File manager - Edit - /home/u816558632/domains/postills.com/public_html/public/spatie.tar
Back
db-dumper/composer.json 0000644 00000001727 15002154456 0011156 0 ustar 00 { "name": "spatie/db-dumper", "description": "Dump databases", "keywords": [ "spatie", "dump", "database", "mysqldump", "db-dumper" ], "homepage": "https://github.com/spatie/db-dumper", "license": "MIT", "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^8.0", "symfony/process": "^5.0|^6.0|^7.0" }, "require-dev": { "pestphp/pest": "^1.22" }, "autoload": { "psr-4": { "Spatie\\DbDumper\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\DbDumper\\Test\\": "tests" } }, "scripts": { "test": "vendor/bin/pest" }, "config": { "allow-plugins": { "pestphp/pest-plugin": true } } } db-dumper/README.md 0000644 00000024012 15002154456 0007703 0 ustar 00 # Dump the contents of a database [](https://packagist.org/packages/spatie/db-dumper)  [](LICENSE.md) [](https://packagist.org/packages/spatie/db-dumper) This repo contains an easy to use class to dump a database using PHP. Currently MySQL, PostgreSQL, SQLite and MongoDB are supported. Behind the scenes `mysqldump`, `pg_dump`, `sqlite3` and `mongodump` are used. Here are simple examples of how to create a database dump with different drivers: **MySQL** ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.sql'); ``` **PostgreSQL** ```php Spatie\DbDumper\Databases\PostgreSql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.sql'); ``` **SQLite** ```php Spatie\DbDumper\Databases\Sqlite::create() ->setDbName($pathToDatabaseFile) ->dumpToFile('dump.sql'); ``` ⚠️ Sqlite version 3.32.0 is required when using the `includeTables` option. **MongoDB** ```php Spatie\DbDumper\Databases\MongoDb::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.gz'); ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/db-dumper.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/db-dumper) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Requirements For dumping MySQL-db's `mysqldump` should be installed. For dumping PostgreSQL-db's `pg_dump` should be installed. For dumping SQLite-db's `sqlite3` should be installed. For dumping MongoDB-db's `mongodump` should be installed. For compressing dump files, `gzip` and/or `bzip2` should be installed. ## Installation You can install the package via composer: ``` bash composer require spatie/db-dumper ``` ## Usage This is the simplest way to create a dump of a MySql db: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.sql'); ``` If you're working with PostgreSQL just use that dumper, most methods are available on both the MySql. and PostgreSql-dumper. ```php Spatie\DbDumper\Databases\PostgreSql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.sql'); ``` If the `mysqldump` (or `pg_dump`) binary is installed in a non default location you can let the package know by using the`setDumpBinaryPath()`-function: ```php Spatie\DbDumper\Databases\MySql::create() ->setDumpBinaryPath('/custom/location') ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->dumpToFile('dump.sql'); ``` If your application is deployed and you need to change the host (default is 127.0.0.1), you can add the `setHost()`-function: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->setHost($host) ->dumpToFile('dump.sql'); ``` ### Use a Database URL In some applications or environments, database credentials are provided as URLs instead of individual components. In this case, you can use the `setDatabaseUrl` method instead of the individual methods. ```php Spatie\DbDumper\Databases\MySql::create() ->setDatabaseUrl($databaseUrl) ->dumpToFile('dump.sql'); ``` When providing a URL, the package will automatically parse it and provide the individual components to the applicable dumper. For example, if you provide the URL `mysql://username:password@hostname:3306/dbname`, the dumper will use the `hostname` host, running on port `3306`, and will connect to `dbname` with `username` and `password`. ### Dump specific tables Using an array: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->includeTables(['table1', 'table2', 'table3']) ->dumpToFile('dump.sql'); ``` Using a string: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->includeTables('table1, table2, table3') ->dumpToFile('dump.sql'); ``` ### Don't use column_statics table with some old version of MySql service. In order to use "_--column-statistics=0_" as option in mysqldump command you can use _doNotUseColumnStatistics()_ method. If you have installed _mysqldump 8_, it queries by default _column_statics_ table in _information_schema_ database. In some old version of MySql (service) like 5.7, this table doesn't exist. So you could have an exception during the execution of mysqldump. To avoid this, you could use _doNotUseColumnStatistics()_ method. ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->doNotUseColumnStatistics() ->dumpToFile('dump.sql'); ``` ### Excluding tables from the dump Using an array: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->excludeTables(['table1', 'table2', 'table3']) ->dumpToFile('dump.sql'); ``` Using a string: ```php Spatie\DbDumper\Databases\MySql::create() ->setDbName($databaseName) ->setUserName($userName) ->setPassword($password) ->excludeTables('table1, table2, table3') ->dumpToFile('dump.sql'); ``` ### Do not write CREATE TABLE statements that create each dumped table. ```php $dumpCommand = MySql::create() ->setDbName('dbname') ->setUserName('username') ->setPassword('password') ->doNotCreateTables() ->getDumpCommand('dump.sql', 'credentials.txt'); ``` ### Adding extra options If you want to add an arbitrary option to the dump command you can use `addExtraOption` ```php $dumpCommand = MySql::create() ->setDbName('dbname') ->setUserName('username') ->setPassword('password') ->addExtraOption('--xml') ->getDumpCommand('dump.sql', 'credentials.txt'); ``` If you're working with MySql you can set the database name using `--databases` as an extra option. This is particularly useful when used in conjunction with the `--add-drop-database` `mysqldump` option (see the [mysqldump docs](https://dev.mysql.com/doc/refman/5.7/en/mysqldump.html#option_mysqldump_add-drop-database)). ```php $dumpCommand = MySql::create() ->setUserName('username') ->setPassword('password') ->addExtraOption('--databases dbname') ->addExtraOption('--add-drop-database') ->getDumpCommand('dump.sql', 'credentials.txt'); ``` With MySql, you also have the option to use the `--all-databases` extra option. This is useful when you want to run a full backup of all the databases in the specified MySQL connection. ```php $dumpCommand = MySql::create() ->setUserName('username') ->setPassword('password') ->addExtraOption('--all-databases') ->getDumpCommand('dump.sql', 'credentials.txt'); ``` Please note that using the `->addExtraOption('--databases dbname')` or `->addExtraOption('--all-databases')` will override the database name set on a previous `->setDbName()` call. ### Using compression If you want the output file to be compressed, you can use a compressor class. There are two compressors that come out of the box: - `GzipCompressor` - This will compress your db dump with `gzip`. Make sure `gzip` is installed on your system before using this. - `Bzip2Compressor` - This will compress your db dump with `bzip2`. Make sure `bzip2` is installed on your system before using this. ```php $dumpCommand = MySql::create() ->setDbName('dbname') ->setUserName('username') ->setPassword('password') ->useCompressor(new GzipCompressor()) // or `new Bzip2Compressor()` ->dumpToFile('dump.sql.gz'); ``` ### Creating your own compressor You can create you own compressor implementing the `Compressor` interface. Here's how that interface looks like: ```php namespace Spatie\DbDumper\Compressors; interface Compressor { public function useCommand(): string; public function useExtension(): string; } ``` The `useCommand` should simply return the compression command the db dump will get pumped to. Here's the implementation of `GzipCompression`. ```php namespace Spatie\DbDumper\Compressors; class GzipCompressor implements Compressor { public function useCommand(): string { return 'gzip'; } public function useExtension(): string { return 'gz'; } } ``` ## Testing ``` bash $ composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) Initial PostgreSQL support was contributed by [Adriano Machado](https://github.com/ammachado). SQlite support was contributed by [Peter Matseykanets](https://twitter.com/pmatseykanets). ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. db-dumper/src/Exceptions/CannotSetParameter.php 0000644 00000000500 15002154456 0015600 0 ustar 00 <?php namespace Spatie\DbDumper\Exceptions; use Exception; class CannotSetParameter extends Exception { public static function conflictingParameters(string $name, string $conflictName): static { return new static("Cannot set `{$name}` because it conflicts with parameter `{$conflictName}`."); } } db-dumper/src/Exceptions/CannotStartDump.php 0000644 00000000371 15002154456 0015135 0 ustar 00 <?php namespace Spatie\DbDumper\Exceptions; use Exception; class CannotStartDump extends Exception { public static function emptyParameter(string $name): static { return new static("Parameter `{$name}` cannot be empty."); } } db-dumper/src/Exceptions/DumpFailed.php 0000644 00000002632 15002154456 0014063 0 ustar 00 <?php namespace Spatie\DbDumper\Exceptions; use Exception; use Symfony\Component\Process\Process; class DumpFailed extends Exception { public static function processDidNotEndSuccessfully(Process $process): static { $processOutput = static::formatProcessOutput($process); return new static("The dump process failed with a none successful exitcode.{$processOutput}"); } public static function dumpfileWasNotCreated(Process $process): static { $processOutput = static::formatProcessOutput($process); return new static("The dumpfile could not be created.{$processOutput}"); } public static function dumpfileWasEmpty(Process $process): static { $processOutput = static::formatProcessOutput($process); return new static("The created dumpfile is empty.{$processOutput}"); } protected static function formatProcessOutput(Process $process): string { $output = $process->getOutput() ?: '<no output>'; $errorOutput = $process->getErrorOutput() ?: '<no output>'; $exitCodeText = $process->getExitCodeText() ?: '<no exit text>'; return <<<CONSOLE Exitcode ======== {$process->getExitCode()}: {$exitCodeText} Output ====== {$output} Error Output ============ {$errorOutput} CONSOLE; } } db-dumper/src/Exceptions/InvalidDatabaseUrl.php 0000644 00000000431 15002154456 0015542 0 ustar 00 <?php namespace Spatie\DbDumper\Exceptions; use Exception; class InvalidDatabaseUrl extends Exception { public static function invalidUrl(string $databaseUrl): static { return new static("Database URL `{$databaseUrl}` is invalid and cannot be parsed."); } } db-dumper/src/DbDumper.php 0000644 00000013763 15002154456 0011441 0 ustar 00 <?php namespace Spatie\DbDumper; use Spatie\DbDumper\Compressors\Compressor; use Spatie\DbDumper\Exceptions\CannotSetParameter; use Spatie\DbDumper\Exceptions\DumpFailed; use Symfony\Component\Process\Process; abstract class DbDumper { protected string $databaseUrl = ''; protected string $dbName = ''; protected string $userName = ''; protected string $password = ''; protected string $host = 'localhost'; protected int $port = 5432; protected string $socket = ''; protected int $timeout = 0; protected string $dumpBinaryPath = ''; protected array $includeTables = []; protected array $excludeTables = []; protected array $extraOptions = []; protected array $extraOptionsAfterDbName = []; protected ?object $compressor = null; public static function create(): static { return new static(); } public function getDbName(): string { return $this->dbName; } public function setDbName(string $dbName): self { $this->dbName = $dbName; return $this; } public function getDatabaseUrl(): string { return $this->databaseUrl; } public function setDatabaseUrl(string $databaseUrl): self { $this->databaseUrl = $databaseUrl; $this->configureFromDatabaseUrl(); return $this; } public function setUserName(string $userName): self { $this->userName = $userName; return $this; } public function setPassword(string $password): self { $this->password = $password; return $this; } public function setHost(string $host): self { $this->host = $host; return $this; } public function getHost(): string { return $this->host; } public function setPort(int $port): self { $this->port = $port; return $this; } public function setSocket(string $socket): self { $this->socket = $socket; return $this; } public function setTimeout(int $timeout): self { $this->timeout = $timeout; return $this; } public function setDumpBinaryPath(string $dumpBinaryPath): self { if ($dumpBinaryPath !== '' && ! str_ends_with($dumpBinaryPath, '/')) { $dumpBinaryPath .= '/'; } $this->dumpBinaryPath = $dumpBinaryPath; return $this; } public function getCompressorExtension(): string { return $this->compressor->useExtension(); } public function useCompressor(Compressor $compressor): self { $this->compressor = $compressor; return $this; } public function includeTables(string | array $includeTables): self { if (! empty($this->excludeTables)) { throw CannotSetParameter::conflictingParameters('includeTables', 'excludeTables'); } if (! is_array($includeTables)) { $includeTables = explode(', ', $includeTables); } $this->includeTables = $includeTables; return $this; } public function excludeTables(string | array $excludeTables): self { if (! empty($this->includeTables)) { throw CannotSetParameter::conflictingParameters('excludeTables', 'includeTables'); } if (! is_array($excludeTables)) { $excludeTables = explode(', ', $excludeTables); } $this->excludeTables = $excludeTables; return $this; } public function addExtraOption(string $extraOption): self { if (! empty($extraOption)) { $this->extraOptions[] = $extraOption; } return $this; } public function addExtraOptionAfterDbName(string $extraOptionAfterDbName): self { if (! empty($extraOptionAfterDbName)) { $this->extraOptionsAfterDbName[] = $extraOptionAfterDbName; } return $this; } abstract public function dumpToFile(string $dumpFile): void; public function checkIfDumpWasSuccessFul(Process $process, string $outputFile): void { if (! $process->isSuccessful()) { throw DumpFailed::processDidNotEndSuccessfully($process); } if (! file_exists($outputFile)) { throw DumpFailed::dumpfileWasNotCreated($process); } if (filesize($outputFile) === 0) { throw DumpFailed::dumpfileWasEmpty($process); } } protected function configureFromDatabaseUrl(): void { $parsed = (new DsnParser($this->databaseUrl))->parse(); $componentMap = [ 'host' => 'setHost', 'port' => 'setPort', 'database' => 'setDbName', 'username' => 'setUserName', 'password' => 'setPassword', ]; foreach ($parsed as $component => $value) { if (isset($componentMap[$component])) { $setterMethod = $componentMap[$component]; if (! $value || in_array($value, ['', 'null'])) { continue; } $this->$setterMethod($value); } } } protected function getCompressCommand(string $command, string $dumpFile): string { $compressCommand = $this->compressor->useCommand(); if ($this->isWindows()) { return "{$command} | {$compressCommand} > {$dumpFile}"; } return "(((({$command}; echo \$? >&3) | {$compressCommand} > {$dumpFile}) 3>&1) | (read x; exit \$x))"; } protected function echoToFile(string $command, string $dumpFile): string { $dumpFile = '"' . addcslashes($dumpFile, '\\"') . '"'; if ($this->compressor) { return $this->getCompressCommand($command, $dumpFile); } return $command . ' > ' . $dumpFile; } protected function determineQuote(): string { return $this->isWindows() ? '"' : "'"; } protected function isWindows(): bool { return str_starts_with(strtoupper(PHP_OS), 'WIN'); } } db-dumper/src/Databases/Sqlite.php 0000644 00000003443 15002154456 0013061 0 ustar 00 <?php namespace Spatie\DbDumper\Databases; use Spatie\DbDumper\DbDumper; use SQLite3; use Symfony\Component\Process\Process; class Sqlite extends DbDumper { public function dumpToFile(string $dumpFile): void { $process = $this->getProcess($dumpFile); $process->run(); $this->checkIfDumpWasSuccessFul($process, $dumpFile); } public function getDbTables(): array { $db = new SQLite3($this->dbName); $query = $db->query("SELECT name FROM sqlite_master WHERE type='table' AND name NOT LIKE 'sqlite_%';"); $tables = []; while ($table = $query->fetchArray(SQLITE3_ASSOC)) { $tables[] = $table['name']; } $db->close(); return $tables; } public function getDumpCommand(string $dumpFile): string { $includeTables = rtrim(' ' . implode(' ', $this->includeTables)); if (empty($includeTables) && ! empty($this->excludeTables)) { $tables = $this->getDbTables(); $includeTables = rtrim(' ' . implode(' ', array_diff($tables, $this->excludeTables))); } $dumpInSqlite = "echo 'BEGIN IMMEDIATE;\n.dump{$includeTables}'"; if ($this->isWindows()) { $dumpInSqlite = "(echo BEGIN IMMEDIATE; & echo .dump{$includeTables})"; } $quote = $this->determineQuote(); $command = sprintf( "{$dumpInSqlite} | {$quote}%ssqlite3{$quote} --bail {$quote}%s{$quote}", $this->dumpBinaryPath, $this->dbName ); return $this->echoToFile($command, $dumpFile); } public function getProcess(string $dumpFile): Process { $command = $this->getDumpCommand($dumpFile); return Process::fromShellCommandline($command, null, null, null, $this->timeout); } } db-dumper/src/Databases/MongoDb.php 0000644 00000005044 15002154456 0013144 0 ustar 00 <?php namespace Spatie\DbDumper\Databases; use Spatie\DbDumper\DbDumper; use Spatie\DbDumper\Exceptions\CannotStartDump; use Symfony\Component\Process\Process; class MongoDb extends DbDumper { protected int $port = 27017; protected ?string $collection = null; protected ?string $authenticationDatabase = null; public function dumpToFile(string $dumpFile): void { $this->guardAgainstIncompleteCredentials(); $process = $this->getProcess($dumpFile); $process->run(); $this->checkIfDumpWasSuccessFul($process, $dumpFile); } /** * Verifies if the dbname and host options are set. * * @throws \Spatie\DbDumper\Exceptions\CannotStartDump * * @return void */ public function guardAgainstIncompleteCredentials(): void { foreach (['dbName', 'host'] as $requiredProperty) { if (strlen($this->$requiredProperty) === 0) { throw CannotStartDump::emptyParameter($requiredProperty); } } } public function setCollection(string $collection): self { $this->collection = $collection; return $this; } public function setAuthenticationDatabase(string $authenticationDatabase): self { $this->authenticationDatabase = $authenticationDatabase; return $this; } public function getDumpCommand(string $filename): string { $quote = $this->determineQuote(); $command = [ "{$quote}{$this->dumpBinaryPath}mongodump{$quote}", "--db {$this->dbName}", '--archive', ]; if ($this->userName) { $command[] = "--username {$quote}{$this->userName}{$quote}"; } if ($this->password) { $command[] = "--password {$quote}{$this->password}{$quote}"; } if (isset($this->host)) { $command[] = "--host {$this->host}"; } if (isset($this->port)) { $command[] = "--port {$this->port}"; } if (isset($this->collection)) { $command[] = "--collection {$this->collection}"; } if ($this->authenticationDatabase) { $command[] = "--authenticationDatabase {$this->authenticationDatabase}"; } return $this->echoToFile(implode(' ', $command), $filename); } public function getProcess(string $dumpFile): Process { $command = $this->getDumpCommand($dumpFile); return Process::fromShellCommandline($command, null, null, null, $this->timeout); } } db-dumper/src/Databases/MySql.php 0000644 00000016062 15002154456 0012666 0 ustar 00 <?php namespace Spatie\DbDumper\Databases; use Spatie\DbDumper\DbDumper; use Spatie\DbDumper\Exceptions\CannotStartDump; use Symfony\Component\Process\Process; class MySql extends DbDumper { protected bool $skipComments = true; protected bool $useExtendedInserts = true; protected bool $useSingleTransaction = false; protected bool $skipLockTables = false; protected bool $doNotUseColumnStatistics = false; protected bool $useQuick = false; protected string $defaultCharacterSet = ''; protected bool $dbNameWasSetAsExtraOption = false; protected bool $allDatabasesWasSetAsExtraOption = false; protected string $setGtidPurged = 'AUTO'; protected bool $createTables = true; /** @var false|resource */ private $tempFileHandle; public function __construct() { $this->port = 3306; } public function skipComments(): self { $this->skipComments = true; return $this; } public function dontSkipComments(): self { $this->skipComments = false; return $this; } public function useExtendedInserts(): self { $this->useExtendedInserts = true; return $this; } public function dontUseExtendedInserts(): self { $this->useExtendedInserts = false; return $this; } public function useSingleTransaction(): self { $this->useSingleTransaction = true; return $this; } public function dontUseSingleTransaction(): self { $this->useSingleTransaction = false; return $this; } public function skipLockTables(): self { $this->skipLockTables = true; return $this; } public function doNotUseColumnStatistics(): self { $this->doNotUseColumnStatistics = true; return $this; } public function dontSkipLockTables(): self { $this->skipLockTables = false; return $this; } public function useQuick(): self { $this->useQuick = true; return $this; } public function dontUseQuick(): self { $this->useQuick = false; return $this; } public function setDefaultCharacterSet(string $characterSet): self { $this->defaultCharacterSet = $characterSet; return $this; } public function setGtidPurged(string $setGtidPurged): self { $this->setGtidPurged = $setGtidPurged; return $this; } public function dumpToFile(string $dumpFile): void { $this->guardAgainstIncompleteCredentials(); $tempFileHandle = tmpfile(); $this->setTempFileHandle($tempFileHandle); $process = $this->getProcess($dumpFile); $process->run(); $this->checkIfDumpWasSuccessFul($process, $dumpFile); } public function addExtraOption(string $extraOption): self { if (str_contains($extraOption, '--all-databases')) { $this->dbNameWasSetAsExtraOption = true; $this->allDatabasesWasSetAsExtraOption = true; } if (preg_match('/^--databases (\S+)/', $extraOption, $matches) === 1) { $this->setDbName($matches[1]); $this->dbNameWasSetAsExtraOption = true; } return parent::addExtraOption($extraOption); } public function doNotCreateTables(): self { $this->createTables = false; return $this; } public function getDumpCommand(string $dumpFile, string $temporaryCredentialsFile): string { $quote = $this->determineQuote(); $command = [ "{$quote}{$this->dumpBinaryPath}mysqldump{$quote}", "--defaults-extra-file=\"{$temporaryCredentialsFile}\"", ]; if (! $this->createTables) { $command[] = '--no-create-info'; } if ($this->skipComments) { $command[] = '--skip-comments'; } $command[] = $this->useExtendedInserts ? '--extended-insert' : '--skip-extended-insert'; if ($this->useSingleTransaction) { $command[] = '--single-transaction'; } if ($this->skipLockTables) { $command[] = '--skip-lock-tables'; } if ($this->doNotUseColumnStatistics) { $command[] = '--column-statistics=0'; } if ($this->useQuick) { $command[] = '--quick'; } if ($this->socket !== '') { $command[] = "--socket={$this->socket}"; } foreach ($this->excludeTables as $tableName) { $command[] = "--ignore-table={$this->dbName}.{$tableName}"; } if (! empty($this->defaultCharacterSet)) { $command[] = '--default-character-set='.$this->defaultCharacterSet; } foreach ($this->extraOptions as $extraOption) { $command[] = $extraOption; } if ($this->setGtidPurged !== 'AUTO') { $command[] = '--set-gtid-purged='.$this->setGtidPurged; } if (! $this->dbNameWasSetAsExtraOption) { $command[] = $this->dbName; } if (! empty($this->includeTables)) { $includeTables = implode(' ', $this->includeTables); $command[] = "--tables {$includeTables}"; } foreach ($this->extraOptionsAfterDbName as $extraOptionAfterDbName) { $command[] = $extraOptionAfterDbName; } return $this->echoToFile(implode(' ', $command), $dumpFile); } public function getContentsOfCredentialsFile(): string { $contents = [ '[client]', "user = '{$this->userName}'", "password = '{$this->password}'", "port = '{$this->port}'", ]; if ($this->socket === '') { $contents[] = "host = '{$this->host}'"; } return implode(PHP_EOL, $contents); } public function guardAgainstIncompleteCredentials(): void { foreach (['userName', 'host'] as $requiredProperty) { if (strlen($this->$requiredProperty) === 0) { throw CannotStartDump::emptyParameter($requiredProperty); } } if (strlen($this->dbName) === 0 && ! $this->allDatabasesWasSetAsExtraOption) { throw CannotStartDump::emptyParameter('dbName'); } } /** * @param string $dumpFile * @return Process */ public function getProcess(string $dumpFile): Process { fwrite($this->getTempFileHandle(), $this->getContentsOfCredentialsFile()); $temporaryCredentialsFile = stream_get_meta_data($this->getTempFileHandle())['uri']; $command = $this->getDumpCommand($dumpFile, $temporaryCredentialsFile); return Process::fromShellCommandline($command, null, null, null, $this->timeout); } /** * @return false|resource */ public function getTempFileHandle(): mixed { return $this->tempFileHandle; } /** * @param false|resource $tempFileHandle */ public function setTempFileHandle($tempFileHandle) { $this->tempFileHandle = $tempFileHandle; } } db-dumper/src/Databases/PostgreSql.php 0000644 00000007531 15002154456 0013725 0 ustar 00 <?php namespace Spatie\DbDumper\Databases; use Spatie\DbDumper\DbDumper; use Spatie\DbDumper\Exceptions\CannotStartDump; use Symfony\Component\Process\Process; class PostgreSql extends DbDumper { protected bool $useInserts = false; protected bool $createTables = true; /** @var false|resource */ private $tempFileHandle; public function __construct() { $this->port = 5432; } public function useInserts(): self { $this->useInserts = true; return $this; } public function dumpToFile(string $dumpFile): void { $this->guardAgainstIncompleteCredentials(); $tempFileHandle = tmpfile(); $this->setTempFileHandle($tempFileHandle); $process = $this->getProcess($dumpFile); $process->run(); $this->checkIfDumpWasSuccessFul($process, $dumpFile); } public function getDumpCommand(string $dumpFile): string { $quote = $this->determineQuote(); $command = [ "{$quote}{$this->dumpBinaryPath}pg_dump{$quote}", "-U \"{$this->userName}\"", '-h '.($this->socket === '' ? $this->host : $this->socket), "-p {$this->port}", ]; if ($this->useInserts) { $command[] = '--inserts'; } if (! $this->createTables) { $command[] = '--data-only'; } foreach ($this->extraOptions as $extraOption) { $command[] = $extraOption; } if (! empty($this->includeTables)) { $command[] = '-t '.implode(' -t ', $this->includeTables); } if (! empty($this->excludeTables)) { $command[] = '-T '.implode(' -T ', $this->excludeTables); } return $this->echoToFile(implode(' ', $command), $dumpFile); } public function getContentsOfCredentialsFile(): string { $contents = [ $this->escapeCredentialEntry($this->host), $this->escapeCredentialEntry((string) $this->port), $this->escapeCredentialEntry($this->dbName), $this->escapeCredentialEntry($this->userName), $this->escapeCredentialEntry($this->password), ]; return implode(':', $contents); } protected function escapeCredentialEntry($entry): string { $entry = str_replace('\\', '\\\\', $entry); $entry = str_replace(':', '\\:', $entry); return $entry; } public function guardAgainstIncompleteCredentials() { foreach (['userName', 'dbName', 'host'] as $requiredProperty) { if (empty($this->$requiredProperty)) { throw CannotStartDump::emptyParameter($requiredProperty); } } } protected function getEnvironmentVariablesForDumpCommand(string $temporaryCredentialsFile): array { return [ 'PGPASSFILE' => $temporaryCredentialsFile, 'PGDATABASE' => $this->dbName, ]; } public function doNotCreateTables(): self { $this->createTables = false; return $this; } public function getProcess(string $dumpFile): Process { $command = $this->getDumpCommand($dumpFile); fwrite($this->getTempFileHandle(), $this->getContentsOfCredentialsFile()); $temporaryCredentialsFile = stream_get_meta_data($this->getTempFileHandle())['uri']; $envVars = $this->getEnvironmentVariablesForDumpCommand($temporaryCredentialsFile); return Process::fromShellCommandline($command, null, $envVars, null, $this->timeout); } /** * @return false|resource */ public function getTempFileHandle() { return $this->tempFileHandle; } /** * @param false|resource $tempFileHandle */ public function setTempFileHandle($tempFileHandle): void { $this->tempFileHandle = $tempFileHandle; } } db-dumper/src/DsnParser.php 0000644 00000004451 15002154456 0011632 0 ustar 00 <?php namespace Spatie\DbDumper; use Spatie\DbDumper\Exceptions\InvalidDatabaseUrl; class DsnParser { protected string $dsn; public function __construct(string $dsn) { $this->dsn = $dsn; } public function parse(): array { $rawComponents = $this->parseUrl($this->dsn); $decodedComponents = $this->parseNativeTypes( array_map('rawurldecode', $rawComponents) ); return array_merge( $this->getPrimaryOptions($decodedComponents), $this->getQueryOptions($rawComponents) ); } protected function getPrimaryOptions($url): array { return array_filter([ 'database' => $this->getDatabase($url), 'host' => $url['host'] ?? null, 'port' => $url['port'] ?? null, 'username' => $url['user'] ?? null, 'password' => $url['pass'] ?? null, ], static fn ($value) => ! is_null($value)); } protected function getDatabase($url): ?string { $path = $url['path'] ?? null; if (! $path) { return null; } if ($path === '/') { return null; } if (isset($url['scheme']) && str_contains($url['scheme'], 'sqlite')) { return $path; } return trim($path, '/'); } protected function getQueryOptions($url) { $queryString = $url['query'] ?? null; if (! $queryString) { return []; } $query = []; parse_str($queryString, $query); return $this->parseNativeTypes($query); } protected function parseUrl($url): array { $url = preg_replace('#^(sqlite3?):///#', '$1://null/', $url); $parsedUrl = parse_url($url); if ($parsedUrl === false) { throw InvalidDatabaseUrl::invalidUrl($url); } return $parsedUrl; } protected function parseNativeTypes($value) { if (is_array($value)) { return array_map([$this, 'parseNativeTypes'], $value); } if (! is_string($value)) { return $value; } $parsedValue = json_decode($value, true); if (json_last_error() === JSON_ERROR_NONE) { return $parsedValue; } return $value; } } db-dumper/src/Compressors/Bzip2Compressor.php 0000644 00000000372 15002154456 0015311 0 ustar 00 <?php namespace Spatie\DbDumper\Compressors; class Bzip2Compressor implements Compressor { public function useCommand(): string { return 'bzip2'; } public function useExtension(): string { return 'bz2'; } } db-dumper/src/Compressors/GzipCompressor.php 0000644 00000000367 15002154456 0015240 0 ustar 00 <?php namespace Spatie\DbDumper\Compressors; class GzipCompressor implements Compressor { public function useCommand(): string { return 'gzip'; } public function useExtension(): string { return 'gz'; } } db-dumper/src/Compressors/Compressor.php 0000644 00000000237 15002154456 0014402 0 ustar 00 <?php namespace Spatie\DbDumper\Compressors; interface Compressor { public function useCommand(): string; public function useExtension(): string; } db-dumper/LICENSE.md 0000644 00000002102 15002154456 0010024 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ignition/composer.json 0000644 00000004727 15002154456 0011122 0 ustar 00 { "name" : "spatie/ignition", "description" : "A beautiful error page for PHP applications.", "keywords" : [ "error", "page", "laravel", "flare" ], "authors" : [ { "name" : "Spatie", "email" : "info@spatie.be", "role" : "Developer" } ], "homepage": "https://flareapp.io/ignition", "license": "MIT", "require": { "php": "^8.0", "ext-json": "*", "ext-mbstring": "*", "spatie/backtrace": "^1.5.3", "spatie/flare-client-php": "^1.4.0", "symfony/console": "^5.4|^6.0|^7.0", "symfony/var-dumper": "^5.4|^6.0|^7.0" }, "require-dev" : { "illuminate/cache" : "^9.52|^10.0|^11.0", "mockery/mockery" : "^1.4", "pestphp/pest" : "^1.20|^2.0", "phpstan/extension-installer" : "^1.1", "phpstan/phpstan-deprecation-rules" : "^1.0", "phpstan/phpstan-phpunit" : "^1.0", "psr/simple-cache-implementation" : "*", "symfony/cache" : "^5.4|^6.0|^7.0", "symfony/process" : "^5.4|^6.0|^7.0", "vlucas/phpdotenv" : "^5.5" }, "suggest" : { "openai-php/client" : "Require get solutions from OpenAI", "simple-cache-implementation" : "To cache solutions from OpenAI" }, "config" : { "sort-packages" : true, "allow-plugins" : { "phpstan/extension-installer": true, "pestphp/pest-plugin": true, "php-http/discovery": false } }, "autoload" : { "psr-4" : { "Spatie\\Ignition\\" : "src" } }, "autoload-dev" : { "psr-4" : { "Spatie\\Ignition\\Tests\\" : "tests" } }, "minimum-stability" : "dev", "prefer-stable" : true, "scripts" : { "analyse" : "vendor/bin/phpstan analyse", "baseline" : "vendor/bin/phpstan analyse --generate-baseline", "format" : "vendor/bin/php-cs-fixer fix --allow-risky=yes", "test" : "vendor/bin/pest", "test-coverage" : "vendor/bin/phpunit --coverage-html coverage" }, "support" : { "issues" : "https://github.com/spatie/ignition/issues", "forum" : "https://twitter.com/flareappio", "source" : "https://github.com/spatie/ignition", "docs" : "https://flareapp.io/docs/ignition-for-laravel/introduction" }, "extra" : { "branch-alias" : { "dev-main" : "1.5.x-dev" } } } ignition/resources/views/errorPage.php 0000644 00000004122 15002154456 0014173 0 ustar 00 <!DOCTYPE html> <?php /** @var \Spatie\Ignition\ErrorPage\ErrorPageViewModel $viewModel */ ?> <html lang="en" class="<?= $viewModel->theme() ?>"> <!-- <?= $viewModel->throwableString() ?> --> <head> <!-- Hide dumps asap --> <style> pre.sf-dump { display: none !important; } </style> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <meta name="robots" content="noindex, nofollow"> <title><?= $viewModel->title() ?></title> <script> // Livewire modals remove CSS classes on the `html` element so we re-add // the theme class again using JavaScript. document.documentElement.classList.add('<?= $viewModel->theme() ?>'); // Process `auto` theme as soon as possible to avoid flashing of white background. if (document.documentElement.classList.contains('auto') && window.matchMedia('(prefers-color-scheme: dark)').matches) { document.documentElement.classList.add('dark'); } </script> <style><?= $viewModel->getAssetContents('ignition.css') ?></style> <?= $viewModel->customHtmlHead() ?> </head> <body class="scrollbar-lg antialiased bg-center bg-dots-darker dark:bg-dots-lighter"> <script> window.data = <?= $viewModel->jsonEncode([ 'report' => $viewModel->report(), 'shareableReport' => $viewModel->shareableReport(), 'config' => $viewModel->config(), 'solutions' => $viewModel->solutions(), 'updateConfigEndpoint' => $viewModel->updateConfigEndpoint(), ]) ?>; </script> <!-- The noscript representation is for HTTP client like Postman that have JS disabled. --> <noscript> <pre><?= $viewModel->throwableString() ?></pre> </noscript> <div id="app"></div> <script> <!-- <?= $viewModel->getAssetContents('ignition.js') ?> --> </script> <script> window.ignite(window.data); </script> <?= $viewModel->customHtmlBody() ?> <!-- <?= $viewModel->throwableString() ?> --> </body> </html> ignition/resources/views/aiPrompt.php 0000644 00000001740 15002154456 0014043 0 ustar 00 <?php /** @var \Spatie\Ignition\Solutions\OpenAi\OpenAiPromptViewModel $viewModel */ ?> You are a very skilled PHP programmer. <?php if($viewModel->applicationType()) { ?> You are working on a <?php echo $viewModel->applicationType() ?> application. <?php } ?> Use the following context to find a possible fix for the exception message at the end. Limit your answer to 4 or 5 sentences. Also include a few links to documentation that might help. Use this format in your answer, make sure links are json: FIX insert the possible fix here ENDFIX LINKS {"title": "Title link 1", "url": "URL link 1"} {"title": "Title link 2", "url": "URL link 2"} ENDLINKS --- Here comes the context and the exception message: Line: <?php echo $viewModel->line() ?> File: <?php echo $viewModel->file() ?> Snippet including line numbers: <?php echo $viewModel->snippet() ?> Exception class: <?php echo $viewModel->exceptionClass() ?> Exception message: <?php echo $viewModel->exceptionMessage() ?> ignition/resources/compiled/.gitignore 0000644 00000000051 15002154456 0014160 0 ustar 00 * !.gitignore !ignition.css !ignition.js ignition/resources/compiled/ignition.js 0000644 00003254762 15002154456 0014375 0 ustar 00 function e(){return(e=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(e[r]=n[r])}return e}).apply(this,arguments)}var t="undefined"!=typeof globalThis?globalThis:"undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};function n(e){var t={exports:{}};return e(t,t.exports),t.exports}var r=Object.getOwnPropertySymbols,a=Object.prototype.hasOwnProperty,o=Object.prototype.propertyIsEnumerable;function i(e){if(null==e)throw new TypeError("Object.assign cannot be called with null or undefined");return Object(e)}var l=function(){try{if(!Object.assign)return!1;var e=new String("abc");if(e[5]="de","5"===Object.getOwnPropertyNames(e)[0])return!1;for(var t={},n=0;n<10;n++)t["_"+String.fromCharCode(n)]=n;if("0123456789"!==Object.getOwnPropertyNames(t).map(function(e){return t[e]}).join(""))return!1;var r={};return"abcdefghijklmnopqrst".split("").forEach(function(e){r[e]=e}),"abcdefghijklmnopqrst"===Object.keys(Object.assign({},r)).join("")}catch(e){return!1}}()?Object.assign:function(e,t){for(var n,l,s=i(e),c=1;c<arguments.length;c++){for(var u in n=Object(arguments[c]))a.call(n,u)&&(s[u]=n[u]);if(r){l=r(n);for(var f=0;f<l.length;f++)o.call(n,l[f])&&(s[l[f]]=n[l[f]])}}return s};n(function(e,t){var n=60103,r=60106;t.Fragment=60107,t.StrictMode=60108,t.Profiler=60114;var a=60109,o=60110,i=60112;t.Suspense=60113;var s=60115,c=60116;if("function"==typeof Symbol&&Symbol.for){var u=Symbol.for;n=u("react.element"),r=u("react.portal"),t.Fragment=u("react.fragment"),t.StrictMode=u("react.strict_mode"),t.Profiler=u("react.profiler"),a=u("react.provider"),o=u("react.context"),i=u("react.forward_ref"),t.Suspense=u("react.suspense"),s=u("react.memo"),c=u("react.lazy")}var f="function"==typeof Symbol&&Symbol.iterator;function d(e){for(var t="https://reactjs.org/docs/error-decoder.html?invariant="+e,n=1;n<arguments.length;n++)t+="&args[]="+encodeURIComponent(arguments[n]);return"Minified React error #"+e+"; visit "+t+" for the full message or use the non-minified dev environment for full errors and additional helpful warnings."}var p={isMounted:function(){return!1},enqueueForceUpdate:function(){},enqueueReplaceState:function(){},enqueueSetState:function(){}},m={};function h(e,t,n){this.props=e,this.context=t,this.refs=m,this.updater=n||p}function g(){}function y(e,t,n){this.props=e,this.context=t,this.refs=m,this.updater=n||p}h.prototype.isReactComponent={},h.prototype.setState=function(e,t){if("object"!=typeof e&&"function"!=typeof e&&null!=e)throw Error(d(85));this.updater.enqueueSetState(this,e,t,"setState")},h.prototype.forceUpdate=function(e){this.updater.enqueueForceUpdate(this,e,"forceUpdate")},g.prototype=h.prototype;var v=y.prototype=new g;v.constructor=y,l(v,h.prototype),v.isPureReactComponent=!0;var b={current:null},E=Object.prototype.hasOwnProperty,T={key:!0,ref:!0,__self:!0,__source:!0};function S(e,t,r){var a,o={},i=null,l=null;if(null!=t)for(a in void 0!==t.ref&&(l=t.ref),void 0!==t.key&&(i=""+t.key),t)E.call(t,a)&&!T.hasOwnProperty(a)&&(o[a]=t[a]);var s=arguments.length-2;if(1===s)o.children=r;else if(1<s){for(var c=Array(s),u=0;u<s;u++)c[u]=arguments[u+2];o.children=c}if(e&&e.defaultProps)for(a in s=e.defaultProps)void 0===o[a]&&(o[a]=s[a]);return{$$typeof:n,type:e,key:i,ref:l,props:o,_owner:b.current}}function w(e){return"object"==typeof e&&null!==e&&e.$$typeof===n}var N=/\/+/g;function R(e,t){return"object"==typeof e&&null!==e&&null!=e.key?function(e){var t={"=":"=0",":":"=2"};return"$"+e.replace(/[=:]/g,function(e){return t[e]})}(""+e.key):t.toString(36)}function O(e,t,a,o,i){var l=typeof e;"undefined"!==l&&"boolean"!==l||(e=null);var s=!1;if(null===e)s=!0;else switch(l){case"string":case"number":s=!0;break;case"object":switch(e.$$typeof){case n:case r:s=!0}}if(s)return i=i(s=e),e=""===o?"."+R(s,0):o,Array.isArray(i)?(a="",null!=e&&(a=e.replace(N,"$&/")+"/"),O(i,t,a,"",function(e){return e})):null!=i&&(w(i)&&(i=function(e,t){return{$$typeof:n,type:e.type,key:t,ref:e.ref,props:e.props,_owner:e._owner}}(i,a+(!i.key||s&&s.key===i.key?"":(""+i.key).replace(N,"$&/")+"/")+e)),t.push(i)),1;if(s=0,o=""===o?".":o+":",Array.isArray(e))for(var c=0;c<e.length;c++){var u=o+R(l=e[c],c);s+=O(l,t,a,u,i)}else if("function"==typeof(u=function(e){return null===e||"object"!=typeof e?null:"function"==typeof(e=f&&e[f]||e["@@iterator"])?e:null}(e)))for(e=u.call(e),c=0;!(l=e.next()).done;)s+=O(l=l.value,t,a,u=o+R(l,c++),i);else if("object"===l)throw t=""+e,Error(d(31,"[object Object]"===t?"object with keys {"+Object.keys(e).join(", ")+"}":t));return s}function C(e,t,n){if(null==e)return e;var r=[],a=0;return O(e,r,"","",function(e){return t.call(n,e,a++)}),r}function x(e){if(-1===e._status){var t=e._result;t=t(),e._status=0,e._result=t,t.then(function(t){0===e._status&&(t=t.default,e._status=1,e._result=t)},function(t){0===e._status&&(e._status=2,e._result=t)})}if(1===e._status)return e._result;throw e._result}var k={current:null};function A(){var e=k.current;if(null===e)throw Error(d(321));return e}var I={ReactCurrentDispatcher:k,ReactCurrentBatchConfig:{transition:0},ReactCurrentOwner:b,IsSomeRendererActing:{current:!1},assign:l};t.Children={map:C,forEach:function(e,t,n){C(e,function(){t.apply(this,arguments)},n)},count:function(e){var t=0;return C(e,function(){t++}),t},toArray:function(e){return C(e,function(e){return e})||[]},only:function(e){if(!w(e))throw Error(d(143));return e}},t.Component=h,t.PureComponent=y,t.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED=I,t.cloneElement=function(e,t,r){if(null==e)throw Error(d(267,e));var a=l({},e.props),o=e.key,i=e.ref,s=e._owner;if(null!=t){if(void 0!==t.ref&&(i=t.ref,s=b.current),void 0!==t.key&&(o=""+t.key),e.type&&e.type.defaultProps)var c=e.type.defaultProps;for(u in t)E.call(t,u)&&!T.hasOwnProperty(u)&&(a[u]=void 0===t[u]&&void 0!==c?c[u]:t[u])}var u=arguments.length-2;if(1===u)a.children=r;else if(1<u){c=Array(u);for(var f=0;f<u;f++)c[f]=arguments[f+2];a.children=c}return{$$typeof:n,type:e.type,key:o,ref:i,props:a,_owner:s}},t.createContext=function(e,t){return void 0===t&&(t=null),(e={$$typeof:o,_calculateChangedBits:t,_currentValue:e,_currentValue2:e,_threadCount:0,Provider:null,Consumer:null}).Provider={$$typeof:a,_context:e},e.Consumer=e},t.createElement=S,t.createFactory=function(e){var t=S.bind(null,e);return t.type=e,t},t.createRef=function(){return{current:null}},t.forwardRef=function(e){return{$$typeof:i,render:e}},t.isValidElement=w,t.lazy=function(e){return{$$typeof:c,_payload:{_status:-1,_result:e},_init:x}},t.memo=function(e,t){return{$$typeof:s,type:e,compare:void 0===t?null:t}},t.useCallback=function(e,t){return A().useCallback(e,t)},t.useContext=function(e,t){return A().useContext(e,t)},t.useDebugValue=function(){},t.useEffect=function(e,t){return A().useEffect(e,t)},t.useImperativeHandle=function(e,t,n){return A().useImperativeHandle(e,t,n)},t.useLayoutEffect=function(e,t){return A().useLayoutEffect(e,t)},t.useMemo=function(e,t){return A().useMemo(e,t)},t.useReducer=function(e,t,n){return A().useReducer(e,t,n)},t.useRef=function(e){return A().useRef(e)},t.useState=function(e){return A().useState(e)},t.version="17.0.2"});var s=n(function(e,t){!function(){var e=l,n=60103,r=60106;t.Fragment=60107,t.StrictMode=60108,t.Profiler=60114;var a=60109,o=60110,i=60112;t.Suspense=60113;var s=60120,c=60115,u=60116,f=60121,d=60122,p=60117,m=60129,h=60131;if("function"==typeof Symbol&&Symbol.for){var g=Symbol.for;n=g("react.element"),r=g("react.portal"),t.Fragment=g("react.fragment"),t.StrictMode=g("react.strict_mode"),t.Profiler=g("react.profiler"),a=g("react.provider"),o=g("react.context"),i=g("react.forward_ref"),t.Suspense=g("react.suspense"),s=g("react.suspense_list"),c=g("react.memo"),u=g("react.lazy"),f=g("react.block"),d=g("react.server.block"),p=g("react.fundamental"),g("react.scope"),g("react.opaque.id"),m=g("react.debug_trace_mode"),g("react.offscreen"),h=g("react.legacy_hidden")}var y="function"==typeof Symbol&&Symbol.iterator;function v(e){if(null===e||"object"!=typeof e)return null;var t=y&&e[y]||e["@@iterator"];return"function"==typeof t?t:null}var b={current:null},E={current:null},T={},S=null;function w(e){S=e}T.setExtraStackFrame=function(e){S=e},T.getCurrentStack=null,T.getStackAddendum=function(){var e="";S&&(e+=S);var t=T.getCurrentStack;return t&&(e+=t()||""),e};var N={ReactCurrentDispatcher:b,ReactCurrentBatchConfig:{transition:0},ReactCurrentOwner:E,IsSomeRendererActing:{current:!1},assign:e};function R(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];C("warn",e,n)}function O(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];C("error",e,n)}function C(e,t,n){var r=N.ReactDebugCurrentFrame.getStackAddendum();""!==r&&(t+="%s",n=n.concat([r]));var a=n.map(function(e){return""+e});a.unshift("Warning: "+t),Function.prototype.apply.call(console[e],console,a)}N.ReactDebugCurrentFrame=T;var x={};function k(e,t){var n=e.constructor,r=n&&(n.displayName||n.name)||"ReactClass",a=r+"."+t;x[a]||(O("Can't call %s on a component that is not yet mounted. This is a no-op, but it might indicate a bug in your application. Instead, assign to `this.state` directly or define a `state = {};` class property with the desired state in the %s component.",t,r),x[a]=!0)}var A={isMounted:function(e){return!1},enqueueForceUpdate:function(e,t,n){k(e,"forceUpdate")},enqueueReplaceState:function(e,t,n,r){k(e,"replaceState")},enqueueSetState:function(e,t,n,r){k(e,"setState")}},I={};function L(e,t,n){this.props=e,this.context=t,this.refs=I,this.updater=n||A}Object.freeze(I),L.prototype.isReactComponent={},L.prototype.setState=function(e,t){if("object"!=typeof e&&"function"!=typeof e&&null!=e)throw Error("setState(...): takes an object of state variables to update or a function which returns an object of state variables.");this.updater.enqueueSetState(this,e,t,"setState")},L.prototype.forceUpdate=function(e){this.updater.enqueueForceUpdate(this,e,"forceUpdate")};var _={isMounted:["isMounted","Instead, make sure to clean up subscriptions and pending requests in componentWillUnmount to prevent memory leaks."],replaceState:["replaceState","Refactor your code to use setState instead (see https://github.com/facebook/react/issues/3236)."]},P=function(e,t){Object.defineProperty(L.prototype,e,{get:function(){R("%s(...) is deprecated in plain JavaScript React classes. %s",t[0],t[1])}})};for(var M in _)_.hasOwnProperty(M)&&P(M,_[M]);function D(){}function U(e,t,n){this.props=e,this.context=t,this.refs=I,this.updater=n||A}D.prototype=L.prototype;var j=U.prototype=new D;function F(e){return e.displayName||"Context"}function z(e){if(null==e)return null;if("number"==typeof e.tag&&O("Received an unexpected object in getComponentName(). This is likely a bug in React. Please file an issue."),"function"==typeof e)return e.displayName||e.name||null;if("string"==typeof e)return e;switch(e){case t.Fragment:return"Fragment";case r:return"Portal";case t.Profiler:return"Profiler";case t.StrictMode:return"StrictMode";case t.Suspense:return"Suspense";case s:return"SuspenseList"}if("object"==typeof e)switch(e.$$typeof){case o:return F(e)+".Consumer";case a:return F(e._context)+".Provider";case i:return p=(d=e.render).displayName||d.name||"",e.displayName||(""!==p?"ForwardRef("+p+")":"ForwardRef");case c:return z(e.type);case f:return z(e._render);case u:var n=e._payload,l=e._init;try{return z(l(n))}catch(e){return null}}var d,p;return null}j.constructor=U,e(j,L.prototype),j.isPureReactComponent=!0;var B,H,V,W=Object.prototype.hasOwnProperty,G={key:!0,ref:!0,__self:!0,__source:!0};function Y(e){if(W.call(e,"ref")){var t=Object.getOwnPropertyDescriptor(e,"ref").get;if(t&&t.isReactWarning)return!1}return void 0!==e.ref}function $(e){if(W.call(e,"key")){var t=Object.getOwnPropertyDescriptor(e,"key").get;if(t&&t.isReactWarning)return!1}return void 0!==e.key}function X(e,t){var n=function(){B||(B=!0,O("%s: `key` is not a prop. Trying to access it will result in `undefined` being returned. If you need to access the same value within the child component, you should pass it as a different prop. (https://reactjs.org/link/special-props)",t))};n.isReactWarning=!0,Object.defineProperty(e,"key",{get:n,configurable:!0})}function q(e,t){var n=function(){H||(H=!0,O("%s: `ref` is not a prop. Trying to access it will result in `undefined` being returned. If you need to access the same value within the child component, you should pass it as a different prop. (https://reactjs.org/link/special-props)",t))};n.isReactWarning=!0,Object.defineProperty(e,"ref",{get:n,configurable:!0})}function K(e){if("string"==typeof e.ref&&E.current&&e.__self&&E.current.stateNode!==e.__self){var t=z(E.current.type);V[t]||(O('Component "%s" contains the string ref "%s". Support for string refs will be removed in a future major release. This case cannot be automatically converted to an arrow function. We ask you to manually fix this case by using useRef() or createRef() instead. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-string-ref',t,e.ref),V[t]=!0)}}V={};var J=function(e,t,r,a,o,i,l){var s={$$typeof:n,type:e,key:t,ref:r,props:l,_owner:i,_store:{}};return Object.defineProperty(s._store,"validated",{configurable:!1,enumerable:!1,writable:!0,value:!1}),Object.defineProperty(s,"_self",{configurable:!1,enumerable:!1,writable:!1,value:a}),Object.defineProperty(s,"_source",{configurable:!1,enumerable:!1,writable:!1,value:o}),Object.freeze&&(Object.freeze(s.props),Object.freeze(s)),s};function Q(e,t,n){var r,a={},o=null,i=null,l=null,s=null;if(null!=t)for(r in Y(t)&&(i=t.ref,K(t)),$(t)&&(o=""+t.key),l=void 0===t.__self?null:t.__self,s=void 0===t.__source?null:t.__source,t)W.call(t,r)&&!G.hasOwnProperty(r)&&(a[r]=t[r]);var c=arguments.length-2;if(1===c)a.children=n;else if(c>1){for(var u=Array(c),f=0;f<c;f++)u[f]=arguments[f+2];Object.freeze&&Object.freeze(u),a.children=u}if(e&&e.defaultProps){var d=e.defaultProps;for(r in d)void 0===a[r]&&(a[r]=d[r])}if(o||i){var p="function"==typeof e?e.displayName||e.name||"Unknown":e;o&&X(a,p),i&&q(a,p)}return J(e,o,i,l,s,E.current,a)}function Z(t,n,r){if(null==t)throw Error("React.cloneElement(...): The argument must be a React element, but you passed "+t+".");var a,o,i=e({},t.props),l=t.key,s=t.ref,c=t._self,u=t._source,f=t._owner;if(null!=n)for(a in Y(n)&&(s=n.ref,f=E.current),$(n)&&(l=""+n.key),t.type&&t.type.defaultProps&&(o=t.type.defaultProps),n)W.call(n,a)&&!G.hasOwnProperty(a)&&(i[a]=void 0===n[a]&&void 0!==o?o[a]:n[a]);var d=arguments.length-2;if(1===d)i.children=r;else if(d>1){for(var p=Array(d),m=0;m<d;m++)p[m]=arguments[m+2];i.children=p}return J(t.type,l,s,c,u,f,i)}function ee(e){return"object"==typeof e&&null!==e&&e.$$typeof===n}var te=!1,ne=/\/+/g;function re(e){return e.replace(ne,"$&/")}function ae(e,t){return"object"==typeof e&&null!==e&&null!=e.key?(n={"=":"=0",":":"=2"},"$"+(""+e.key).replace(/[=:]/g,function(e){return n[e]})):t.toString(36);var n}function oe(e,t,a,o,i){var l=typeof e;"undefined"!==l&&"boolean"!==l||(e=null);var s,c,u,f=!1;if(null===e)f=!0;else switch(l){case"string":case"number":f=!0;break;case"object":switch(e.$$typeof){case n:case r:f=!0}}if(f){var d=e,p=i(d),m=""===o?"."+ae(d,0):o;if(Array.isArray(p)){var h="";null!=m&&(h=re(m)+"/"),oe(p,t,h,"",function(e){return e})}else null!=p&&(ee(p)&&(s=p,c=a+(!p.key||d&&d.key===p.key?"":re(""+p.key)+"/")+m,p=J(s.type,c,s.ref,s._self,s._source,s._owner,s.props)),t.push(p));return 1}var g=0,y=""===o?".":o+":";if(Array.isArray(e))for(var b=0;b<e.length;b++)g+=oe(u=e[b],t,a,y+ae(u,b),i);else{var E=v(e);if("function"==typeof E){var T=e;E===T.entries&&(te||R("Using Maps as children is not supported. Use an array of keyed ReactElements instead."),te=!0);for(var S,w=E.call(T),N=0;!(S=w.next()).done;)g+=oe(u=S.value,t,a,y+ae(u,N++),i)}else if("object"===l){var O=""+e;throw Error("Objects are not valid as a React child (found: "+("[object Object]"===O?"object with keys {"+Object.keys(e).join(", ")+"}":O)+"). If you meant to render a collection of children, use an array instead.")}}return g}function ie(e,t,n){if(null==e)return e;var r=[],a=0;return oe(e,r,"","",function(e){return t.call(n,e,a++)}),r}function le(e){if(-1===e._status){var t=(0,e._result)(),n=e;n._status=0,n._result=t,t.then(function(t){if(0===e._status){var n=t.default;void 0===n&&O("lazy: Expected the result of a dynamic import() call. Instead received: %s\n\nYour code should look like: \n const MyComponent = lazy(() => import('./MyComponent'))",t);var r=e;r._status=1,r._result=n}},function(t){if(0===e._status){var n=e;n._status=2,n._result=t}})}if(1===e._status)return e._result;throw e._result}function se(e){return"string"==typeof e||"function"==typeof e||e===t.Fragment||e===t.Profiler||e===m||e===t.StrictMode||e===t.Suspense||e===s||e===h||"object"==typeof e&&null!==e&&(e.$$typeof===u||e.$$typeof===c||e.$$typeof===a||e.$$typeof===o||e.$$typeof===i||e.$$typeof===p||e.$$typeof===f||e[0]===d)}function ce(){var e=b.current;if(null===e)throw Error("Invalid hook call. Hooks can only be called inside of the body of a function component. This could happen for one of the following reasons:\n1. You might have mismatching versions of React and the renderer (such as React DOM)\n2. You might be breaking the Rules of Hooks\n3. You might have more than one copy of React in the same app\nSee https://reactjs.org/link/invalid-hook-call for tips about how to debug and fix this problem.");return e}var ue,fe,de,pe,me,he,ge,ye=0;function ve(){}ve.__reactDisabledLog=!0;var be,Ee=N.ReactCurrentDispatcher;function Te(e,t,n){if(void 0===be)try{throw Error()}catch(e){var r=e.stack.trim().match(/\n( *(at )?)/);be=r&&r[1]||""}return"\n"+be+e}var Se,we=!1,Ne="function"==typeof WeakMap?WeakMap:Map;function Re(t,n){if(!t||we)return"";var r,a=Se.get(t);if(void 0!==a)return a;we=!0;var o,i=Error.prepareStackTrace;Error.prepareStackTrace=void 0,o=Ee.current,Ee.current=null,function(){if(0===ye){ue=console.log,fe=console.info,de=console.warn,pe=console.error,me=console.group,he=console.groupCollapsed,ge=console.groupEnd;var e={configurable:!0,enumerable:!0,value:ve,writable:!0};Object.defineProperties(console,{info:e,log:e,warn:e,error:e,group:e,groupCollapsed:e,groupEnd:e})}ye++}();try{if(n){var l=function(){throw Error()};if(Object.defineProperty(l.prototype,"props",{set:function(){throw Error()}}),"object"==typeof Reflect&&Reflect.construct){try{Reflect.construct(l,[])}catch(e){r=e}Reflect.construct(t,[],l)}else{try{l.call()}catch(e){r=e}t.call(l.prototype)}}else{try{throw Error()}catch(e){r=e}t()}}catch(e){if(e&&r&&"string"==typeof e.stack){for(var s=e.stack.split("\n"),c=r.stack.split("\n"),u=s.length-1,f=c.length-1;u>=1&&f>=0&&s[u]!==c[f];)f--;for(;u>=1&&f>=0;u--,f--)if(s[u]!==c[f]){if(1!==u||1!==f)do{if(u--,--f<0||s[u]!==c[f]){var d="\n"+s[u].replace(" at new "," at ");return"function"==typeof t&&Se.set(t,d),d}}while(u>=1&&f>=0);break}}}finally{we=!1,Ee.current=o,function(){if(0==--ye){var t={configurable:!0,enumerable:!0,writable:!0};Object.defineProperties(console,{log:e({},t,{value:ue}),info:e({},t,{value:fe}),warn:e({},t,{value:de}),error:e({},t,{value:pe}),group:e({},t,{value:me}),groupCollapsed:e({},t,{value:he}),groupEnd:e({},t,{value:ge})})}ye<0&&O("disabledDepth fell below zero. This is a bug in React. Please file an issue.")}(),Error.prepareStackTrace=i}var p=t?t.displayName||t.name:"",m=p?Te(p):"";return"function"==typeof t&&Se.set(t,m),m}function Oe(e,t,n){return Re(e,!1)}function Ce(e,n,r){if(null==e)return"";if("function"==typeof e)return Re(e,function(e){var t=e.prototype;return!(!t||!t.isReactComponent)}(e));if("string"==typeof e)return Te(e);switch(e){case t.Suspense:return Te("Suspense");case s:return Te("SuspenseList")}if("object"==typeof e)switch(e.$$typeof){case i:return Oe(e.render);case c:return Ce(e.type,n,r);case f:return Oe(e._render);case u:var a=e._payload,o=e._init;try{return Ce(o(a),n,r)}catch(e){}}return""}Se=new Ne;var xe,ke={},Ae=N.ReactDebugCurrentFrame;function Ie(e){if(e){var t=e._owner,n=Ce(e.type,e._source,t?t.type:null);Ae.setExtraStackFrame(n)}else Ae.setExtraStackFrame(null)}function Le(e){if(e){var t=e._owner;w(Ce(e.type,e._source,t?t.type:null))}else w(null)}function _e(){if(E.current){var e=z(E.current.type);if(e)return"\n\nCheck the render method of `"+e+"`."}return""}function Pe(e){return null!=e?function(e){return void 0!==e?"\n\nCheck your code at "+e.fileName.replace(/^.*[\\\/]/,"")+":"+e.lineNumber+".":""}(e.__source):""}xe=!1;var Me={};function De(e,t){if(e._store&&!e._store.validated&&null==e.key){e._store.validated=!0;var n=function(e){var t=_e();if(!t){var n="string"==typeof e?e:e.displayName||e.name;n&&(t="\n\nCheck the top-level render call using <"+n+">.")}return t}(t);if(!Me[n]){Me[n]=!0;var r="";e&&e._owner&&e._owner!==E.current&&(r=" It was passed a child from "+z(e._owner.type)+"."),Le(e),O('Each child in a list should have a unique "key" prop.%s%s See https://reactjs.org/link/warning-keys for more information.',n,r),Le(null)}}}function Ue(e,t){if("object"==typeof e)if(Array.isArray(e))for(var n=0;n<e.length;n++){var r=e[n];ee(r)&&De(r,t)}else if(ee(e))e._store&&(e._store.validated=!0);else if(e){var a=v(e);if("function"==typeof a&&a!==e.entries)for(var o,i=a.call(e);!(o=i.next()).done;)ee(o.value)&&De(o.value,t)}}function je(e){var t,n=e.type;if(null!=n&&"string"!=typeof n){if("function"==typeof n)t=n.propTypes;else{if("object"!=typeof n||n.$$typeof!==i&&n.$$typeof!==c)return;t=n.propTypes}if(t){var r=z(n);!function(e,t,n,r,a){var o=Function.call.bind(Object.prototype.hasOwnProperty);for(var i in e)if(o(e,i)){var l=void 0;try{if("function"!=typeof e[i]){var s=Error((r||"React class")+": prop type `"+i+"` is invalid; it must be a function, usually from the `prop-types` package, but received `"+typeof e[i]+"`.This often happens because of typos such as `PropTypes.function` instead of `PropTypes.func`.");throw s.name="Invariant Violation",s}l=e[i](t,i,r,n,null,"SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED")}catch(e){l=e}!l||l instanceof Error||(Ie(a),O("%s: type specification of %s `%s` is invalid; the type checker function must return `null` or an `Error` but returned a %s. You may have forgotten to pass an argument to the type checker creator (arrayOf, instanceOf, objectOf, oneOf, oneOfType, and shape all require an argument).",r||"React class",n,i,typeof l),Ie(null)),l instanceof Error&&!(l.message in ke)&&(ke[l.message]=!0,Ie(a),O("Failed %s type: %s",n,l.message),Ie(null))}}(t,e.props,"prop",r,e)}else void 0===n.PropTypes||xe||(xe=!0,O("Component %s declared `PropTypes` instead of `propTypes`. Did you misspell the property assignment?",z(n)||"Unknown"));"function"!=typeof n.getDefaultProps||n.getDefaultProps.isReactClassApproved||O("getDefaultProps is only used on classic React.createClass definitions. Use a static property named `defaultProps` instead.")}}function Fe(e){for(var t=Object.keys(e.props),n=0;n<t.length;n++){var r=t[n];if("children"!==r&&"key"!==r){Le(e),O("Invalid prop `%s` supplied to `React.Fragment`. React.Fragment can only have `key` and `children` props.",r),Le(null);break}}null!==e.ref&&(Le(e),O("Invalid attribute `ref` supplied to `React.Fragment`."),Le(null))}function ze(e,r,a){var o=se(e);if(!o){var i="";(void 0===e||"object"==typeof e&&null!==e&&0===Object.keys(e).length)&&(i+=" You likely forgot to export your component from the file it's defined in, or you might have mixed up default and named imports.");var l,s=Pe(r);i+=s||_e(),null===e?l="null":Array.isArray(e)?l="array":void 0!==e&&e.$$typeof===n?(l="<"+(z(e.type)||"Unknown")+" />",i=" Did you accidentally export a JSX literal instead of a component?"):l=typeof e,O("React.createElement: type is invalid -- expected a string (for built-in components) or a class/function (for composite components) but got: %s.%s",l,i)}var c=Q.apply(this,arguments);if(null==c)return c;if(o)for(var u=2;u<arguments.length;u++)Ue(arguments[u],e);return e===t.Fragment?Fe(c):je(c),c}var Be=!1;try{var He=Object.freeze({});new Map([[He,null]]),new Set([He])}catch(e){}var Ve=ze;t.Children={map:ie,forEach:function(e,t,n){ie(e,function(){t.apply(this,arguments)},n)},count:function(e){var t=0;return ie(e,function(){t++}),t},toArray:function(e){return ie(e,function(e){return e})||[]},only:function(e){if(!ee(e))throw Error("React.Children.only expected to receive a single React element child.");return e}},t.Component=L,t.PureComponent=U,t.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED=N,t.cloneElement=function(e,t,n){for(var r=Z.apply(this,arguments),a=2;a<arguments.length;a++)Ue(arguments[a],r.type);return je(r),r},t.createContext=function(e,t){void 0===t?t=null:null!==t&&"function"!=typeof t&&O("createContext: Expected the optional second argument to be a function. Instead received: %s",t);var n={$$typeof:o,_calculateChangedBits:t,_currentValue:e,_currentValue2:e,_threadCount:0,Provider:null,Consumer:null};n.Provider={$$typeof:a,_context:n};var r=!1,i=!1,l=!1,s={$$typeof:o,_context:n,_calculateChangedBits:n._calculateChangedBits};return Object.defineProperties(s,{Provider:{get:function(){return i||(i=!0,O("Rendering <Context.Consumer.Provider> is not supported and will be removed in a future major release. Did you mean to render <Context.Provider> instead?")),n.Provider},set:function(e){n.Provider=e}},_currentValue:{get:function(){return n._currentValue},set:function(e){n._currentValue=e}},_currentValue2:{get:function(){return n._currentValue2},set:function(e){n._currentValue2=e}},_threadCount:{get:function(){return n._threadCount},set:function(e){n._threadCount=e}},Consumer:{get:function(){return r||(r=!0,O("Rendering <Context.Consumer.Consumer> is not supported and will be removed in a future major release. Did you mean to render <Context.Consumer> instead?")),n.Consumer}},displayName:{get:function(){return n.displayName},set:function(e){l||(R("Setting `displayName` on Context.Consumer has no effect. You should set it directly on the context with Context.displayName = '%s'.",e),l=!0)}}}),n.Consumer=s,n._currentRenderer=null,n._currentRenderer2=null,n},t.createElement=Ve,t.createFactory=function(e){var t=ze.bind(null,e);return t.type=e,Be||(Be=!0,R("React.createFactory() is deprecated and will be removed in a future major release. Consider using JSX or use React.createElement() directly instead.")),Object.defineProperty(t,"type",{enumerable:!1,get:function(){return R("Factory.type is deprecated. Access the class directly before passing it to createFactory."),Object.defineProperty(this,"type",{value:e}),e}}),t},t.createRef=function(){var e={current:null};return Object.seal(e),e},t.forwardRef=function(e){null!=e&&e.$$typeof===c?O("forwardRef requires a render function but received a `memo` component. Instead of forwardRef(memo(...)), use memo(forwardRef(...))."):"function"!=typeof e?O("forwardRef requires a render function but was given %s.",null===e?"null":typeof e):0!==e.length&&2!==e.length&&O("forwardRef render functions accept exactly two parameters: props and ref. %s",1===e.length?"Did you forget to use the ref parameter?":"Any additional parameter will be undefined."),null!=e&&(null==e.defaultProps&&null==e.propTypes||O("forwardRef render functions do not support propTypes or defaultProps. Did you accidentally pass a React component?"));var t,n={$$typeof:i,render:e};return Object.defineProperty(n,"displayName",{enumerable:!1,configurable:!0,get:function(){return t},set:function(n){t=n,null==e.displayName&&(e.displayName=n)}}),n},t.isValidElement=ee,t.lazy=function(e){var t,n,r={$$typeof:u,_payload:{_status:-1,_result:e},_init:le};return Object.defineProperties(r,{defaultProps:{configurable:!0,get:function(){return t},set:function(e){O("React.lazy(...): It is not supported to assign `defaultProps` to a lazy component import. Either specify them where the component is defined, or create a wrapping component around it."),t=e,Object.defineProperty(r,"defaultProps",{enumerable:!0})}},propTypes:{configurable:!0,get:function(){return n},set:function(e){O("React.lazy(...): It is not supported to assign `propTypes` to a lazy component import. Either specify them where the component is defined, or create a wrapping component around it."),n=e,Object.defineProperty(r,"propTypes",{enumerable:!0})}}}),r},t.memo=function(e,t){se(e)||O("memo: The first argument must be a component. Instead received: %s",null===e?"null":typeof e);var n,r={$$typeof:c,type:e,compare:void 0===t?null:t};return Object.defineProperty(r,"displayName",{enumerable:!1,configurable:!0,get:function(){return n},set:function(t){n=t,null==e.displayName&&(e.displayName=t)}}),r},t.useCallback=function(e,t){return ce().useCallback(e,t)},t.useContext=function(e,t){var n=ce();if(void 0!==t&&O("useContext() second argument is reserved for future use in React. Passing it is not supported. You passed: %s.%s",t,"number"==typeof t&&Array.isArray(arguments[2])?"\n\nDid you call array.map(useContext)? Calling Hooks inside a loop is not supported. Learn more at https://reactjs.org/link/rules-of-hooks":""),void 0!==e._context){var r=e._context;r.Consumer===e?O("Calling useContext(Context.Consumer) is not supported, may cause bugs, and will be removed in a future major release. Did you mean to call useContext(Context) instead?"):r.Provider===e&&O("Calling useContext(Context.Provider) is not supported. Did you mean to call useContext(Context) instead?")}return n.useContext(e,t)},t.useDebugValue=function(e,t){return ce().useDebugValue(e,t)},t.useEffect=function(e,t){return ce().useEffect(e,t)},t.useImperativeHandle=function(e,t,n){return ce().useImperativeHandle(e,t,n)},t.useLayoutEffect=function(e,t){return ce().useLayoutEffect(e,t)},t.useMemo=function(e,t){return ce().useMemo(e,t)},t.useReducer=function(e,t,n){return ce().useReducer(e,t,n)},t.useRef=function(e){return ce().useRef(e)},t.useState=function(e){return ce().useState(e)},t.version="17.0.2"}()}),c=n(function(e){e.exports=s});n(function(e,t){var n,r,a,o;if("object"==typeof performance&&"function"==typeof performance.now){var i=performance;t.unstable_now=function(){return i.now()}}else{var l=Date,s=l.now();t.unstable_now=function(){return l.now()-s}}if("undefined"==typeof window||"function"!=typeof MessageChannel){var c=null,u=null,f=function(){if(null!==c)try{var e=t.unstable_now();c(!0,e),c=null}catch(e){throw setTimeout(f,0),e}};n=function(e){null!==c?setTimeout(n,0,e):(c=e,setTimeout(f,0))},r=function(e,t){u=setTimeout(e,t)},a=function(){clearTimeout(u)},t.unstable_shouldYield=function(){return!1},o=t.unstable_forceFrameRate=function(){}}else{var d=window.setTimeout,p=window.clearTimeout;if("undefined"!=typeof console){var m=window.cancelAnimationFrame;"function"!=typeof window.requestAnimationFrame&&console.error("This browser doesn't support requestAnimationFrame. Make sure that you load a polyfill in older browsers. https://reactjs.org/link/react-polyfills"),"function"!=typeof m&&console.error("This browser doesn't support cancelAnimationFrame. Make sure that you load a polyfill in older browsers. https://reactjs.org/link/react-polyfills")}var h=!1,g=null,y=-1,v=5,b=0;t.unstable_shouldYield=function(){return t.unstable_now()>=b},o=function(){},t.unstable_forceFrameRate=function(e){0>e||125<e?console.error("forceFrameRate takes a positive int between 0 and 125, forcing frame rates higher than 125 fps is not supported"):v=0<e?Math.floor(1e3/e):5};var E=new MessageChannel,T=E.port2;E.port1.onmessage=function(){if(null!==g){var e=t.unstable_now();b=e+v;try{g(!0,e)?T.postMessage(null):(h=!1,g=null)}catch(e){throw T.postMessage(null),e}}else h=!1},n=function(e){g=e,h||(h=!0,T.postMessage(null))},r=function(e,n){y=d(function(){e(t.unstable_now())},n)},a=function(){p(y),y=-1}}function S(e,t){var n=e.length;e.push(t);e:for(;;){var r=n-1>>>1,a=e[r];if(!(void 0!==a&&0<R(a,t)))break e;e[r]=t,e[n]=a,n=r}}function w(e){return void 0===(e=e[0])?null:e}function N(e){var t=e[0];if(void 0!==t){var n=e.pop();if(n!==t){e[0]=n;e:for(var r=0,a=e.length;r<a;){var o=2*(r+1)-1,i=e[o],l=o+1,s=e[l];if(void 0!==i&&0>R(i,n))void 0!==s&&0>R(s,i)?(e[r]=s,e[l]=n,r=l):(e[r]=i,e[o]=n,r=o);else{if(!(void 0!==s&&0>R(s,n)))break e;e[r]=s,e[l]=n,r=l}}}return t}return null}function R(e,t){var n=e.sortIndex-t.sortIndex;return 0!==n?n:e.id-t.id}var O=[],C=[],x=1,k=null,A=3,I=!1,L=!1,_=!1;function P(e){for(var t=w(C);null!==t;){if(null===t.callback)N(C);else{if(!(t.startTime<=e))break;N(C),t.sortIndex=t.expirationTime,S(O,t)}t=w(C)}}function M(e){if(_=!1,P(e),!L)if(null!==w(O))L=!0,n(D);else{var t=w(C);null!==t&&r(M,t.startTime-e)}}function D(e,n){L=!1,_&&(_=!1,a()),I=!0;var o=A;try{for(P(n),k=w(O);null!==k&&(!(k.expirationTime>n)||e&&!t.unstable_shouldYield());){var i=k.callback;if("function"==typeof i){k.callback=null,A=k.priorityLevel;var l=i(k.expirationTime<=n);n=t.unstable_now(),"function"==typeof l?k.callback=l:k===w(O)&&N(O),P(n)}else N(O);k=w(O)}if(null!==k)var s=!0;else{var c=w(C);null!==c&&r(M,c.startTime-n),s=!1}return s}finally{k=null,A=o,I=!1}}var U=o;t.unstable_IdlePriority=5,t.unstable_ImmediatePriority=1,t.unstable_LowPriority=4,t.unstable_NormalPriority=3,t.unstable_Profiling=null,t.unstable_UserBlockingPriority=2,t.unstable_cancelCallback=function(e){e.callback=null},t.unstable_continueExecution=function(){L||I||(L=!0,n(D))},t.unstable_getCurrentPriorityLevel=function(){return A},t.unstable_getFirstCallbackNode=function(){return w(O)},t.unstable_next=function(e){switch(A){case 1:case 2:case 3:var t=3;break;default:t=A}var n=A;A=t;try{return e()}finally{A=n}},t.unstable_pauseExecution=function(){},t.unstable_requestPaint=U,t.unstable_runWithPriority=function(e,t){switch(e){case 1:case 2:case 3:case 4:case 5:break;default:e=3}var n=A;A=e;try{return t()}finally{A=n}},t.unstable_scheduleCallback=function(e,o,i){var l=t.unstable_now();switch(i="object"==typeof i&&null!==i&&"number"==typeof(i=i.delay)&&0<i?l+i:l,e){case 1:var s=-1;break;case 2:s=250;break;case 5:s=1073741823;break;case 4:s=1e4;break;default:s=5e3}return e={id:x++,callback:o,priorityLevel:e,startTime:i,expirationTime:s=i+s,sortIndex:-1},i>l?(e.sortIndex=i,S(C,e),null===w(O)&&e===w(C)&&(_?a():_=!0,r(M,i-l))):(e.sortIndex=s,S(O,e),L||I||(L=!0,n(D))),e},t.unstable_wrapCallback=function(e){var t=A;return function(){var n=A;A=t;try{return e.apply(this,arguments)}finally{A=n}}}});var u=n(function(e,t){!function(){var e,n,r,a;if("object"==typeof performance&&"function"==typeof performance.now){var o=performance;t.unstable_now=function(){return o.now()}}else{var i=Date,l=i.now();t.unstable_now=function(){return i.now()-l}}if("undefined"==typeof window||"function"!=typeof MessageChannel){var s=null,c=null,u=function(){if(null!==s)try{var e=t.unstable_now();s(!0,e),s=null}catch(e){throw setTimeout(u,0),e}};e=function(t){null!==s?setTimeout(e,0,t):(s=t,setTimeout(u,0))},n=function(e,t){c=setTimeout(e,t)},r=function(){clearTimeout(c)},t.unstable_shouldYield=function(){return!1},a=t.unstable_forceFrameRate=function(){}}else{var f=window.setTimeout,d=window.clearTimeout;if("undefined"!=typeof console){var p=window.requestAnimationFrame,m=window.cancelAnimationFrame;"function"!=typeof p&&console.error("This browser doesn't support requestAnimationFrame. Make sure that you load a polyfill in older browsers. https://reactjs.org/link/react-polyfills"),"function"!=typeof m&&console.error("This browser doesn't support cancelAnimationFrame. Make sure that you load a polyfill in older browsers. https://reactjs.org/link/react-polyfills")}var h=!1,g=null,y=-1,v=5,b=0;t.unstable_shouldYield=function(){return t.unstable_now()>=b},a=function(){},t.unstable_forceFrameRate=function(e){e<0||e>125?console.error("forceFrameRate takes a positive int between 0 and 125, forcing frame rates higher than 125 fps is not supported"):v=e>0?Math.floor(1e3/e):5};var E=new MessageChannel,T=E.port2;E.port1.onmessage=function(){if(null!==g){var e=t.unstable_now();b=e+v;try{g(!0,e)?T.postMessage(null):(h=!1,g=null)}catch(e){throw T.postMessage(null),e}}else h=!1},e=function(e){g=e,h||(h=!0,T.postMessage(null))},n=function(e,n){y=f(function(){e(t.unstable_now())},n)},r=function(){d(y),y=-1}}function S(e,t){var n=e.length;e.push(t),function(e,t,n){for(var r=n;;){var a=r-1>>>1,o=e[a];if(!(void 0!==o&&R(o,t)>0))return;e[a]=t,e[r]=o,r=a}}(e,t,n)}function w(e){var t=e[0];return void 0===t?null:t}function N(e){var t=e[0];if(void 0!==t){var n=e.pop();return n!==t&&(e[0]=n,function(e,t,n){for(var r=0,a=e.length;r<a;){var o=2*(r+1)-1,i=e[o],l=o+1,s=e[l];if(void 0!==i&&R(i,t)<0)void 0!==s&&R(s,i)<0?(e[r]=s,e[l]=t,r=l):(e[r]=i,e[o]=t,r=o);else{if(!(void 0!==s&&R(s,t)<0))return;e[r]=s,e[l]=t,r=l}}}(e,n)),t}return null}function R(e,t){var n=e.sortIndex-t.sortIndex;return 0!==n?n:e.id-t.id}var O=[],C=[],x=1,k=null,A=3,I=!1,L=!1,_=!1;function P(e){for(var t=w(C);null!==t;){if(null===t.callback)N(C);else{if(!(t.startTime<=e))return;N(C),t.sortIndex=t.expirationTime,S(O,t)}t=w(C)}}function M(t){if(_=!1,P(t),!L)if(null!==w(O))L=!0,e(D);else{var r=w(C);null!==r&&n(M,r.startTime-t)}}function D(e,a){L=!1,_&&(_=!1,r()),I=!0;var o=A;try{return function(e,r){var a=r;for(P(a),k=w(O);null!==k&&(!(k.expirationTime>a)||e&&!t.unstable_shouldYield());){var o=k.callback;if("function"==typeof o){k.callback=null,A=k.priorityLevel;var i=o(k.expirationTime<=a);a=t.unstable_now(),"function"==typeof i?k.callback=i:k===w(O)&&N(O),P(a)}else N(O);k=w(O)}if(null!==k)return!0;var l=w(C);return null!==l&&n(M,l.startTime-a),!1}(e,a)}finally{k=null,A=o,I=!1}}var U=a;t.unstable_IdlePriority=5,t.unstable_ImmediatePriority=1,t.unstable_LowPriority=4,t.unstable_NormalPriority=3,t.unstable_Profiling=null,t.unstable_UserBlockingPriority=2,t.unstable_cancelCallback=function(e){e.callback=null},t.unstable_continueExecution=function(){L||I||(L=!0,e(D))},t.unstable_getCurrentPriorityLevel=function(){return A},t.unstable_getFirstCallbackNode=function(){return w(O)},t.unstable_next=function(e){var t;switch(A){case 1:case 2:case 3:t=3;break;default:t=A}var n=A;A=t;try{return e()}finally{A=n}},t.unstable_pauseExecution=function(){},t.unstable_requestPaint=U,t.unstable_runWithPriority=function(e,t){switch(e){case 1:case 2:case 3:case 4:case 5:break;default:e=3}var n=A;A=e;try{return t()}finally{A=n}},t.unstable_scheduleCallback=function(a,o,i){var l,s,c=t.unstable_now();if("object"==typeof i&&null!==i){var u=i.delay;l="number"==typeof u&&u>0?c+u:c}else l=c;switch(a){case 1:s=-1;break;case 2:s=250;break;case 5:s=1073741823;break;case 4:s=1e4;break;case 3:default:s=5e3}var f=l+s,d={id:x++,callback:o,priorityLevel:a,startTime:l,expirationTime:f,sortIndex:-1};return l>c?(d.sortIndex=l,S(C,d),null===w(O)&&d===w(C)&&(_?r():_=!0,n(M,l-c))):(d.sortIndex=f,S(O,d),L||I||(L=!0,e(D))),d},t.unstable_wrapCallback=function(e){var t=A;return function(){var n=A;A=t;try{return e.apply(this,arguments)}finally{A=n}}}}()}),f=n(function(e){e.exports=u});function d(e){for(var t="https://reactjs.org/docs/error-decoder.html?invariant="+e,n=1;n<arguments.length;n++)t+="&args[]="+encodeURIComponent(arguments[n]);return"Minified React error #"+e+"; visit "+t+" for the full message or use the non-minified dev environment for full errors and additional helpful warnings."}if(!c)throw Error(d(227));var p=new Set;function m(e,t){h(e,t),h(e+"Capture",t)}function h(e,t){for(e=0;e<t.length;e++)p.add(t[e])}var g=!("undefined"==typeof window||void 0===window.document||void 0===window.document.createElement);function y(e,t,n,r,a,o,i){this.acceptsBooleans=2===t||3===t||4===t,this.attributeName=r,this.attributeNamespace=a,this.mustUseProperty=n,this.propertyName=e,this.type=t,this.sanitizeURL=o,this.removeEmptyString=i}["contentEditable","draggable","spellCheck","value"].forEach(function(e){new y(e,2,!1,e.toLowerCase(),null,!1,!1)}),"allowFullScreen async autoFocus autoPlay controls default defer disabled disablePictureInPicture disableRemotePlayback formNoValidate hidden loop noModule noValidate open playsInline readOnly required reversed scoped seamless itemScope".split(" ").forEach(function(e){new y(e,3,!1,e.toLowerCase(),null,!1,!1)}),["rowSpan","start"].forEach(function(e){new y(e,5,!1,e.toLowerCase(),null,!1,!1)});var v=/[\-:]([a-z])/g;function b(e){return e[1].toUpperCase()}"accent-height alignment-baseline arabic-form baseline-shift cap-height clip-path clip-rule color-interpolation color-interpolation-filters color-profile color-rendering dominant-baseline enable-background fill-opacity fill-rule flood-color flood-opacity font-family font-size font-size-adjust font-stretch font-style font-variant font-weight glyph-name glyph-orientation-horizontal glyph-orientation-vertical horiz-adv-x horiz-origin-x image-rendering letter-spacing lighting-color marker-end marker-mid marker-start overline-position overline-thickness paint-order panose-1 pointer-events rendering-intent shape-rendering stop-color stop-opacity strikethrough-position strikethrough-thickness stroke-dasharray stroke-dashoffset stroke-linecap stroke-linejoin stroke-miterlimit stroke-opacity stroke-width text-anchor text-decoration text-rendering underline-position underline-thickness unicode-bidi unicode-range units-per-em v-alphabetic v-hanging v-ideographic v-mathematical vector-effect vert-adv-y vert-origin-x vert-origin-y word-spacing writing-mode xmlns:xlink x-height".split(" ").forEach(function(e){e.replace(v,b)}),"xlink:actuate xlink:arcrole xlink:role xlink:show xlink:title xlink:type".split(" ").forEach(function(e){e.replace(v,b)}),["xml:base","xml:lang","xml:space"].forEach(function(e){e.replace(v,b)}),["tabIndex","crossOrigin"].forEach(function(e){new y(e,1,!1,e.toLowerCase(),null,!1,!1)}),["src","href","action","formAction"].forEach(function(e){new y(e,1,!1,e.toLowerCase(),null,!0,!0)});var E=c.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED;if("function"==typeof Symbol&&Symbol.for){var T=Symbol.for;T("react.element"),T("react.portal"),T("react.fragment"),T("react.strict_mode"),T("react.profiler"),T("react.provider"),T("react.context"),T("react.forward_ref"),T("react.suspense"),T("react.suspense_list"),T("react.memo"),T("react.lazy"),T("react.block"),T("react.scope"),T("react.opaque.id"),T("react.debug_trace_mode"),T("react.offscreen"),T("react.legacy_hidden")}"undefined"!=typeof MSApp&&MSApp;var S={animationIterationCount:!0,borderImageOutset:!0,borderImageSlice:!0,borderImageWidth:!0,boxFlex:!0,boxFlexGroup:!0,boxOrdinalGroup:!0,columnCount:!0,columns:!0,flex:!0,flexGrow:!0,flexPositive:!0,flexShrink:!0,flexNegative:!0,flexOrder:!0,gridArea:!0,gridRow:!0,gridRowEnd:!0,gridRowSpan:!0,gridRowStart:!0,gridColumn:!0,gridColumnEnd:!0,gridColumnSpan:!0,gridColumnStart:!0,fontWeight:!0,lineClamp:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,tabSize:!0,widows:!0,zIndex:!0,zoom:!0,fillOpacity:!0,floodOpacity:!0,stopOpacity:!0,strokeDasharray:!0,strokeDashoffset:!0,strokeMiterlimit:!0,strokeOpacity:!0,strokeWidth:!0},w=["Webkit","ms","Moz","O"];if(Object.keys(S).forEach(function(e){w.forEach(function(t){t=t+e.charAt(0).toUpperCase()+e.substring(1),S[t]=S[e]})}),l({menuitem:!0},{area:!0,base:!0,br:!0,col:!0,embed:!0,hr:!0,img:!0,input:!0,keygen:!0,link:!0,meta:!0,param:!0,source:!0,track:!0,wbr:!0}),g)try{var N={};Object.defineProperty(N,"passive",{get:function(){}}),window.addEventListener("test",N,N),window.removeEventListener("test",N,N)}catch(e){}function R(e){var t=e,n=e;if(e.alternate)for(;t.return;)t=t.return;else{e=t;do{0!=(1026&(t=e).flags)&&(n=t.return),e=t.return}while(e)}return 3===t.tag?n:null}function O(e){if(R(e)!==e)throw Error(d(188))}function C(e,t){var n={};return n[e.toLowerCase()]=t.toLowerCase(),n["Webkit"+e]="webkit"+t,n["Moz"+e]="moz"+t,n}var x={animationend:C("Animation","AnimationEnd"),animationiteration:C("Animation","AnimationIteration"),animationstart:C("Animation","AnimationStart"),transitionend:C("Transition","TransitionEnd")},k={},A={};function I(e){if(k[e])return k[e];if(!x[e])return e;var t,n=x[e];for(t in n)if(n.hasOwnProperty(t)&&t in A)return k[e]=n[t];return e}g&&(A=document.createElement("div").style,"AnimationEvent"in window||(delete x.animationend.animation,delete x.animationiteration.animation,delete x.animationstart.animation),"TransitionEvent"in window||delete x.transitionend.transition);var L=I("animationend"),_=I("animationiteration"),P=I("animationstart"),M=I("transitionend"),D=new Map,U=new Map,j=["abort","abort",L,"animationEnd",_,"animationIteration",P,"animationStart","canplay","canPlay","canplaythrough","canPlayThrough","durationchange","durationChange","emptied","emptied","encrypted","encrypted","ended","ended","error","error","gotpointercapture","gotPointerCapture","load","load","loadeddata","loadedData","loadedmetadata","loadedMetadata","loadstart","loadStart","lostpointercapture","lostPointerCapture","playing","playing","progress","progress","seeking","seeking","stalled","stalled","suspend","suspend","timeupdate","timeUpdate",M,"transitionEnd","waiting","waiting"];function F(e,t){for(var n=0;n<e.length;n+=2){var r=e[n],a=e[n+1];a="on"+(a[0].toUpperCase()+a.slice(1)),U.set(r,t),D.set(r,a),m(a,[r])}}function z(e){var t=e.keyCode;return"charCode"in e?0===(e=e.charCode)&&13===t&&(e=13):e=t,10===e&&(e=13),32<=e||13===e?e:0}function B(){return!0}function H(){return!1}function V(e){function t(t,n,r,a,o){for(var i in this._reactName=t,this._targetInst=r,this.type=n,this.nativeEvent=a,this.target=o,this.currentTarget=null,e)e.hasOwnProperty(i)&&(this[i]=(t=e[i])?t(a):a[i]);return this.isDefaultPrevented=(null!=a.defaultPrevented?a.defaultPrevented:!1===a.returnValue)?B:H,this.isPropagationStopped=H,this}return l(t.prototype,{preventDefault:function(){this.defaultPrevented=!0;var e=this.nativeEvent;e&&(e.preventDefault?e.preventDefault():"unknown"!=typeof e.returnValue&&(e.returnValue=!1),this.isDefaultPrevented=B)},stopPropagation:function(){var e=this.nativeEvent;e&&(e.stopPropagation?e.stopPropagation():"unknown"!=typeof e.cancelBubble&&(e.cancelBubble=!0),this.isPropagationStopped=B)},persist:function(){},isPersistent:B}),t}(0,f.unstable_now)();var W={eventPhase:0,bubbles:0,cancelable:0,timeStamp:function(e){return e.timeStamp||Date.now()},defaultPrevented:0,isTrusted:0};V(W);var G=l({},W,{view:0,detail:0});V(G);var Y,$,X,q=l({},G,{screenX:0,screenY:0,clientX:0,clientY:0,pageX:0,pageY:0,ctrlKey:0,shiftKey:0,altKey:0,metaKey:0,getModifierState:ee,button:0,buttons:0,relatedTarget:function(e){return void 0===e.relatedTarget?e.fromElement===e.srcElement?e.toElement:e.fromElement:e.relatedTarget},movementX:function(e){return"movementX"in e?e.movementX:(e!==X&&(X&&"mousemove"===e.type?(Y=e.screenX-X.screenX,$=e.screenY-X.screenY):$=Y=0,X=e),Y)},movementY:function(e){return"movementY"in e?e.movementY:$}});V(q),V(l({},q,{dataTransfer:0})),V(l({},G,{relatedTarget:0})),V(l({},W,{animationName:0,elapsedTime:0,pseudoElement:0})),V(l({},W,{clipboardData:function(e){return"clipboardData"in e?e.clipboardData:window.clipboardData}})),V(l({},W,{data:0}));var K={Esc:"Escape",Spacebar:" ",Left:"ArrowLeft",Up:"ArrowUp",Right:"ArrowRight",Down:"ArrowDown",Del:"Delete",Win:"OS",Menu:"ContextMenu",Apps:"ContextMenu",Scroll:"ScrollLock",MozPrintableKey:"Unidentified"},J={8:"Backspace",9:"Tab",12:"Clear",13:"Enter",16:"Shift",17:"Control",18:"Alt",19:"Pause",20:"CapsLock",27:"Escape",32:" ",33:"PageUp",34:"PageDown",35:"End",36:"Home",37:"ArrowLeft",38:"ArrowUp",39:"ArrowRight",40:"ArrowDown",45:"Insert",46:"Delete",112:"F1",113:"F2",114:"F3",115:"F4",116:"F5",117:"F6",118:"F7",119:"F8",120:"F9",121:"F10",122:"F11",123:"F12",144:"NumLock",145:"ScrollLock",224:"Meta"},Q={Alt:"altKey",Control:"ctrlKey",Meta:"metaKey",Shift:"shiftKey"};function Z(e){var t=this.nativeEvent;return t.getModifierState?t.getModifierState(e):!!(e=Q[e])&&!!t[e]}function ee(){return Z}if(V(l({},G,{key:function(e){if(e.key){var t=K[e.key]||e.key;if("Unidentified"!==t)return t}return"keypress"===e.type?13===(e=z(e))?"Enter":String.fromCharCode(e):"keydown"===e.type||"keyup"===e.type?J[e.keyCode]||"Unidentified":""},code:0,location:0,ctrlKey:0,shiftKey:0,altKey:0,metaKey:0,repeat:0,locale:0,getModifierState:ee,charCode:function(e){return"keypress"===e.type?z(e):0},keyCode:function(e){return"keydown"===e.type||"keyup"===e.type?e.keyCode:0},which:function(e){return"keypress"===e.type?z(e):"keydown"===e.type||"keyup"===e.type?e.keyCode:0}})),V(l({},q,{pointerId:0,width:0,height:0,pressure:0,tangentialPressure:0,tiltX:0,tiltY:0,twist:0,pointerType:0,isPrimary:0})),V(l({},G,{touches:0,targetTouches:0,changedTouches:0,altKey:0,metaKey:0,ctrlKey:0,shiftKey:0,getModifierState:ee})),V(l({},W,{propertyName:0,elapsedTime:0,pseudoElement:0})),V(l({},q,{deltaX:function(e){return"deltaX"in e?e.deltaX:"wheelDeltaX"in e?-e.wheelDeltaX:0},deltaY:function(e){return"deltaY"in e?e.deltaY:"wheelDeltaY"in e?-e.wheelDeltaY:"wheelDelta"in e?-e.wheelDelta:0},deltaZ:0,deltaMode:0})),g&&g){var te="oninput"in document;if(!te){var ne=document.createElement("div");ne.setAttribute("oninput","return;"),te="function"==typeof ne.oninput}}F("cancel cancel click click close close contextmenu contextMenu copy copy cut cut auxclick auxClick dblclick doubleClick dragend dragEnd dragstart dragStart drop drop focusin focus focusout blur input input invalid invalid keydown keyDown keypress keyPress keyup keyUp mousedown mouseDown mouseup mouseUp paste paste pause pause play play pointercancel pointerCancel pointerdown pointerDown pointerup pointerUp ratechange rateChange reset reset seeked seeked submit submit touchcancel touchCancel touchend touchEnd touchstart touchStart volumechange volumeChange".split(" "),0),F("drag drag dragenter dragEnter dragexit dragExit dragleave dragLeave dragover dragOver mousemove mouseMove mouseout mouseOut mouseover mouseOver pointermove pointerMove pointerout pointerOut pointerover pointerOver scroll scroll toggle toggle touchmove touchMove wheel wheel".split(" "),1),F(j,2);for(var re="change selectionchange textInput compositionstart compositionend compositionupdate".split(" "),ae=0;ae<re.length;ae++)U.set(re[ae],0);function oe(e){e=e.previousSibling;for(var t=0;e;){if(8===e.nodeType){var n=e.data;if("$"===n||"$!"===n||"$?"===n){if(0===t)return e;t--}else"/$"===n&&t++}e=e.previousSibling}return null}h("onMouseEnter",["mouseout","mouseover"]),h("onMouseLeave",["mouseout","mouseover"]),h("onPointerEnter",["pointerout","pointerover"]),h("onPointerLeave",["pointerout","pointerover"]),m("onChange","change click focusin focusout input keydown keyup selectionchange".split(" ")),m("onSelect","focusout contextmenu dragend focusin keydown keyup mousedown mouseup selectionchange".split(" ")),m("onBeforeInput",["compositionend","keypress","textInput","paste"]),m("onCompositionEnd","compositionend focusout keydown keypress keyup mousedown".split(" ")),m("onCompositionStart","compositionstart focusout keydown keypress keyup mousedown".split(" ")),m("onCompositionUpdate","compositionupdate focusout keydown keypress keyup mousedown".split(" ")),Math.random().toString(36).slice(2);var ie=Math.random().toString(36).slice(2),le="__reactFiber$"+ie,se="__reactContainer$"+ie;(0,f.unstable_now)(),new c.Component;var ce={findFiberByHostInstance:function(e){var t=e[le];if(t)return t;for(var n=e.parentNode;n;){if(t=n[se]||n[le]){if(n=t.alternate,null!==t.child||null!==n&&null!==n.child)for(e=oe(e);null!==e;){if(n=e[le])return n;e=oe(e)}return t}n=(e=n).parentNode}return null},bundleType:0,version:"17.0.2",rendererPackageName:"react-dom"},ue={bundleType:ce.bundleType,version:ce.version,rendererPackageName:ce.rendererPackageName,rendererConfig:ce.rendererConfig,overrideHookState:null,overrideHookStateDeletePath:null,overrideHookStateRenamePath:null,overrideProps:null,overridePropsDeletePath:null,overridePropsRenamePath:null,setSuspenseHandler:null,scheduleUpdate:null,currentDispatcherRef:E.ReactCurrentDispatcher,findHostInstanceByFiber:function(e){return null===(e=function(e){if(!(e=function(e){var t=e.alternate;if(!t){if(null===(t=R(e)))throw Error(d(188));return t!==e?null:e}for(var n=e,r=t;;){var a=n.return;if(null===a)break;var o=a.alternate;if(null===o){if(null!==(r=a.return)){n=r;continue}break}if(a.child===o.child){for(o=a.child;o;){if(o===n)return O(a),e;if(o===r)return O(a),t;o=o.sibling}throw Error(d(188))}if(n.return!==r.return)n=a,r=o;else{for(var i=!1,l=a.child;l;){if(l===n){i=!0,n=a,r=o;break}if(l===r){i=!0,r=a,n=o;break}l=l.sibling}if(!i){for(l=o.child;l;){if(l===n){i=!0,n=o,r=a;break}if(l===r){i=!0,r=o,n=a;break}l=l.sibling}if(!i)throw Error(d(189))}}if(n.alternate!==r)throw Error(d(190))}if(3!==n.tag)throw Error(d(188));return n.stateNode.current===n?e:t}(e)))return null;for(var t=e;;){if(5===t.tag||6===t.tag)return t;if(t.child)t.child.return=t,t=t.child;else{if(t===e)break;for(;!t.sibling;){if(!t.return||t.return===e)return null;t=t.return}t.sibling.return=t.return,t=t.sibling}}return null}(e))?null:e.stateNode},findFiberByHostInstance:ce.findFiberByHostInstance||function(){return null},findHostInstancesForRefresh:null,scheduleRefresh:null,scheduleRoot:null,setRefreshHandler:null,getCurrentFiber:null};if("undefined"!=typeof __REACT_DEVTOOLS_GLOBAL_HOOK__){var fe=__REACT_DEVTOOLS_GLOBAL_HOOK__;if(!fe.isDisabled&&fe.supportsFiber)try{fe.inject(ue)}catch(e){}}var de=n(function(e,t){!function(){var e=0,n=0;t.__interactionsRef=null,t.__subscriberRef=null,t.__interactionsRef={current:new Set},t.__subscriberRef={current:null};var r=null;function a(e){var t=!1,n=null;if(r.forEach(function(r){try{r.onInteractionTraced(e)}catch(e){t||(t=!0,n=e)}}),t)throw n}function o(e){var t=!1,n=null;if(r.forEach(function(r){try{r.onInteractionScheduledWorkCompleted(e)}catch(e){t||(t=!0,n=e)}}),t)throw n}function i(e,t){var n=!1,a=null;if(r.forEach(function(r){try{r.onWorkScheduled(e,t)}catch(e){n||(n=!0,a=e)}}),n)throw a}function l(e,t){var n=!1,a=null;if(r.forEach(function(r){try{r.onWorkStarted(e,t)}catch(e){n||(n=!0,a=e)}}),n)throw a}function s(e,t){var n=!1,a=null;if(r.forEach(function(r){try{r.onWorkStopped(e,t)}catch(e){n||(n=!0,a=e)}}),n)throw a}function c(e,t){var n=!1,a=null;if(r.forEach(function(r){try{r.onWorkCanceled(e,t)}catch(e){n||(n=!0,a=e)}}),n)throw a}r=new Set,t.unstable_clear=function(e){var n=t.__interactionsRef.current;t.__interactionsRef.current=new Set;try{return e()}finally{t.__interactionsRef.current=n}},t.unstable_getCurrent=function(){return t.__interactionsRef.current},t.unstable_getThreadID=function(){return++n},t.unstable_subscribe=function(e){r.add(e),1===r.size&&(t.__subscriberRef.current={onInteractionScheduledWorkCompleted:o,onInteractionTraced:a,onWorkCanceled:c,onWorkScheduled:i,onWorkStarted:l,onWorkStopped:s})},t.unstable_trace=function(n,r,a){var o=arguments.length>3&&void 0!==arguments[3]?arguments[3]:0,i={__count:1,id:e++,name:n,timestamp:r},l=t.__interactionsRef.current,s=new Set(l);s.add(i),t.__interactionsRef.current=s;var c,u=t.__subscriberRef.current;try{null!==u&&u.onInteractionTraced(i)}finally{try{null!==u&&u.onWorkStarted(s,o)}finally{try{c=a()}finally{t.__interactionsRef.current=l;try{null!==u&&u.onWorkStopped(s,o)}finally{i.__count--,null!==u&&0===i.__count&&u.onInteractionScheduledWorkCompleted(i)}}}}return c},t.unstable_unsubscribe=function(e){r.delete(e),0===r.size&&(t.__subscriberRef.current=null)},t.unstable_wrap=function(e){var n=arguments.length>1&&void 0!==arguments[1]?arguments[1]:0,r=t.__interactionsRef.current,a=t.__subscriberRef.current;null!==a&&a.onWorkScheduled(r,n),r.forEach(function(e){e.__count++});var o=!1;function i(){var i=t.__interactionsRef.current;t.__interactionsRef.current=r,a=t.__subscriberRef.current;try{var l;try{null!==a&&a.onWorkStarted(r,n)}finally{try{l=e.apply(void 0,arguments)}finally{t.__interactionsRef.current=i,null!==a&&a.onWorkStopped(r,n)}}return l}finally{o||(o=!0,r.forEach(function(e){e.__count--,null!==a&&0===e.__count&&a.onInteractionScheduledWorkCompleted(e)}))}}return i.cancel=function(){a=t.__subscriberRef.current;try{null!==a&&a.onWorkCanceled(r,n)}finally{r.forEach(function(e){e.__count--,a&&0===e.__count&&a.onInteractionScheduledWorkCompleted(e)})}},i}}()}),pe=n(function(e){e.exports=de}),me=n(function(e,t){!function(){var e=c,n=l,r=f,a=pe,o=e.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED;function i(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];u("warn",e,n)}function s(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];u("error",e,n)}function u(e,t,n){var r=o.ReactDebugCurrentFrame.getStackAddendum();""!==r&&(t+="%s",n=n.concat([r]));var a=n.map(function(e){return""+e});a.unshift("Warning: "+t),Function.prototype.apply.call(console[e],console,a)}if(!e)throw Error("ReactDOM was loaded before React. Make sure you load the React package before loading ReactDOM.");var d=10,p=11,m=12,h=13,g=14,y=15,v=17,b=19,E=20,T=22,S=23,w=24,N=!1,R=new Set,O={},C={};function x(e,t){k(e,t),k(e+"Capture",t)}function k(e,t){O[e]&&s("EventRegistry: More than one plugin attempted to publish the same registration name, `%s`.",e),O[e]=t;var n=e.toLowerCase();C[n]=e,"onDoubleClick"===e&&(C.ondblclick=e);for(var r=0;r<t.length;r++)R.add(t[r])}var A=!("undefined"==typeof window||void 0===window.document||void 0===window.document.createElement),I=":A-Z_a-z\\u00C0-\\u00D6\\u00D8-\\u00F6\\u00F8-\\u02FF\\u0370-\\u037D\\u037F-\\u1FFF\\u200C-\\u200D\\u2070-\\u218F\\u2C00-\\u2FEF\\u3001-\\uD7FF\\uF900-\\uFDCF\\uFDF0-\\uFFFD",L=I+"\\-.0-9\\u00B7\\u0300-\\u036F\\u203F-\\u2040",_="data-reactroot",P=new RegExp("^["+I+"]["+L+"]*$"),M=Object.prototype.hasOwnProperty,D={},U={};function j(e){return!!M.call(U,e)||!M.call(D,e)&&(P.test(e)?(U[e]=!0,!0):(D[e]=!0,s("Invalid attribute name: `%s`",e),!1))}function F(e,t,n){return null!==t?0===t.type:!n&&e.length>2&&("o"===e[0]||"O"===e[0])&&("n"===e[1]||"N"===e[1])}function z(e,t,n,r){if(null!==n&&0===n.type)return!1;switch(typeof t){case"function":case"symbol":return!0;case"boolean":if(r)return!1;if(null!==n)return!n.acceptsBooleans;var a=e.toLowerCase().slice(0,5);return"data-"!==a&&"aria-"!==a;default:return!1}}function B(e,t,n,r){if(null==t)return!0;if(z(e,t,n,r))return!0;if(r)return!1;if(null!==n)switch(n.type){case 3:return!t;case 4:return!1===t;case 5:return isNaN(t);case 6:return isNaN(t)||t<1}return!1}function H(e){return W.hasOwnProperty(e)?W[e]:null}function V(e,t,n,r,a,o,i){this.acceptsBooleans=2===t||3===t||4===t,this.attributeName=r,this.attributeNamespace=a,this.mustUseProperty=n,this.propertyName=e,this.type=t,this.sanitizeURL=o,this.removeEmptyString=i}var W={};["children","dangerouslySetInnerHTML","defaultValue","defaultChecked","innerHTML","suppressContentEditableWarning","suppressHydrationWarning","style"].forEach(function(e){W[e]=new V(e,0,!1,e,null,!1,!1)}),[["acceptCharset","accept-charset"],["className","class"],["htmlFor","for"],["httpEquiv","http-equiv"]].forEach(function(e){var t=e[0];W[t]=new V(t,1,!1,e[1],null,!1,!1)}),["contentEditable","draggable","spellCheck","value"].forEach(function(e){W[e]=new V(e,2,!1,e.toLowerCase(),null,!1,!1)}),["autoReverse","externalResourcesRequired","focusable","preserveAlpha"].forEach(function(e){W[e]=new V(e,2,!1,e,null,!1,!1)}),["allowFullScreen","async","autoFocus","autoPlay","controls","default","defer","disabled","disablePictureInPicture","disableRemotePlayback","formNoValidate","hidden","loop","noModule","noValidate","open","playsInline","readOnly","required","reversed","scoped","seamless","itemScope"].forEach(function(e){W[e]=new V(e,3,!1,e.toLowerCase(),null,!1,!1)}),["checked","multiple","muted","selected"].forEach(function(e){W[e]=new V(e,3,!0,e,null,!1,!1)}),["capture","download"].forEach(function(e){W[e]=new V(e,4,!1,e,null,!1,!1)}),["cols","rows","size","span"].forEach(function(e){W[e]=new V(e,6,!1,e,null,!1,!1)}),["rowSpan","start"].forEach(function(e){W[e]=new V(e,5,!1,e.toLowerCase(),null,!1,!1)});var G=/[\-\:]([a-z])/g,Y=function(e){return e[1].toUpperCase()};["accent-height","alignment-baseline","arabic-form","baseline-shift","cap-height","clip-path","clip-rule","color-interpolation","color-interpolation-filters","color-profile","color-rendering","dominant-baseline","enable-background","fill-opacity","fill-rule","flood-color","flood-opacity","font-family","font-size","font-size-adjust","font-stretch","font-style","font-variant","font-weight","glyph-name","glyph-orientation-horizontal","glyph-orientation-vertical","horiz-adv-x","horiz-origin-x","image-rendering","letter-spacing","lighting-color","marker-end","marker-mid","marker-start","overline-position","overline-thickness","paint-order","panose-1","pointer-events","rendering-intent","shape-rendering","stop-color","stop-opacity","strikethrough-position","strikethrough-thickness","stroke-dasharray","stroke-dashoffset","stroke-linecap","stroke-linejoin","stroke-miterlimit","stroke-opacity","stroke-width","text-anchor","text-decoration","text-rendering","underline-position","underline-thickness","unicode-bidi","unicode-range","units-per-em","v-alphabetic","v-hanging","v-ideographic","v-mathematical","vector-effect","vert-adv-y","vert-origin-x","vert-origin-y","word-spacing","writing-mode","xmlns:xlink","x-height"].forEach(function(e){var t=e.replace(G,Y);W[t]=new V(t,1,!1,e,null,!1,!1)}),["xlink:actuate","xlink:arcrole","xlink:role","xlink:show","xlink:title","xlink:type"].forEach(function(e){var t=e.replace(G,Y);W[t]=new V(t,1,!1,e,"http://www.w3.org/1999/xlink",!1,!1)}),["xml:base","xml:lang","xml:space"].forEach(function(e){var t=e.replace(G,Y);W[t]=new V(t,1,!1,e,"http://www.w3.org/XML/1998/namespace",!1,!1)}),["tabIndex","crossOrigin"].forEach(function(e){W[e]=new V(e,1,!1,e.toLowerCase(),null,!1,!1)}),W.xlinkHref=new V("xlinkHref",1,!1,"xlink:href","http://www.w3.org/1999/xlink",!0,!1),["src","href","action","formAction"].forEach(function(e){W[e]=new V(e,1,!1,e.toLowerCase(),null,!0,!0)});var $=/^[\u0000-\u001F ]*j[\r\n\t]*a[\r\n\t]*v[\r\n\t]*a[\r\n\t]*s[\r\n\t]*c[\r\n\t]*r[\r\n\t]*i[\r\n\t]*p[\r\n\t]*t[\r\n\t]*\:/i,X=!1;function q(e){!X&&$.test(e)&&(X=!0,s("A future version of React will block javascript: URLs as a security precaution. Use event handlers instead if you can. If you need to generate unsafe HTML try using dangerouslySetInnerHTML instead. React was passed %s.",JSON.stringify(e)))}function K(e,t,n,r){if(r.mustUseProperty)return e[r.propertyName];r.sanitizeURL&&q(""+n);var a=r.attributeName,o=null;if(4===r.type){if(e.hasAttribute(a)){var i=e.getAttribute(a);return""===i||(B(t,n,r,!1)?i:i===""+n?n:i)}}else if(e.hasAttribute(a)){if(B(t,n,r,!1))return e.getAttribute(a);if(3===r.type)return n;o=e.getAttribute(a)}return B(t,n,r,!1)?null===o?n:o:o===""+n?n:o}function J(e,t,n){if(j(t)){if(function(e){return null!==e&&"object"==typeof e&&e.$$typeof===me}(n))return n;if(!e.hasAttribute(t))return void 0===n?void 0:null;var r=e.getAttribute(t);return r===""+n?n:r}}function Q(e,t,n,r){var a=H(t);if(!F(t,a,r))if(B(t,n,a,r)&&(n=null),r||null===a){if(j(t)){var o=t;null===n?e.removeAttribute(o):e.setAttribute(o,""+n)}}else if(a.mustUseProperty)e[a.propertyName]=null===n?3!==a.type&&"":n;else{var i=a.attributeName,l=a.attributeNamespace;if(null===n)e.removeAttribute(i);else{var s,c=a.type;3===c||4===c&&!0===n?s="":(s=""+n,a.sanitizeURL&&q(s.toString())),l?e.setAttributeNS(l,i,s):e.setAttribute(i,s)}}}var Z=60103,ee=60106,te=60107,ne=60108,re=60114,ae=60109,oe=60110,ie=60112,le=60113,se=60120,ce=60115,ue=60116,fe=60121,de=60119,me=60128,he=60129,ge=60130,ye=60131;if("function"==typeof Symbol&&Symbol.for){var ve=Symbol.for;Z=ve("react.element"),ee=ve("react.portal"),te=ve("react.fragment"),ne=ve("react.strict_mode"),re=ve("react.profiler"),ae=ve("react.provider"),oe=ve("react.context"),ie=ve("react.forward_ref"),le=ve("react.suspense"),se=ve("react.suspense_list"),ce=ve("react.memo"),ue=ve("react.lazy"),fe=ve("react.block"),ve("react.server.block"),ve("react.fundamental"),de=ve("react.scope"),me=ve("react.opaque.id"),he=ve("react.debug_trace_mode"),ge=ve("react.offscreen"),ye=ve("react.legacy_hidden")}var be="function"==typeof Symbol&&Symbol.iterator;function Ee(e){if(null===e||"object"!=typeof e)return null;var t=be&&e[be]||e["@@iterator"];return"function"==typeof t?t:null}var Te,Se,we,Ne,Re,Oe,Ce,xe=0;function ke(){}function Ae(){if(0===xe){Te=console.log,Se=console.info,we=console.warn,Ne=console.error,Re=console.group,Oe=console.groupCollapsed,Ce=console.groupEnd;var e={configurable:!0,enumerable:!0,value:ke,writable:!0};Object.defineProperties(console,{info:e,log:e,warn:e,error:e,group:e,groupCollapsed:e,groupEnd:e})}xe++}function Ie(){if(0==--xe){var e={configurable:!0,enumerable:!0,writable:!0};Object.defineProperties(console,{log:n({},e,{value:Te}),info:n({},e,{value:Se}),warn:n({},e,{value:we}),error:n({},e,{value:Ne}),group:n({},e,{value:Re}),groupCollapsed:n({},e,{value:Oe}),groupEnd:n({},e,{value:Ce})})}xe<0&&s("disabledDepth fell below zero. This is a bug in React. Please file an issue.")}ke.__reactDisabledLog=!0;var Le,_e=o.ReactCurrentDispatcher;function Pe(e,t,n){if(void 0===Le)try{throw Error()}catch(e){var r=e.stack.trim().match(/\n( *(at )?)/);Le=r&&r[1]||""}return"\n"+Le+e}var Me,De=!1,Ue="function"==typeof WeakMap?WeakMap:Map;function je(e,t){if(!e||De)return"";var n,r=Me.get(e);if(void 0!==r)return r;De=!0;var a,o=Error.prepareStackTrace;Error.prepareStackTrace=void 0,a=_e.current,_e.current=null,Ae();try{if(t){var i=function(){throw Error()};if(Object.defineProperty(i.prototype,"props",{set:function(){throw Error()}}),"object"==typeof Reflect&&Reflect.construct){try{Reflect.construct(i,[])}catch(e){n=e}Reflect.construct(e,[],i)}else{try{i.call()}catch(e){n=e}e.call(i.prototype)}}else{try{throw Error()}catch(e){n=e}e()}}catch(t){if(t&&n&&"string"==typeof t.stack){for(var l=t.stack.split("\n"),s=n.stack.split("\n"),c=l.length-1,u=s.length-1;c>=1&&u>=0&&l[c]!==s[u];)u--;for(;c>=1&&u>=0;c--,u--)if(l[c]!==s[u]){if(1!==c||1!==u)do{if(c--,--u<0||l[c]!==s[u]){var f="\n"+l[c].replace(" at new "," at ");return"function"==typeof e&&Me.set(e,f),f}}while(c>=1&&u>=0);break}}}finally{De=!1,_e.current=a,Ie(),Error.prepareStackTrace=o}var d=e?e.displayName||e.name:"",p=d?Pe(d):"";return"function"==typeof e&&Me.set(e,p),p}function Fe(e,t,n){return je(e,!1)}function ze(e,t,n){if(null==e)return"";if("function"==typeof e)return je(e,!(!(r=e.prototype)||!r.isReactComponent));var r;if("string"==typeof e)return Pe(e);switch(e){case le:return Pe("Suspense");case se:return Pe("SuspenseList")}if("object"==typeof e)switch(e.$$typeof){case ie:return Fe(e.render);case ce:return ze(e.type,t,n);case fe:return Fe(e._render);case ue:var a=e._payload,o=e._init;try{return ze(o(a),t,n)}catch(e){}}return""}function Be(e){switch(e.tag){case 5:return Pe(e.type);case 16:return Pe("Lazy");case h:return Pe("Suspense");case b:return Pe("SuspenseList");case 0:case 2:case y:return Fe(e.type);case p:return Fe(e.type.render);case T:return Fe(e.type._render);case 1:return je(e.type,!0);default:return""}}function He(e){try{var t="",n=e;do{t+=Be(n),n=n.return}while(n);return t}catch(e){return"\nError generating stack: "+e.message+"\n"+e.stack}}function Ve(e){return e.displayName||"Context"}function We(e){if(null==e)return null;if("number"==typeof e.tag&&s("Received an unexpected object in getComponentName(). This is likely a bug in React. Please file an issue."),"function"==typeof e)return e.displayName||e.name||null;if("string"==typeof e)return e;switch(e){case te:return"Fragment";case ee:return"Portal";case re:return"Profiler";case ne:return"StrictMode";case le:return"Suspense";case se:return"SuspenseList"}if("object"==typeof e)switch(e.$$typeof){case oe:return Ve(e)+".Consumer";case ae:return Ve(e._context)+".Provider";case ie:return a=(r=e.render).displayName||r.name||"",e.displayName||(""!==a?"ForwardRef("+a+")":"ForwardRef");case ce:return We(e.type);case fe:return We(e._render);case ue:var t=e._payload,n=e._init;try{return We(n(t))}catch(e){return null}}var r,a;return null}Me=new Ue;var Ge=o.ReactDebugCurrentFrame,Ye=null,$e=!1;function Xe(){if(null===Ye)return null;var e=Ye._debugOwner;return null!=e?We(e.type):null}function qe(){return null===Ye?"":He(Ye)}function Ke(){Ge.getCurrentStack=null,Ye=null,$e=!1}function Je(e){Ge.getCurrentStack=qe,Ye=e,$e=!1}function Qe(e){$e=e}function Ze(e){return""+e}function et(e){switch(typeof e){case"boolean":case"number":case"object":case"string":case"undefined":return e;default:return""}}var tt={button:!0,checkbox:!0,image:!0,hidden:!0,radio:!0,reset:!0,submit:!0};function nt(e,t){tt[t.type]||t.onChange||t.onInput||t.readOnly||t.disabled||null==t.value||s("You provided a `value` prop to a form field without an `onChange` handler. This will render a read-only field. If the field should be mutable use `defaultValue`. Otherwise, set either `onChange` or `readOnly`."),t.onChange||t.readOnly||t.disabled||null==t.checked||s("You provided a `checked` prop to a form field without an `onChange` handler. This will render a read-only field. If the field should be mutable use `defaultChecked`. Otherwise, set either `onChange` or `readOnly`.")}function rt(e){var t=e.type,n=e.nodeName;return n&&"input"===n.toLowerCase()&&("checkbox"===t||"radio"===t)}function at(e){return e._valueTracker}function ot(e){at(e)||(e._valueTracker=function(e){var t=rt(e)?"checked":"value",n=Object.getOwnPropertyDescriptor(e.constructor.prototype,t),r=""+e[t];if(!e.hasOwnProperty(t)&&void 0!==n&&"function"==typeof n.get&&"function"==typeof n.set){var a=n.get,o=n.set;return Object.defineProperty(e,t,{configurable:!0,get:function(){return a.call(this)},set:function(e){r=""+e,o.call(this,e)}}),Object.defineProperty(e,t,{enumerable:n.enumerable}),{getValue:function(){return r},setValue:function(e){r=""+e},stopTracking:function(){!function(e){e._valueTracker=null}(e),delete e[t]}}}}(e))}function it(e){if(!e)return!1;var t=at(e);if(!t)return!0;var n=t.getValue(),r=function(e){var t="";return e?t=rt(e)?e.checked?"true":"false":e.value:t}(e);return r!==n&&(t.setValue(r),!0)}function lt(e){if(void 0===(e=e||("undefined"!=typeof document?document:void 0)))return null;try{return e.activeElement||e.body}catch(t){return e.body}}var st=!1,ct=!1,ut=!1,ft=!1;function dt(e){return"checkbox"===e.type||"radio"===e.type?null!=e.checked:null!=e.value}function pt(e,t){var r=t.checked;return n({},t,{defaultChecked:void 0,defaultValue:void 0,value:void 0,checked:null!=r?r:e._wrapperState.initialChecked})}function mt(e,t){nt(0,t),void 0===t.checked||void 0===t.defaultChecked||ct||(s("%s contains an input of type %s with both checked and defaultChecked props. Input elements must be either controlled or uncontrolled (specify either the checked prop, or the defaultChecked prop, but not both). Decide between using a controlled or uncontrolled input element and remove one of these props. More info: https://reactjs.org/link/controlled-components",Xe()||"A component",t.type),ct=!0),void 0===t.value||void 0===t.defaultValue||st||(s("%s contains an input of type %s with both value and defaultValue props. Input elements must be either controlled or uncontrolled (specify either the value prop, or the defaultValue prop, but not both). Decide between using a controlled or uncontrolled input element and remove one of these props. More info: https://reactjs.org/link/controlled-components",Xe()||"A component",t.type),st=!0),e._wrapperState={initialChecked:null!=t.checked?t.checked:t.defaultChecked,initialValue:et(null!=t.value?t.value:null==t.defaultValue?"":t.defaultValue),controlled:dt(t)}}function ht(e,t){var n=t.checked;null!=n&&Q(e,"checked",n,!1)}function gt(e,t){var n=e,r=dt(t);n._wrapperState.controlled||!r||ft||(s("A component is changing an uncontrolled input to be controlled. This is likely caused by the value changing from undefined to a defined value, which should not happen. Decide between using a controlled or uncontrolled input element for the lifetime of the component. More info: https://reactjs.org/link/controlled-components"),ft=!0),!n._wrapperState.controlled||r||ut||(s("A component is changing a controlled input to be uncontrolled. This is likely caused by the value changing from a defined to undefined, which should not happen. Decide between using a controlled or uncontrolled input element for the lifetime of the component. More info: https://reactjs.org/link/controlled-components"),ut=!0),ht(e,t);var a=et(t.value),o=t.type;if(null!=a)"number"===o?(0===a&&""===n.value||n.value!=a)&&(n.value=Ze(a)):n.value!==Ze(a)&&(n.value=Ze(a));else if("submit"===o||"reset"===o)return void n.removeAttribute("value");t.hasOwnProperty("value")?vt(n,t.type,a):t.hasOwnProperty("defaultValue")&&vt(n,t.type,et(t.defaultValue)),null==t.checked&&null!=t.defaultChecked&&(n.defaultChecked=!!t.defaultChecked)}function yt(e,t,n){var r=e;if(t.hasOwnProperty("value")||t.hasOwnProperty("defaultValue")){var a=t.type;if(("submit"===a||"reset"===a)&&null==t.value)return;var o=Ze(r._wrapperState.initialValue);n||o!==r.value&&(r.value=o),r.defaultValue=o}var i=r.name;""!==i&&(r.name=""),r.defaultChecked=!r.defaultChecked,r.defaultChecked=!!r._wrapperState.initialChecked,""!==i&&(r.name=i)}function vt(e,t,n){"number"===t&<(e.ownerDocument)===e||(null==n?e.defaultValue=Ze(e._wrapperState.initialValue):e.defaultValue!==Ze(n)&&(e.defaultValue=Ze(n)))}var bt,Et=!1,Tt=!1;function St(t,n){"object"==typeof n.children&&null!==n.children&&e.Children.forEach(n.children,function(e){null!=e&&"string"!=typeof e&&"number"!=typeof e&&"string"==typeof e.type&&(Tt||(Tt=!0,s("Only strings and numbers are supported as <option> children.")))}),null==n.selected||Et||(s("Use the `defaultValue` or `value` props on <select> instead of setting `selected` on <option>."),Et=!0)}function wt(t,r){var a=n({children:void 0},r),o=function(t){var n="";return e.Children.forEach(t,function(e){null!=e&&(n+=e)}),n}(r.children);return o&&(a.children=o),a}function Nt(){var e=Xe();return e?"\n\nCheck the render method of `"+e+"`.":""}bt=!1;var Rt=["value","defaultValue"];function Ot(e,t,n,r){var a=e.options;if(t){for(var o=n,i={},l=0;l<o.length;l++)i["$"+o[l]]=!0;for(var s=0;s<a.length;s++){var c=i.hasOwnProperty("$"+a[s].value);a[s].selected!==c&&(a[s].selected=c),c&&r&&(a[s].defaultSelected=!0)}}else{for(var u=Ze(et(n)),f=null,d=0;d<a.length;d++){if(a[d].value===u)return a[d].selected=!0,void(r&&(a[d].defaultSelected=!0));null!==f||a[d].disabled||(f=a[d])}null!==f&&(f.selected=!0)}}function Ct(e,t){return n({},t,{value:void 0})}function xt(e,t){var n=e;!function(e){nt(0,e);for(var t=0;t<Rt.length;t++){var n=Rt[t];if(null!=e[n]){var r=Array.isArray(e[n]);e.multiple&&!r?s("The `%s` prop supplied to <select> must be an array if `multiple` is true.%s",n,Nt()):!e.multiple&&r&&s("The `%s` prop supplied to <select> must be a scalar value if `multiple` is false.%s",n,Nt())}}}(t),n._wrapperState={wasMultiple:!!t.multiple},void 0===t.value||void 0===t.defaultValue||bt||(s("Select elements must be either controlled or uncontrolled (specify either the value prop, or the defaultValue prop, but not both). Decide between using a controlled or uncontrolled select element and remove one of these props. More info: https://reactjs.org/link/controlled-components"),bt=!0)}var kt=!1;function At(e,t){var r=e;if(null!=t.dangerouslySetInnerHTML)throw Error("`dangerouslySetInnerHTML` does not make sense on <textarea>.");return n({},t,{value:void 0,defaultValue:void 0,children:Ze(r._wrapperState.initialValue)})}function It(e,t){var n=e;nt(0,t),void 0===t.value||void 0===t.defaultValue||kt||(s("%s contains a textarea with both value and defaultValue props. Textarea elements must be either controlled or uncontrolled (specify either the value prop, or the defaultValue prop, but not both). Decide between using a controlled or uncontrolled textarea and remove one of these props. More info: https://reactjs.org/link/controlled-components",Xe()||"A component"),kt=!0);var r=t.value;if(null==r){var a=t.children,o=t.defaultValue;if(null!=a){if(s("Use the `defaultValue` or `value` props instead of setting children on <textarea>."),null!=o)throw Error("If you supply `defaultValue` on a <textarea>, do not pass children.");if(Array.isArray(a)){if(!(a.length<=1))throw Error("<textarea> can only have at most one child.");a=a[0]}o=a}null==o&&(o=""),r=o}n._wrapperState={initialValue:et(r)}}function Lt(e,t){var n=e,r=et(t.value),a=et(t.defaultValue);if(null!=r){var o=Ze(r);o!==n.value&&(n.value=o),null==t.defaultValue&&n.defaultValue!==o&&(n.defaultValue=o)}null!=a&&(n.defaultValue=Ze(a))}function _t(e,t){var n=e.textContent;n===e._wrapperState.initialValue&&""!==n&&null!==n&&(e.value=n)}var Pt="http://www.w3.org/1999/xhtml",Mt="http://www.w3.org/2000/svg";function Dt(e){switch(e){case"svg":return Mt;case"math":return"http://www.w3.org/1998/Math/MathML";default:return Pt}}function Ut(e,t){return null==e||e===Pt?Dt(t):e===Mt&&"foreignObject"===t?Pt:e}var jt,Ft,zt=(Ft=function(e,t){if("http://www.w3.org/2000/svg"!==e.namespaceURI||"innerHTML"in e)e.innerHTML=t;else{(jt=jt||document.createElement("div")).innerHTML="<svg>"+t.valueOf().toString()+"</svg>";for(var n=jt.firstChild;e.firstChild;)e.removeChild(e.firstChild);for(;n.firstChild;)e.appendChild(n.firstChild)}},"undefined"!=typeof MSApp&&MSApp.execUnsafeLocalFunction?function(e,t,n,r){MSApp.execUnsafeLocalFunction(function(){return Ft(e,t)})}:Ft),Bt=function(e,t){if(t){var n=e.firstChild;if(n&&n===e.lastChild&&3===n.nodeType)return void(n.nodeValue=t)}e.textContent=t},Ht={animation:["animationDelay","animationDirection","animationDuration","animationFillMode","animationIterationCount","animationName","animationPlayState","animationTimingFunction"],background:["backgroundAttachment","backgroundClip","backgroundColor","backgroundImage","backgroundOrigin","backgroundPositionX","backgroundPositionY","backgroundRepeat","backgroundSize"],backgroundPosition:["backgroundPositionX","backgroundPositionY"],border:["borderBottomColor","borderBottomStyle","borderBottomWidth","borderImageOutset","borderImageRepeat","borderImageSlice","borderImageSource","borderImageWidth","borderLeftColor","borderLeftStyle","borderLeftWidth","borderRightColor","borderRightStyle","borderRightWidth","borderTopColor","borderTopStyle","borderTopWidth"],borderBlockEnd:["borderBlockEndColor","borderBlockEndStyle","borderBlockEndWidth"],borderBlockStart:["borderBlockStartColor","borderBlockStartStyle","borderBlockStartWidth"],borderBottom:["borderBottomColor","borderBottomStyle","borderBottomWidth"],borderColor:["borderBottomColor","borderLeftColor","borderRightColor","borderTopColor"],borderImage:["borderImageOutset","borderImageRepeat","borderImageSlice","borderImageSource","borderImageWidth"],borderInlineEnd:["borderInlineEndColor","borderInlineEndStyle","borderInlineEndWidth"],borderInlineStart:["borderInlineStartColor","borderInlineStartStyle","borderInlineStartWidth"],borderLeft:["borderLeftColor","borderLeftStyle","borderLeftWidth"],borderRadius:["borderBottomLeftRadius","borderBottomRightRadius","borderTopLeftRadius","borderTopRightRadius"],borderRight:["borderRightColor","borderRightStyle","borderRightWidth"],borderStyle:["borderBottomStyle","borderLeftStyle","borderRightStyle","borderTopStyle"],borderTop:["borderTopColor","borderTopStyle","borderTopWidth"],borderWidth:["borderBottomWidth","borderLeftWidth","borderRightWidth","borderTopWidth"],columnRule:["columnRuleColor","columnRuleStyle","columnRuleWidth"],columns:["columnCount","columnWidth"],flex:["flexBasis","flexGrow","flexShrink"],flexFlow:["flexDirection","flexWrap"],font:["fontFamily","fontFeatureSettings","fontKerning","fontLanguageOverride","fontSize","fontSizeAdjust","fontStretch","fontStyle","fontVariant","fontVariantAlternates","fontVariantCaps","fontVariantEastAsian","fontVariantLigatures","fontVariantNumeric","fontVariantPosition","fontWeight","lineHeight"],fontVariant:["fontVariantAlternates","fontVariantCaps","fontVariantEastAsian","fontVariantLigatures","fontVariantNumeric","fontVariantPosition"],gap:["columnGap","rowGap"],grid:["gridAutoColumns","gridAutoFlow","gridAutoRows","gridTemplateAreas","gridTemplateColumns","gridTemplateRows"],gridArea:["gridColumnEnd","gridColumnStart","gridRowEnd","gridRowStart"],gridColumn:["gridColumnEnd","gridColumnStart"],gridColumnGap:["columnGap"],gridGap:["columnGap","rowGap"],gridRow:["gridRowEnd","gridRowStart"],gridRowGap:["rowGap"],gridTemplate:["gridTemplateAreas","gridTemplateColumns","gridTemplateRows"],listStyle:["listStyleImage","listStylePosition","listStyleType"],margin:["marginBottom","marginLeft","marginRight","marginTop"],marker:["markerEnd","markerMid","markerStart"],mask:["maskClip","maskComposite","maskImage","maskMode","maskOrigin","maskPositionX","maskPositionY","maskRepeat","maskSize"],maskPosition:["maskPositionX","maskPositionY"],outline:["outlineColor","outlineStyle","outlineWidth"],overflow:["overflowX","overflowY"],padding:["paddingBottom","paddingLeft","paddingRight","paddingTop"],placeContent:["alignContent","justifyContent"],placeItems:["alignItems","justifyItems"],placeSelf:["alignSelf","justifySelf"],textDecoration:["textDecorationColor","textDecorationLine","textDecorationStyle"],textEmphasis:["textEmphasisColor","textEmphasisStyle"],transition:["transitionDelay","transitionDuration","transitionProperty","transitionTimingFunction"],wordWrap:["overflowWrap"]},Vt={animationIterationCount:!0,borderImageOutset:!0,borderImageSlice:!0,borderImageWidth:!0,boxFlex:!0,boxFlexGroup:!0,boxOrdinalGroup:!0,columnCount:!0,columns:!0,flex:!0,flexGrow:!0,flexPositive:!0,flexShrink:!0,flexNegative:!0,flexOrder:!0,gridArea:!0,gridRow:!0,gridRowEnd:!0,gridRowSpan:!0,gridRowStart:!0,gridColumn:!0,gridColumnEnd:!0,gridColumnSpan:!0,gridColumnStart:!0,fontWeight:!0,lineClamp:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,tabSize:!0,widows:!0,zIndex:!0,zoom:!0,fillOpacity:!0,floodOpacity:!0,stopOpacity:!0,strokeDasharray:!0,strokeDashoffset:!0,strokeMiterlimit:!0,strokeOpacity:!0,strokeWidth:!0},Wt=["Webkit","ms","Moz","O"];function Gt(e,t,n){return null==t||"boolean"==typeof t||""===t?"":n||"number"!=typeof t||0===t||Vt.hasOwnProperty(e)&&Vt[e]?(""+t).trim():t+"px"}Object.keys(Vt).forEach(function(e){Wt.forEach(function(t){Vt[function(e,t){return e+t.charAt(0).toUpperCase()+t.substring(1)}(t,e)]=Vt[e]})});var Yt=/([A-Z])/g,$t=/^ms-/,Xt=/^(?:webkit|moz|o)[A-Z]/,qt=/^-ms-/,Kt=/-(.)/g,Jt=/;\s*$/,Qt={},Zt={},en=!1,tn=!1;function nn(e){var t="",n="";for(var r in e)if(e.hasOwnProperty(r)){var a=e[r];if(null!=a){var o=0===r.indexOf("--");t+=n+(o?r:r.replace(Yt,"-$1").toLowerCase().replace($t,"-ms-"))+":",t+=Gt(r,a,o),n=";"}}return t||null}function rn(e,t){var n,r,a=e.style;for(var o in t)if(t.hasOwnProperty(o)){var i=0===o.indexOf("--");i||(r=t[o],(n=o).indexOf("-")>-1?function(e){Qt.hasOwnProperty(e)&&Qt[e]||(Qt[e]=!0,s("Unsupported style property %s. Did you mean %s?",e,e.replace(qt,"ms-").replace(Kt,function(e,t){return t.toUpperCase()})))}(n):Xt.test(n)?function(e){Qt.hasOwnProperty(e)&&Qt[e]||(Qt[e]=!0,s("Unsupported vendor-prefixed style property %s. Did you mean %s?",e,e.charAt(0).toUpperCase()+e.slice(1)))}(n):Jt.test(r)&&function(e,t){Zt.hasOwnProperty(t)&&Zt[t]||(Zt[t]=!0,s('Style property values shouldn\'t contain a semicolon. Try "%s: %s" instead.',e,t.replace(Jt,"")))}(n,r),"number"==typeof r&&(isNaN(r)?function(e,t){en||(en=!0,s("`NaN` is an invalid value for the `%s` css style property.",e))}(n):isFinite(r)||function(e,t){tn||(tn=!0,s("`Infinity` is an invalid value for the `%s` css style property.",e))}(n)));var l=Gt(o,t[o],i);"float"===o&&(o="cssFloat"),i?a.setProperty(o,l):a[o]=l}}function an(e){var t={};for(var n in e)for(var r=Ht[n]||[n],a=0;a<r.length;a++)t[r[a]]=n;return t}var on=n({menuitem:!0},{area:!0,base:!0,br:!0,col:!0,embed:!0,hr:!0,img:!0,input:!0,keygen:!0,link:!0,meta:!0,param:!0,source:!0,track:!0,wbr:!0});function ln(e,t){if(t){if(on[e]&&(null!=t.children||null!=t.dangerouslySetInnerHTML))throw Error(e+" is a void element tag and must neither have `children` nor use `dangerouslySetInnerHTML`.");if(null!=t.dangerouslySetInnerHTML){if(null!=t.children)throw Error("Can only set one of `children` or `props.dangerouslySetInnerHTML`.");if("object"!=typeof t.dangerouslySetInnerHTML||!("__html"in t.dangerouslySetInnerHTML))throw Error("`props.dangerouslySetInnerHTML` must be in the form `{__html: ...}`. Please visit https://reactjs.org/link/dangerously-set-inner-html for more information.")}if(!t.suppressContentEditableWarning&&t.contentEditable&&null!=t.children&&s("A component is `contentEditable` and contains `children` managed by React. It is now your responsibility to guarantee that none of those nodes are unexpectedly modified or duplicated. This is probably not intentional."),null!=t.style&&"object"!=typeof t.style)throw Error("The `style` prop expects a mapping from style properties to values, not a string. For example, style={{marginRight: spacing + 'em'}} when using JSX.")}}function sn(e,t){if(-1===e.indexOf("-"))return"string"==typeof t.is;switch(e){case"annotation-xml":case"color-profile":case"font-face":case"font-face-src":case"font-face-uri":case"font-face-format":case"font-face-name":case"missing-glyph":return!1;default:return!0}}var cn={accept:"accept",acceptcharset:"acceptCharset","accept-charset":"acceptCharset",accesskey:"accessKey",action:"action",allowfullscreen:"allowFullScreen",alt:"alt",as:"as",async:"async",autocapitalize:"autoCapitalize",autocomplete:"autoComplete",autocorrect:"autoCorrect",autofocus:"autoFocus",autoplay:"autoPlay",autosave:"autoSave",capture:"capture",cellpadding:"cellPadding",cellspacing:"cellSpacing",challenge:"challenge",charset:"charSet",checked:"checked",children:"children",cite:"cite",class:"className",classid:"classID",classname:"className",cols:"cols",colspan:"colSpan",content:"content",contenteditable:"contentEditable",contextmenu:"contextMenu",controls:"controls",controlslist:"controlsList",coords:"coords",crossorigin:"crossOrigin",dangerouslysetinnerhtml:"dangerouslySetInnerHTML",data:"data",datetime:"dateTime",default:"default",defaultchecked:"defaultChecked",defaultvalue:"defaultValue",defer:"defer",dir:"dir",disabled:"disabled",disablepictureinpicture:"disablePictureInPicture",disableremoteplayback:"disableRemotePlayback",download:"download",draggable:"draggable",enctype:"encType",enterkeyhint:"enterKeyHint",for:"htmlFor",form:"form",formmethod:"formMethod",formaction:"formAction",formenctype:"formEncType",formnovalidate:"formNoValidate",formtarget:"formTarget",frameborder:"frameBorder",headers:"headers",height:"height",hidden:"hidden",high:"high",href:"href",hreflang:"hrefLang",htmlfor:"htmlFor",httpequiv:"httpEquiv","http-equiv":"httpEquiv",icon:"icon",id:"id",innerhtml:"innerHTML",inputmode:"inputMode",integrity:"integrity",is:"is",itemid:"itemID",itemprop:"itemProp",itemref:"itemRef",itemscope:"itemScope",itemtype:"itemType",keyparams:"keyParams",keytype:"keyType",kind:"kind",label:"label",lang:"lang",list:"list",loop:"loop",low:"low",manifest:"manifest",marginwidth:"marginWidth",marginheight:"marginHeight",max:"max",maxlength:"maxLength",media:"media",mediagroup:"mediaGroup",method:"method",min:"min",minlength:"minLength",multiple:"multiple",muted:"muted",name:"name",nomodule:"noModule",nonce:"nonce",novalidate:"noValidate",open:"open",optimum:"optimum",pattern:"pattern",placeholder:"placeholder",playsinline:"playsInline",poster:"poster",preload:"preload",profile:"profile",radiogroup:"radioGroup",readonly:"readOnly",referrerpolicy:"referrerPolicy",rel:"rel",required:"required",reversed:"reversed",role:"role",rows:"rows",rowspan:"rowSpan",sandbox:"sandbox",scope:"scope",scoped:"scoped",scrolling:"scrolling",seamless:"seamless",selected:"selected",shape:"shape",size:"size",sizes:"sizes",span:"span",spellcheck:"spellCheck",src:"src",srcdoc:"srcDoc",srclang:"srcLang",srcset:"srcSet",start:"start",step:"step",style:"style",summary:"summary",tabindex:"tabIndex",target:"target",title:"title",type:"type",usemap:"useMap",value:"value",width:"width",wmode:"wmode",wrap:"wrap",about:"about",accentheight:"accentHeight","accent-height":"accentHeight",accumulate:"accumulate",additive:"additive",alignmentbaseline:"alignmentBaseline","alignment-baseline":"alignmentBaseline",allowreorder:"allowReorder",alphabetic:"alphabetic",amplitude:"amplitude",arabicform:"arabicForm","arabic-form":"arabicForm",ascent:"ascent",attributename:"attributeName",attributetype:"attributeType",autoreverse:"autoReverse",azimuth:"azimuth",basefrequency:"baseFrequency",baselineshift:"baselineShift","baseline-shift":"baselineShift",baseprofile:"baseProfile",bbox:"bbox",begin:"begin",bias:"bias",by:"by",calcmode:"calcMode",capheight:"capHeight","cap-height":"capHeight",clip:"clip",clippath:"clipPath","clip-path":"clipPath",clippathunits:"clipPathUnits",cliprule:"clipRule","clip-rule":"clipRule",color:"color",colorinterpolation:"colorInterpolation","color-interpolation":"colorInterpolation",colorinterpolationfilters:"colorInterpolationFilters","color-interpolation-filters":"colorInterpolationFilters",colorprofile:"colorProfile","color-profile":"colorProfile",colorrendering:"colorRendering","color-rendering":"colorRendering",contentscripttype:"contentScriptType",contentstyletype:"contentStyleType",cursor:"cursor",cx:"cx",cy:"cy",d:"d",datatype:"datatype",decelerate:"decelerate",descent:"descent",diffuseconstant:"diffuseConstant",direction:"direction",display:"display",divisor:"divisor",dominantbaseline:"dominantBaseline","dominant-baseline":"dominantBaseline",dur:"dur",dx:"dx",dy:"dy",edgemode:"edgeMode",elevation:"elevation",enablebackground:"enableBackground","enable-background":"enableBackground",end:"end",exponent:"exponent",externalresourcesrequired:"externalResourcesRequired",fill:"fill",fillopacity:"fillOpacity","fill-opacity":"fillOpacity",fillrule:"fillRule","fill-rule":"fillRule",filter:"filter",filterres:"filterRes",filterunits:"filterUnits",floodopacity:"floodOpacity","flood-opacity":"floodOpacity",floodcolor:"floodColor","flood-color":"floodColor",focusable:"focusable",fontfamily:"fontFamily","font-family":"fontFamily",fontsize:"fontSize","font-size":"fontSize",fontsizeadjust:"fontSizeAdjust","font-size-adjust":"fontSizeAdjust",fontstretch:"fontStretch","font-stretch":"fontStretch",fontstyle:"fontStyle","font-style":"fontStyle",fontvariant:"fontVariant","font-variant":"fontVariant",fontweight:"fontWeight","font-weight":"fontWeight",format:"format",from:"from",fx:"fx",fy:"fy",g1:"g1",g2:"g2",glyphname:"glyphName","glyph-name":"glyphName",glyphorientationhorizontal:"glyphOrientationHorizontal","glyph-orientation-horizontal":"glyphOrientationHorizontal",glyphorientationvertical:"glyphOrientationVertical","glyph-orientation-vertical":"glyphOrientationVertical",glyphref:"glyphRef",gradienttransform:"gradientTransform",gradientunits:"gradientUnits",hanging:"hanging",horizadvx:"horizAdvX","horiz-adv-x":"horizAdvX",horizoriginx:"horizOriginX","horiz-origin-x":"horizOriginX",ideographic:"ideographic",imagerendering:"imageRendering","image-rendering":"imageRendering",in2:"in2",in:"in",inlist:"inlist",intercept:"intercept",k1:"k1",k2:"k2",k3:"k3",k4:"k4",k:"k",kernelmatrix:"kernelMatrix",kernelunitlength:"kernelUnitLength",kerning:"kerning",keypoints:"keyPoints",keysplines:"keySplines",keytimes:"keyTimes",lengthadjust:"lengthAdjust",letterspacing:"letterSpacing","letter-spacing":"letterSpacing",lightingcolor:"lightingColor","lighting-color":"lightingColor",limitingconeangle:"limitingConeAngle",local:"local",markerend:"markerEnd","marker-end":"markerEnd",markerheight:"markerHeight",markermid:"markerMid","marker-mid":"markerMid",markerstart:"markerStart","marker-start":"markerStart",markerunits:"markerUnits",markerwidth:"markerWidth",mask:"mask",maskcontentunits:"maskContentUnits",maskunits:"maskUnits",mathematical:"mathematical",mode:"mode",numoctaves:"numOctaves",offset:"offset",opacity:"opacity",operator:"operator",order:"order",orient:"orient",orientation:"orientation",origin:"origin",overflow:"overflow",overlineposition:"overlinePosition","overline-position":"overlinePosition",overlinethickness:"overlineThickness","overline-thickness":"overlineThickness",paintorder:"paintOrder","paint-order":"paintOrder",panose1:"panose1","panose-1":"panose1",pathlength:"pathLength",patterncontentunits:"patternContentUnits",patterntransform:"patternTransform",patternunits:"patternUnits",pointerevents:"pointerEvents","pointer-events":"pointerEvents",points:"points",pointsatx:"pointsAtX",pointsaty:"pointsAtY",pointsatz:"pointsAtZ",prefix:"prefix",preservealpha:"preserveAlpha",preserveaspectratio:"preserveAspectRatio",primitiveunits:"primitiveUnits",property:"property",r:"r",radius:"radius",refx:"refX",refy:"refY",renderingintent:"renderingIntent","rendering-intent":"renderingIntent",repeatcount:"repeatCount",repeatdur:"repeatDur",requiredextensions:"requiredExtensions",requiredfeatures:"requiredFeatures",resource:"resource",restart:"restart",result:"result",results:"results",rotate:"rotate",rx:"rx",ry:"ry",scale:"scale",security:"security",seed:"seed",shaperendering:"shapeRendering","shape-rendering":"shapeRendering",slope:"slope",spacing:"spacing",specularconstant:"specularConstant",specularexponent:"specularExponent",speed:"speed",spreadmethod:"spreadMethod",startoffset:"startOffset",stddeviation:"stdDeviation",stemh:"stemh",stemv:"stemv",stitchtiles:"stitchTiles",stopcolor:"stopColor","stop-color":"stopColor",stopopacity:"stopOpacity","stop-opacity":"stopOpacity",strikethroughposition:"strikethroughPosition","strikethrough-position":"strikethroughPosition",strikethroughthickness:"strikethroughThickness","strikethrough-thickness":"strikethroughThickness",string:"string",stroke:"stroke",strokedasharray:"strokeDasharray","stroke-dasharray":"strokeDasharray",strokedashoffset:"strokeDashoffset","stroke-dashoffset":"strokeDashoffset",strokelinecap:"strokeLinecap","stroke-linecap":"strokeLinecap",strokelinejoin:"strokeLinejoin","stroke-linejoin":"strokeLinejoin",strokemiterlimit:"strokeMiterlimit","stroke-miterlimit":"strokeMiterlimit",strokewidth:"strokeWidth","stroke-width":"strokeWidth",strokeopacity:"strokeOpacity","stroke-opacity":"strokeOpacity",suppresscontenteditablewarning:"suppressContentEditableWarning",suppresshydrationwarning:"suppressHydrationWarning",surfacescale:"surfaceScale",systemlanguage:"systemLanguage",tablevalues:"tableValues",targetx:"targetX",targety:"targetY",textanchor:"textAnchor","text-anchor":"textAnchor",textdecoration:"textDecoration","text-decoration":"textDecoration",textlength:"textLength",textrendering:"textRendering","text-rendering":"textRendering",to:"to",transform:"transform",typeof:"typeof",u1:"u1",u2:"u2",underlineposition:"underlinePosition","underline-position":"underlinePosition",underlinethickness:"underlineThickness","underline-thickness":"underlineThickness",unicode:"unicode",unicodebidi:"unicodeBidi","unicode-bidi":"unicodeBidi",unicoderange:"unicodeRange","unicode-range":"unicodeRange",unitsperem:"unitsPerEm","units-per-em":"unitsPerEm",unselectable:"unselectable",valphabetic:"vAlphabetic","v-alphabetic":"vAlphabetic",values:"values",vectoreffect:"vectorEffect","vector-effect":"vectorEffect",version:"version",vertadvy:"vertAdvY","vert-adv-y":"vertAdvY",vertoriginx:"vertOriginX","vert-origin-x":"vertOriginX",vertoriginy:"vertOriginY","vert-origin-y":"vertOriginY",vhanging:"vHanging","v-hanging":"vHanging",videographic:"vIdeographic","v-ideographic":"vIdeographic",viewbox:"viewBox",viewtarget:"viewTarget",visibility:"visibility",vmathematical:"vMathematical","v-mathematical":"vMathematical",vocab:"vocab",widths:"widths",wordspacing:"wordSpacing","word-spacing":"wordSpacing",writingmode:"writingMode","writing-mode":"writingMode",x1:"x1",x2:"x2",x:"x",xchannelselector:"xChannelSelector",xheight:"xHeight","x-height":"xHeight",xlinkactuate:"xlinkActuate","xlink:actuate":"xlinkActuate",xlinkarcrole:"xlinkArcrole","xlink:arcrole":"xlinkArcrole",xlinkhref:"xlinkHref","xlink:href":"xlinkHref",xlinkrole:"xlinkRole","xlink:role":"xlinkRole",xlinkshow:"xlinkShow","xlink:show":"xlinkShow",xlinktitle:"xlinkTitle","xlink:title":"xlinkTitle",xlinktype:"xlinkType","xlink:type":"xlinkType",xmlbase:"xmlBase","xml:base":"xmlBase",xmllang:"xmlLang","xml:lang":"xmlLang",xmlns:"xmlns","xml:space":"xmlSpace",xmlnsxlink:"xmlnsXlink","xmlns:xlink":"xmlnsXlink",xmlspace:"xmlSpace",y1:"y1",y2:"y2",y:"y",ychannelselector:"yChannelSelector",z:"z",zoomandpan:"zoomAndPan"},un={"aria-current":0,"aria-details":0,"aria-disabled":0,"aria-hidden":0,"aria-invalid":0,"aria-keyshortcuts":0,"aria-label":0,"aria-roledescription":0,"aria-autocomplete":0,"aria-checked":0,"aria-expanded":0,"aria-haspopup":0,"aria-level":0,"aria-modal":0,"aria-multiline":0,"aria-multiselectable":0,"aria-orientation":0,"aria-placeholder":0,"aria-pressed":0,"aria-readonly":0,"aria-required":0,"aria-selected":0,"aria-sort":0,"aria-valuemax":0,"aria-valuemin":0,"aria-valuenow":0,"aria-valuetext":0,"aria-atomic":0,"aria-busy":0,"aria-live":0,"aria-relevant":0,"aria-dropeffect":0,"aria-grabbed":0,"aria-activedescendant":0,"aria-colcount":0,"aria-colindex":0,"aria-colspan":0,"aria-controls":0,"aria-describedby":0,"aria-errormessage":0,"aria-flowto":0,"aria-labelledby":0,"aria-owns":0,"aria-posinset":0,"aria-rowcount":0,"aria-rowindex":0,"aria-rowspan":0,"aria-setsize":0},fn={},dn=new RegExp("^(aria)-["+L+"]*$"),pn=new RegExp("^(aria)[A-Z]["+L+"]*$"),mn=Object.prototype.hasOwnProperty;function hn(e,t){if(mn.call(fn,t)&&fn[t])return!0;if(pn.test(t)){var n="aria-"+t.slice(4).toLowerCase(),r=un.hasOwnProperty(n)?n:null;if(null==r)return s("Invalid ARIA attribute `%s`. ARIA attributes follow the pattern aria-* and must be lowercase.",t),fn[t]=!0,!0;if(t!==r)return s("Invalid ARIA attribute `%s`. Did you mean `%s`?",t,r),fn[t]=!0,!0}if(dn.test(t)){var a=t.toLowerCase(),o=un.hasOwnProperty(a)?a:null;if(null==o)return fn[t]=!0,!1;if(t!==o)return s("Unknown ARIA attribute `%s`. Did you mean `%s`?",t,o),fn[t]=!0,!0}return!0}var gn,yn=!1,vn={},bn=Object.prototype.hasOwnProperty,En=/^on./,Tn=/^on[^A-Z]/,Sn=new RegExp("^(aria)-["+L+"]*$"),wn=new RegExp("^(aria)[A-Z]["+L+"]*$");function Nn(e){var t=e.target||e.srcElement||window;return t.correspondingUseElement&&(t=t.correspondingUseElement),3===t.nodeType?t.parentNode:t}gn=function(e,t,n,r){if(bn.call(vn,t)&&vn[t])return!0;var a=t.toLowerCase();if("onfocusin"===a||"onfocusout"===a)return s("React uses onFocus and onBlur instead of onFocusIn and onFocusOut. All React events are normalized to bubble, so onFocusIn and onFocusOut are not needed/supported by React."),vn[t]=!0,!0;if(null!=r){var o=r.possibleRegistrationNames;if(r.registrationNameDependencies.hasOwnProperty(t))return!0;var i=o.hasOwnProperty(a)?o[a]:null;if(null!=i)return s("Invalid event handler property `%s`. Did you mean `%s`?",t,i),vn[t]=!0,!0;if(En.test(t))return s("Unknown event handler property `%s`. It will be ignored.",t),vn[t]=!0,!0}else if(En.test(t))return Tn.test(t)&&s("Invalid event handler property `%s`. React events use the camelCase naming convention, for example `onClick`.",t),vn[t]=!0,!0;if(Sn.test(t)||wn.test(t))return!0;if("innerhtml"===a)return s("Directly setting property `innerHTML` is not permitted. For more information, lookup documentation on `dangerouslySetInnerHTML`."),vn[t]=!0,!0;if("aria"===a)return s("The `aria` attribute is reserved for future use in React. Pass individual `aria-` attributes instead."),vn[t]=!0,!0;if("is"===a&&null!=n&&"string"!=typeof n)return s("Received a `%s` for a string attribute `is`. If this is expected, cast the value to a string.",typeof n),vn[t]=!0,!0;if("number"==typeof n&&isNaN(n))return s("Received NaN for the `%s` attribute. If this is expected, cast the value to a string.",t),vn[t]=!0,!0;var l=H(t),c=null!==l&&0===l.type;if(cn.hasOwnProperty(a)){var u=cn[a];if(u!==t)return s("Invalid DOM property `%s`. Did you mean `%s`?",t,u),vn[t]=!0,!0}else if(!c&&t!==a)return s("React does not recognize the `%s` prop on a DOM element. If you intentionally want it to appear in the DOM as a custom attribute, spell it as lowercase `%s` instead. If you accidentally passed it from a parent component, remove it from the DOM element.",t,a),vn[t]=!0,!0;return"boolean"==typeof n&&z(t,n,l,!1)?(n?s('Received `%s` for a non-boolean attribute `%s`.\n\nIf you want to write it to the DOM, pass a string instead: %s="%s" or %s={value.toString()}.',n,t,t,n,t):s('Received `%s` for a non-boolean attribute `%s`.\n\nIf you want to write it to the DOM, pass a string instead: %s="%s" or %s={value.toString()}.\n\nIf you used to conditionally omit it with %s={condition && value}, pass %s={condition ? value : undefined} instead.',n,t,t,n,t,t,t),vn[t]=!0,!0):!!c||(z(t,n,l,!1)?(vn[t]=!0,!1):("false"!==n&&"true"!==n||null===l||3!==l.type||(s("Received the string `%s` for the boolean attribute `%s`. %s Did you mean %s={%s}?",n,t,"false"===n?"The browser will interpret it as a truthy value.":'Although this works, it will not work as expected if you pass the string "false".',t,n),vn[t]=!0),!0))};var Rn=null,On=null,Cn=null;function xn(e){var t=Ul(e);if(t){if("function"!=typeof Rn)throw Error("setRestoreImplementation() needs to be called to handle a target for controlled events. This error is likely caused by a bug in React. Please file an issue.");var n=t.stateNode;if(n){var r=Fl(n);Rn(t.stateNode,t.type,r)}}}function kn(e){On?Cn?Cn.push(e):Cn=[e]:On=e}function An(){if(On){var e=On,t=Cn;if(On=null,Cn=null,xn(e),t)for(var n=0;n<t.length;n++)xn(t[n])}}var In=function(e,t){return e(t)},Ln=function(e,t,n,r,a){return e(t,n,r,a)},_n=function(){},Pn=In,Mn=!1,Dn=!1;function Un(){(null!==On||null!==Cn)&&(_n(),An())}function jn(e,t){var n=e.stateNode;if(null===n)return null;var r=Fl(n);if(null===r)return null;var a=r[t];if(function(e,t,n){switch(e){case"onClick":case"onClickCapture":case"onDoubleClick":case"onDoubleClickCapture":case"onMouseDown":case"onMouseDownCapture":case"onMouseMove":case"onMouseMoveCapture":case"onMouseUp":case"onMouseUpCapture":case"onMouseEnter":return!(!n.disabled||!function(e){return"button"===e||"input"===e||"select"===e||"textarea"===e}(t));default:return!1}}(t,e.type,r))return null;if(a&&"function"!=typeof a)throw Error("Expected `"+t+"` listener to be a function, instead got a value of `"+typeof a+"` type.");return a}var Fn=!1;if(A)try{var zn={};Object.defineProperty(zn,"passive",{get:function(){Fn=!0}}),window.addEventListener("test",zn,zn),window.removeEventListener("test",zn,zn)}catch(e){Fn=!1}function Bn(e,t,n,r,a,o,i,l,s){var c=Array.prototype.slice.call(arguments,3);try{t.apply(n,c)}catch(e){this.onError(e)}}var Hn=Bn;if("undefined"!=typeof window&&"function"==typeof window.dispatchEvent&&"undefined"!=typeof document&&"function"==typeof document.createEvent){var Vn=document.createElement("react");Hn=function(e,t,n,r,a,o,i,l,s){if("undefined"==typeof document)throw Error("The `document` global was defined when React was initialized, but is not defined anymore. This can happen in a test environment if a component schedules an update from an asynchronous callback, but the test has already finished running. To solve this, you can either unmount the component at the end of your test (and ensure that any asynchronous operations get canceled in `componentWillUnmount`), or you can change the test itself to be asynchronous.");var c=document.createEvent("Event"),u=!1,f=!0,d=window.event,p=Object.getOwnPropertyDescriptor(window,"event");function m(){Vn.removeEventListener(T,y,!1),void 0!==window.event&&window.hasOwnProperty("event")&&(window.event=d)}var h,g=Array.prototype.slice.call(arguments,3);function y(){u=!0,m(),t.apply(n,g),f=!1}var v=!1,b=!1;function E(e){if(v=!0,null===(h=e.error)&&0===e.colno&&0===e.lineno&&(b=!0),e.defaultPrevented&&null!=h&&"object"==typeof h)try{h._suppressLogging=!0}catch(e){}}var T="react-"+(e||"invokeguardedcallback");if(window.addEventListener("error",E),Vn.addEventListener(T,y,!1),c.initEvent(T,!1,!1),Vn.dispatchEvent(c),p&&Object.defineProperty(window,"event",p),u&&f&&(v?b&&(h=new Error("A cross-origin error was thrown. React doesn't have access to the actual error object in development. See https://reactjs.org/link/crossorigin-error for more information.")):h=new Error("An error was thrown inside one of your components, but React doesn't know what it was. This is likely due to browser flakiness. React does its best to preserve the \"Pause on exceptions\" behavior of the DevTools, which requires some DEV-mode only tricks. It's possible that these don't work in your browser. Try triggering the error in production mode, or switching to a modern browser. If you suspect that this is actually an issue with React, please file an issue."),this.onError(h)),window.removeEventListener("error",E),!u)return m(),Bn.apply(this,arguments)}}var Wn=Hn,Gn=!1,Yn=null,$n=!1,Xn=null,qn={onError:function(e){Gn=!0,Yn=e}};function Kn(e,t,n,r,a,o,i,l,s){Gn=!1,Yn=null,Wn.apply(qn,arguments)}function Jn(){return Gn}function Qn(){if(Gn){var e=Yn;return Gn=!1,Yn=null,e}throw Error("clearCaughtError was called but no error was captured. This error is likely caused by a bug in React. Please file an issue.")}function Zn(e){return e._reactInternals}var er,tr,nr,rr,ar=64,or=128,ir=256,lr=8192,sr=2048,cr=4096,ur=16384,fr=o.ReactCurrentOwner;function dr(e){var t=e,n=e;if(e.alternate)for(;t.return;)t=t.return;else{var r=t;do{0!=(1026&(t=r).flags)&&(n=t.return),r=t.return}while(r)}return 3===t.tag?n:null}function pr(e){if(e.tag===h){var t=e.memoizedState;if(null===t){var n=e.alternate;null!==n&&(t=n.memoizedState)}if(null!==t)return t.dehydrated}return null}function mr(e){return 3===e.tag?e.stateNode.containerInfo:null}function hr(e){if(dr(e)!==e)throw Error("Unable to find node on an unmounted component.")}function gr(e){var t=e.alternate;if(!t){var n=dr(e);if(null===n)throw Error("Unable to find node on an unmounted component.");return n!==e?null:e}for(var r=e,a=t;;){var o=r.return;if(null===o)break;var i=o.alternate;if(null===i){var l=o.return;if(null!==l){r=a=l;continue}break}if(o.child===i.child){for(var s=o.child;s;){if(s===r)return hr(o),e;if(s===a)return hr(o),t;s=s.sibling}throw Error("Unable to find node on an unmounted component.")}if(r.return!==a.return)r=o,a=i;else{for(var c=!1,u=o.child;u;){if(u===r){c=!0,r=o,a=i;break}if(u===a){c=!0,a=o,r=i;break}u=u.sibling}if(!c){for(u=i.child;u;){if(u===r){c=!0,r=i,a=o;break}if(u===a){c=!0,a=i,r=o;break}u=u.sibling}if(!c)throw Error("Child was not found in either parent set. This indicates a bug in React related to the return pointer. Please file an issue.")}}if(r.alternate!==a)throw Error("Return fibers should always be each others' alternates. This error is likely caused by a bug in React. Please file an issue.")}if(3!==r.tag)throw Error("Unable to find node on an unmounted component.");return r.stateNode.current===r?e:t}function yr(e){var t=gr(e);if(!t)return null;for(var n=t;;){if(5===n.tag||6===n.tag)return n;if(n.child)n.child.return=n,n=n.child;else{if(n===t)return null;for(;!n.sibling;){if(!n.return||n.return===t)return null;n=n.return}n.sibling.return=n.return,n=n.sibling}}return null}var vr=!1,br=[],Er=null,Tr=null,Sr=null,wr=new Map,Nr=new Map,Rr=[],Or=["mousedown","mouseup","touchcancel","touchend","touchstart","auxclick","dblclick","pointercancel","pointerdown","pointerup","dragend","dragstart","drop","compositionend","compositionstart","keydown","keypress","keyup","input","textInput","copy","cut","paste","click","change","contextmenu","reset","submit"];function Cr(e){return Or.indexOf(e)>-1}function xr(e,t,n,r,a){return{blockedOn:e,domEventName:t,eventSystemFlags:16|n,nativeEvent:a,targetContainers:[r]}}function kr(e,t,n,r,a){var o=xr(e,t,n,r,a);br.push(o)}function Ar(e,t){switch(e){case"focusin":case"focusout":Er=null;break;case"dragenter":case"dragleave":Tr=null;break;case"mouseover":case"mouseout":Sr=null;break;case"pointerover":case"pointerout":wr.delete(t.pointerId);break;case"gotpointercapture":case"lostpointercapture":Nr.delete(t.pointerId)}}function Ir(e,t,n,r,a,o){if(null===e||e.nativeEvent!==o){var i=xr(t,n,r,a,o);if(null!==t){var l=Ul(t);null!==l&&tr(l)}return i}e.eventSystemFlags|=r;var s=e.targetContainers;return null!==a&&-1===s.indexOf(a)&&s.push(a),e}function Lr(e){var t=Dl(e.target);if(null!==t){var n=dr(t);if(null!==n){var a=n.tag;if(a===h){var o=pr(n);if(null!==o)return e.blockedOn=o,void rr(e.lanePriority,function(){r.unstable_runWithPriority(e.priority,function(){nr(n)})})}else if(3===a&&n.stateNode.hydrate)return void(e.blockedOn=mr(n))}}e.blockedOn=null}function _r(e){if(null!==e.blockedOn)return!1;for(var t=e.targetContainers;t.length>0;){var n=ja(e.domEventName,e.eventSystemFlags,t[0],e.nativeEvent);if(null!==n){var r=Ul(n);return null!==r&&tr(r),e.blockedOn=n,!1}t.shift()}return!0}function Pr(e,t,n){_r(e)&&n.delete(t)}function Mr(){for(vr=!1;br.length>0;){var e=br[0];if(null!==e.blockedOn){var t=Ul(e.blockedOn);null!==t&&er(t);break}for(var n=e.targetContainers;n.length>0;){var r=ja(e.domEventName,e.eventSystemFlags,n[0],e.nativeEvent);if(null!==r){e.blockedOn=r;break}n.shift()}null===e.blockedOn&&br.shift()}null!==Er&&_r(Er)&&(Er=null),null!==Tr&&_r(Tr)&&(Tr=null),null!==Sr&&_r(Sr)&&(Sr=null),wr.forEach(Pr),Nr.forEach(Pr)}function Dr(e,t){e.blockedOn===t&&(e.blockedOn=null,vr||(vr=!0,r.unstable_scheduleCallback(r.unstable_NormalPriority,Mr)))}function Ur(e){if(br.length>0){Dr(br[0],e);for(var t=1;t<br.length;t++){var n=br[t];n.blockedOn===e&&(n.blockedOn=null)}}null!==Er&&Dr(Er,e),null!==Tr&&Dr(Tr,e),null!==Sr&&Dr(Sr,e);var r=function(t){return Dr(t,e)};wr.forEach(r),Nr.forEach(r);for(var a=0;a<Rr.length;a++){var o=Rr[a];o.blockedOn===e&&(o.blockedOn=null)}for(;Rr.length>0;){var i=Rr[0];if(null!==i.blockedOn)break;Lr(i),null===i.blockedOn&&Rr.shift()}}function jr(e,t){var n={};return n[e.toLowerCase()]=t.toLowerCase(),n["Webkit"+e]="webkit"+t,n["Moz"+e]="moz"+t,n}var Fr={animationend:jr("Animation","AnimationEnd"),animationiteration:jr("Animation","AnimationIteration"),animationstart:jr("Animation","AnimationStart"),transitionend:jr("Transition","TransitionEnd")},zr={},Br={};function Hr(e){if(zr[e])return zr[e];if(!Fr[e])return e;var t=Fr[e];for(var n in t)if(t.hasOwnProperty(n)&&n in Br)return zr[e]=t[n];return e}A&&(Br=document.createElement("div").style,"AnimationEvent"in window||(delete Fr.animationend.animation,delete Fr.animationiteration.animation,delete Fr.animationstart.animation),"TransitionEvent"in window||delete Fr.transitionend.transition);var Vr=Hr("animationend"),Wr=Hr("animationiteration"),Gr=Hr("animationstart"),Yr=Hr("transitionend"),$r=new Map,Xr=new Map,qr=["abort","abort",Vr,"animationEnd",Wr,"animationIteration",Gr,"animationStart","canplay","canPlay","canplaythrough","canPlayThrough","durationchange","durationChange","emptied","emptied","encrypted","encrypted","ended","ended","error","error","gotpointercapture","gotPointerCapture","load","load","loadeddata","loadedData","loadedmetadata","loadedMetadata","loadstart","loadStart","lostpointercapture","lostPointerCapture","playing","playing","progress","progress","seeking","seeking","stalled","stalled","suspend","suspend","timeupdate","timeUpdate",Yr,"transitionEnd","waiting","waiting"];function Kr(e,t){for(var n=0;n<e.length;n+=2){var r=e[n],a=e[n+1],o="on"+(a[0].toUpperCase()+a.slice(1));Xr.set(r,t),$r.set(r,o),x(o,[r])}}var Jr=r.unstable_now;if(null==a.__interactionsRef||null==a.__interactionsRef.current)throw Error("It is not supported to run the profiling version of a renderer (for example, `react-dom/profiling`) without also replacing the `scheduler/tracing` module with `scheduler/tracing-profiling`. Your bundler might have a setting for aliasing both modules. Learn more at https://reactjs.org/link/profiling");Jr();var Qr=15,Zr=10,ea=3584,ta=4186112,na=62914560,ra=33554432,aa=67108864,oa=134217727,ia=134217728,la=805306368,sa=1073741824,ca=-1,ua=8;function fa(e){if(0!=(1&e))return ua=Qr,1;if(0!=(2&e))return ua=14,2;if(0!=(4&e))return ua=13,4;var t=24&e;if(0!==t)return ua=12,t;if(0!=(32&e))return ua=11,32;var n=192&e;if(0!==n)return ua=Zr,n;if(0!=(256&e))return ua=9,256;var r=ea&e;if(0!==r)return ua=8,r;if(0!=(4096&e))return ua=7,4096;var a=ta&e;if(0!==a)return ua=6,a;var o=na&e;if(0!==o)return ua=5,o;if(e&aa)return ua=4,aa;if(0!=(e&ia))return ua=3,ia;var i=la&e;return 0!==i?(ua=2,i):0!=(sa&e)?(ua=1,sa):(s("Should have found matching lanes. This is a bug in React."),ua=8,e)}function da(e,t){var n=e.pendingLanes;if(0===n)return ua=0,0;var r=0,a=0,o=e.expiredLanes,i=e.suspendedLanes,l=e.pingedLanes;if(0!==o)r=o,a=ua=Qr;else{var s=n&oa;if(0!==s){var c=s&~i;if(0!==c)r=fa(c),a=ua;else{var u=s&l;0!==u&&(r=fa(u),a=ua)}}else{var f=n&~i;0!==f?(r=fa(f),a=ua):0!==l&&(r=fa(l),a=ua)}}if(0===r)return 0;if(r=n&function(e){return(function(e){var t=31-xa(e);return t<0?0:1<<t}(e)<<1)-1}(r),0!==t&&t!==r&&0==(t&i)){if(fa(t),a<=ua)return t;ua=a}var d=e.entangledLanes;if(0!==d)for(var p=e.entanglements,m=r&d;m>0;){var h=ba(m);r|=p[h],m&=~(1<<h)}return r}function pa(e,t){return fa(e),ua>=Zr?t+250:ua>=6?t+5e3:ca}function ma(e){var t=-1073741825&e.pendingLanes;return 0!==t?t:t&sa?sa:0}function ha(e){return 0!=(e&oa)}function ga(e){return(e&na)===e}function ya(e,t){switch(e){case 0:break;case Qr:return 1;case 14:return 2;case 12:var n=va(24&~t);return 0===n?ya(Zr,t):n;case Zr:var r=va(192&~t);return 0===r?ya(8,t):r;case 8:var a=va(ea&~t);return 0===a&&0===(a=va(ta&~t))&&(a=va(ea)),a;case 6:case 5:break;case 2:var o=va(la&~t);return 0===o&&(o=va(la)),o}throw Error("Invalid update priority: "+e+". This is a bug in React.")}function va(e){return function(e){return e&-e}(e)}function ba(e){return 31-xa(e)}function Ea(e,t){return 0!=(e&t)}function Ta(e,t){return(e&t)===t}function Sa(e,t){return e|t}function wa(e,t){return e&~t}function Na(e){for(var t=[],n=0;n<31;n++)t.push(e);return t}function Ra(e,t,n){e.pendingLanes|=t;var r=t-1;e.suspendedLanes&=r,e.pingedLanes&=r,e.eventTimes[ba(t)]=n}function Oa(e,t,n){e.pingedLanes|=e.suspendedLanes&t}function Ca(e,t){e.mutableReadLanes|=t&e.pendingLanes}var xa=Math.clz32?Math.clz32:function(e){return 0===e?32:31-(ka(e)/Aa|0)|0},ka=Math.log,Aa=Math.LN2,Ia=r.unstable_UserBlockingPriority,La=r.unstable_runWithPriority,_a=!0;function Pa(e){_a=!!e}function Ma(e,t,n,r){Mn||_n(),function(e,t,n,r,a){var o=Mn;Mn=!0;try{Ln(e,t,n,r,a)}finally{(Mn=o)||Un()}}(Ua,e,t,n,r)}function Da(e,t,n,r){La(Ia,Ua.bind(null,e,t,n,r))}function Ua(e,t,n,r){var a;if(_a)if((a=0==(4&t))&&br.length>0&&Cr(e))kr(null,e,t,n,r);else{var o=ja(e,t,n,r);if(null!==o){if(a){if(Cr(e))return void kr(o,e,t,n,r);if(function(e,t,n,r,a){switch(t){case"focusin":return Er=Ir(Er,e,t,n,r,a),!0;case"dragenter":return Tr=Ir(Tr,e,t,n,r,a),!0;case"mouseover":return Sr=Ir(Sr,e,t,n,r,a),!0;case"pointerover":var o=a,i=o.pointerId;return wr.set(i,Ir(wr.get(i)||null,e,t,n,r,o)),!0;case"gotpointercapture":var l=a,s=l.pointerId;return Nr.set(s,Ir(Nr.get(s)||null,e,t,n,r,l)),!0}return!1}(o,e,t,n,r))return;Ar(e,r)}Ti(e,t,r,null,n)}else a&&Ar(e,r)}}function ja(e,t,n,r){var a=Dl(Nn(r));if(null!==a){var o=dr(a);if(null===o)a=null;else{var i=o.tag;if(i===h){var l=pr(o);if(null!==l)return l;a=null}else if(3===i){if(o.stateNode.hydrate)return mr(o);a=null}else o!==a&&(a=null)}}return Ti(e,t,r,a,n),null}var Fa=null,za=null,Ba=null;function Ha(){if(Ba)return Ba;var e,t,n=za,r=n.length,a=Va(),o=a.length;for(e=0;e<r&&n[e]===a[e];e++);var i=r-e;for(t=1;t<=i&&n[r-t]===a[o-t];t++);return Ba=a.slice(e,t>1?1-t:void 0)}function Va(){return"value"in Fa?Fa.value:Fa.textContent}function Wa(e){var t,n=e.keyCode;return"charCode"in e?0===(t=e.charCode)&&13===n&&(t=13):t=n,10===t&&(t=13),t>=32||13===t?t:0}function Ga(){return!0}function Ya(){return!1}function $a(e){function t(t,n,r,a,o){for(var i in this._reactName=t,this._targetInst=r,this.type=n,this.nativeEvent=a,this.target=o,this.currentTarget=null,e)if(e.hasOwnProperty(i)){var l=e[i];this[i]=l?l(a):a[i]}return this.isDefaultPrevented=(null!=a.defaultPrevented?a.defaultPrevented:!1===a.returnValue)?Ga:Ya,this.isPropagationStopped=Ya,this}return n(t.prototype,{preventDefault:function(){this.defaultPrevented=!0;var e=this.nativeEvent;e&&(e.preventDefault?e.preventDefault():"unknown"!=typeof e.returnValue&&(e.returnValue=!1),this.isDefaultPrevented=Ga)},stopPropagation:function(){var e=this.nativeEvent;e&&(e.stopPropagation?e.stopPropagation():"unknown"!=typeof e.cancelBubble&&(e.cancelBubble=!0),this.isPropagationStopped=Ga)},persist:function(){},isPersistent:Ga}),t}var Xa,qa,Ka,Ja={eventPhase:0,bubbles:0,cancelable:0,timeStamp:function(e){return e.timeStamp||Date.now()},defaultPrevented:0,isTrusted:0},Qa=$a(Ja),Za=n({},Ja,{view:0,detail:0}),eo=$a(Za),to=n({},Za,{screenX:0,screenY:0,clientX:0,clientY:0,pageX:0,pageY:0,ctrlKey:0,shiftKey:0,altKey:0,metaKey:0,getModifierState:mo,button:0,buttons:0,relatedTarget:function(e){return void 0===e.relatedTarget?e.fromElement===e.srcElement?e.toElement:e.fromElement:e.relatedTarget},movementX:function(e){return"movementX"in e?e.movementX:(function(e){e!==Ka&&(Ka&&"mousemove"===e.type?(Xa=e.screenX-Ka.screenX,qa=e.screenY-Ka.screenY):(Xa=0,qa=0),Ka=e)}(e),Xa)},movementY:function(e){return"movementY"in e?e.movementY:qa}}),no=$a(to),ro=$a(n({},to,{dataTransfer:0})),ao=$a(n({},Za,{relatedTarget:0})),oo=$a(n({},Ja,{animationName:0,elapsedTime:0,pseudoElement:0})),io=$a(n({},Ja,{clipboardData:function(e){return"clipboardData"in e?e.clipboardData:window.clipboardData}})),lo=$a(n({},Ja,{data:0})),so=lo,co={Esc:"Escape",Spacebar:" ",Left:"ArrowLeft",Up:"ArrowUp",Right:"ArrowRight",Down:"ArrowDown",Del:"Delete",Win:"OS",Menu:"ContextMenu",Apps:"ContextMenu",Scroll:"ScrollLock",MozPrintableKey:"Unidentified"},uo={8:"Backspace",9:"Tab",12:"Clear",13:"Enter",16:"Shift",17:"Control",18:"Alt",19:"Pause",20:"CapsLock",27:"Escape",32:" ",33:"PageUp",34:"PageDown",35:"End",36:"Home",37:"ArrowLeft",38:"ArrowUp",39:"ArrowRight",40:"ArrowDown",45:"Insert",46:"Delete",112:"F1",113:"F2",114:"F3",115:"F4",116:"F5",117:"F6",118:"F7",119:"F8",120:"F9",121:"F10",122:"F11",123:"F12",144:"NumLock",145:"ScrollLock",224:"Meta"},fo={Alt:"altKey",Control:"ctrlKey",Meta:"metaKey",Shift:"shiftKey"};function po(e){var t=this.nativeEvent;if(t.getModifierState)return t.getModifierState(e);var n=fo[e];return!!n&&!!t[n]}function mo(e){return po}var ho=$a(n({},Za,{key:function(e){if(e.key){var t=co[e.key]||e.key;if("Unidentified"!==t)return t}if("keypress"===e.type){var n=Wa(e);return 13===n?"Enter":String.fromCharCode(n)}return"keydown"===e.type||"keyup"===e.type?uo[e.keyCode]||"Unidentified":""},code:0,location:0,ctrlKey:0,shiftKey:0,altKey:0,metaKey:0,repeat:0,locale:0,getModifierState:mo,charCode:function(e){return"keypress"===e.type?Wa(e):0},keyCode:function(e){return"keydown"===e.type||"keyup"===e.type?e.keyCode:0},which:function(e){return"keypress"===e.type?Wa(e):"keydown"===e.type||"keyup"===e.type?e.keyCode:0}})),go=$a(n({},to,{pointerId:0,width:0,height:0,pressure:0,tangentialPressure:0,tiltX:0,tiltY:0,twist:0,pointerType:0,isPrimary:0})),yo=$a(n({},Za,{touches:0,targetTouches:0,changedTouches:0,altKey:0,metaKey:0,ctrlKey:0,shiftKey:0,getModifierState:mo})),vo=$a(n({},Ja,{propertyName:0,elapsedTime:0,pseudoElement:0})),bo=$a(n({},to,{deltaX:function(e){return"deltaX"in e?e.deltaX:"wheelDeltaX"in e?-e.wheelDeltaX:0},deltaY:function(e){return"deltaY"in e?e.deltaY:"wheelDeltaY"in e?-e.wheelDeltaY:"wheelDelta"in e?-e.wheelDelta:0},deltaZ:0,deltaMode:0})),Eo=[9,13,27,32],To=A&&"CompositionEvent"in window,So=null;A&&"documentMode"in document&&(So=document.documentMode);var wo=A&&"TextEvent"in window&&!So,No=A&&(!To||So&&So>8&&So<=11),Ro=String.fromCharCode(32),Oo=!1;function Co(e,t){switch(e){case"keyup":return-1!==Eo.indexOf(t.keyCode);case"keydown":return 229!==t.keyCode;case"keypress":case"mousedown":case"focusout":return!0;default:return!1}}function xo(e){var t=e.detail;return"object"==typeof t&&"data"in t?t.data:null}function ko(e){return"ko"===e.locale}var Ao=!1,Io={color:!0,date:!0,datetime:!0,"datetime-local":!0,email:!0,month:!0,number:!0,password:!0,range:!0,search:!0,tel:!0,text:!0,time:!0,url:!0,week:!0};function Lo(e){var t=e&&e.nodeName&&e.nodeName.toLowerCase();return"input"===t?!!Io[e.type]:"textarea"===t}function _o(e,t,n,r){kn(r);var a=wi(t,"onChange");if(a.length>0){var o=new Qa("onChange","change",null,n,r);e.push({event:o,listeners:a})}}var Po=null,Mo=null;function Do(e){mi(e,0)}function Uo(e){if(it(jl(e)))return e}function jo(e,t){if("change"===e)return t}var Fo=!1;function zo(){Po&&(Po.detachEvent("onpropertychange",Bo),Po=null,Mo=null)}function Bo(e){"value"===e.propertyName&&Uo(Mo)&&function(e){var t=[];_o(t,Mo,e,Nn(e)),function(e,t){if(Mn)return e(t);Mn=!0;try{In(e,t)}finally{Mn=!1,Un()}}(Do,t)}(e)}function Ho(e,t,n){"focusin"===e?(zo(),function(e,t){Mo=t,(Po=e).attachEvent("onpropertychange",Bo)}(t,n)):"focusout"===e&&zo()}function Vo(e,t){if("selectionchange"===e||"keyup"===e||"keydown"===e)return Uo(Mo)}function Wo(e,t){if("click"===e)return Uo(t)}function Go(e,t){if("input"===e||"change"===e)return Uo(t)}A&&(Fo=function(e){if(!A)return!1;var t="oninput",n=t in document;if(!n){var r=document.createElement("div");r.setAttribute(t,"return;"),n="function"==typeof r.oninput}return n}()&&(!document.documentMode||document.documentMode>9));var Yo="function"==typeof Object.is?Object.is:function(e,t){return e===t&&(0!==e||1/e==1/t)||e!=e&&t!=t},$o=Object.prototype.hasOwnProperty;function Xo(e,t){if(Yo(e,t))return!0;if("object"!=typeof e||null===e||"object"!=typeof t||null===t)return!1;var n=Object.keys(e),r=Object.keys(t);if(n.length!==r.length)return!1;for(var a=0;a<n.length;a++)if(!$o.call(t,n[a])||!Yo(e[n[a]],t[n[a]]))return!1;return!0}function qo(e){for(;e&&e.firstChild;)e=e.firstChild;return e}function Ko(e){for(;e;){if(e.nextSibling)return e.nextSibling;e=e.parentNode}}function Jo(e,t){for(var n=qo(e),r=0,a=0;n;){if(3===n.nodeType){if(a=r+n.textContent.length,r<=t&&a>=t)return{node:n,offset:t-r};r=a}n=qo(Ko(n))}}function Qo(e){return e&&3===e.nodeType}function Zo(e,t){return!(!e||!t)&&(e===t||!Qo(e)&&(Qo(t)?Zo(e,t.parentNode):"contains"in e?e.contains(t):!!e.compareDocumentPosition&&!!(16&e.compareDocumentPosition(t))))}function ei(e){return e&&e.ownerDocument&&Zo(e.ownerDocument.documentElement,e)}function ti(e){try{return"string"==typeof e.contentWindow.location.href}catch(e){return!1}}function ni(){for(var e=window,t=lt();t instanceof e.HTMLIFrameElement;){if(!ti(t))return t;t=lt((e=t.contentWindow).document)}return t}function ri(e){var t=e&&e.nodeName&&e.nodeName.toLowerCase();return t&&("input"===t&&("text"===e.type||"search"===e.type||"tel"===e.type||"url"===e.type||"password"===e.type)||"textarea"===t||"true"===e.contentEditable)}var ai=A&&"documentMode"in document&&document.documentMode<=11,oi=null,ii=null,li=null,si=!1;function ci(e,t,n){var r,a=(r=n).window===r?r.document:9===r.nodeType?r:r.ownerDocument;if(!si&&null!=oi&&oi===lt(a)){var o=function(e){if("selectionStart"in e&&ri(e))return{start:e.selectionStart,end:e.selectionEnd};var t=(e.ownerDocument&&e.ownerDocument.defaultView||window).getSelection();return{anchorNode:t.anchorNode,anchorOffset:t.anchorOffset,focusNode:t.focusNode,focusOffset:t.focusOffset}}(oi);if(!li||!Xo(li,o)){li=o;var i=wi(ii,"onSelect");if(i.length>0){var l=new Qa("onSelect","select",null,t,n);e.push({event:l,listeners:i}),l.target=oi}}}}Kr(["cancel","cancel","click","click","close","close","contextmenu","contextMenu","copy","copy","cut","cut","auxclick","auxClick","dblclick","doubleClick","dragend","dragEnd","dragstart","dragStart","drop","drop","focusin","focus","focusout","blur","input","input","invalid","invalid","keydown","keyDown","keypress","keyPress","keyup","keyUp","mousedown","mouseDown","mouseup","mouseUp","paste","paste","pause","pause","play","play","pointercancel","pointerCancel","pointerdown","pointerDown","pointerup","pointerUp","ratechange","rateChange","reset","reset","seeked","seeked","submit","submit","touchcancel","touchCancel","touchend","touchEnd","touchstart","touchStart","volumechange","volumeChange"],0),Kr(["drag","drag","dragenter","dragEnter","dragexit","dragExit","dragleave","dragLeave","dragover","dragOver","mousemove","mouseMove","mouseout","mouseOut","mouseover","mouseOver","pointermove","pointerMove","pointerout","pointerOut","pointerover","pointerOver","scroll","scroll","toggle","toggle","touchmove","touchMove","wheel","wheel"],1),Kr(qr,2),function(e,t){for(var n=0;n<e.length;n++)Xr.set(e[n],0)}(["change","selectionchange","textInput","compositionstart","compositionend","compositionupdate"]),k("onMouseEnter",["mouseout","mouseover"]),k("onMouseLeave",["mouseout","mouseover"]),k("onPointerEnter",["pointerout","pointerover"]),k("onPointerLeave",["pointerout","pointerover"]),x("onChange",["change","click","focusin","focusout","input","keydown","keyup","selectionchange"]),x("onSelect",["focusout","contextmenu","dragend","focusin","keydown","keyup","mousedown","mouseup","selectionchange"]),x("onBeforeInput",["compositionend","keypress","textInput","paste"]),x("onCompositionEnd",["compositionend","focusout","keydown","keypress","keyup","mousedown"]),x("onCompositionStart",["compositionstart","focusout","keydown","keypress","keyup","mousedown"]),x("onCompositionUpdate",["compositionupdate","focusout","keydown","keypress","keyup","mousedown"]);var ui=["abort","canplay","canplaythrough","durationchange","emptied","encrypted","ended","error","loadeddata","loadedmetadata","loadstart","pause","play","playing","progress","ratechange","seeked","seeking","stalled","suspend","timeupdate","volumechange","waiting"],fi=new Set(["cancel","close","invalid","load","scroll","toggle"].concat(ui));function di(e,t,n){var r=e.type||"unknown-event";e.currentTarget=n,function(e,t,n,r,a,o,i,l,s){if(Kn.apply(this,arguments),Gn){var c=Qn();$n||($n=!0,Xn=c)}}(r,t,void 0,e),e.currentTarget=null}function pi(e,t,n){var r;if(n)for(var a=t.length-1;a>=0;a--){var o=t[a],i=o.instance,l=o.currentTarget,s=o.listener;if(i!==r&&e.isPropagationStopped())return;di(e,s,l),r=i}else for(var c=0;c<t.length;c++){var u=t[c],f=u.instance,d=u.currentTarget,p=u.listener;if(f!==r&&e.isPropagationStopped())return;di(e,p,d),r=f}}function mi(e,t){for(var n=0!=(4&t),r=0;r<e.length;r++){var a=e[r];pi(a.event,a.listeners,n)}!function(){if($n){var e=Xn;throw $n=!1,Xn=null,e}}()}function hi(e,t){var n=Bl(t),r=Oi(e,!1);n.has(r)||(bi(t,e,2,!1),n.add(r))}var gi="_reactListening"+Math.random().toString(36).slice(2);function yi(e){e[gi]||(e[gi]=!0,R.forEach(function(t){fi.has(t)||vi(t,!1,e,null),vi(t,!0,e,null)}))}function vi(e,t,n,r){var a=arguments.length>4&&void 0!==arguments[4]?arguments[4]:0,o=n;if("selectionchange"===e&&9!==n.nodeType&&(o=n.ownerDocument),null!==r&&!t&&fi.has(e)){if("scroll"!==e)return;a|=2,o=r}var i=Bl(o),l=Oi(e,t);i.has(l)||(t&&(a|=4),bi(o,e,a,t),i.add(l))}function bi(e,t,n,r,a){var o=function(e,t,n){var r;switch(function(e){var t=Xr.get(e);return void 0===t?2:t}(t)){case 0:r=Ma;break;case 1:r=Da;break;case 2:default:r=Ua}return r.bind(null,t,n,e)}(e,t,n),i=void 0;Fn&&("touchstart"!==t&&"touchmove"!==t&&"wheel"!==t||(i=!0)),e=e,r?void 0!==i?function(e,t,n,r){e.addEventListener(t,n,{capture:!0,passive:r})}(e,t,o,i):function(e,t,n){e.addEventListener(t,n,!0)}(e,t,o):void 0!==i?function(e,t,n,r){e.addEventListener(t,n,{passive:r})}(e,t,o,i):function(e,t,n){e.addEventListener(t,n,!1)}(e,t,o)}function Ei(e,t){return e===t||8===e.nodeType&&e.parentNode===t}function Ti(e,t,n,r,a){var o=r;if(0==(1&t)&&0==(2&t)){var i=a;if(null!==r){var l=r;e:for(;;){if(null===l)return;var s=l.tag;if(3===s||4===s){var c=l.stateNode.containerInfo;if(Ei(c,i))break;if(4===s)for(var u=l.return;null!==u;){var f=u.tag;if((3===f||4===f)&&Ei(u.stateNode.containerInfo,i))return;u=u.return}for(;null!==c;){var d=Dl(c);if(null===d)return;var p=d.tag;if(5===p||6===p){l=o=d;continue e}c=c.parentNode}}l=l.return}}}!function(e,t,n){if(Dn)return e();Dn=!0;try{Pn(e,void 0,void 0)}finally{Dn=!1,Un()}}(function(){return function(e,t,n,r,a){var o=[];(function(e,t,n,r,a,o,i){(function(e,t,n,r,a,o,i){var l=$r.get(t);if(void 0!==l){var s=Qa,c=t;switch(t){case"keypress":if(0===Wa(r))return;case"keydown":case"keyup":s=ho;break;case"focusin":c="focus",s=ao;break;case"focusout":c="blur",s=ao;break;case"beforeblur":case"afterblur":s=ao;break;case"click":if(2===r.button)return;case"auxclick":case"dblclick":case"mousedown":case"mousemove":case"mouseup":case"mouseout":case"mouseover":case"contextmenu":s=no;break;case"drag":case"dragend":case"dragenter":case"dragexit":case"dragleave":case"dragover":case"dragstart":case"drop":s=ro;break;case"touchcancel":case"touchend":case"touchmove":case"touchstart":s=yo;break;case Vr:case Wr:case Gr:s=oo;break;case Yr:s=vo;break;case"scroll":s=eo;break;case"wheel":s=bo;break;case"copy":case"cut":case"paste":s=io;break;case"gotpointercapture":case"lostpointercapture":case"pointercancel":case"pointerdown":case"pointermove":case"pointerout":case"pointerover":case"pointerup":s=go}var u=0!=(4&o),f=function(e,t,n,r,a){for(var o=r?null!==t?t+"Capture":null:t,i=[],l=e,s=null;null!==l;){var c=l.stateNode;if(5===l.tag&&null!==c&&(s=c,null!==o)){var u=jn(l,o);null!=u&&i.push(Si(l,u,s))}if(a)break;l=l.return}return i}(n,l,0,u,!u&&"scroll"===t);if(f.length>0){var d=new s(l,c,null,r,a);e.push({event:d,listeners:f})}}})(e,t,n,r,a,o),0==(7&o)&&(function(e,t,n,r,a,o,i){var l="mouseover"===t||"pointerover"===t,s="mouseout"===t||"pointerout"===t;if(l&&0==(16&o)){var c=r.relatedTarget||r.fromElement;if(c&&(Dl(c)||Ml(c)))return}if(s||l){var u,f,d;if(a.window===a)u=a;else{var p=a.ownerDocument;u=p?p.defaultView||p.parentWindow:window}if(s){var m=r.relatedTarget||r.toElement;f=n,null!==(d=m?Dl(m):null)&&(d!==dr(d)||5!==d.tag&&6!==d.tag)&&(d=null)}else f=null,d=n;if(f!==d){var h=no,g="onMouseLeave",y="onMouseEnter",v="mouse";"pointerout"!==t&&"pointerover"!==t||(h=go,g="onPointerLeave",y="onPointerEnter",v="pointer");var b=null==f?u:jl(f),E=null==d?u:jl(d),T=new h(g,v+"leave",f,r,a);T.target=b,T.relatedTarget=E;var S=null;if(Dl(a)===n){var w=new h(y,v+"enter",d,r,a);w.target=E,w.relatedTarget=b,S=w}!function(e,t,n,r,a){var o=r&&a?function(e,t){for(var n=e,r=t,a=0,o=n;o;o=Ni(o))a++;for(var i=0,l=r;l;l=Ni(l))i++;for(;a-i>0;)n=Ni(n),a--;for(;i-a>0;)r=Ni(r),i--;for(var s=a;s--;){if(n===r||null!==r&&n===r.alternate)return n;n=Ni(n),r=Ni(r)}return null}(r,a):null;null!==r&&Ri(e,t,r,o,!1),null!==a&&null!==n&&Ri(e,n,a,o,!0)}(e,T,S,f,d)}}}(e,t,n,r,a,o),function(e,t,n,r,a,o,i){var l,s,c,u,f=n?jl(n):window;if("select"===(u=(c=f).nodeName&&c.nodeName.toLowerCase())||"input"===u&&"file"===c.type?l=jo:Lo(f)?Fo?l=Go:(l=Vo,s=Ho):function(e){var t=e.nodeName;return t&&"input"===t.toLowerCase()&&("checkbox"===e.type||"radio"===e.type)}(f)&&(l=Wo),l){var d=l(t,n);if(d)return void _o(e,d,r,a)}s&&s(t,f,n),"focusout"===t&&function(e){var t=e._wrapperState;t&&t.controlled&&"number"===e.type&&vt(e,"number",e.value)}(f)}(e,t,n,r,a),function(e,t,n,r,a,o,i){var l=n?jl(n):window;switch(t){case"focusin":(Lo(l)||"true"===l.contentEditable)&&(oi=l,ii=n,li=null);break;case"focusout":oi=null,ii=null,li=null;break;case"mousedown":si=!0;break;case"contextmenu":case"mouseup":case"dragend":si=!1,ci(e,r,a);break;case"selectionchange":if(ai)break;case"keydown":case"keyup":ci(e,r,a)}}(e,t,n,r,a),function(e,t,n,r,a,o,i){(function(e,t,n,r,a){var o,i;if(To?o=function(e){switch(e){case"compositionstart":return"onCompositionStart";case"compositionend":return"onCompositionEnd";case"compositionupdate":return"onCompositionUpdate"}}(t):Ao?Co(t,r)&&(o="onCompositionEnd"):function(e,t){return"keydown"===e&&229===t.keyCode}(t,r)&&(o="onCompositionStart"),!o)return null;No&&!ko(r)&&(Ao||"onCompositionStart"!==o?"onCompositionEnd"===o&&Ao&&(i=Ha()):Ao=function(e){return Fa=e,za=Va(),!0}(a));var l=wi(n,o);if(l.length>0){var s=new lo(o,t,null,r,a);if(e.push({event:s,listeners:l}),i)s.data=i;else{var c=xo(r);null!==c&&(s.data=c)}}})(e,t,n,r,a),function(e,t,n,r,a){var o;if(!(o=wo?function(e,t){switch(e){case"compositionend":return xo(t);case"keypress":return 32!==t.which?null:(Oo=!0,Ro);case"textInput":var n=t.data;return n===Ro&&Oo?null:n;default:return null}}(t,r):function(e,t){if(Ao){if("compositionend"===e||!To&&Co(e,t)){var n=Ha();return Fa=null,za=null,Ba=null,Ao=!1,n}return null}switch(e){case"paste":return null;case"keypress":if(!function(e){return(e.ctrlKey||e.altKey||e.metaKey)&&!(e.ctrlKey&&e.altKey)}(t)){if(t.char&&t.char.length>1)return t.char;if(t.which)return String.fromCharCode(t.which)}return null;case"compositionend":return No&&!ko(t)?null:t.data;default:return null}}(t,r)))return null;var i=wi(n,"onBeforeInput");if(i.length>0){var l=new so("onBeforeInput","beforeinput",null,r,a);e.push({event:l,listeners:i}),l.data=o}}(e,t,n,r,a)}(e,t,n,r,a))})(o,e,r,n,Nn(n),t),mi(o,t)}(e,t,n,o)})}function Si(e,t,n){return{instance:e,listener:t,currentTarget:n}}function wi(e,t){for(var n=t+"Capture",r=[],a=e;null!==a;){var o=a.stateNode;if(5===a.tag&&null!==o){var i=o,l=jn(a,n);null!=l&&r.unshift(Si(a,l,i));var s=jn(a,t);null!=s&&r.push(Si(a,s,i))}a=a.return}return r}function Ni(e){if(null===e)return null;do{e=e.return}while(e&&5!==e.tag);return e||null}function Ri(e,t,n,r,a){for(var o=t._reactName,i=[],l=n;null!==l&&l!==r;){var s=l.alternate,c=l.stateNode,u=l.tag;if(null!==s&&s===r)break;if(5===u&&null!==c){var f=c;if(a){var d=jn(l,o);null!=d&&i.unshift(Si(l,d,f))}else if(!a){var p=jn(l,o);null!=p&&i.push(Si(l,p,f))}}l=l.return}0!==i.length&&e.push({event:t,listeners:i})}function Oi(e,t){return e+"__"+(t?"capture":"bubble")}var Ci,xi,ki,Ai,Ii,Li,_i,Pi,Mi,Di,Ui=!1,ji="dangerouslySetInnerHTML",Fi="suppressContentEditableWarning",zi="suppressHydrationWarning",Bi="autoFocus",Hi="children",Vi="style",Wi="http://www.w3.org/1999/xhtml";Ci={dialog:!0,webview:!0},ki=function(e,t){(function(e,t){sn(e,t)||function(e,t){var n=[];for(var r in t)hn(0,r)||n.push(r);var a=n.map(function(e){return"`"+e+"`"}).join(", ");1===n.length?s("Invalid aria prop %s on <%s> tag. For details, see https://reactjs.org/link/invalid-aria-props",a,e):n.length>1&&s("Invalid aria props %s on <%s> tag. For details, see https://reactjs.org/link/invalid-aria-props",a,e)}(e,t)})(e,t),function(e,t){"input"!==e&&"textarea"!==e&&"select"!==e||null==t||null!==t.value||yn||(yn=!0,s("select"===e&&t.multiple?"`value` prop on `%s` should not be null. Consider using an empty array when `multiple` is set to `true` to clear the component or `undefined` for uncontrolled components.":"`value` prop on `%s` should not be null. Consider using an empty string to clear the component or `undefined` for uncontrolled components.",e))}(e,t),function(e,t,n){sn(e,t)||function(e,t,n){var r=[];for(var a in t)gn(0,a,t[a],n)||r.push(a);var o=r.map(function(e){return"`"+e+"`"}).join(", ");1===r.length?s("Invalid value for prop %s on <%s> tag. Either remove it from the element, or pass a string or number value to keep it in the DOM. For details, see https://reactjs.org/link/attribute-behavior ",o,e):r.length>1&&s("Invalid values for props %s on <%s> tag. Either remove them from the element, or pass a string or number value to keep them in the DOM. For details, see https://reactjs.org/link/attribute-behavior ",o,e)}(e,t,n)}(e,t,{registrationNameDependencies:O,possibleRegistrationNames:C})},Pi=A&&!document.documentMode;var Gi=/\r\n?/g,Yi=/\u0000|\uFFFD/g;function $i(e){return 9===e.nodeType?e:e.ownerDocument}function Xi(){}function qi(e){e.onclick=Xi}function Ki(e,t){Ai(e.nodeValue,t)}function Ji(e,t){Ui||(Ui=!0,s("Did not expect server HTML to contain a <%s> in <%s>.",t.nodeName.toLowerCase(),e.nodeName.toLowerCase()))}function Qi(e,t){Ui||(Ui=!0,s('Did not expect server HTML to contain the text node "%s" in <%s>.',t.nodeValue,e.nodeName.toLowerCase()))}function Zi(e,t,n){Ui||(Ui=!0,s("Expected server HTML to contain a matching <%s> in <%s>.",t,e.nodeName.toLowerCase()))}function el(e,t){""!==t&&(Ui||(Ui=!0,s('Expected server HTML to contain a matching text node for "%s" in <%s>.',t,e.nodeName.toLowerCase())))}Mi=function(e){return("string"==typeof e?e:""+e).replace(Gi,"\n").replace(Yi,"")},Ai=function(e,t){if(!Ui){var n=Mi(t),r=Mi(e);r!==n&&(Ui=!0,s('Text content did not match. Server: "%s" Client: "%s"',r,n))}},Ii=function(e,t,n){if(!Ui){var r=Mi(n),a=Mi(t);a!==r&&(Ui=!0,s("Prop `%s` did not match. Server: %s Client: %s",e,JSON.stringify(a),JSON.stringify(r)))}},Li=function(e){if(!Ui){Ui=!0;var t=[];e.forEach(function(e){t.push(e)}),s("Extra attributes from the server: %s",t)}},_i=function(e,t){!1===t?s("Expected `%s` listener to be a function, instead got `false`.\n\nIf you used to conditionally omit it with %s={condition && value}, pass %s={condition ? value : undefined} instead.",e,e,e):s("Expected `%s` listener to be a function, instead got a value of `%s` type.",e,typeof t)},Di=function(e,t){var n=e.namespaceURI===Wi?e.ownerDocument.createElement(e.tagName):e.ownerDocument.createElementNS(e.namespaceURI,e.tagName);return n.innerHTML=t,n.innerHTML};var tl,nl,rl=["address","applet","area","article","aside","base","basefont","bgsound","blockquote","body","br","button","caption","center","col","colgroup","dd","details","dir","div","dl","dt","embed","fieldset","figcaption","figure","footer","form","frame","frameset","h1","h2","h3","h4","h5","h6","head","header","hgroup","hr","html","iframe","img","input","isindex","li","link","listing","main","marquee","menu","menuitem","meta","nav","noembed","noframes","noscript","object","ol","p","param","plaintext","pre","script","section","select","source","style","summary","table","tbody","td","template","textarea","tfoot","th","thead","title","tr","track","ul","wbr","xmp"],al=["applet","caption","html","table","td","th","marquee","object","template","foreignObject","desc","title"],ol=al.concat(["button"]),il=["dd","dt","li","option","optgroup","p","rp","rt"],ll={current:null,formTag:null,aTagInScope:null,buttonTagInScope:null,nobrTagInScope:null,pTagInButtonScope:null,listItemTagAutoclosing:null,dlItemTagAutoclosing:null};nl=function(e,t){var r=n({},e||ll),a={tag:t};return-1!==al.indexOf(t)&&(r.aTagInScope=null,r.buttonTagInScope=null,r.nobrTagInScope=null),-1!==ol.indexOf(t)&&(r.pTagInButtonScope=null),-1!==rl.indexOf(t)&&"address"!==t&&"div"!==t&&"p"!==t&&(r.listItemTagAutoclosing=null,r.dlItemTagAutoclosing=null),r.current=a,"form"===t&&(r.formTag=a),"a"===t&&(r.aTagInScope=a),"button"===t&&(r.buttonTagInScope=a),"nobr"===t&&(r.nobrTagInScope=a),"p"===t&&(r.pTagInButtonScope=a),"li"===t&&(r.listItemTagAutoclosing=a),"dd"!==t&&"dt"!==t||(r.dlItemTagAutoclosing=a),r};var sl={};tl=function(e,t,n){var r=(n=n||ll).current,a=r&&r.tag;null!=t&&(null!=e&&s("validateDOMNesting: when childText is passed, childTag should be null"),e="#text");var o=function(e,t){switch(t){case"select":return"option"===e||"optgroup"===e||"#text"===e;case"optgroup":return"option"===e||"#text"===e;case"option":return"#text"===e;case"tr":return"th"===e||"td"===e||"style"===e||"script"===e||"template"===e;case"tbody":case"thead":case"tfoot":return"tr"===e||"style"===e||"script"===e||"template"===e;case"colgroup":return"col"===e||"template"===e;case"table":return"caption"===e||"colgroup"===e||"tbody"===e||"tfoot"===e||"thead"===e||"style"===e||"script"===e||"template"===e;case"head":return"base"===e||"basefont"===e||"bgsound"===e||"link"===e||"meta"===e||"title"===e||"noscript"===e||"noframes"===e||"style"===e||"script"===e||"template"===e;case"html":return"head"===e||"body"===e||"frameset"===e;case"frameset":return"frame"===e;case"#document":return"html"===e}switch(e){case"h1":case"h2":case"h3":case"h4":case"h5":case"h6":return"h1"!==t&&"h2"!==t&&"h3"!==t&&"h4"!==t&&"h5"!==t&&"h6"!==t;case"rp":case"rt":return-1===il.indexOf(t);case"body":case"caption":case"col":case"colgroup":case"frameset":case"frame":case"head":case"html":case"tbody":case"td":case"tfoot":case"th":case"thead":case"tr":return null==t}return!0}(e,a)?null:r,i=o?null:function(e,t){switch(e){case"address":case"article":case"aside":case"blockquote":case"center":case"details":case"dialog":case"dir":case"div":case"dl":case"fieldset":case"figcaption":case"figure":case"footer":case"header":case"hgroup":case"main":case"menu":case"nav":case"ol":case"p":case"section":case"summary":case"ul":case"pre":case"listing":case"table":case"hr":case"xmp":case"h1":case"h2":case"h3":case"h4":case"h5":case"h6":return t.pTagInButtonScope;case"form":return t.formTag||t.pTagInButtonScope;case"li":return t.listItemTagAutoclosing;case"dd":case"dt":return t.dlItemTagAutoclosing;case"button":return t.buttonTagInScope;case"a":return t.aTagInScope;case"nobr":return t.nobrTagInScope}return null}(e,n),l=o||i;if(l){var c=l.tag,u=!!o+"|"+e+"|"+c;if(!sl[u]){sl[u]=!0;var f=e,d="";if("#text"===e?/\S/.test(t)?f="Text nodes":(f="Whitespace text nodes",d=" Make sure you don't have any extra whitespace between tags on each line of your source code."):f="<"+e+">",o){var p="";"table"===c&&"tr"===e&&(p+=" Add a <tbody>, <thead> or <tfoot> to your code to match the DOM tree generated by the browser."),s("validateDOMNesting(...): %s cannot appear as a child of <%s>.%s%s",f,c,d,p)}else s("validateDOMNesting(...): %s cannot appear as a descendant of <%s>.",f,c)}}};var cl="$?",ul="$!",fl=null,dl=null;function pl(e,t){switch(e){case"button":case"input":case"select":case"textarea":return!!t.autoFocus}return!1}function ml(e,t){return"textarea"===e||"option"===e||"noscript"===e||"string"==typeof t.children||"number"==typeof t.children||"object"==typeof t.dangerouslySetInnerHTML&&null!==t.dangerouslySetInnerHTML&&null!=t.dangerouslySetInnerHTML.__html}var hl="function"==typeof setTimeout?setTimeout:void 0,gl="function"==typeof clearTimeout?clearTimeout:void 0;function yl(e){Bt(e,"")}function vl(e,t){e.removeChild(t)}function bl(e){var t=(e=e).style;"function"==typeof t.setProperty?t.setProperty("display","none","important"):t.display="none"}function El(e,t){e=e;var n=t.style,r=null!=n&&n.hasOwnProperty("display")?n.display:null;e.style.display=Gt("display",r)}function Tl(e){if(1===e.nodeType)e.textContent="";else if(9===e.nodeType){var t=e.body;null!=t&&(t.textContent="")}}function Sl(e){for(;null!=e;e=e.nextSibling){var t=e.nodeType;if(1===t||3===t)break}return e}function wl(e){return Sl(e.nextSibling)}function Nl(e){return Sl(e.firstChild)}function Rl(e){for(var t=e.previousSibling,n=0;t;){if(8===t.nodeType){var r=t.data;if("$"===r||r===ul||r===cl){if(0===n)return t;n--}else"/$"===r&&n++}t=t.previousSibling}return null}var Ol=0;function Cl(e){var t="r:"+(Ol++).toString(36);return{toString:function(){return e(),t},valueOf:function(){return e(),t}}}var xl=Math.random().toString(36).slice(2),kl="__reactFiber$"+xl,Al="__reactProps$"+xl,Il="__reactContainer$"+xl,Ll="__reactEvents$"+xl;function _l(e,t){t[kl]=e}function Pl(e){e[Il]=null}function Ml(e){return!!e[Il]}function Dl(e){var t=e[kl];if(t)return t;for(var n=e.parentNode;n;){if(t=n[Il]||n[kl]){var r=t.alternate;if(null!==t.child||null!==r&&null!==r.child)for(var a=Rl(e);null!==a;){var o=a[kl];if(o)return o;a=Rl(a)}return t}n=(e=n).parentNode}return null}function Ul(e){var t=e[kl]||e[Il];return!t||5!==t.tag&&6!==t.tag&&t.tag!==h&&3!==t.tag?null:t}function jl(e){if(5===e.tag||6===e.tag)return e.stateNode;throw Error("getNodeFromInstance: Invalid argument.")}function Fl(e){return e[Al]||null}function zl(e,t){e[Al]=t}function Bl(e){var t=e[Ll];return void 0===t&&(t=e[Ll]=new Set),t}var Hl={},Vl=o.ReactDebugCurrentFrame;function Wl(e){if(e){var t=e._owner,n=ze(e.type,e._source,t?t.type:null);Vl.setExtraStackFrame(n)}else Vl.setExtraStackFrame(null)}function Gl(e,t,n,r,a){var o=Function.call.bind(Object.prototype.hasOwnProperty);for(var i in e)if(o(e,i)){var l=void 0;try{if("function"!=typeof e[i]){var c=Error((r||"React class")+": "+n+" type `"+i+"` is invalid; it must be a function, usually from the `prop-types` package, but received `"+typeof e[i]+"`.This often happens because of typos such as `PropTypes.function` instead of `PropTypes.func`.");throw c.name="Invariant Violation",c}l=e[i](t,i,r,n,null,"SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED")}catch(e){l=e}!l||l instanceof Error||(Wl(a),s("%s: type specification of %s `%s` is invalid; the type checker function must return `null` or an `Error` but returned a %s. You may have forgotten to pass an argument to the type checker creator (arrayOf, instanceOf, objectOf, oneOf, oneOfType, and shape all require an argument).",r||"React class",n,i,typeof l),Wl(null)),l instanceof Error&&!(l.message in Hl)&&(Hl[l.message]=!0,Wl(a),s("Failed %s type: %s",n,l.message),Wl(null))}}var Yl,$l=[];Yl=[];var Xl,ql=-1;function Kl(e){return{current:e}}function Jl(e,t){ql<0?s("Unexpected pop."):(t!==Yl[ql]&&s("Unexpected Fiber popped."),e.current=$l[ql],$l[ql]=null,Yl[ql]=null,ql--)}function Ql(e,t,n){ql++,$l[ql]=e.current,Yl[ql]=n,e.current=t}Xl={};var Zl={};Object.freeze(Zl);var es=Kl(Zl),ts=Kl(!1),ns=Zl;function rs(e,t,n){return n&&ls(t)?ns:es.current}function as(e,t,n){var r=e.stateNode;r.__reactInternalMemoizedUnmaskedChildContext=t,r.__reactInternalMemoizedMaskedChildContext=n}function os(e,t){var n=e.type,r=n.contextTypes;if(!r)return Zl;var a=e.stateNode;if(a&&a.__reactInternalMemoizedUnmaskedChildContext===t)return a.__reactInternalMemoizedMaskedChildContext;var o={};for(var i in r)o[i]=t[i];return Gl(r,o,"context",We(n)||"Unknown"),a&&as(e,t,o),o}function is(){return ts.current}function ls(e){return null!=e.childContextTypes}function ss(e){Jl(ts,e),Jl(es,e)}function cs(e){Jl(ts,e),Jl(es,e)}function us(e,t,n){if(es.current!==Zl)throw Error("Unexpected context found on stack. This error is likely caused by a bug in React. Please file an issue.");Ql(es,t,e),Ql(ts,n,e)}function fs(e,t,r){var a=e.stateNode,o=t.childContextTypes;if("function"!=typeof a.getChildContext){var i=We(t)||"Unknown";return Xl[i]||(Xl[i]=!0,s("%s.childContextTypes is specified but there is no getChildContext() method on the instance. You can either define getChildContext() on %s or remove childContextTypes from it.",i,i)),r}var l=a.getChildContext();for(var c in l)if(!(c in o))throw Error((We(t)||"Unknown")+'.getChildContext(): key "'+c+'" is not defined in childContextTypes.');return Gl(o,l,"child context",We(t)||"Unknown"),n({},r,l)}function ds(e){var t=e.stateNode;return ns=es.current,Ql(es,t&&t.__reactInternalMemoizedMergedChildContext||Zl,e),Ql(ts,ts.current,e),!0}function ps(e,t,n){var r=e.stateNode;if(!r)throw Error("Expected to have an instance by this point. This error is likely caused by a bug in React. Please file an issue.");if(n){var a=fs(e,t,ns);r.__reactInternalMemoizedMergedChildContext=a,Jl(ts,e),Jl(es,e),Ql(es,a,e),Ql(ts,n,e)}else Jl(ts,e),Ql(ts,n,e)}var ms=null,hs=null,gs=!1,ys="undefined"!=typeof __REACT_DEVTOOLS_GLOBAL_HOOK__;function vs(e,t){if(hs&&"function"==typeof hs.onCommitFiberRoot)try{hs.onCommitFiberRoot(ms,e,t,(e.current.flags&ar)===ar)}catch(e){gs||(gs=!0,s("React instrumentation encountered an error: %s",e))}}var bs=r.unstable_runWithPriority,Es=r.unstable_scheduleCallback,Ts=r.unstable_cancelCallback,Ss=r.unstable_shouldYield,ws=r.unstable_requestPaint,Ns=r.unstable_now,Rs=r.unstable_getCurrentPriorityLevel,Os=r.unstable_ImmediatePriority,Cs=r.unstable_UserBlockingPriority,xs=r.unstable_NormalPriority,ks=r.unstable_LowPriority,As=r.unstable_IdlePriority;if(null==a.__interactionsRef||null==a.__interactionsRef.current)throw Error("It is not supported to run the profiling version of a renderer (for example, `react-dom/profiling`) without also replacing the `scheduler/tracing` module with `scheduler/tracing-profiling`. Your bundler might have a setting for aliasing both modules. Learn more at https://reactjs.org/link/profiling");var Is={},Ls=99,_s=98,Ps=97,Ms=Ss,Ds=void 0!==ws?ws:function(){},Us=null,js=null,Fs=!1,zs=Ns(),Bs=zs<1e4?Ns:function(){return Ns()-zs};function Hs(){switch(Rs()){case Os:return Ls;case Cs:return _s;case xs:return Ps;case ks:return 96;case As:return 95;default:throw Error("Unknown priority level.")}}function Vs(e){switch(e){case Ls:return Os;case _s:return Cs;case Ps:return xs;case 96:return ks;case 95:return As;default:throw Error("Unknown priority level.")}}function Ws(e,t){var n=Vs(e);return bs(n,t)}function Gs(e,t,n){var r=Vs(e);return Es(r,t,n)}function Ys(e){e!==Is&&Ts(e)}function $s(){if(null!==js){var e=js;js=null,Ts(e)}Xs()}function Xs(){if(!Fs&&null!==Us){Fs=!0;var e=0;try{var t=Us;Ws(Ls,function(){for(;e<t.length;e++){var n=t[e];do{n=n(!0)}while(null!==n)}}),Us=null}catch(t){throw null!==Us&&(Us=Us.slice(e+1)),Es(Os,$s),t}finally{Fs=!1}}}var qs="17.0.2",Ks=o.ReactCurrentBatchConfig,Js={recordUnsafeLifecycleWarnings:function(e,t){},flushPendingUnsafeLifecycleWarnings:function(){},recordLegacyContextWarning:function(e,t){},flushLegacyContextWarning:function(){},discardPendingWarnings:function(){}},Qs=function(e){var t=[];return e.forEach(function(e){t.push(e)}),t.sort().join(", ")},Zs=[],ec=[],tc=[],nc=[],rc=[],ac=[],oc=new Set;Js.recordUnsafeLifecycleWarnings=function(e,t){oc.has(e.type)||("function"==typeof t.componentWillMount&&!0!==t.componentWillMount.__suppressDeprecationWarning&&Zs.push(e),1&e.mode&&"function"==typeof t.UNSAFE_componentWillMount&&ec.push(e),"function"==typeof t.componentWillReceiveProps&&!0!==t.componentWillReceiveProps.__suppressDeprecationWarning&&tc.push(e),1&e.mode&&"function"==typeof t.UNSAFE_componentWillReceiveProps&&nc.push(e),"function"==typeof t.componentWillUpdate&&!0!==t.componentWillUpdate.__suppressDeprecationWarning&&rc.push(e),1&e.mode&&"function"==typeof t.UNSAFE_componentWillUpdate&&ac.push(e))},Js.flushPendingUnsafeLifecycleWarnings=function(){var e=new Set;Zs.length>0&&(Zs.forEach(function(t){e.add(We(t.type)||"Component"),oc.add(t.type)}),Zs=[]);var t=new Set;ec.length>0&&(ec.forEach(function(e){t.add(We(e.type)||"Component"),oc.add(e.type)}),ec=[]);var n=new Set;tc.length>0&&(tc.forEach(function(e){n.add(We(e.type)||"Component"),oc.add(e.type)}),tc=[]);var r=new Set;nc.length>0&&(nc.forEach(function(e){r.add(We(e.type)||"Component"),oc.add(e.type)}),nc=[]);var a=new Set;rc.length>0&&(rc.forEach(function(e){a.add(We(e.type)||"Component"),oc.add(e.type)}),rc=[]);var o=new Set;ac.length>0&&(ac.forEach(function(e){o.add(We(e.type)||"Component"),oc.add(e.type)}),ac=[]),t.size>0&&s("Using UNSAFE_componentWillMount in strict mode is not recommended and may indicate bugs in your code. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move code with side effects to componentDidMount, and set initial state in the constructor.\n\nPlease update the following components: %s",Qs(t)),r.size>0&&s("Using UNSAFE_componentWillReceiveProps in strict mode is not recommended and may indicate bugs in your code. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move data fetching code or side effects to componentDidUpdate.\n* If you're updating state whenever props change, refactor your code to use memoization techniques or move it to static getDerivedStateFromProps. Learn more at: https://reactjs.org/link/derived-state\n\nPlease update the following components: %s",Qs(r)),o.size>0&&s("Using UNSAFE_componentWillUpdate in strict mode is not recommended and may indicate bugs in your code. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move data fetching code or side effects to componentDidUpdate.\n\nPlease update the following components: %s",Qs(o)),e.size>0&&i("componentWillMount has been renamed, and is not recommended for use. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move code with side effects to componentDidMount, and set initial state in the constructor.\n* Rename componentWillMount to UNSAFE_componentWillMount to suppress this warning in non-strict mode. In React 18.x, only the UNSAFE_ name will work. To rename all deprecated lifecycles to their new names, you can run `npx react-codemod rename-unsafe-lifecycles` in your project source folder.\n\nPlease update the following components: %s",Qs(e)),n.size>0&&i("componentWillReceiveProps has been renamed, and is not recommended for use. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move data fetching code or side effects to componentDidUpdate.\n* If you're updating state whenever props change, refactor your code to use memoization techniques or move it to static getDerivedStateFromProps. Learn more at: https://reactjs.org/link/derived-state\n* Rename componentWillReceiveProps to UNSAFE_componentWillReceiveProps to suppress this warning in non-strict mode. In React 18.x, only the UNSAFE_ name will work. To rename all deprecated lifecycles to their new names, you can run `npx react-codemod rename-unsafe-lifecycles` in your project source folder.\n\nPlease update the following components: %s",Qs(n)),a.size>0&&i("componentWillUpdate has been renamed, and is not recommended for use. See https://reactjs.org/link/unsafe-component-lifecycles for details.\n\n* Move data fetching code or side effects to componentDidUpdate.\n* Rename componentWillUpdate to UNSAFE_componentWillUpdate to suppress this warning in non-strict mode. In React 18.x, only the UNSAFE_ name will work. To rename all deprecated lifecycles to their new names, you can run `npx react-codemod rename-unsafe-lifecycles` in your project source folder.\n\nPlease update the following components: %s",Qs(a))};var ic=new Map,lc=new Set;function sc(e,t){if(e&&e.defaultProps){var r=n({},t),a=e.defaultProps;for(var o in a)void 0===r[o]&&(r[o]=a[o]);return r}return t}Js.recordLegacyContextWarning=function(e,t){var n=function(e){for(var t=null,n=e;null!==n;)1&n.mode&&(t=n),n=n.return;return t}(e);if(null!==n){if(!lc.has(e.type)){var r=ic.get(n);(null!=e.type.contextTypes||null!=e.type.childContextTypes||null!==t&&"function"==typeof t.getChildContext)&&(void 0===r&&ic.set(n,r=[]),r.push(e))}}else s("Expected to find a StrictMode component in a strict mode tree. This error is likely caused by a bug in React. Please file an issue.")},Js.flushLegacyContextWarning=function(){ic.forEach(function(e,t){if(0!==e.length){var n=e[0],r=new Set;e.forEach(function(e){r.add(We(e.type)||"Component"),lc.add(e.type)});var a=Qs(r);try{Je(n),s("Legacy context API has been detected within a strict-mode tree.\n\nThe old API will be supported in all 16.x releases, but applications using it should migrate to the new version.\n\nPlease update the following components: %s\n\nLearn more about this warning here: https://reactjs.org/link/legacy-context",a)}finally{Ke()}}})},Js.discardPendingWarnings=function(){Zs=[],ec=[],tc=[],nc=[],rc=[],ac=[],ic=new Map};var cc,uc=1073741823,fc=Kl(null);cc={};var dc=null,pc=null,mc=null,hc=!1;function gc(){dc=null,pc=null,mc=null,hc=!1}function yc(){hc=!0}function vc(){hc=!1}function bc(e,t){var n=e.type._context;Ql(fc,n._currentValue,e),n._currentValue=t,null!=n._currentRenderer&&n._currentRenderer!==cc&&s("Detected multiple renderers concurrently rendering the same context provider. This is currently unsupported."),n._currentRenderer=cc}function Ec(e){var t=fc.current;Jl(fc,e),e.type._context._currentValue=t}function Tc(e,t){for(var n=e;null!==n;){var r=n.alternate;if(Ta(n.childLanes,t)){if(null===r||Ta(r.childLanes,t))break;r.childLanes=Sa(r.childLanes,t)}else n.childLanes=Sa(n.childLanes,t),null!==r&&(r.childLanes=Sa(r.childLanes,t));n=n.return}}function Sc(e,t){dc=e,pc=null,mc=null;var n=e.dependencies;null!==n&&null!==n.firstContext&&(Ea(n.lanes,t)&&hp(),n.firstContext=null)}function wc(e,t){if(hc&&s("Context can only be read while React is rendering. In classes, you can read it in the render method or getDerivedStateFromProps. In function components, you can read it directly in the function body, but not inside Hooks like useReducer() or useMemo()."),mc===e);else if(!1===t||0===t);else{var n;"number"!=typeof t||t===uc?(mc=e,n=uc):n=t;var r={context:e,observedBits:n,next:null};if(null===pc){if(null===dc)throw Error("Context can only be read while React is rendering. In classes, you can read it in the render method or getDerivedStateFromProps. In function components, you can read it directly in the function body, but not inside Hooks like useReducer() or useMemo().");pc=r,dc.dependencies={lanes:0,firstContext:r,responders:null}}else pc=pc.next=r}return e._currentValue}var Nc,Rc,Oc=!1;function Cc(e){e.updateQueue={baseState:e.memoizedState,firstBaseUpdate:null,lastBaseUpdate:null,shared:{pending:null},effects:null}}function xc(e,t){var n=e.updateQueue;t.updateQueue===n&&(t.updateQueue={baseState:n.baseState,firstBaseUpdate:n.firstBaseUpdate,lastBaseUpdate:n.lastBaseUpdate,shared:n.shared,effects:n.effects})}function kc(e,t){return{eventTime:e,lane:t,tag:0,payload:null,callback:null,next:null}}function Ac(e,t){var n=e.updateQueue;if(null!==n){var r=n.shared,a=r.pending;null===a?t.next=t:(t.next=a.next,a.next=t),r.pending=t,Rc!==r||Nc||(s("An update (setState, replaceState, or forceUpdate) was scheduled from inside an update function. Update functions should be pure, with zero side-effects. Consider using componentDidUpdate or a callback."),Nc=!0)}}function Ic(e,t){var n=e.updateQueue,r=e.alternate;if(null!==r){var a=r.updateQueue;if(n===a){var o=null,i=null,l=n.firstBaseUpdate;if(null!==l){var s=l;do{var c={eventTime:s.eventTime,lane:s.lane,tag:s.tag,payload:s.payload,callback:s.callback,next:null};null===i?o=i=c:(i.next=c,i=c),s=s.next}while(null!==s);null===i?o=i=t:(i.next=t,i=t)}else o=i=t;return void(e.updateQueue=n={baseState:a.baseState,firstBaseUpdate:o,lastBaseUpdate:i,shared:a.shared,effects:a.effects})}}var u=n.lastBaseUpdate;null===u?n.firstBaseUpdate=t:u.next=t,n.lastBaseUpdate=t}function Lc(e,t,r,a,o,i){switch(r.tag){case 1:var l=r.payload;if("function"==typeof l){yc();var s=l.call(i,a,o);if(1&e.mode){Ae();try{l.call(i,a,o)}finally{Ie()}}return vc(),s}return l;case 3:e.flags=-4097&e.flags|ar;case 0:var c,u=r.payload;if("function"==typeof u){if(yc(),c=u.call(i,a,o),1&e.mode){Ae();try{u.call(i,a,o)}finally{Ie()}}vc()}else c=u;return null==c?a:n({},a,c);case 2:return Oc=!0,a}return a}function _c(e,t,n,r){var a=e.updateQueue;Oc=!1,Rc=a.shared;var o=a.firstBaseUpdate,i=a.lastBaseUpdate,l=a.shared.pending;if(null!==l){a.shared.pending=null;var s=l,c=s.next;s.next=null,null===i?o=c:i.next=c,i=s;var u=e.alternate;if(null!==u){var f=u.updateQueue,d=f.lastBaseUpdate;d!==i&&(null===d?f.firstBaseUpdate=c:d.next=c,f.lastBaseUpdate=s)}}if(null!==o){for(var p=a.baseState,m=0,h=null,g=null,y=null,v=o;;){var b=v.lane,E=v.eventTime;if(Ta(r,b)){if(null!==y&&(y=y.next={eventTime:E,lane:0,tag:v.tag,payload:v.payload,callback:v.callback,next:null}),p=Lc(e,0,v,p,t,n),null!==v.callback){e.flags|=32;var T=a.effects;null===T?a.effects=[v]:T.push(v)}}else{var S={eventTime:E,lane:b,tag:v.tag,payload:v.payload,callback:v.callback,next:null};null===y?(g=y=S,h=p):y=y.next=S,m=Sa(m,b)}if(null===(v=v.next)){if(null===(l=a.shared.pending))break;var w=l,N=w.next;w.next=null,v=N,a.lastBaseUpdate=w,a.shared.pending=null}}null===y&&(h=p),a.baseState=h,a.firstBaseUpdate=g,a.lastBaseUpdate=y,ih(m),e.lanes=m,e.memoizedState=p}Rc=null}function Pc(e,t){if("function"!=typeof e)throw Error("Invalid argument passed as callback. Expected a function. Instead received: "+e);e.call(t)}function Mc(){Oc=!1}function Dc(){return Oc}function Uc(e,t,n){var r=t.effects;if(t.effects=null,null!==r)for(var a=0;a<r.length;a++){var o=r[a],i=o.callback;null!==i&&(o.callback=null,Pc(i,n))}}Nc=!1,Rc=null;var jc,Fc,zc,Bc,Hc,Vc,Wc,Gc,Yc,$c,Xc={},qc=Array.isArray,Kc=(new e.Component).refs;jc=new Set,Fc=new Set,zc=new Set,Bc=new Set,Gc=new Set,Hc=new Set,Yc=new Set,$c=new Set;var Jc=new Set;function Qc(e,t,r,a){var o=e.memoizedState;if(1&e.mode){Ae();try{r(a,o)}finally{Ie()}}var i=r(a,o);Vc(t,i);var l=null==i?o:n({},o,i);e.memoizedState=l,0===e.lanes&&(e.updateQueue.baseState=l)}Wc=function(e,t){if(null!==e&&"function"!=typeof e){var n=t+"_"+e;Jc.has(n)||(Jc.add(n),s("%s(...): Expected the last optional `callback` argument to be a function. Instead received: %s.",t,e))}},Vc=function(e,t){if(void 0===t){var n=We(e)||"Component";Hc.has(n)||(Hc.add(n),s("%s.getDerivedStateFromProps(): A valid state object (or null) must be returned. You have returned undefined.",n))}},Object.defineProperty(Xc,"_processChildContext",{enumerable:!1,value:function(){throw Error("_processChildContext is not available in React 16+. This likely means you have multiple copies of React and are attempting to nest a React 15 tree inside a React 16 tree using unstable_renderSubtreeIntoContainer, which isn't supported. Try to make sure you have only one copy of React (and ideally, switch to ReactDOM.createPortal).")}}),Object.freeze(Xc);var Zc,eu,tu,nu,ru,au,ou={isMounted:function(e){var t=fr.current;if(null!==t&&1===t.tag){var n=t.stateNode;n._warnedAboutRefsInRender||s("%s is accessing isMounted inside its render() function. render() should be a pure function of props and state. It should never access something that requires stale data from the previous render, such as refs. Move this logic to componentDidMount and componentDidUpdate instead.",We(t.type)||"A component"),n._warnedAboutRefsInRender=!0}var r=Zn(e);return!!r&&dr(r)===r},enqueueSetState:function(e,t,n){var r=Zn(e),a=Bm(),o=Hm(r),i=kc(a,o);i.payload=t,null!=n&&(Wc(n,"setState"),i.callback=n),Ac(r,i),Vm(r,o,a)},enqueueReplaceState:function(e,t,n){var r=Zn(e),a=Bm(),o=Hm(r),i=kc(a,o);i.tag=1,i.payload=t,null!=n&&(Wc(n,"replaceState"),i.callback=n),Ac(r,i),Vm(r,o,a)},enqueueForceUpdate:function(e,t){var n=Zn(e),r=Bm(),a=Hm(n),o=kc(r,a);o.tag=2,null!=t&&(Wc(t,"forceUpdate"),o.callback=t),Ac(n,o),Vm(n,a,r)}};function iu(e,t,n,r,a,o,i){var l=e.stateNode;if("function"==typeof l.shouldComponentUpdate){if(1&e.mode){Ae();try{l.shouldComponentUpdate(r,o,i)}finally{Ie()}}var c=l.shouldComponentUpdate(r,o,i);return void 0===c&&s("%s.shouldComponentUpdate(): Returned undefined instead of a boolean value. Make sure to return true or false.",We(t)||"Component"),c}return!(t.prototype&&t.prototype.isPureReactComponent&&Xo(n,r)&&Xo(a,o))}function lu(e,t){t.updater=ou,e.stateNode=t,t._reactInternals=e,t._reactInternalInstance=Xc}function su(e,t,n){var r,a=!1,o=Zl,i=Zl,l=t.contextType;if(!("contextType"in t)||null===l||void 0!==l&&l.$$typeof===oe&&void 0===l._context||$c.has(t)||($c.add(t),r=void 0===l?" However, it is set to undefined. This can be caused by a typo or by mixing up named and default imports. This can also happen due to a circular dependency, so try moving the createContext() call to a separate file.":"object"!=typeof l?" However, it is set to a "+typeof l+".":l.$$typeof===ae?" Did you accidentally pass the Context.Provider instead?":void 0!==l._context?" Did you accidentally pass the Context.Consumer instead?":" However, it is set to an object with keys {"+Object.keys(l).join(", ")+"}.",s("%s defines an invalid contextType. contextType should point to the Context object returned by React.createContext().%s",We(t)||"Component",r)),"object"==typeof l&&null!==l?i=wc(l):(o=rs(0,t,!0),i=(a=null!=t.contextTypes)?os(e,o):Zl),1&e.mode){Ae();try{new t(n,i)}finally{Ie()}}var c=new t(n,i),u=e.memoizedState=null!=c.state?c.state:null;if(lu(e,c),"function"==typeof t.getDerivedStateFromProps&&null===u){var f=We(t)||"Component";Fc.has(f)||(Fc.add(f),s("`%s` uses `getDerivedStateFromProps` but its initial state is %s. This is not recommended. Instead, define the initial state by assigning an object to `this.state` in the constructor of `%s`. This ensures that `getDerivedStateFromProps` arguments have a consistent shape.",f,null===c.state?"null":"undefined",f))}if("function"==typeof t.getDerivedStateFromProps||"function"==typeof c.getSnapshotBeforeUpdate){var d=null,p=null,m=null;if("function"==typeof c.componentWillMount&&!0!==c.componentWillMount.__suppressDeprecationWarning?d="componentWillMount":"function"==typeof c.UNSAFE_componentWillMount&&(d="UNSAFE_componentWillMount"),"function"==typeof c.componentWillReceiveProps&&!0!==c.componentWillReceiveProps.__suppressDeprecationWarning?p="componentWillReceiveProps":"function"==typeof c.UNSAFE_componentWillReceiveProps&&(p="UNSAFE_componentWillReceiveProps"),"function"==typeof c.componentWillUpdate&&!0!==c.componentWillUpdate.__suppressDeprecationWarning?m="componentWillUpdate":"function"==typeof c.UNSAFE_componentWillUpdate&&(m="UNSAFE_componentWillUpdate"),null!==d||null!==p||null!==m){var h=We(t)||"Component",g="function"==typeof t.getDerivedStateFromProps?"getDerivedStateFromProps()":"getSnapshotBeforeUpdate()";Bc.has(h)||(Bc.add(h),s("Unsafe legacy lifecycles will not be called for components using new component APIs.\n\n%s uses %s but also contains the following legacy lifecycles:%s%s%s\n\nThe above lifecycles should be removed. Learn more about this warning here:\nhttps://reactjs.org/link/unsafe-component-lifecycles",h,g,null!==d?"\n "+d:"",null!==p?"\n "+p:"",null!==m?"\n "+m:""))}}return a&&as(e,o,i),c}function cu(e,t,n,r){var a=t.state;if("function"==typeof t.componentWillReceiveProps&&t.componentWillReceiveProps(n,r),"function"==typeof t.UNSAFE_componentWillReceiveProps&&t.UNSAFE_componentWillReceiveProps(n,r),t.state!==a){var o=We(e.type)||"Component";jc.has(o)||(jc.add(o),s("%s.componentWillReceiveProps(): Assigning directly to this.state is deprecated (except inside a component's constructor). Use setState instead.",o)),ou.enqueueReplaceState(t,t.state,null)}}function uu(e,t,n,r){!function(e,t,n){var r=e.stateNode,a=We(t)||"Component";r.render||s(t.prototype&&"function"==typeof t.prototype.render?"%s(...): No `render` method found on the returned component instance: did you accidentally return an object from the constructor?":"%s(...): No `render` method found on the returned component instance: you may have forgotten to define `render`.",a),!r.getInitialState||r.getInitialState.isReactClassApproved||r.state||s("getInitialState was defined on %s, a plain JavaScript class. This is only supported for classes created using React.createClass. Did you mean to define a state property instead?",a),r.getDefaultProps&&!r.getDefaultProps.isReactClassApproved&&s("getDefaultProps was defined on %s, a plain JavaScript class. This is only supported for classes created using React.createClass. Use a static property to define defaultProps instead.",a),r.propTypes&&s("propTypes was defined as an instance property on %s. Use a static property to define propTypes instead.",a),r.contextType&&s("contextType was defined as an instance property on %s. Use a static property to define contextType instead.",a),r.contextTypes&&s("contextTypes was defined as an instance property on %s. Use a static property to define contextTypes instead.",a),t.contextType&&t.contextTypes&&!Yc.has(t)&&(Yc.add(t),s("%s declares both contextTypes and contextType static properties. The legacy contextTypes property will be ignored.",a)),"function"==typeof r.componentShouldUpdate&&s("%s has a method called componentShouldUpdate(). Did you mean shouldComponentUpdate()? The name is phrased as a question because the function is expected to return a value.",a),t.prototype&&t.prototype.isPureReactComponent&&void 0!==r.shouldComponentUpdate&&s("%s has a method called shouldComponentUpdate(). shouldComponentUpdate should not be used when extending React.PureComponent. Please extend React.Component if shouldComponentUpdate is used.",We(t)||"A pure component"),"function"==typeof r.componentDidUnmount&&s("%s has a method called componentDidUnmount(). But there is no such lifecycle method. Did you mean componentWillUnmount()?",a),"function"==typeof r.componentDidReceiveProps&&s("%s has a method called componentDidReceiveProps(). But there is no such lifecycle method. If you meant to update the state in response to changing props, use componentWillReceiveProps(). If you meant to fetch data or run side-effects or mutations after React has updated the UI, use componentDidUpdate().",a),"function"==typeof r.componentWillRecieveProps&&s("%s has a method called componentWillRecieveProps(). Did you mean componentWillReceiveProps()?",a),"function"==typeof r.UNSAFE_componentWillRecieveProps&&s("%s has a method called UNSAFE_componentWillRecieveProps(). Did you mean UNSAFE_componentWillReceiveProps()?",a),void 0!==r.props&&r.props!==n&&s("%s(...): When calling super() in `%s`, make sure to pass up the same props that your component's constructor was passed.",a,a),r.defaultProps&&s("Setting defaultProps as an instance property on %s is not supported and will be ignored. Instead, define defaultProps as a static property on %s.",a,a),"function"!=typeof r.getSnapshotBeforeUpdate||"function"==typeof r.componentDidUpdate||zc.has(t)||(zc.add(t),s("%s: getSnapshotBeforeUpdate() should be used with componentDidUpdate(). This component defines getSnapshotBeforeUpdate() only.",We(t))),"function"==typeof r.getDerivedStateFromProps&&s("%s: getDerivedStateFromProps() is defined as an instance method and will be ignored. Instead, declare it as a static method.",a),"function"==typeof r.getDerivedStateFromError&&s("%s: getDerivedStateFromError() is defined as an instance method and will be ignored. Instead, declare it as a static method.",a),"function"==typeof t.getSnapshotBeforeUpdate&&s("%s: getSnapshotBeforeUpdate() is defined as a static method and will be ignored. Instead, declare it as an instance method.",a);var o=r.state;o&&("object"!=typeof o||qc(o))&&s("%s.state: must be set to an object or null",a),"function"==typeof r.getChildContext&&"object"!=typeof t.childContextTypes&&s("%s.getChildContext(): childContextTypes must be defined in order to use getChildContext().",a)}(e,t,n);var a=e.stateNode;a.props=n,a.state=e.memoizedState,a.refs=Kc,Cc(e);var o=t.contextType;if("object"==typeof o&&null!==o)a.context=wc(o);else{var i=rs(0,t,!0);a.context=os(e,i)}if(a.state===n){var l=We(t)||"Component";Gc.has(l)||(Gc.add(l),s("%s: It is not recommended to assign props directly to state because updates to props won't be reflected in state. In most cases, it is better to use props directly.",l))}1&e.mode&&Js.recordLegacyContextWarning(e,a),Js.recordUnsafeLifecycleWarnings(e,a),_c(e,n,a,r),a.state=e.memoizedState;var c=t.getDerivedStateFromProps;"function"==typeof c&&(Qc(e,t,c,n),a.state=e.memoizedState),"function"==typeof t.getDerivedStateFromProps||"function"==typeof a.getSnapshotBeforeUpdate||"function"!=typeof a.UNSAFE_componentWillMount&&"function"!=typeof a.componentWillMount||(function(e,t){var n=t.state;"function"==typeof t.componentWillMount&&t.componentWillMount(),"function"==typeof t.UNSAFE_componentWillMount&&t.UNSAFE_componentWillMount(),n!==t.state&&(s("%s.componentWillMount(): Assigning directly to this.state is deprecated (except inside a component's constructor). Use setState instead.",We(e.type)||"Component"),ou.enqueueReplaceState(t,t.state,null))}(e,a),_c(e,n,a,r),a.state=e.memoizedState),"function"==typeof a.componentDidMount&&(e.flags|=4)}Zc=!1,eu=!1,tu={},nu={},ru={},au=function(e,t){if(null!==e&&"object"==typeof e&&e._store&&!e._store.validated&&null==e.key){if("object"!=typeof e._store)throw Error("React Component in warnForMissingKey should have a _store. This error is likely caused by a bug in React. Please file an issue.");e._store.validated=!0;var n=We(t.type)||"Component";nu[n]||(nu[n]=!0,s('Each child in a list should have a unique "key" prop. See https://reactjs.org/link/warning-keys for more information.'))}};var fu=Array.isArray;function du(e,t,n){var r=n.ref;if(null!==r&&"function"!=typeof r&&"object"!=typeof r){if(1&e.mode&&(!n._owner||!n._self||n._owner.stateNode===n._self)){var a=We(e.type)||"Component";tu[a]||(s('A string ref, "%s", has been found within a strict mode tree. String refs are a source of potential bugs and should be avoided. We recommend using useRef() or createRef() instead. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-string-ref',r),tu[a]=!0)}if(n._owner){var o,i=n._owner;if(i){var l=i;if(1!==l.tag)throw Error("Function components cannot have string refs. We recommend using useRef() instead. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-string-ref");o=l.stateNode}if(!o)throw Error("Missing owner for string ref "+r+". This error is likely caused by a bug in React. Please file an issue.");var c=""+r;if(null!==t&&null!==t.ref&&"function"==typeof t.ref&&t.ref._stringRef===c)return t.ref;var u=function(e){var t=o.refs;t===Kc&&(t=o.refs={}),null===e?delete t[c]:t[c]=e};return u._stringRef=c,u}if("string"!=typeof r)throw Error("Expected ref to be a function, a string, an object returned by React.createRef(), or null.");if(!n._owner)throw Error("Element ref was specified as a string ("+r+") but no owner was set. This could happen for one of the following reasons:\n1. You may be adding a ref to a function component\n2. You may be adding a ref to a component that was not created inside a component's render method\n3. You have multiple copies of React loaded\nSee https://reactjs.org/link/refs-must-have-owner for more information.")}return r}function pu(e,t){if("textarea"!==e.type)throw Error("Objects are not valid as a React child (found: "+("[object Object]"===Object.prototype.toString.call(t)?"object with keys {"+Object.keys(t).join(", ")+"}":t)+"). If you meant to render a collection of children, use an array instead.")}function mu(e){var t=We(e.type)||"Component";ru[t]||(ru[t]=!0,s("Functions are not valid as a React child. This may happen if you return a Component instead of <Component /> from render. Or maybe you meant to call this function rather than return it."))}function hu(e){function t(t,n){if(e){var r=t.lastEffect;null!==r?(r.nextEffect=n,t.lastEffect=n):t.firstEffect=t.lastEffect=n,n.nextEffect=null,n.flags=8}}function n(n,r){if(!e)return null;for(var a=r;null!==a;)t(n,a),a=a.sibling;return null}function r(e,t){for(var n=new Map,r=t;null!==r;)n.set(null!==r.key?r.key:r.index,r),r=r.sibling;return n}function a(e,t){var n=fg(e,t);return n.index=0,n.sibling=null,n}function o(t,n,r){if(t.index=r,!e)return n;var a=t.alternate;if(null!==a){var o=a.index;return o<n?(t.flags=2,n):o}return t.flags=2,n}function i(t){return e&&null===t.alternate&&(t.flags=2),t}function l(e,t,n,r){if(null===t||6!==t.tag){var o=yg(n,e.mode,r);return o.return=e,o}var i=a(t,n);return i.return=e,i}function c(e,t,n,r){if(null!==t&&(t.elementType===n.type||eg(t,n))){var o=a(t,n.props);return o.ref=du(e,t,n),o.return=e,o._debugSource=n._source,o._debugOwner=n._owner,o}var i=mg(n,e.mode,r);return i.ref=du(e,t,n),i.return=e,i}function u(e,t,n,r){if(null===t||4!==t.tag||t.stateNode.containerInfo!==n.containerInfo||t.stateNode.implementation!==n.implementation){var o=vg(n,e.mode,r);return o.return=e,o}var i=a(t,n.children||[]);return i.return=e,i}function f(e,t,n,r,o){if(null===t||7!==t.tag){var i=hg(n,e.mode,r,o);return i.return=e,i}var l=a(t,n);return l.return=e,l}function d(e,t,n){if("string"==typeof t||"number"==typeof t){var r=yg(""+t,e.mode,n);return r.return=e,r}if("object"==typeof t&&null!==t){switch(t.$$typeof){case Z:var a=mg(t,e.mode,n);return a.ref=du(e,null,t),a.return=e,a;case ee:var o=vg(t,e.mode,n);return o.return=e,o}if(fu(t)||Ee(t)){var i=hg(t,e.mode,n,null);return i.return=e,i}pu(e,t)}return"function"==typeof t&&mu(e),null}function m(e,t,n,r){var a=null!==t?t.key:null;if("string"==typeof n||"number"==typeof n)return null!==a?null:l(e,t,""+n,r);if("object"==typeof n&&null!==n){switch(n.$$typeof){case Z:return n.key===a?n.type===te?f(e,t,n.props.children,r,a):c(e,t,n,r):null;case ee:return n.key===a?u(e,t,n,r):null}if(fu(n)||Ee(n))return null!==a?null:f(e,t,n,r,null);pu(e,n)}return"function"==typeof n&&mu(e),null}function h(e,t,n,r,a){if("string"==typeof r||"number"==typeof r)return l(t,e.get(n)||null,""+r,a);if("object"==typeof r&&null!==r){switch(r.$$typeof){case Z:var o=e.get(null===r.key?n:r.key)||null;return r.type===te?f(t,o,r.props.children,a,r.key):c(t,o,r,a);case ee:return u(t,e.get(null===r.key?n:r.key)||null,r,a)}if(fu(r)||Ee(r))return f(t,e.get(n)||null,r,a,null);pu(t,r)}return"function"==typeof r&&mu(t),null}function g(e,t,n){if("object"!=typeof e||null===e)return t;switch(e.$$typeof){case Z:case ee:au(e,n);var r=e.key;if("string"!=typeof r)break;if(null===t){(t=new Set).add(r);break}if(!t.has(r)){t.add(r);break}s("Encountered two children with the same key, `%s`. Keys should be unique so that components maintain their identity across updates. Non-unique keys may cause children to be duplicated and/or omitted — the behavior is unsupported and could change in a future version.",r)}return t}return function(l,c,u,f){var v="object"==typeof u&&null!==u&&u.type===te&&null===u.key;v&&(u=u.props.children);var b="object"==typeof u&&null!==u;if(b)switch(u.$$typeof){case Z:return i(function(e,r,o,i){for(var l=o.key,s=r;null!==s;){if(s.key===l){switch(s.tag){case 7:if(o.type===te){n(e,s.sibling);var c=a(s,o.props.children);return c.return=e,c._debugSource=o._source,c._debugOwner=o._owner,c}break;case T:default:if(s.elementType===o.type||eg(s,o)){n(e,s.sibling);var u=a(s,o.props);return u.ref=du(e,s,o),u.return=e,u._debugSource=o._source,u._debugOwner=o._owner,u}}n(e,s);break}t(e,s),s=s.sibling}if(o.type===te){var f=hg(o.props.children,e.mode,i,o.key);return f.return=e,f}var d=mg(o,e.mode,i);return d.ref=du(e,r,o),d.return=e,d}(l,c,u,f));case ee:return i(function(e,r,o,i){for(var l=o.key,s=r;null!==s;){if(s.key===l){if(4===s.tag&&s.stateNode.containerInfo===o.containerInfo&&s.stateNode.implementation===o.implementation){n(e,s.sibling);var c=a(s,o.children||[]);return c.return=e,c}n(e,s);break}t(e,s),s=s.sibling}var u=vg(o,e.mode,i);return u.return=e,u}(l,c,u,f))}if("string"==typeof u||"number"==typeof u)return i(function(e,t,r,o){if(null!==t&&6===t.tag){n(e,t.sibling);var i=a(t,r);return i.return=e,i}n(e,t);var l=yg(r,e.mode,o);return l.return=e,l}(l,c,""+u,f));if(fu(u))return function(a,i,l,s){for(var c=null,u=0;u<l.length;u++)c=g(l[u],c,a);for(var f=null,p=null,y=i,v=0,b=0,E=null;null!==y&&b<l.length;b++){y.index>b?(E=y,y=null):E=y.sibling;var T=m(a,y,l[b],s);if(null===T){null===y&&(y=E);break}e&&y&&null===T.alternate&&t(a,y),v=o(T,v,b),null===p?f=T:p.sibling=T,p=T,y=E}if(b===l.length)return n(a,y),f;if(null===y){for(;b<l.length;b++){var S=d(a,l[b],s);null!==S&&(v=o(S,v,b),null===p?f=S:p.sibling=S,p=S)}return f}for(var w=r(0,y);b<l.length;b++){var N=h(w,a,b,l[b],s);null!==N&&(e&&null!==N.alternate&&w.delete(null===N.key?b:N.key),v=o(N,v,b),null===p?f=N:p.sibling=N,p=N)}return e&&w.forEach(function(e){return t(a,e)}),f}(l,c,u,f);if(Ee(u))return function(a,i,l,c){var u=Ee(l);if("function"!=typeof u)throw Error("An object is not an iterable. This error is likely caused by a bug in React. Please file an issue.");"function"==typeof Symbol&&"Generator"===l[Symbol.toStringTag]&&(eu||s("Using Generators as children is unsupported and will likely yield unexpected results because enumerating a generator mutates it. You may convert it to an array with `Array.from()` or the `[...spread]` operator before rendering. Keep in mind you might need to polyfill these features for older browsers."),eu=!0),l.entries===u&&(Zc||s("Using Maps as children is not supported. Use an array of keyed ReactElements instead."),Zc=!0);var f=u.call(l);if(f)for(var p=null,y=f.next();!y.done;y=f.next())p=g(y.value,p,a);var v=u.call(l);if(null==v)throw Error("An iterable object provided no iterator.");for(var b=null,E=null,T=i,S=0,w=0,N=null,R=v.next();null!==T&&!R.done;w++,R=v.next()){T.index>w?(N=T,T=null):N=T.sibling;var O=m(a,T,R.value,c);if(null===O){null===T&&(T=N);break}e&&T&&null===O.alternate&&t(a,T),S=o(O,S,w),null===E?b=O:E.sibling=O,E=O,T=N}if(R.done)return n(a,T),b;if(null===T){for(;!R.done;w++,R=v.next()){var C=d(a,R.value,c);null!==C&&(S=o(C,S,w),null===E?b=C:E.sibling=C,E=C)}return b}for(var x=r(0,T);!R.done;w++,R=v.next()){var k=h(x,a,w,R.value,c);null!==k&&(e&&null!==k.alternate&&x.delete(null===k.key?w:k.key),S=o(k,S,w),null===E?b=k:E.sibling=k,E=k)}return e&&x.forEach(function(e){return t(a,e)}),b}(l,c,u,f);if(b&&pu(l,u),"function"==typeof u&&mu(l),void 0===u&&!v)switch(l.tag){case 1:if(l.stateNode.render._isMockFunction)break;case T:case 0:case p:case y:throw Error((We(l.type)||"Component")+"(...): Nothing was returned from render. This usually means a return statement is missing. Or, to render nothing, return null.")}return n(l,c)}}var gu=hu(!0),yu=hu(!1);function vu(e,t){for(var n=e.child;null!==n;)dg(n,t),n=n.sibling}var bu={},Eu=Kl(bu),Tu=Kl(bu),Su=Kl(bu);function wu(e){if(e===bu)throw Error("Expected host context to exist. This error is likely caused by a bug in React. Please file an issue.");return e}function Nu(){return wu(Su.current)}function Ru(e,t){Ql(Su,t,e),Ql(Tu,e,e),Ql(Eu,bu,e);var n=function(e){var t,n,r=e.nodeType;switch(r){case 9:case 11:t=9===r?"#document":"#fragment";var a=e.documentElement;n=a?a.namespaceURI:Ut(null,"");break;default:var o=8===r?e.parentNode:e;n=Ut(o.namespaceURI||null,t=o.tagName)}var i=t.toLowerCase();return{namespace:n,ancestorInfo:nl(null,i)}}(t);Jl(Eu,e),Ql(Eu,n,e)}function Ou(e){Jl(Eu,e),Jl(Tu,e),Jl(Su,e)}function Cu(){return wu(Eu.current)}function xu(e){wu(Su.current);var t,n,r=wu(Eu.current),a={namespace:Ut((n=r).namespace,t=e.type),ancestorInfo:nl(n.ancestorInfo,t)};r!==a&&(Ql(Tu,e,e),Ql(Eu,a,e))}function ku(e){Tu.current===e&&(Jl(Eu,e),Jl(Tu,e))}var Au=Kl(0);function Iu(e,t){return 0!=(e&t)}function Lu(e){return 1&e}function _u(e,t){return 1&e|t}function Pu(e,t){Ql(Au,t,e)}function Mu(e){Jl(Au,e)}function Du(e,t){var n=e.memoizedState;if(null!==n)return null!==n.dehydrated;var r=e.memoizedProps;return void 0!==r.fallback&&(!0!==r.unstable_avoidThisFallback||!t)}function Uu(e){for(var t=e;null!==t;){if(t.tag===h){var n=t.memoizedState;if(null!==n){var r=n.dehydrated;if(null===r||r.data===cl||r.data===ul)return t}}else if(t.tag===b&&void 0!==t.memoizedProps.revealOrder){if(0!=(t.flags&ar))return t}else if(null!==t.child){t.child.return=t,t=t.child;continue}if(t===e)return null;for(;null===t.sibling;){if(null===t.return||t.return===e)return null;t=t.return}t.sibling.return=t.return,t=t.sibling}return null}var ju=null,Fu=null,zu=!1;function Bu(e,t){switch(e.tag){case 3:!function(e,t){1===t.nodeType?Ji(e,t):8===t.nodeType||Qi(e,t)}(e.stateNode.containerInfo,t);break;case 5:!function(e,t,n,r){!0!==t.suppressHydrationWarning&&(1===r.nodeType?Ji(n,r):8===r.nodeType||Qi(n,r))}(0,e.memoizedProps,e.stateNode,t)}var n,r=((n=cg(5,null,null,0)).elementType="DELETED",n.type="DELETED",n);r.stateNode=t,r.return=e,r.flags=8,null!==e.lastEffect?(e.lastEffect.nextEffect=r,e.lastEffect=r):e.firstEffect=e.lastEffect=r}function Hu(e,t){switch(t.flags=-1025&t.flags|2,e.tag){case 3:var n=e.stateNode.containerInfo;switch(t.tag){case 5:!function(e,t,n){Zi(e,t)}(n,t.type);break;case 6:!function(e,t){el(e,t)}(n,t.pendingProps)}break;case 5:var r=e.memoizedProps,a=e.stateNode;switch(t.tag){case 5:!function(e,t,n,r,a){!0!==t.suppressHydrationWarning&&Zi(n,r)}(0,r,a,t.type);break;case 6:!function(e,t,n,r){!0!==t.suppressHydrationWarning&&el(n,r)}(0,r,a,t.pendingProps)}break;default:return}}function Vu(e,t){switch(e.tag){case 5:var n=function(e,t,n){return 1!==e.nodeType||t.toLowerCase()!==e.nodeName.toLowerCase()?null:e}(t,e.type);return null!==n&&(e.stateNode=n,!0);case 6:var r=function(e,t){return""===t||3!==e.nodeType?null:e}(t,e.pendingProps);return null!==r&&(e.stateNode=r,!0);case h:default:return!1}}function Wu(e){if(zu){var t=Fu;if(!t)return Hu(ju,e),zu=!1,void(ju=e);var n=t;if(!Vu(e,t)){if(!(t=wl(n))||!Vu(e,t))return Hu(ju,e),zu=!1,void(ju=e);Bu(ju,n)}ju=e,Fu=Nl(t)}}function Gu(e){for(var t=e.return;null!==t&&5!==t.tag&&3!==t.tag&&t.tag!==h;)t=t.return;ju=t}function Yu(e){if(e!==ju)return!1;if(!zu)return Gu(e),zu=!0,!1;var t=e.type;if(5!==e.tag||"head"!==t&&"body"!==t&&!ml(t,e.memoizedProps))for(var n=Fu;n;)Bu(e,n),n=wl(n);return Gu(e),Fu=e.tag===h?function(e){var t=e.memoizedState,n=null!==t?t.dehydrated:null;if(!n)throw Error("Expected to have a hydrated suspense instance. This error is likely caused by a bug in React. Please file an issue.");return function(e){for(var t=e.nextSibling,n=0;t;){if(8===t.nodeType){var r=t.data;if("/$"===r){if(0===n)return wl(t);n--}else"$"!==r&&r!==ul&&r!==cl||n++}t=t.nextSibling}return null}(n)}(e):ju?wl(e.stateNode):null,!0}function $u(){ju=null,Fu=null,zu=!1}function Xu(){return zu}var qu,Ku=[];function Ju(){for(var e=0;e<Ku.length;e++)Ku[e]._workInProgressVersionPrimary=null;Ku.length=0}function Qu(e,t){e._workInProgressVersionPrimary=t,Ku.push(e)}qu={};var Zu,ef,tf=o.ReactCurrentDispatcher,nf=o.ReactCurrentBatchConfig;ef={},Zu=new Set;var rf=0,af=null,of=null,lf=null,sf=!1,cf=!1,uf=null,ff=null,df=-1,pf=!1;function mf(){null===ff?ff=[uf]:ff.push(uf)}function hf(){null!==ff&&(df++,ff[df]!==uf&&function(e){var t=We(af.type);if(!Zu.has(t)&&(Zu.add(t),null!==ff)){for(var n="",r=0;r<=df;r++){for(var a=ff[r],o=r===df?e:a,i=r+1+". "+a;i.length<30;)i+=" ";n+=i+=o+"\n"}s("React has detected a change in the order of Hooks called by %s. This will lead to bugs and errors if not fixed. For more information, read the Rules of Hooks: https://reactjs.org/link/rules-of-hooks\n\n Previous render Next render\n ------------------------------------------------------\n%s ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^\n",t,n)}}(uf))}function gf(e){null==e||Array.isArray(e)||s("%s received a final argument that is not an array (instead, received `%s`). When specified, the final argument must be an array.",uf,typeof e)}function yf(){throw Error("Invalid hook call. Hooks can only be called inside of the body of a function component. This could happen for one of the following reasons:\n1. You might have mismatching versions of React and the renderer (such as React DOM)\n2. You might be breaking the Rules of Hooks\n3. You might have more than one copy of React in the same app\nSee https://reactjs.org/link/invalid-hook-call for tips about how to debug and fix this problem.")}function vf(e,t){if(pf)return!1;if(null===t)return s("%s received a final argument during this render, but not during the previous render. Even though the final argument is optional, its type cannot change between renders.",uf),!1;e.length!==t.length&&s("The final argument passed to %s changed size between renders. The order and size of this array must remain constant.\n\nPrevious: %s\nIncoming: %s",uf,"["+t.join(", ")+"]","["+e.join(", ")+"]");for(var n=0;n<t.length&&n<e.length;n++)if(!Yo(e[n],t[n]))return!1;return!0}function bf(e,t,n,r,a,o){rf=o,af=t,ff=null!==e?e._debugHookTypes:null,df=-1,pf=null!==e&&e.type!==t.type,t.memoizedState=null,t.updateQueue=null,t.lanes=0,tf.current=null!==e&&null!==e.memoizedState?pd:null!==ff?dd:fd;var i=n(r,a);if(cf){var l=0;do{if(cf=!1,!(l<25))throw Error("Too many re-renders. React limits the number of renders to prevent an infinite loop.");l+=1,pf=!1,of=null,lf=null,t.updateQueue=null,df=-1,tf.current=md,i=n(r,a)}while(cf)}tf.current=ud,t._debugHookTypes=ff;var s=null!==of&&null!==of.next;if(rf=0,af=null,of=null,lf=null,uf=null,ff=null,df=-1,sf=!1,s)throw Error("Rendered fewer hooks than expected. This may be caused by an accidental early return statement.");return i}function Ef(e,t,n){t.updateQueue=e.updateQueue,t.flags&=-517,e.lanes=wa(e.lanes,n)}function Tf(){if(tf.current=ud,sf){for(var e=af.memoizedState;null!==e;){var t=e.queue;null!==t&&(t.pending=null),e=e.next}sf=!1}rf=0,af=null,of=null,lf=null,ff=null,df=-1,uf=null,ad=!1,cf=!1}function Sf(){var e={memoizedState:null,baseState:null,baseQueue:null,queue:null,next:null};return null===lf?af.memoizedState=lf=e:lf=lf.next=e,lf}function wf(){var e,t;if(null===of){var n=af.alternate;e=null!==n?n.memoizedState:null}else e=of.next;if(null!==(t=null===lf?af.memoizedState:lf.next))t=(lf=t).next,of=e;else{if(null===e)throw Error("Rendered more hooks than during the previous render.");var r={memoizedState:(of=e).memoizedState,baseState:of.baseState,baseQueue:of.baseQueue,queue:of.queue,next:null};null===lf?af.memoizedState=lf=r:lf=lf.next=r}return lf}function Nf(e,t){return"function"==typeof t?t(e):t}function Rf(e,t,n){var r,a=Sf();r=void 0!==n?n(t):t,a.memoizedState=a.baseState=r;var o=a.queue={pending:null,dispatch:null,lastRenderedReducer:e,lastRenderedState:r},i=o.dispatch=cd.bind(null,af,o);return[a.memoizedState,i]}function Of(e,t,n){var r=wf(),a=r.queue;if(null===a)throw Error("Should have a queue. This is likely a bug in React. Please file an issue.");a.lastRenderedReducer=e;var o=of,i=o.baseQueue,l=a.pending;if(null!==l){if(null!==i){var c=i.next;i.next=l.next,l.next=c}o.baseQueue!==i&&s("Internal error: Expected work-in-progress queue to be a clone. This is a bug in React."),o.baseQueue=i=l,a.pending=null}if(null!==i){var u=i.next,f=o.baseState,d=null,p=null,m=null,h=u;do{var g=h.lane;if(Ta(rf,g))null!==m&&(m=m.next={lane:0,action:h.action,eagerReducer:h.eagerReducer,eagerState:h.eagerState,next:null}),f=h.eagerReducer===e?h.eagerState:e(f,h.action);else{var y={lane:g,action:h.action,eagerReducer:h.eagerReducer,eagerState:h.eagerState,next:null};null===m?(p=m=y,d=f):m=m.next=y,af.lanes=Sa(af.lanes,g),ih(g)}h=h.next}while(null!==h&&h!==u);null===m?d=f:m.next=p,Yo(f,r.memoizedState)||hp(),r.memoizedState=f,r.baseState=d,r.baseQueue=m,a.lastRenderedState=f}return[r.memoizedState,a.dispatch]}function Cf(e,t,n){var r=wf(),a=r.queue;if(null===a)throw Error("Should have a queue. This is likely a bug in React. Please file an issue.");a.lastRenderedReducer=e;var o=a.dispatch,i=a.pending,l=r.memoizedState;if(null!==i){a.pending=null;var s=i.next,c=s;do{l=e(l,c.action),c=c.next}while(c!==s);Yo(l,r.memoizedState)||hp(),r.memoizedState=l,null===r.baseQueue&&(r.baseState=l),a.lastRenderedState=l}return[l,o]}function xf(e,t,n){var r;null==(r=t)._currentPrimaryRenderer?r._currentPrimaryRenderer=qu:r._currentPrimaryRenderer!==qu&&s("Detected multiple renderers concurrently rendering the same mutable source. This is currently unsupported.");var a=(0,t._getVersion)(t._source),o=!1,i=function(e){return e._workInProgressVersionPrimary}(t);if(null!==i?o=i===a:(o=Ta(rf,e.mutableReadLanes))&&Qu(t,a),o){var l=n(t._source);return"function"==typeof l&&s("Mutable source should not return a function as the snapshot value. Functions may close over mutable values and cause tearing."),l}throw function(e){Ku.push(e)}(t),Error("Cannot read from mutable source during the current render without tearing. This is a bug in React. Please file an issue.")}function kf(e,t,n,r){var a=im;if(null===a)throw Error("Expected a work-in-progress root. This is a bug in React. Please file an issue.");var o=t._getVersion,i=o(t._source),l=tf.current,c=l.useState(function(){return xf(a,t,n)}),u=c[1],f=c[0],d=lf,p=e.memoizedState,m=p.refs,h=m.getSnapshot,g=p.source,y=p.subscribe,v=af;if(e.memoizedState={refs:m,source:t,subscribe:r},l.useEffect(function(){m.getSnapshot=n,m.setSnapshot=u;var e=o(t._source);if(!Yo(i,e)){var r=n(t._source);if("function"==typeof r&&s("Mutable source should not return a function as the snapshot value. Functions may close over mutable values and cause tearing."),!Yo(f,r)){u(r);var l=Hm(v);Ca(a,l)}!function(e,t){e.entangledLanes|=t;for(var n=e.entanglements,r=t;r>0;){var a=ba(r),o=1<<a;n[a]|=t,r&=~o}}(a,a.mutableReadLanes)}},[n,t,r]),l.useEffect(function(){var e=r(t._source,function(){var e=m.getSnapshot,n=m.setSnapshot;try{n(e(t._source));var r=Hm(v);Ca(a,r)}catch(e){n(function(){throw e})}});return"function"!=typeof e&&s("Mutable source subscribe function must return an unsubscribe function."),e},[t,r]),!Yo(h,n)||!Yo(g,t)||!Yo(y,r)){var b={pending:null,dispatch:null,lastRenderedReducer:Nf,lastRenderedState:f};b.dispatch=u=cd.bind(null,af,b),d.queue=b,d.baseQueue=null,f=xf(a,t,n),d.memoizedState=d.baseState=f}return f}function Af(e,t,n){var r=Sf();return r.memoizedState={refs:{getSnapshot:t,setSnapshot:null},source:e,subscribe:n},kf(r,e,t,n)}function If(e,t,n){return kf(wf(),e,t,n)}function Lf(e){var t=Sf();"function"==typeof e&&(e=e()),t.memoizedState=t.baseState=e;var n=t.queue={pending:null,dispatch:null,lastRenderedReducer:Nf,lastRenderedState:e},r=n.dispatch=cd.bind(null,af,n);return[t.memoizedState,r]}function _f(e){return Of(Nf)}function Pf(e){return Cf(Nf)}function Mf(e,t,n,r){var a={tag:e,create:t,destroy:n,deps:r,next:null},o=af.updateQueue;if(null===o)af.updateQueue=o={lastEffect:null},o.lastEffect=a.next=a;else{var i=o.lastEffect;if(null===i)o.lastEffect=a.next=a;else{var l=i.next;i.next=a,a.next=l,o.lastEffect=a}}return a}function Df(e){var t=Sf(),n={current:e};return Object.seal(n),t.memoizedState=n,n}function Uf(e){return wf().memoizedState}function jf(e,t,n,r){var a=Sf(),o=void 0===r?null:r;af.flags|=e,a.memoizedState=Mf(1|t,n,void 0,o)}function Ff(e,t,n,r){var a=wf(),o=void 0===r?null:r,i=void 0;if(null!==of){var l=of.memoizedState;if(i=l.destroy,null!==o&&vf(o,l.deps))return void Mf(t,n,i,o)}af.flags|=e,a.memoizedState=Mf(1|t,n,i,o)}function zf(e,t){return"undefined"!=typeof jest&&Uh(af),jf(516,4,e,t)}function Bf(e,t){return"undefined"!=typeof jest&&Uh(af),Ff(516,4,e,t)}function Hf(e,t){return jf(4,2,e,t)}function Vf(e,t){return Ff(4,2,e,t)}function Wf(e,t){if("function"==typeof t){var n=t,r=e();return n(r),function(){n(null)}}if(null!=t){var a=t;a.hasOwnProperty("current")||s("Expected useImperativeHandle() first argument to either be a ref callback or React.createRef() object. Instead received: %s.","an object with keys {"+Object.keys(a).join(", ")+"}");var o=e();return a.current=o,function(){a.current=null}}}function Gf(e,t,n){"function"!=typeof t&&s("Expected useImperativeHandle() second argument to be a function that creates a handle. Instead received: %s.",null!==t?typeof t:"null");var r=null!=n?n.concat([e]):null;return jf(4,2,Wf.bind(null,t,e),r)}function Yf(e,t,n){"function"!=typeof t&&s("Expected useImperativeHandle() second argument to be a function that creates a handle. Instead received: %s.",null!==t?typeof t:"null");var r=null!=n?n.concat([e]):null;return Ff(4,2,Wf.bind(null,t,e),r)}function $f(e,t){return Sf().memoizedState=[e,void 0===t?null:t],e}function Xf(e,t){var n=wf(),r=void 0===t?null:t,a=n.memoizedState;return null!==a&&null!==r&&vf(r,a[1])?a[0]:(n.memoizedState=[e,r],e)}function qf(e,t){var n=Sf(),r=void 0===t?null:t,a=e();return n.memoizedState=[a,r],a}function Kf(e,t){var n=wf(),r=void 0===t?null:t,a=n.memoizedState;if(null!==a&&null!==r&&vf(r,a[1]))return a[0];var o=e();return n.memoizedState=[o,r],o}function Jf(e){var t=Lf(e),n=t[0],r=t[1];return zf(function(){var t=nf.transition;nf.transition=1;try{r(e)}finally{nf.transition=t}},[e]),n}function Qf(e){var t=_f(),n=t[0],r=t[1];return Bf(function(){var t=nf.transition;nf.transition=1;try{r(e)}finally{nf.transition=t}},[e]),n}function Zf(e){var t=Pf(),n=t[0],r=t[1];return Bf(function(){var t=nf.transition;nf.transition=1;try{r(e)}finally{nf.transition=t}},[e]),n}function ed(e,t){var n=Hs();Ws(n<_s?_s:n,function(){e(!0)}),Ws(n>Ps?Ps:n,function(){var n=nf.transition;nf.transition=1;try{e(!1),t()}finally{nf.transition=n}})}function td(){var e=Lf(!1),t=e[0],n=ed.bind(null,e[1]);return Df(n),[n,t]}function nd(){var e=_f()[0];return[Uf().current,e]}function rd(){var e=Pf()[0];return[Uf().current,e]}var ad=!1;function od(e){var t=We(e.type)||"Unknown";$e&&!ef[t]&&(s("The object passed back from useOpaqueIdentifier is meant to be passed through to attributes only. Do not read the value directly."),ef[t]=!0)}function id(){var e=Cl.bind(null,od.bind(null,af));if(Xu()){var t=!1,n=af,r={$$typeof:me,toString:o=function(){throw t||(t=!0,ad=!0,a(e()),ad=!1,od(n)),Error("The object passed back from useOpaqueIdentifier is meant to be passed through to attributes only. Do not read the value directly.")},valueOf:o},a=Lf(r)[1];return 0==(2&af.mode)&&(af.flags|=516,Mf(5,function(){a(e())},void 0,null)),r}var o,i=e();return Lf(i),i}function ld(){return _f()[0]}function sd(){return Pf()[0]}function cd(e,t,n){"function"==typeof arguments[3]&&s("State updates from the useState() and useReducer() Hooks don't support the second callback argument. To execute a side effect after rendering, declare it in the component body with useEffect().");var r=Bm(),a=Hm(e),o={lane:a,action:n,eagerReducer:null,eagerState:null,next:null},i=t.pending;null===i?o.next=o:(o.next=i.next,i.next=o),t.pending=o;var l=e.alternate;if(e===af||null!==l&&l===af)cf=sf=!0;else{if(0===e.lanes&&(null===l||0===l.lanes)){var c=t.lastRenderedReducer;if(null!==c){var u;u=tf.current,tf.current=gd;try{var f=t.lastRenderedState,d=c(f,n);if(o.eagerReducer=c,o.eagerState=d,Yo(d,f))return}catch(e){}finally{tf.current=u}}}"undefined"!=typeof jest&&(Dh(e),jh(e)),Vm(e,a,r)}}var ud={readContext:wc,useCallback:yf,useContext:yf,useEffect:yf,useImperativeHandle:yf,useLayoutEffect:yf,useMemo:yf,useReducer:yf,useRef:yf,useState:yf,useDebugValue:yf,useDeferredValue:yf,useTransition:yf,useMutableSource:yf,useOpaqueIdentifier:yf,unstable_isNewReconciler:N},fd=null,dd=null,pd=null,md=null,hd=null,gd=null,yd=null,vd=function(){s("Context can only be read while React is rendering. In classes, you can read it in the render method or getDerivedStateFromProps. In function components, you can read it directly in the function body, but not inside Hooks like useReducer() or useMemo().")},bd=function(){s("Do not call Hooks inside useEffect(...), useMemo(...), or other built-in Hooks. You can only call Hooks at the top level of your React function. For more information, see https://reactjs.org/link/rules-of-hooks")};fd={readContext:function(e,t){return wc(e,t)},useCallback:function(e,t){return uf="useCallback",mf(),gf(t),$f(e,t)},useContext:function(e,t){return uf="useContext",mf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",mf(),gf(t),zf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",mf(),gf(n),Gf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",mf(),gf(t),Hf(e,t)},useMemo:function(e,t){uf="useMemo",mf(),gf(t);var n=tf.current;tf.current=hd;try{return qf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",mf();var r=tf.current;tf.current=hd;try{return Rf(e,t,n)}finally{tf.current=r}},useRef:function(e){return uf="useRef",mf(),Df(e)},useState:function(e){uf="useState",mf();var t=tf.current;tf.current=hd;try{return Lf(e)}finally{tf.current=t}},useDebugValue:function(e,t){uf="useDebugValue",mf()},useDeferredValue:function(e){return uf="useDeferredValue",mf(),Jf(e)},useTransition:function(){return uf="useTransition",mf(),td()},useMutableSource:function(e,t,n){return uf="useMutableSource",mf(),Af(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",mf(),id()},unstable_isNewReconciler:N},dd={readContext:function(e,t){return wc(e,t)},useCallback:function(e,t){return uf="useCallback",hf(),$f(e,t)},useContext:function(e,t){return uf="useContext",hf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",hf(),zf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",hf(),Gf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",hf(),Hf(e,t)},useMemo:function(e,t){uf="useMemo",hf();var n=tf.current;tf.current=hd;try{return qf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",hf();var r=tf.current;tf.current=hd;try{return Rf(e,t,n)}finally{tf.current=r}},useRef:function(e){return uf="useRef",hf(),Df(e)},useState:function(e){uf="useState",hf();var t=tf.current;tf.current=hd;try{return Lf(e)}finally{tf.current=t}},useDebugValue:function(e,t){uf="useDebugValue",hf()},useDeferredValue:function(e){return uf="useDeferredValue",hf(),Jf(e)},useTransition:function(){return uf="useTransition",hf(),td()},useMutableSource:function(e,t,n){return uf="useMutableSource",hf(),Af(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",hf(),id()},unstable_isNewReconciler:N},pd={readContext:function(e,t){return wc(e,t)},useCallback:function(e,t){return uf="useCallback",hf(),Xf(e,t)},useContext:function(e,t){return uf="useContext",hf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",hf(),Bf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",hf(),Yf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",hf(),Vf(e,t)},useMemo:function(e,t){uf="useMemo",hf();var n=tf.current;tf.current=gd;try{return Kf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",hf();var r=tf.current;tf.current=gd;try{return Of(e)}finally{tf.current=r}},useRef:function(e){return uf="useRef",hf(),Uf()},useState:function(e){uf="useState",hf();var t=tf.current;tf.current=gd;try{return _f()}finally{tf.current=t}},useDebugValue:function(e,t){return uf="useDebugValue",void hf()},useDeferredValue:function(e){return uf="useDeferredValue",hf(),Qf(e)},useTransition:function(){return uf="useTransition",hf(),nd()},useMutableSource:function(e,t,n){return uf="useMutableSource",hf(),If(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",hf(),ld()},unstable_isNewReconciler:N},md={readContext:function(e,t){return wc(e,t)},useCallback:function(e,t){return uf="useCallback",hf(),Xf(e,t)},useContext:function(e,t){return uf="useContext",hf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",hf(),Bf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",hf(),Yf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",hf(),Vf(e,t)},useMemo:function(e,t){uf="useMemo",hf();var n=tf.current;tf.current=yd;try{return Kf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",hf();var r=tf.current;tf.current=yd;try{return Cf(e)}finally{tf.current=r}},useRef:function(e){return uf="useRef",hf(),Uf()},useState:function(e){uf="useState",hf();var t=tf.current;tf.current=yd;try{return Pf()}finally{tf.current=t}},useDebugValue:function(e,t){return uf="useDebugValue",void hf()},useDeferredValue:function(e){return uf="useDeferredValue",hf(),Zf(e)},useTransition:function(){return uf="useTransition",hf(),rd()},useMutableSource:function(e,t,n){return uf="useMutableSource",hf(),If(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",hf(),sd()},unstable_isNewReconciler:N},hd={readContext:function(e,t){return vd(),wc(e,t)},useCallback:function(e,t){return uf="useCallback",bd(),mf(),$f(e,t)},useContext:function(e,t){return uf="useContext",bd(),mf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",bd(),mf(),zf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",bd(),mf(),Gf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",bd(),mf(),Hf(e,t)},useMemo:function(e,t){uf="useMemo",bd(),mf();var n=tf.current;tf.current=hd;try{return qf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",bd(),mf();var r=tf.current;tf.current=hd;try{return Rf(e,t,n)}finally{tf.current=r}},useRef:function(e){return uf="useRef",bd(),mf(),Df(e)},useState:function(e){uf="useState",bd(),mf();var t=tf.current;tf.current=hd;try{return Lf(e)}finally{tf.current=t}},useDebugValue:function(e,t){uf="useDebugValue",bd(),mf()},useDeferredValue:function(e){return uf="useDeferredValue",bd(),mf(),Jf(e)},useTransition:function(){return uf="useTransition",bd(),mf(),td()},useMutableSource:function(e,t,n){return uf="useMutableSource",bd(),mf(),Af(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",bd(),mf(),id()},unstable_isNewReconciler:N},gd={readContext:function(e,t){return vd(),wc(e,t)},useCallback:function(e,t){return uf="useCallback",bd(),hf(),Xf(e,t)},useContext:function(e,t){return uf="useContext",bd(),hf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",bd(),hf(),Bf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",bd(),hf(),Yf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",bd(),hf(),Vf(e,t)},useMemo:function(e,t){uf="useMemo",bd(),hf();var n=tf.current;tf.current=gd;try{return Kf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",bd(),hf();var r=tf.current;tf.current=gd;try{return Of(e)}finally{tf.current=r}},useRef:function(e){return uf="useRef",bd(),hf(),Uf()},useState:function(e){uf="useState",bd(),hf();var t=tf.current;tf.current=gd;try{return _f()}finally{tf.current=t}},useDebugValue:function(e,t){return uf="useDebugValue",bd(),void hf()},useDeferredValue:function(e){return uf="useDeferredValue",bd(),hf(),Qf(e)},useTransition:function(){return uf="useTransition",bd(),hf(),nd()},useMutableSource:function(e,t,n){return uf="useMutableSource",bd(),hf(),If(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",bd(),hf(),ld()},unstable_isNewReconciler:N},yd={readContext:function(e,t){return vd(),wc(e,t)},useCallback:function(e,t){return uf="useCallback",bd(),hf(),Xf(e,t)},useContext:function(e,t){return uf="useContext",bd(),hf(),wc(e,t)},useEffect:function(e,t){return uf="useEffect",bd(),hf(),Bf(e,t)},useImperativeHandle:function(e,t,n){return uf="useImperativeHandle",bd(),hf(),Yf(e,t,n)},useLayoutEffect:function(e,t){return uf="useLayoutEffect",bd(),hf(),Vf(e,t)},useMemo:function(e,t){uf="useMemo",bd(),hf();var n=tf.current;tf.current=gd;try{return Kf(e,t)}finally{tf.current=n}},useReducer:function(e,t,n){uf="useReducer",bd(),hf();var r=tf.current;tf.current=gd;try{return Cf(e)}finally{tf.current=r}},useRef:function(e){return uf="useRef",bd(),hf(),Uf()},useState:function(e){uf="useState",bd(),hf();var t=tf.current;tf.current=gd;try{return Pf()}finally{tf.current=t}},useDebugValue:function(e,t){return uf="useDebugValue",bd(),void hf()},useDeferredValue:function(e){return uf="useDeferredValue",bd(),hf(),Zf(e)},useTransition:function(){return uf="useTransition",bd(),hf(),rd()},useMutableSource:function(e,t,n){return uf="useMutableSource",bd(),hf(),If(e,t,n)},useOpaqueIdentifier:function(){return uf="useOpaqueIdentifier",bd(),hf(),sd()},unstable_isNewReconciler:N};var Ed=r.unstable_now,Td=0,Sd=-1;function wd(){return Td}function Nd(){Td=Ed()}function Rd(e){Sd=Ed(),e.actualStartTime<0&&(e.actualStartTime=Ed())}function Od(e){Sd=-1}function Cd(e,t){if(Sd>=0){var n=Ed()-Sd;e.actualDuration+=n,t&&(e.selfBaseDuration=n),Sd=-1}}function xd(e){for(var t=e.child;t;)e.actualDuration+=t.actualDuration,t=t.sibling}var kd,Ad,Id,Ld,_d,Pd,Md,Dd,Ud=o.ReactCurrentOwner,jd=!1;function Fd(e,t,n,r){t.child=null===e?yu(t,null,n,r):gu(t,e.child,n,r)}function zd(e,t,n,r,a){if(t.type!==t.elementType){var o=n.propTypes;o&&Gl(o,r,"prop",We(n))}var i,l=n.render,s=t.ref;if(Sc(t,a),Ud.current=t,Qe(!0),i=bf(e,t,l,r,s,a),1&t.mode){Ae();try{i=bf(e,t,l,r,s,a)}finally{Ie()}}return Qe(!1),null===e||jd?(t.flags|=1,Fd(e,t,i,a),t.child):(Ef(e,t,a),gp(e,t,a))}function Bd(e,t,n,r,a,o){if(null===e){var i,l=n.type;if(function(e){return"function"==typeof e&&!ug(e)&&void 0===e.defaultProps}(l)&&null===n.compare&&void 0===n.defaultProps)return i=Jh(l),t.tag=y,t.type=i,Kd(t,l),Hd(e,t,i,r,a,o);var s=l.propTypes;s&&Gl(s,r,"prop",We(l));var c=pg(n.type,null,r,t,t.mode,o);return c.ref=t.ref,c.return=t,t.child=c,c}var u=n.type,f=u.propTypes;f&&Gl(f,r,"prop",We(u));var d=e.child;if(!Ea(a,o)){var p=n.compare;if((p=null!==p?p:Xo)(d.memoizedProps,r)&&e.ref===t.ref)return gp(e,t,o)}t.flags|=1;var m=fg(d,r);return m.ref=t.ref,m.return=t,t.child=m,m}function Hd(e,t,n,r,a,o){if(t.type!==t.elementType){var i=t.elementType;if(i.$$typeof===ue){var l=i._payload,s=i._init;try{i=s(l)}catch(e){i=null}var c=i&&i.propTypes;c&&Gl(c,r,"prop",We(i))}}if(null!==e&&Xo(e.memoizedProps,r)&&e.ref===t.ref&&t.type===e.type){if(jd=!1,!Ea(o,a))return t.lanes=e.lanes,gp(e,t,o);0!=(e.flags&ur)&&(jd=!0)}return Yd(e,t,n,r,o)}function Vd(e,t,n){var r,a=t.pendingProps,o=a.children,i=null!==e?e.memoizedState:null;if("hidden"===a.mode||"unstable-defer-without-hiding"===a.mode)if(0==(4&t.mode))t.memoizedState={baseLanes:0},Qm(t,n);else{var l;if(!Ea(n,sa))return l=null!==i?Sa(i.baseLanes,n):n,Bh(sa),t.lanes=t.childLanes=sa,t.memoizedState={baseLanes:l},Qm(t,l),null;t.memoizedState={baseLanes:0},Qm(t,null!==i?i.baseLanes:n)}else null!==i?(r=Sa(i.baseLanes,n),t.memoizedState=null):r=n,Qm(t,r);return Fd(e,t,o,n),t.child}kd={},Ad={},Id={},Ld={},_d={},Pd=!1,Md={},Dd={};var Wd=Vd;function Gd(e,t){var n=t.ref;(null===e&&null!==n||null!==e&&e.ref!==n)&&(t.flags|=or)}function Yd(e,t,n,r,a){if(t.type!==t.elementType){var o=n.propTypes;o&&Gl(o,r,"prop",We(n))}var i,l;if(i=os(t,rs(0,n,!0)),Sc(t,a),Ud.current=t,Qe(!0),l=bf(e,t,n,r,i,a),1&t.mode){Ae();try{l=bf(e,t,n,r,i,a)}finally{Ie()}}return Qe(!1),null===e||jd?(t.flags|=1,Fd(e,t,l,a),t.child):(Ef(e,t,a),gp(e,t,a))}function $d(e,t,n,r,a){if(t.type!==t.elementType){var o=n.propTypes;o&&Gl(o,r,"prop",We(n))}var i,l;ls(n)?(i=!0,ds(t)):i=!1,Sc(t,a),null===t.stateNode?(null!==e&&(e.alternate=null,t.alternate=null,t.flags|=2),su(t,n,r),uu(t,n,r,a),l=!0):l=null===e?function(e,t,n,r){var a=e.stateNode,o=e.memoizedProps;a.props=o;var i,l=a.context,s=t.contextType;i="object"==typeof s&&null!==s?wc(s):os(e,rs(0,t,!0));var c=t.getDerivedStateFromProps,u="function"==typeof c||"function"==typeof a.getSnapshotBeforeUpdate;u||"function"!=typeof a.UNSAFE_componentWillReceiveProps&&"function"!=typeof a.componentWillReceiveProps||o===n&&l===i||cu(e,a,n,i),Mc();var f=e.memoizedState,d=a.state=f;if(_c(e,n,a,r),d=e.memoizedState,o===n&&f===d&&!is()&&!Dc())return"function"==typeof a.componentDidMount&&(e.flags|=4),!1;"function"==typeof c&&(Qc(e,t,c,n),d=e.memoizedState);var p=Dc()||iu(e,t,o,n,f,d,i);return p?(u||"function"!=typeof a.UNSAFE_componentWillMount&&"function"!=typeof a.componentWillMount||("function"==typeof a.componentWillMount&&a.componentWillMount(),"function"==typeof a.UNSAFE_componentWillMount&&a.UNSAFE_componentWillMount()),"function"==typeof a.componentDidMount&&(e.flags|=4)):("function"==typeof a.componentDidMount&&(e.flags|=4),e.memoizedProps=n,e.memoizedState=d),a.props=n,a.state=d,a.context=i,p}(t,n,r,a):function(e,t,n,r,a){var o=t.stateNode;xc(e,t);var i=t.memoizedProps,l=t.type===t.elementType?i:sc(t.type,i);o.props=l;var s,c=t.pendingProps,u=o.context,f=n.contextType;s="object"==typeof f&&null!==f?wc(f):os(t,rs(0,n,!0));var d=n.getDerivedStateFromProps,p="function"==typeof d||"function"==typeof o.getSnapshotBeforeUpdate;p||"function"!=typeof o.UNSAFE_componentWillReceiveProps&&"function"!=typeof o.componentWillReceiveProps||i===c&&u===s||cu(t,o,r,s),Mc();var m=t.memoizedState,h=o.state=m;if(_c(t,r,o,a),h=t.memoizedState,i===c&&m===h&&!is()&&!Dc())return"function"==typeof o.componentDidUpdate&&(i===e.memoizedProps&&m===e.memoizedState||(t.flags|=4)),"function"==typeof o.getSnapshotBeforeUpdate&&(i===e.memoizedProps&&m===e.memoizedState||(t.flags|=ir)),!1;"function"==typeof d&&(Qc(t,n,d,r),h=t.memoizedState);var g=Dc()||iu(t,n,l,r,m,h,s);return g?(p||"function"!=typeof o.UNSAFE_componentWillUpdate&&"function"!=typeof o.componentWillUpdate||("function"==typeof o.componentWillUpdate&&o.componentWillUpdate(r,h,s),"function"==typeof o.UNSAFE_componentWillUpdate&&o.UNSAFE_componentWillUpdate(r,h,s)),"function"==typeof o.componentDidUpdate&&(t.flags|=4),"function"==typeof o.getSnapshotBeforeUpdate&&(t.flags|=ir)):("function"==typeof o.componentDidUpdate&&(i===e.memoizedProps&&m===e.memoizedState||(t.flags|=4)),"function"==typeof o.getSnapshotBeforeUpdate&&(i===e.memoizedProps&&m===e.memoizedState||(t.flags|=ir)),t.memoizedProps=r,t.memoizedState=h),o.props=r,o.state=h,o.context=s,g}(e,t,n,r,a);var c=Xd(e,t,n,l,i,a);return l&&t.stateNode.props!==r&&(Pd||s("It looks like %s is reassigning its own `this.props` while rendering. This is not supported and can lead to confusing bugs.",We(t.type)||"a component"),Pd=!0),c}function Xd(e,t,n,r,a,o){Gd(e,t);var i=0!=(t.flags&ar);if(!r&&!i)return a&&ps(t,n,!1),gp(e,t,o);var l,s=t.stateNode;if(Ud.current=t,i&&"function"!=typeof n.getDerivedStateFromError)l=null,Od();else{if(Qe(!0),l=s.render(),1&t.mode){Ae();try{s.render()}finally{Ie()}}Qe(!1)}return t.flags|=1,null!==e&&i?function(e,t,n,r){t.child=gu(t,e.child,null,r),t.child=gu(t,null,n,r)}(e,t,l,o):Fd(e,t,l,o),t.memoizedState=s.state,a&&ps(t,n,!0),t.child}function qd(e){var t=e.stateNode;t.pendingContext?us(e,t.pendingContext,t.pendingContext!==t.context):t.context&&us(e,t.context,!1),Ru(e,t.containerInfo)}function Kd(e,t){if(t&&t.childContextTypes&&s("%s(...): childContextTypes cannot be defined on a function component.",t.displayName||t.name||"Component"),null!==e.ref){var n="",r=Xe();r&&(n+="\n\nCheck the render method of `"+r+"`.");var a=r||e._debugID||"",o=e._debugSource;o&&(a=o.fileName+":"+o.lineNumber),_d[a]||(_d[a]=!0,s("Function components cannot be given refs. Attempts to access this ref will fail. Did you mean to use React.forwardRef()?%s",n))}if("function"==typeof t.getDerivedStateFromProps){var i=We(t)||"Unknown";Ld[i]||(s("%s: Function components do not support getDerivedStateFromProps.",i),Ld[i]=!0)}if("object"==typeof t.contextType&&null!==t.contextType){var l=We(t)||"Unknown";Id[l]||(s("%s: Function components do not support contextType.",l),Id[l]=!0)}}var Jd={dehydrated:null,retryLane:0};function Qd(e){return{baseLanes:e}}function Zd(e,t){return{baseLanes:Sa(e.baseLanes,t)}}function ep(e,t){return wa(e.childLanes,t)}function tp(e,t,n){var r=t.pendingProps;Dg(t)&&(t.flags|=ar);var a=Au.current,o=!1;if(0!=(t.flags&ar)||function(e,t,n,r){return(null===t||null!==t.memoizedState)&&Iu(e,2)}(a,e)?(o=!0,t.flags&=-65):null!==e&&null===e.memoizedState||void 0!==r.fallback&&!0!==r.unstable_avoidThisFallback&&(a|=1),Pu(t,a=Lu(a)),null===e){void 0!==r.fallback&&Wu(t);var i=r.children,l=r.fallback;if(o){var s=np(t,i,l,n);return t.child.memoizedState=Qd(n),t.memoizedState=Jd,s}if("number"==typeof r.unstable_expectedLoadTime){var c=np(t,i,l,n);return t.child.memoizedState=Qd(n),t.memoizedState=Jd,t.lanes=ra,Bh(ra),c}return function(e,t,n){var r=gg({mode:"visible",children:t},e.mode,n,null);return r.return=e,e.child=r,r}(t,i,n)}if(null!==e.memoizedState){if(o){var u=op(e,t,r.children,r.fallback,n),f=t.child,d=e.child.memoizedState;return f.memoizedState=null===d?Qd(n):Zd(d,n),f.childLanes=ep(e,n),t.memoizedState=Jd,u}var p=ap(e,t,r.children,n);return t.memoizedState=null,p}if(o){var m=op(e,t,r.children,r.fallback,n),h=t.child,g=e.child.memoizedState;return h.memoizedState=null===g?Qd(n):Zd(g,n),h.childLanes=ep(e,n),t.memoizedState=Jd,m}var y=ap(e,t,r.children,n);return t.memoizedState=null,y}function np(e,t,n,r){var a,o,i=e.mode,l=e.child,s={mode:"hidden",children:t};return 0==(2&i)&&null!==l?((a=l).childLanes=0,a.pendingProps=s,8&e.mode&&(a.actualDuration=0,a.actualStartTime=-1,a.selfBaseDuration=0,a.treeBaseDuration=0),o=hg(n,i,r,null)):(a=gg(s,i,0,null),o=hg(n,i,r,null)),a.return=e,o.return=e,a.sibling=o,e.child=a,o}function rp(e,t){return fg(e,t)}function ap(e,t,n,r){var a=e.child,o=a.sibling,i=rp(a,{mode:"visible",children:n});return 0==(2&t.mode)&&(i.lanes=r),i.return=t,i.sibling=null,null!==o&&(o.nextEffect=null,o.flags=8,t.firstEffect=t.lastEffect=o),t.child=i,i}function op(e,t,n,r,a){var o,i,l=t.mode,s=e.child,c=s.sibling,u={mode:"hidden",children:n};if(0==(2&l)&&t.child!==s){(o=t.child).childLanes=0,o.pendingProps=u,8&t.mode&&(o.actualDuration=0,o.actualStartTime=-1,o.selfBaseDuration=s.selfBaseDuration,o.treeBaseDuration=s.treeBaseDuration);var f=o.lastEffect;null!==f?(t.firstEffect=o.firstEffect,t.lastEffect=f,f.nextEffect=null):t.firstEffect=t.lastEffect=null}else o=rp(s,u);return null!==c?i=fg(c,r):(i=hg(r,l,a,null)).flags|=2,i.return=t,o.return=t,o.sibling=i,t.child=o,i}function ip(e,t){e.lanes=Sa(e.lanes,t);var n=e.alternate;null!==n&&(n.lanes=Sa(n.lanes,t)),Tc(e.return,t)}function lp(e,t){var n=Array.isArray(e),r=!n&&"function"==typeof Ee(e);if(n||r){var a=n?"array":"iterable";return s("A nested %s was passed to row #%s in <SuspenseList />. Wrap it in an additional SuspenseList to configure its revealOrder: <SuspenseList revealOrder=...> ... <SuspenseList revealOrder=...>{%s}</SuspenseList> ... </SuspenseList>",a,t,a),!1}return!0}function sp(e,t,n,r,a,o){var i=e.memoizedState;null===i?e.memoizedState={isBackwards:t,rendering:null,renderingStartTime:0,last:r,tail:n,tailMode:a,lastEffect:o}:(i.isBackwards=t,i.rendering=null,i.renderingStartTime=0,i.last=r,i.tail=n,i.tailMode=a,i.lastEffect=o)}function cp(e,t,n){var r=t.pendingProps,a=r.revealOrder,o=r.tail,i=r.children;!function(e){if(void 0!==e&&"forwards"!==e&&"backwards"!==e&&"together"!==e&&!Md[e])if(Md[e]=!0,"string"==typeof e)switch(e.toLowerCase()){case"together":case"forwards":case"backwards":s('"%s" is not a valid value for revealOrder on <SuspenseList />. Use lowercase "%s" instead.',e,e.toLowerCase());break;case"forward":case"backward":s('"%s" is not a valid value for revealOrder on <SuspenseList />. React uses the -s suffix in the spelling. Use "%ss" instead.',e,e.toLowerCase());break;default:s('"%s" is not a supported revealOrder on <SuspenseList />. Did you mean "together", "forwards" or "backwards"?',e)}else s('%s is not a supported value for revealOrder on <SuspenseList />. Did you mean "together", "forwards" or "backwards"?',e)}(a),function(e,t){void 0===e||Dd[e]||("collapsed"!==e&&"hidden"!==e?(Dd[e]=!0,s('"%s" is not a supported value for tail on <SuspenseList />. Did you mean "collapsed" or "hidden"?',e)):"forwards"!==t&&"backwards"!==t&&(Dd[e]=!0,s('<SuspenseList tail="%s" /> is only valid if revealOrder is "forwards" or "backwards". Did you mean to specify revealOrder="forwards"?',e)))}(o,a),function(e,t){if(("forwards"===t||"backwards"===t)&&null!=e&&!1!==e)if(Array.isArray(e)){for(var n=0;n<e.length;n++)if(!lp(e[n],n))return}else{var r=Ee(e);if("function"==typeof r){var a=r.call(e);if(a)for(var o=a.next(),i=0;!o.done;o=a.next()){if(!lp(o.value,i))return;i++}}else s('A single row was passed to a <SuspenseList revealOrder="%s" />. This is not useful since it needs multiple rows. Did you mean to pass multiple children or an array?',t)}}(i,a),Fd(e,t,i,n);var l=Au.current;if(Iu(l,2)?(l=_u(l,2),t.flags|=ar):(null!==e&&0!=(e.flags&ar)&&function(e,t,n){for(var r=t;null!==r;){if(r.tag===h)null!==r.memoizedState&&ip(r,n);else if(r.tag===b)ip(r,n);else if(null!==r.child){r.child.return=r,r=r.child;continue}if(r===e)return;for(;null===r.sibling;){if(null===r.return||r.return===e)return;r=r.return}r.sibling.return=r.return,r=r.sibling}}(t,t.child,n),l=Lu(l)),Pu(t,l),0==(2&t.mode))t.memoizedState=null;else switch(a){case"forwards":var c,u=function(e){for(var t=e,n=null;null!==t;){var r=t.alternate;null!==r&&null===Uu(r)&&(n=t),t=t.sibling}return n}(t.child);null===u?(c=t.child,t.child=null):(c=u.sibling,u.sibling=null),sp(t,!1,c,u,o,t.lastEffect);break;case"backwards":var f=null,d=t.child;for(t.child=null;null!==d;){var p=d.alternate;if(null!==p&&null===Uu(p)){t.child=d;break}var m=d.sibling;d.sibling=f,f=d,d=m}sp(t,!0,f,null,o,t.lastEffect);break;case"together":sp(t,!1,null,null,void 0,t.lastEffect);break;default:t.memoizedState=null}return t.child}var up,fp,dp,pp=!1,mp=!1;function hp(){jd=!0}function gp(e,t,n){return null!==e&&(t.dependencies=e.dependencies),Od(),ih(t.lanes),Ea(n,t.childLanes)?(function(e,t){if(null!==e&&t.child!==e.child)throw Error("Resuming work not yet implemented.");if(null!==t.child){var n=t.child,r=fg(n,n.pendingProps);for(t.child=r,r.return=t;null!==n.sibling;)(r=r.sibling=fg(n=n.sibling,n.pendingProps)).return=t;r.sibling=null}}(e,t),t.child):null}function yp(e,t,n){var r=t.lanes;if(t._debugNeedsRemount&&null!==e)return function(e,t,n){var r=t.return;if(null===r)throw new Error("Cannot swap the root fiber.");if(e.alternate=null,t.alternate=null,n.index=t.index,n.sibling=t.sibling,n.return=t.return,n.ref=t.ref,t===r.child)r.child=n;else{var a=r.child;if(null===a)throw new Error("Expected parent to have a child.");for(;a.sibling!==t;)if(null===(a=a.sibling))throw new Error("Expected to find the previous sibling.");a.sibling=n}var o=r.lastEffect;return null!==o?(o.nextEffect=e,r.lastEffect=e):r.firstEffect=r.lastEffect=e,e.nextEffect=null,e.flags=8,n.flags|=2,n}(e,t,pg(t.type,t.key,t.pendingProps,t._debugOwner||null,t.mode,t.lanes));if(null!==e)if(e.memoizedProps!==t.pendingProps||is()||t.type!==e.type)jd=!0;else{if(!Ea(n,r)){switch(jd=!1,t.tag){case 3:qd(t),$u();break;case 5:xu(t);break;case 1:ls(t.type)&&ds(t);break;case 4:Ru(t,t.stateNode.containerInfo);break;case d:bc(t,t.memoizedProps.value);break;case m:Ea(n,t.childLanes)&&(t.flags|=4);var a=t.stateNode;a.effectDuration=0,a.passiveEffectDuration=0;break;case h:if(null!==t.memoizedState){if(Ea(n,t.child.childLanes))return tp(e,t,n);Pu(t,Lu(Au.current));var o=gp(e,t,n);return null!==o?o.sibling:null}Pu(t,Lu(Au.current));break;case b:var i=0!=(e.flags&ar),l=Ea(n,t.childLanes);if(i){if(l)return cp(e,t,n);t.flags|=ar}var c=t.memoizedState;if(null!==c&&(c.rendering=null,c.tail=null,c.lastEffect=null),Pu(t,Au.current),l)break;return null;case S:case w:return t.lanes=0,Vd(e,t,n)}return gp(e,t,n)}jd=0!=(e.flags&ur)}else jd=!1;switch(t.lanes=0,t.tag){case 2:return function(e,t,n,r){null!==e&&(e.alternate=null,t.alternate=null,t.flags|=2);var a,o,i=t.pendingProps;if(a=os(t,rs(0,n,!1)),Sc(t,r),n.prototype&&"function"==typeof n.prototype.render){var l=We(n)||"Unknown";kd[l]||(s("The <%s /> component appears to have a render method, but doesn't extend React.Component. This is likely to cause errors. Change %s to extend React.Component instead.",l,l),kd[l]=!0)}if(1&t.mode&&Js.recordLegacyContextWarning(t,null),Qe(!0),Ud.current=t,o=bf(null,t,n,i,a,r),Qe(!1),t.flags|=1,"object"==typeof o&&null!==o&&"function"==typeof o.render&&void 0===o.$$typeof){var c=We(n)||"Unknown";Ad[c]||(s("The <%s /> component appears to be a function component that returns a class instance. Change %s to a class that extends React.Component instead. If you can't use a class try assigning the prototype on the function as a workaround. `%s.prototype = React.Component.prototype`. Don't use an arrow function since it cannot be called with `new` by React.",c,c,c),Ad[c]=!0)}if("object"==typeof o&&null!==o&&"function"==typeof o.render&&void 0===o.$$typeof){var u=We(n)||"Unknown";Ad[u]||(s("The <%s /> component appears to be a function component that returns a class instance. Change %s to a class that extends React.Component instead. If you can't use a class try assigning the prototype on the function as a workaround. `%s.prototype = React.Component.prototype`. Don't use an arrow function since it cannot be called with `new` by React.",u,u,u),Ad[u]=!0),t.tag=1,t.memoizedState=null,t.updateQueue=null;var f=!1;ls(n)?(f=!0,ds(t)):f=!1,t.memoizedState=null!=o.state?o.state:null,Cc(t);var d=n.getDerivedStateFromProps;return"function"==typeof d&&Qc(t,n,d,i),lu(t,o),uu(t,n,i,r),Xd(null,t,n,!0,f,r)}if(t.tag=0,1&t.mode){Ae();try{o=bf(null,t,n,i,a,r)}finally{Ie()}}return Fd(null,t,o,r),Kd(t,n),t.child}(e,t,t.type,n);case 16:return function(e,t,n,r,a){null!==e&&(e.alternate=null,t.alternate=null,t.flags|=2);var o=t.pendingProps,i=(0,n._init)(n._payload);t.type=i;var l=t.tag=function(e){if("function"==typeof e)return ug(e)?1:0;if(null!=e){var t=e.$$typeof;if(t===ie)return p;if(t===ce)return g}return 2}(i),s=sc(i,o);switch(l){case 0:return Kd(t,i),t.type=i=Jh(i),Yd(null,t,i,s,a);case 1:return t.type=i=Qh(i),$d(null,t,i,s,a);case p:return t.type=i=Zh(i),zd(null,t,i,s,a);case g:if(t.type!==t.elementType){var c=i.propTypes;c&&Gl(c,s,"prop",We(i))}return Bd(null,t,i,sc(i.type,s),r,a)}var u="";throw null!==i&&"object"==typeof i&&i.$$typeof===ue&&(u=" Did you wrap a component in React.lazy() more than once?"),Error("Element type is invalid. Received a promise that resolves to: "+i+". Lazy element type must resolve to a class or function."+u)}(e,t,t.elementType,r,n);case 0:var u=t.type,f=t.pendingProps;return Yd(e,t,u,t.elementType===u?f:sc(u,f),n);case 1:var N=t.type,R=t.pendingProps;return $d(e,t,N,t.elementType===N?R:sc(N,R),n);case 3:return function(e,t,n){if(qd(t),null===e||null===t.updateQueue)throw Error("If the root does not have an updateQueue, we should have already bailed out. This error is likely caused by a bug in React. Please file an issue.");var r=t.pendingProps,a=t.memoizedState,o=null!==a?a.element:null;xc(e,t),_c(t,r,null,n);var i=t.memoizedState.element;if(i===o)return $u(),gp(e,t,n);var l,s=t.stateNode;if(s.hydrate&&(Fu=Nl((l=t).stateNode.containerInfo),ju=l,zu=!0,1)){var c=s.mutableSourceEagerHydrationData;if(null!=c)for(var u=0;u<c.length;u+=2)Qu(c[u],c[u+1]);var f=yu(t,null,i,n);t.child=f;for(var d=f;d;)d.flags=-3&d.flags|1024,d=d.sibling}else Fd(e,t,i,n),$u();return t.child}(e,t,n);case 5:return function(e,t,n){xu(t),null===e&&Wu(t);var r=t.type,a=t.pendingProps,o=null!==e?e.memoizedProps:null,i=a.children;return ml(r,a)?i=null:null!==o&&ml(r,o)&&(t.flags|=16),Gd(e,t),Fd(e,t,i,n),t.child}(e,t,n);case 6:return function(e,t){return null===e&&Wu(t),null}(e,t);case h:return tp(e,t,n);case 4:return function(e,t,n){Ru(t,t.stateNode.containerInfo);var r=t.pendingProps;return null===e?t.child=gu(t,null,r,n):Fd(e,t,r,n),t.child}(e,t,n);case p:var O=t.type,C=t.pendingProps;return zd(e,t,O,t.elementType===O?C:sc(O,C),n);case 7:return function(e,t,n){return Fd(e,t,t.pendingProps,n),t.child}(e,t,n);case 8:return function(e,t,n){return Fd(e,t,t.pendingProps.children,n),t.child}(e,t,n);case m:return function(e,t,n){t.flags|=4;var r=t.stateNode;return r.effectDuration=0,r.passiveEffectDuration=0,Fd(e,t,t.pendingProps.children,n),t.child}(e,t,n);case d:return function(e,t,n){var r=t.type._context,a=t.pendingProps,o=t.memoizedProps,i=a.value;"value"in a||pp||(pp=!0,s("The `value` prop is required for the `<Context.Provider>`. Did you misspell it or forget to pass it?"));var l=t.type.propTypes;if(l&&Gl(l,a,"prop","Context.Provider"),bc(t,i),null!==o){var c=function(e,t,n){if(Yo(n,t))return 0;var r="function"==typeof e._calculateChangedBits?e._calculateChangedBits(n,t):uc;return(r&uc)!==r&&s("calculateChangedBits: Expected the return value to be a 31-bit integer. Instead received: %s",r),0|r}(r,i,o.value);if(0===c){if(o.children===a.children&&!is())return gp(e,t,n)}else!function(e,t,n,r){var a=e.child;for(null!==a&&(a.return=e);null!==a;){var o=void 0,i=a.dependencies;if(null!==i){o=a.child;for(var l=i.firstContext;null!==l;){if(l.context===t&&0!=(l.observedBits&n)){if(1===a.tag){var s=kc(ca,va(r));s.tag=2,Ac(a,s)}a.lanes=Sa(a.lanes,r);var c=a.alternate;null!==c&&(c.lanes=Sa(c.lanes,r)),Tc(a.return,r),i.lanes=Sa(i.lanes,r);break}l=l.next}}else o=a.tag===d&&a.type===e.type?null:a.child;if(null!==o)o.return=a;else for(o=a;null!==o;){if(o===e){o=null;break}var u=o.sibling;if(null!==u){u.return=o.return,o=u;break}o=o.return}a=o}}(t,r,c,n)}return Fd(e,t,a.children,n),t.child}(e,t,n);case 9:return function(e,t,n){var r=t.type;void 0===r._context?r!==r.Consumer&&(mp||(mp=!0,s("Rendering <Context> directly is not supported and will be removed in a future major release. Did you mean to render <Context.Consumer> instead?"))):r=r._context;var a=t.pendingProps,o=a.children;"function"!=typeof o&&s("A context consumer was rendered with multiple children, or a child that isn't a function. A context consumer expects a single child that is a function. If you did pass a function, make sure there is no trailing or leading whitespace around it."),Sc(t,n);var i,l=wc(r,a.unstable_observedBits);return Ud.current=t,Qe(!0),i=o(l),Qe(!1),t.flags|=1,Fd(e,t,i,n),t.child}(e,t,n);case g:var x=t.type,k=sc(x,t.pendingProps);if(t.type!==t.elementType){var A=x.propTypes;A&&Gl(A,k,"prop",We(x))}return Bd(e,t,x,k=sc(x.type,k),r,n);case y:return Hd(e,t,t.type,t.pendingProps,r,n);case v:var I=t.type,L=t.pendingProps;return function(e,t,n,r,a){var o;return null!==e&&(e.alternate=null,t.alternate=null,t.flags|=2),t.tag=1,ls(n)?(o=!0,ds(t)):o=!1,Sc(t,a),su(t,n,r),uu(t,n,r,a),Xd(null,t,n,!0,o,a)}(e,t,I,t.elementType===I?L:sc(I,L),n);case b:return cp(e,t,n);case E:case 21:case T:break;case S:return Vd(e,t,n);case w:return Wd(e,t,n)}throw Error("Unknown unit of work tag ("+t.tag+"). This error is likely caused by a bug in React. Please file an issue.")}function vp(e){e.flags|=4}function bp(e){e.flags|=or}function Ep(e,t){if(!Xu())switch(e.tailMode){case"hidden":for(var n=e.tail,r=null;null!==n;)null!==n.alternate&&(r=n),n=n.sibling;null===r?e.tail=null:r.sibling=null;break;case"collapsed":for(var a=e.tail,o=null;null!==a;)null!==a.alternate&&(o=a),a=a.sibling;null===o?t||null===e.tail?e.tail=null:e.tail.sibling=null:o.sibling=null}}function Tp(e,t,n){var r,a,o=t.pendingProps;switch(t.tag){case 2:case 16:case y:case 0:case p:case 7:case 8:case m:case 9:case g:return null;case 1:return ls(t.type)&&ss(t),null;case 3:Ou(t),cs(t),Ju();var i=t.stateNode;return i.pendingContext&&(i.context=i.pendingContext,i.pendingContext=null),(null===e||null===e.child)&&(Yu(t)?vp(t):i.hydrate||(t.flags|=ir)),null;case 5:ku(t);var l=Nu(),c=t.type;if(null!==e&&null!=t.stateNode)fp(e,t,c,o),e.ref!==t.ref&&bp(t);else{if(!o){if(null===t.stateNode)throw Error("We must have new props for new mounts. This error is likely caused by a bug in React. Please file an issue.");return null}var u=Cu();if(Yu(t))a=function(e,t,n,r,a,o){return _l(o,e),zl(e,n),function(e,t,n,r,a){var o,i;switch(xi=!0===n.suppressHydrationWarning,o=sn(t,n),ki(t,n),t){case"dialog":hi("cancel",e),hi("close",e);break;case"iframe":case"object":case"embed":hi("load",e);break;case"video":case"audio":for(var l=0;l<ui.length;l++)hi(ui[l],e);break;case"source":hi("error",e);break;case"img":case"image":case"link":hi("error",e),hi("load",e);break;case"details":hi("toggle",e);break;case"input":mt(e,n),hi("invalid",e);break;case"option":St(0,n);break;case"select":xt(e,n),hi("invalid",e);break;case"textarea":It(e,n),hi("invalid",e)}ln(t,n),i=new Set;for(var s=e.attributes,c=0;c<s.length;c++)switch(s[c].name.toLowerCase()){case"data-reactroot":case"value":case"checked":case"selected":break;default:i.add(s[c].name)}var u,f=null;for(var d in n)if(n.hasOwnProperty(d)){var p=n[d];if(d===Hi)"string"==typeof p?e.textContent!==p&&(xi||Ai(e.textContent,p),f=[Hi,p]):"number"==typeof p&&e.textContent!==""+p&&(xi||Ai(e.textContent,p),f=[Hi,""+p]);else if(O.hasOwnProperty(d))null!=p&&("function"!=typeof p&&_i(d,p),"onScroll"===d&&hi("scroll",e));else if("boolean"==typeof o){var m=void 0,h=H(d);if(xi);else if(d===Fi||d===zi||"value"===d||"checked"===d||"selected"===d);else if(d===ji){var g=e.innerHTML,y=p?p.__html:void 0;if(null!=y){var v=Di(e,y);v!==g&&Ii(d,g,v)}}else if(d===Vi){if(i.delete(d),Pi){var b=nn(p);b!==(m=e.getAttribute("style"))&&Ii(d,m,b)}}else if(o)i.delete(d.toLowerCase()),p!==(m=J(e,d,p))&&Ii(d,m,p);else if(!F(d,h,o)&&!B(d,p,h,o)){var E=!1;if(null!==h)i.delete(h.attributeName),m=K(e,d,p,h);else{var T=r;if(T===Wi&&(T=Dt(t)),T===Wi)i.delete(d.toLowerCase());else{var S=(u=d.toLowerCase(),cn.hasOwnProperty(u)&&cn[u]||null);null!==S&&S!==d&&(E=!0,i.delete(S)),i.delete(d)}m=J(e,d,p)}p===m||E||Ii(d,m,p)}}}switch(i.size>0&&!xi&&Li(i),t){case"input":ot(e),yt(e,n,!0);break;case"textarea":ot(e),_t(e);break;case"select":case"option":break;default:"function"==typeof n.onClick&&qi(e)}return f}(e,t,n,a.namespace)}((r=t).stateNode,r.type,r.memoizedProps,0,u,r),r.updateQueue=a,null!==a&&vp(t);else{var f=function(e,t,n,r,a){var o=r;if(tl(e,null,o.ancestorInfo),"string"==typeof t.children||"number"==typeof t.children){var i=""+t.children,l=nl(o.ancestorInfo,e);tl(null,i,l)}var c=function(e,t,n,r){var a,o,i=$i(n),l=r;if(l===Wi&&(l=Dt(e)),l===Wi){if((a=sn(e,t))||e===e.toLowerCase()||s("<%s /> is using incorrect casing. Use PascalCase for React components, or lowercase for HTML elements.",e),"script"===e){var c=i.createElement("div");c.innerHTML="<script><\/script>",o=c.removeChild(c.firstChild)}else if("string"==typeof t.is)o=i.createElement(e,{is:t.is});else if(o=i.createElement(e),"select"===e){var u=o;t.multiple?u.multiple=!0:t.size&&(u.size=t.size)}}else o=i.createElementNS(l,e);return l===Wi&&(a||"[object HTMLUnknownElement]"!==Object.prototype.toString.call(o)||Object.prototype.hasOwnProperty.call(Ci,e)||(Ci[e]=!0,s("The tag <%s> is unrecognized in this browser. If you meant to render a React component, start its name with an uppercase letter.",e))),o}(e,t,n,o.namespace);return _l(a,c),zl(c,t),c}(c,o,l,u,t);up(f,t),t.stateNode=f,function(e,t,n,r,a){return function(e,t,n,r){var a,o=sn(t,n);switch(ki(t,n),t){case"dialog":hi("cancel",e),hi("close",e),a=n;break;case"iframe":case"object":case"embed":hi("load",e),a=n;break;case"video":case"audio":for(var i=0;i<ui.length;i++)hi(ui[i],e);a=n;break;case"source":hi("error",e),a=n;break;case"img":case"image":case"link":hi("error",e),hi("load",e),a=n;break;case"details":hi("toggle",e),a=n;break;case"input":mt(e,n),a=pt(e,n),hi("invalid",e);break;case"option":St(0,n),a=wt(0,n);break;case"select":xt(e,n),a=Ct(0,n),hi("invalid",e);break;case"textarea":It(e,n),a=At(e,n),hi("invalid",e);break;default:a=n}switch(ln(t,a),function(e,t,n,r,a){for(var o in r)if(r.hasOwnProperty(o)){var i=r[o];if(o===Vi)i&&Object.freeze(i),rn(t,i);else if(o===ji){var l=i?i.__html:void 0;null!=l&&zt(t,l)}else o===Hi?"string"==typeof i?("textarea"!==e||""!==i)&&Bt(t,i):"number"==typeof i&&Bt(t,""+i):o===Fi||o===zi||o===Bi||(O.hasOwnProperty(o)?null!=i&&("function"!=typeof i&&_i(o,i),"onScroll"===o&&hi("scroll",t)):null!=i&&Q(t,o,i,a))}}(t,e,0,a,o),t){case"input":ot(e),yt(e,n,!1);break;case"textarea":ot(e),_t(e);break;case"option":!function(e,t){null!=t.value&&e.setAttribute("value",Ze(et(t.value)))}(e,n);break;case"select":!function(e,t){var n=e;n.multiple=!!t.multiple;var r=t.value;null!=r?Ot(n,!!t.multiple,r,!1):null!=t.defaultValue&&Ot(n,!!t.multiple,t.defaultValue,!0)}(e,n);break;default:"function"==typeof a.onClick&&qi(e)}}(e,t,n),pl(t,n)}(f,c,o)&&vp(t)}null!==t.ref&&bp(t)}return null;case 6:var N=o;if(e&&null!=t.stateNode)dp(0,t,e.memoizedProps,N);else{if("string"!=typeof N&&null===t.stateNode)throw Error("We must have new props for new mounts. This error is likely caused by a bug in React. Please file an issue.");var R=Nu(),C=Cu();Yu(t)?function(e){var t=e.stateNode,n=e.memoizedProps,r=function(e,t,n){return _l(n,e),function(e,t){return e.nodeValue!==t}(e,t)}(t,n,e);if(r){var a=ju;if(null!==a)switch(a.tag){case 3:!function(e,t,n){Ki(t,n)}(0,t,n);break;case 5:!function(e,t,n,r,a){!0!==t.suppressHydrationWarning&&Ki(r,a)}(0,a.memoizedProps,0,t,n)}}return r}(t)&&vp(t):t.stateNode=function(e,t,n,r){tl(null,e,n.ancestorInfo);var a=function(e,t){return $i(t).createTextNode(e)}(e,t);return _l(r,a),a}(N,R,C,t)}return null;case h:Mu(t);var x=t.memoizedState;if(0!=(t.flags&ar))return t.lanes=n,0!=(8&t.mode)&&xd(t),t;var k=null!==x,A=!1;return null===e?void 0!==t.memoizedProps.fallback&&Yu(t):A=null!==e.memoizedState,k&&!A&&0!=(2&t.mode)&&(null===e&&!0!==t.memoizedProps.unstable_avoidThisFallback||Iu(Au.current,1)?0===fm&&(fm=3):(0!==fm&&3!==fm||(fm=4),null!==im&&(ha(mm)||ha(hm))&&$m(im,sm))),(k||A)&&(t.flags|=4),null;case 4:return Ou(t),null===e&&yi(t.stateNode.containerInfo),null;case d:return Ec(t),null;case v:return ls(t.type)&&ss(t),null;case b:Mu(t);var I=t.memoizedState;if(null===I)return null;var L=0!=(t.flags&ar),_=I.rendering;if(null===_)if(L)Ep(I,!1);else{if(0!==fm||null!==e&&0!=(e.flags&ar))for(var P=t.child;null!==P;){var M=Uu(P);if(null!==M){L=!0,t.flags|=ar,Ep(I,!1);var D=M.updateQueue;return null!==D&&(t.updateQueue=D,t.flags|=4),null===I.lastEffect&&(t.firstEffect=null),t.lastEffect=I.lastEffect,vu(t,n),Pu(t,_u(Au.current,2)),t.child}P=P.sibling}null!==I.tail&&Bs()>Tm()&&(t.flags|=ar,L=!0,Ep(I,!1),t.lanes=ra,Bh(ra))}else{if(!L){var U=Uu(_);if(null!==U){t.flags|=ar,L=!0;var j=U.updateQueue;if(null!==j&&(t.updateQueue=j,t.flags|=4),Ep(I,!0),null===I.tail&&"hidden"===I.tailMode&&!_.alternate&&!Xu()){var z=t.lastEffect=I.lastEffect;return null!==z&&(z.nextEffect=null),null}}else 2*Bs()-I.renderingStartTime>Tm()&&n!==sa&&(t.flags|=ar,L=!0,Ep(I,!1),t.lanes=ra,Bh(ra))}if(I.isBackwards)_.sibling=t.child,t.child=_;else{var V=I.last;null!==V?V.sibling=_:t.child=_,I.last=_}}if(null!==I.tail){var W=I.tail;I.rendering=W,I.tail=W.sibling,I.lastEffect=t.lastEffect,I.renderingStartTime=Bs(),W.sibling=null;var G=Au.current;return Pu(t,G=L?_u(G,2):Lu(G)),W}return null;case E:case 21:case T:break;case S:case w:return Zm(t),null!==e&&null!==e.memoizedState!=(null!==t.memoizedState)&&"unstable-defer-without-hiding"!==o.mode&&(t.flags|=4),null}throw Error("Unknown unit of work tag ("+t.tag+"). This error is likely caused by a bug in React. Please file an issue.")}function Sp(e,t){switch(e.tag){case 1:ls(e.type)&&ss(e);var n=e.flags;return n&cr?(e.flags=-4097&n|ar,0!=(8&e.mode)&&xd(e),e):null;case 3:Ou(e),cs(e),Ju();var r=e.flags;if(0!=(r&ar))throw Error("The root failed to unmount after an error. This is likely a bug in React. Please file an issue.");return e.flags=-4097&r|ar,e;case 5:return ku(e),null;case h:Mu(e);var a=e.flags;return a&cr?(e.flags=-4097&a|ar,0!=(8&e.mode)&&xd(e),e):null;case b:return Mu(e),null;case 4:return Ou(e),null;case d:return Ec(e),null;case S:case w:return Zm(e),null;default:return null}}function wp(e){switch(e.tag){case 1:null!=e.type.childContextTypes&&ss(e);break;case 3:Ou(e),cs(e),Ju();break;case 5:ku(e);break;case 4:Ou(e);break;case h:case b:Mu(e);break;case d:Ec(e);break;case S:case w:Zm(e)}}function Np(e,t){return{value:e,source:t,stack:He(t)}}function Rp(e,t){try{var n=t.value,r=t.source,a=t.stack,o=null!==a?a:"";if(null!=n&&n._suppressLogging){if(1===e.tag)return;console.error(n)}var i=r?We(r.type):null,l=i?"The above error occurred in the <"+i+"> component:":"The above error occurred in one of your React components:",s=We(e.type);console.error(l+"\n"+o+"\n\n"+(s?"React will try to recreate this component tree from scratch using the error boundary you provided, "+s+".":"Consider adding an error boundary to your tree to customize error handling behavior.\nVisit https://reactjs.org/link/error-boundaries to learn more about error boundaries."))}catch(e){setTimeout(function(){throw e})}}up=function(e,t,n,r){for(var a=t.child;null!==a;){if(5===a.tag||6===a.tag)e.appendChild(a.stateNode);else if(4===a.tag);else if(null!==a.child){a.child.return=a,a=a.child;continue}if(a===t)return;for(;null===a.sibling;){if(null===a.return||a.return===t)return;a=a.return}a.sibling.return=a.return,a=a.sibling}},fp=function(e,t,n,r,a){var o=e.memoizedProps;if(o!==r){var i=function(e,t,n,r,a,o){if(typeof r.children!=typeof n.children&&("string"==typeof r.children||"number"==typeof r.children)){var i=""+r.children,l=nl(o.ancestorInfo,t);tl(null,i,l)}return function(e,t,n,r,a){ki(t,r);var o,i,l,c,u=null;switch(t){case"input":o=pt(e,n),i=pt(e,r),u=[];break;case"option":o=wt(0,n),i=wt(0,r),u=[];break;case"select":o=Ct(0,n),i=Ct(0,r),u=[];break;case"textarea":o=At(e,n),i=At(e,r),u=[];break;default:i=r,"function"!=typeof(o=n).onClick&&"function"==typeof i.onClick&&qi(e)}ln(t,i);var f=null;for(l in o)if(!i.hasOwnProperty(l)&&o.hasOwnProperty(l)&&null!=o[l])if(l===Vi){var d=o[l];for(c in d)d.hasOwnProperty(c)&&(f||(f={}),f[c]="")}else l===ji||l===Hi||l===Fi||l===zi||l===Bi||(O.hasOwnProperty(l)?u||(u=[]):(u=u||[]).push(l,null));for(l in i){var p=i[l],m=null!=o?o[l]:void 0;if(i.hasOwnProperty(l)&&p!==m&&(null!=p||null!=m))if(l===Vi)if(p&&Object.freeze(p),m){for(c in m)!m.hasOwnProperty(c)||p&&p.hasOwnProperty(c)||(f||(f={}),f[c]="");for(c in p)p.hasOwnProperty(c)&&m[c]!==p[c]&&(f||(f={}),f[c]=p[c])}else f||(u||(u=[]),u.push(l,f)),f=p;else if(l===ji){var h=p?p.__html:void 0;null!=h&&(m?m.__html:void 0)!==h&&(u=u||[]).push(l,h)}else l===Hi?"string"!=typeof p&&"number"!=typeof p||(u=u||[]).push(l,""+p):l===Fi||l===zi||(O.hasOwnProperty(l)?(null!=p&&("function"!=typeof p&&_i(l,p),"onScroll"===l&&hi("scroll",e)),u||m===p||(u=[])):"object"==typeof p&&null!==p&&p.$$typeof===me?p.toString():(u=u||[]).push(l,p))}return f&&(function(e,t){if(t){var n,r=an(e),a=an(t),o={};for(var i in r){var l=r[i],c=a[i];if(c&&l!==c){var u=l+","+c;if(o[u])continue;o[u]=!0,s("%s a style property during rerender (%s) when a conflicting property is set (%s) can lead to styling bugs. To avoid this, don't mix shorthand and non-shorthand properties for the same value; instead, replace the shorthand with separate values.",null==(n=e[l])||"boolean"==typeof n||""===n?"Removing":"Updating",l,c)}}}}(f,i.style),(u=u||[]).push(Vi,f)),u}(e,t,n,r)}(t.stateNode,n,o,r,0,Cu());t.updateQueue=i,i&&vp(t)}},dp=function(e,t,n,r){n!==r&&vp(t)};var Op,Cp="function"==typeof WeakMap?WeakMap:Map;function xp(e,t,n){var r=kc(ca,n);r.tag=3,r.payload={element:null};var a=t.value;return r.callback=function(){Nh(a),Rp(e,t)},r}function kp(e,t,n){var r=kc(ca,n);r.tag=3;var a=e.type.getDerivedStateFromError;if("function"==typeof a){var o=t.value;r.payload=function(){return Rp(e,t),a(o)}}var i=e.stateNode;return r.callback=null!==i&&"function"==typeof i.componentDidCatch?function(){tg(e),"function"!=typeof a&&(null===Rm?Rm=new Set([this]):Rm.add(this),Rp(e,t));var n=t.stack;this.componentDidCatch(t.value,{componentStack:null!==n?n:""}),"function"!=typeof a&&(Ea(e.lanes,1)||s("%s: Error boundaries should implement getDerivedStateFromError(). In that method, return a state update to display an error message or fallback UI.",We(e.type)||"Unknown"))}:function(){tg(e)},r}function Ap(e,t,n){var r,a=e.pingCache;if(null===a?(a=e.pingCache=new Cp,r=new Set,a.set(t,r)):void 0===(r=a.get(t))&&(r=new Set,a.set(t,r)),!r.has(n)){r.add(n);var o=Ch.bind(null,e,t,n);t.then(o,o)}}function Ip(e,t,n,r,a){if(n.flags|=sr,n.firstEffect=n.lastEffect=null,null!==r&&"object"==typeof r&&"function"==typeof r.then){var o=r;if(0==(2&n.mode)){var i=n.alternate;i?(n.updateQueue=i.updateQueue,n.memoizedState=i.memoizedState,n.lanes=i.lanes):(n.updateQueue=null,n.memoizedState=null)}var l=Iu(Au.current,1),s=t;do{if(s.tag===h&&Du(s,l)){var c=s.updateQueue;if(null===c){var u=new Set;u.add(o),s.updateQueue=u}else c.add(o);if(0==(2&s.mode)){if(s.flags|=ar,n.flags|=ur,n.flags&=-2981,1===n.tag)if(null===n.alternate)n.tag=v;else{var f=kc(ca,1);f.tag=2,Ac(n,f)}return void(n.lanes=Sa(n.lanes,1))}return Ap(e,o,a),s.flags|=cr,void(s.lanes=a)}s=s.return}while(null!==s);r=new Error((We(n.type)||"A React component")+" suspended while rendering, but no fallback UI was specified.\n\nAdd a <Suspense fallback=...> component higher in the tree to provide a loading indicator or placeholder to display.")}5!==fm&&(fm=2),r=Np(r,n);var d=t;do{switch(d.tag){case 3:var p=r;d.flags|=cr;var m=va(a);return d.lanes=Sa(d.lanes,m),void Ic(d,xp(d,p,m));case 1:var g=r,y=d.stateNode;if(0==(d.flags&ar)&&("function"==typeof d.type.getDerivedStateFromError||null!==y&&"function"==typeof y.componentDidCatch&&!wh(y))){d.flags|=cr;var b=va(a);return d.lanes=Sa(d.lanes,b),void Ic(d,kp(d,g,b))}}d=d.return}while(null!==d)}Op=new Set;var Lp="function"==typeof WeakSet?WeakSet:Set,_p=function(e,t){t.props=e.memoizedProps,t.state=e.memoizedState,t.componentWillUnmount()};function Pp(e){var t=e.ref;null!==t&&("function"==typeof t?(Kn(null,t,null,null),Jn()&&Oh(e,Qn())):t.current=null)}function Mp(e,t){Kn(null,t,null),Jn()&&Oh(e,Qn())}function Dp(e,t){switch(t.tag){case 0:case p:case y:case T:return;case 1:if(t.flags&ir&&null!==e){var n=e.memoizedProps,r=e.memoizedState,a=t.stateNode;t.type!==t.elementType||Pd||(a.props!==t.memoizedProps&&s("Expected %s props to match memoized props before getSnapshotBeforeUpdate. This might either be because of a bug in React, or because a component reassigns its own `this.props`. Please file an issue.",We(t.type)||"instance"),a.state!==t.memoizedState&&s("Expected %s state to match memoized state before getSnapshotBeforeUpdate. This might either be because of a bug in React, or because a component reassigns its own `this.state`. Please file an issue.",We(t.type)||"instance"));var o=a.getSnapshotBeforeUpdate(t.elementType===t.type?n:sc(t.type,n),r),i=Op;void 0!==o||i.has(t.type)||(i.add(t.type),s("%s.getSnapshotBeforeUpdate(): A snapshot value (or null) must be returned. You have returned undefined.",We(t.type))),a.__reactInternalSnapshotBeforeUpdate=o}return;case 3:return void(t.flags&ir&&Tl(t.stateNode.containerInfo));case 5:case 6:case 4:case v:return}throw Error("This unit of work tag should not have side-effects. This error is likely caused by a bug in React. Please file an issue.")}function Up(e,t,n,r){switch(n.tag){case 0:case p:case y:case T:return function(e,t){var n=t.updateQueue,r=null!==n?n.lastEffect:null;if(null!==r){var a=r.next,o=a;do{if(3==(3&o.tag)){o.destroy=(0,o.create)();var i=o.destroy;void 0!==i&&"function"!=typeof i&&s("An effect function must not return anything besides a function, which is used for clean-up.%s",null===i?" You returned null. If your effect does not require clean up, return undefined (or nothing).":"function"==typeof i.then?"\n\nIt looks like you wrote useEffect(async () => ...) or returned a Promise. Instead, write the async function inside your effect and call it immediately:\n\nuseEffect(() => {\n async function fetchData() {\n // You can await here\n const response = await MyAPI.getData(someId);\n // ...\n }\n fetchData();\n}, [someId]); // Or [] if effect doesn't need props or state\n\nLearn more about data fetching with Hooks: https://reactjs.org/link/hooks-data-fetching":" You returned: "+i)}o=o.next}while(o!==a)}}(0,n),void function(e){var t=e.updateQueue,n=null!==t?t.lastEffect:null;if(null!==n){var r=n.next,a=r;do{var o=a.next,i=a.tag;0!=(4&i)&&0!=(1&i)&&(Eh(e,a),bh(e,a)),a=o}while(a!==r)}}(n);case 1:var a=n.stateNode;if(4&n.flags)if(null===t)n.type!==n.elementType||Pd||(a.props!==n.memoizedProps&&s("Expected %s props to match memoized props before componentDidMount. This might either be because of a bug in React, or because a component reassigns its own `this.props`. Please file an issue.",We(n.type)||"instance"),a.state!==n.memoizedState&&s("Expected %s state to match memoized state before componentDidMount. This might either be because of a bug in React, or because a component reassigns its own `this.state`. Please file an issue.",We(n.type)||"instance")),a.componentDidMount();else{var o=n.elementType===n.type?t.memoizedProps:sc(n.type,t.memoizedProps),i=t.memoizedState;n.type!==n.elementType||Pd||(a.props!==n.memoizedProps&&s("Expected %s props to match memoized props before componentDidUpdate. This might either be because of a bug in React, or because a component reassigns its own `this.props`. Please file an issue.",We(n.type)||"instance"),a.state!==n.memoizedState&&s("Expected %s state to match memoized state before componentDidUpdate. This might either be because of a bug in React, or because a component reassigns its own `this.state`. Please file an issue.",We(n.type)||"instance")),a.componentDidUpdate(o,i,a.__reactInternalSnapshotBeforeUpdate)}var l=n.updateQueue;return void(null!==l&&(n.type!==n.elementType||Pd||(a.props!==n.memoizedProps&&s("Expected %s props to match memoized props before processing the update queue. This might either be because of a bug in React, or because a component reassigns its own `this.props`. Please file an issue.",We(n.type)||"instance"),a.state!==n.memoizedState&&s("Expected %s state to match memoized state before processing the update queue. This might either be because of a bug in React, or because a component reassigns its own `this.state`. Please file an issue.",We(n.type)||"instance")),Uc(0,l,a)));case 3:var c=n.updateQueue;if(null!==c){var u=null;if(null!==n.child)switch(n.child.tag){case 5:case 1:u=n.child.stateNode}Uc(0,c,u)}return;case 5:return void(null===t&&4&n.flags&&(g=n.stateNode,N=n.type,R=n.memoizedProps,pl(N,R)&&g.focus()));case 6:case 4:return;case m:var f=n.memoizedProps.onRender,d=wd();return void("function"==typeof f&&f(n.memoizedProps.id,null===t?"mount":"update",n.actualDuration,n.treeBaseDuration,n.actualStartTime,d,e.memoizedInteractions));case h:return void function(e,t){if(null===t.memoizedState){var n=t.alternate;if(null!==n){var r=n.memoizedState;if(null!==r){var a=r.dehydrated;null!==a&&function(e){Ur(e)}(a)}}}}(0,n);case b:case v:case E:case 21:case S:case w:return}var g,N,R;throw Error("This unit of work tag should not have side-effects. This error is likely caused by a bug in React. Please file an issue.")}function jp(e,t){for(var n=e;;){if(5===n.tag)t?bl(n.stateNode):El(n.stateNode,n.memoizedProps);else if(6===n.tag)n.stateNode.nodeValue=t?"":n.memoizedProps;else if((n.tag!==S&&n.tag!==w||null===n.memoizedState||n===e)&&null!==n.child){n.child.return=n,n=n.child;continue}if(n===e)return;for(;null===n.sibling;){if(null===n.return||n.return===e)return;n=n.return}n.sibling.return=n.return,n=n.sibling}}function Fp(e){var t=e.ref;if(null!==t){var n,r=e.stateNode;switch(e.tag){case 5:n=r;break;default:n=r}"function"==typeof t?t(n):(t.hasOwnProperty("current")||s("Unexpected ref object provided for %s. Use either a ref-setter function or React.createRef().",We(e.type)),t.current=n)}}function zp(e){var t=e.ref;null!==t&&("function"==typeof t?t(null):t.current=null)}function Bp(e,t,n){switch(function(e){if(hs&&"function"==typeof hs.onCommitFiberUnmount)try{hs.onCommitFiberUnmount(ms,e)}catch(e){gs||(gs=!0,s("React instrumentation encountered an error: %s",e))}}(t),t.tag){case 0:case p:case g:case y:case T:var r=t.updateQueue;if(null!==r){var a=r.lastEffect;if(null!==a){var o=a.next,i=o;do{var l=i.destroy;void 0!==l&&(0!=(4&i.tag)?Eh(t,i):Mp(t,l)),i=i.next}while(i!==o)}}return;case 1:Pp(t);var c=t.stateNode;return void("function"==typeof c.componentWillUnmount&&function(e,t){Kn(null,_p,null,e,t),Jn()&&Oh(e,Qn())}(t,c));case 5:return void Pp(t);case 4:return void Xp(e,t);case E:case 18:case 21:return}}function Hp(e,t,n){for(var r=t;;)if(Bp(e,r),null===r.child||4===r.tag){if(r===t)return;for(;null===r.sibling;){if(null===r.return||r.return===t)return;r=r.return}r.sibling.return=r.return,r=r.sibling}else r.child.return=r,r=r.child}function Vp(e){e.alternate=null,e.child=null,e.dependencies=null,e.firstEffect=null,e.lastEffect=null,e.memoizedProps=null,e.memoizedState=null,e.pendingProps=null,e.return=null,e.updateQueue=null,e._debugOwner=null}function Wp(e){return 5===e.tag||3===e.tag||4===e.tag}function Gp(e){var t,n,r=function(e){for(var t=e.return;null!==t;){if(Wp(t))return t;t=t.return}throw Error("Expected to find a host parent. This error is likely caused by a bug in React. Please file an issue.")}(e),a=r.stateNode;switch(r.tag){case 5:t=a,n=!1;break;case 3:case 4:t=a.containerInfo,n=!0;break;case E:default:throw Error("Invalid host parent fiber. This error is likely caused by a bug in React. Please file an issue.")}16&r.flags&&(yl(t),r.flags&=-17);var o=function(e){var t=e;e:for(;;){for(;null===t.sibling;){if(null===t.return||Wp(t.return))return null;t=t.return}for(t.sibling.return=t.return,t=t.sibling;5!==t.tag&&6!==t.tag&&18!==t.tag;){if(2&t.flags)continue e;if(null===t.child||4===t.tag)continue e;t.child.return=t,t=t.child}if(!(2&t.flags))return t.stateNode}}(e);n?Yp(e,o,t):$p(e,o,t)}function Yp(e,t,n){var r=e.tag,a=5===r||6===r;if(a){var o=a?e.stateNode:e.stateNode.instance;t?function(e,t,n){8===e.nodeType?e.parentNode.insertBefore(t,n):e.insertBefore(t,n)}(n,o,t):function(e,t){var n;8===e.nodeType?(n=e.parentNode).insertBefore(t,e):(n=e).appendChild(t),null==e._reactRootContainer&&null===n.onclick&&qi(n)}(n,o)}else if(4===r);else{var i=e.child;if(null!==i){Yp(i,t,n);for(var l=i.sibling;null!==l;)Yp(l,t,n),l=l.sibling}}}function $p(e,t,n){var r=e.tag,a=5===r||6===r;if(a){var o=a?e.stateNode:e.stateNode.instance;t?function(e,t,n){e.insertBefore(t,n)}(n,o,t):function(e,t){e.appendChild(t)}(n,o)}else if(4===r);else{var i=e.child;if(null!==i){$p(i,t,n);for(var l=i.sibling;null!==l;)$p(l,t,n),l=l.sibling}}}function Xp(e,t,n){for(var r,a,o,i,l=t,s=!1;;){if(!s){var c=l.return;e:for(;;){if(null===c)throw Error("Expected to find a host parent. This error is likely caused by a bug in React. Please file an issue.");var u=c.stateNode;switch(c.tag){case 5:r=u,a=!1;break e;case 3:case 4:r=u.containerInfo,a=!0;break e}c=c.return}s=!0}if(5===l.tag||6===l.tag)Hp(e,l),a?(i=l.stateNode,8===(o=r).nodeType?o.parentNode.removeChild(i):o.removeChild(i)):vl(r,l.stateNode);else if(4===l.tag){if(null!==l.child){r=l.stateNode.containerInfo,a=!0,l.child.return=l,l=l.child;continue}}else if(Bp(e,l),null!==l.child){l.child.return=l,l=l.child;continue}if(l===t)return;for(;null===l.sibling;){if(null===l.return||l.return===t)return;4===(l=l.return).tag&&(s=!1)}l.sibling.return=l.return,l=l.sibling}}function qp(e,t,n){Xp(e,t);var r=t.alternate;Vp(t),null!==r&&Vp(r)}function Kp(e,t){switch(t.tag){case 0:case p:case g:case y:case T:return void function(e,t){var n=t.updateQueue,r=null!==n?n.lastEffect:null;if(null!==r){var a=r.next,o=a;do{if(3==(3&o.tag)){var i=o.destroy;o.destroy=void 0,void 0!==i&&i()}o=o.next}while(o!==a)}}(0,t);case 1:return;case 5:var n=t.stateNode;if(null!=n){var r=t.memoizedProps,a=null!==e?e.memoizedProps:r,o=t.type,i=t.updateQueue;t.updateQueue=null,null!==i&&function(e,t,n,r,a,o){zl(e,a),function(e,t,n,r,a){switch("input"===n&&"radio"===a.type&&null!=a.name&&ht(e,a),sn(n,r),function(e,t,n,r){for(var a=0;a<t.length;a+=2){var o=t[a],i=t[a+1];o===Vi?rn(e,i):o===ji?zt(e,i):o===Hi?Bt(e,i):Q(e,o,i,r)}}(e,t,0,sn(n,a)),n){case"input":gt(e,a);break;case"textarea":Lt(e,a);break;case"select":!function(e,t){var n=e,r=n._wrapperState.wasMultiple;n._wrapperState.wasMultiple=!!t.multiple;var a=t.value;null!=a?Ot(n,!!t.multiple,a,!1):r!==!!t.multiple&&(null!=t.defaultValue?Ot(n,!!t.multiple,t.defaultValue,!0):Ot(n,!!t.multiple,t.multiple?[]:"",!1))}(e,a)}}(e,t,n,r,a)}(n,i,o,a,r)}return;case 6:if(null===t.stateNode)throw Error("This should have a text node initialized. This error is likely caused by a bug in React. Please file an issue.");return void(t.stateNode.nodeValue=t.memoizedProps);case 3:var l=t.stateNode;return void(l.hydrate&&(l.hydrate=!1,Ur(l.containerInfo)));case m:return;case h:return function(e){null!==e.memoizedState&&(vm=Bs(),jp(e.child,!0))}(t),void Jp(t);case b:return void Jp(t);case v:return;case E:case 21:break;case S:case w:return void jp(t,null!==t.memoizedState)}throw Error("This unit of work tag should not have side-effects. This error is likely caused by a bug in React. Please file an issue.")}function Jp(e){var t=e.updateQueue;if(null!==t){e.updateQueue=null;var n=e.stateNode;null===n&&(n=e.stateNode=new Lp),t.forEach(function(t){var r=xh.bind(null,e,t);n.has(t)||(!0!==t.__reactDoNotTraceInteractions&&(r=a.unstable_wrap(r)),n.add(t),t.then(r,r))})}}function Qp(e){yl(e.stateNode)}if("function"==typeof Symbol&&Symbol.for){var Zp=Symbol.for;Zp("selector.component"),Zp("selector.has_pseudo_class"),Zp("selector.role"),Zp("selector.test_id"),Zp("selector.text")}var em=[],tm=Math.ceil,nm=o.ReactCurrentDispatcher,rm=o.ReactCurrentOwner,am=o.IsSomeRendererActing,om=0,im=null,lm=null,sm=0,cm=0,um=Kl(0),fm=0,dm=null,pm=0,mm=0,hm=0,gm=0,ym=null,vm=0,bm=Infinity;function Em(){bm=Bs()+500}function Tm(){return bm}var Sm=null,wm=!1,Nm=null,Rm=null,Om=!1,Cm=null,xm=90,km=0,Am=[],Im=[],Lm=null,_m=0,Pm=null,Mm=0,Dm=null,Um=ca,jm=0,Fm=0,zm=!1;function Bm(){return 0!=(48&om)?Bs():Um!==ca?Um:Um=Bs()}function Hm(e){var t,n,r=e.mode;if(0==(2&r))return 1;if(0==(4&r))return Hs()===Ls?1:2;if(0===jm&&(jm=pm),0!==Ks.transition)return 0!==Fm&&(Fm=null!==ym?ym.pendingLanes:0),t=jm,0===(n=va(ta&~Fm))&&0===(n=va(ta&~t))&&(n=va(ta)),n;var a=Hs();return ya(0!=(4&om)&&a===_s?12:function(e){switch(e){case 99:return Qr;case 98:return Zr;case 97:case 96:return 8;case 95:return 2;default:return 0}}(a),jm)}function Vm(e,t,n){!function(){if(_m>50)throw _m=0,Pm=null,Error("Maximum update depth exceeded. This can happen when a component repeatedly calls setState inside componentWillUpdate or componentDidUpdate. React limits the number of nested updates to prevent infinite loops.");Mm>50&&(Mm=0,s("Maximum update depth exceeded. This can happen when a component calls setState inside useEffect, but useEffect either doesn't have a dependency array, or one of the dependencies changes on every render."))}(),function(e){if($e&&0!=(16&om)&&!ad)switch(e.tag){case 0:case p:case y:var t=lm&&We(lm.type)||"Unknown",n=t;_h.has(n)||(_h.add(n),s("Cannot update a component (`%s`) while rendering a different component (`%s`). To locate the bad setState() call inside `%s`, follow the stack trace as described in https://reactjs.org/link/setstate-in-render",We(e.type)||"Unknown",t,t));break;case 1:Ph||(s("Cannot update during an existing state transition (such as within `render`). Render methods should be a pure function of props and state."),Ph=!0)}}(e);var r=Wm(e,t);if(null===r)return function(e){var t=e.tag;if((3===t||1===t||0===t||t===p||t===g||t===y||t===T)&&0==(e.flags&lr)){var n=We(e.type)||"ReactComponent";if(null!==Lh){if(Lh.has(n))return;Lh.add(n)}else Lh=new Set([n]);if(zm);else{var r=Ye;try{Je(e),s("Can't perform a React state update on an unmounted component. This is a no-op, but it indicates a memory leak in your application. To fix, cancel all subscriptions and asynchronous tasks in %s.",1===t?"the componentWillUnmount method":"a useEffect cleanup function")}finally{r?Je(e):Ke()}}}}(e),null;Ra(r,t,n),r===im&&(hm=Sa(hm,t),4===fm&&$m(r,sm));var a=Hs();1===t?0!=(8&om)&&0==(48&om)?(Vh(r,t),Xm(r)):(Gm(r,n),Vh(r,t),0===om&&(Em(),$s())):(0==(4&om)||a!==_s&&a!==Ls||(null===Lm?Lm=new Set([r]):Lm.add(r)),Gm(r,n),Vh(r,t)),ym=r}function Wm(e,t){e.lanes=Sa(e.lanes,t);var n=e.alternate;null!==n&&(n.lanes=Sa(n.lanes,t)),null===n&&0!=(1026&e.flags)&&Ah(e);for(var r=e,a=e.return;null!==a;)a.childLanes=Sa(a.childLanes,t),null!==(n=a.alternate)?n.childLanes=Sa(n.childLanes,t):0!=(1026&a.flags)&&Ah(e),r=a,a=a.return;return 3===r.tag?r.stateNode:null}function Gm(e,t){var n=e.callbackNode;!function(e,t){for(var n=e.suspendedLanes,r=e.pingedLanes,a=e.expirationTimes,o=e.pendingLanes;o>0;){var i=ba(o),l=1<<i,s=a[i];s===ca?0!=(l&n)&&0==(l&r)||(a[i]=pa(l,t)):s<=t&&(e.expiredLanes|=l),o&=~l}}(e,t);var r=da(e,e===im?sm:0),a=ua;if(0!==r){if(null!==n){if(e.callbackPriority===a)return;Ys(n)}var o,i;a===Qr?(i=Xm.bind(null,e),null===Us?(Us=[i],js=Es(Os,Xs)):Us.push(i),o=Is):o=14===a?Gs(Ls,Xm.bind(null,e)):Gs(function(e){switch(e){case Qr:case 14:return 99;case 13:case 12:case 11:case Zr:return 98;case 9:case 8:case 7:case 6:case 4:case 5:return 97;case 3:case 2:case 1:return 95;case 0:return 90;default:throw Error("Invalid update priority: "+e+". This is a bug in React.")}}(a),Ym.bind(null,e)),e.callbackPriority=a,e.callbackNode=o}else null!==n&&(Ys(n),e.callbackNode=null,e.callbackPriority=0)}function Ym(e){if(Um=ca,jm=0,Fm=0,0!=(48&om))throw Error("Should not already be working.");var t=e.callbackNode;if(vh()&&e.callbackNode!==t)return null;var n=da(e,e===im?sm:0);if(0===n)return null;var r=function(e,t){var n=om;om|=16;var r=nh();im===e&&sm===t||(Em(),eh(e,t),Wh(e,t));for(var a=ah(e);;)try{ch();break}catch(t){th(e,t)}return gc(),oh(a),rh(r),om=n,null!==lm?0:(im=null,sm=0,fm)}(e,n);if(Ea(pm,hm))eh(e,0);else if(0!==r){if(2===r&&(om|=64,e.hydrate&&(e.hydrate=!1,Tl(e.containerInfo)),0!==(n=ma(e))&&(r=lh(e,n))),1===r){var a=dm;throw eh(e,0),$m(e,n),Gm(e,Bs()),a}e.finishedWork=e.current.alternate,e.finishedLanes=n,function(e,t,n){switch(t){case 0:case 1:throw Error("Root did not complete. This is a bug in React.");case 2:ph(e);break;case 3:if($m(e,n),ga(n)&&!(Yh>0)){var r=vm+500-Bs();if(r>10){if(0!==da(e,0))break;var a=e.suspendedLanes;if(!Ta(a,n)){Bm(),Oa(e,a);break}e.timeoutHandle=hl(ph.bind(null,e),r);break}}ph(e);break;case 4:if($m(e,n),function(e){return(e&ta)===e}(n))break;var o=function(e,t){for(var n=e.eventTimes,r=ca;t>0;){var a=ba(t),o=n[a];o>r&&(r=o),t&=~(1<<a)}return r}(e,n),i=Bs()-o,l=((s=i)<120?120:s<480?480:s<1080?1080:s<1920?1920:s<3e3?3e3:s<4320?4320:1960*tm(s/1960))-i;if(l>10){e.timeoutHandle=hl(ph.bind(null,e),l);break}ph(e);break;case 5:ph(e);break;default:throw Error("Unknown root exit status.")}var s}(e,r,n)}return Gm(e,Bs()),e.callbackNode===t?Ym.bind(null,e):null}function $m(e,t){t=wa(t,gm),function(e,t){e.suspendedLanes|=t,e.pingedLanes&=~t;for(var n=e.expirationTimes,r=t;r>0;){var a=ba(r),o=1<<a;n[a]=ca,r&=~o}}(e,t=wa(t,hm))}function Xm(e){if(0!=(48&om))throw Error("Should not already be working.");var t,n;if(vh(),e===im&&Ea(e.expiredLanes,sm)?(n=lh(e,t=sm),Ea(pm,hm)&&(n=lh(e,t=da(e,t)))):n=lh(e,t=da(e,0)),0!==e.tag&&2===n&&(om|=64,e.hydrate&&(e.hydrate=!1,Tl(e.containerInfo)),0!==(t=ma(e))&&(n=lh(e,t))),1===n){var r=dm;throw eh(e,0),$m(e,t),Gm(e,Bs()),r}return e.finishedWork=e.current.alternate,e.finishedLanes=t,ph(e),Gm(e,Bs()),null}function qm(e,t){var n=om;om|=1;try{return e(t)}finally{0===(om=n)&&(Em(),$s())}}function Km(e,t){var n=om;om&=-2,om|=8;try{return e(t)}finally{0===(om=n)&&(Em(),$s())}}function Jm(e,t){var n=om;if(0!=(48&n))return s("flushSync was called from inside a lifecycle method. React cannot flush when React is already rendering. Consider moving this call to a scheduler task or micro task."),e(t);om|=1;try{return e?Ws(Ls,e.bind(null,t)):void 0}finally{om=n,$s()}}function Qm(e,t){Ql(um,cm,e),cm=Sa(cm,t),pm=Sa(pm,t)}function Zm(e){cm=um.current,Jl(um,e)}function eh(e,t){e.finishedWork=null,e.finishedLanes=0;var n=e.timeoutHandle;if(-1!==n&&(e.timeoutHandle=-1,gl(n)),null!==lm)for(var r=lm.return;null!==r;)wp(r),r=r.return;im=e,lm=fg(e.current,null),sm=cm=pm=t,fm=0,dm=null,mm=0,hm=0,gm=0,Dm=null,Js.discardPendingWarnings()}function th(e,t){for(;;){var n=lm;try{if(gc(),Tf(),Ke(),rm.current=null,null===n||null===n.return)return fm=1,dm=t,void(lm=null);8&n.mode&&Cd(n,!0),Ip(e,n.return,n,t,sm),fh(n)}catch(e){t=e,lm===n&&null!==n?lm=n=n.return:n=lm;continue}return}}function nh(){var e=nm.current;return nm.current=ud,null===e?ud:e}function rh(e){nm.current=e}function ah(e){var t=a.__interactionsRef.current;return a.__interactionsRef.current=e.memoizedInteractions,t}function oh(e){a.__interactionsRef.current=e}function ih(e){mm=Sa(e,mm)}function lh(e,t){var n=om;om|=16;var r=nh();im===e&&sm===t||(eh(e,t),Wh(e,t));for(var a=ah(e);;)try{sh();break}catch(t){th(e,t)}if(gc(),oh(a),om=n,rh(r),null!==lm)throw Error("Cannot commit an incomplete root. This error is likely caused by a bug in React. Please file an issue.");return im=null,sm=0,fm}function sh(){for(;null!==lm;)uh(lm)}function ch(){for(;null!==lm&&!Ms();)uh(lm)}function uh(e){var t,n=e.alternate;Je(e),0!=(8&e.mode)?(Rd(e),t=Ih(n,e,cm),Cd(e,!0)):t=Ih(n,e,cm),Ke(),e.memoizedProps=e.pendingProps,null===t?fh(e):lm=t,rm.current=null}function fh(e){var t=e;do{var n=t.alternate,r=t.return;if(0==(t.flags&sr)){Je(t);var a=void 0;if(0==(8&t.mode)?a=Tp(n,t,cm):(Rd(t),a=Tp(n,t,cm),Cd(t,!1)),Ke(),null!==a)return void(lm=a);dh(t),null!==r&&0==(r.flags&sr)&&(null===r.firstEffect&&(r.firstEffect=t.firstEffect),null!==t.lastEffect&&(null!==r.lastEffect&&(r.lastEffect.nextEffect=t.firstEffect),r.lastEffect=t.lastEffect),t.flags>1&&(null!==r.lastEffect?r.lastEffect.nextEffect=t:r.firstEffect=t,r.lastEffect=t))}else{var o=Sp(t);if(null!==o)return o.flags&=2047,void(lm=o);if(0!=(8&t.mode)){Cd(t,!1);for(var i=t.actualDuration,l=t.child;null!==l;)i+=l.actualDuration,l=l.sibling;t.actualDuration=i}null!==r&&(r.firstEffect=r.lastEffect=null,r.flags|=sr)}var s=t.sibling;if(null!==s)return void(lm=s);lm=t=r}while(null!==t);0===fm&&(fm=5)}function dh(e){if(e.tag!==w&&e.tag!==S||null===e.memoizedState||Ea(cm,sa)||0==(4&e.mode)){var t=0;if(0!=(8&e.mode)){for(var n=e.actualDuration,r=e.selfBaseDuration,a=null===e.alternate||e.child!==e.alternate.child,o=e.child;null!==o;)t=Sa(t,Sa(o.lanes,o.childLanes)),a&&(n+=o.actualDuration),r+=o.treeBaseDuration,o=o.sibling;if(e.tag===h&&null!==e.memoizedState){var i=e.child;null!==i&&(r-=i.treeBaseDuration)}e.actualDuration=n,e.treeBaseDuration=r}else for(var l=e.child;null!==l;)t=Sa(t,Sa(l.lanes,l.childLanes)),l=l.sibling;e.childLanes=t}}function ph(e){var t=Hs();return Ws(Ls,mh.bind(null,e,t)),null}function mh(e,t){do{vh()}while(null!==Cm);if(Js.flushLegacyContextWarning(),Js.flushPendingUnsafeLifecycleWarnings(),0!=(48&om))throw Error("Should not already be working.");var n=e.finishedWork,r=e.finishedLanes;if(null===n)return null;if(e.finishedWork=null,e.finishedLanes=0,n===e.current)throw Error("Cannot commit the same tree as before. This error is likely caused by a bug in React. Please file an issue.");e.callbackNode=null;var a,o,i,l,s,c,u,f=Sa(n.lanes,n.childLanes);if(function(e,t){var n=e.pendingLanes&~t;e.pendingLanes=t,e.suspendedLanes=0,e.pingedLanes=0,e.expiredLanes&=t,e.mutableReadLanes&=t,e.entangledLanes&=t;for(var r=e.entanglements,a=e.eventTimes,o=e.expirationTimes,i=n;i>0;){var l=ba(i),s=1<<l;r[l]=0,a[l]=ca,o[l]=ca,i&=~s}}(e,f),null!==Lm&&!function(e){return 0!=(24&e)}(f)&&Lm.has(e)&&Lm.delete(e),e===im&&(im=null,lm=null,sm=0),n.flags>1?null!==n.lastEffect?(n.lastEffect.nextEffect=n,a=n.firstEffect):a=n:a=n.firstEffect,null!==a){var d=om;om|=32;var p=ah(e);rm.current=null,fl=_a,o=ni(),dl={focusedElem:o,selectionRange:ri(o)?(i=o,("selectionStart"in i?{start:i.selectionStart,end:i.selectionEnd}:(u=(c=(s=(l=i).ownerDocument)&&s.defaultView||window).getSelection&&c.getSelection())&&0!==u.rangeCount?function(e,t,n,r,a){var o=0,i=-1,l=-1,s=0,c=0,u=e,f=null;e:for(;;){for(var d=null;u!==t||0!==n&&3!==u.nodeType||(i=o+n),u!==r||0!==a&&3!==u.nodeType||(l=o+a),3===u.nodeType&&(o+=u.nodeValue.length),null!==(d=u.firstChild);)f=u,u=d;for(;;){if(u===e)break e;if(f===t&&++s===n&&(i=o),f===r&&++c===a&&(l=o),null!==(d=u.nextSibling))break;f=(u=f).parentNode}u=d}return-1===i||-1===l?null:{start:i,end:l}}(l,u.anchorNode,u.anchorOffset,u.focusNode,u.focusOffset):null)||{start:0,end:0}):null},Pa(!1),Sm=a;do{if(Kn(null,hh,null),Jn()){if(null===Sm)throw Error("Should be working on an effect.");var m=Qn();Oh(Sm,m),Sm=Sm.nextEffect}}while(null!==Sm);Nd(),Sm=a;do{if(Kn(null,gh,null,e,t),Jn()){if(null===Sm)throw Error("Should be working on an effect.");var h=Qn();Oh(Sm,h),Sm=Sm.nextEffect}}while(null!==Sm);(function(e){var t=ni(),n=e.focusedElem,r=e.selectionRange;if(t!==n&&ei(n)){null!==r&&ri(n)&&function(e,t){var n=t.start,r=t.end;void 0===r&&(r=n),"selectionStart"in e?(e.selectionStart=n,e.selectionEnd=Math.min(r,e.value.length)):function(e,t){var n=e.ownerDocument||document,r=n&&n.defaultView||window;if(r.getSelection){var a=r.getSelection(),o=e.textContent.length,i=Math.min(t.start,o),l=void 0===t.end?i:Math.min(t.end,o);if(!a.extend&&i>l){var s=l;l=i,i=s}var c=Jo(e,i),u=Jo(e,l);if(c&&u){if(1===a.rangeCount&&a.anchorNode===c.node&&a.anchorOffset===c.offset&&a.focusNode===u.node&&a.focusOffset===u.offset)return;var f=n.createRange();f.setStart(c.node,c.offset),a.removeAllRanges(),i>l?(a.addRange(f),a.extend(u.node,u.offset)):(f.setEnd(u.node,u.offset),a.addRange(f))}}}(e,t)}(n,r);for(var a=[],o=n;o=o.parentNode;)1===o.nodeType&&a.push({element:o,left:o.scrollLeft,top:o.scrollTop});"function"==typeof n.focus&&n.focus();for(var i=0;i<a.length;i++){var l=a[i];l.element.scrollLeft=l.left,l.element.scrollTop=l.top}}})(dl),Pa(fl),fl=null,dl=null,e.current=n,Sm=a;do{if(Kn(null,yh,null,e,r),Jn()){if(null===Sm)throw Error("Should be working on an effect.");var g=Qn();Oh(Sm,g),Sm=Sm.nextEffect}}while(null!==Sm);Sm=null,Ds(),oh(p),om=d}else e.current=n,Nd();var y=Om;if(Om)Om=!1,Cm=e,km=r,xm=t;else for(Sm=a;null!==Sm;){var v=Sm.nextEffect;Sm.nextEffect=null,8&Sm.flags&&$h(Sm),Sm=v}if(0!==(f=e.pendingLanes)){if(null!==Dm){var b=Dm;Dm=null;for(var E=0;E<b.length;E++)Hh(e,b[E],e.memoizedInteractions)}Vh(e,f)}else Rm=null;if(y||Gh(e,r),1===f?e===Pm?_m++:(_m=0,Pm=e):_m=0,vs(n.stateNode,t),em.forEach(function(e){return e()}),Gm(e,Bs()),wm){wm=!1;var T=Nm;throw Nm=null,T}return 0!=(8&om)||$s(),null}function hh(){for(;null!==Sm;){var e=Sm.alternate,t=Sm.flags;0!=(t&ir)&&(Je(Sm),Dp(e,Sm),Ke()),0!=(512&t)&&(Om||(Om=!0,Gs(Ps,function(){return vh(),null}))),Sm=Sm.nextEffect}}function gh(e,t){for(;null!==Sm;){Je(Sm);var n=Sm.flags;if(16&n&&Qp(Sm),n&or){var r=Sm.alternate;null!==r&&zp(r)}switch(1038&n){case 2:Gp(Sm),Sm.flags&=-3;break;case 6:Gp(Sm),Sm.flags&=-3,Kp(Sm.alternate,Sm);break;case 1024:Sm.flags&=-1025;break;case 1028:Sm.flags&=-1025,Kp(Sm.alternate,Sm);break;case 4:Kp(Sm.alternate,Sm);break;case 8:qp(e,Sm)}Ke(),Sm=Sm.nextEffect}}function yh(e,t){for(;null!==Sm;){Je(Sm);var n=Sm.flags;36&n&&Up(e,Sm.alternate,Sm),n&or&&Fp(Sm),Ke(),Sm=Sm.nextEffect}}function vh(){if(90!==xm){var e=xm>Ps?Ps:xm;return xm=90,Ws(e,Sh)}return!1}function bh(e,t){Am.push(t,e),Om||(Om=!0,Gs(Ps,function(){return vh(),null}))}function Eh(e,t){Im.push(t,e),e.flags|=lr;var n=e.alternate;null!==n&&(n.flags|=lr),Om||(Om=!0,Gs(Ps,function(){return vh(),null}))}function Th(e){e.destroy=(0,e.create)()}function Sh(){if(null===Cm)return!1;var e=Cm,t=km;if(Cm=null,km=0,0!=(48&om))throw Error("Cannot flush passive effects while already rendering.");zm=!0;var n=om;om|=32;var r=ah(e),a=Im;Im=[];for(var o=0;o<a.length;o+=2){var i=a[o],l=a[o+1],s=i.destroy;i.destroy=void 0,l.flags&=-8193;var c=l.alternate;if(null!==c&&(c.flags&=-8193),"function"==typeof s){if(Je(l),Kn(null,s,null),Jn()){if(null===l)throw Error("Should be working on an effect.");Oh(l,Qn())}Ke()}}var u=Am;Am=[];for(var f=0;f<u.length;f+=2){var d=u[f],p=u[f+1];if(Je(p),Kn(null,Th,null,d),Jn()){if(null===p)throw Error("Should be working on an effect.");Oh(p,Qn())}Ke()}for(var m=e.current.firstEffect;null!==m;){var h=m.nextEffect;m.nextEffect=null,8&m.flags&&$h(m),m=h}return oh(r),Gh(e,t),zm=!1,om=n,$s(),Mm=null===Cm?0:Mm+1,!0}function wh(e){return null!==Rm&&Rm.has(e)}var Nh=function(e){wm||(wm=!0,Nm=e)};function Rh(e,t,n){Ac(e,xp(e,Np(n,t),1));var r=Bm(),a=Wm(e,1);null!==a&&(Ra(a,1,r),Gm(a,r),Vh(a,1))}function Oh(e,t){if(3!==e.tag)for(var n=e.return;null!==n;){if(3===n.tag)return void Rh(n,e,t);if(1===n.tag){var r=n.stateNode;if("function"==typeof n.type.getDerivedStateFromError||"function"==typeof r.componentDidCatch&&!wh(r)){var a=Np(t,e);Ac(n,kp(n,a,1));var o=Bm(),i=Wm(n,1);if(null!==i)Ra(i,1,o),Gm(i,o),Vh(i,1);else if("function"==typeof r.componentDidCatch&&!wh(r))try{r.componentDidCatch(t,a)}catch(e){}return}}n=n.return}else Rh(e,e,t)}function Ch(e,t,n){var r=e.pingCache;null!==r&&r.delete(t);var a=Bm();Oa(e,n),im===e&&Ta(sm,n)&&(4===fm||3===fm&&ga(sm)&&Bs()-vm<500?eh(e,0):gm=Sa(gm,n)),Gm(e,a),Vh(e,n)}function xh(e,t){var n;null!==(n=e.stateNode)&&n.delete(t),function(e,t){var n,r;0===t&&(t=0==(2&(r=e.mode))?1:0==(4&r)?Hs()===Ls?1:2:(0===jm&&(jm=pm),0===(n=va(na&~jm))&&(n=va(na)),n));var a=Bm(),o=Wm(e,t);null!==o&&(Ra(o,t,a),Gm(o,a),Vh(o,t))}(e,0)}var kh=null;function Ah(e){if(0==(16&om)&&6&e.mode){var t=e.tag;if(2===t||3===t||1===t||0===t||t===p||t===g||t===y||t===T){var n=We(e.type)||"ReactComponent";if(null!==kh){if(kh.has(n))return;kh.add(n)}else kh=new Set([n]);var r=Ye;try{Je(e),s("Can't perform a React state update on a component that hasn't mounted yet. This indicates that you have a side-effect in your render function that asynchronously later calls tries to update the component. Move this work to useEffect instead.")}finally{r?Je(e):Ke()}}}}var Ih,Lh=null;Ih=function(e,t,n){var r=bg(null,t);try{return yp(e,t,n)}catch(a){if(null!==a&&"object"==typeof a&&"function"==typeof a.then)throw a;if(gc(),Tf(),wp(t),bg(t,r),8&t.mode&&Rd(t),Kn(null,yp,null,e,t,n),Jn())throw Qn();throw a}};var _h,Ph=!1;_h=new Set;var Mh={current:!1};function Dh(e){if(!0===am.current&&!0!==Mh.current){var t=Ye;try{Je(e),s("It looks like you're using the wrong act() around your test interactions.\nBe sure to use the matching version of act() corresponding to your renderer:\n\n// for react-dom:\nimport {act} from 'react-dom/test-utils';\n// ...\nact(() => ...);\n\n// for react-test-renderer:\nimport TestRenderer from react-test-renderer';\nconst {act} = TestRenderer;\n// ...\nact(() => ...);")}finally{t?Je(e):Ke()}}}function Uh(e){0!=(1&e.mode)&&!1===am.current&&!1===Mh.current&&s("An update to %s ran an effect, but was not wrapped in act(...).\n\nWhen testing, code that causes React state updates should be wrapped into act(...):\n\nact(() => {\n /* fire events that update state */\n});\n/* assert on the output */\n\nThis ensures that you're testing the behavior the user would see in the browser. Learn more at https://reactjs.org/link/wrap-tests-with-act",We(e.type))}var jh=function(e){if(0===om&&!1===am.current&&!1===Mh.current){var t=Ye;try{Je(e),s("An update to %s inside a test was not wrapped in act(...).\n\nWhen testing, code that causes React state updates should be wrapped into act(...):\n\nact(() => {\n /* fire events that update state */\n});\n/* assert on the output */\n\nThis ensures that you're testing the behavior the user would see in the browser. Learn more at https://reactjs.org/link/wrap-tests-with-act",We(e.type))}finally{t?Je(e):Ke()}}},Fh=!1;function zh(e,t){return 1e3*t+e.interactionThreadID}function Bh(e){null===Dm?Dm=[e]:Dm.push(e)}function Hh(e,t,n){if(n.size>0){var r=e.pendingInteractionMap,o=r.get(t);null!=o?n.forEach(function(e){o.has(e)||e.__count++,o.add(e)}):(r.set(t,new Set(n)),n.forEach(function(e){e.__count++}));var i=a.__subscriberRef.current;if(null!==i){var l=zh(e,t);i.onWorkScheduled(n,l)}}}function Vh(e,t){Hh(e,t,a.__interactionsRef.current)}function Wh(e,t){var n=new Set;if(e.pendingInteractionMap.forEach(function(e,r){Ea(t,r)&&e.forEach(function(e){return n.add(e)})}),e.memoizedInteractions=n,n.size>0){var r=a.__subscriberRef.current;if(null!==r){var o=zh(e,t);try{r.onWorkStarted(n,o)}catch(e){Gs(Ls,function(){throw e})}}}}function Gh(e,t){var n,r=e.pendingLanes;try{if(null!==(n=a.__subscriberRef.current)&&e.memoizedInteractions.size>0){var o=zh(e,t);n.onWorkStopped(e.memoizedInteractions,o)}}catch(e){Gs(Ls,function(){throw e})}finally{var i=e.pendingInteractionMap;i.forEach(function(e,t){Ea(r,t)||(i.delete(t),e.forEach(function(e){if(e.__count--,null!==n&&0===e.__count)try{n.onInteractionScheduledWorkCompleted(e)}catch(e){Gs(Ls,function(){throw e})}}))})}}var Yh=0;function $h(e){e.sibling=null,e.stateNode=null}var Xh,qh=null,Kh=null;function Jh(e){if(null===qh)return e;var t=qh(e);return void 0===t?e:t.current}function Qh(e){return Jh(e)}function Zh(e){if(null===qh)return e;var t=qh(e);if(void 0===t){if(null!=e&&"function"==typeof e.render){var n=Jh(e.render);if(e.render!==n){var r={$$typeof:ie,render:n};return void 0!==e.displayName&&(r.displayName=e.displayName),r}}return e}return t.current}function eg(e,t){if(null===qh)return!1;var n=e.elementType,r=t.type,a=!1,o="object"==typeof r&&null!==r?r.$$typeof:null;switch(e.tag){case 1:"function"==typeof r&&(a=!0);break;case 0:("function"==typeof r||o===ue)&&(a=!0);break;case p:(o===ie||o===ue)&&(a=!0);break;case g:case y:(o===ce||o===ue)&&(a=!0);break;default:return!1}if(a){var i=qh(n);if(void 0!==i&&i===qh(r))return!0}return!1}function tg(e){null!==qh&&"function"==typeof WeakSet&&(null===Kh&&(Kh=new WeakSet),Kh.add(e))}function ng(e,t,n){var r=e.alternate,a=e.child,o=e.sibling,i=e.tag,l=e.type,s=null;switch(i){case 0:case y:case 1:s=l;break;case p:s=l.render}if(null===qh)throw new Error("Expected resolveFamily to be set during hot reload.");var c=!1,u=!1;if(null!==s){var f=qh(s);void 0!==f&&(n.has(f)?u=!0:t.has(f)&&(1===i?u=!0:c=!0))}null!==Kh&&(Kh.has(e)||null!==r&&Kh.has(r))&&(u=!0),u&&(e._debugNeedsRemount=!0),(u||c)&&Vm(e,1,ca),null===a||u||ng(a,t,n),null!==o&&ng(o,t,n)}function rg(e,t,n){var r=e.child,a=e.sibling,o=e.type,i=null;switch(e.tag){case 0:case y:case 1:i=o;break;case p:i=o.render}var l=!1;null!==i&&t.has(i)&&(l=!0),l?function(e,t){if(!function(e,t){for(var n=e,r=!1;;){if(5===n.tag)r=!0,t.add(n.stateNode);else if(null!==n.child){n.child.return=n,n=n.child;continue}if(n===e)return r;for(;null===n.sibling;){if(null===n.return||n.return===e)return r;n=n.return}n.sibling.return=n.return,n=n.sibling}return!1}(e,t))for(var n=e;;){switch(n.tag){case 5:return void t.add(n.stateNode);case 4:case 3:return void t.add(n.stateNode.containerInfo)}if(null===n.return)throw new Error("Expected to reach root first.");n=n.return}}(e,n):null!==r&&rg(r,t,n),null!==a&&rg(a,t,n)}Xh=!1;try{var ag=Object.preventExtensions({});new Map([[ag,null]]),new Set([ag])}catch(e){Xh=!0}var og=1;function ig(e,t,n,r){this.tag=e,this.key=n,this.elementType=null,this.type=null,this.stateNode=null,this.return=null,this.child=null,this.sibling=null,this.index=0,this.ref=null,this.pendingProps=t,this.memoizedProps=null,this.updateQueue=null,this.memoizedState=null,this.dependencies=null,this.mode=r,this.flags=0,this.nextEffect=null,this.firstEffect=null,this.lastEffect=null,this.lanes=0,this.childLanes=0,this.alternate=null,this.actualDuration=Number.NaN,this.actualStartTime=Number.NaN,this.selfBaseDuration=Number.NaN,this.treeBaseDuration=Number.NaN,this.actualDuration=0,this.actualStartTime=-1,this.selfBaseDuration=0,this.treeBaseDuration=0,this._debugID=og++,this._debugSource=null,this._debugOwner=null,this._debugNeedsRemount=!1,this._debugHookTypes=null,Xh||"function"!=typeof Object.preventExtensions||Object.preventExtensions(this)}var lg,sg,cg=function(e,t,n,r){return new ig(e,t,n,r)};function ug(e){var t=e.prototype;return!(!t||!t.isReactComponent)}function fg(e,t){var n=e.alternate;null===n?((n=cg(e.tag,t,e.key,e.mode)).elementType=e.elementType,n.type=e.type,n.stateNode=e.stateNode,n._debugID=e._debugID,n._debugSource=e._debugSource,n._debugOwner=e._debugOwner,n._debugHookTypes=e._debugHookTypes,n.alternate=e,e.alternate=n):(n.pendingProps=t,n.type=e.type,n.flags=0,n.nextEffect=null,n.firstEffect=null,n.lastEffect=null,n.actualDuration=0,n.actualStartTime=-1),n.childLanes=e.childLanes,n.lanes=e.lanes,n.child=e.child,n.memoizedProps=e.memoizedProps,n.memoizedState=e.memoizedState,n.updateQueue=e.updateQueue;var r=e.dependencies;switch(n.dependencies=null===r?null:{lanes:r.lanes,firstContext:r.firstContext},n.sibling=e.sibling,n.index=e.index,n.ref=e.ref,n.selfBaseDuration=e.selfBaseDuration,n.treeBaseDuration=e.treeBaseDuration,n._debugNeedsRemount=e._debugNeedsRemount,n.tag){case 2:case 0:case y:n.type=Jh(e.type);break;case 1:n.type=Qh(e.type);break;case p:n.type=Zh(e.type)}return n}function dg(e,t){e.flags&=2,e.nextEffect=null,e.firstEffect=null,e.lastEffect=null;var n=e.alternate;if(null===n)e.childLanes=0,e.lanes=t,e.child=null,e.memoizedProps=null,e.memoizedState=null,e.updateQueue=null,e.dependencies=null,e.stateNode=null,e.selfBaseDuration=0,e.treeBaseDuration=0;else{e.childLanes=n.childLanes,e.lanes=n.lanes,e.child=n.child,e.memoizedProps=n.memoizedProps,e.memoizedState=n.memoizedState,e.updateQueue=n.updateQueue,e.type=n.type;var r=n.dependencies;e.dependencies=null===r?null:{lanes:r.lanes,firstContext:r.firstContext},e.selfBaseDuration=n.selfBaseDuration,e.treeBaseDuration=n.treeBaseDuration}return e}function pg(e,t,n,r,a,o){var i=2,l=e;if("function"==typeof e)ug(e)?(i=1,l=Qh(l)):l=Jh(l);else if("string"==typeof e)i=5;else e:switch(e){case te:return hg(n.children,a,o,t);case he:i=8,a|=16;break;case ne:i=8,a|=1;break;case re:return function(e,t,n,r){"string"!=typeof e.id&&s('Profiler must specify an "id" as a prop');var a=cg(m,e,r,8|t);return a.elementType=re,a.type=re,a.lanes=n,a.stateNode={effectDuration:0,passiveEffectDuration:0},a}(n,a,o,t);case le:return function(e,t,n,r){var a=cg(h,e,r,t);return a.type=le,a.elementType=le,a.lanes=n,a}(n,a,o,t);case se:return function(e,t,n,r){var a=cg(b,e,r,t);return a.type=se,a.elementType=se,a.lanes=n,a}(n,a,o,t);case ge:return gg(n,a,o,t);case ye:return function(e,t,n,r){var a=cg(w,e,r,t);return a.type=ye,a.elementType=ye,a.lanes=n,a}(n,a,o,t);case de:default:if("object"==typeof e&&null!==e)switch(e.$$typeof){case ae:i=d;break e;case oe:i=9;break e;case ie:i=p,l=Zh(l);break e;case ce:i=g;break e;case ue:i=16,l=null;break e;case fe:i=T;break e}var c="";(void 0===e||"object"==typeof e&&null!==e&&0===Object.keys(e).length)&&(c+=" You likely forgot to export your component from the file it's defined in, or you might have mixed up default and named imports.");var u=r?We(r.type):null;throw u&&(c+="\n\nCheck the render method of `"+u+"`."),Error("Element type is invalid: expected a string (for built-in components) or a class/function (for composite components) but got: "+(null==e?e:typeof e)+"."+c)}var f=cg(i,n,t,a);return f.elementType=e,f.type=l,f.lanes=o,f._debugOwner=r,f}function mg(e,t,n){var r=pg(e.type,e.key,e.props,e._owner,t,n);return r._debugSource=e._source,r._debugOwner=e._owner,r}function hg(e,t,n,r){var a=cg(7,e,r,t);return a.lanes=n,a}function gg(e,t,n,r){var a=cg(S,e,r,t);return a.type=ge,a.elementType=ge,a.lanes=n,a}function yg(e,t,n){var r=cg(6,e,null,t);return r.lanes=n,r}function vg(e,t,n){var r=cg(4,null!==e.children?e.children:[],e.key,t);return r.lanes=n,r.stateNode={containerInfo:e.containerInfo,pendingChildren:null,implementation:e.implementation},r}function bg(e,t){return null===e&&(e=cg(2,null,null,0)),e.tag=t.tag,e.key=t.key,e.elementType=t.elementType,e.type=t.type,e.stateNode=t.stateNode,e.return=t.return,e.child=t.child,e.sibling=t.sibling,e.index=t.index,e.ref=t.ref,e.pendingProps=t.pendingProps,e.memoizedProps=t.memoizedProps,e.updateQueue=t.updateQueue,e.memoizedState=t.memoizedState,e.dependencies=t.dependencies,e.mode=t.mode,e.flags=t.flags,e.nextEffect=t.nextEffect,e.firstEffect=t.firstEffect,e.lastEffect=t.lastEffect,e.lanes=t.lanes,e.childLanes=t.childLanes,e.alternate=t.alternate,e.actualDuration=t.actualDuration,e.actualStartTime=t.actualStartTime,e.selfBaseDuration=t.selfBaseDuration,e.treeBaseDuration=t.treeBaseDuration,e._debugID=t._debugID,e._debugSource=t._debugSource,e._debugOwner=t._debugOwner,e._debugNeedsRemount=t._debugNeedsRemount,e._debugHookTypes=t._debugHookTypes,e}function Eg(e,t,n){switch(this.tag=t,this.containerInfo=e,this.pendingChildren=null,this.current=null,this.pingCache=null,this.finishedWork=null,this.timeoutHandle=-1,this.context=null,this.pendingContext=null,this.hydrate=n,this.callbackNode=null,this.callbackPriority=0,this.eventTimes=Na(0),this.expirationTimes=Na(ca),this.pendingLanes=0,this.suspendedLanes=0,this.pingedLanes=0,this.expiredLanes=0,this.mutableReadLanes=0,this.finishedLanes=0,this.entangledLanes=0,this.entanglements=Na(0),this.mutableSourceEagerHydrationData=null,this.interactionThreadID=a.unstable_getThreadID(),this.memoizedInteractions=new Set,this.pendingInteractionMap=new Map,t){case 1:this._debugRootType="createBlockingRoot()";break;case 2:this._debugRootType="createRoot()";break;case 0:this._debugRootType="createLegacyRoot()"}}function Tg(e,t){var n=(0,t._getVersion)(t._source);null==e.mutableSourceEagerHydrationData?e.mutableSourceEagerHydrationData=[t,n]:e.mutableSourceEagerHydrationData.push(t,n)}function Sg(e,t,n){var r=arguments.length>3&&void 0!==arguments[3]?arguments[3]:null;return{$$typeof:ee,key:null==r?null:""+r,children:e,containerInfo:t,implementation:n}}function wg(e,t,n,a){!function(e,t){if(hs&&"function"==typeof hs.onScheduleFiberRoot)try{hs.onScheduleFiberRoot(ms,e,t)}catch(e){gs||(gs=!0,s("React instrumentation encountered an error: %s",e))}}(t,e);var o,i=t.current,l=Bm();"undefined"!=typeof jest&&(o=i,!1===Fh&&void 0===r.unstable_flushAllWithoutAsserting&&(2&o.mode||4&o.mode)&&(Fh=!0,s("In Concurrent or Sync modes, the \"scheduler\" module needs to be mocked to guarantee consistent behaviour across tests and browsers. For example, with jest: \njest.mock('scheduler', () => require('scheduler/unstable_mock'));\n\nFor more info, visit https://reactjs.org/link/mock-scheduler")),Dh(i));var c=Hm(i),u=function(e){if(!e)return Zl;var t=Zn(e),n=function(e){if(!function(e){return dr(e)===e}(e)||1!==e.tag)throw Error("Expected subtree parent to be a mounted class component. This error is likely caused by a bug in React. Please file an issue.");var t=e;do{switch(t.tag){case 3:return t.stateNode.context;case 1:if(ls(t.type))return t.stateNode.__reactInternalMemoizedMergedChildContext}t=t.return}while(null!==t);throw Error("Found unexpected detached subtree parent. This error is likely caused by a bug in React. Please file an issue.")}(t);if(1===t.tag){var r=t.type;if(ls(r))return fs(t,r,n)}return n}(n);null===t.context?t.context=u:t.pendingContext=u,$e&&null!==Ye&&!lg&&(lg=!0,s("Render methods should be a pure function of props and state; triggering nested component updates from render is not allowed. If necessary, trigger nested updates in componentDidUpdate.\n\nCheck the render method of %s.",We(Ye.type)||"Unknown"));var f=kc(l,c);return f.payload={element:e},null!==(a=void 0===a?null:a)&&("function"!=typeof a&&s("render(...): Expected the last optional `callback` argument to be a function. Instead received: %s.",a),f.callback=a),Ac(i,f),Vm(i,c,l),c}function Ng(e){var t=e.current;if(!t.child)return null;switch(t.child.tag){case 5:default:return t.child.stateNode}}function Rg(e,t){var n=e.memoizedState;null!==n&&null!==n.dehydrated&&(n.retryLane=function(e,t){return 0!==e&&e<t?e:t}(n.retryLane,t))}function Og(e,t){Rg(e,t);var n=e.alternate;n&&Rg(n,t)}function Cg(e){var t=function(e){var t=gr(e);if(!t)return null;for(var n=t;;){if(5===n.tag||6===n.tag)return n;if(n.child&&4!==n.tag)n.child.return=n,n=n.child;else{if(n===t)return null;for(;!n.sibling;){if(!n.return||n.return===t)return null;n=n.return}n.sibling.return=n.return,n=n.sibling}}return null}(e);return null===t?null:t.tag===E?t.stateNode.instance:t.stateNode}lg=!1,sg={};var xg,kg,Ag,Ig,Lg,_g,Pg,Mg,Dg=function(e){return!1},Ug=function(e,t,r){var a=t[r],o=Array.isArray(e)?e.slice():n({},e);return r+1===t.length?(Array.isArray(o)?o.splice(a,1):delete o[a],o):(o[a]=Ug(e[a],t,r+1),o)},jg=function(e,t){return Ug(e,t,0)},Fg=function(e,t,r,a){var o=t[a],i=Array.isArray(e)?e.slice():n({},e);return a+1===t.length?(i[r[a]]=i[o],Array.isArray(i)?i.splice(o,1):delete i[o]):i[o]=Fg(e[o],t,r,a+1),i},zg=function(e,t,n){if(t.length===n.length){for(var r=0;r<n.length-1;r++)if(t[r]!==n[r])return void i("copyWithRename() expects paths to be the same except for the deepest key");return Fg(e,t,n,0)}i("copyWithRename() expects paths of the same length")},Bg=function(e,t,r,a){if(r>=t.length)return a;var o=t[r],i=Array.isArray(e)?e.slice():n({},e);return i[o]=Bg(e[o],t,r+1,a),i},Hg=function(e,t,n){return Bg(e,t,0,n)},Vg=function(e,t){for(var n=e.memoizedState;null!==n&&t>0;)n=n.next,t--;return n};function Wg(e,t,n){this._internalRoot=function(e,t,n){var r=null!=n&&null!=n.hydrationOptions&&n.hydrationOptions.mutableSources||null,a=function(e,t,n,r){return function(e,t,n,r){var a=new Eg(e,t,n),o=function(e){var t;return t=2===e?7:1===e?3:0,ys&&(t|=8),cg(3,null,null,t)}(t);return a.current=o,o.stateNode=a,Cc(o),a}(e,t,n)}(e,t,null!=n&&!0===n.hydrate);if(function(e,t){t[Il]=e}(a.current,e),yi(8===e.nodeType?e.parentNode:e),r)for(var o=0;o<r.length;o++)Tg(a,r[o]);return a}(e,t,n)}function Gg(e){return!(!e||1!==e.nodeType&&9!==e.nodeType&&11!==e.nodeType&&(8!==e.nodeType||" react-mount-point-unstable "!==e.nodeValue))}xg=function(e,t,r,a){var o=Vg(e,t);if(null!==o){var i=Hg(o.memoizedState,r,a);o.memoizedState=i,o.baseState=i,e.memoizedProps=n({},e.memoizedProps),Vm(e,1,ca)}},kg=function(e,t,r){var a=Vg(e,t);if(null!==a){var o=jg(a.memoizedState,r);a.memoizedState=o,a.baseState=o,e.memoizedProps=n({},e.memoizedProps),Vm(e,1,ca)}},Ag=function(e,t,r,a){var o=Vg(e,t);if(null!==o){var i=zg(o.memoizedState,r,a);o.memoizedState=i,o.baseState=i,e.memoizedProps=n({},e.memoizedProps),Vm(e,1,ca)}},Ig=function(e,t,n){e.pendingProps=Hg(e.memoizedProps,t,n),e.alternate&&(e.alternate.pendingProps=e.pendingProps),Vm(e,1,ca)},Lg=function(e,t){e.pendingProps=jg(e.memoizedProps,t),e.alternate&&(e.alternate.pendingProps=e.pendingProps),Vm(e,1,ca)},_g=function(e,t,n){e.pendingProps=zg(e.memoizedProps,t,n),e.alternate&&(e.alternate.pendingProps=e.pendingProps),Vm(e,1,ca)},Pg=function(e){Vm(e,1,ca)},Mg=function(e){Dg=e},Wg.prototype.render=function(e){var t=this._internalRoot;"function"==typeof arguments[1]&&s("render(...): does not support the second callback argument. To execute a side effect after rendering, declare it in a component body with useEffect().");var n=t.containerInfo;if(8!==n.nodeType){var r=Cg(t.current);r&&r.parentNode!==n&&s("render(...): It looks like the React-rendered content of the root container was removed without using React. This is not supported and will cause errors. Instead, call root.unmount() to empty a root's container.")}wg(e,t,null,null)},Wg.prototype.unmount=function(){"function"==typeof arguments[0]&&s("unmount(...): does not support a callback argument. To execute a side effect after rendering, declare it in a component body with useEffect().");var e=this._internalRoot,t=e.containerInfo;wg(null,e,null,function(){Pl(t)})};var Yg,$g=o.ReactCurrentOwner,Xg=!1;function qg(e){return e?9===e.nodeType?e.documentElement:e.firstChild:null}function Kg(e,t,n,r,a){Yg(n),function(e,t){null!==e&&"function"!=typeof e&&s("%s(...): Expected the last optional `callback` argument to be a function. Instead received: %s.","render",e)}(void 0===a?null:a);var o,l=n._reactRootContainer;if(l){if("function"==typeof a){var c=a;a=function(){var e=Ng(o);c.call(e)}}wg(t,o=l._internalRoot,e,a)}else{if(l=n._reactRootContainer=function(e,t){var n=t||function(e){var t=qg(e);return!(!t||1!==t.nodeType||!t.hasAttribute(_))}(e);if(!n)for(var r,a=!1;r=e.lastChild;)!a&&1===r.nodeType&&r.hasAttribute(_)&&(a=!0,s("render(): Target node has markup rendered by React, but there are unrelated nodes as well. This is most commonly caused by white-space inserted around server-rendered markup.")),e.removeChild(r);return!n||t||Xg||(Xg=!0,i("render(): Calling ReactDOM.render() to hydrate server-rendered markup will stop working in React v18. Replace the ReactDOM.render() call with ReactDOM.hydrate() if you want React to attach to the server HTML.")),function(e,t){return new Wg(e,0,t)}(e,n?{hydrate:!0}:void 0)}(n,r),o=l._internalRoot,"function"==typeof a){var u=a;a=function(){var e=Ng(o);u.call(e)}}Km(function(){wg(t,o,e,a)})}return Ng(o)}Yg=function(e){if(e._reactRootContainer&&8!==e.nodeType){var t=Cg(e._reactRootContainer._internalRoot.current);t&&t.parentNode!==e&&s("render(...): It looks like the React-rendered content of this container was removed without using React. This is not supported and will cause errors. Instead, call ReactDOM.unmountComponentAtNode to empty a container.")}var n=!!e._reactRootContainer,r=qg(e);!(!r||!Ul(r))&&!n&&s("render(...): Replacing React-rendered children with a new root component. If you intended to update the children of this node, you should instead have the existing children update their state and render the new components instead of calling ReactDOM.render."),1===e.nodeType&&e.tagName&&"BODY"===e.tagName.toUpperCase()&&s("render(): Rendering components directly into document.body is discouraged, since its children are often manipulated by third-party scripts and browser extensions. This may lead to subtle reconciliation issues. Try rendering into a container element created for your app.")},er=function(e){e.tag===h&&(Vm(e,4,Bm()),Og(e,4))},tr=function(e){if(e.tag===h){var t=Bm(),n=aa;Vm(e,n,t),Og(e,n)}},nr=function(e){if(e.tag===h){var t=Bm(),n=Hm(e);Vm(e,n,t),Og(e,n)}},rr=function(e,t){try{return t()}finally{}};var Jg=!1;function Qg(e,t){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:null;if(!Gg(t))throw Error("Target container is not a DOM element.");return Sg(e,t,null,n)}"function"==typeof Map&&null!=Map.prototype&&"function"==typeof Map.prototype.forEach&&"function"==typeof Set&&null!=Set.prototype&&"function"==typeof Set.prototype.clear&&"function"==typeof Set.prototype.forEach||s("React depends on Map and Set built-in types. Make sure that you load a polyfill in older browsers. https://reactjs.org/link/react-polyfills"),Rn=function(e,t,n){switch(t){case"input":return void function(e,t){var n=e;gt(n,t),function(e,t){var n=t.name;if("radio"===t.type&&null!=n){for(var r=e;r.parentNode;)r=r.parentNode;for(var a=r.querySelectorAll("input[name="+JSON.stringify(""+n)+'][type="radio"]'),o=0;o<a.length;o++){var i=a[o];if(i!==e&&i.form===e.form){var l=Fl(i);if(!l)throw Error("ReactDOMInput: Mixing React and non-React radio inputs with the same `name` is not supported.");it(i),gt(i,l)}}}}(n,t)}(e,n);case"textarea":return void function(e,t){Lt(e,t)}(e,n);case"select":return void function(e,t){var n=t.value;null!=n&&Ot(e,!!t.multiple,n,!1)}(e,n)}},In=qm,Ln=function(e,t,n,r,a){var o=om;om|=4;try{return Ws(_s,e.bind(null,t,n,r,a))}finally{0===(om=o)&&(Em(),$s())}},_n=function(){0==(49&om)?(function(){if(null!==Lm){var e=Lm;Lm=null,e.forEach(function(e){!function(e){e.expiredLanes|=24&e.pendingLanes}(e),Gm(e,Bs())})}$s()}(),vh()):0!=(16&om)&&s("unstable_flushDiscreteUpdates: Cannot flush updates when React is already rendering.")},Pn=function(e,t){var n=om;om|=2;try{return e(t)}finally{0===(om=n)&&(Em(),$s())}};var Zg,ey={Events:[Ul,jl,Fl,kn,An,vh,Mh]};if(!function(e){if("undefined"==typeof __REACT_DEVTOOLS_GLOBAL_HOOK__)return!1;var t=__REACT_DEVTOOLS_GLOBAL_HOOK__;if(t.isDisabled)return!0;if(!t.supportsFiber)return s("The installed version of React DevTools is too old and will not work with the current version of React. Please update React DevTools. https://reactjs.org/link/react-devtools"),!0;try{ms=t.inject(e),hs=t}catch(e){s("React instrumentation encountered an error: %s.",e)}return!0}({bundleType:(Zg={findFiberByHostInstance:Dl,bundleType:1,version:qs,rendererPackageName:"react-dom"}).bundleType,version:Zg.version,rendererPackageName:Zg.rendererPackageName,rendererConfig:Zg.rendererConfig,overrideHookState:xg,overrideHookStateDeletePath:kg,overrideHookStateRenamePath:Ag,overrideProps:Ig,overridePropsDeletePath:Lg,overridePropsRenamePath:_g,setSuspenseHandler:Mg,scheduleUpdate:Pg,currentDispatcherRef:o.ReactCurrentDispatcher,findHostInstanceByFiber:function(e){var t=yr(e);return null===t?null:t.stateNode},findFiberByHostInstance:Zg.findFiberByHostInstance||function(e){return null},findHostInstancesForRefresh:function(e,t){var n=new Set,r=new Set(t.map(function(e){return e.current}));return rg(e.current,r,n),n},scheduleRefresh:function(e,t){if(null!==qh){var n=t.staleFamilies,r=t.updatedFamilies;vh(),Jm(function(){ng(e.current,r,n)})}},scheduleRoot:function(e,t){e.context===Zl&&(vh(),Jm(function(){wg(t,e,null,null)}))},setRefreshHandler:function(e){qh=e},getCurrentFiber:function(){return Ye}})&&A&&window.top===window.self&&(navigator.userAgent.indexOf("Chrome")>-1&&-1===navigator.userAgent.indexOf("Edge")||navigator.userAgent.indexOf("Firefox")>-1)){var ty=window.location.protocol;/^(https?|file):$/.test(ty)&&console.info("%cDownload the React DevTools for a better development experience: https://reactjs.org/link/react-devtools"+("file:"===ty?"\nYou might need to use a local HTTP server (instead of file://): https://reactjs.org/link/react-devtools-faq":""),"font-weight:bold")}t.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED=ey,t.createPortal=Qg,t.findDOMNode=function(e){var t=$g.current;return null!==t&&null!==t.stateNode&&(t.stateNode._warnedAboutRefsInRender||s("%s is accessing findDOMNode inside its render(). render() should be a pure function of props and state. It should never access something that requires stale data from the previous render, such as refs. Move this logic to componentDidMount and componentDidUpdate instead.",We(t.type)||"A component"),t.stateNode._warnedAboutRefsInRender=!0),null==e?null:1===e.nodeType?e:function(e,t){var n=Zn(e);if(void 0===n)throw"function"==typeof e.render?Error("Unable to find node on an unmounted component."):Error("Argument appears to not be a ReactComponent. Keys: "+Object.keys(e));var r=yr(n);if(null===r)return null;if(1&r.mode){var a=We(n.type)||"Component";if(!sg[a]){sg[a]=!0;var o=Ye;try{Je(r),s(1&n.mode?"%s is deprecated in StrictMode. %s was passed an instance of %s which is inside StrictMode. Instead, add a ref directly to the element you want to reference. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-find-node":"%s is deprecated in StrictMode. %s was passed an instance of %s which renders StrictMode children. Instead, add a ref directly to the element you want to reference. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-find-node",t,t,a)}finally{o?Je(o):Ke()}}}return r.stateNode}(e,"findDOMNode")},t.flushSync=Jm,t.hydrate=function(e,t,n){if(!Gg(t))throw Error("Target container is not a DOM element.");return Ml(t)&&void 0===t._reactRootContainer&&s("You are calling ReactDOM.hydrate() on a container that was previously passed to ReactDOM.createRoot(). This is not supported. Did you mean to call createRoot(container, {hydrate: true}).render(element)?"),Kg(null,e,t,!0,n)},t.render=function(e,t,n){if(!Gg(t))throw Error("Target container is not a DOM element.");return Ml(t)&&void 0===t._reactRootContainer&&s("You are calling ReactDOM.render() on a container that was previously passed to ReactDOM.createRoot(). This is not supported. Did you mean to call root.render(element)?"),Kg(null,e,t,!1,n)},t.unmountComponentAtNode=function(e){if(!Gg(e))throw Error("unmountComponentAtNode(...): Target container is not a DOM element.");if(Ml(e)&&void 0===e._reactRootContainer&&s("You are calling ReactDOM.unmountComponentAtNode() on a container that was previously passed to ReactDOM.createRoot(). This is not supported. Did you mean to call root.unmount()?"),e._reactRootContainer){var t=qg(e);return t&&!Ul(t)&&s("unmountComponentAtNode(): The node you're attempting to unmount was rendered by another copy of React."),Km(function(){Kg(null,null,e,!1,function(){e._reactRootContainer=null,Pl(e)})}),!0}var n=qg(e),r=!(!n||!Ul(n)),a=1===e.nodeType&&Gg(e.parentNode)&&!!e.parentNode._reactRootContainer;return r&&s("unmountComponentAtNode(): The node you're attempting to unmount was rendered by React and is not a top-level container. %s",a?"You may have accidentally passed in a React root node instead of its container.":"Instead, have the parent component update its state and rerender in order to remove this component."),!1},t.unstable_batchedUpdates=qm,t.unstable_createPortal=function(e,t){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:null;return Jg||(Jg=!0,i('The ReactDOM.unstable_createPortal() alias has been deprecated, and will be removed in React 18+. Update your code to use ReactDOM.createPortal() instead. It has the exact same API, but without the "unstable_" prefix.')),Qg(e,t,n)},t.unstable_renderSubtreeIntoContainer=function(e,t,n,r){return function(e,t,n,r){if(!Gg(n))throw Error("Target container is not a DOM element.");if(null==e||void 0===e._reactInternals)throw Error("parentComponent must be a valid React Component");return Kg(e,t,n,!1,r)}(e,t,n,r)},t.version=qs}()}),he=n(function(e){e.exports=me});Sfdump=window.Sfdump||function(e){var t=e.createElement("style"),n=/([.*+?^${}()|\[\]\/\\])/g,r=/\bsf-dump-\d+-ref[012]\w+\b/,a=0<=navigator.platform.toUpperCase().indexOf("MAC")?"Cmd":"Ctrl",o=function(e,t,n){e.addEventListener(t,n,!1)};function i(t,n){var r,a,o=t.nextSibling||{},i=o.className;if(/\bsf-dump-compact\b/.test(i))r="▼",a="sf-dump-expanded";else{if(!/\bsf-dump-expanded\b/.test(i))return!1;r="▶",a="sf-dump-compact"}if(e.createEvent&&o.dispatchEvent){var l=e.createEvent("Event");l.initEvent("sf-dump-expanded"===a?"sfbeforedumpexpand":"sfbeforedumpcollapse",!0,!1),o.dispatchEvent(l)}if(t.lastChild.innerHTML=r,o.className=o.className.replace(/\bsf-dump-(compact|expanded)\b/,a),n)try{for(t=o.querySelectorAll("."+i),o=0;o<t.length;++o)-1==t[o].className.indexOf(a)&&(t[o].className=a,t[o].previousSibling.lastChild.innerHTML=r)}catch(e){}return!0}function l(e,t){return!!/\bsf-dump-compact\b/.test((e.nextSibling||{}).className)&&(i(e,t),!0)}function s(e){var t=e.querySelector("a.sf-dump-toggle");return!!t&&(function(e,t){/\bsf-dump-expanded\b/.test((e.nextSibling||{}).className)&&i(e,!0)}(t),l(t),!0)}function c(e){Array.from(e.querySelectorAll(".sf-dump-str, .sf-dump-key, .sf-dump-public, .sf-dump-protected, .sf-dump-private")).forEach(function(e){e.className=e.className.replace(/\bsf-dump-highlight\b/,""),e.className=e.className.replace(/\bsf-dump-highlight-active\b/,"")})}return t.innerHTML="pre.sf-dump .sf-dump-compact, .sf-dump-str-collapse .sf-dump-str-collapse, .sf-dump-str-expand .sf-dump-str-expand { display: none; }",(e.documentElement.firstElementChild||e.documentElement.children[0]).appendChild(t),t=e.createElement("style"),(e.documentElement.firstElementChild||e.documentElement.children[0]).appendChild(t),e.addEventListener||(o=function(e,t,n){e.attachEvent("on"+t,function(e){e.preventDefault=function(){e.returnValue=!1},e.target=e.srcElement,n(e)})}),function(u,f){u=e.getElementById(u);for(var d,p,m=new RegExp("^("+(u.getAttribute("data-indent-pad")||" ").replace(n,"\\$1")+")+","m"),h={maxDepth:1,maxStringLength:160,fileLinkFormat:null},g=u.getElementsByTagName("A"),y=g.length,v=0,b=[];v<y;)b.push(g[v++]);for(v in f)h[v]=f[v];function E(e,t){o(u,e,function(e,n){"A"==e.target.tagName?t(e.target,e):"A"==e.target.parentNode.tagName?t(e.target.parentNode,e):(n=(n=/\bsf-dump-ellipsis\b/.test(e.target.className)?e.target.parentNode:e.target).nextElementSibling)&&"A"==n.tagName&&(/\bsf-dump-toggle\b/.test(n.className)||(n=n.nextElementSibling||n),t(n,e,!0))})}function T(e){return e.ctrlKey||e.metaKey}function S(e){return"concat("+e.match(/[^'"]+|['"]/g).map(function(e){return"'"==e?'"\'"':'"'==e?"'\"'":"'"+e+"'"}).join(",")+", '')"}function w(e){return"contains(concat(' ', normalize-space(@class), ' '), ' "+e+" ')"}for(o(u,"mouseover",function(e){""!=t.innerHTML&&(t.innerHTML="")}),E("mouseover",function(e,n,a){if(a)n.target.style.cursor="pointer";else if(e=r.exec(e.className))try{t.innerHTML="pre.sf-dump ."+e[0]+"{background-color: #B729D9; color: #FFF !important; border-radius: 2px}"}catch(n){}}),E("click",function(t,r,a){if(/\bsf-dump-toggle\b/.test(t.className)){if(r.preventDefault(),!i(t,T(r))){var o=e.getElementById(t.getAttribute("href").substr(1)),l=o.previousSibling,s=o.parentNode,c=t.parentNode;c.replaceChild(o,t),s.replaceChild(t,l),c.insertBefore(l,o),s=s.firstChild.nodeValue.match(m),c=c.firstChild.nodeValue.match(m),s&&c&&s[0]!==c[0]&&(o.innerHTML=o.innerHTML.replace(new RegExp("^"+s[0].replace(n,"\\$1"),"mg"),c[0])),/\bsf-dump-compact\b/.test(o.className)&&i(l,T(r))}if(a);else if(e.getSelection)try{e.getSelection().removeAllRanges()}catch(r){e.getSelection().empty()}else e.selection.empty()}else/\bsf-dump-str-toggle\b/.test(t.className)&&(r.preventDefault(),(r=t.parentNode.parentNode).className=r.className.replace(/\bsf-dump-str-(expand|collapse)\b/,t.parentNode.className))}),y=(g=u.getElementsByTagName("SAMP")).length,v=0;v<y;)b.push(g[v++]);for(y=b.length,v=0;v<y;++v)if("SAMP"==(g=b[v]).tagName)"A"!=(E=g.previousSibling||{}).tagName?((E=e.createElement("A")).className="sf-dump-ref",g.parentNode.insertBefore(E,g)):E.innerHTML+=" ",E.title=(E.title?E.title+"\n[":"[")+a+"+click] Expand all children",E.innerHTML+="sf-dump-compact"==g.className?"<span>▶</span>":"<span>▼</span>",E.className+=" sf-dump-toggle",f=1,"sf-dump"!=g.parentNode.className&&(f+=g.parentNode.getAttribute("data-depth")/1);else if(/\bsf-dump-ref\b/.test(g.className)&&(E=g.getAttribute("href"))&&(E=E.substr(1),g.className+=" "+E,/[\[{]$/.test(g.previousSibling.nodeValue))){E=E!=g.nextSibling.id&&e.getElementById(E);try{d=E.nextSibling,g.appendChild(E),d.parentNode.insertBefore(E,d),/^[@#]/.test(g.innerHTML)?g.innerHTML+=" <span>▶</span>":(g.innerHTML="<span>▶</span>",g.className="sf-dump-ref"),g.className+=" sf-dump-toggle"}catch(e){"&"==g.innerHTML.charAt(0)&&(g.innerHTML="…",g.className="sf-dump-ref")}}if(e.evaluate&&Array.from&&u.children.length>1){var N=function(e){var t,n,r=e.current();r&&(function(e){for(var t,n=[];(e=e.parentNode||{})&&(t=e.previousSibling)&&"A"===t.tagName;)n.push(t);0!==n.length&&n.forEach(function(e){l(e)})}(r),function(e,t,n){c(e),Array.from(n||[]).forEach(function(e){/\bsf-dump-highlight\b/.test(e.className)||(e.className=e.className+" sf-dump-highlight")}),/\bsf-dump-highlight-active\b/.test(t.className)||(t.className=t.className+" sf-dump-highlight-active")}(u,r,e.nodes),"scrollIntoView"in r&&(r.scrollIntoView(!0),t=r.getBoundingClientRect(),n=R.getBoundingClientRect(),t.top<n.top+n.height&&window.scrollBy(0,-(n.top+n.height+5)))),x.textContent=(e.isEmpty()?0:e.idx+1)+" of "+e.count()};u.setAttribute("tabindex",0),SearchState=function(){this.nodes=[],this.idx=0},SearchState.prototype={next:function(){return this.isEmpty()||(this.idx=this.idx<this.nodes.length-1?this.idx+1:0),this.current()},previous:function(){return this.isEmpty()||(this.idx=this.idx>0?this.idx-1:this.nodes.length-1),this.current()},isEmpty:function(){return 0===this.count()},current:function(){return this.isEmpty()?null:this.nodes[this.idx]},reset:function(){this.nodes=[],this.idx=0},count:function(){return this.nodes.length}};var R=e.createElement("div");R.className="sf-dump-search-wrapper sf-dump-search-hidden",R.innerHTML='\n <input type="text" class="sf-dump-search-input">\n <span class="sf-dump-search-count">0 of 0</span>\n <button type="button" class="sf-dump-search-input-previous" tabindex="-1">\n <svg viewBox="0 0 1792 1792" xmlns="http://www.w3.org/2000/svg"><path d="M1683 1331l-166 165q-19 19-45 19t-45-19L896 965l-531 531q-19 19-45 19t-45-19l-166-165q-19-19-19-45.5t19-45.5l742-741q19-19 45-19t45 19l742 741q19 19 19 45.5t-19 45.5z"/></svg>\n </button>\n <button type="button" class="sf-dump-search-input-next" tabindex="-1">\n <svg viewBox="0 0 1792 1792" xmlns="http://www.w3.org/2000/svg"><path d="M1683 808l-742 741q-19 19-45 19t-45-19L109 808q-19-19-19-45.5t19-45.5l166-165q19-19 45-19t45 19l531 531 531-531q19-19 45-19t45 19l166 165q19 19 19 45.5t-19 45.5z"/></svg>\n </button>\n ',u.insertBefore(R,u.firstChild);var O=new SearchState,C=R.querySelector(".sf-dump-search-input"),x=R.querySelector(".sf-dump-search-count"),k=0,A="";o(C,"keyup",function(t){var n=t.target.value;n!==A&&(A=n,clearTimeout(k),k=setTimeout(function(){if(O.reset(),s(u),c(u),""!==n){for(var t=["sf-dump-str","sf-dump-key","sf-dump-public","sf-dump-protected","sf-dump-private"].map(w).join(" or "),r=e.evaluate(".//span["+t+"][contains(translate(child::text(), "+S(n.toUpperCase())+", "+S(n.toLowerCase())+"), "+S(n.toLowerCase())+")]",u,null,XPathResult.ORDERED_NODE_ITERATOR_TYPE,null);node=r.iterateNext();)O.nodes.push(node);N(O)}else x.textContent="0 of 0"},400))}),Array.from(R.querySelectorAll(".sf-dump-search-input-next, .sf-dump-search-input-previous")).forEach(function(e){o(e,"click",function(e){e.preventDefault(),-1!==e.target.className.indexOf("next")?O.next():O.previous(),C.focus(),s(u),N(O)})}),o(u,"keydown",function(e){var t=!/\bsf-dump-search-hidden\b/.test(R.className);if(114===e.keyCode&&!t||T(e)&&70===e.keyCode){if(70===e.keyCode&&document.activeElement===C)return;e.preventDefault(),R.className=R.className.replace(/\bsf-dump-search-hidden\b/,""),C.focus()}else t&&(27===e.keyCode?(R.className+=" sf-dump-search-hidden",e.preventDefault(),c(u),C.value=""):(T(e)&&71===e.keyCode||13===e.keyCode||114===e.keyCode)&&(e.preventDefault(),e.shiftKey?O.previous():O.next(),s(u),N(O)))})}if(!(0>=h.maxStringLength))try{for(y=(g=u.querySelectorAll(".sf-dump-str")).length,v=0,b=[];v<y;)b.push(g[v++]);for(y=b.length,v=0;v<y;++v)0<(f=(d=(g=b[v]).innerText||g.textContent).length-h.maxStringLength)&&(p=g.innerHTML,g[g.innerText?"innerText":"textContent"]=d.substring(0,h.maxStringLength),g.className+=" sf-dump-str-collapse",g.innerHTML='<span class="sf-dump-str-collapse">'+p+'<a class="sf-dump-ref sf-dump-str-toggle" title="Collapse"> ◀</a></span><span class="sf-dump-str-expand">'+g.innerHTML+'<a class="sf-dump-ref sf-dump-str-toggle" title="'+f+' remaining characters"> ▶</a></span>')}catch(e){}}}(document);var ge=c.createContext({inView:[],setInView:function(){}});function ye(e){var t=e.children,n=c.useState([]);return c.createElement(ge.Provider,{value:{inView:n[0],setInView:n[1]}},t)}function ve(e){var t,n,r=e.name,a=e.href,o=void 0===a?null:a,i=e.icon,l=e.iconOpacity,s=void 0===l?"opacity-50":l,u=e.important,f=void 0!==u&&u,d=e.children,p=void 0===d?null:d,m=e.onClick,h=void 0===m?null:m,g=e.label,y=void 0===g||g,v=e.navRef,b=c.useContext(ge);return c.createElement("li",{ref:v},c.createElement("a",{href:o||"#"+r,target:o?"_blank":"_self",onClick:function(e){h&&(e.preventDefault(),h())}},c.createElement("button",{className:"\n group px-3 sm:px-5 h-10 uppercase tracking-wider text-xs font-medium\n hover:text-red-500\n "+(f?"mr-2":"")+"\n "+(t=b.inView,n=null==t?0:t.length,((n?t[n-1]:void 0)===r?"text-red-500":"")+"\n ")},i&&c.createElement("span",{className:"mr-1.5 "+(null!=s?s:"opacity-50")},i),y&&c.createElement("span",null,r.charAt(0).toUpperCase()+r.slice(1)),f&&c.createElement("span",{className:"right-2 top-2.5 absolute w-2 h-2 bg-red-500 rounded-full shadow"}))),p)}var be=c.createContext(),Ee=function(){},Te=c.createContext({ignitionConfig:{},setIgnitionConfig:Ee});function Se({children:e,ignitionConfig:t}){const[n,r]=c.useState(t),a=(()=>{let[e,t]=c.useState(()=>{if("function"!=typeof window.matchMedia)return"no-preference";let e=window.matchMedia("(prefers-color-scheme: dark)"),t=window.matchMedia("(prefers-color-scheme: light)");return e.matches?"dark":t.matches?"light":"no-preference"});return c.useEffect(()=>{if("function"!=typeof window.matchMedia)return;let e=window.matchMedia("(prefers-color-scheme: dark)"),n=window.matchMedia("(prefers-color-scheme: light)");if("function"==typeof n.addEventListener){let r=({matches:e})=>{e&&t("dark")},a=({matches:e})=>{e&&t("light")};return e.addEventListener("change",r),n.addEventListener("change",a),()=>{e.removeEventListener("change",r),n.removeEventListener("change",a)}}if("function"==typeof n.addListener){let r=()=>t(e.matches?"dark":n.matches?"light":"no-preference");return e.addListener(r),n.addListener(r),()=>{e.removeListener(r),n.removeListener(r)}}},[]),e})(),o="auto"===n.theme?"no-preference"!==a?a:"light":n.theme;return c.useEffect(()=>{document.documentElement.classList.remove("light","dark","auto"),document.documentElement.classList.add(o)},[o]),c.useEffect(()=>{r(t)},[t]),c.createElement(Te.Provider,{value:{ignitionConfig:n,setIgnitionConfig:r,theme:o}},e)}function we(){we=function(e,t){return new n(e,void 0,t)};var e=RegExp.prototype,t=new WeakMap;function n(e,r,a){var o=new RegExp(e,r);return t.set(o,a||t.get(e)),Oe(o,n.prototype)}function r(e,n){var r=t.get(n);return Object.keys(r).reduce(function(t,n){return t[n]=e[r[n]],t},Object.create(null))}return Re(n,RegExp),n.prototype.exec=function(t){var n=e.exec.call(this,t);return n&&(n.groups=r(n,this)),n},n.prototype[Symbol.replace]=function(n,a){if("string"==typeof a){var o=t.get(this);return e[Symbol.replace].call(this,n,a.replace(/\$<([^>]+)>/g,function(e,t){return"$"+o[t]}))}if("function"==typeof a){var i=this;return e[Symbol.replace].call(this,n,function(){var e=arguments;return"object"!=typeof e[e.length-1]&&(e=[].slice.call(e)).push(r(e,i)),a.apply(this,e)})}return e[Symbol.replace].call(this,n,a)},we.apply(this,arguments)}function Ne(){return(Ne=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(e[r]=n[r])}return e}).apply(this,arguments)}function Re(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),Object.defineProperty(e,"prototype",{writable:!1}),t&&Oe(e,t)}function Oe(e,t){return(Oe=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function Ce(e,t){if(null==e)return{};var n,r,a={},o=Object.keys(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||(a[n]=e[n]);return a}function xe(e){return e.map((t,n)=>Ne({},t,{frame_number:e.length-n}))}function ke(e){return e.application_frame?"unknown"===e.relative_file?"unknown":"application":"vendor"}var Ae="undefined"!=typeof globalThis?globalThis:"undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};function Ie(e){return e&&e.__esModule&&Object.prototype.hasOwnProperty.call(e,"default")?e.default:e}function Le(e){var t={exports:{}};return e(t,t.exports),t.exports}var _e,Pe="object"==typeof Ae&&Ae&&Ae.Object===Object&&Ae,Me="object"==typeof self&&self&&self.Object===Object&&self,De=Pe||Me||Function("return this")(),Ue=De.Symbol,je=Object.prototype,Fe=je.hasOwnProperty,ze=je.toString,Be=Ue?Ue.toStringTag:void 0,He=Object.prototype.toString,Ve=Ue?Ue.toStringTag:void 0,We=function(e){return null==e?void 0===e?"[object Undefined]":"[object Null]":Ve&&Ve in Object(e)?function(e){var t=Fe.call(e,Be),n=e[Be];try{e[Be]=void 0;var r=!0}catch(e){}var a=ze.call(e);return r&&(t?e[Be]=n:delete e[Be]),a}(e):function(e){return He.call(e)}(e)},Ge=function(e){var t=typeof e;return null!=e&&("object"==t||"function"==t)},Ye=function(e){if(!Ge(e))return!1;var t=We(e);return"[object Function]"==t||"[object GeneratorFunction]"==t||"[object AsyncFunction]"==t||"[object Proxy]"==t},$e=De["__core-js_shared__"],Xe=(_e=/[^.]+$/.exec($e&&$e.keys&&$e.keys.IE_PROTO||""))?"Symbol(src)_1."+_e:"",qe=Function.prototype.toString,Ke=function(e){if(null!=e){try{return qe.call(e)}catch(e){}try{return e+""}catch(e){}}return""},Je=/^\[object .+?Constructor\]$/,Qe=RegExp("^"+Function.prototype.toString.call(Object.prototype.hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g,"\\$&").replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g,"$1.*?")+"$"),Ze=function(e,t){var n=function(e,t){return null==e?void 0:e[t]}(e,t);return function(e){return!(!Ge(e)||(t=e,Xe&&Xe in t))&&(Ye(e)?Qe:Je).test(Ke(e));var t}(n)?n:void 0},et=Ze(Object,"create"),tt=Object.prototype.hasOwnProperty,nt=Object.prototype.hasOwnProperty;function rt(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}rt.prototype.clear=function(){this.__data__=et?et(null):{},this.size=0},rt.prototype.delete=function(e){var t=this.has(e)&&delete this.__data__[e];return this.size-=t?1:0,t},rt.prototype.get=function(e){var t=this.__data__;if(et){var n=t[e];return"__lodash_hash_undefined__"===n?void 0:n}return tt.call(t,e)?t[e]:void 0},rt.prototype.has=function(e){var t=this.__data__;return et?void 0!==t[e]:nt.call(t,e)},rt.prototype.set=function(e,t){var n=this.__data__;return this.size+=this.has(e)?0:1,n[e]=et&&void 0===t?"__lodash_hash_undefined__":t,this};var at=rt,ot=function(e,t){return e===t||e!=e&&t!=t},it=function(e,t){for(var n=e.length;n--;)if(ot(e[n][0],t))return n;return-1},lt=Array.prototype.splice;function st(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}st.prototype.clear=function(){this.__data__=[],this.size=0},st.prototype.delete=function(e){var t=this.__data__,n=it(t,e);return!(n<0||(n==t.length-1?t.pop():lt.call(t,n,1),--this.size,0))},st.prototype.get=function(e){var t=this.__data__,n=it(t,e);return n<0?void 0:t[n][1]},st.prototype.has=function(e){return it(this.__data__,e)>-1},st.prototype.set=function(e,t){var n=this.__data__,r=it(n,e);return r<0?(++this.size,n.push([e,t])):n[r][1]=t,this};var ct=st,ut=Ze(De,"Map"),ft=function(e,t){var n,r,a=e.__data__;return("string"==(r=typeof(n=t))||"number"==r||"symbol"==r||"boolean"==r?"__proto__"!==n:null===n)?a["string"==typeof t?"string":"hash"]:a.map};function dt(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}dt.prototype.clear=function(){this.size=0,this.__data__={hash:new at,map:new(ut||ct),string:new at}},dt.prototype.delete=function(e){var t=ft(this,e).delete(e);return this.size-=t?1:0,t},dt.prototype.get=function(e){return ft(this,e).get(e)},dt.prototype.has=function(e){return ft(this,e).has(e)},dt.prototype.set=function(e,t){var n=ft(this,e),r=n.size;return n.set(e,t),this.size+=n.size==r?0:1,this};var pt=dt;function mt(e){var t=-1,n=null==e?0:e.length;for(this.__data__=new pt;++t<n;)this.add(e[t])}mt.prototype.add=mt.prototype.push=function(e){return this.__data__.set(e,"__lodash_hash_undefined__"),this},mt.prototype.has=function(e){return this.__data__.has(e)};var ht=mt,gt=function(e,t,n,r){for(var a=e.length,o=n+(r?1:-1);r?o--:++o<a;)if(t(e[o],o,e))return o;return-1},yt=function(e){return e!=e},vt=function(e,t){return!(null==e||!e.length)&&function(e,t,n){return t==t?function(e,t,n){for(var r=-1,a=e.length;++r<a;)if(e[r]===t)return r;return-1}(e,t):gt(e,yt,0)}(e,t)>-1},bt=function(e,t){return e.has(t)},Et=Ze(De,"Set"),Tt=function(e){var t=-1,n=Array(e.size);return e.forEach(function(e){n[++t]=e}),n},St=Et&&1/Tt(new Et([,-0]))[1]==1/0?function(e){return new Et(e)}:Ee,wt=function(e){return e&&e.length?function(e,t,n){var r=-1,a=vt,o=e.length,i=!0,l=[],s=l;if(o>=200){var c=St(e);if(c)return Tt(c);i=!1,a=bt,s=new ht}else s=l;e:for(;++r<o;){var u=e[r],f=u;if(u=0!==u?u:0,i&&f==f){for(var d=s.length;d--;)if(s[d]===f)continue e;l.push(u)}else a(s,f,void 0)||(s!==l&&s.push(f),l.push(u))}return l}(e):[]};function Nt(e,t){switch(t.type){case"EXPAND_FRAMES":return Ne({},e,{expanded:wt([...e.expanded,...t.frames])});case"EXPAND_ALL_VENDOR_FRAMES":return Ne({},e,{expanded:xe(e.frames).filter(e=>"unknown"!==e.relative_file).map(e=>e.frame_number)});case"COLLAPSE_ALL_VENDOR_FRAMES":{const t=xe(e.frames).filter(e=>"application"===ke(e)).map(e=>e.frame_number);return Ne({},e,{expanded:wt([...t])})}case"SELECT_FRAME":{const n=xe(e.frames).filter(e=>"unknown"!==e.relative_file).map(e=>e.frame_number).includes(t.frame)?t.frame:e.selected;return Ne({},e,{expanded:wt([...e.expanded,n]),selected:n})}case"SELECT_NEXT_FRAME":{const t=xe(e.frames).filter(e=>"unknown"!==e.relative_file).map(e=>e.frame_number),n=t.indexOf(e.selected),r=n===t.length-1?t[0]:t[n+1];return Ne({},e,{expanded:wt([...e.expanded,r]),selected:r})}case"SELECT_PREVIOUS_FRAME":{const t=xe(e.frames).filter(e=>"unknown"!==e.relative_file).map(e=>e.frame_number),n=t.indexOf(e.selected),r=0===n?t[t.length-1]:t[n-1];return Ne({},e,{expanded:wt([...e.expanded,r]),selected:r})}default:return e}}const Rt={type:"application",relative_file:"",expanded:!0,frames:[]};function Ot(e,t,{ignoreWhenActiveElementMatches:n="input, select, textarea, [contenteditable=true]"}={}){c.useEffect(()=>{function r(r){n&&document.activeElement&&document.activeElement.matches(n)||r.key===e&&t(r)}return window.addEventListener("keyup",r),()=>{window.removeEventListener("keyup",r)}},[e,t])}const Ct=["children","className"];function xt(e){let{children:t,className:n=""}=e,r=Ce(e,Ct);return c.createElement("button",Ne({type:r.type||"button",className:`group inline-flex gap-2 items-center h-6 px-2 rounded-sm ~bg-white shadow text-xs font-medium whitespace-nowrap\n transform\n transition-animation\n hover:shadow-md\n active:shadow-inner\n active:translate-y-px\n ${n}\n `},r),t)}function kt({path:e,lineNumber:t=null}){const n=e.split("\\"),r=String.fromCharCode(8201);return c.createElement("span",{className:"inline-flex flex-wrap items-baseline"},n.map((e,t)=>c.createElement(c.Fragment,{key:t},c.createElement("span",{key:t},e),t!==n.length-1&&c.createElement("span",null,r,"\\",r))),t&&c.createElement(c.Fragment,null,r,c.createElement("span",{className:"whitespace-nowrap"},":",r,c.createElement("span",{className:"font-mono text-xs"},t))))}function At(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function It(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?At(Object(n),!0).forEach(function(t){_t(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):At(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}function Lt(e){return(Lt="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function _t(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function Pt(e,t){return function(e){if(Array.isArray(e))return e}(e)||function(e,t){var n=null==e?null:"undefined"!=typeof Symbol&&e[Symbol.iterator]||e["@@iterator"];if(null!=n){var r,a,o=[],i=!0,l=!1;try{for(n=n.call(e);!(i=(r=n.next()).done)&&(o.push(r.value),!t||o.length!==t);i=!0);}catch(e){l=!0,a=e}finally{try{i||null==n.return||n.return()}finally{if(l)throw a}}return o}}(e,t)||Dt(e,t)||function(){throw new TypeError("Invalid attempt to destructure non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function Mt(e){return function(e){if(Array.isArray(e))return Ut(e)}(e)||function(e){if("undefined"!=typeof Symbol&&null!=e[Symbol.iterator]||null!=e["@@iterator"])return Array.from(e)}(e)||Dt(e)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function Dt(e,t){if(e){if("string"==typeof e)return Ut(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?Ut(e,t):void 0}}function Ut(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}var jt=function(){},Ft={},zt={},Bt=null,Ht={mark:jt,measure:jt};try{"undefined"!=typeof window&&(Ft=window),"undefined"!=typeof document&&(zt=document),"undefined"!=typeof MutationObserver&&(Bt=MutationObserver),"undefined"!=typeof performance&&(Ht=performance)}catch(pa){}var Vt=(Ft.navigator||{}).userAgent,Wt=void 0===Vt?"":Vt,Gt=Ft,Yt=zt,$t=Bt,Xt=Ht,qt=!!Yt.documentElement&&!!Yt.head&&"function"==typeof Yt.addEventListener&&"function"==typeof Yt.createElement,Kt=~Wt.indexOf("MSIE")||~Wt.indexOf("Trident/"),Jt=["HTML","HEAD","STYLE","SCRIPT"],Qt=function(){try{return!0}catch(e){return!1}}(),Zt={fas:"solid","fa-solid":"solid",far:"regular","fa-regular":"regular",fal:"light","fa-light":"light",fat:"thin","fa-thin":"thin",fad:"duotone","fa-duotone":"duotone",fab:"brands","fa-brands":"brands",fak:"kit","fa-kit":"kit",fa:"solid"},en={solid:"fas",regular:"far",light:"fal",thin:"fat",duotone:"fad",brands:"fab",kit:"fak"},tn={fab:"fa-brands",fad:"fa-duotone",fak:"fa-kit",fal:"fa-light",far:"fa-regular",fas:"fa-solid",fat:"fa-thin"},nn={"fa-brands":"fab","fa-duotone":"fad","fa-kit":"fak","fa-light":"fal","fa-regular":"far","fa-solid":"fas","fa-thin":"fat"},rn=/fa[srltdbk\-\ ]/,an=/Font ?Awesome ?([56 ]*)(Solid|Regular|Light|Thin|Duotone|Brands|Free|Pro|Kit)?.*/i,on={900:"fas",400:"far",normal:"far",300:"fal",100:"fat"},ln=[1,2,3,4,5,6,7,8,9,10],sn=ln.concat([11,12,13,14,15,16,17,18,19,20]),cn=["class","data-prefix","data-icon","data-fa-transform","data-fa-mask"],un=[].concat(Mt(Object.keys(en)),["2xs","xs","sm","lg","xl","2xl","beat","border","fade","beat-fade","bounce","flip-both","flip-horizontal","flip-vertical","flip","fw","inverse","layers-counter","layers-text","layers","li","pull-left","pull-right","pulse","rotate-180","rotate-270","rotate-90","rotate-by","shake","spin-pulse","spin-reverse","spin","stack-1x","stack-2x","stack","ul","duotone-group","swap-opacity","primary","secondary"]).concat(ln.map(function(e){return"".concat(e,"x")})).concat(sn.map(function(e){return"w-".concat(e)})),fn=Gt.FontAwesomeConfig||{};Yt&&"function"==typeof Yt.querySelector&&[["data-family-prefix","familyPrefix"],["data-style-default","styleDefault"],["data-replacement-class","replacementClass"],["data-auto-replace-svg","autoReplaceSvg"],["data-auto-add-css","autoAddCss"],["data-auto-a11y","autoA11y"],["data-search-pseudo-elements","searchPseudoElements"],["data-observe-mutations","observeMutations"],["data-mutate-approach","mutateApproach"],["data-keep-original-source","keepOriginalSource"],["data-measure-performance","measurePerformance"],["data-show-missing-icons","showMissingIcons"]].forEach(function(e){var t=Pt(e,2),n=t[1],r=function(e){return""===e||"false"!==e&&("true"===e||e)}(function(e){var t=Yt.querySelector("script["+e+"]");if(t)return t.getAttribute(e)}(t[0]));null!=r&&(fn[n]=r)});var dn=It(It({},{familyPrefix:"fa",styleDefault:"solid",replacementClass:"svg-inline--fa",autoReplaceSvg:!0,autoAddCss:!0,autoA11y:!0,searchPseudoElements:!1,observeMutations:!0,mutateApproach:"async",keepOriginalSource:!0,measurePerformance:!1,showMissingIcons:!0}),fn);dn.autoReplaceSvg||(dn.observeMutations=!1);var pn={};Object.keys(dn).forEach(function(e){Object.defineProperty(pn,e,{enumerable:!0,set:function(t){dn[e]=t,mn.forEach(function(e){return e(pn)})},get:function(){return dn[e]}})}),Gt.FontAwesomeConfig=pn;var mn=[],hn={size:16,x:0,y:0,rotate:0,flipX:!1,flipY:!1};function gn(){for(var e=12,t="";e-- >0;)t+="0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"[62*Math.random()|0];return t}function yn(e){for(var t=[],n=(e||[]).length>>>0;n--;)t[n]=e[n];return t}function vn(e){return e.classList?yn(e.classList):(e.getAttribute("class")||"").split(" ").filter(function(e){return e})}function bn(e){return"".concat(e).replace(/&/g,"&").replace(/"/g,""").replace(/'/g,"'").replace(/</g,"<").replace(/>/g,">")}function En(e){return Object.keys(e||{}).reduce(function(t,n){return t+"".concat(n,": ").concat(e[n].trim(),";")},"")}function Tn(e){return e.size!==hn.size||e.x!==hn.x||e.y!==hn.y||e.rotate!==hn.rotate||e.flipX||e.flipY}function Sn(){var e="fa",t="svg-inline--fa",n=pn.familyPrefix,r=pn.replacementClass,a=':root, :host {\n --fa-font-solid: normal 900 1em/1 "Font Awesome 6 Solid";\n --fa-font-regular: normal 400 1em/1 "Font Awesome 6 Regular";\n --fa-font-light: normal 300 1em/1 "Font Awesome 6 Light";\n --fa-font-thin: normal 100 1em/1 "Font Awesome 6 Thin";\n --fa-font-duotone: normal 900 1em/1 "Font Awesome 6 Duotone";\n --fa-font-brands: normal 400 1em/1 "Font Awesome 6 Brands";\n}\n\nsvg:not(:root).svg-inline--fa, svg:not(:host).svg-inline--fa {\n overflow: visible;\n box-sizing: content-box;\n}\n\n.svg-inline--fa {\n display: var(--fa-display, inline-block);\n height: 1em;\n overflow: visible;\n vertical-align: -0.125em;\n}\n.svg-inline--fa.fa-2xs {\n vertical-align: 0.1em;\n}\n.svg-inline--fa.fa-xs {\n vertical-align: 0em;\n}\n.svg-inline--fa.fa-sm {\n vertical-align: -0.0714285705em;\n}\n.svg-inline--fa.fa-lg {\n vertical-align: -0.2em;\n}\n.svg-inline--fa.fa-xl {\n vertical-align: -0.25em;\n}\n.svg-inline--fa.fa-2xl {\n vertical-align: -0.3125em;\n}\n.svg-inline--fa.fa-pull-left {\n margin-right: var(--fa-pull-margin, 0.3em);\n width: auto;\n}\n.svg-inline--fa.fa-pull-right {\n margin-left: var(--fa-pull-margin, 0.3em);\n width: auto;\n}\n.svg-inline--fa.fa-li {\n width: var(--fa-li-width, 2em);\n top: 0.25em;\n}\n.svg-inline--fa.fa-fw {\n width: var(--fa-fw-width, 1.25em);\n}\n\n.fa-layers svg.svg-inline--fa {\n bottom: 0;\n left: 0;\n margin: auto;\n position: absolute;\n right: 0;\n top: 0;\n}\n\n.fa-layers-counter, .fa-layers-text {\n display: inline-block;\n position: absolute;\n text-align: center;\n}\n\n.fa-layers {\n display: inline-block;\n height: 1em;\n position: relative;\n text-align: center;\n vertical-align: -0.125em;\n width: 1em;\n}\n.fa-layers svg.svg-inline--fa {\n -webkit-transform-origin: center center;\n transform-origin: center center;\n}\n\n.fa-layers-text {\n left: 50%;\n top: 50%;\n -webkit-transform: translate(-50%, -50%);\n transform: translate(-50%, -50%);\n -webkit-transform-origin: center center;\n transform-origin: center center;\n}\n\n.fa-layers-counter {\n background-color: var(--fa-counter-background-color, #ff253a);\n border-radius: var(--fa-counter-border-radius, 1em);\n box-sizing: border-box;\n color: var(--fa-inverse, #fff);\n line-height: var(--fa-counter-line-height, 1);\n max-width: var(--fa-counter-max-width, 5em);\n min-width: var(--fa-counter-min-width, 1.5em);\n overflow: hidden;\n padding: var(--fa-counter-padding, 0.25em 0.5em);\n right: var(--fa-right, 0);\n text-overflow: ellipsis;\n top: var(--fa-top, 0);\n -webkit-transform: scale(var(--fa-counter-scale, 0.25));\n transform: scale(var(--fa-counter-scale, 0.25));\n -webkit-transform-origin: top right;\n transform-origin: top right;\n}\n\n.fa-layers-bottom-right {\n bottom: var(--fa-bottom, 0);\n right: var(--fa-right, 0);\n top: auto;\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: bottom right;\n transform-origin: bottom right;\n}\n\n.fa-layers-bottom-left {\n bottom: var(--fa-bottom, 0);\n left: var(--fa-left, 0);\n right: auto;\n top: auto;\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: bottom left;\n transform-origin: bottom left;\n}\n\n.fa-layers-top-right {\n top: var(--fa-top, 0);\n right: var(--fa-right, 0);\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: top right;\n transform-origin: top right;\n}\n\n.fa-layers-top-left {\n left: var(--fa-left, 0);\n right: auto;\n top: var(--fa-top, 0);\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: top left;\n transform-origin: top left;\n}\n\n.fa-1x {\n font-size: 1em;\n}\n\n.fa-2x {\n font-size: 2em;\n}\n\n.fa-3x {\n font-size: 3em;\n}\n\n.fa-4x {\n font-size: 4em;\n}\n\n.fa-5x {\n font-size: 5em;\n}\n\n.fa-6x {\n font-size: 6em;\n}\n\n.fa-7x {\n font-size: 7em;\n}\n\n.fa-8x {\n font-size: 8em;\n}\n\n.fa-9x {\n font-size: 9em;\n}\n\n.fa-10x {\n font-size: 10em;\n}\n\n.fa-2xs {\n font-size: 0.625em;\n line-height: 0.1em;\n vertical-align: 0.225em;\n}\n\n.fa-xs {\n font-size: 0.75em;\n line-height: 0.0833333337em;\n vertical-align: 0.125em;\n}\n\n.fa-sm {\n font-size: 0.875em;\n line-height: 0.0714285718em;\n vertical-align: 0.0535714295em;\n}\n\n.fa-lg {\n font-size: 1.25em;\n line-height: 0.05em;\n vertical-align: -0.075em;\n}\n\n.fa-xl {\n font-size: 1.5em;\n line-height: 0.0416666682em;\n vertical-align: -0.125em;\n}\n\n.fa-2xl {\n font-size: 2em;\n line-height: 0.03125em;\n vertical-align: -0.1875em;\n}\n\n.fa-fw {\n text-align: center;\n width: 1.25em;\n}\n\n.fa-ul {\n list-style-type: none;\n margin-left: var(--fa-li-margin, 2.5em);\n padding-left: 0;\n}\n.fa-ul > li {\n position: relative;\n}\n\n.fa-li {\n left: calc(var(--fa-li-width, 2em) * -1);\n position: absolute;\n text-align: center;\n width: var(--fa-li-width, 2em);\n line-height: inherit;\n}\n\n.fa-border {\n border-color: var(--fa-border-color, #eee);\n border-radius: var(--fa-border-radius, 0.1em);\n border-style: var(--fa-border-style, solid);\n border-width: var(--fa-border-width, 0.08em);\n padding: var(--fa-border-padding, 0.2em 0.25em 0.15em);\n}\n\n.fa-pull-left {\n float: left;\n margin-right: var(--fa-pull-margin, 0.3em);\n}\n\n.fa-pull-right {\n float: right;\n margin-left: var(--fa-pull-margin, 0.3em);\n}\n\n.fa-beat {\n -webkit-animation-name: fa-beat;\n animation-name: fa-beat;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, ease-in-out);\n animation-timing-function: var(--fa-animation-timing, ease-in-out);\n}\n\n.fa-bounce {\n -webkit-animation-name: fa-bounce;\n animation-name: fa-bounce;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.28, 0.84, 0.42, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.28, 0.84, 0.42, 1));\n}\n\n.fa-fade {\n -webkit-animation-name: fa-fade;\n animation-name: fa-fade;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n}\n\n.fa-beat-fade {\n -webkit-animation-name: fa-beat-fade;\n animation-name: fa-beat-fade;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n}\n\n.fa-flip {\n -webkit-animation-name: fa-flip;\n animation-name: fa-flip;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, ease-in-out);\n animation-timing-function: var(--fa-animation-timing, ease-in-out);\n}\n\n.fa-shake {\n -webkit-animation-name: fa-shake;\n animation-name: fa-shake;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, linear);\n animation-timing-function: var(--fa-animation-timing, linear);\n}\n\n.fa-spin {\n -webkit-animation-name: fa-spin;\n animation-name: fa-spin;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 2s);\n animation-duration: var(--fa-animation-duration, 2s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, linear);\n animation-timing-function: var(--fa-animation-timing, linear);\n}\n\n.fa-spin-reverse {\n --fa-animation-direction: reverse;\n}\n\n.fa-pulse,\n.fa-spin-pulse {\n -webkit-animation-name: fa-spin;\n animation-name: fa-spin;\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, steps(8));\n animation-timing-function: var(--fa-animation-timing, steps(8));\n}\n\n@media (prefers-reduced-motion: reduce) {\n .fa-beat,\n.fa-bounce,\n.fa-fade,\n.fa-beat-fade,\n.fa-flip,\n.fa-pulse,\n.fa-shake,\n.fa-spin,\n.fa-spin-pulse {\n -webkit-animation-delay: -1ms;\n animation-delay: -1ms;\n -webkit-animation-duration: 1ms;\n animation-duration: 1ms;\n -webkit-animation-iteration-count: 1;\n animation-iteration-count: 1;\n transition-delay: 0s;\n transition-duration: 0s;\n }\n}\n@-webkit-keyframes fa-beat {\n 0%, 90% {\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 45% {\n -webkit-transform: scale(var(--fa-beat-scale, 1.25));\n transform: scale(var(--fa-beat-scale, 1.25));\n }\n}\n@keyframes fa-beat {\n 0%, 90% {\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 45% {\n -webkit-transform: scale(var(--fa-beat-scale, 1.25));\n transform: scale(var(--fa-beat-scale, 1.25));\n }\n}\n@-webkit-keyframes fa-bounce {\n 0% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 10% {\n -webkit-transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n }\n 30% {\n -webkit-transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n }\n 50% {\n -webkit-transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n }\n 57% {\n -webkit-transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n }\n 64% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 100% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n}\n@keyframes fa-bounce {\n 0% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 10% {\n -webkit-transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n }\n 30% {\n -webkit-transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n }\n 50% {\n -webkit-transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n }\n 57% {\n -webkit-transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n }\n 64% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 100% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n}\n@-webkit-keyframes fa-fade {\n 50% {\n opacity: var(--fa-fade-opacity, 0.4);\n }\n}\n@keyframes fa-fade {\n 50% {\n opacity: var(--fa-fade-opacity, 0.4);\n }\n}\n@-webkit-keyframes fa-beat-fade {\n 0%, 100% {\n opacity: var(--fa-beat-fade-opacity, 0.4);\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 50% {\n opacity: 1;\n -webkit-transform: scale(var(--fa-beat-fade-scale, 1.125));\n transform: scale(var(--fa-beat-fade-scale, 1.125));\n }\n}\n@keyframes fa-beat-fade {\n 0%, 100% {\n opacity: var(--fa-beat-fade-opacity, 0.4);\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 50% {\n opacity: 1;\n -webkit-transform: scale(var(--fa-beat-fade-scale, 1.125));\n transform: scale(var(--fa-beat-fade-scale, 1.125));\n }\n}\n@-webkit-keyframes fa-flip {\n 50% {\n -webkit-transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n }\n}\n@keyframes fa-flip {\n 50% {\n -webkit-transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n }\n}\n@-webkit-keyframes fa-shake {\n 0% {\n -webkit-transform: rotate(-15deg);\n transform: rotate(-15deg);\n }\n 4% {\n -webkit-transform: rotate(15deg);\n transform: rotate(15deg);\n }\n 8%, 24% {\n -webkit-transform: rotate(-18deg);\n transform: rotate(-18deg);\n }\n 12%, 28% {\n -webkit-transform: rotate(18deg);\n transform: rotate(18deg);\n }\n 16% {\n -webkit-transform: rotate(-22deg);\n transform: rotate(-22deg);\n }\n 20% {\n -webkit-transform: rotate(22deg);\n transform: rotate(22deg);\n }\n 32% {\n -webkit-transform: rotate(-12deg);\n transform: rotate(-12deg);\n }\n 36% {\n -webkit-transform: rotate(12deg);\n transform: rotate(12deg);\n }\n 40%, 100% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n}\n@keyframes fa-shake {\n 0% {\n -webkit-transform: rotate(-15deg);\n transform: rotate(-15deg);\n }\n 4% {\n -webkit-transform: rotate(15deg);\n transform: rotate(15deg);\n }\n 8%, 24% {\n -webkit-transform: rotate(-18deg);\n transform: rotate(-18deg);\n }\n 12%, 28% {\n -webkit-transform: rotate(18deg);\n transform: rotate(18deg);\n }\n 16% {\n -webkit-transform: rotate(-22deg);\n transform: rotate(-22deg);\n }\n 20% {\n -webkit-transform: rotate(22deg);\n transform: rotate(22deg);\n }\n 32% {\n -webkit-transform: rotate(-12deg);\n transform: rotate(-12deg);\n }\n 36% {\n -webkit-transform: rotate(12deg);\n transform: rotate(12deg);\n }\n 40%, 100% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n}\n@-webkit-keyframes fa-spin {\n 0% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n 100% {\n -webkit-transform: rotate(360deg);\n transform: rotate(360deg);\n }\n}\n@keyframes fa-spin {\n 0% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n 100% {\n -webkit-transform: rotate(360deg);\n transform: rotate(360deg);\n }\n}\n.fa-rotate-90 {\n -webkit-transform: rotate(90deg);\n transform: rotate(90deg);\n}\n\n.fa-rotate-180 {\n -webkit-transform: rotate(180deg);\n transform: rotate(180deg);\n}\n\n.fa-rotate-270 {\n -webkit-transform: rotate(270deg);\n transform: rotate(270deg);\n}\n\n.fa-flip-horizontal {\n -webkit-transform: scale(-1, 1);\n transform: scale(-1, 1);\n}\n\n.fa-flip-vertical {\n -webkit-transform: scale(1, -1);\n transform: scale(1, -1);\n}\n\n.fa-flip-both,\n.fa-flip-horizontal.fa-flip-vertical {\n -webkit-transform: scale(-1, -1);\n transform: scale(-1, -1);\n}\n\n.fa-rotate-by {\n -webkit-transform: rotate(var(--fa-rotate-angle, none));\n transform: rotate(var(--fa-rotate-angle, none));\n}\n\n.fa-stack {\n display: inline-block;\n vertical-align: middle;\n height: 2em;\n position: relative;\n width: 2.5em;\n}\n\n.fa-stack-1x,\n.fa-stack-2x {\n bottom: 0;\n left: 0;\n margin: auto;\n position: absolute;\n right: 0;\n top: 0;\n z-index: var(--fa-stack-z-index, auto);\n}\n\n.svg-inline--fa.fa-stack-1x {\n height: 1em;\n width: 1.25em;\n}\n.svg-inline--fa.fa-stack-2x {\n height: 2em;\n width: 2.5em;\n}\n\n.fa-inverse {\n color: var(--fa-inverse, #fff);\n}\n\n.sr-only,\n.fa-sr-only {\n position: absolute;\n width: 1px;\n height: 1px;\n padding: 0;\n margin: -1px;\n overflow: hidden;\n clip: rect(0, 0, 0, 0);\n white-space: nowrap;\n border-width: 0;\n}\n\n.sr-only-focusable:not(:focus),\n.fa-sr-only-focusable:not(:focus) {\n position: absolute;\n width: 1px;\n height: 1px;\n padding: 0;\n margin: -1px;\n overflow: hidden;\n clip: rect(0, 0, 0, 0);\n white-space: nowrap;\n border-width: 0;\n}\n\n.svg-inline--fa .fa-primary {\n fill: var(--fa-primary-color, currentColor);\n opacity: var(--fa-primary-opacity, 1);\n}\n\n.svg-inline--fa .fa-secondary {\n fill: var(--fa-secondary-color, currentColor);\n opacity: var(--fa-secondary-opacity, 0.4);\n}\n\n.svg-inline--fa.fa-swap-opacity .fa-primary {\n opacity: var(--fa-secondary-opacity, 0.4);\n}\n\n.svg-inline--fa.fa-swap-opacity .fa-secondary {\n opacity: var(--fa-primary-opacity, 1);\n}\n\n.svg-inline--fa mask .fa-primary,\n.svg-inline--fa mask .fa-secondary {\n fill: black;\n}\n\n.fad.fa-inverse,\n.fa-duotone.fa-inverse {\n color: var(--fa-inverse, #fff);\n}';if(n!==e||r!==t){var o=new RegExp("\\.".concat(e,"\\-"),"g"),i=new RegExp("\\--".concat(e,"\\-"),"g"),l=new RegExp("\\.".concat(t),"g");a=a.replace(o,".".concat(n,"-")).replace(i,"--".concat(n,"-")).replace(l,".".concat(r))}return a}var wn=!1;function Nn(){pn.autoAddCss&&!wn&&(function(e){if(e&&qt){var t=Yt.createElement("style");t.setAttribute("type","text/css"),t.innerHTML=e;for(var n=Yt.head.childNodes,r=null,a=n.length-1;a>-1;a--){var o=n[a],i=(o.tagName||"").toUpperCase();["STYLE","LINK"].indexOf(i)>-1&&(r=o)}Yt.head.insertBefore(t,r)}}(Sn()),wn=!0)}var Rn={mixout:function(){return{dom:{css:Sn,insertCss:Nn}}},hooks:function(){return{beforeDOMElementCreation:function(){Nn()},beforeI2svg:function(){Nn()}}}},On=Gt||{};On.___FONT_AWESOME___||(On.___FONT_AWESOME___={}),On.___FONT_AWESOME___.styles||(On.___FONT_AWESOME___.styles={}),On.___FONT_AWESOME___.hooks||(On.___FONT_AWESOME___.hooks={}),On.___FONT_AWESOME___.shims||(On.___FONT_AWESOME___.shims=[]);var Cn=On.___FONT_AWESOME___,xn=[],kn=!1;function An(e){qt&&(kn?setTimeout(e,0):xn.push(e))}function In(e){var t=e.tag,n=e.attributes,r=void 0===n?{}:n,a=e.children,o=void 0===a?[]:a;return"string"==typeof e?bn(e):"<".concat(t," ").concat(function(e){return Object.keys(e||{}).reduce(function(t,n){return t+"".concat(n,'="').concat(bn(e[n]),'" ')},"").trim()}(r),">").concat(o.map(In).join(""),"</").concat(t,">")}function Ln(e,t,n){if(e&&e[t]&&e[t][n])return{prefix:t,iconName:n,icon:e[t][n]}}qt&&((kn=(Yt.documentElement.doScroll?/^loaded|^c/:/^loaded|^i|^c/).test(Yt.readyState))||Yt.addEventListener("DOMContentLoaded",function e(){Yt.removeEventListener("DOMContentLoaded",e),kn=1,xn.map(function(e){return e()})}));var _n=function(e,t,n,r){var a,o,i,l=Object.keys(e),s=l.length,c=void 0!==r?function(e,t){return function(n,r,a,o){return e.call(t,n,r,a,o)}}(t,r):t;for(void 0===n?(a=1,i=e[l[0]]):(a=0,i=n);a<s;a++)i=c(i,e[o=l[a]],o,e);return i};function Pn(e){var t=function(e){for(var t=[],n=0,r=e.length;n<r;){var a=e.charCodeAt(n++);if(a>=55296&&a<=56319&&n<r){var o=e.charCodeAt(n++);56320==(64512&o)?t.push(((1023&a)<<10)+(1023&o)+65536):(t.push(a),n--)}else t.push(a)}return t}(e);return 1===t.length?t[0].toString(16):null}function Mn(e){return Object.keys(e).reduce(function(t,n){var r=e[n];return r.icon?t[r.iconName]=r.icon:t[n]=r,t},{})}function Dn(e,t){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{},r=n.skipHooks,a=void 0!==r&&r,o=Mn(t);"function"!=typeof Cn.hooks.addPack||a?Cn.styles[e]=It(It({},Cn.styles[e]||{}),o):Cn.hooks.addPack(e,Mn(t)),"fas"===e&&Dn("fa",t)}var Un=Cn.styles,jn=Cn.shims,Fn=Object.values(tn),zn=null,Bn={},Hn={},Vn={},Wn={},Gn={},Yn=Object.keys(Zt);function $n(e,t){var n=t.split("-"),r=n[0],a=n.slice(1).join("-");return r!==e||""===a||~un.indexOf(a)?null:a}var Xn=function(){var e=function(e){return _n(Un,function(t,n,r){return t[r]=_n(n,e,{}),t},{})};Bn=e(function(e,t,n){return t[3]&&(e[t[3]]=n),t[2]&&t[2].filter(function(e){return"number"==typeof e}).forEach(function(t){e[t.toString(16)]=n}),e}),Hn=e(function(e,t,n){return e[n]=n,t[2]&&t[2].filter(function(e){return"string"==typeof e}).forEach(function(t){e[t]=n}),e}),Gn=e(function(e,t,n){var r=t[2];return e[n]=n,r.forEach(function(t){e[t]=n}),e});var t="far"in Un||pn.autoFetchSvg,n=_n(jn,function(e,n){var r=n[0],a=n[1],o=n[2];return"far"!==a||t||(a="fas"),"string"==typeof r&&(e.names[r]={prefix:a,iconName:o}),"number"==typeof r&&(e.unicodes[r.toString(16)]={prefix:a,iconName:o}),e},{names:{},unicodes:{}});Vn=n.names,Wn=n.unicodes,zn=Zn(pn.styleDefault)};function qn(e,t){return(Bn[e]||{})[t]}function Kn(e,t){return(Gn[e]||{})[t]}function Jn(e){return Vn[e]||{prefix:null,iconName:null}}function Qn(){return zn}function Zn(e){return en[e]||en[Zt[e]]||(e in Cn.styles?e:null)||null}function er(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.skipLookups,r=void 0!==n&&n,a=null,o=e.reduce(function(e,t){var n=$n(pn.familyPrefix,t);if(Un[t]?(t=Fn.includes(t)?nn[t]:t,a=t,e.prefix=t):Yn.indexOf(t)>-1?(a=t,e.prefix=Zn(t)):n?e.iconName=n:t!==pn.replacementClass&&e.rest.push(t),!r&&e.prefix&&e.iconName){var o="fa"===a?Jn(e.iconName):{},i=Kn(e.prefix,e.iconName);o.prefix&&(a=null),e.iconName=o.iconName||i||e.iconName,e.prefix=o.prefix||e.prefix,"far"!==e.prefix||Un.far||!Un.fas||pn.autoFetchSvg||(e.prefix="fas")}return e},{prefix:null,iconName:null,rest:[]});return"fa"!==o.prefix&&"fa"!==a||(o.prefix=Qn()||"fas"),o}mn.push(function(e){zn=Zn(e.styleDefault)}),Xn();var tr=/*#__PURE__*/function(){function e(){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.definitions={}}var t,n;return t=e,(n=[{key:"add",value:function(){for(var e=this,t=arguments.length,n=new Array(t),r=0;r<t;r++)n[r]=arguments[r];var a=n.reduce(this._pullDefinitions,{});Object.keys(a).forEach(function(t){e.definitions[t]=It(It({},e.definitions[t]||{}),a[t]),Dn(t,a[t]);var n=tn[t];n&&Dn(n,a[t]),Xn()})}},{key:"reset",value:function(){this.definitions={}}},{key:"_pullDefinitions",value:function(e,t){var n=t.prefix&&t.iconName&&t.icon?{0:t}:t;return Object.keys(n).map(function(t){var r=n[t],a=r.prefix,o=r.iconName,i=r.icon,l=i[2];e[a]||(e[a]={}),l.length>0&&l.forEach(function(t){"string"==typeof t&&(e[a][t]=i)}),e[a][o]=i}),e}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(t.prototype,n),Object.defineProperty(t,"prototype",{writable:!1}),e}(),nr=[],rr={},ar={},or=Object.keys(ar);function ir(e,t){for(var n=arguments.length,r=new Array(n>2?n-2:0),a=2;a<n;a++)r[a-2]=arguments[a];var o=rr[e]||[];return o.forEach(function(e){t=e.apply(null,[t].concat(r))}),t}function lr(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];var a=rr[e]||[];a.forEach(function(e){e.apply(null,n)})}function sr(){var e=arguments[0],t=Array.prototype.slice.call(arguments,1);return ar[e]?ar[e].apply(null,t):void 0}function cr(e){"fa"===e.prefix&&(e.prefix="fas");var t=e.iconName,n=e.prefix||Qn();if(t)return t=Kn(n,t)||t,Ln(ur.definitions,n,t)||Ln(Cn.styles,n,t)}var ur=new tr,fr={i2svg:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};return qt?(lr("beforeI2svg",e),sr("pseudoElements2svg",e),sr("i2svg",e)):Promise.reject("Operation requires a DOM of some kind.")},watch:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=e.autoReplaceSvgRoot;!1===pn.autoReplaceSvg&&(pn.autoReplaceSvg=!0),pn.observeMutations=!0,An(function(){pr({autoReplaceSvgRoot:t}),lr("watch",e)})}},dr={noAuto:function(){pn.autoReplaceSvg=!1,pn.observeMutations=!1,lr("noAuto")},config:pn,dom:fr,parse:{icon:function(e){if(null===e)return null;if("object"===Lt(e)&&e.prefix&&e.iconName)return{prefix:e.prefix,iconName:Kn(e.prefix,e.iconName)||e.iconName};if(Array.isArray(e)&&2===e.length){var t=0===e[1].indexOf("fa-")?e[1].slice(3):e[1],n=Zn(e[0]);return{prefix:n,iconName:Kn(n,t)||t}}if("string"==typeof e&&(e.indexOf("".concat(pn.familyPrefix,"-"))>-1||e.match(rn))){var r=er(e.split(" "),{skipLookups:!0});return{prefix:r.prefix||Qn(),iconName:Kn(r.prefix,r.iconName)||r.iconName}}if("string"==typeof e){var a=Qn();return{prefix:a,iconName:Kn(a,e)||e}}}},library:ur,findIconDefinition:cr,toHtml:In},pr=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=e.autoReplaceSvgRoot,n=void 0===t?Yt:t;(Object.keys(Cn.styles).length>0||pn.autoFetchSvg)&&qt&&pn.autoReplaceSvg&&dr.dom.i2svg({node:n})};function mr(e,t){return Object.defineProperty(e,"abstract",{get:t}),Object.defineProperty(e,"html",{get:function(){return e.abstract.map(function(e){return In(e)})}}),Object.defineProperty(e,"node",{get:function(){if(qt){var t=Yt.createElement("div");return t.innerHTML=e.html,t.children}}}),e}function hr(e){var t=e.icons,n=t.main,r=t.mask,a=e.prefix,o=e.iconName,i=e.transform,l=e.symbol,s=e.title,c=e.maskId,u=e.titleId,f=e.extra,d=e.watchable,p=void 0!==d&&d,m=r.found?r:n,h=m.width,g=m.height,y="fak"===a,v=[pn.replacementClass,o?"".concat(pn.familyPrefix,"-").concat(o):""].filter(function(e){return-1===f.classes.indexOf(e)}).filter(function(e){return""!==e||!!e}).concat(f.classes).join(" "),b={children:[],attributes:It(It({},f.attributes),{},{"data-prefix":a,"data-icon":o,class:v,role:f.attributes.role||"img",xmlns:"http://www.w3.org/2000/svg",viewBox:"0 0 ".concat(h," ").concat(g)})},E=y&&!~f.classes.indexOf("fa-fw")?{width:"".concat(h/g*16*.0625,"em")}:{};p&&(b.attributes["data-fa-i2svg"]=""),s&&(b.children.push({tag:"title",attributes:{id:b.attributes["aria-labelledby"]||"title-".concat(u||gn())},children:[s]}),delete b.attributes.title);var T=It(It({},b),{},{prefix:a,iconName:o,main:n,mask:r,maskId:c,transform:i,symbol:l,styles:It(It({},E),f.styles)}),S=r.found&&n.found?sr("generateAbstractMask",T)||{children:[],attributes:{}}:sr("generateAbstractIcon",T)||{children:[],attributes:{}},w=S.attributes;return T.children=S.children,T.attributes=w,l?function(e){var t=e.iconName,n=e.children,r=e.attributes,a=e.symbol,o=!0===a?"".concat(e.prefix,"-").concat(pn.familyPrefix,"-").concat(t):a;return[{tag:"svg",attributes:{style:"display: none;"},children:[{tag:"symbol",attributes:It(It({},r),{},{id:o}),children:n}]}]}(T):function(e){var t=e.children,n=e.main,r=e.mask,a=e.attributes,o=e.styles,i=e.transform;if(Tn(i)&&n.found&&!r.found){var l={x:n.width/n.height/2,y:.5};a.style=En(It(It({},o),{},{"transform-origin":"".concat(l.x+i.x/16,"em ").concat(l.y+i.y/16,"em")}))}return[{tag:"svg",attributes:a,children:t}]}(T)}function gr(e){var t=e.content,n=e.width,r=e.height,a=e.transform,o=e.title,i=e.extra,l=e.watchable,s=void 0!==l&&l,c=It(It(It({},i.attributes),o?{title:o}:{}),{},{class:i.classes.join(" ")});s&&(c["data-fa-i2svg"]="");var u=It({},i.styles);Tn(a)&&(u.transform=function(e){var t=e.transform,n=e.width,r=e.height,a=void 0===r?16:r,o=e.startCentered,i=void 0!==o&&o,l="";return l+=i&&Kt?"translate(".concat(t.x/16-(void 0===n?16:n)/2,"em, ").concat(t.y/16-a/2,"em) "):i?"translate(calc(-50% + ".concat(t.x/16,"em), calc(-50% + ").concat(t.y/16,"em)) "):"translate(".concat(t.x/16,"em, ").concat(t.y/16,"em) "),(l+="scale(".concat(t.size/16*(t.flipX?-1:1),", ").concat(t.size/16*(t.flipY?-1:1),") "))+"rotate(".concat(t.rotate,"deg) ")}({transform:a,startCentered:!0,width:n,height:r}),u["-webkit-transform"]=u.transform);var f=En(u);f.length>0&&(c.style=f);var d=[];return d.push({tag:"span",attributes:c,children:[t]}),o&&d.push({tag:"span",attributes:{class:"sr-only"},children:[o]}),d}function yr(e){var t=e.content,n=e.title,r=e.extra,a=It(It(It({},r.attributes),n?{title:n}:{}),{},{class:r.classes.join(" ")}),o=En(r.styles);o.length>0&&(a.style=o);var i=[];return i.push({tag:"span",attributes:a,children:[t]}),n&&i.push({tag:"span",attributes:{class:"sr-only"},children:[n]}),i}var vr=Cn.styles;function br(e){var t=e[0],n=e[1],r=Pt(e.slice(4),1)[0];return{found:!0,width:t,height:n,icon:Array.isArray(r)?{tag:"g",attributes:{class:"".concat(pn.familyPrefix,"-").concat("duotone-group")},children:[{tag:"path",attributes:{class:"".concat(pn.familyPrefix,"-").concat("secondary"),fill:"currentColor",d:r[0]}},{tag:"path",attributes:{class:"".concat(pn.familyPrefix,"-").concat("primary"),fill:"currentColor",d:r[1]}}]}:{tag:"path",attributes:{fill:"currentColor",d:r}}}}var Er={found:!1,width:512,height:512};function Tr(e,t){var n=t;return"fa"===t&&null!==pn.styleDefault&&(t=Qn()),new Promise(function(r,a){if(sr("missingIconAbstract"),"fa"===n){var o=Jn(e)||{};e=o.iconName||e,t=o.prefix||t}if(e&&t&&vr[t]&&vr[t][e])return r(br(vr[t][e]));!function(e,t){Qt||pn.showMissingIcons||!e||console.error('Icon with name "'.concat(e,'" and prefix "').concat(t,'" is missing.'))}(e,t),r(It(It({},Er),{},{icon:pn.showMissingIcons&&e&&sr("missingIconAbstract")||{}}))})}var Sr=function(){},wr=pn.measurePerformance&&Xt&&Xt.mark&&Xt.measure?Xt:{mark:Sr,measure:Sr},Nr=function(e){return wr.mark("".concat('FA "6.1.1"'," ").concat(e," begins")),function(){return function(e){wr.mark("".concat('FA "6.1.1"'," ").concat(e," ends")),wr.measure("".concat('FA "6.1.1"'," ").concat(e),"".concat('FA "6.1.1"'," ").concat(e," begins"),"".concat('FA "6.1.1"'," ").concat(e," ends"))}(e)}},Rr=function(){};function Or(e){return"string"==typeof(e.getAttribute?e.getAttribute("data-fa-i2svg"):null)}function Cr(e){return Yt.createElementNS("http://www.w3.org/2000/svg",e)}function xr(e){return Yt.createElement(e)}function kr(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.ceFn,r=void 0===n?"svg"===e.tag?Cr:xr:n;if("string"==typeof e)return Yt.createTextNode(e);var a=r(e.tag);Object.keys(e.attributes||[]).forEach(function(t){a.setAttribute(t,e.attributes[t])});var o=e.children||[];return o.forEach(function(e){a.appendChild(kr(e,{ceFn:r}))}),a}var Ar={replace:function(e){var t=e[0];if(t.parentNode)if(e[1].forEach(function(e){t.parentNode.insertBefore(kr(e),t)}),null===t.getAttribute("data-fa-i2svg")&&pn.keepOriginalSource){var n=Yt.createComment(function(e){var t=" ".concat(e.outerHTML," ");return"".concat(t,"Font Awesome fontawesome.com ")}(t));t.parentNode.replaceChild(n,t)}else t.remove()},nest:function(e){var t=e[0],n=e[1];if(~vn(t).indexOf(pn.replacementClass))return Ar.replace(e);var r=new RegExp("".concat(pn.familyPrefix,"-.*"));if(delete n[0].attributes.id,n[0].attributes.class){var a=n[0].attributes.class.split(" ").reduce(function(e,t){return t===pn.replacementClass||t.match(r)?e.toSvg.push(t):e.toNode.push(t),e},{toNode:[],toSvg:[]});n[0].attributes.class=a.toSvg.join(" "),0===a.toNode.length?t.removeAttribute("class"):t.setAttribute("class",a.toNode.join(" "))}var o=n.map(function(e){return In(e)}).join("\n");t.setAttribute("data-fa-i2svg",""),t.innerHTML=o}};function Ir(e){e()}function Lr(e,t){var n="function"==typeof t?t:Rr;if(0===e.length)n();else{var r=Ir;"async"===pn.mutateApproach&&(r=Gt.requestAnimationFrame||Ir),r(function(){var t=!0===pn.autoReplaceSvg?Ar.replace:Ar[pn.autoReplaceSvg]||Ar.replace,r=Nr("mutate");e.map(t),r(),n()})}}var _r=!1;function Pr(){_r=!0}function Mr(){_r=!1}var Dr=null;function Ur(e){if($t&&pn.observeMutations){var t=e.treeCallback,n=void 0===t?Rr:t,r=e.nodeCallback,a=void 0===r?Rr:r,o=e.pseudoElementsCallback,i=void 0===o?Rr:o,l=e.observeMutationsRoot,s=void 0===l?Yt:l;Dr=new $t(function(e){if(!_r){var t=Qn();yn(e).forEach(function(e){if("childList"===e.type&&e.addedNodes.length>0&&!Or(e.addedNodes[0])&&(pn.searchPseudoElements&&i(e.target),n(e.target)),"attributes"===e.type&&e.target.parentNode&&pn.searchPseudoElements&&i(e.target.parentNode),"attributes"===e.type&&Or(e.target)&&~cn.indexOf(e.attributeName))if("class"===e.attributeName&&function(e){var t=e.getAttribute?e.getAttribute("data-prefix"):null,n=e.getAttribute?e.getAttribute("data-icon"):null;return t&&n}(e.target)){var r=er(vn(e.target)),o=r.iconName;e.target.setAttribute("data-prefix",r.prefix||t),o&&e.target.setAttribute("data-icon",o)}else(function(e){return e&&e.classList&&e.classList.contains&&e.classList.contains(pn.replacementClass)})(e.target)&&a(e.target)})}}),qt&&Dr.observe(s,{childList:!0,attributes:!0,characterData:!0,subtree:!0})}}function jr(e){var t=e.getAttribute("style"),n=[];return t&&(n=t.split(";").reduce(function(e,t){var n=t.split(":"),r=n[0],a=n.slice(1);return r&&a.length>0&&(e[r]=a.join(":").trim()),e},{})),n}function Fr(e){var t=e.getAttribute("data-prefix"),n=e.getAttribute("data-icon"),r=void 0!==e.innerText?e.innerText.trim():"",a=er(vn(e));return a.prefix||(a.prefix=Qn()),t&&n&&(a.prefix=t,a.iconName=n),a.iconName&&a.prefix||a.prefix&&r.length>0&&(a.iconName=(Hn[a.prefix]||{})[e.innerText]||qn(a.prefix,Pn(e.innerText))),a}function zr(e){var t=yn(e.attributes).reduce(function(e,t){return"class"!==e.name&&"style"!==e.name&&(e[t.name]=t.value),e},{}),n=e.getAttribute("title"),r=e.getAttribute("data-fa-title-id");return pn.autoA11y&&(n?t["aria-labelledby"]="".concat(pn.replacementClass,"-title-").concat(r||gn()):(t["aria-hidden"]="true",t.focusable="false")),t}function Br(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{styleParser:!0},n=Fr(e),r=n.iconName,a=n.prefix,o=n.rest,i=zr(e),l=ir("parseNodeAttributes",{},e),s=t.styleParser?jr(e):[];return It({iconName:r,title:e.getAttribute("title"),titleId:e.getAttribute("data-fa-title-id"),prefix:a,transform:hn,mask:{iconName:null,prefix:null,rest:[]},maskId:null,symbol:!1,extra:{classes:o,styles:s,attributes:i}},l)}var Hr=Cn.styles;function Vr(e){var t="nest"===pn.autoReplaceSvg?Br(e,{styleParser:!1}):Br(e);return~t.extra.classes.indexOf("fa-layers-text")?sr("generateLayersText",e,t):sr("generateSvgReplacementMutation",e,t)}function Wr(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:null;if(!qt)return Promise.resolve();var n=Yt.documentElement.classList,r=function(e){return n.add("".concat("fontawesome-i2svg","-").concat(e))},a=function(e){return n.remove("".concat("fontawesome-i2svg","-").concat(e))},o=Object.keys(pn.autoFetchSvg?Zt:Hr),i=[".".concat("fa-layers-text",":not([").concat("data-fa-i2svg","])")].concat(o.map(function(e){return".".concat(e,":not([").concat("data-fa-i2svg","])")})).join(", ");if(0===i.length)return Promise.resolve();var l=[];try{l=yn(e.querySelectorAll(i))}catch(e){}if(!(l.length>0))return Promise.resolve();r("pending"),a("complete");var s=Nr("onTree"),c=l.reduce(function(e,t){try{var n=Vr(t);n&&e.push(n)}catch(e){Qt||"MissingIcon"===e.name&&console.error(e)}return e},[]);return new Promise(function(e,n){Promise.all(c).then(function(n){Lr(n,function(){r("active"),r("complete"),a("pending"),"function"==typeof t&&t(),s(),e()})}).catch(function(e){s(),n(e)})})}function Gr(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:null;Vr(e).then(function(e){e&&Lr([e],t)})}var Yr=function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.transform,r=void 0===n?hn:n,a=t.symbol,o=void 0!==a&&a,i=t.mask,l=void 0===i?null:i,s=t.maskId,c=void 0===s?null:s,u=t.title,f=void 0===u?null:u,d=t.titleId,p=void 0===d?null:d,m=t.classes,h=void 0===m?[]:m,g=t.attributes,y=void 0===g?{}:g,v=t.styles,b=void 0===v?{}:v;if(e){var E=e.prefix,T=e.iconName,S=e.icon;return mr(It({type:"icon"},e),function(){return lr("beforeDOMElementCreation",{iconDefinition:e,params:t}),pn.autoA11y&&(f?y["aria-labelledby"]="".concat(pn.replacementClass,"-title-").concat(p||gn()):(y["aria-hidden"]="true",y.focusable="false")),hr({icons:{main:br(S),mask:l?br(l.icon):{found:!1,width:null,height:null,icon:{}}},prefix:E,iconName:T,transform:It(It({},hn),r),symbol:o,title:f,maskId:c,titleId:p,extra:{attributes:y,styles:b,classes:h}})})}},$r={mixout:function(){return{icon:(e=Yr,function(t){var n=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},r=(t||{}).icon?t:cr(t||{}),a=n.mask;return a&&(a=(a||{}).icon?a:cr(a||{})),e(r,It(It({},n),{},{mask:a}))})};var e},hooks:function(){return{mutationObserverCallbacks:function(e){return e.treeCallback=Wr,e.nodeCallback=Gr,e}}},provides:function(e){e.i2svg=function(e){var t=e.node,n=e.callback;return Wr(void 0===t?Yt:t,void 0===n?function(){}:n)},e.generateSvgReplacementMutation=function(e,t){var n=t.iconName,r=t.title,a=t.titleId,o=t.prefix,i=t.transform,l=t.symbol,s=t.mask,c=t.maskId,u=t.extra;return new Promise(function(t,f){Promise.all([Tr(n,o),s.iconName?Tr(s.iconName,s.prefix):Promise.resolve({found:!1,width:512,height:512,icon:{}})]).then(function(s){var f=Pt(s,2);t([e,hr({icons:{main:f[0],mask:f[1]},prefix:o,iconName:n,transform:i,symbol:l,maskId:c,title:r,titleId:a,extra:u,watchable:!0})])}).catch(f)})},e.generateAbstractIcon=function(e){var t,n=e.children,r=e.attributes,a=e.main,o=e.transform,i=En(e.styles);return i.length>0&&(r.style=i),Tn(o)&&(t=sr("generateAbstractTransformGrouping",{main:a,transform:o,containerWidth:a.width,iconWidth:a.width})),n.push(t||a.icon),{children:n,attributes:r}}}},Xr={mixout:function(){return{layer:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.classes,r=void 0===n?[]:n;return mr({type:"layer"},function(){lr("beforeDOMElementCreation",{assembler:e,params:t});var n=[];return e(function(e){Array.isArray(e)?e.map(function(e){n=n.concat(e.abstract)}):n=n.concat(e.abstract)}),[{tag:"span",attributes:{class:["".concat(pn.familyPrefix,"-layers")].concat(Mt(r)).join(" ")},children:n}]})}}}},qr={mixout:function(){return{counter:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.title,r=void 0===n?null:n,a=t.classes,o=void 0===a?[]:a,i=t.attributes,l=void 0===i?{}:i,s=t.styles,c=void 0===s?{}:s;return mr({type:"counter",content:e},function(){return lr("beforeDOMElementCreation",{content:e,params:t}),yr({content:e.toString(),title:r,extra:{attributes:l,styles:c,classes:["".concat(pn.familyPrefix,"-layers-counter")].concat(Mt(o))}})})}}}},Kr={mixout:function(){return{text:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.transform,r=void 0===n?hn:n,a=t.title,o=void 0===a?null:a,i=t.classes,l=void 0===i?[]:i,s=t.attributes,c=void 0===s?{}:s,u=t.styles,f=void 0===u?{}:u;return mr({type:"text",content:e},function(){return lr("beforeDOMElementCreation",{content:e,params:t}),gr({content:e,transform:It(It({},hn),r),title:o,extra:{attributes:c,styles:f,classes:["".concat(pn.familyPrefix,"-layers-text")].concat(Mt(l))}})})}}},provides:function(e){e.generateLayersText=function(e,t){var n=t.title,r=t.transform,a=t.extra,o=null,i=null;if(Kt){var l=parseInt(getComputedStyle(e).fontSize,10),s=e.getBoundingClientRect();o=s.width/l,i=s.height/l}return pn.autoA11y&&!n&&(a.attributes["aria-hidden"]="true"),Promise.resolve([e,gr({content:e.innerHTML,width:o,height:i,transform:r,title:n,extra:a,watchable:!0})])}}},Jr=new RegExp('"',"ug"),Qr=[1105920,1112319];function Zr(e,t){var n="".concat("data-fa-pseudo-element-pending").concat(t.replace(":","-"));return new Promise(function(r,a){if(null!==e.getAttribute(n))return r();var o,i,l,s=yn(e.children).filter(function(e){return e.getAttribute("data-fa-pseudo-element")===t})[0],c=Gt.getComputedStyle(e,t),u=c.getPropertyValue("font-family").match(an),f=c.getPropertyValue("font-weight"),d=c.getPropertyValue("content");if(s&&!u)return e.removeChild(s),r();if(u&&"none"!==d&&""!==d){var p=c.getPropertyValue("content"),m=~["Solid","Regular","Light","Thin","Duotone","Brands","Kit"].indexOf(u[2])?en[u[2].toLowerCase()]:on[f],h=function(e){var t,n,r,a,o=e.replace(Jr,""),i=(r=(t=o).length,(a=t.charCodeAt(0))>=55296&&a<=56319&&r>1&&(n=t.charCodeAt(1))>=56320&&n<=57343?1024*(a-55296)+n-56320+65536:a),l=i>=Qr[0]&&i<=Qr[1],s=2===o.length&&o[0]===o[1];return{value:Pn(s?o[0]:o),isSecondary:l||s}}(p),g=h.value,y=h.isSecondary,v=u[0].startsWith("FontAwesome"),b=qn(m,g),E=b;if(v){var T=(i=Wn[o=g],l=qn("fas",o),i||(l?{prefix:"fas",iconName:l}:null)||{prefix:null,iconName:null});T.iconName&&T.prefix&&(b=T.iconName,m=T.prefix)}if(!b||y||s&&s.getAttribute("data-prefix")===m&&s.getAttribute("data-icon")===E)r();else{e.setAttribute(n,E),s&&e.removeChild(s);var S={iconName:null,title:null,titleId:null,prefix:null,transform:hn,symbol:!1,mask:{iconName:null,prefix:null,rest:[]},maskId:null,extra:{classes:[],styles:{},attributes:{}}},w=S.extra;w.attributes["data-fa-pseudo-element"]=t,Tr(b,m).then(function(a){var o=hr(It(It({},S),{},{icons:{main:a,mask:{prefix:null,iconName:null,rest:[]}},prefix:m,iconName:E,extra:w,watchable:!0})),i=Yt.createElement("svg");"::before"===t?e.insertBefore(i,e.firstChild):e.appendChild(i),i.outerHTML=o.map(function(e){return In(e)}).join("\n"),e.removeAttribute(n),r()}).catch(a)}}else r()})}function ea(e){return Promise.all([Zr(e,"::before"),Zr(e,"::after")])}function ta(e){return!(e.parentNode===document.head||~Jt.indexOf(e.tagName.toUpperCase())||e.getAttribute("data-fa-pseudo-element")||e.parentNode&&"svg"===e.parentNode.tagName)}function na(e){if(qt)return new Promise(function(t,n){var r=yn(e.querySelectorAll("*")).filter(ta).map(ea),a=Nr("searchPseudoElements");Pr(),Promise.all(r).then(function(){a(),Mr(),t()}).catch(function(){a(),Mr(),n()})})}var ra,aa=!1,oa=function(e){return e.toLowerCase().split(" ").reduce(function(e,t){var n=t.toLowerCase().split("-"),r=n[0],a=n.slice(1).join("-");if(r&&"h"===a)return e.flipX=!0,e;if(r&&"v"===a)return e.flipY=!0,e;if(a=parseFloat(a),isNaN(a))return e;switch(r){case"grow":e.size=e.size+a;break;case"shrink":e.size=e.size-a;break;case"left":e.x=e.x-a;break;case"right":e.x=e.x+a;break;case"up":e.y=e.y-a;break;case"down":e.y=e.y+a;break;case"rotate":e.rotate=e.rotate+a}return e},{size:16,x:0,y:0,flipX:!1,flipY:!1,rotate:0})},ia={x:0,y:0,width:"100%",height:"100%"};function la(e){var t=!(arguments.length>1&&void 0!==arguments[1])||arguments[1];return e.attributes&&(e.attributes.fill||t)&&(e.attributes.fill="black"),e}ra=dr,nr=[Rn,$r,Xr,qr,Kr,{hooks:function(){return{mutationObserverCallbacks:function(e){return e.pseudoElementsCallback=na,e}}},provides:function(e){e.pseudoElements2svg=function(e){var t=e.node;pn.searchPseudoElements&&na(void 0===t?Yt:t)}}},{mixout:function(){return{dom:{unwatch:function(){Pr(),aa=!0}}}},hooks:function(){return{bootstrap:function(){Ur(ir("mutationObserverCallbacks",{}))},noAuto:function(){Dr&&Dr.disconnect()},watch:function(e){var t=e.observeMutationsRoot;aa?Mr():Ur(ir("mutationObserverCallbacks",{observeMutationsRoot:t}))}}}},{mixout:function(){return{parse:{transform:function(e){return oa(e)}}}},hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-transform");return n&&(e.transform=oa(n)),e}}},provides:function(e){e.generateAbstractTransformGrouping=function(e){var t=e.main,n=e.transform,r=e.iconWidth,a={transform:"translate(".concat(e.containerWidth/2," 256)")},o="translate(".concat(32*n.x,", ").concat(32*n.y,") "),i="scale(".concat(n.size/16*(n.flipX?-1:1),", ").concat(n.size/16*(n.flipY?-1:1),") "),l="rotate(".concat(n.rotate," 0 0)"),s={outer:a,inner:{transform:"".concat(o," ").concat(i," ").concat(l)},path:{transform:"translate(".concat(r/2*-1," -256)")}};return{tag:"g",attributes:It({},s.outer),children:[{tag:"g",attributes:It({},s.inner),children:[{tag:t.icon.tag,children:t.icon.children,attributes:It(It({},t.icon.attributes),s.path)}]}]}}}},{hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-mask"),r=n?er(n.split(" ").map(function(e){return e.trim()})):{prefix:null,iconName:null,rest:[]};return r.prefix||(r.prefix=Qn()),e.mask=r,e.maskId=t.getAttribute("data-fa-mask-id"),e}}},provides:function(e){e.generateAbstractMask=function(e){var t,n=e.children,r=e.attributes,a=e.main,o=e.mask,i=e.maskId,l=a.icon,s=o.icon,c=function(e){var t=e.transform,n=e.iconWidth,r={transform:"translate(".concat(e.containerWidth/2," 256)")},a="translate(".concat(32*t.x,", ").concat(32*t.y,") "),o="scale(".concat(t.size/16*(t.flipX?-1:1),", ").concat(t.size/16*(t.flipY?-1:1),") "),i="rotate(".concat(t.rotate," 0 0)");return{outer:r,inner:{transform:"".concat(a," ").concat(o," ").concat(i)},path:{transform:"translate(".concat(n/2*-1," -256)")}}}({transform:e.transform,containerWidth:o.width,iconWidth:a.width}),u={tag:"rect",attributes:It(It({},ia),{},{fill:"white"})},f=l.children?{children:l.children.map(la)}:{},d={tag:"g",attributes:It({},c.inner),children:[la(It({tag:l.tag,attributes:It(It({},l.attributes),c.path)},f))]},p={tag:"g",attributes:It({},c.outer),children:[d]},m="mask-".concat(i||gn()),h="clip-".concat(i||gn()),g={tag:"mask",attributes:It(It({},ia),{},{id:m,maskUnits:"userSpaceOnUse",maskContentUnits:"userSpaceOnUse"}),children:[u,p]},y={tag:"defs",children:[{tag:"clipPath",attributes:{id:h},children:(t=s,"g"===t.tag?t.children:[t])},g]};return n.push(y,{tag:"rect",attributes:It({fill:"currentColor","clip-path":"url(#".concat(h,")"),mask:"url(#".concat(m,")")},ia)}),{children:n,attributes:r}}}},{provides:function(e){var t=!1;Gt.matchMedia&&(t=Gt.matchMedia("(prefers-reduced-motion: reduce)").matches),e.missingIconAbstract=function(){var e=[],n={fill:"currentColor"},r={attributeType:"XML",repeatCount:"indefinite",dur:"2s"};e.push({tag:"path",attributes:It(It({},n),{},{d:"M156.5,447.7l-12.6,29.5c-18.7-9.5-35.9-21.2-51.5-34.9l22.7-22.7C127.6,430.5,141.5,440,156.5,447.7z M40.6,272H8.5 c1.4,21.2,5.4,41.7,11.7,61.1L50,321.2C45.1,305.5,41.8,289,40.6,272z M40.6,240c1.4-18.8,5.2-37,11.1-54.1l-29.5-12.6 C14.7,194.3,10,216.7,8.5,240H40.6z M64.3,156.5c7.8-14.9,17.2-28.8,28.1-41.5L69.7,92.3c-13.7,15.6-25.5,32.8-34.9,51.5 L64.3,156.5z M397,419.6c-13.9,12-29.4,22.3-46.1,30.4l11.9,29.8c20.7-9.9,39.8-22.6,56.9-37.6L397,419.6z M115,92.4 c13.9-12,29.4-22.3,46.1-30.4l-11.9-29.8c-20.7,9.9-39.8,22.6-56.8,37.6L115,92.4z M447.7,355.5c-7.8,14.9-17.2,28.8-28.1,41.5 l22.7,22.7c13.7-15.6,25.5-32.9,34.9-51.5L447.7,355.5z M471.4,272c-1.4,18.8-5.2,37-11.1,54.1l29.5,12.6 c7.5-21.1,12.2-43.5,13.6-66.8H471.4z M321.2,462c-15.7,5-32.2,8.2-49.2,9.4v32.1c21.2-1.4,41.7-5.4,61.1-11.7L321.2,462z M240,471.4c-18.8-1.4-37-5.2-54.1-11.1l-12.6,29.5c21.1,7.5,43.5,12.2,66.8,13.6V471.4z M462,190.8c5,15.7,8.2,32.2,9.4,49.2h32.1 c-1.4-21.2-5.4-41.7-11.7-61.1L462,190.8z M92.4,397c-12-13.9-22.3-29.4-30.4-46.1l-29.8,11.9c9.9,20.7,22.6,39.8,37.6,56.9 L92.4,397z M272,40.6c18.8,1.4,36.9,5.2,54.1,11.1l12.6-29.5C317.7,14.7,295.3,10,272,8.5V40.6z M190.8,50 c15.7-5,32.2-8.2,49.2-9.4V8.5c-21.2,1.4-41.7,5.4-61.1,11.7L190.8,50z M442.3,92.3L419.6,115c12,13.9,22.3,29.4,30.5,46.1 l29.8-11.9C470,128.5,457.3,109.4,442.3,92.3z M397,92.4l22.7-22.7c-15.6-13.7-32.8-25.5-51.5-34.9l-12.6,29.5 C370.4,72.1,384.4,81.5,397,92.4z"})});var a=It(It({},r),{},{attributeName:"opacity"}),o={tag:"circle",attributes:It(It({},n),{},{cx:"256",cy:"364",r:"28"}),children:[]};return t||o.children.push({tag:"animate",attributes:It(It({},r),{},{attributeName:"r",values:"28;14;28;28;14;28;"})},{tag:"animate",attributes:It(It({},a),{},{values:"1;0;1;1;0;1;"})}),e.push(o),e.push({tag:"path",attributes:It(It({},n),{},{opacity:"1",d:"M263.7,312h-16c-6.6,0-12-5.4-12-12c0-71,77.4-63.9,77.4-107.8c0-20-17.8-40.2-57.4-40.2c-29.1,0-44.3,9.6-59.2,28.7 c-3.9,5-11.1,6-16.2,2.4l-13.1-9.2c-5.6-3.9-6.9-11.8-2.6-17.2c21.2-27.2,46.4-44.7,91.2-44.7c52.3,0,97.4,29.8,97.4,80.2 c0,67.6-77.4,63.5-77.4,107.8C275.7,306.6,270.3,312,263.7,312z"}),children:t?[]:[{tag:"animate",attributes:It(It({},a),{},{values:"1;0;0;0;0;1;"})}]}),t||e.push({tag:"path",attributes:It(It({},n),{},{opacity:"0",d:"M232.5,134.5l7,168c0.3,6.4,5.6,11.5,12,11.5h9c6.4,0,11.7-5.1,12-11.5l7-168c0.3-6.8-5.2-12.5-12-12.5h-23 C237.7,122,232.2,127.7,232.5,134.5z"}),children:[{tag:"animate",attributes:It(It({},a),{},{values:"0;0;1;1;0;0;"})}]}),{tag:"g",attributes:{class:"missing"},children:e}}}},{hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-symbol");return e.symbol=null!==n&&(""===n||n),e}}}}],rr={},Object.keys(ar).forEach(function(e){-1===or.indexOf(e)&&delete ar[e]}),nr.forEach(function(e){var t=e.mixout?e.mixout():{};if(Object.keys(t).forEach(function(e){"function"==typeof t[e]&&(ra[e]=t[e]),"object"===Lt(t[e])&&Object.keys(t[e]).forEach(function(n){ra[e]||(ra[e]={}),ra[e][n]=t[e][n]})}),e.hooks){var n=e.hooks();Object.keys(n).forEach(function(e){rr[e]||(rr[e]=[]),rr[e].push(n[e])})}e.provides&&e.provides(ar)});var sa=dr.parse,ca=dr.icon,ua="function"==typeof Symbol&&Symbol.for,fa=ua?Symbol.for("react.element"):60103,da=ua?Symbol.for("react.portal"):60106,pa=ua?Symbol.for("react.fragment"):60107,ma=ua?Symbol.for("react.strict_mode"):60108,ha=ua?Symbol.for("react.profiler"):60114,ga=ua?Symbol.for("react.provider"):60109,ya=ua?Symbol.for("react.context"):60110,va=ua?Symbol.for("react.async_mode"):60111,ba=ua?Symbol.for("react.concurrent_mode"):60111,Ea=ua?Symbol.for("react.forward_ref"):60112,Ta=ua?Symbol.for("react.suspense"):60113,Sa=ua?Symbol.for("react.suspense_list"):60120,wa=ua?Symbol.for("react.memo"):60115,Na=ua?Symbol.for("react.lazy"):60116,Ra=ua?Symbol.for("react.block"):60121,Oa=ua?Symbol.for("react.fundamental"):60117,Ca=ua?Symbol.for("react.responder"):60118,xa=ua?Symbol.for("react.scope"):60119;function ka(e){if("object"==typeof e&&null!==e){var t=e.$$typeof;switch(t){case fa:switch(e=e.type){case va:case ba:case pa:case ha:case ma:case Ta:return e;default:switch(e=e&&e.$$typeof){case ya:case Ea:case Na:case wa:case ga:return e;default:return t}}case da:return t}}}function Aa(e){return ka(e)===ba}var Ia={AsyncMode:va,ConcurrentMode:ba,ContextConsumer:ya,ContextProvider:ga,Element:fa,ForwardRef:Ea,Fragment:pa,Lazy:Na,Memo:wa,Portal:da,Profiler:ha,StrictMode:ma,Suspense:Ta,isAsyncMode:function(e){return Aa(e)||ka(e)===va},isConcurrentMode:Aa,isContextConsumer:function(e){return ka(e)===ya},isContextProvider:function(e){return ka(e)===ga},isElement:function(e){return"object"==typeof e&&null!==e&&e.$$typeof===fa},isForwardRef:function(e){return ka(e)===Ea},isFragment:function(e){return ka(e)===pa},isLazy:function(e){return ka(e)===Na},isMemo:function(e){return ka(e)===wa},isPortal:function(e){return ka(e)===da},isProfiler:function(e){return ka(e)===ha},isStrictMode:function(e){return ka(e)===ma},isSuspense:function(e){return ka(e)===Ta},isValidElementType:function(e){return"string"==typeof e||"function"==typeof e||e===pa||e===ba||e===ha||e===ma||e===Ta||e===Sa||"object"==typeof e&&null!==e&&(e.$$typeof===Na||e.$$typeof===wa||e.$$typeof===ga||e.$$typeof===ya||e.$$typeof===Ea||e.$$typeof===Oa||e.$$typeof===Ca||e.$$typeof===xa||e.$$typeof===Ra)},typeOf:ka};function La(){}function _a(){}Le(function(e,t){}),Le(function(e){e.exports=Ia}),_a.resetWarningCache=La;var Pa=Le(function(e){e.exports=function(){function e(e,t,n,r,a,o){if("SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED"!==o){var i=new Error("Calling PropTypes validators directly is not supported by the `prop-types` package. Use PropTypes.checkPropTypes() to call them. Read more at http://fb.me/use-check-prop-types");throw i.name="Invariant Violation",i}}function t(){return e}e.isRequired=e;var n={array:e,bigint:e,bool:e,func:e,number:e,object:e,string:e,symbol:e,any:e,arrayOf:t,element:e,elementType:e,instanceOf:t,node:e,objectOf:t,oneOf:t,oneOfType:t,shape:t,exact:t,checkPropTypes:_a,resetWarningCache:La};return n.PropTypes=n,n}()});function Ma(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function Da(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?Ma(Object(n),!0).forEach(function(t){ja(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):Ma(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}function Ua(e){return(Ua="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function ja(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function Fa(e,t){if(null==e)return{};var n,r,a=function(e,t){if(null==e)return{};var n,r,a={},o=Object.keys(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||(a[n]=e[n]);return a}(e,t);if(Object.getOwnPropertySymbols){var o=Object.getOwnPropertySymbols(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||Object.prototype.propertyIsEnumerable.call(e,n)&&(a[n]=e[n])}return a}function za(e){return function(e){if(Array.isArray(e))return Ba(e)}(e)||function(e){if("undefined"!=typeof Symbol&&null!=e[Symbol.iterator]||null!=e["@@iterator"])return Array.from(e)}(e)||function(e,t){if(e){if("string"==typeof e)return Ba(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?Ba(e,t):void 0}}(e)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function Ba(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}function Ha(e){return t=e,(t-=0)==t?e:(e=e.replace(/[\-_\s]+(.)?/g,function(e,t){return t?t.toUpperCase():""})).substr(0,1).toLowerCase()+e.substr(1);var t}var Va=["style"];function Wa(e){return e.split(";").map(function(e){return e.trim()}).filter(function(e){return e}).reduce(function(e,t){var n,r=t.indexOf(":"),a=Ha(t.slice(0,r)),o=t.slice(r+1).trim();return a.startsWith("webkit")?e[(n=a,n.charAt(0).toUpperCase()+n.slice(1))]=o:e[a]=o,e},{})}var Ga=!1;try{Ga=!0}catch(pa){}function Ya(e){return e&&"object"===Ua(e)&&e.prefix&&e.iconName&&e.icon?e:sa.icon?sa.icon(e):null===e?null:e&&"object"===Ua(e)&&e.prefix&&e.iconName?e:Array.isArray(e)&&2===e.length?{prefix:e[0],iconName:e[1]}:"string"==typeof e?{prefix:"fas",iconName:e}:void 0}function $a(e,t){return Array.isArray(t)&&t.length>0||!Array.isArray(t)&&t?ja({},e,t):{}}var Xa=["forwardedRef"];function qa(e){var t=e.forwardedRef,n=Fa(e,Xa),r=n.mask,a=n.symbol,o=n.className,i=n.title,l=n.titleId,s=n.maskId,c=Ya(n.icon),u=$a("classes",[].concat(za(function(e){var t,n=e.flip,r=e.size,a=e.rotation,o=e.pull,i=(ja(t={"fa-beat":e.beat,"fa-fade":e.fade,"fa-beat-fade":e.beatFade,"fa-bounce":e.bounce,"fa-shake":e.shake,"fa-flash":e.flash,"fa-spin":e.spin,"fa-spin-reverse":e.spinReverse,"fa-spin-pulse":e.spinPulse,"fa-pulse":e.pulse,"fa-fw":e.fixedWidth,"fa-inverse":e.inverse,"fa-border":e.border,"fa-li":e.listItem,"fa-flip-horizontal":"horizontal"===n||"both"===n,"fa-flip-vertical":"vertical"===n||"both"===n},"fa-".concat(r),null!=r),ja(t,"fa-rotate-".concat(a),null!=a&&0!==a),ja(t,"fa-pull-".concat(o),null!=o),ja(t,"fa-swap-opacity",e.swapOpacity),t);return Object.keys(i).map(function(e){return i[e]?e:null}).filter(function(e){return e})}(n)),za(o.split(" ")))),f=$a("transform","string"==typeof n.transform?sa.transform(n.transform):n.transform),d=$a("mask",Ya(r)),p=ca(c,Da(Da(Da(Da({},u),f),d),{},{symbol:a,title:i,titleId:l,maskId:s}));if(!p)return function(){var e;!Ga&&console&&"function"==typeof console.error&&(e=console).error.apply(e,arguments)}("Could not find icon",c),null;var m=p.abstract,h={ref:t};return Object.keys(n).forEach(function(e){qa.defaultProps.hasOwnProperty(e)||(h[e]=n[e])}),Ka(m[0],h)}qa.displayName="FontAwesomeIcon",qa.propTypes={beat:Pa.bool,border:Pa.bool,bounce:Pa.bool,className:Pa.string,fade:Pa.bool,flash:Pa.bool,mask:Pa.oneOfType([Pa.object,Pa.array,Pa.string]),maskId:Pa.string,fixedWidth:Pa.bool,inverse:Pa.bool,flip:Pa.oneOf(["horizontal","vertical","both"]),icon:Pa.oneOfType([Pa.object,Pa.array,Pa.string]),listItem:Pa.bool,pull:Pa.oneOf(["right","left"]),pulse:Pa.bool,rotation:Pa.oneOf([0,90,180,270]),shake:Pa.bool,size:Pa.oneOf(["2xs","xs","sm","lg","xl","2xl","1x","2x","3x","4x","5x","6x","7x","8x","9x","10x"]),spin:Pa.bool,spinPulse:Pa.bool,spinReverse:Pa.bool,symbol:Pa.oneOfType([Pa.bool,Pa.string]),title:Pa.string,titleId:Pa.string,transform:Pa.oneOfType([Pa.string,Pa.object]),swapOpacity:Pa.bool},qa.defaultProps={border:!1,className:"",mask:null,maskId:null,fixedWidth:!1,inverse:!1,flip:null,icon:null,listItem:!1,pull:null,pulse:!1,rotation:null,size:null,spin:!1,beat:!1,fade:!1,beatFade:!1,bounce:!1,shake:!1,symbol:!1,title:"",titleId:null,transform:null,swapOpacity:!1};var Ka=function e(t,n){var r=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{};if("string"==typeof n)return n;var a=(n.children||[]).map(function(n){return e(t,n)}),o=Object.keys(n.attributes||{}).reduce(function(e,t){var r=n.attributes[t];switch(t){case"class":e.attrs.className=r,delete n.attributes.class;break;case"style":e.attrs.style=Wa(r);break;default:0===t.indexOf("aria-")||0===t.indexOf("data-")?e.attrs[t.toLowerCase()]=r:e.attrs[Ha(t)]=r}return e},{attrs:{}}),i=r.style,l=void 0===i?{}:i,s=Fa(r,Va);return o.attrs.style=Da(Da({},o.attrs.style),l),t.apply(void 0,[n.tag,Da(Da({},o.attrs),s)].concat(za(a)))}.bind(null,c.createElement),Ja={prefix:"fas",iconName:"angle-down",icon:[384,512,[8964],"f107","M192 384c-8.188 0-16.38-3.125-22.62-9.375l-160-160c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0L192 306.8l137.4-137.4c12.5-12.5 32.75-12.5 45.25 0s12.5 32.75 0 45.25l-160 160C208.4 380.9 200.2 384 192 384z"]},Qa={prefix:"fas",iconName:"angle-right",icon:[256,512,[8250],"f105","M64 448c-8.188 0-16.38-3.125-22.62-9.375c-12.5-12.5-12.5-32.75 0-45.25L178.8 256L41.38 118.6c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0l160 160c12.5 12.5 12.5 32.75 0 45.25l-160 160C80.38 444.9 72.19 448 64 448z"]},Za={prefix:"fas",iconName:"angle-up",icon:[384,512,[8963],"f106","M352 352c-8.188 0-16.38-3.125-22.62-9.375L192 205.3l-137.4 137.4c-12.5 12.5-32.75 12.5-45.25 0s-12.5-32.75 0-45.25l160-160c12.5-12.5 32.75-12.5 45.25 0l160 160c12.5 12.5 12.5 32.75 0 45.25C368.4 348.9 360.2 352 352 352z"]},eo={prefix:"fas",iconName:"asterisk",icon:[448,512,[10033,61545],"2a","M417.1 368c-5.937 10.27-16.69 16-27.75 16c-5.422 0-10.92-1.375-15.97-4.281L256 311.4V448c0 17.67-14.33 32-31.1 32S192 465.7 192 448V311.4l-118.3 68.29C68.67 382.6 63.17 384 57.75 384c-11.06 0-21.81-5.734-27.75-16c-8.828-15.31-3.594-34.88 11.72-43.72L159.1 256L41.72 187.7C26.41 178.9 21.17 159.3 29.1 144C36.63 132.5 49.26 126.7 61.65 128.2C65.78 128.7 69.88 130.1 73.72 132.3L192 200.6V64c0-17.67 14.33-32 32-32S256 46.33 256 64v136.6l118.3-68.29c3.838-2.213 7.939-3.539 12.07-4.051C398.7 126.7 411.4 132.5 417.1 144c8.828 15.31 3.594 34.88-11.72 43.72L288 256l118.3 68.28C421.6 333.1 426.8 352.7 417.1 368z"]},to={prefix:"fas",iconName:"bomb",icon:[512,512,[128163],"f1e2","M440.8 4.994C441.9 1.99 444.8 0 448 0C451.2 0 454.1 1.99 455.2 4.994L469.3 42.67L507 56.79C510 57.92 512 60.79 512 64C512 67.21 510 70.08 507 71.21L469.3 85.33L455.2 123C454.1 126 451.2 128 448 128C444.8 128 441.9 126 440.8 123L426.7 85.33L388.1 71.21C385.1 70.08 384 67.21 384 64C384 60.79 385.1 57.92 388.1 56.79L426.7 42.67L440.8 4.994zM289.4 97.37C301.9 84.88 322.1 84.88 334.6 97.37L363.3 126.1L380.7 108.7C386.9 102.4 397.1 102.4 403.3 108.7C409.6 114.9 409.6 125.1 403.3 131.3L385.9 148.7L414.6 177.4C427.1 189.9 427.1 210.1 414.6 222.6L403.8 233.5C411.7 255.5 416 279.3 416 304C416 418.9 322.9 512 208 512C93.12 512 0 418.9 0 304C0 189.1 93.12 96 208 96C232.7 96 256.5 100.3 278.5 108.3L289.4 97.37zM95.1 296C95.1 238.6 142.6 192 199.1 192H207.1C216.8 192 223.1 184.8 223.1 176C223.1 167.2 216.8 160 207.1 160H199.1C124.9 160 63.1 220.9 63.1 296V304C63.1 312.8 71.16 320 79.1 320C88.84 320 95.1 312.8 95.1 304V296z"]},no={prefix:"fas",iconName:"check",icon:[448,512,[10004,10003],"f00c","M438.6 105.4C451.1 117.9 451.1 138.1 438.6 150.6L182.6 406.6C170.1 419.1 149.9 419.1 137.4 406.6L9.372 278.6C-3.124 266.1-3.124 245.9 9.372 233.4C21.87 220.9 42.13 220.9 54.63 233.4L159.1 338.7L393.4 105.4C405.9 92.88 426.1 92.88 438.6 105.4H438.6z"]},ro={prefix:"fas",iconName:"circle-question",icon:[512,512,[62108,"question-circle"],"f059","M256 0C114.6 0 0 114.6 0 256s114.6 256 256 256s256-114.6 256-256S397.4 0 256 0zM256 400c-18 0-32-14-32-32s13.1-32 32-32c17.1 0 32 14 32 32S273.1 400 256 400zM325.1 258L280 286V288c0 13-11 24-24 24S232 301 232 288V272c0-8 4-16 12-21l57-34C308 213 312 206 312 198C312 186 301.1 176 289.1 176h-51.1C225.1 176 216 186 216 198c0 13-11 24-24 24s-24-11-24-24C168 159 199 128 237.1 128h51.1C329 128 360 159 360 198C360 222 347 245 325.1 258z"]},ao={prefix:"fas",iconName:"code",icon:[640,512,[],"f121","M414.8 40.79L286.8 488.8C281.9 505.8 264.2 515.6 247.2 510.8C230.2 505.9 220.4 488.2 225.2 471.2L353.2 23.21C358.1 6.216 375.8-3.624 392.8 1.232C409.8 6.087 419.6 23.8 414.8 40.79H414.8zM518.6 121.4L630.6 233.4C643.1 245.9 643.1 266.1 630.6 278.6L518.6 390.6C506.1 403.1 485.9 403.1 473.4 390.6C460.9 378.1 460.9 357.9 473.4 345.4L562.7 256L473.4 166.6C460.9 154.1 460.9 133.9 473.4 121.4C485.9 108.9 506.1 108.9 518.6 121.4V121.4zM166.6 166.6L77.25 256L166.6 345.4C179.1 357.9 179.1 378.1 166.6 390.6C154.1 403.1 133.9 403.1 121.4 390.6L9.372 278.6C-3.124 266.1-3.124 245.9 9.372 233.4L121.4 121.4C133.9 108.9 154.1 108.9 166.6 121.4C179.1 133.9 179.1 154.1 166.6 166.6V166.6z"]},oo={prefix:"fas",iconName:"code-branch",icon:[448,512,[],"f126","M160 80C160 112.8 140.3 140.1 112 153.3V241.1C130.8 230.2 152.7 224 176 224H272C307.3 224 336 195.3 336 160V153.3C307.7 140.1 288 112.8 288 80C288 35.82 323.8 0 368 0C412.2 0 448 35.82 448 80C448 112.8 428.3 140.1 400 153.3V160C400 230.7 342.7 288 272 288H176C140.7 288 112 316.7 112 352V358.7C140.3 371 160 399.2 160 432C160 476.2 124.2 512 80 512C35.82 512 0 476.2 0 432C0 399.2 19.75 371 48 358.7V153.3C19.75 140.1 0 112.8 0 80C0 35.82 35.82 0 80 0C124.2 0 160 35.82 160 80V80zM80 104C93.25 104 104 93.25 104 80C104 66.75 93.25 56 80 56C66.75 56 56 66.75 56 80C56 93.25 66.75 104 80 104zM368 56C354.7 56 344 66.75 344 80C344 93.25 354.7 104 368 104C381.3 104 392 93.25 392 80C392 66.75 381.3 56 368 56zM80 456C93.25 456 104 445.3 104 432C104 418.7 93.25 408 80 408C66.75 408 56 418.7 56 432C56 445.3 66.75 456 80 456z"]},io={prefix:"fas",iconName:"cookie-bite",icon:[512,512,[],"f564","M494.6 255.9c-65.63-.8203-118.6-54.14-118.6-119.9c-65.74 0-119.1-52.97-119.8-118.6c-25.66-3.867-51.8 .2346-74.77 12.07L116.7 62.41C93.35 74.36 74.36 93.35 62.41 116.7L29.6 181.2c-11.95 23.44-16.17 49.92-12.07 75.94l11.37 71.48c4.102 25.9 16.29 49.8 34.81 68.32l51.36 51.39C133.6 466.9 157.3 479 183.2 483.1l71.84 11.37c25.9 4.101 52.27-.1172 75.59-11.95l64.81-33.05c23.32-11.84 42.31-30.82 54.14-54.14l32.93-64.57C494.3 307.7 498.5 281.4 494.6 255.9zM176 367.1c-17.62 0-32-14.37-32-31.1s14.38-31.1 32-31.1s32 14.37 32 31.1S193.6 367.1 176 367.1zM208 208c-17.62 0-32-14.37-32-31.1s14.38-31.1 32-31.1s32 14.37 32 31.1S225.6 208 208 208zM368 335.1c-17.62 0-32-14.37-32-31.1s14.38-31.1 32-31.1s32 14.37 32 31.1S385.6 335.1 368 335.1z"]},lo={prefix:"fas",iconName:"copy",icon:[512,512,[],"f0c5","M384 96L384 0h-112c-26.51 0-48 21.49-48 48v288c0 26.51 21.49 48 48 48H464c26.51 0 48-21.49 48-48V128h-95.1C398.4 128 384 113.6 384 96zM416 0v96h96L416 0zM192 352V128h-144c-26.51 0-48 21.49-48 48v288c0 26.51 21.49 48 48 48h192c26.51 0 48-21.49 48-48L288 416h-32C220.7 416 192 387.3 192 352z"]},so={prefix:"fas",iconName:"database",icon:[448,512,[],"f1c0","M448 80V128C448 172.2 347.7 208 224 208C100.3 208 0 172.2 0 128V80C0 35.82 100.3 0 224 0C347.7 0 448 35.82 448 80zM393.2 214.7C413.1 207.3 433.1 197.8 448 186.1V288C448 332.2 347.7 368 224 368C100.3 368 0 332.2 0 288V186.1C14.93 197.8 34.02 207.3 54.85 214.7C99.66 230.7 159.5 240 224 240C288.5 240 348.3 230.7 393.2 214.7V214.7zM54.85 374.7C99.66 390.7 159.5 400 224 400C288.5 400 348.3 390.7 393.2 374.7C413.1 367.3 433.1 357.8 448 346.1V432C448 476.2 347.7 512 224 512C100.3 512 0 476.2 0 432V346.1C14.93 357.8 34.02 367.3 54.85 374.7z"]},co={prefix:"fas",iconName:"file",icon:[384,512,[128459,61462,128196],"f15b","M0 64C0 28.65 28.65 0 64 0H224V128C224 145.7 238.3 160 256 160H384V448C384 483.3 355.3 512 320 512H64C28.65 512 0 483.3 0 448V64zM256 128V0L384 128H256z"]},uo={prefix:"fas",iconName:"hourglass",icon:[384,512,[62032,9203,"hourglass-2","hourglass-half"],"f254","M352 0C369.7 0 384 14.33 384 32C384 49.67 369.7 64 352 64V74.98C352 117.4 335.1 158.1 305.1 188.1L237.3 256L305.1 323.9C335.1 353.9 352 394.6 352 437V448C369.7 448 384 462.3 384 480C384 497.7 369.7 512 352 512H32C14.33 512 0 497.7 0 480C0 462.3 14.33 448 32 448V437C32 394.6 48.86 353.9 78.86 323.9L146.7 256L78.86 188.1C48.86 158.1 32 117.4 32 74.98V64C14.33 64 0 49.67 0 32C0 14.33 14.33 0 32 0H352zM111.1 128H272C282.4 112.4 288 93.98 288 74.98V64H96V74.98C96 93.98 101.6 112.4 111.1 128zM111.1 384H272C268.5 378.7 264.5 373.7 259.9 369.1L192 301.3L124.1 369.1C119.5 373.7 115.5 378.7 111.1 384V384z"]},fo={prefix:"fas",iconName:"layer-group",icon:[512,512,[],"f5fd","M232.5 5.171C247.4-1.718 264.6-1.718 279.5 5.171L498.1 106.2C506.6 110.1 512 118.6 512 127.1C512 137.3 506.6 145.8 498.1 149.8L279.5 250.8C264.6 257.7 247.4 257.7 232.5 250.8L13.93 149.8C5.438 145.8 0 137.3 0 127.1C0 118.6 5.437 110.1 13.93 106.2L232.5 5.171zM498.1 234.2C506.6 238.1 512 246.6 512 255.1C512 265.3 506.6 273.8 498.1 277.8L279.5 378.8C264.6 385.7 247.4 385.7 232.5 378.8L13.93 277.8C5.438 273.8 0 265.3 0 255.1C0 246.6 5.437 238.1 13.93 234.2L67.13 209.6L219.1 279.8C242.5 290.7 269.5 290.7 292.9 279.8L444.9 209.6L498.1 234.2zM292.9 407.8L444.9 337.6L498.1 362.2C506.6 366.1 512 374.6 512 383.1C512 393.3 506.6 401.8 498.1 405.8L279.5 506.8C264.6 513.7 247.4 513.7 232.5 506.8L13.93 405.8C5.438 401.8 0 393.3 0 383.1C0 374.6 5.437 366.1 13.93 362.2L67.13 337.6L219.1 407.8C242.5 418.7 269.5 418.7 292.9 407.8V407.8z"]},po={prefix:"fas",iconName:"lightbulb",icon:[384,512,[128161],"f0eb","M112.1 454.3c0 6.297 1.816 12.44 5.284 17.69l17.14 25.69c5.25 7.875 17.17 14.28 26.64 14.28h61.67c9.438 0 21.36-6.401 26.61-14.28l17.08-25.68c2.938-4.438 5.348-12.37 5.348-17.7L272 415.1h-160L112.1 454.3zM191.4 .0132C89.44 .3257 16 82.97 16 175.1c0 44.38 16.44 84.84 43.56 115.8c16.53 18.84 42.34 58.23 52.22 91.45c.0313 .25 .0938 .5166 .125 .7823h160.2c.0313-.2656 .0938-.5166 .125-.7823c9.875-33.22 35.69-72.61 52.22-91.45C351.6 260.8 368 220.4 368 175.1C368 78.61 288.9-.2837 191.4 .0132zM192 96.01c-44.13 0-80 35.89-80 79.1C112 184.8 104.8 192 96 192S80 184.8 80 176c0-61.76 50.25-111.1 112-111.1c8.844 0 16 7.159 16 16S200.8 96.01 192 96.01z"]},mo={prefix:"fas",iconName:"list-ul",icon:[512,512,["list-dots"],"f0ca","M16 96C16 69.49 37.49 48 64 48C90.51 48 112 69.49 112 96C112 122.5 90.51 144 64 144C37.49 144 16 122.5 16 96zM480 64C497.7 64 512 78.33 512 96C512 113.7 497.7 128 480 128H192C174.3 128 160 113.7 160 96C160 78.33 174.3 64 192 64H480zM480 224C497.7 224 512 238.3 512 256C512 273.7 497.7 288 480 288H192C174.3 288 160 273.7 160 256C160 238.3 174.3 224 192 224H480zM480 384C497.7 384 512 398.3 512 416C512 433.7 497.7 448 480 448H192C174.3 448 160 433.7 160 416C160 398.3 174.3 384 192 384H480zM16 416C16 389.5 37.49 368 64 368C90.51 368 112 389.5 112 416C112 442.5 90.51 464 64 464C37.49 464 16 442.5 16 416zM112 256C112 282.5 90.51 304 64 304C37.49 304 16 282.5 16 256C16 229.5 37.49 208 64 208C90.51 208 112 229.5 112 256z"]},ho={prefix:"fas",iconName:"paint-roller",icon:[512,512,[],"f5aa","M0 64C0 28.65 28.65 0 64 0H352C387.3 0 416 28.65 416 64V128C416 163.3 387.3 192 352 192H64C28.65 192 0 163.3 0 128V64zM160 352C160 334.3 174.3 320 192 320V304C192 259.8 227.8 224 272 224H416C433.7 224 448 209.7 448 192V69.46C485.3 82.64 512 118.2 512 160V192C512 245 469 288 416 288H272C263.2 288 256 295.2 256 304V320C273.7 320 288 334.3 288 352V480C288 497.7 273.7 512 256 512H192C174.3 512 160 497.7 160 480V352z"]},go={prefix:"fas",iconName:"pencil",icon:[512,512,[61504,9999,"pencil-alt"],"f303","M421.7 220.3L188.5 453.4L154.6 419.5L158.1 416H112C103.2 416 96 408.8 96 400V353.9L92.51 357.4C87.78 362.2 84.31 368 82.42 374.4L59.44 452.6L137.6 429.6C143.1 427.7 149.8 424.2 154.6 419.5L188.5 453.4C178.1 463.8 165.2 471.5 151.1 475.6L30.77 511C22.35 513.5 13.24 511.2 7.03 504.1C.8198 498.8-1.502 489.7 .976 481.2L36.37 360.9C40.53 346.8 48.16 333.9 58.57 323.5L291.7 90.34L421.7 220.3zM492.7 58.75C517.7 83.74 517.7 124.3 492.7 149.3L444.3 197.7L314.3 67.72L362.7 19.32C387.7-5.678 428.3-5.678 453.3 19.32L492.7 58.75z"]},yo={prefix:"fas",iconName:"right-left",icon:[512,512,["exchange-alt"],"f362","M32 160h319.9l.0791 72c0 9.547 5.652 18.19 14.41 22c8.754 3.812 18.93 2.078 25.93-4.406l112-104c10.24-9.5 10.24-25.69 0-35.19l-112-104c-6.992-6.484-17.17-8.217-25.93-4.408c-8.758 3.816-14.41 12.46-14.41 22L351.9 96H32C14.31 96 0 110.3 0 127.1S14.31 160 32 160zM480 352H160.1L160 279.1c0-9.547-5.652-18.19-14.41-22C136.9 254.2 126.7 255.9 119.7 262.4l-112 104c-10.24 9.5-10.24 25.69 0 35.19l112 104c6.992 6.484 17.17 8.219 25.93 4.406C154.4 506.2 160 497.5 160 488L160.1 416H480c17.69 0 32-14.31 32-32S497.7 352 480 352z"]},vo={prefix:"fas",iconName:"robot",icon:[640,512,[129302],"f544","M9.375 233.4C3.375 239.4 0 247.5 0 256v128c0 8.5 3.375 16.62 9.375 22.62S23.5 416 32 416h32V224H32C23.5 224 15.38 227.4 9.375 233.4zM464 96H352V32c0-17.62-14.38-32-32-32S288 14.38 288 32v64H176C131.8 96 96 131.8 96 176V448c0 35.38 28.62 64 64 64h320c35.38 0 64-28.62 64-64V176C544 131.8 508.3 96 464 96zM256 416H192v-32h64V416zM224 296C201.9 296 184 278.1 184 256S201.9 216 224 216S264 233.9 264 256S246.1 296 224 296zM352 416H288v-32h64V416zM448 416h-64v-32h64V416zM416 296c-22.12 0-40-17.88-40-40S393.9 216 416 216S456 233.9 456 256S438.1 296 416 296zM630.6 233.4C624.6 227.4 616.5 224 608 224h-32v192h32c8.5 0 16.62-3.375 22.62-9.375S640 392.5 640 384V256C640 247.5 636.6 239.4 630.6 233.4z"]},bo={prefix:"fas",iconName:"rotate-right",icon:[512,512,["redo-alt","rotate-forward"],"f2f9","M468.9 32.11c13.87 0 27.18 10.77 27.18 27.04v145.9c0 10.59-8.584 19.17-19.17 19.17h-145.7c-16.28 0-27.06-13.32-27.06-27.2c0-6.634 2.461-13.4 7.96-18.9l45.12-45.14c-28.22-23.14-63.85-36.64-101.3-36.64c-88.09 0-159.8 71.69-159.8 159.8S167.8 415.9 255.9 415.9c73.14 0 89.44-38.31 115.1-38.31c18.48 0 31.97 15.04 31.97 31.96c0 35.04-81.59 70.41-147 70.41c-123.4 0-223.9-100.5-223.9-223.9S132.6 32.44 256 32.44c54.6 0 106.2 20.39 146.4 55.26l47.6-47.63C455.5 34.57 462.3 32.11 468.9 32.11z"]},Eo={prefix:"fas",iconName:"satellite-dish",icon:[512,512,[128225],"f7c0","M216 104C202.8 104 192 114.8 192 128s10.75 24 24 24c79.41 0 144 64.59 144 144C360 309.3 370.8 320 384 320s24-10.75 24-24C408 190.1 321.9 104 216 104zM224 0C206.3 0 192 14.31 192 32s14.33 32 32 32c123.5 0 224 100.5 224 224c0 17.69 14.33 32 32 32s32-14.31 32-32C512 129.2 382.8 0 224 0zM188.9 346l27.37-27.37c2.625 .625 5.059 1.506 7.809 1.506c17.75 0 31.99-14.26 31.99-32c0-17.62-14.24-32.01-31.99-32.01c-17.62 0-31.99 14.38-31.99 32.01c0 2.875 .8099 5.25 1.56 7.875L166.2 323.4L49.37 206.5c-7.25-7.25-20.12-6-24.1 3c-41.75 77.88-29.88 176.7 35.75 242.4c65.62 65.62 164.6 77.5 242.4 35.75c9.125-5 10.38-17.75 3-25L188.9 346z"]},To={prefix:"fas",iconName:"shuffle",icon:[512,512,[128256,"random"],"f074","M424.1 287c-15.13-15.12-40.1-4.426-40.1 16.97V352H336L153.6 108.8C147.6 100.8 138.1 96 128 96H32C14.31 96 0 110.3 0 128s14.31 32 32 32h80l182.4 243.2C300.4 411.3 309.9 416 320 416h63.97v47.94c0 21.39 25.86 32.12 40.99 17l79.1-79.98c9.387-9.387 9.387-24.59 0-33.97L424.1 287zM336 160h47.97v48.03c0 21.39 25.87 32.09 40.1 16.97l79.1-79.98c9.387-9.391 9.385-24.59-.0013-33.97l-79.1-79.98c-15.13-15.12-40.99-4.391-40.99 17V96H320c-10.06 0-19.56 4.75-25.59 12.81L254 162.7L293.1 216L336 160zM112 352H32c-17.69 0-32 14.31-32 32s14.31 32 32 32h96c10.06 0 19.56-4.75 25.59-12.81l40.4-53.87L154 296L112 352z"]},So={prefix:"fas",iconName:"sliders",icon:[512,512,["sliders-h"],"f1de","M0 416C0 398.3 14.33 384 32 384H86.66C99 355.7 127.2 336 160 336C192.8 336 220.1 355.7 233.3 384H480C497.7 384 512 398.3 512 416C512 433.7 497.7 448 480 448H233.3C220.1 476.3 192.8 496 160 496C127.2 496 99 476.3 86.66 448H32C14.33 448 0 433.7 0 416V416zM192 416C192 398.3 177.7 384 160 384C142.3 384 128 398.3 128 416C128 433.7 142.3 448 160 448C177.7 448 192 433.7 192 416zM352 176C384.8 176 412.1 195.7 425.3 224H480C497.7 224 512 238.3 512 256C512 273.7 497.7 288 480 288H425.3C412.1 316.3 384.8 336 352 336C319.2 336 291 316.3 278.7 288H32C14.33 288 0 273.7 0 256C0 238.3 14.33 224 32 224H278.7C291 195.7 319.2 176 352 176zM384 256C384 238.3 369.7 224 352 224C334.3 224 320 238.3 320 256C320 273.7 334.3 288 352 288C369.7 288 384 273.7 384 256zM480 64C497.7 64 512 78.33 512 96C512 113.7 497.7 128 480 128H265.3C252.1 156.3 224.8 176 192 176C159.2 176 131 156.3 118.7 128H32C14.33 128 0 113.7 0 96C0 78.33 14.33 64 32 64H118.7C131 35.75 159.2 16 192 16C224.8 16 252.1 35.75 265.3 64H480zM160 96C160 113.7 174.3 128 192 128C209.7 128 224 113.7 224 96C224 78.33 209.7 64 192 64C174.3 64 160 78.33 160 96z"]},wo={prefix:"fas",iconName:"stopwatch",icon:[448,512,[9201],"f2f2","M272 0C289.7 0 304 14.33 304 32C304 49.67 289.7 64 272 64H256V98.45C293.5 104.2 327.7 120 355.7 143L377.4 121.4C389.9 108.9 410.1 108.9 422.6 121.4C435.1 133.9 435.1 154.1 422.6 166.6L398.5 190.8C419.7 223.3 432 262.2 432 304C432 418.9 338.9 512 224 512C109.1 512 16 418.9 16 304C16 200 92.32 113.8 192 98.45V64H176C158.3 64 144 49.67 144 32C144 14.33 158.3 0 176 0L272 0zM248 192C248 178.7 237.3 168 224 168C210.7 168 200 178.7 200 192V320C200 333.3 210.7 344 224 344C237.3 344 248 333.3 248 320V192z"]},No={prefix:"fas",iconName:"table-cells",icon:[512,512,["th"],"f00a","M448 32C483.3 32 512 60.65 512 96V416C512 451.3 483.3 480 448 480H64C28.65 480 0 451.3 0 416V96C0 60.65 28.65 32 64 32H448zM152 96H64V160H152V96zM208 160H296V96H208V160zM448 96H360V160H448V96zM64 288H152V224H64V288zM296 224H208V288H296V224zM360 288H448V224H360V288zM152 352H64V416H152V352zM208 416H296V352H208V416zM448 352H360V416H448V352z"]},Ro={prefix:"fas",iconName:"terminal",icon:[576,512,[],"f120","M9.372 86.63C-3.124 74.13-3.124 53.87 9.372 41.37C21.87 28.88 42.13 28.88 54.63 41.37L246.6 233.4C259.1 245.9 259.1 266.1 246.6 278.6L54.63 470.6C42.13 483.1 21.87 483.1 9.372 470.6C-3.124 458.1-3.124 437.9 9.372 425.4L178.7 256L9.372 86.63zM544 416C561.7 416 576 430.3 576 448C576 465.7 561.7 480 544 480H256C238.3 480 224 465.7 224 448C224 430.3 238.3 416 256 416H544z"]},Oo={prefix:"fas",iconName:"triangle-exclamation",icon:[512,512,[9888,"exclamation-triangle","warning"],"f071","M506.3 417l-213.3-364c-16.33-28-57.54-28-73.98 0l-213.2 364C-10.59 444.9 9.849 480 42.74 480h426.6C502.1 480 522.6 445 506.3 417zM232 168c0-13.25 10.75-24 24-24S280 154.8 280 168v128c0 13.25-10.75 24-23.1 24S232 309.3 232 296V168zM256 416c-17.36 0-31.44-14.08-31.44-31.44c0-17.36 14.07-31.44 31.44-31.44s31.44 14.08 31.44 31.44C287.4 401.9 273.4 416 256 416z"]},Co={prefix:"fas",iconName:"up-right-from-square",icon:[512,512,["external-link-alt"],"f35d","M384 320c-17.67 0-32 14.33-32 32v96H64V160h96c17.67 0 32-14.32 32-32s-14.33-32-32-32L64 96c-35.35 0-64 28.65-64 64V448c0 35.34 28.65 64 64 64h288c35.35 0 64-28.66 64-64v-96C416 334.3 401.7 320 384 320zM488 0H352c-12.94 0-24.62 7.797-29.56 19.75c-4.969 11.97-2.219 25.72 6.938 34.88L370.8 96L169.4 297.4c-12.5 12.5-12.5 32.75 0 45.25C175.6 348.9 183.8 352 192 352s16.38-3.125 22.62-9.375L416 141.3l41.38 41.38c9.156 9.141 22.88 11.84 34.88 6.938C504.2 184.6 512 172.9 512 160V24C512 10.74 501.3 0 488 0z"]},xo={prefix:"fas",iconName:"user",icon:[448,512,[62144,128100],"f007","M224 256c70.7 0 128-57.31 128-128s-57.3-128-128-128C153.3 0 96 57.31 96 128S153.3 256 224 256zM274.7 304H173.3C77.61 304 0 381.6 0 477.3c0 19.14 15.52 34.67 34.66 34.67h378.7C432.5 512 448 496.5 448 477.3C448 381.6 370.4 304 274.7 304z"]},ko={prefix:"fas",iconName:"window-maximize",icon:[512,512,[128470],"f2d0","M448 32C483.3 32 512 60.65 512 96V416C512 451.3 483.3 480 448 480H64C28.65 480 0 451.3 0 416V96C0 60.65 28.65 32 64 32H448zM96 96C78.33 96 64 110.3 64 128C64 145.7 78.33 160 96 160H416C433.7 160 448 145.7 448 128C448 110.3 433.7 96 416 96H96z"]},Ao={prefix:"fas",iconName:"wrench",icon:[512,512,[128295],"f0ad","M507.6 122.8c-2.904-12.09-18.25-16.13-27.04-7.338l-76.55 76.56l-83.1-.0002l0-83.1l76.55-76.56c8.791-8.789 4.75-24.14-7.336-27.04c-23.69-5.693-49.34-6.111-75.92 .2484c-61.45 14.7-109.4 66.9-119.2 129.3C189.8 160.8 192.3 186.7 200.1 210.1l-178.1 178.1c-28.12 28.12-28.12 73.69 0 101.8C35.16 504.1 53.56 512 71.1 512s36.84-7.031 50.91-21.09l178.1-178.1c23.46 7.736 49.31 10.24 76.17 6.004c62.41-9.84 114.6-57.8 129.3-119.2C513.7 172.1 513.3 146.5 507.6 122.8zM80 456c-13.25 0-24-10.75-24-24c0-13.26 10.75-24 24-24s24 10.74 24 24C104 445.3 93.25 456 80 456z"]},Io={prefix:"fas",iconName:"xmark",icon:[320,512,[128473,10005,10006,10060,215,"close","multiply","remove","times"],"f00d","M310.6 361.4c12.5 12.5 12.5 32.75 0 45.25C304.4 412.9 296.2 416 288 416s-16.38-3.125-22.62-9.375L160 301.3L54.63 406.6C48.38 412.9 40.19 416 32 416S15.63 412.9 9.375 406.6c-12.5-12.5-12.5-32.75 0-45.25l105.4-105.4L9.375 150.6c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0L160 210.8l105.4-105.4c12.5-12.5 32.75-12.5 45.25 0s12.5 32.75 0 45.25l-105.4 105.4L310.6 361.4z"]};function Lo({path:e,lineNumber:t=null}){var n;const{application_path:r}=c.useContext(be),a=e.replace(r+"/","").replace(/\/Users\/.*?\//,"~/").split("/"),o=(null==(n=a.pop())?void 0:n.split("."))||[],i=o.pop(),l=o.join("."),s=String.fromCharCode(8201);return c.createElement("span",{className:"inline-flex flex-wrap items-baseline"},a.map((e,t)=>c.createElement(c.Fragment,{key:t},c.createElement("span",{key:t},e),c.createElement("span",null,s,"/",s))),c.createElement("span",{className:"font-semibold"},l),c.createElement("span",null,".",i),t&&c.createElement(c.Fragment,null,s,c.createElement("span",{className:"whitespace-nowrap"},":",s,c.createElement("span",{className:"font-mono text-xs"},t))))}function _o({frameGroup:e,onExpand:t,onSelect:n}){return"vendor"!==e.type||e.expanded?c.createElement(c.Fragment,null,e.frames.map((e,t)=>c.createElement(c.Fragment,{key:t},c.createElement("li",{key:e.frame_number,className:`px-6 sm:px-10 py-4\n ${e.selected?"bg-red-500 text-white":"cursor-pointer border-b ~border-gray-200 hover:~bg-red-500/10"}\n `,onClick:()=>n(e.frame_number)},c.createElement("div",{className:"flex items-baseline"},e.class?c.createElement(kt,{path:e.class,lineNumber:e.line_number}):c.createElement(Lo,{path:e.file,lineNumber:e.line_number})),c.createElement("div",{className:"font-semibold"},e.method)),e.selected&&c.createElement("li",{className:"z-10 mt-[-4px] sticky top-0 bg-red-500 h-[4px]"})))):c.createElement("li",{className:"group cursor-pointer px-6 @lg:px-10 py-4 flex gap-2 border-b ~border-gray-200 hover:~bg-gray-500/5 items-center",onClick:t},e.frames.length>1?`${e.frames.length} vendor frames`:"1 vendor frame",c.createElement(qa,{icon:Ja,className:"~text-gray-500 group-hover:text-indigo-500"}))}function Po({file:e,lineNumber:t=1}){const{ignitionConfig:n}=c.useContext(Te);e=(n.remoteSitesPath||"").length>0&&(n.localSitesPath||"").length>0?e.replace(n.remoteSitesPath,n.localSitesPath):e;const r=function(e){const t=e.editor||"";return Object.keys(e.editorOptions||{}).includes(t)?e.editorOptions[t]:(console.warn(`Editor '${t}' is not supported. Support editors are: ${Object.keys(e.editorOptions||{}).join(", ")}`),null)}(n);return r?r.clipboard?{url:r.url.replace("%path",e).replace("%line",t.toString()),clipboard:!0}:{url:r.url.replace("%path",encodeURIComponent(e)).replace("%line",encodeURIComponent(t)),clipboard:!1}:{url:e+":"+t,clipboard:!0}}function Mo(e){return new Date(1e3*e)}function Do(e){return JSON.stringify(e,null,4)}function Uo(e){return!!(e.glows.length||Object.values(e.context_items.dumps||[]).length||Object.values(e.context_items.logs||[]).length||Object.values(e.context_items.queries||[]).length)}const jo=["children","className"];function Fo(e){let{children:t,className:n=""}=e,r=Ce(e,jo);return c.createElement("button",Ne({type:r.type||"button",className:`w-6 h-6 rounded-full flex items-center justify-center\n text-xs ~bg-white text-indigo-500 hover:~text-indigo-600 \n transform transition-animation shadow-md hover:shadow-lg\n active:shadow-sm active:translate-y-px"\n ${n}\n `},r),t)}function zo({value:e,className:t="",alwaysVisible:n=!1,direction:r="right",outside:a=!1,children:o}){const[i,l]=c.useState(!1);function s(t){t.preventDefault(),function(e){const t=document.createElement("textarea");t.value=e,document.body.appendChild(t),t.select(),document.execCommand("copy"),document.body.removeChild(t)}(e),l(!0)}return c.useEffect(()=>{let e;return i&&(e=window.setTimeout(()=>l(!1),3e3)),()=>window.clearTimeout(e)},[i]),c.createElement("div",{className:t},o&&c.createElement("button",{onClick:s,title:"Copy to clipboard"},o),!o&&c.createElement(Fo,{onClick:s,title:"Copy to clipboard",className:`\n ${n?"":"opacity-0 transform scale-80 transition-animation delay-100"}\n ${i?"opacity-0":"group-hover:opacity-100 group-hover:scale-100"}\n `},c.createElement(qa,{icon:lo})),i&&c.createElement("p",{className:`\n absolute top-0 pointer-events-none select-none\n ${r}-0\n ${a&&"bottom"===r?"translate-y-full right-0":""}\n ${a&&"right"===r?"translate-x-full":""}\n hidden z-10 sm:inline-flex gap-2 items-center h-6 px-2 rounded-sm ~bg-white shadow text-xs font-medium whitespace-nowrap text-emerald-500\n `,onClick:()=>l(!1)},"Copied!"))}function Bo({path:e,lineNumber:t,className:n}){const{url:r,clipboard:a}=Po({file:e,lineNumber:t});return a?c.createElement(zo,{value:e,outside:!0,direction:"bottom"},c.createElement("span",{className:`hover:underline ${n}`},c.createElement(Lo,{path:e,lineNumber:t}))):c.createElement("a",{href:r,className:`hover:underline ${n}`},c.createElement(Lo,{path:e,lineNumber:t}))}function Ho(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}function Vo(e){return function(e){if(Array.isArray(e))return Ho(e)}(e)||function(e){if("undefined"!=typeof Symbol&&null!=e[Symbol.iterator]||null!=e["@@iterator"])return Array.from(e)}(e)||function(e,t){if(e){if("string"==typeof e)return Ho(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?Ho(e,t):void 0}}(e)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function Wo(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function Go(){return(Go=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(e[r]=n[r])}return e}).apply(this,arguments)}function Yo(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function $o(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?Yo(Object(n),!0).forEach(function(t){Wo(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):Yo(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}var Xo={};function qo(e){if(0===e.length||1===e.length)return e;var t,n,r=e.join(".");return Xo[r]||(Xo[r]=0===(n=(t=e).length)||1===n?t:2===n?[t[0],t[1],"".concat(t[0],".").concat(t[1]),"".concat(t[1],".").concat(t[0])]:3===n?[t[0],t[1],t[2],"".concat(t[0],".").concat(t[1]),"".concat(t[0],".").concat(t[2]),"".concat(t[1],".").concat(t[0]),"".concat(t[1],".").concat(t[2]),"".concat(t[2],".").concat(t[0]),"".concat(t[2],".").concat(t[1]),"".concat(t[0],".").concat(t[1],".").concat(t[2]),"".concat(t[0],".").concat(t[2],".").concat(t[1]),"".concat(t[1],".").concat(t[0],".").concat(t[2]),"".concat(t[1],".").concat(t[2],".").concat(t[0]),"".concat(t[2],".").concat(t[0],".").concat(t[1]),"".concat(t[2],".").concat(t[1],".").concat(t[0])]:n>=4?[t[0],t[1],t[2],t[3],"".concat(t[0],".").concat(t[1]),"".concat(t[0],".").concat(t[2]),"".concat(t[0],".").concat(t[3]),"".concat(t[1],".").concat(t[0]),"".concat(t[1],".").concat(t[2]),"".concat(t[1],".").concat(t[3]),"".concat(t[2],".").concat(t[0]),"".concat(t[2],".").concat(t[1]),"".concat(t[2],".").concat(t[3]),"".concat(t[3],".").concat(t[0]),"".concat(t[3],".").concat(t[1]),"".concat(t[3],".").concat(t[2]),"".concat(t[0],".").concat(t[1],".").concat(t[2]),"".concat(t[0],".").concat(t[1],".").concat(t[3]),"".concat(t[0],".").concat(t[2],".").concat(t[1]),"".concat(t[0],".").concat(t[2],".").concat(t[3]),"".concat(t[0],".").concat(t[3],".").concat(t[1]),"".concat(t[0],".").concat(t[3],".").concat(t[2]),"".concat(t[1],".").concat(t[0],".").concat(t[2]),"".concat(t[1],".").concat(t[0],".").concat(t[3]),"".concat(t[1],".").concat(t[2],".").concat(t[0]),"".concat(t[1],".").concat(t[2],".").concat(t[3]),"".concat(t[1],".").concat(t[3],".").concat(t[0]),"".concat(t[1],".").concat(t[3],".").concat(t[2]),"".concat(t[2],".").concat(t[0],".").concat(t[1]),"".concat(t[2],".").concat(t[0],".").concat(t[3]),"".concat(t[2],".").concat(t[1],".").concat(t[0]),"".concat(t[2],".").concat(t[1],".").concat(t[3]),"".concat(t[2],".").concat(t[3],".").concat(t[0]),"".concat(t[2],".").concat(t[3],".").concat(t[1]),"".concat(t[3],".").concat(t[0],".").concat(t[1]),"".concat(t[3],".").concat(t[0],".").concat(t[2]),"".concat(t[3],".").concat(t[1],".").concat(t[0]),"".concat(t[3],".").concat(t[1],".").concat(t[2]),"".concat(t[3],".").concat(t[2],".").concat(t[0]),"".concat(t[3],".").concat(t[2],".").concat(t[1]),"".concat(t[0],".").concat(t[1],".").concat(t[2],".").concat(t[3]),"".concat(t[0],".").concat(t[1],".").concat(t[3],".").concat(t[2]),"".concat(t[0],".").concat(t[2],".").concat(t[1],".").concat(t[3]),"".concat(t[0],".").concat(t[2],".").concat(t[3],".").concat(t[1]),"".concat(t[0],".").concat(t[3],".").concat(t[1],".").concat(t[2]),"".concat(t[0],".").concat(t[3],".").concat(t[2],".").concat(t[1]),"".concat(t[1],".").concat(t[0],".").concat(t[2],".").concat(t[3]),"".concat(t[1],".").concat(t[0],".").concat(t[3],".").concat(t[2]),"".concat(t[1],".").concat(t[2],".").concat(t[0],".").concat(t[3]),"".concat(t[1],".").concat(t[2],".").concat(t[3],".").concat(t[0]),"".concat(t[1],".").concat(t[3],".").concat(t[0],".").concat(t[2]),"".concat(t[1],".").concat(t[3],".").concat(t[2],".").concat(t[0]),"".concat(t[2],".").concat(t[0],".").concat(t[1],".").concat(t[3]),"".concat(t[2],".").concat(t[0],".").concat(t[3],".").concat(t[1]),"".concat(t[2],".").concat(t[1],".").concat(t[0],".").concat(t[3]),"".concat(t[2],".").concat(t[1],".").concat(t[3],".").concat(t[0]),"".concat(t[2],".").concat(t[3],".").concat(t[0],".").concat(t[1]),"".concat(t[2],".").concat(t[3],".").concat(t[1],".").concat(t[0]),"".concat(t[3],".").concat(t[0],".").concat(t[1],".").concat(t[2]),"".concat(t[3],".").concat(t[0],".").concat(t[2],".").concat(t[1]),"".concat(t[3],".").concat(t[1],".").concat(t[0],".").concat(t[2]),"".concat(t[3],".").concat(t[1],".").concat(t[2],".").concat(t[0]),"".concat(t[3],".").concat(t[2],".").concat(t[0],".").concat(t[1]),"".concat(t[3],".").concat(t[2],".").concat(t[1],".").concat(t[0])]:void 0),Xo[r]}function Ko(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=arguments.length>2?arguments[2]:void 0,r=e.filter(function(e){return"token"!==e}),a=qo(r);return a.reduce(function(e,t){return $o($o({},e),n[t])},t)}function Jo(e){return e.join(" ")}function Qo(e){var t=e.node,n=e.stylesheet,r=e.style,a=void 0===r?{}:r,o=e.useInlineStyles,i=e.key,l=t.properties,s=t.tagName;if("text"===t.type)return t.value;if(s){var u,f=function(e,t){var n=0;return function(r){return n+=1,r.map(function(r,a){return Qo({node:r,stylesheet:e,useInlineStyles:t,key:"code-segment-".concat(n,"-").concat(a)})})}}(n,o);if(o){var d=Object.keys(n).reduce(function(e,t){return t.split(".").forEach(function(t){e.includes(t)||e.push(t)}),e},[]),p=l.className&&l.className.includes("token")?["token"]:[],m=l.className&&p.concat(l.className.filter(function(e){return!d.includes(e)}));u=$o($o({},l),{},{className:Jo(m)||void 0,style:Ko(l.className,Object.assign({},l.style,a),n)})}else u=$o($o({},l),{},{className:Jo(l.className)});var h=f(t.children);/*#__PURE__*/ return c.createElement(s,Go({key:i},u),h)}}var Zo=["language","children","style","customStyle","codeTagProps","useInlineStyles","showLineNumbers","showInlineLineNumbers","startingLineNumber","lineNumberContainerStyle","lineNumberStyle","wrapLines","wrapLongLines","lineProps","renderer","PreTag","CodeTag","code","astGenerator"];function ei(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function ti(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?ei(Object(n),!0).forEach(function(t){Wo(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):ei(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}var ni=/\n/g;function ri(e){var t=e.codeString,n=e.containerStyle,r=e.numberStyle,a=void 0===r?{}:r,o=e.startingLineNumber;/*#__PURE__*/ return c.createElement("code",{style:Object.assign({},e.codeStyle,void 0===n?{float:"left",paddingRight:"10px"}:n)},function(e){var t=e.startingLineNumber,n=e.style;return e.lines.map(function(e,r){var a=r+t;/*#__PURE__*/ return c.createElement("span",{key:"line-".concat(r),className:"react-syntax-highlighter-line-number",style:"function"==typeof n?n(a):n},"".concat(a,"\n"))})}({lines:t.replace(/\n$/,"").split("\n"),style:a,startingLineNumber:o}))}function ai(e,t){return{type:"element",tagName:"span",properties:{key:"line-number--".concat(e),className:["comment","linenumber","react-syntax-highlighter-line-number"],style:t},children:[{type:"text",value:e}]}}function oi(e,t,n){var r,a={display:"inline-block",minWidth:(r=n,"".concat(r.toString().length,".25em")),paddingRight:"1em",textAlign:"right",userSelect:"none"},o="function"==typeof e?e(t):e;return ti(ti({},a),o)}function ii(e){var t=e.children,n=e.lineNumber,r=e.lineNumberStyle,a=e.largestLineNumber,o=e.showInlineLineNumbers,i=e.lineProps,l=void 0===i?{}:i,s=e.className,c=void 0===s?[]:s,u=e.showLineNumbers,f=e.wrapLongLines,d="function"==typeof l?l(n):l;if(d.className=c,n&&o){var p=oi(r,n,a);t.unshift(ai(n,p))}return f&u&&(d.style=ti(ti({},d.style),{},{display:"flex"})),{type:"element",tagName:"span",properties:d,children:t}}function li(e){for(var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:[],n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:[],r=0;r<e.length;r++){var a=e[r];if("text"===a.type)n.push(ii({children:[a],className:Vo(new Set(t))}));else if(a.children){var o=t.concat(a.properties.className);li(a.children,o).forEach(function(e){return n.push(e)})}}return n}function si(e,t,n,r,a,o,i,l,s){var c,u=li(e.value),f=[],d=-1,p=0;function m(e,t){var o=arguments.length>2&&void 0!==arguments[2]?arguments[2]:[];return ii({children:e,lineNumber:t,lineNumberStyle:l,largestLineNumber:i,showInlineLineNumbers:a,lineProps:n,className:o,showLineNumbers:r,wrapLongLines:s})}function h(e,t){if(r&&t&&a){var n=oi(l,t,i);e.unshift(ai(t,n))}return e}function g(e,n){var r=arguments.length>2&&void 0!==arguments[2]?arguments[2]:[];return t||r.length>0?m(e,n,r):h(e,n)}for(var y=function(){var e=u[p],t=e.children[0].value;if(t.match(ni)){var n=t.split("\n");n.forEach(function(t,a){var i=r&&f.length+o,l={type:"text",value:"".concat(t,"\n")};if(0===a){var s=g(u.slice(d+1,p).concat(ii({children:[l],className:e.properties.className})),i);f.push(s)}else if(a===n.length-1){var c=u[p+1]&&u[p+1].children&&u[p+1].children[0],m={type:"text",value:"".concat(t)};if(c){var h=ii({children:[m],className:e.properties.className});u.splice(p+1,0,h)}else{var y=g([m],i,e.properties.className);f.push(y)}}else{var v=g([l],i,e.properties.className);f.push(v)}}),d=p}p++};p<u.length;)y();if(d!==u.length-1){var v=u.slice(d+1,u.length);if(v&&v.length){var b=g(v,r&&f.length+o);f.push(b)}}return t?f:(c=[]).concat.apply(c,f)}function ci(e){var t=e.stylesheet,n=e.useInlineStyles;return e.rows.map(function(e,r){return Qo({node:e,stylesheet:t,useInlineStyles:n,key:"code-segement".concat(r)})})}function ui(e){return e&&void 0!==e.highlightAuto}function fi(e){return e instanceof Map?e.clear=e.delete=e.set=function(){throw new Error("map is read-only")}:e instanceof Set&&(e.add=e.clear=e.delete=function(){throw new Error("set is read-only")}),Object.freeze(e),Object.getOwnPropertyNames(e).forEach(function(t){var n=e[t];"object"!=typeof n||Object.isFrozen(n)||fi(n)}),e}var di=fi;di.default=fi;class pi{constructor(e){void 0===e.data&&(e.data={}),this.data=e.data,this.isMatchIgnored=!1}ignoreMatch(){this.isMatchIgnored=!0}}function mi(e){return e.replace(/&/g,"&").replace(/</g,"<").replace(/>/g,">").replace(/"/g,""").replace(/'/g,"'")}function hi(e,...t){const n=Object.create(null);for(const t in e)n[t]=e[t];return t.forEach(function(e){for(const t in e)n[t]=e[t]}),n}const gi=e=>!!e.kind;class yi{constructor(e,t){this.buffer="",this.classPrefix=t.classPrefix,e.walk(this)}addText(e){this.buffer+=mi(e)}openNode(e){if(!gi(e))return;let t=e.kind;e.sublanguage||(t=`${this.classPrefix}${t}`),this.span(t)}closeNode(e){gi(e)&&(this.buffer+="</span>")}value(){return this.buffer}span(e){this.buffer+=`<span class="${e}">`}}class vi{constructor(){this.rootNode={children:[]},this.stack=[this.rootNode]}get top(){return this.stack[this.stack.length-1]}get root(){return this.rootNode}add(e){this.top.children.push(e)}openNode(e){const t={kind:e,children:[]};this.add(t),this.stack.push(t)}closeNode(){if(this.stack.length>1)return this.stack.pop()}closeAllNodes(){for(;this.closeNode(););}toJSON(){return JSON.stringify(this.rootNode,null,4)}walk(e){return this.constructor._walk(e,this.rootNode)}static _walk(e,t){return"string"==typeof t?e.addText(t):t.children&&(e.openNode(t),t.children.forEach(t=>this._walk(e,t)),e.closeNode(t)),e}static _collapse(e){"string"!=typeof e&&e.children&&(e.children.every(e=>"string"==typeof e)?e.children=[e.children.join("")]:e.children.forEach(e=>{vi._collapse(e)}))}}class bi extends vi{constructor(e){super(),this.options=e}addKeyword(e,t){""!==e&&(this.openNode(t),this.addText(e),this.closeNode())}addText(e){""!==e&&this.add(e)}addSublanguage(e,t){const n=e.root;n.kind=t,n.sublanguage=!0,this.add(n)}toHTML(){return new yi(this,this.options).value()}finalize(){return!0}}function Ei(e){return e?"string"==typeof e?e:e.source:null}const Ti=/\[(?:[^\\\]]|\\.)*\]|\(\??|\\([1-9][0-9]*)|\\./,Si="(-?)(\\b0[xX][a-fA-F0-9]+|(\\b\\d+(\\.\\d*)?|\\.\\d+)([eE][-+]?\\d+)?)",wi={begin:"\\\\[\\s\\S]",relevance:0},Ni={className:"string",begin:"'",end:"'",illegal:"\\n",contains:[wi]},Ri={className:"string",begin:'"',end:'"',illegal:"\\n",contains:[wi]},Oi={begin:/\b(a|an|the|are|I'm|isn't|don't|doesn't|won't|but|just|should|pretty|simply|enough|gonna|going|wtf|so|such|will|you|your|they|like|more)\b/},Ci=function(e,t,n={}){const r=hi({className:"comment",begin:e,end:t,contains:[]},n);return r.contains.push(Oi),r.contains.push({className:"doctag",begin:"(?:TODO|FIXME|NOTE|BUG|OPTIMIZE|HACK|XXX):",relevance:0}),r},xi=Ci("//","$"),ki=Ci("/\\*","\\*/"),Ai=Ci("#","$");var Ii=/*#__PURE__*/Object.freeze({__proto__:null,MATCH_NOTHING_RE:/\b\B/,IDENT_RE:"[a-zA-Z]\\w*",UNDERSCORE_IDENT_RE:"[a-zA-Z_]\\w*",NUMBER_RE:"\\b\\d+(\\.\\d+)?",C_NUMBER_RE:Si,BINARY_NUMBER_RE:"\\b(0b[01]+)",RE_STARTERS_RE:"!|!=|!==|%|%=|&|&&|&=|\\*|\\*=|\\+|\\+=|,|-|-=|/=|/|:|;|<<|<<=|<=|<|===|==|=|>>>=|>>=|>=|>>>|>>|>|\\?|\\[|\\{|\\(|\\^|\\^=|\\||\\|=|\\|\\||~",SHEBANG:(e={})=>{const t=/^#![ ]*\//;return e.binary&&(e.begin=function(...e){return e.map(e=>Ei(e)).join("")}(t,/.*\b/,e.binary,/\b.*/)),hi({className:"meta",begin:t,end:/$/,relevance:0,"on:begin":(e,t)=>{0!==e.index&&t.ignoreMatch()}},e)},BACKSLASH_ESCAPE:wi,APOS_STRING_MODE:Ni,QUOTE_STRING_MODE:Ri,PHRASAL_WORDS_MODE:Oi,COMMENT:Ci,C_LINE_COMMENT_MODE:xi,C_BLOCK_COMMENT_MODE:ki,HASH_COMMENT_MODE:Ai,NUMBER_MODE:{className:"number",begin:"\\b\\d+(\\.\\d+)?",relevance:0},C_NUMBER_MODE:{className:"number",begin:Si,relevance:0},BINARY_NUMBER_MODE:{className:"number",begin:"\\b(0b[01]+)",relevance:0},CSS_NUMBER_MODE:{className:"number",begin:"\\b\\d+(\\.\\d+)?(%|em|ex|ch|rem|vw|vh|vmin|vmax|cm|mm|in|pt|pc|px|deg|grad|rad|turn|s|ms|Hz|kHz|dpi|dpcm|dppx)?",relevance:0},REGEXP_MODE:{begin:/(?=\/[^/\n]*\/)/,contains:[{className:"regexp",begin:/\//,end:/\/[gimuy]*/,illegal:/\n/,contains:[wi,{begin:/\[/,end:/\]/,relevance:0,contains:[wi]}]}]},TITLE_MODE:{className:"title",begin:"[a-zA-Z]\\w*",relevance:0},UNDERSCORE_TITLE_MODE:{className:"title",begin:"[a-zA-Z_]\\w*",relevance:0},METHOD_GUARD:{begin:"\\.\\s*[a-zA-Z_]\\w*",relevance:0},END_SAME_AS_BEGIN:function(e){return Object.assign(e,{"on:begin":(e,t)=>{t.data._beginMatch=e[1]},"on:end":(e,t)=>{t.data._beginMatch!==e[1]&&t.ignoreMatch()}})}});function Li(e,t){"."===e.input[e.index-1]&&t.ignoreMatch()}function _i(e,t){t&&e.beginKeywords&&(e.begin="\\b("+e.beginKeywords.split(" ").join("|")+")(?!\\.)(?=\\b|\\s)",e.__beforeBegin=Li,e.keywords=e.keywords||e.beginKeywords,delete e.beginKeywords,void 0===e.relevance&&(e.relevance=0))}function Pi(e,t){Array.isArray(e.illegal)&&(e.illegal=function(...e){return"("+e.map(e=>Ei(e)).join("|")+")"}(...e.illegal))}function Mi(e,t){if(e.match){if(e.begin||e.end)throw new Error("begin & end are not supported with match");e.begin=e.match,delete e.match}}function Di(e,t){void 0===e.relevance&&(e.relevance=1)}const Ui=["of","and","for","in","not","or","if","then","parent","list","value"];function ji(e,t,n="keyword"){const r={};return"string"==typeof e?a(n,e.split(" ")):Array.isArray(e)?a(n,e):Object.keys(e).forEach(function(n){Object.assign(r,ji(e[n],t,n))}),r;function a(e,n){t&&(n=n.map(e=>e.toLowerCase())),n.forEach(function(t){const n=t.split("|");r[n[0]]=[e,Fi(n[0],n[1])]})}}function Fi(e,t){return t?Number(t):function(e){return Ui.includes(e.toLowerCase())}(e)?0:1}function zi(e,{}){function t(t,n){return new RegExp(Ei(t),"m"+(e.case_insensitive?"i":"")+(n?"g":""))}class n{constructor(){this.matchIndexes={},this.regexes=[],this.matchAt=1,this.position=0}addRule(e,t){t.position=this.position++,this.matchIndexes[this.matchAt]=t,this.regexes.push([t,e]),this.matchAt+=function(e){return new RegExp(e.toString()+"|").exec("").length-1}(e)+1}compile(){0===this.regexes.length&&(this.exec=()=>null);const e=this.regexes.map(e=>e[1]);this.matcherRe=t(function(e,t="|"){let n=0;return e.map(e=>{n+=1;const t=n;let r=Ei(e),a="";for(;r.length>0;){const e=Ti.exec(r);if(!e){a+=r;break}a+=r.substring(0,e.index),r=r.substring(e.index+e[0].length),"\\"===e[0][0]&&e[1]?a+="\\"+String(Number(e[1])+t):(a+=e[0],"("===e[0]&&n++)}return a}).map(e=>`(${e})`).join(t)}(e),!0),this.lastIndex=0}exec(e){this.matcherRe.lastIndex=this.lastIndex;const t=this.matcherRe.exec(e);if(!t)return null;const n=t.findIndex((e,t)=>t>0&&void 0!==e),r=this.matchIndexes[n];return t.splice(0,n),Object.assign(t,r)}}class r{constructor(){this.rules=[],this.multiRegexes=[],this.count=0,this.lastIndex=0,this.regexIndex=0}getMatcher(e){if(this.multiRegexes[e])return this.multiRegexes[e];const t=new n;return this.rules.slice(e).forEach(([e,n])=>t.addRule(e,n)),t.compile(),this.multiRegexes[e]=t,t}resumingScanAtSamePosition(){return 0!==this.regexIndex}considerAll(){this.regexIndex=0}addRule(e,t){this.rules.push([e,t]),"begin"===t.type&&this.count++}exec(e){const t=this.getMatcher(this.regexIndex);t.lastIndex=this.lastIndex;let n=t.exec(e);if(this.resumingScanAtSamePosition())if(n&&n.index===this.lastIndex);else{const t=this.getMatcher(0);t.lastIndex=this.lastIndex+1,n=t.exec(e)}return n&&(this.regexIndex+=n.position+1,this.regexIndex===this.count&&this.considerAll()),n}}if(e.compilerExtensions||(e.compilerExtensions=[]),e.contains&&e.contains.includes("self"))throw new Error("ERR: contains `self` is not supported at the top-level of a language. See documentation.");return e.classNameAliases=hi(e.classNameAliases||{}),function n(a,o){const i=a;if(a.isCompiled)return i;[Mi].forEach(e=>e(a,o)),e.compilerExtensions.forEach(e=>e(a,o)),a.__beforeBegin=null,[_i,Pi,Di].forEach(e=>e(a,o)),a.isCompiled=!0;let l=null;if("object"==typeof a.keywords&&(l=a.keywords.$pattern,delete a.keywords.$pattern),a.keywords&&(a.keywords=ji(a.keywords,e.case_insensitive)),a.lexemes&&l)throw new Error("ERR: Prefer `keywords.$pattern` to `mode.lexemes`, BOTH are not allowed. (see mode reference) ");return l=l||a.lexemes||/\w+/,i.keywordPatternRe=t(l,!0),o&&(a.begin||(a.begin=/\B|\b/),i.beginRe=t(a.begin),a.endSameAsBegin&&(a.end=a.begin),a.end||a.endsWithParent||(a.end=/\B|\b/),a.end&&(i.endRe=t(a.end)),i.terminatorEnd=Ei(a.end)||"",a.endsWithParent&&o.terminatorEnd&&(i.terminatorEnd+=(a.end?"|":"")+o.terminatorEnd)),a.illegal&&(i.illegalRe=t(a.illegal)),a.contains||(a.contains=[]),a.contains=[].concat(...a.contains.map(function(e){return function(e){return e.variants&&!e.cachedVariants&&(e.cachedVariants=e.variants.map(function(t){return hi(e,{variants:null},t)})),e.cachedVariants?e.cachedVariants:Bi(e)?hi(e,{starts:e.starts?hi(e.starts):null}):Object.isFrozen(e)?hi(e):e}("self"===e?a:e)})),a.contains.forEach(function(e){n(e,i)}),a.starts&&n(a.starts,o),i.matcher=function(e){const t=new r;return e.contains.forEach(e=>t.addRule(e.begin,{rule:e,type:"begin"})),e.terminatorEnd&&t.addRule(e.terminatorEnd,{type:"end"}),e.illegal&&t.addRule(e.illegal,{type:"illegal"}),t}(i),i}(e)}function Bi(e){return!!e&&(e.endsWithParent||Bi(e.starts))}function Hi(e){const t={props:["language","code","autodetect"],data:function(){return{detectedLanguage:"",unknownLanguage:!1}},computed:{className(){return this.unknownLanguage?"":"hljs "+this.detectedLanguage},highlighted(){if(!this.autoDetect&&!e.getLanguage(this.language))return console.warn(`The language "${this.language}" you specified could not be found.`),this.unknownLanguage=!0,mi(this.code);let t={};return this.autoDetect?(t=e.highlightAuto(this.code),this.detectedLanguage=t.language):(t=e.highlight(this.language,this.code,this.ignoreIllegals),this.detectedLanguage=this.language),t.value},autoDetect(){return!this.language||(e=this.autodetect,Boolean(e||""===e));var e},ignoreIllegals:()=>!0},render(e){return e("pre",{},[e("code",{class:this.className,domProps:{innerHTML:this.highlighted}})])}};return{Component:t,VuePlugin:{install(e){e.component("highlightjs",t)}}}}const Vi={"after:highlightElement":({el:e,result:t,text:n})=>{const r=Gi(e);if(!r.length)return;const a=document.createElement("div");a.innerHTML=t.value,t.value=function(e,t,n){let r=0,a="";const o=[];function i(){return e.length&&t.length?e[0].offset!==t[0].offset?e[0].offset<t[0].offset?e:t:"start"===t[0].event?e:t:e.length?e:t}function l(e){a+="<"+Wi(e)+[].map.call(e.attributes,function(e){return" "+e.nodeName+'="'+mi(e.value)+'"'}).join("")+">"}function s(e){a+="</"+Wi(e)+">"}function c(e){("start"===e.event?l:s)(e.node)}for(;e.length||t.length;){let t=i();if(a+=mi(n.substring(r,t[0].offset)),r=t[0].offset,t===e){o.reverse().forEach(s);do{c(t.splice(0,1)[0]),t=i()}while(t===e&&t.length&&t[0].offset===r);o.reverse().forEach(l)}else"start"===t[0].event?o.push(t[0].node):o.pop(),c(t.splice(0,1)[0])}return a+mi(n.substr(r))}(r,Gi(a),n)}};function Wi(e){return e.nodeName.toLowerCase()}function Gi(e){const t=[];return function e(n,r){for(let a=n.firstChild;a;a=a.nextSibling)3===a.nodeType?r+=a.nodeValue.length:1===a.nodeType&&(t.push({event:"start",offset:r,node:a}),r=e(a,r),Wi(a).match(/br|hr|img|input/)||t.push({event:"stop",offset:r,node:a}));return r}(e,0),t}const Yi={},$i=e=>{console.error(e)},Xi=(e,...t)=>{console.log(`WARN: ${e}`,...t)},qi=(e,t)=>{Yi[`${e}/${t}`]||(console.log(`Deprecated as of ${e}. ${t}`),Yi[`${e}/${t}`]=!0)},Ki=mi,Ji=hi,Qi=Symbol("nomatch");var Zi=function(e){const t=Object.create(null),n=Object.create(null),r=[];let a=!0;const o=/(^(<[^>]+>|\t|)+|\n)/gm,i="Could not find the language '{}', did you forget to load/include a language module?",l={disableAutodetect:!0,name:"Plain text",contains:[]};let s={noHighlightRe:/^(no-?highlight)$/i,languageDetectRe:/\blang(?:uage)?-([\w-]+)\b/i,classPrefix:"hljs-",tabReplace:null,useBR:!1,languages:null,__emitter:bi};function c(e){return s.noHighlightRe.test(e)}function u(e,t,n,r){let a="",o="";"object"==typeof t?(a=e,n=t.ignoreIllegals,o=t.language,r=void 0):(qi("10.7.0","highlight(lang, code, ...args) has been deprecated."),qi("10.7.0","Please use highlight(code, options) instead.\nhttps://github.com/highlightjs/highlight.js/issues/2277"),o=e,a=t);const i={code:a,language:o};w("before:highlight",i);const l=i.result?i.result:f(i.language,i.code,n,r);return l.code=i.code,w("after:highlight",l),l}function f(e,n,o,l){function c(e,t){const n=b.case_insensitive?t[0].toLowerCase():t[0];return Object.prototype.hasOwnProperty.call(e.keywords,n)&&e.keywords[n]}function u(){null!=w.subLanguage?function(){if(""===O)return;let e=null;if("string"==typeof w.subLanguage){if(!t[w.subLanguage])return void R.addText(O);e=f(w.subLanguage,O,!0,N[w.subLanguage]),N[w.subLanguage]=e.top}else e=d(O,w.subLanguage.length?w.subLanguage:null);w.relevance>0&&(C+=e.relevance),R.addSublanguage(e.emitter,e.language)}():function(){if(!w.keywords)return void R.addText(O);let e=0;w.keywordPatternRe.lastIndex=0;let t=w.keywordPatternRe.exec(O),n="";for(;t;){n+=O.substring(e,t.index);const r=c(w,t);if(r){const[e,a]=r;R.addText(n),n="",C+=a,e.startsWith("_")?n+=t[0]:R.addKeyword(t[0],b.classNameAliases[e]||e)}else n+=t[0];e=w.keywordPatternRe.lastIndex,t=w.keywordPatternRe.exec(O)}n+=O.substr(e),R.addText(n)}(),O=""}function p(e){return e.className&&R.openNode(b.classNameAliases[e.className]||e.className),w=Object.create(e,{parent:{value:w}}),w}function m(e,t,n){let r=function(e,t){const n=e&&e.exec(t);return n&&0===n.index}(e.endRe,n);if(r){if(e["on:end"]){const n=new pi(e);e["on:end"](t,n),n.isMatchIgnored&&(r=!1)}if(r){for(;e.endsParent&&e.parent;)e=e.parent;return e}}if(e.endsWithParent)return m(e.parent,t,n)}function h(e){return 0===w.matcher.regexIndex?(O+=e[0],1):(A=!0,0)}function g(e){const t=e[0],r=n.substr(e.index),a=m(w,e,r);if(!a)return Qi;const o=w;o.skip?O+=t:(o.returnEnd||o.excludeEnd||(O+=t),u(),o.excludeEnd&&(O=t));do{w.className&&R.closeNode(),w.skip||w.subLanguage||(C+=w.relevance),w=w.parent}while(w!==a.parent);return a.starts&&(a.endSameAsBegin&&(a.starts.endRe=a.endRe),p(a.starts)),o.returnEnd?0:t.length}let y={};function v(t,r){const i=r&&r[0];if(O+=t,null==i)return u(),0;if("begin"===y.type&&"end"===r.type&&y.index===r.index&&""===i){if(O+=n.slice(r.index,r.index+1),!a){const t=new Error("0 width match regex");throw t.languageName=e,t.badRule=y.rule,t}return 1}if(y=r,"begin"===r.type)return function(e){const t=e[0],n=e.rule,r=new pi(n),a=[n.__beforeBegin,n["on:begin"]];for(const n of a)if(n&&(n(e,r),r.isMatchIgnored))return h(t);return n&&n.endSameAsBegin&&(n.endRe=new RegExp(t.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&"),"m")),n.skip?O+=t:(n.excludeBegin&&(O+=t),u(),n.returnBegin||n.excludeBegin||(O=t)),p(n),n.returnBegin?0:t.length}(r);if("illegal"===r.type&&!o){const e=new Error('Illegal lexeme "'+i+'" for mode "'+(w.className||"<unnamed>")+'"');throw e.mode=w,e}if("end"===r.type){const e=g(r);if(e!==Qi)return e}if("illegal"===r.type&&""===i)return 1;if(k>1e5&&k>3*r.index)throw new Error("potential infinite loop, way more iterations than matches");return O+=i,i.length}const b=E(e);if(!b)throw $i(i.replace("{}",e)),new Error('Unknown language: "'+e+'"');const T=zi(b,{plugins:r});let S="",w=l||T;const N={},R=new s.__emitter(s);!function(){const e=[];for(let t=w;t!==b;t=t.parent)t.className&&e.unshift(t.className);e.forEach(e=>R.openNode(e))}();let O="",C=0,x=0,k=0,A=!1;try{for(w.matcher.considerAll();;){k++,A?A=!1:w.matcher.considerAll(),w.matcher.lastIndex=x;const e=w.matcher.exec(n);if(!e)break;const t=v(n.substring(x,e.index),e);x=e.index+t}return v(n.substr(x)),R.closeAllNodes(),R.finalize(),S=R.toHTML(),{relevance:Math.floor(C),value:S,language:e,illegal:!1,emitter:R,top:w}}catch(t){if(t.message&&t.message.includes("Illegal"))return{illegal:!0,illegalBy:{msg:t.message,context:n.slice(x-100,x+100),mode:t.mode},sofar:S,relevance:0,value:Ki(n),emitter:R};if(a)return{illegal:!1,relevance:0,value:Ki(n),emitter:R,language:e,top:w,errorRaised:t};throw t}}function d(e,n){n=n||s.languages||Object.keys(t);const r=function(e){const t={relevance:0,emitter:new s.__emitter(s),value:Ki(e),illegal:!1,top:l};return t.emitter.addText(e),t}(e),a=n.filter(E).filter(S).map(t=>f(t,e,!1));a.unshift(r);const o=a.sort((e,t)=>{if(e.relevance!==t.relevance)return t.relevance-e.relevance;if(e.language&&t.language){if(E(e.language).supersetOf===t.language)return 1;if(E(t.language).supersetOf===e.language)return-1}return 0}),[i,c]=o,u=i;return u.second_best=c,u}const p={"before:highlightElement":({el:e})=>{s.useBR&&(e.innerHTML=e.innerHTML.replace(/\n/g,"").replace(/<br[ /]*>/g,"\n"))},"after:highlightElement":({result:e})=>{s.useBR&&(e.value=e.value.replace(/\n/g,"<br>"))}},m=/^(<[^>]+>|\t)+/gm,h={"after:highlightElement":({result:e})=>{s.tabReplace&&(e.value=e.value.replace(m,e=>e.replace(/\t/g,s.tabReplace)))}};function g(e){let t=null;const r=function(e){let t=e.className+" ";t+=e.parentNode?e.parentNode.className:"";const n=s.languageDetectRe.exec(t);if(n){const t=E(n[1]);return t||(Xi(i.replace("{}",n[1])),Xi("Falling back to no-highlight mode for this block.",e)),t?n[1]:"no-highlight"}return t.split(/\s+/).find(e=>c(e)||E(e))}(e);if(c(r))return;w("before:highlightElement",{el:e,language:r}),t=e;const a=t.textContent,o=r?u(a,{language:r,ignoreIllegals:!0}):d(a);w("after:highlightElement",{el:e,result:o,text:a}),e.innerHTML=o.value,function(e,t,r){const a=t?n[t]:r;e.classList.add("hljs"),a&&e.classList.add(a)}(e,r,o.language),e.result={language:o.language,re:o.relevance,relavance:o.relevance},o.second_best&&(e.second_best={language:o.second_best.language,re:o.second_best.relevance,relavance:o.second_best.relevance})}const y=()=>{y.called||(y.called=!0,qi("10.6.0","initHighlighting() is deprecated. Use highlightAll() instead."),document.querySelectorAll("pre code").forEach(g))};let v=!1;function b(){"loading"!==document.readyState?document.querySelectorAll("pre code").forEach(g):v=!0}function E(e){return e=(e||"").toLowerCase(),t[e]||t[n[e]]}function T(e,{languageName:t}){"string"==typeof e&&(e=[e]),e.forEach(e=>{n[e.toLowerCase()]=t})}function S(e){const t=E(e);return t&&!t.disableAutodetect}function w(e,t){const n=e;r.forEach(function(e){e[n]&&e[n](t)})}"undefined"!=typeof window&&window.addEventListener&&window.addEventListener("DOMContentLoaded",function(){v&&b()},!1),Object.assign(e,{highlight:u,highlightAuto:d,highlightAll:b,fixMarkup:function(e){return qi("10.2.0","fixMarkup will be removed entirely in v11.0"),qi("10.2.0","Please see https://github.com/highlightjs/highlight.js/issues/2534"),t=e,s.tabReplace||s.useBR?t.replace(o,e=>"\n"===e?s.useBR?"<br>":e:s.tabReplace?e.replace(/\t/g,s.tabReplace):e):t;var t},highlightElement:g,highlightBlock:function(e){return qi("10.7.0","highlightBlock will be removed entirely in v12.0"),qi("10.7.0","Please use highlightElement now."),g(e)},configure:function(e){e.useBR&&(qi("10.3.0","'useBR' will be removed entirely in v11.0"),qi("10.3.0","Please see https://github.com/highlightjs/highlight.js/issues/2559")),s=Ji(s,e)},initHighlighting:y,initHighlightingOnLoad:function(){qi("10.6.0","initHighlightingOnLoad() is deprecated. Use highlightAll() instead."),v=!0},registerLanguage:function(n,r){let o=null;try{o=r(e)}catch(e){if($i("Language definition for '{}' could not be registered.".replace("{}",n)),!a)throw e;$i(e),o=l}o.name||(o.name=n),t[n]=o,o.rawDefinition=r.bind(null,e),o.aliases&&T(o.aliases,{languageName:n})},unregisterLanguage:function(e){delete t[e];for(const t of Object.keys(n))n[t]===e&&delete n[t]},listLanguages:function(){return Object.keys(t)},getLanguage:E,registerAliases:T,requireLanguage:function(e){qi("10.4.0","requireLanguage will be removed entirely in v11."),qi("10.4.0","Please see https://github.com/highlightjs/highlight.js/pull/2844");const t=E(e);if(t)return t;throw new Error("The '{}' language is required, but not loaded.".replace("{}",e))},autoDetection:S,inherit:Ji,addPlugin:function(e){!function(e){e["before:highlightBlock"]&&!e["before:highlightElement"]&&(e["before:highlightElement"]=t=>{e["before:highlightBlock"](Object.assign({block:t.el},t))}),e["after:highlightBlock"]&&!e["after:highlightElement"]&&(e["after:highlightElement"]=t=>{e["after:highlightBlock"](Object.assign({block:t.el},t))})}(e),r.push(e)},vuePlugin:Hi(e).VuePlugin}),e.debugMode=function(){a=!1},e.safeMode=function(){a=!0},e.versionString="10.7.3";for(const e in Ii)"object"==typeof Ii[e]&&di(Ii[e]);return Object.assign(e,Ii),e.addPlugin(p),e.addPlugin(Vi),e.addPlugin(h),e}({}),el=Le(function(e){!function(){var t;function n(e){for(var t,n,r,a,o=1,i=[].slice.call(arguments),l=0,s=e.length,c="",u=!1,f=!1,d=function(){return i[o++]},p=function(){for(var n="";/\d/.test(e[l]);)n+=e[l++],t=e[l];return n.length>0?parseInt(n):null};l<s;++l)if(t=e[l],u)switch(u=!1,"."==t?(f=!1,t=e[++l]):"0"==t&&"."==e[l+1]?(f=!0,t=e[l+=2]):f=!0,a=p(),t){case"b":c+=parseInt(d(),10).toString(2);break;case"c":c+="string"==typeof(n=d())||n instanceof String?n:String.fromCharCode(parseInt(n,10));break;case"d":c+=parseInt(d(),10);break;case"f":r=String(parseFloat(d()).toFixed(a||6)),c+=f?r:r.replace(/^0/,"");break;case"j":c+=JSON.stringify(d());break;case"o":c+="0"+parseInt(d(),10).toString(8);break;case"s":c+=d();break;case"x":c+="0x"+parseInt(d(),10).toString(16);break;case"X":c+="0x"+parseInt(d(),10).toString(16).toUpperCase();break;default:c+=t}else"%"===t?u=!0:c+=t;return c}(t=e.exports=n).format=n,t.vsprintf=function(e,t){return n.apply(null,[e].concat(t))},"undefined"!=typeof console&&"function"==typeof console.log&&(t.printf=function(){console.log(n.apply(null,arguments))})}()}),tl=rl(Error),nl=tl;function rl(e){return t.displayName=e.displayName||e.name,t;function t(t){return t&&(t=el.apply(null,arguments)),new e(t)}}tl.eval=rl(EvalError),tl.range=rl(RangeError),tl.reference=rl(ReferenceError),tl.syntax=rl(SyntaxError),tl.type=rl(TypeError),tl.uri=rl(URIError),tl.create=rl;var al=ol;function ol(e,t,n){var r,a=Zi.configure({}),o=(n||{}).prefix;if("string"!=typeof e)throw nl("Expected `string` for name, got `%s`",e);if(!Zi.getLanguage(e))throw nl("Unknown language: `%s` is not registered",e);if("string"!=typeof t)throw nl("Expected `string` for value, got `%s`",t);if(null==o&&(o="hljs-"),Zi.configure({__emitter:il,classPrefix:o}),r=Zi.highlight(t,{language:e,ignoreIllegals:!0}),Zi.configure(a||{}),r.errorRaised)throw r.errorRaised;return{relevance:r.relevance,language:r.language,value:r.emitter.rootNode.children}}function il(e){this.options=e,this.rootNode={children:[]},this.stack=[this.rootNode]}function ll(){}il.prototype.addText=function(e){var t,n,r=this.stack;""!==e&&((n=(t=r[r.length-1]).children[t.children.length-1])&&"text"===n.type?n.value+=e:t.children.push({type:"text",value:e}))},il.prototype.addKeyword=function(e,t){this.openNode(t),this.addText(e),this.closeNode()},il.prototype.addSublanguage=function(e,t){var n=this.stack,r=n[n.length-1],a=e.rootNode.children;r.children=r.children.concat(t?{type:"element",tagName:"span",properties:{className:[t]},children:a}:a)},il.prototype.openNode=function(e){var t=this.stack,n={type:"element",tagName:"span",properties:{className:[this.options.classPrefix+e]},children:[]};t[t.length-1].children.push(n),t.push(n)},il.prototype.closeNode=function(){this.stack.pop()},il.prototype.closeAllNodes=ll,il.prototype.finalize=ll,il.prototype.toHTML=function(){return""};var sl={highlight:al,highlightAuto:function(e,t){var n,r,a,o,i=(t||{}).subset||Zi.listLanguages(),l=i.length,s=-1;if("string"!=typeof e)throw nl("Expected `string` for value, got `%s`",e);for(r={relevance:0,language:null,value:[]},n={relevance:0,language:null,value:[]};++s<l;)Zi.getLanguage(o=i[s])&&((a=ol(o,e,t)).language=o,a.relevance>r.relevance&&(r=a),a.relevance>n.relevance&&(r=n,n=a));return r.language&&(n.secondBest=r),n},registerLanguage:function(e,t){Zi.registerLanguage(e,t)},listLanguages:function(){return Zi.listLanguages()},registerAlias:function(e,t){var n,r=e;for(n in t&&((r={})[e]=t),r)Zi.registerAliases(r[n],{languageName:n})}};function cl(e){return e?"string"==typeof e?e:e.source:null}function ul(e){return fl("(?=",e,")")}function fl(...e){return e.map(e=>cl(e)).join("")}function dl(...e){return"("+e.map(e=>cl(e)).join("|")+")"}const pl=["a","abbr","address","article","aside","audio","b","blockquote","body","button","canvas","caption","cite","code","dd","del","details","dfn","div","dl","dt","em","fieldset","figcaption","figure","footer","form","h1","h2","h3","h4","h5","h6","header","hgroup","html","i","iframe","img","input","ins","kbd","label","legend","li","main","mark","menu","nav","object","ol","p","q","quote","samp","section","span","strong","summary","sup","table","tbody","td","textarea","tfoot","th","thead","time","tr","ul","var","video"],ml=["any-hover","any-pointer","aspect-ratio","color","color-gamut","color-index","device-aspect-ratio","device-height","device-width","display-mode","forced-colors","grid","height","hover","inverted-colors","monochrome","orientation","overflow-block","overflow-inline","pointer","prefers-color-scheme","prefers-contrast","prefers-reduced-motion","prefers-reduced-transparency","resolution","scan","scripting","update","width","min-width","max-width","min-height","max-height"],hl=["active","any-link","blank","checked","current","default","defined","dir","disabled","drop","empty","enabled","first","first-child","first-of-type","fullscreen","future","focus","focus-visible","focus-within","has","host","host-context","hover","indeterminate","in-range","invalid","is","lang","last-child","last-of-type","left","link","local-link","not","nth-child","nth-col","nth-last-child","nth-last-col","nth-last-of-type","nth-of-type","only-child","only-of-type","optional","out-of-range","past","placeholder-shown","read-only","read-write","required","right","root","scope","target","target-within","user-invalid","valid","visited","where"],gl=["after","backdrop","before","cue","cue-region","first-letter","first-line","grammar-error","marker","part","placeholder","selection","slotted","spelling-error"],yl=["align-content","align-items","align-self","animation","animation-delay","animation-direction","animation-duration","animation-fill-mode","animation-iteration-count","animation-name","animation-play-state","animation-timing-function","auto","backface-visibility","background","background-attachment","background-clip","background-color","background-image","background-origin","background-position","background-repeat","background-size","border","border-bottom","border-bottom-color","border-bottom-left-radius","border-bottom-right-radius","border-bottom-style","border-bottom-width","border-collapse","border-color","border-image","border-image-outset","border-image-repeat","border-image-slice","border-image-source","border-image-width","border-left","border-left-color","border-left-style","border-left-width","border-radius","border-right","border-right-color","border-right-style","border-right-width","border-spacing","border-style","border-top","border-top-color","border-top-left-radius","border-top-right-radius","border-top-style","border-top-width","border-width","bottom","box-decoration-break","box-shadow","box-sizing","break-after","break-before","break-inside","caption-side","clear","clip","clip-path","color","column-count","column-fill","column-gap","column-rule","column-rule-color","column-rule-style","column-rule-width","column-span","column-width","columns","content","counter-increment","counter-reset","cursor","direction","display","empty-cells","filter","flex","flex-basis","flex-direction","flex-flow","flex-grow","flex-shrink","flex-wrap","float","font","font-display","font-family","font-feature-settings","font-kerning","font-language-override","font-size","font-size-adjust","font-smoothing","font-stretch","font-style","font-variant","font-variant-ligatures","font-variation-settings","font-weight","height","hyphens","icon","image-orientation","image-rendering","image-resolution","ime-mode","inherit","initial","justify-content","left","letter-spacing","line-height","list-style","list-style-image","list-style-position","list-style-type","margin","margin-bottom","margin-left","margin-right","margin-top","marks","mask","max-height","max-width","min-height","min-width","nav-down","nav-index","nav-left","nav-right","nav-up","none","normal","object-fit","object-position","opacity","order","orphans","outline","outline-color","outline-offset","outline-style","outline-width","overflow","overflow-wrap","overflow-x","overflow-y","padding","padding-bottom","padding-left","padding-right","padding-top","page-break-after","page-break-before","page-break-inside","perspective","perspective-origin","pointer-events","position","quotes","resize","right","src","tab-size","table-layout","text-align","text-align-last","text-decoration","text-decoration-color","text-decoration-line","text-decoration-style","text-indent","text-overflow","text-rendering","text-shadow","text-transform","text-underline-position","top","transform","transform-origin","transform-style","transition","transition-delay","transition-duration","transition-property","transition-timing-function","unicode-bidi","vertical-align","visibility","white-space","widows","width","word-break","word-spacing","word-wrap","z-index"].reverse();function vl(e){return e?"string"==typeof e?e:e.source:null}function bl(...e){return e.map(e=>vl(e)).join("")}const El=["as","in","of","if","for","while","finally","var","new","function","do","return","void","else","break","catch","instanceof","with","throw","case","default","try","switch","continue","typeof","delete","let","yield","const","class","debugger","async","await","static","import","from","export","extends"],Tl=["true","false","null","undefined","NaN","Infinity"],Sl=[].concat(["setInterval","setTimeout","clearInterval","clearTimeout","require","exports","eval","isFinite","isNaN","parseFloat","parseInt","decodeURI","decodeURIComponent","encodeURI","encodeURIComponent","escape","unescape"],["arguments","this","super","console","window","document","localStorage","module","global"],["Intl","DataView","Number","Math","Date","String","RegExp","Object","Function","Boolean","Error","Symbol","Set","Map","WeakSet","WeakMap","Proxy","Reflect","JSON","Promise","Float64Array","Int16Array","Int32Array","Int8Array","Uint16Array","Uint32Array","Float32Array","Array","Uint8Array","Uint8ClampedArray","ArrayBuffer","BigInt64Array","BigUint64Array","BigInt"],["EvalError","InternalError","RangeError","ReferenceError","SyntaxError","TypeError","URIError"]);function wl(e){return Nl("(?=",e,")")}function Nl(...e){return e.map(e=>{return(t=e)?"string"==typeof t?t:t.source:null;var t}).join("")}function Rl(e){return e?"string"==typeof e?e:e.source:null}function Ol(...e){return e.map(e=>Rl(e)).join("")}function Cl(...e){return"("+e.map(e=>Rl(e)).join("|")+")"}var xl,kl,Al=(xl=sl,kl={},function(e){var t=e.language,n=e.children,r=e.style,a=void 0===r?kl:r,o=e.customStyle,i=void 0===o?{}:o,l=e.codeTagProps,s=void 0===l?{className:t?"language-".concat(t):void 0,style:ti(ti({},a['code[class*="language-"]']),a['code[class*="language-'.concat(t,'"]')])}:l,u=e.useInlineStyles,f=void 0===u||u,d=e.showLineNumbers,p=void 0!==d&&d,m=e.showInlineLineNumbers,h=void 0===m||m,g=e.startingLineNumber,y=void 0===g?1:g,v=e.lineNumberContainerStyle,b=e.lineNumberStyle,E=void 0===b?{}:b,T=e.wrapLines,S=e.wrapLongLines,w=void 0!==S&&S,N=e.lineProps,R=void 0===N?{}:N,O=e.renderer,C=e.PreTag,x=void 0===C?"pre":C,k=e.CodeTag,A=void 0===k?"code":k,I=e.code,L=void 0===I?(Array.isArray(n)?n[0]:n)||"":I,_=e.astGenerator,P=function(e,t){if(null==e)return{};var n,r,a=function(e,t){if(null==e)return{};var n,r,a={},o=Object.keys(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||(a[n]=e[n]);return a}(e,t);if(Object.getOwnPropertySymbols){var o=Object.getOwnPropertySymbols(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||Object.prototype.propertyIsEnumerable.call(e,n)&&(a[n]=e[n])}return a}(e,Zo);_=_||xl;var M=p?/*#__PURE__*/c.createElement(ri,{containerStyle:v,codeStyle:s.style||{},numberStyle:E,startingLineNumber:y,codeString:L}):null,D=a.hljs||a['pre[class*="language-"]']||{backgroundColor:"#fff"},U=ui(_)?"hljs":"prismjs",j=Object.assign({},P,f?{style:Object.assign({},D,i)}:{className:P.className?"".concat(U," ").concat(P.className):U,style:Object.assign({},i)});if(s.style=ti(ti({},s.style),{},w?{whiteSpace:"pre-wrap"}:{whiteSpace:"pre"}),!_)/*#__PURE__*/ return c.createElement(x,j,M,/*#__PURE__*/c.createElement(A,s,L));(void 0===T&&O||w)&&(T=!0),O=O||ci;var F=[{type:"text",value:L}],z=function(e){var t=e.astGenerator,n=e.language,r=e.code,a=e.defaultCodeValue;if(ui(t)){var o=function(e,t){return-1!==e.listLanguages().indexOf(t)}(t,n);return"text"===n?{value:a,language:"text"}:o?t.highlight(n,r):t.highlightAuto(r)}try{return n&&"text"!==n?{value:t.highlight(r,n)}:{value:a}}catch(e){return{value:a}}}({astGenerator:_,language:t,code:L,defaultCodeValue:F});null===z.language&&(z.value=F);var B=si(z,T,R,p,h,y,z.value.length+y,E,w);/*#__PURE__*/ return c.createElement(x,j,/*#__PURE__*/c.createElement(A,s,!h&&M,O({rows:B,stylesheet:a,useInlineStyles:f})))});Al.registerLanguage=sl.registerLanguage;var Il=Al;function Ll({highlight:e,row:t,frame:n,lineNumber:r}){const{url:a,clipboard:o}=Po({file:n.file,lineNumber:r});return c.createElement("span",{className:`\n flex group leading-loose hover:~bg-red-500/10\n ${e?" ~bg-red-500/20":""}\n `},!o&&c.createElement("span",{className:"z-30 opacity-0 group-hover:opacity-100 sticky left-10 w-0 h-full"},c.createElement("a",{href:a,className:"-ml-3 block"},c.createElement(Fo,null,c.createElement(qa,{className:"text-xs",icon:go})))),o&&c.createElement("span",{className:"z-30 opacity-0 group-hover:opacity-100 sticky w-0 h-full"},c.createElement(zo,{value:a,outside:!0,direction:"right"})),c.createElement("span",{className:"pl-6"},Qo({node:t,useInlineStyles:!1,key:`code-segement-${r}`})))}function _l({frame:e}){const t=Object.values(e.code_snippet).join("\n"),n=Object.keys(e.code_snippet).map(e=>Number(e)),r=n.indexOf(e.line_number),a=c.useMemo(()=>({rows:t})=>t.map((t,a)=>c.createElement(Ll,{key:n[a],frame:e,highlight:a===r,row:t,lineNumber:n[a]})),[e]);return c.createElement("main",{className:"flex items-stretch flex-grow overflow-x-auto overflow-y-hidden scrollbar-hidden-x mask-fade-r text-sm"},c.createElement("nav",{className:"sticky left-0 flex flex-none z-20"},c.createElement("div",{className:"select-none text-right"},n.map(t=>c.createElement("p",{key:t,className:`\n px-2 font-mono leading-loose select-none\n ${Number(t)===e.line_number?" text-opacity-75 ~text-red-700 ~bg-red-500/30":""}\n `},c.createElement("span",{className:"~text-gray-500"},t))))),c.createElement("div",{className:"flex-grow pr-10"},c.createElement(Il,{language:(o=e.relative_file,o.endsWith(".blade.php")?"blade":o.match(/^resources\/views\//)?"php-template":"php"),renderer:a,customStyle:{background:"transparent"}},t)));var o}function Pl(e){var t=this.__data__=new ct(e);this.size=t.size}Il.registerLanguage("php",function(e){const t={className:"variable",begin:"\\$+[a-zA-Z_-ÿ][a-zA-Z0-9_-ÿ]*(?![A-Za-z0-9])(?![$])"},n={className:"meta",variants:[{begin:/<\?php/,relevance:10},{begin:/<\?[=]?/},{begin:/\?>/}]},r={className:"subst",variants:[{begin:/\$\w+/},{begin:/\{\$/,end:/\}/}]},a=e.inherit(e.APOS_STRING_MODE,{illegal:null}),o=e.inherit(e.QUOTE_STRING_MODE,{illegal:null,contains:e.QUOTE_STRING_MODE.contains.concat(r)}),i=e.END_SAME_AS_BEGIN({begin:/<<<[ \t]*(\w+)\n/,end:/[ \t]*(\w+)\b/,contains:e.QUOTE_STRING_MODE.contains.concat(r)}),l={className:"string",contains:[e.BACKSLASH_ESCAPE,n],variants:[e.inherit(a,{begin:"b'",end:"'"}),e.inherit(o,{begin:'b"',end:'"'}),o,a,i]},s={className:"number",variants:[{begin:"\\b0b[01]+(?:_[01]+)*\\b"},{begin:"\\b0o[0-7]+(?:_[0-7]+)*\\b"},{begin:"\\b0x[\\da-f]+(?:_[\\da-f]+)*\\b"},{begin:"(?:\\b\\d+(?:_\\d+)*(\\.(?:\\d+(?:_\\d+)*))?|\\B\\.\\d+)(?:e[+-]?\\d+)?"}],relevance:0},c={keyword:"__CLASS__ __DIR__ __FILE__ __FUNCTION__ __LINE__ __METHOD__ __NAMESPACE__ __TRAIT__ die echo exit include include_once print require require_once array abstract and as binary bool boolean break callable case catch class clone const continue declare default do double else elseif empty enddeclare endfor endforeach endif endswitch endwhile enum eval extends final finally float for foreach from global goto if implements instanceof insteadof int integer interface isset iterable list match|0 mixed new object or private protected public real return string switch throw trait try unset use var void while xor yield",literal:"false null true",built_in:"Error|0 AppendIterator ArgumentCountError ArithmeticError ArrayIterator ArrayObject AssertionError BadFunctionCallException BadMethodCallException CachingIterator CallbackFilterIterator CompileError Countable DirectoryIterator DivisionByZeroError DomainException EmptyIterator ErrorException Exception FilesystemIterator FilterIterator GlobIterator InfiniteIterator InvalidArgumentException IteratorIterator LengthException LimitIterator LogicException MultipleIterator NoRewindIterator OutOfBoundsException OutOfRangeException OuterIterator OverflowException ParentIterator ParseError RangeException RecursiveArrayIterator RecursiveCachingIterator RecursiveCallbackFilterIterator RecursiveDirectoryIterator RecursiveFilterIterator RecursiveIterator RecursiveIteratorIterator RecursiveRegexIterator RecursiveTreeIterator RegexIterator RuntimeException SeekableIterator SplDoublyLinkedList SplFileInfo SplFileObject SplFixedArray SplHeap SplMaxHeap SplMinHeap SplObjectStorage SplObserver SplObserver SplPriorityQueue SplQueue SplStack SplSubject SplSubject SplTempFileObject TypeError UnderflowException UnexpectedValueException UnhandledMatchError ArrayAccess Closure Generator Iterator IteratorAggregate Serializable Stringable Throwable Traversable WeakReference WeakMap Directory __PHP_Incomplete_Class parent php_user_filter self static stdClass"};return{aliases:["php3","php4","php5","php6","php7","php8"],case_insensitive:!0,keywords:c,contains:[e.HASH_COMMENT_MODE,e.COMMENT("//","$",{contains:[n]}),e.COMMENT("/\\*","\\*/",{contains:[{className:"doctag",begin:"@[A-Za-z]+"}]}),e.COMMENT("__halt_compiler.+?;",!1,{endsWithParent:!0,keywords:"__halt_compiler"}),n,{className:"keyword",begin:/\$this\b/},t,{begin:/(::|->)+[a-zA-Z_\x7f-\xff][a-zA-Z0-9_\x7f-\xff]*/},{className:"function",relevance:0,beginKeywords:"fn function",end:/[;{]/,excludeEnd:!0,illegal:"[$%\\[]",contains:[{beginKeywords:"use"},e.UNDERSCORE_TITLE_MODE,{begin:"=>",endsParent:!0},{className:"params",begin:"\\(",end:"\\)",excludeBegin:!0,excludeEnd:!0,keywords:c,contains:["self",t,e.C_BLOCK_COMMENT_MODE,l,s]}]},{className:"class",variants:[{beginKeywords:"enum",illegal:/[($"]/},{beginKeywords:"class interface trait",illegal:/[:($"]/}],relevance:0,end:/\{/,excludeEnd:!0,contains:[{beginKeywords:"extends implements"},e.UNDERSCORE_TITLE_MODE]},{beginKeywords:"namespace",relevance:0,end:";",illegal:/[.']/,contains:[e.UNDERSCORE_TITLE_MODE]},{beginKeywords:"use",relevance:0,end:";",contains:[e.UNDERSCORE_TITLE_MODE]},l,s]}}),Il.registerLanguage("php-template",function(e){return{name:"PHP template",subLanguage:"xml",contains:[{begin:/<\?(php|=)?/,end:/\?>/,subLanguage:"php",contains:[{begin:"/\\*",end:"\\*/",skip:!0},{begin:'b"',end:'"',skip:!0},{begin:"b'",end:"'",skip:!0},e.inherit(e.APOS_STRING_MODE,{illegal:null,className:null,contains:null,skip:!0}),e.inherit(e.QUOTE_STRING_MODE,{illegal:null,className:null,contains:null,skip:!0})]}]}}),Il.registerLanguage("blade",function(e){return{name:"Blade",case_insensitive:!0,subLanguage:"php-template",contains:[e.COMMENT(/\{\{--/,/--\}\}/),{className:"template-variable",begin:/\{\{/,starts:{end:/\}\}/,returnEnd:!0,subLanguage:"php"}},{className:"template-variable",begin:/\}\}/},{className:"template-variable",begin:/\{\{\{/,starts:{end:/\}\}\}/,returnEnd:!0,subLanguage:"php"}},{className:"template-variable",begin:/\}\}\}/},{className:"template-variable",begin:/\{!!/,starts:{end:/!!\}/,returnEnd:!0,subLanguage:"php"}},{className:"template-variable",begin:/!!\}/},{className:"template-tag",begin:/@php\(/,starts:{end:/\)/,returnEnd:!0,subLanguage:"php"},relevance:15},{className:"template-tag",begin:/@php/,starts:{end:/@endphp/,returnEnd:!0,subLanguage:"php"},relevance:10},{className:"attr",begin:/:[\w-]+="/,starts:{end:/"(?=\s|\n|\/)/,returnEnd:!0,subLanguage:"php"}},{begin:/@\w+/,end:/\W/,excludeEnd:!0,className:"template-tag"}]}}),Il.registerLanguage("xml",function(e){const t=fl(/[A-Z_]/,fl("(",/[A-Z0-9_.-]*:/,")?"),/[A-Z0-9_.-]*/),n={className:"symbol",begin:/&[a-z]+;|&#[0-9]+;|&#x[a-f0-9]+;/},r={begin:/\s/,contains:[{className:"meta-keyword",begin:/#?[a-z_][a-z1-9_-]+/,illegal:/\n/}]},a=e.inherit(r,{begin:/\(/,end:/\)/}),o=e.inherit(e.APOS_STRING_MODE,{className:"meta-string"}),i=e.inherit(e.QUOTE_STRING_MODE,{className:"meta-string"}),l={endsWithParent:!0,illegal:/</,relevance:0,contains:[{className:"attr",begin:/[A-Za-z0-9._:-]+/,relevance:0},{begin:/=\s*/,relevance:0,contains:[{className:"string",endsParent:!0,variants:[{begin:/"/,end:/"/,contains:[n]},{begin:/'/,end:/'/,contains:[n]},{begin:/[^\s"'=<>`]+/}]}]}]};return{name:"HTML, XML",aliases:["html","xhtml","rss","atom","xjb","xsd","xsl","plist","wsf","svg"],case_insensitive:!0,contains:[{className:"meta",begin:/<![a-z]/,end:/>/,relevance:10,contains:[r,i,o,a,{begin:/\[/,end:/\]/,contains:[{className:"meta",begin:/<![a-z]/,end:/>/,contains:[r,a,i,o]}]}]},e.COMMENT(/<!--/,/-->/,{relevance:10}),{begin:/<!\[CDATA\[/,end:/\]\]>/,relevance:10},n,{className:"meta",begin:/<\?xml/,end:/\?>/,relevance:10},{className:"tag",begin:/<style(?=\s|>)/,end:/>/,keywords:{name:"style"},contains:[l],starts:{end:/<\/style>/,returnEnd:!0,subLanguage:["css","xml"]}},{className:"tag",begin:/<script(?=\s|>)/,end:/>/,keywords:{name:"script"},contains:[l],starts:{end:/<\/script>/,returnEnd:!0,subLanguage:["javascript","handlebars","xml"]}},{className:"tag",begin:/<>|<\/>/},{className:"tag",begin:fl(/</,ul(fl(t,dl(/\/>/,/>/,/\s/)))),end:/\/?>/,contains:[{className:"name",begin:t,relevance:0,starts:l}]},{className:"tag",begin:fl(/<\//,ul(fl(t,/>/))),contains:[{className:"name",begin:t,relevance:0},{begin:/>/,relevance:0,endsParent:!0}]}]}}),Il.registerLanguage("css",function(e){const t=(e=>({IMPORTANT:{className:"meta",begin:"!important"},HEXCOLOR:{className:"number",begin:"#([a-fA-F0-9]{6}|[a-fA-F0-9]{3})"},ATTRIBUTE_SELECTOR_MODE:{className:"selector-attr",begin:/\[/,end:/\]/,illegal:"$",contains:[e.APOS_STRING_MODE,e.QUOTE_STRING_MODE]}}))(e),n=[e.APOS_STRING_MODE,e.QUOTE_STRING_MODE];return{name:"CSS",case_insensitive:!0,illegal:/[=|'\$]/,keywords:{keyframePosition:"from to"},classNameAliases:{keyframePosition:"selector-tag"},contains:[e.C_BLOCK_COMMENT_MODE,{begin:/-(webkit|moz|ms|o)-(?=[a-z])/},e.CSS_NUMBER_MODE,{className:"selector-id",begin:/#[A-Za-z0-9_-]+/,relevance:0},{className:"selector-class",begin:"\\.[a-zA-Z-][a-zA-Z0-9_-]*",relevance:0},t.ATTRIBUTE_SELECTOR_MODE,{className:"selector-pseudo",variants:[{begin:":("+hl.join("|")+")"},{begin:"::("+gl.join("|")+")"}]},{className:"attribute",begin:"\\b("+yl.join("|")+")\\b"},{begin:":",end:"[;}]",contains:[t.HEXCOLOR,t.IMPORTANT,e.CSS_NUMBER_MODE,...n,{begin:/(url|data-uri)\(/,end:/\)/,relevance:0,keywords:{built_in:"url data-uri"},contains:[{className:"string",begin:/[^)]/,endsWithParent:!0,excludeEnd:!0}]},{className:"built_in",begin:/[\w-]+(?=\()/}]},{begin:(r=/@/,function(...e){return e.map(e=>function(e){return e?"string"==typeof e?e:e.source:null}(e)).join("")}("(?=",r,")")),end:"[{;]",relevance:0,illegal:/:/,contains:[{className:"keyword",begin:/@-?\w[\w]*(-\w+)*/},{begin:/\s/,endsWithParent:!0,excludeEnd:!0,relevance:0,keywords:{$pattern:/[a-z-]+/,keyword:"and or not only",attribute:ml.join(" ")},contains:[{begin:/[a-z-]+(?=:)/,className:"attribute"},...n,e.CSS_NUMBER_MODE]}]},{className:"selector-tag",begin:"\\b("+pl.join("|")+")\\b"}]};var r}),Il.registerLanguage("javascript",function(e){const t="[A-Za-z$_][0-9A-Za-z$_]*",n={begin:/<[A-Za-z0-9\\._:-]+/,end:/\/[A-Za-z0-9\\._:-]+>|\/>/,isTrulyOpeningTag:(e,t)=>{const n=e[0].length+e.index,r=e.input[n];"<"!==r?">"===r&&(((e,{after:t})=>{const n="</"+e[0].slice(1);return-1!==e.input.indexOf(n,t)})(e,{after:n})||t.ignoreMatch()):t.ignoreMatch()}},r={$pattern:"[A-Za-z$_][0-9A-Za-z$_]*",keyword:El,literal:Tl,built_in:Sl},a="\\.([0-9](_?[0-9])*)",o="0|[1-9](_?[0-9])*|0[0-7]*[89][0-9]*",i={className:"number",variants:[{begin:`(\\b(${o})((${a})|\\.)?|(${a}))[eE][+-]?([0-9](_?[0-9])*)\\b`},{begin:`\\b(${o})\\b((${a})\\b|\\.)?|(${a})\\b`},{begin:"\\b(0|[1-9](_?[0-9])*)n\\b"},{begin:"\\b0[xX][0-9a-fA-F](_?[0-9a-fA-F])*n?\\b"},{begin:"\\b0[bB][0-1](_?[0-1])*n?\\b"},{begin:"\\b0[oO][0-7](_?[0-7])*n?\\b"},{begin:"\\b0[0-7]+n?\\b"}],relevance:0},l={className:"subst",begin:"\\$\\{",end:"\\}",keywords:r,contains:[]},s={begin:"html`",end:"",starts:{end:"`",returnEnd:!1,contains:[e.BACKSLASH_ESCAPE,l],subLanguage:"xml"}},c={begin:"css`",end:"",starts:{end:"`",returnEnd:!1,contains:[e.BACKSLASH_ESCAPE,l],subLanguage:"css"}},u={className:"string",begin:"`",end:"`",contains:[e.BACKSLASH_ESCAPE,l]},f={className:"comment",variants:[e.COMMENT(/\/\*\*(?!\/)/,"\\*/",{relevance:0,contains:[{className:"doctag",begin:"@[A-Za-z]+",contains:[{className:"type",begin:"\\{",end:"\\}",relevance:0},{className:"variable",begin:t+"(?=\\s*(-)|$)",endsParent:!0,relevance:0},{begin:/(?=[^\n])\s/,relevance:0}]}]}),e.C_BLOCK_COMMENT_MODE,e.C_LINE_COMMENT_MODE]},d=[e.APOS_STRING_MODE,e.QUOTE_STRING_MODE,s,c,u,i,e.REGEXP_MODE];l.contains=d.concat({begin:/\{/,end:/\}/,keywords:r,contains:["self"].concat(d)});const p=[].concat(f,l.contains),m=p.concat([{begin:/\(/,end:/\)/,keywords:r,contains:["self"].concat(p)}]),h={className:"params",begin:/\(/,end:/\)/,excludeBegin:!0,excludeEnd:!0,keywords:r,contains:m};return{name:"Javascript",aliases:["js","jsx","mjs","cjs"],keywords:r,exports:{PARAMS_CONTAINS:m},illegal:/#(?![$_A-z])/,contains:[e.SHEBANG({label:"shebang",binary:"node",relevance:5}),{label:"use_strict",className:"meta",relevance:10,begin:/^\s*['"]use (strict|asm)['"]/},e.APOS_STRING_MODE,e.QUOTE_STRING_MODE,s,c,u,f,i,{begin:Nl(/[{,\n]\s*/,wl(Nl(/(((\/\/.*$)|(\/\*(\*[^/]|[^*])*\*\/))\s*)*/,t+"\\s*:"))),relevance:0,contains:[{className:"attr",begin:t+wl("\\s*:"),relevance:0}]},{begin:"("+e.RE_STARTERS_RE+"|\\b(case|return|throw)\\b)\\s*",keywords:"return throw case",contains:[f,e.REGEXP_MODE,{className:"function",begin:"(\\([^()]*(\\([^()]*(\\([^()]*\\)[^()]*)*\\)[^()]*)*\\)|"+e.UNDERSCORE_IDENT_RE+")\\s*=>",returnBegin:!0,end:"\\s*=>",contains:[{className:"params",variants:[{begin:e.UNDERSCORE_IDENT_RE,relevance:0},{className:null,begin:/\(\s*\)/,skip:!0},{begin:/\(/,end:/\)/,excludeBegin:!0,excludeEnd:!0,keywords:r,contains:m}]}]},{begin:/,/,relevance:0},{className:"",begin:/\s/,end:/\s*/,skip:!0},{variants:[{begin:"<>",end:"</>"},{begin:n.begin,"on:begin":n.isTrulyOpeningTag,end:n.end}],subLanguage:"xml",contains:[{begin:n.begin,end:n.end,skip:!0,contains:["self"]}]}],relevance:0},{className:"function",beginKeywords:"function",end:/[{;]/,excludeEnd:!0,keywords:r,contains:["self",e.inherit(e.TITLE_MODE,{begin:t}),h],illegal:/%/},{beginKeywords:"while if switch catch for"},{className:"function",begin:e.UNDERSCORE_IDENT_RE+"\\([^()]*(\\([^()]*(\\([^()]*\\)[^()]*)*\\)[^()]*)*\\)\\s*\\{",returnBegin:!0,contains:[h,e.inherit(e.TITLE_MODE,{begin:t})]},{variants:[{begin:"\\."+t},{begin:"\\$"+t}],relevance:0},{className:"class",beginKeywords:"class",end:/[{;=]/,excludeEnd:!0,illegal:/[:"[\]]/,contains:[{beginKeywords:"extends"},e.UNDERSCORE_TITLE_MODE]},{begin:/\b(?=constructor)/,end:/[{;]/,excludeEnd:!0,contains:[e.inherit(e.TITLE_MODE,{begin:t}),"self",h]},{begin:"(get|set)\\s+(?="+t+"\\()",end:/\{/,keywords:"get set",contains:[e.inherit(e.TITLE_MODE,{begin:t}),{begin:/\(\)/},h]},{begin:/\$[(.]/}]}}),Il.registerLanguage("handlebars",function(e){const t={"builtin-name":["action","bindattr","collection","component","concat","debugger","each","each-in","get","hash","if","in","input","link-to","loc","log","lookup","mut","outlet","partial","query-params","render","template","textarea","unbound","unless","view","with","yield"]},n=/\[\]|\[[^\]]+\]/,r=/[^\s!"#%&'()*+,.\/;<=>@\[\\\]^`{|}~]+/,a=function(...e){return"("+e.map(e=>vl(e)).join("|")+")"}(/""|"[^"]+"/,/''|'[^']+'/,n,r),o=bl(bl("(",/\.|\.\/|\//,")?"),a,(d=bl(/(\.|\/)/,a),bl("(",d,")*"))),i=bl("(",n,"|",r,")(?==)"),l={begin:o,lexemes:/[\w.\/]+/},s=e.inherit(l,{keywords:{literal:["true","false","undefined","null"]}}),c={begin:/\(/,end:/\)/},u={contains:[e.NUMBER_MODE,e.QUOTE_STRING_MODE,e.APOS_STRING_MODE,{begin:/as\s+\|/,keywords:{keyword:"as"},end:/\|/,contains:[{begin:/\w+/}]},{className:"attr",begin:i,relevance:0,starts:{begin:/=/,end:/=/,starts:{contains:[e.NUMBER_MODE,e.QUOTE_STRING_MODE,e.APOS_STRING_MODE,s,c]}}},s,c],returnEnd:!0},f=e.inherit(l,{className:"name",keywords:t,starts:e.inherit(u,{end:/\)/})});var d;c.contains=[f];const p=e.inherit(l,{keywords:t,className:"name",starts:e.inherit(u,{end:/\}\}/})}),m=e.inherit(l,{keywords:t,className:"name"}),h=e.inherit(l,{className:"name",keywords:t,starts:e.inherit(u,{end:/\}\}/})});return{name:"Handlebars",aliases:["hbs","html.hbs","html.handlebars","htmlbars"],case_insensitive:!0,subLanguage:"xml",contains:[{begin:/\\\{\{/,skip:!0},{begin:/\\\\(?=\{\{)/,skip:!0},e.COMMENT(/\{\{!--/,/--\}\}/),e.COMMENT(/\{\{!/,/\}\}/),{className:"template-tag",begin:/\{\{\{\{(?!\/)/,end:/\}\}\}\}/,contains:[p],starts:{end:/\{\{\{\{\//,returnEnd:!0,subLanguage:"xml"}},{className:"template-tag",begin:/\{\{\{\{\//,end:/\}\}\}\}/,contains:[m]},{className:"template-tag",begin:/\{\{#/,end:/\}\}/,contains:[p]},{className:"template-tag",begin:/\{\{(?=else\}\})/,end:/\}\}/,keywords:"else"},{className:"template-tag",begin:/\{\{(?=else if)/,end:/\}\}/,keywords:"else if"},{className:"template-tag",begin:/\{\{\//,end:/\}\}/,contains:[m]},{className:"template-variable",begin:/\{\{\{/,end:/\}\}\}/,contains:[h]},{className:"template-variable",begin:/\{\{/,end:/\}\}/,contains:[h]}]}}),Il.registerLanguage("sql",function(e){const t=e.COMMENT("--","$"),n=["true","false","unknown"],r=["bigint","binary","blob","boolean","char","character","clob","date","dec","decfloat","decimal","float","int","integer","interval","nchar","nclob","national","numeric","real","row","smallint","time","timestamp","varchar","varying","varbinary"],a=["abs","acos","array_agg","asin","atan","avg","cast","ceil","ceiling","coalesce","corr","cos","cosh","count","covar_pop","covar_samp","cume_dist","dense_rank","deref","element","exp","extract","first_value","floor","json_array","json_arrayagg","json_exists","json_object","json_objectagg","json_query","json_table","json_table_primitive","json_value","lag","last_value","lead","listagg","ln","log","log10","lower","max","min","mod","nth_value","ntile","nullif","percent_rank","percentile_cont","percentile_disc","position","position_regex","power","rank","regr_avgx","regr_avgy","regr_count","regr_intercept","regr_r2","regr_slope","regr_sxx","regr_sxy","regr_syy","row_number","sin","sinh","sqrt","stddev_pop","stddev_samp","substring","substring_regex","sum","tan","tanh","translate","translate_regex","treat","trim","trim_array","unnest","upper","value_of","var_pop","var_samp","width_bucket"],o=["create table","insert into","primary key","foreign key","not null","alter table","add constraint","grouping sets","on overflow","character set","respect nulls","ignore nulls","nulls first","nulls last","depth first","breadth first"],i=a,l=["abs","acos","all","allocate","alter","and","any","are","array","array_agg","array_max_cardinality","as","asensitive","asin","asymmetric","at","atan","atomic","authorization","avg","begin","begin_frame","begin_partition","between","bigint","binary","blob","boolean","both","by","call","called","cardinality","cascaded","case","cast","ceil","ceiling","char","char_length","character","character_length","check","classifier","clob","close","coalesce","collate","collect","column","commit","condition","connect","constraint","contains","convert","copy","corr","corresponding","cos","cosh","count","covar_pop","covar_samp","create","cross","cube","cume_dist","current","current_catalog","current_date","current_default_transform_group","current_path","current_role","current_row","current_schema","current_time","current_timestamp","current_path","current_role","current_transform_group_for_type","current_user","cursor","cycle","date","day","deallocate","dec","decimal","decfloat","declare","default","define","delete","dense_rank","deref","describe","deterministic","disconnect","distinct","double","drop","dynamic","each","element","else","empty","end","end_frame","end_partition","end-exec","equals","escape","every","except","exec","execute","exists","exp","external","extract","false","fetch","filter","first_value","float","floor","for","foreign","frame_row","free","from","full","function","fusion","get","global","grant","group","grouping","groups","having","hold","hour","identity","in","indicator","initial","inner","inout","insensitive","insert","int","integer","intersect","intersection","interval","into","is","join","json_array","json_arrayagg","json_exists","json_object","json_objectagg","json_query","json_table","json_table_primitive","json_value","lag","language","large","last_value","lateral","lead","leading","left","like","like_regex","listagg","ln","local","localtime","localtimestamp","log","log10","lower","match","match_number","match_recognize","matches","max","member","merge","method","min","minute","mod","modifies","module","month","multiset","national","natural","nchar","nclob","new","no","none","normalize","not","nth_value","ntile","null","nullif","numeric","octet_length","occurrences_regex","of","offset","old","omit","on","one","only","open","or","order","out","outer","over","overlaps","overlay","parameter","partition","pattern","per","percent","percent_rank","percentile_cont","percentile_disc","period","portion","position","position_regex","power","precedes","precision","prepare","primary","procedure","ptf","range","rank","reads","real","recursive","ref","references","referencing","regr_avgx","regr_avgy","regr_count","regr_intercept","regr_r2","regr_slope","regr_sxx","regr_sxy","regr_syy","release","result","return","returns","revoke","right","rollback","rollup","row","row_number","rows","running","savepoint","scope","scroll","search","second","seek","select","sensitive","session_user","set","show","similar","sin","sinh","skip","smallint","some","specific","specifictype","sql","sqlexception","sqlstate","sqlwarning","sqrt","start","static","stddev_pop","stddev_samp","submultiset","subset","substring","substring_regex","succeeds","sum","symmetric","system","system_time","system_user","table","tablesample","tan","tanh","then","time","timestamp","timezone_hour","timezone_minute","to","trailing","translate","translate_regex","translation","treat","trigger","trim","trim_array","true","truncate","uescape","union","unique","unknown","unnest","update ","upper","user","using","value","values","value_of","var_pop","var_samp","varbinary","varchar","varying","versioning","when","whenever","where","width_bucket","window","with","within","without","year","add","asc","collation","desc","final","first","last","view"].filter(e=>!a.includes(e)),s={begin:Ol(/\b/,Cl(...i),/\s*\(/),keywords:{built_in:i}};return{name:"SQL",case_insensitive:!0,illegal:/[{}]|<\//,keywords:{$pattern:/\b[\w\.]+/,keyword:function(e,{exceptions:t,when:n}={}){const r=n;return t=t||[],e.map(e=>e.match(/\|\d+$/)||t.includes(e)?e:r(e)?`${e}|0`:e)}(l,{when:e=>e.length<3}),literal:n,type:r,built_in:["current_catalog","current_date","current_default_transform_group","current_path","current_role","current_schema","current_transform_group_for_type","current_user","session_user","system_time","system_user","current_time","localtime","current_timestamp","localtimestamp"]},contains:[{begin:Cl(...o),keywords:{$pattern:/[\w\.]+/,keyword:l.concat(o),literal:n,type:r}},{className:"type",begin:Cl("double precision","large object","with timezone","without timezone")},s,{className:"variable",begin:/@[a-z0-9]+/},{className:"string",variants:[{begin:/'/,end:/'/,contains:[{begin:/''/}]}]},{begin:/"/,end:/"/,contains:[{begin:/""/}]},e.C_NUMBER_MODE,e.C_BLOCK_COMMENT_MODE,t,{className:"operator",begin:/[-+*/=%^~]|&&?|\|\|?|!=?|<(?:=>?|<|>)?|>[>=]?/,relevance:0}]}}),Il.registerLanguage("curl",function(e){return{name:"curl",aliases:["curl"],keywords:"curl",case_insensitive:!0,contains:[{className:"literal",begin:/(--request|-X)\s/,contains:[{className:"symbol",begin:/(get|post|delete|options|head|put|patch|trace|connect)/,end:/\s/,returnEnd:!0}],returnEnd:!0,relevance:10},{className:"literal",begin:/--/,end:/[\s"]/,returnEnd:!0,relevance:0},{className:"literal",begin:/-\w/,end:/[\s"]/,returnEnd:!0,relevance:0},{className:"string",begin:/"/,end:/"/,contains:[e.BACKSLASH_ESCAPE,{className:"variable",begin:/\$\(/,end:/\)/,contains:[e.BACKSLASH_ESCAPE]}],relevance:0},{className:"string",begin:/\\"/,relevance:0},{className:"string",begin:/'/,end:/'/,relevance:0},e.APOS_STRING_MODE,e.QUOTE_STRING_MODE,{className:"number",variants:[{begin:e.C_NUMBER_RE}],relevance:0},{match:/(\/[a-z._-]+)+/}]}}),Il.registerLanguage("json",function(e){const t={literal:"true false null"},n=[e.C_LINE_COMMENT_MODE,e.C_BLOCK_COMMENT_MODE],r=[e.QUOTE_STRING_MODE,e.C_NUMBER_MODE],a={end:",",endsWithParent:!0,excludeEnd:!0,contains:r,keywords:t},o={begin:/\{/,end:/\}/,contains:[{className:"attr",begin:/"/,end:/"/,contains:[e.BACKSLASH_ESCAPE],illegal:"\\n"},e.inherit(a,{begin:/:/})].concat(n),illegal:"\\S"},i={begin:"\\[",end:"\\]",contains:[e.inherit(a)],illegal:"\\S"};return r.push(o,i),n.forEach(function(e){r.push(e)}),{name:"JSON",contains:r,keywords:t,illegal:"\\S"}}),Pl.prototype.clear=function(){this.__data__=new ct,this.size=0},Pl.prototype.delete=function(e){var t=this.__data__,n=t.delete(e);return this.size=t.size,n},Pl.prototype.get=function(e){return this.__data__.get(e)},Pl.prototype.has=function(e){return this.__data__.has(e)},Pl.prototype.set=function(e,t){var n=this.__data__;if(n instanceof ct){var r=n.__data__;if(!ut||r.length<199)return r.push([e,t]),this.size=++n.size,this;n=this.__data__=new pt(r)}return n.set(e,t),this.size=n.size,this};var Ml=Pl,Dl=function(e,t){for(var n=-1,r=null==e?0:e.length;++n<r;)if(t(e[n],n,e))return!0;return!1},Ul=function(e,t,n,r,a,o){var i=1&n,l=e.length,s=t.length;if(l!=s&&!(i&&s>l))return!1;var c=o.get(e),u=o.get(t);if(c&&u)return c==t&&u==e;var f=-1,d=!0,p=2&n?new ht:void 0;for(o.set(e,t),o.set(t,e);++f<l;){var m=e[f],h=t[f];if(r)var g=i?r(h,m,f,t,e,o):r(m,h,f,e,t,o);if(void 0!==g){if(g)continue;d=!1;break}if(p){if(!Dl(t,function(e,t){if(!bt(p,t)&&(m===e||a(m,e,n,r,o)))return p.push(t)})){d=!1;break}}else if(m!==h&&!a(m,h,n,r,o)){d=!1;break}}return o.delete(e),o.delete(t),d},jl=De.Uint8Array,Fl=function(e){var t=-1,n=Array(e.size);return e.forEach(function(e,r){n[++t]=[r,e]}),n},zl=Ue?Ue.prototype:void 0,Bl=zl?zl.valueOf:void 0,Hl=function(e,t){for(var n=-1,r=t.length,a=e.length;++n<r;)e[a+n]=t[n];return e},Vl=Array.isArray,Wl=function(e,t,n){var r=t(e);return Vl(e)?r:Hl(r,n(e))},Gl=function(){return[]},Yl=Object.prototype.propertyIsEnumerable,$l=Object.getOwnPropertySymbols,Xl=$l?function(e){return null==e?[]:(e=Object(e),function(t,n){for(var r=-1,a=null==t?0:t.length,o=0,i=[];++r<a;){var l=t[r];Yl.call(e,l)&&(i[o++]=l)}return i}($l(e)))}:Gl,ql=function(e){return null!=e&&"object"==typeof e},Kl=function(e){return ql(e)&&"[object Arguments]"==We(e)},Jl=Object.prototype,Ql=Jl.hasOwnProperty,Zl=Jl.propertyIsEnumerable,es=Kl(function(){return arguments}())?Kl:function(e){return ql(e)&&Ql.call(e,"callee")&&!Zl.call(e,"callee")},ts=function(){return!1},ns=Le(function(e,t){var n=t&&!t.nodeType&&t,r=n&&e&&!e.nodeType&&e,a=r&&r.exports===n?De.Buffer:void 0;e.exports=(a?a.isBuffer:void 0)||ts}),rs=/^(?:0|[1-9]\d*)$/,as=function(e,t){var n=typeof e;return!!(t=null==t?9007199254740991:t)&&("number"==n||"symbol"!=n&&rs.test(e))&&e>-1&&e%1==0&&e<t},os=function(e){return"number"==typeof e&&e>-1&&e%1==0&&e<=9007199254740991},is={};is["[object Float32Array]"]=is["[object Float64Array]"]=is["[object Int8Array]"]=is["[object Int16Array]"]=is["[object Int32Array]"]=is["[object Uint8Array]"]=is["[object Uint8ClampedArray]"]=is["[object Uint16Array]"]=is["[object Uint32Array]"]=!0,is["[object Arguments]"]=is["[object Array]"]=is["[object ArrayBuffer]"]=is["[object Boolean]"]=is["[object DataView]"]=is["[object Date]"]=is["[object Error]"]=is["[object Function]"]=is["[object Map]"]=is["[object Number]"]=is["[object Object]"]=is["[object RegExp]"]=is["[object Set]"]=is["[object String]"]=is["[object WeakMap]"]=!1;var ls,ss=Le(function(e,t){var n=t&&!t.nodeType&&t,r=n&&e&&!e.nodeType&&e,a=r&&r.exports===n&&Pe.process,o=function(){try{return r&&r.require&&r.require("util").types||a&&a.binding&&a.binding("util")}catch(e){}}();e.exports=o}),cs=ss&&ss.isTypedArray,us=cs?(ls=cs,function(e){return ls(e)}):function(e){return ql(e)&&os(e.length)&&!!is[We(e)]},fs=Object.prototype.hasOwnProperty,ds=function(e,t){var n=Vl(e),r=!n&&es(e),a=!n&&!r&&ns(e),o=!n&&!r&&!a&&us(e),i=n||r||a||o,l=i?function(e,t){for(var n=-1,r=Array(e);++n<e;)r[n]=t(n);return r}(e.length,String):[],s=l.length;for(var c in e)!t&&!fs.call(e,c)||i&&("length"==c||a&&("offset"==c||"parent"==c)||o&&("buffer"==c||"byteLength"==c||"byteOffset"==c)||as(c,s))||l.push(c);return l},ps=Object.prototype,ms=function(e){var t=e&&e.constructor;return e===("function"==typeof t&&t.prototype||ps)},hs=function(e,t){return function(n){return e(t(n))}},gs=hs(Object.keys,Object),ys=Object.prototype.hasOwnProperty,vs=function(e){if(!ms(e))return gs(e);var t=[];for(var n in Object(e))ys.call(e,n)&&"constructor"!=n&&t.push(n);return t},bs=function(e){return null!=e&&os(e.length)&&!Ye(e)},Es=function(e){return bs(e)?ds(e):vs(e)},Ts=function(e){return Wl(e,Es,Xl)},Ss=Object.prototype.hasOwnProperty,ws=Ze(De,"DataView"),Ns=Ze(De,"Promise"),Rs=Ze(De,"WeakMap"),Os=Ke(ws),Cs=Ke(ut),xs=Ke(Ns),ks=Ke(Et),As=Ke(Rs),Is=We;(ws&&"[object DataView]"!=Is(new ws(new ArrayBuffer(1)))||ut&&"[object Map]"!=Is(new ut)||Ns&&"[object Promise]"!=Is(Ns.resolve())||Et&&"[object Set]"!=Is(new Et)||Rs&&"[object WeakMap]"!=Is(new Rs))&&(Is=function(e){var t=We(e),n="[object Object]"==t?e.constructor:void 0,r=n?Ke(n):"";if(r)switch(r){case Os:return"[object DataView]";case Cs:return"[object Map]";case xs:return"[object Promise]";case ks:return"[object Set]";case As:return"[object WeakMap]"}return t});var Ls=Is,_s=Object.prototype.hasOwnProperty,Ps=function e(t,n,r,a,o){return t===n||(null==t||null==n||!ql(t)&&!ql(n)?t!=t&&n!=n:function(e,t,n,r,a,o){var i=Vl(e),l=Vl(t),s=i?"[object Array]":Ls(e),c=l?"[object Array]":Ls(t),u="[object Object]"==(s="[object Arguments]"==s?"[object Object]":s),f="[object Object]"==(c="[object Arguments]"==c?"[object Object]":c),d=s==c;if(d&&ns(e)){if(!ns(t))return!1;i=!0,u=!1}if(d&&!u)return o||(o=new Ml),i||us(e)?Ul(e,t,n,r,a,o):function(e,t,n,r,a,o,i){switch(n){case"[object DataView]":if(e.byteLength!=t.byteLength||e.byteOffset!=t.byteOffset)return!1;e=e.buffer,t=t.buffer;case"[object ArrayBuffer]":return!(e.byteLength!=t.byteLength||!o(new jl(e),new jl(t)));case"[object Boolean]":case"[object Date]":case"[object Number]":return ot(+e,+t);case"[object Error]":return e.name==t.name&&e.message==t.message;case"[object RegExp]":case"[object String]":return e==t+"";case"[object Map]":var l=Fl;case"[object Set]":if(l||(l=Tt),e.size!=t.size&&!(1&r))return!1;var s=i.get(e);if(s)return s==t;r|=2,i.set(e,t);var c=Ul(l(e),l(t),r,a,o,i);return i.delete(e),c;case"[object Symbol]":if(Bl)return Bl.call(e)==Bl.call(t)}return!1}(e,t,s,n,r,a,o);if(!(1&n)){var p=u&&_s.call(e,"__wrapped__"),m=f&&_s.call(t,"__wrapped__");if(p||m){var h=p?e.value():e,g=m?t.value():t;return o||(o=new Ml),a(h,g,n,r,o)}}return!!d&&(o||(o=new Ml),function(e,t,n,r,a,o){var i=1&n,l=Ts(e),s=l.length;if(s!=Ts(t).length&&!i)return!1;for(var c=s;c--;){var u=l[c];if(!(i?u in t:Ss.call(t,u)))return!1}var f=o.get(e),d=o.get(t);if(f&&d)return f==t&&d==e;var p=!0;o.set(e,t),o.set(t,e);for(var m=i;++c<s;){var h=e[u=l[c]],g=t[u];if(r)var y=i?r(g,h,u,t,e,o):r(h,g,u,e,t,o);if(!(void 0===y?h===g||a(h,g,n,r,o):y)){p=!1;break}m||(m="constructor"==u)}if(p&&!m){var v=e.constructor,b=t.constructor;v==b||!("constructor"in e)||!("constructor"in t)||"function"==typeof v&&v instanceof v&&"function"==typeof b&&b instanceof b||(p=!1)}return o.delete(e),o.delete(t),p}(e,t,n,r,a,o))}(t,n,r,a,e,o))},Ms=function(e){return e==e&&!Ge(e)},Ds=function(e,t){return function(n){return null!=n&&n[e]===t&&(void 0!==t||e in Object(n))}},Us=function(e){return"symbol"==typeof e||ql(e)&&"[object Symbol]"==We(e)},js=/\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,Fs=/^\w*$/,zs=function(e,t){if(Vl(e))return!1;var n=typeof e;return!("number"!=n&&"symbol"!=n&&"boolean"!=n&&null!=e&&!Us(e))||Fs.test(e)||!js.test(e)||null!=t&&e in Object(t)};function Bs(e,t){if("function"!=typeof e||null!=t&&"function"!=typeof t)throw new TypeError("Expected a function");var n=function n(){var r=arguments,a=t?t.apply(this,r):r[0],o=n.cache;if(o.has(a))return o.get(a);var i=e.apply(this,r);return n.cache=o.set(a,i)||o,i};return n.cache=new(Bs.Cache||pt),n}Bs.Cache=pt;var Hs,Vs,Ws=/[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g,Gs=/\\(\\)?/g,Ys=(Hs=Bs(function(e){var t=[];return 46===e.charCodeAt(0)&&t.push(""),e.replace(Ws,function(e,n,r,a){t.push(r?a.replace(Gs,"$1"):n||e)}),t},function(e){return 500===Vs.size&&Vs.clear(),e}),Vs=Hs.cache,Hs),$s=function(e,t){for(var n=-1,r=null==e?0:e.length,a=Array(r);++n<r;)a[n]=t(e[n],n,e);return a},Xs=Ue?Ue.prototype:void 0,qs=Xs?Xs.toString:void 0,Ks=function e(t){if("string"==typeof t)return t;if(Vl(t))return $s(t,e)+"";if(Us(t))return qs?qs.call(t):"";var n=t+"";return"0"==n&&1/t==-Infinity?"-0":n},Js=function(e){return null==e?"":Ks(e)},Qs=function(e,t){return Vl(e)?e:zs(e,t)?[e]:Ys(Js(e))},Zs=function(e){if("string"==typeof e||Us(e))return e;var t=e+"";return"0"==t&&1/e==-Infinity?"-0":t},ec=function(e,t){for(var n=0,r=(t=Qs(t,e)).length;null!=e&&n<r;)e=e[Zs(t[n++])];return n&&n==r?e:void 0},tc=function(e,t){return null!=e&&t in Object(e)},nc=function(e){return e},rc=function(e){return"function"==typeof e?e:null==e?nc:"object"==typeof e?Vl(e)?function(e,t){return zs(e)&&Ms(t)?Ds(Zs(e),t):function(n){var r=function(e,t,n){var r=null==e?void 0:ec(e,t);return void 0===r?void 0:r}(n,e);return void 0===r&&r===t?function(e,t){return null!=e&&function(e,t,n){for(var r=-1,a=(t=Qs(t,e)).length,o=!1;++r<a;){var i=Zs(t[r]);if(!(o=null!=e&&n(e,i)))break;e=e[i]}return o||++r!=a?o:!!(a=null==e?0:e.length)&&os(a)&&as(i,a)&&(Vl(e)||es(e))}(e,t,tc)}(n,e):Ps(t,r,3)}}(e[0],e[1]):function(e){var t=function(e){for(var t=Es(e),n=t.length;n--;){var r=t[n],a=e[r];t[n]=[r,a,Ms(a)]}return t}(e);return 1==t.length&&t[0][2]?Ds(t[0][0],t[0][1]):function(n){return n===e||function(e,t,n,r){var a=n.length,o=a;if(null==e)return!o;for(e=Object(e);a--;){var i=n[a];if(i[2]?i[1]!==e[i[0]]:!(i[0]in e))return!1}for(;++a<o;){var l=(i=n[a])[0],s=e[l],c=i[1];if(i[2]){if(void 0===s&&!(l in e))return!1}else{var u=new Ml;if(!Ps(c,s,3,void 0,u))return!1}}return!0}(n,0,t)}}(e):zs(t=e)?(n=Zs(t),function(e){return null==e?void 0:e[n]}):function(e){return function(t){return ec(t,e)}}(t);var t,n},ac=(parseInt,Math.max),oc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0,t.default={WORD:"word",STRING:"string",RESERVED:"reserved",RESERVED_TOP_LEVEL:"reserved-top-level",RESERVED_TOP_LEVEL_NO_INDENT:"reserved-top-level-no-indent",RESERVED_NEWLINE:"reserved-newline",OPERATOR:"operator",OPEN_PAREN:"open-paren",CLOSE_PAREN:"close-paren",LINE_COMMENT:"line-comment",BLOCK_COMMENT:"block-comment",NUMBER:"number",PLACEHOLDER:"placeholder"},e.exports=t.default}),ic=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.sortByLengthDesc=t.escapeRegExp=t.isEmpty=t.last=t.trimSpacesEnd=void 0,t.trimSpacesEnd=function(e){return e.replace(/[\t ]+$/,"")},t.last=function(e){return e[e.length-1]},t.isEmpty=function(e){return!Array.isArray(e)||0===e.length},t.escapeRegExp=function(e){return e.replace(/[\$\(-\+\.\?\[-\^\{-\}]/g,"\\$&")},t.sortByLengthDesc=function(e){return e.sort(function(e,t){return t.length-e.length||e.localeCompare(t)})}}),lc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var n="top-level";t.default=/*#__PURE__*/function(){function e(t){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.indent=t||" ",this.indentTypes=[]}var t;return(t=[{key:"getIndent",value:function(){return this.indent.repeat(this.indentTypes.length)}},{key:"increaseTopLevel",value:function(){this.indentTypes.push(n)}},{key:"increaseBlockLevel",value:function(){this.indentTypes.push("block-level")}},{key:"decreaseTopLevel",value:function(){this.indentTypes.length>0&&(0,ic.last)(this.indentTypes)===n&&this.indentTypes.pop()}},{key:"decreaseBlockLevel",value:function(){for(;this.indentTypes.length>0&&this.indentTypes.pop()===n;);}},{key:"resetIndentation",value:function(){this.indentTypes=[]}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(e.prototype,t),e}(),e.exports=t.default}),sc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var n,r=(n=oc)&&n.__esModule?n:{default:n};t.default=/*#__PURE__*/function(){function e(){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.level=0}var t;return(t=[{key:"beginIfPossible",value:function(e,t){0===this.level&&this.isInlineBlock(e,t)?this.level=1:this.level>0?this.level++:this.level=0}},{key:"end",value:function(){this.level--}},{key:"isActive",value:function(){return this.level>0}},{key:"isInlineBlock",value:function(e,t){for(var n=0,a=0,o=t;o<e.length;o++){var i=e[o];if((n+=i.value.length)>50)return!1;if(i.type===r.default.OPEN_PAREN)a++;else if(i.type===r.default.CLOSE_PAREN&&0==--a)return!0;if(this.isForbiddenToken(i))return!1}return!1}},{key:"isForbiddenToken",value:function(e){var t=e.type;return t===r.default.RESERVED_TOP_LEVEL||t===r.default.RESERVED_NEWLINE||t===r.default.COMMENT||t===r.default.BLOCK_COMMENT||";"===e.value}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(e.prototype,t),e}(),e.exports=t.default}),cc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0,t.default=/*#__PURE__*/function(){function e(t){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.params=t,this.index=0}var t;return(t=[{key:"get",value:function(e){var t=e.key,n=e.value;return this.params?t?this.params[t]:this.params[this.index++]:n}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(e.prototype,t),e}(),e.exports=t.default}),uc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.isEnd=t.isWindow=t.isBy=t.isSet=t.isLimit=t.isBetween=t.isAnd=void 0;var n,r=(n=oc)&&n.__esModule?n:{default:n},a=function(e,t){return function(n){return(null==n?void 0:n.type)===e&&t.test(null==n?void 0:n.value)}},o=a(r.default.RESERVED_NEWLINE,/^AND$/i);t.isAnd=o;var i=a(r.default.RESERVED,/^BETWEEN$/i);t.isBetween=i;var l=a(r.default.RESERVED_TOP_LEVEL,/^LIMIT$/i);t.isLimit=l;var s=a(r.default.RESERVED_TOP_LEVEL,/^[S\u017F]ET$/i);t.isSet=s;var c=a(r.default.RESERVED,/^BY$/i);t.isBy=c;var u=a(r.default.RESERVED_TOP_LEVEL,/^WINDOW$/i);t.isWindow=u;var f=a(r.default.CLOSE_PAREN,/^END$/i);t.isEnd=f}),fc=Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var n=i(oc),r=i(lc),a=i(sc),o=i(cc);function i(e){return e&&e.__esModule?e:{default:e}}function l(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}t.default=/*#__PURE__*/function(){function e(t){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.cfg=t,this.indentation=new r.default(this.cfg.indent),this.inlineBlock=new a.default,this.params=new o.default(this.cfg.params),this.previousReservedToken={},this.tokens=[],this.index=0}var t;return(t=[{key:"tokenizer",value:function(){throw new Error("tokenizer() not implemented by subclass")}},{key:"tokenOverride",value:function(e){return e}},{key:"format",value:function(e){return this.tokens=this.tokenizer().tokenize(e),this.getFormattedQueryFromTokens().trim()}},{key:"getFormattedQueryFromTokens",value:function(){var e=this,t="";return this.tokens.forEach(function(r,a){e.index=a,(r=e.tokenOverride(r)).type===n.default.LINE_COMMENT?t=e.formatLineComment(r,t):r.type===n.default.BLOCK_COMMENT?t=e.formatBlockComment(r,t):r.type===n.default.RESERVED_TOP_LEVEL?(t=e.formatTopLevelReservedWord(r,t),e.previousReservedToken=r):r.type===n.default.RESERVED_TOP_LEVEL_NO_INDENT?(t=e.formatTopLevelReservedWordNoIndent(r,t),e.previousReservedToken=r):r.type===n.default.RESERVED_NEWLINE?(t=e.formatNewlineReservedWord(r,t),e.previousReservedToken=r):r.type===n.default.RESERVED?(t=e.formatWithSpaces(r,t),e.previousReservedToken=r):t=r.type===n.default.OPEN_PAREN?e.formatOpeningParentheses(r,t):r.type===n.default.CLOSE_PAREN?e.formatClosingParentheses(r,t):r.type===n.default.PLACEHOLDER?e.formatPlaceholder(r,t):","===r.value?e.formatComma(r,t):":"===r.value?e.formatWithSpaceAfter(r,t):"."===r.value?e.formatWithoutSpaces(r,t):";"===r.value?e.formatQuerySeparator(r,t):e.formatWithSpaces(r,t)}),t}},{key:"formatLineComment",value:function(e,t){return this.addNewline(t+this.show(e))}},{key:"formatBlockComment",value:function(e,t){return this.addNewline(this.addNewline(t)+this.indentComment(e.value))}},{key:"indentComment",value:function(e){return e.replace(/\n[\t ]*/g,"\n"+this.indentation.getIndent()+" ")}},{key:"formatTopLevelReservedWordNoIndent",value:function(e,t){return this.indentation.decreaseTopLevel(),t=this.addNewline(t)+this.equalizeWhitespace(this.show(e)),this.addNewline(t)}},{key:"formatTopLevelReservedWord",value:function(e,t){return this.indentation.decreaseTopLevel(),t=this.addNewline(t),this.indentation.increaseTopLevel(),t+=this.equalizeWhitespace(this.show(e)),this.addNewline(t)}},{key:"formatNewlineReservedWord",value:function(e,t){return(0,uc.isAnd)(e)&&(0,uc.isBetween)(this.tokenLookBehind(2))?this.formatWithSpaces(e,t):this.addNewline(t)+this.equalizeWhitespace(this.show(e))+" "}},{key:"equalizeWhitespace",value:function(e){return e.replace(/[\t-\r \xA0\u1680\u2000-\u200A\u2028\u2029\u202F\u205F\u3000\uFEFF]+/g," ")}},{key:"formatOpeningParentheses",value:function(e,t){var r,a,o=(l(r={},n.default.OPEN_PAREN,!0),l(r,n.default.LINE_COMMENT,!0),l(r,n.default.OPERATOR,!0),r);return 0!==e.whitespaceBefore.length||o[null===(a=this.tokenLookBehind())||void 0===a?void 0:a.type]||(t=(0,ic.trimSpacesEnd)(t)),t+=this.show(e),this.inlineBlock.beginIfPossible(this.tokens,this.index),this.inlineBlock.isActive()||(this.indentation.increaseBlockLevel(),t=this.addNewline(t)),t}},{key:"formatClosingParentheses",value:function(e,t){return this.inlineBlock.isActive()?(this.inlineBlock.end(),this.formatWithSpaceAfter(e,t)):(this.indentation.decreaseBlockLevel(),this.formatWithSpaces(e,this.addNewline(t)))}},{key:"formatPlaceholder",value:function(e,t){return t+this.params.get(e)+" "}},{key:"formatComma",value:function(e,t){return t=(0,ic.trimSpacesEnd)(t)+this.show(e)+" ",this.inlineBlock.isActive()||(0,uc.isLimit)(this.previousReservedToken)?t:this.addNewline(t)}},{key:"formatWithSpaceAfter",value:function(e,t){return(0,ic.trimSpacesEnd)(t)+this.show(e)+" "}},{key:"formatWithoutSpaces",value:function(e,t){return(0,ic.trimSpacesEnd)(t)+this.show(e)}},{key:"formatWithSpaces",value:function(e,t){return t+this.show(e)+" "}},{key:"formatQuerySeparator",value:function(e,t){return this.indentation.resetIndentation(),(0,ic.trimSpacesEnd)(t)+this.show(e)+"\n".repeat(this.cfg.linesBetweenQueries||1)}},{key:"show",value:function(e){var t=e.type,r=e.value;return!this.cfg.uppercase||t!==n.default.RESERVED&&t!==n.default.RESERVED_TOP_LEVEL&&t!==n.default.RESERVED_TOP_LEVEL_NO_INDENT&&t!==n.default.RESERVED_NEWLINE&&t!==n.default.OPEN_PAREN&&t!==n.default.CLOSE_PAREN?r:r.toUpperCase()}},{key:"addNewline",value:function(e){return(e=(0,ic.trimSpacesEnd)(e)).endsWith("\n")||(e+="\n"),e+this.indentation.getIndent()}},{key:"tokenLookBehind",value:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:1;return this.tokens[this.index-e]}},{key:"tokenLookAhead",value:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:1;return this.tokens[this.index+e]}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(e.prototype,t),e}(),e.exports=t.default});function dc(e){var t={"``":"((`[^`]*($|`))+)","{}":"((\\{[^\\}]*($|\\}))+)","[]":"((\\[[^\\]]*($|\\]))(\\][^\\]]*($|\\]))*)",'""':'(("[^"\\\\]*(?:\\\\.[^"\\\\]*)*("|$))+)',"''":"(('[^'\\\\]*(?:\\\\.[^'\\\\]*)*('|$))+)","N''":"((N'[^'\\\\]*(?:\\\\.[^'\\\\]*)*('|$))+)","U&''":"((U&'[^'\\\\]*(?:\\\\.[^'\\\\]*)*('|$))+)",'U&""':'((U&"[^"\\\\]*(?:\\\\.[^"\\\\]*)*("|$))+)',$$:"((?<tag>\\$\\w*\\$)[\\s\\S]*?(?:\\k<tag>|$))"};return e.map(function(e){return t[e]}).join("|")}function pc(e){return 1===e.length?(0,ic.escapeRegExp)(e):"\\b"+e+"\\b"}var mc=/*#__PURE__*/Object.defineProperty({createOperatorRegex:function(e){return new RegExp("^(".concat((0,ic.sortByLengthDesc)(e).map(ic.escapeRegExp).join("|"),"|.)"),"u")},createLineCommentRegex:function(e){return new RegExp("^((?:".concat(e.map(function(e){return(0,ic.escapeRegExp)(e)}).join("|"),").*?)(?:\r\n|\r|\n|$)"),"u")},createReservedWordRegex:function(e){if(0===e.length)return new RegExp("^\b$","u");var t=(0,ic.sortByLengthDesc)(e).join("|").replace(/ /g,"\\s+");return new RegExp("^(".concat(t,")\\b"),"iu")},createWordRegex:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:[];return new RegExp("^([\\p{Alphabetic}\\p{Mark}\\p{Decimal_Number}\\p{Connector_Punctuation}\\p{Join_Control}".concat(e.join(""),"]+)"),"u")},createStringRegex:function(e){return new RegExp("^("+dc(e)+")","u")},createStringPattern:dc,createParenRegex:function(e){return new RegExp("^("+e.map(pc).join("|")+")","iu")},createPlaceholderRegex:function(e,t){if((0,ic.isEmpty)(e))return!1;var n=e.map(ic.escapeRegExp).join("|");return new RegExp("^((?:".concat(n,")(?:").concat(t,"))"),"u")}},"__esModule",{value:!0}),hc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r,a=(r=oc)&&r.__esModule?r:{default:r},o=function(e){if(e&&e.__esModule)return e;if(null===e||"object"!==n(e)&&"function"!=typeof e)return{default:e};var t=i();if(t&&t.has(e))return t.get(e);var r={},a=Object.defineProperty&&Object.getOwnPropertyDescriptor;for(var o in e)if(Object.prototype.hasOwnProperty.call(e,o)){var l=a?Object.getOwnPropertyDescriptor(e,o):null;l&&(l.get||l.set)?Object.defineProperty(r,o,l):r[o]=e[o]}return r.default=e,t&&t.set(e,r),r}(mc);function i(){if("function"!=typeof WeakMap)return null;var e=new WeakMap;return i=function(){return e},e}function l(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function s(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?l(Object(n),!0).forEach(function(t){c(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):l(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}function c(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function u(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}t.default=/*#__PURE__*/function(){function e(t){var n;!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.WHITESPACE_REGEX=/^([\t-\r \xA0\u1680\u2000-\u200A\u2028\u2029\u202F\u205F\u3000\uFEFF]+)/,this.NUMBER_REGEX=/^((\x2D[\t-\r \xA0\u1680\u2000-\u200A\u2028\u2029\u202F\u205F\u3000\uFEFF]*)?[0-9]+(\.[0-9]+)?([Ee]\x2D?[0-9]+(\.[0-9]+)?)?|0x[0-9A-Fa-f]+|0b[01]+)\b/,this.OPERATOR_REGEX=o.createOperatorRegex(["<>","<=",">="].concat(function(e){if(Array.isArray(e))return u(e)}(n=t.operators||[])||function(e){if("undefined"!=typeof Symbol&&Symbol.iterator in Object(e))return Array.from(e)}(n)||function(e,t){if(e){if("string"==typeof e)return u(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?u(e,t):void 0}}(n)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}())),this.BLOCK_COMMENT_REGEX=/^(\/\*(?:(?![])[\s\S])*?(?:\*\/|$))/,this.LINE_COMMENT_REGEX=o.createLineCommentRegex(t.lineCommentTypes),this.RESERVED_TOP_LEVEL_REGEX=o.createReservedWordRegex(t.reservedTopLevelWords),this.RESERVED_TOP_LEVEL_NO_INDENT_REGEX=o.createReservedWordRegex(t.reservedTopLevelWordsNoIndent),this.RESERVED_NEWLINE_REGEX=o.createReservedWordRegex(t.reservedNewlineWords),this.RESERVED_PLAIN_REGEX=o.createReservedWordRegex(t.reservedWords),this.WORD_REGEX=o.createWordRegex(t.specialWordChars),this.STRING_REGEX=o.createStringRegex(t.stringTypes),this.OPEN_PAREN_REGEX=o.createParenRegex(t.openParens),this.CLOSE_PAREN_REGEX=o.createParenRegex(t.closeParens),this.INDEXED_PLACEHOLDER_REGEX=o.createPlaceholderRegex(t.indexedPlaceholderTypes,"[0-9]*"),this.IDENT_NAMED_PLACEHOLDER_REGEX=o.createPlaceholderRegex(t.namedPlaceholderTypes,"[a-zA-Z0-9._$]+"),this.STRING_NAMED_PLACEHOLDER_REGEX=o.createPlaceholderRegex(t.namedPlaceholderTypes,o.createStringPattern(t.stringTypes))}var t;return(t=[{key:"tokenize",value:function(e){for(var t,n=[];e.length;){var r=this.getWhitespace(e);(e=e.substring(r.length)).length&&(t=this.getNextToken(e,t),e=e.substring(t.value.length),n.push(s(s({},t),{},{whitespaceBefore:r})))}return n}},{key:"getWhitespace",value:function(e){var t=e.match(this.WHITESPACE_REGEX);return t?t[1]:""}},{key:"getNextToken",value:function(e,t){return this.getCommentToken(e)||this.getStringToken(e)||this.getOpenParenToken(e)||this.getCloseParenToken(e)||this.getPlaceholderToken(e)||this.getNumberToken(e)||this.getReservedWordToken(e,t)||this.getWordToken(e)||this.getOperatorToken(e)}},{key:"getCommentToken",value:function(e){return this.getLineCommentToken(e)||this.getBlockCommentToken(e)}},{key:"getLineCommentToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.LINE_COMMENT,regex:this.LINE_COMMENT_REGEX})}},{key:"getBlockCommentToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.BLOCK_COMMENT,regex:this.BLOCK_COMMENT_REGEX})}},{key:"getStringToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.STRING,regex:this.STRING_REGEX})}},{key:"getOpenParenToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.OPEN_PAREN,regex:this.OPEN_PAREN_REGEX})}},{key:"getCloseParenToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.CLOSE_PAREN,regex:this.CLOSE_PAREN_REGEX})}},{key:"getPlaceholderToken",value:function(e){return this.getIdentNamedPlaceholderToken(e)||this.getStringNamedPlaceholderToken(e)||this.getIndexedPlaceholderToken(e)}},{key:"getIdentNamedPlaceholderToken",value:function(e){return this.getPlaceholderTokenWithKey({input:e,regex:this.IDENT_NAMED_PLACEHOLDER_REGEX,parseKey:function(e){return e.slice(1)}})}},{key:"getStringNamedPlaceholderToken",value:function(e){var t=this;return this.getPlaceholderTokenWithKey({input:e,regex:this.STRING_NAMED_PLACEHOLDER_REGEX,parseKey:function(e){return t.getEscapedPlaceholderKey({key:e.slice(2,-1),quoteChar:e.slice(-1)})}})}},{key:"getIndexedPlaceholderToken",value:function(e){return this.getPlaceholderTokenWithKey({input:e,regex:this.INDEXED_PLACEHOLDER_REGEX,parseKey:function(e){return e.slice(1)}})}},{key:"getPlaceholderTokenWithKey",value:function(e){var t=e.parseKey,n=this.getTokenOnFirstMatch({input:e.input,regex:e.regex,type:a.default.PLACEHOLDER});return n&&(n.key=t(n.value)),n}},{key:"getEscapedPlaceholderKey",value:function(e){var t=e.quoteChar;return e.key.replace(new RegExp((0,ic.escapeRegExp)("\\"+t),"gu"),t)}},{key:"getNumberToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.NUMBER,regex:this.NUMBER_REGEX})}},{key:"getOperatorToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.OPERATOR,regex:this.OPERATOR_REGEX})}},{key:"getReservedWordToken",value:function(e,t){if(!t||!t.value||"."!==t.value)return this.getTopLevelReservedToken(e)||this.getNewlineReservedToken(e)||this.getTopLevelReservedTokenNoIndent(e)||this.getPlainReservedToken(e)}},{key:"getTopLevelReservedToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.RESERVED_TOP_LEVEL,regex:this.RESERVED_TOP_LEVEL_REGEX})}},{key:"getNewlineReservedToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.RESERVED_NEWLINE,regex:this.RESERVED_NEWLINE_REGEX})}},{key:"getTopLevelReservedTokenNoIndent",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.RESERVED_TOP_LEVEL_NO_INDENT,regex:this.RESERVED_TOP_LEVEL_NO_INDENT_REGEX})}},{key:"getPlainReservedToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.RESERVED,regex:this.RESERVED_PLAIN_REGEX})}},{key:"getWordToken",value:function(e){return this.getTokenOnFirstMatch({input:e,type:a.default.WORD,regex:this.WORD_REGEX})}},{key:"getTokenOnFirstMatch",value:function(e){var t=e.type,n=e.input.match(e.regex);return n?{type:t,value:n[1]}:void 0}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(e.prototype,t),e}(),e.exports=t.default}),gc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ABS","ACTIVATE","ALIAS","ALL","ALLOCATE","ALLOW","ALTER","ANY","ARE","ARRAY","AS","ASC","ASENSITIVE","ASSOCIATE","ASUTIME","ASYMMETRIC","AT","ATOMIC","ATTRIBUTES","AUDIT","AUTHORIZATION","AUX","AUXILIARY","AVG","BEFORE","BEGIN","BETWEEN","BIGINT","BINARY","BLOB","BOOLEAN","BOTH","BUFFERPOOL","BY","CACHE","CALL","CALLED","CAPTURE","CARDINALITY","CASCADED","CASE","CAST","CCSID","CEIL","CEILING","CHAR","CHARACTER","CHARACTER_LENGTH","CHAR_LENGTH","CHECK","CLOB","CLONE","CLOSE","CLUSTER","COALESCE","COLLATE","COLLECT","COLLECTION","COLLID","COLUMN","COMMENT","COMMIT","CONCAT","CONDITION","CONNECT","CONNECTION","CONSTRAINT","CONTAINS","CONTINUE","CONVERT","CORR","CORRESPONDING","COUNT","COUNT_BIG","COVAR_POP","COVAR_SAMP","CREATE","CROSS","CUBE","CUME_DIST","CURRENT","CURRENT_DATE","CURRENT_DEFAULT_TRANSFORM_GROUP","CURRENT_LC_CTYPE","CURRENT_PATH","CURRENT_ROLE","CURRENT_SCHEMA","CURRENT_SERVER","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_TIMEZONE","CURRENT_TRANSFORM_GROUP_FOR_TYPE","CURRENT_USER","CURSOR","CYCLE","DATA","DATABASE","DATAPARTITIONNAME","DATAPARTITIONNUM","DATE","DAY","DAYS","DB2GENERAL","DB2GENRL","DB2SQL","DBINFO","DBPARTITIONNAME","DBPARTITIONNUM","DEALLOCATE","DEC","DECIMAL","DECLARE","DEFAULT","DEFAULTS","DEFINITION","DELETE","DENSERANK","DENSE_RANK","DEREF","DESCRIBE","DESCRIPTOR","DETERMINISTIC","DIAGNOSTICS","DISABLE","DISALLOW","DISCONNECT","DISTINCT","DO","DOCUMENT","DOUBLE","DROP","DSSIZE","DYNAMIC","EACH","EDITPROC","ELEMENT","ELSE","ELSEIF","ENABLE","ENCODING","ENCRYPTION","END","END-EXEC","ENDING","ERASE","ESCAPE","EVERY","EXCEPTION","EXCLUDING","EXCLUSIVE","EXEC","EXECUTE","EXISTS","EXIT","EXP","EXPLAIN","EXTENDED","EXTERNAL","EXTRACT","FALSE","FENCED","FETCH","FIELDPROC","FILE","FILTER","FINAL","FIRST","FLOAT","FLOOR","FOR","FOREIGN","FREE","FULL","FUNCTION","FUSION","GENERAL","GENERATED","GET","GLOBAL","GOTO","GRANT","GRAPHIC","GROUP","GROUPING","HANDLER","HASH","HASHED_VALUE","HINT","HOLD","HOUR","HOURS","IDENTITY","IF","IMMEDIATE","IN","INCLUDING","INCLUSIVE","INCREMENT","INDEX","INDICATOR","INDICATORS","INF","INFINITY","INHERIT","INNER","INOUT","INSENSITIVE","INSERT","INT","INTEGER","INTEGRITY","INTERSECTION","INTERVAL","INTO","IS","ISOBID","ISOLATION","ITERATE","JAR","JAVA","KEEP","KEY","LABEL","LANGUAGE","LARGE","LATERAL","LC_CTYPE","LEADING","LEAVE","LEFT","LIKE","LINKTYPE","LN","LOCAL","LOCALDATE","LOCALE","LOCALTIME","LOCALTIMESTAMP","LOCATOR","LOCATORS","LOCK","LOCKMAX","LOCKSIZE","LONG","LOOP","LOWER","MAINTAINED","MATCH","MATERIALIZED","MAX","MAXVALUE","MEMBER","MERGE","METHOD","MICROSECOND","MICROSECONDS","MIN","MINUTE","MINUTES","MINVALUE","MOD","MODE","MODIFIES","MODULE","MONTH","MONTHS","MULTISET","NAN","NATIONAL","NATURAL","NCHAR","NCLOB","NEW","NEW_TABLE","NEXTVAL","NO","NOCACHE","NOCYCLE","NODENAME","NODENUMBER","NOMAXVALUE","NOMINVALUE","NONE","NOORDER","NORMALIZE","NORMALIZED","NOT","NULL","NULLIF","NULLS","NUMERIC","NUMPARTS","OBID","OCTET_LENGTH","OF","OFFSET","OLD","OLD_TABLE","ON","ONLY","OPEN","OPTIMIZATION","OPTIMIZE","OPTION","ORDER","OUT","OUTER","OVER","OVERLAPS","OVERLAY","OVERRIDING","PACKAGE","PADDED","PAGESIZE","PARAMETER","PART","PARTITION","PARTITIONED","PARTITIONING","PARTITIONS","PASSWORD","PATH","PERCENTILE_CONT","PERCENTILE_DISC","PERCENT_RANK","PIECESIZE","PLAN","POSITION","POWER","PRECISION","PREPARE","PREVVAL","PRIMARY","PRIQTY","PRIVILEGES","PROCEDURE","PROGRAM","PSID","PUBLIC","QUERY","QUERYNO","RANGE","RANK","READ","READS","REAL","RECOVERY","RECURSIVE","REF","REFERENCES","REFERENCING","REFRESH","REGR_AVGX","REGR_AVGY","REGR_COUNT","REGR_INTERCEPT","REGR_R2","REGR_SLOPE","REGR_SXX","REGR_SXY","REGR_SYY","RELEASE","RENAME","REPEAT","RESET","RESIGNAL","RESTART","RESTRICT","RESULT","RESULT_SET_LOCATOR","RETURN","RETURNS","REVOKE","RIGHT","ROLE","ROLLBACK","ROLLUP","ROUND_CEILING","ROUND_DOWN","ROUND_FLOOR","ROUND_HALF_DOWN","ROUND_HALF_EVEN","ROUND_HALF_UP","ROUND_UP","ROUTINE","ROW","ROWNUMBER","ROWS","ROWSET","ROW_NUMBER","RRN","RUN","SAVEPOINT","SCHEMA","SCOPE","SCRATCHPAD","SCROLL","SEARCH","SECOND","SECONDS","SECQTY","SECURITY","SENSITIVE","SEQUENCE","SESSION","SESSION_USER","SIGNAL","SIMILAR","SIMPLE","SMALLINT","SNAN","SOME","SOURCE","SPECIFIC","SPECIFICTYPE","SQL","SQLEXCEPTION","SQLID","SQLSTATE","SQLWARNING","SQRT","STACKED","STANDARD","START","STARTING","STATEMENT","STATIC","STATMENT","STAY","STDDEV_POP","STDDEV_SAMP","STOGROUP","STORES","STYLE","SUBMULTISET","SUBSTRING","SUM","SUMMARY","SYMMETRIC","SYNONYM","SYSFUN","SYSIBM","SYSPROC","SYSTEM","SYSTEM_USER","TABLE","TABLESAMPLE","TABLESPACE","THEN","TIME","TIMESTAMP","TIMEZONE_HOUR","TIMEZONE_MINUTE","TO","TRAILING","TRANSACTION","TRANSLATE","TRANSLATION","TREAT","TRIGGER","TRIM","TRUE","TRUNCATE","TYPE","UESCAPE","UNDO","UNIQUE","UNKNOWN","UNNEST","UNTIL","UPPER","USAGE","USER","USING","VALIDPROC","VALUE","VARCHAR","VARIABLE","VARIANT","VARYING","VAR_POP","VAR_SAMP","VCAT","VERSION","VIEW","VOLATILE","VOLUMES","WHEN","WHENEVER","WHILE","WIDTH_BUCKET","WINDOW","WITH","WITHIN","WITHOUT","WLM","WRITE","XMLELEMENT","XMLEXISTS","XMLNAMESPACES","YEAR","YEARS"],f=["ADD","AFTER","ALTER COLUMN","ALTER TABLE","DELETE FROM","EXCEPT","FETCH FIRST","FROM","GROUP BY","GO","HAVING","INSERT INTO","INTERSECT","LIMIT","ORDER BY","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","MINUS","UNION","UNION ALL"],p=["AND","OR","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"''","``","[]"],openParens:["("],closeParens:[")"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:[":"],lineCommentTypes:["--"],specialWordChars:["#","@"],operators:["**","!=","!>","!>","||"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),yc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ACCESSIBLE","ADD","ALL","ALTER","ANALYZE","AND","AS","ASC","ASENSITIVE","BEFORE","BETWEEN","BIGINT","BINARY","BLOB","BOTH","BY","CALL","CASCADE","CASE","CHANGE","CHAR","CHARACTER","CHECK","COLLATE","COLUMN","CONDITION","CONSTRAINT","CONTINUE","CONVERT","CREATE","CROSS","CURRENT_DATE","CURRENT_ROLE","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_USER","CURSOR","DATABASE","DATABASES","DAY_HOUR","DAY_MICROSECOND","DAY_MINUTE","DAY_SECOND","DEC","DECIMAL","DECLARE","DEFAULT","DELAYED","DELETE","DESC","DESCRIBE","DETERMINISTIC","DISTINCT","DISTINCTROW","DIV","DO_DOMAIN_IDS","DOUBLE","DROP","DUAL","EACH","ELSE","ELSEIF","ENCLOSED","ESCAPED","EXCEPT","EXISTS","EXIT","EXPLAIN","FALSE","FETCH","FLOAT","FLOAT4","FLOAT8","FOR","FORCE","FOREIGN","FROM","FULLTEXT","GENERAL","GRANT","GROUP","HAVING","HIGH_PRIORITY","HOUR_MICROSECOND","HOUR_MINUTE","HOUR_SECOND","IF","IGNORE","IGNORE_DOMAIN_IDS","IGNORE_SERVER_IDS","IN","INDEX","INFILE","INNER","INOUT","INSENSITIVE","INSERT","INT","INT1","INT2","INT3","INT4","INT8","INTEGER","INTERSECT","INTERVAL","INTO","IS","ITERATE","JOIN","KEY","KEYS","KILL","LEADING","LEAVE","LEFT","LIKE","LIMIT","LINEAR","LINES","LOAD","LOCALTIME","LOCALTIMESTAMP","LOCK","LONG","LONGBLOB","LONGTEXT","LOOP","LOW_PRIORITY","MASTER_HEARTBEAT_PERIOD","MASTER_SSL_VERIFY_SERVER_CERT","MATCH","MAXVALUE","MEDIUMBLOB","MEDIUMINT","MEDIUMTEXT","MIDDLEINT","MINUTE_MICROSECOND","MINUTE_SECOND","MOD","MODIFIES","NATURAL","NOT","NO_WRITE_TO_BINLOG","NULL","NUMERIC","ON","OPTIMIZE","OPTION","OPTIONALLY","OR","ORDER","OUT","OUTER","OUTFILE","OVER","PAGE_CHECKSUM","PARSE_VCOL_EXPR","PARTITION","POSITION","PRECISION","PRIMARY","PROCEDURE","PURGE","RANGE","READ","READS","READ_WRITE","REAL","RECURSIVE","REF_SYSTEM_ID","REFERENCES","REGEXP","RELEASE","RENAME","REPEAT","REPLACE","REQUIRE","RESIGNAL","RESTRICT","RETURN","RETURNING","REVOKE","RIGHT","RLIKE","ROWS","SCHEMA","SCHEMAS","SECOND_MICROSECOND","SELECT","SENSITIVE","SEPARATOR","SET","SHOW","SIGNAL","SLOW","SMALLINT","SPATIAL","SPECIFIC","SQL","SQLEXCEPTION","SQLSTATE","SQLWARNING","SQL_BIG_RESULT","SQL_CALC_FOUND_ROWS","SQL_SMALL_RESULT","SSL","STARTING","STATS_AUTO_RECALC","STATS_PERSISTENT","STATS_SAMPLE_PAGES","STRAIGHT_JOIN","TABLE","TERMINATED","THEN","TINYBLOB","TINYINT","TINYTEXT","TO","TRAILING","TRIGGER","TRUE","UNDO","UNION","UNIQUE","UNLOCK","UNSIGNED","UPDATE","USAGE","USE","USING","UTC_DATE","UTC_TIME","UTC_TIMESTAMP","VALUES","VARBINARY","VARCHAR","VARCHARACTER","VARYING","WHEN","WHERE","WHILE","WINDOW","WITH","WRITE","XOR","YEAR_MONTH","ZEROFILL"],f=["ADD","ALTER COLUMN","ALTER TABLE","DELETE FROM","EXCEPT","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","ORDER BY","SELECT","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","UNION","UNION ALL"],p=["AND","ELSE","OR","WHEN","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","CROSS JOIN","NATURAL JOIN","STRAIGHT_JOIN","NATURAL LEFT JOIN","NATURAL LEFT OUTER JOIN","NATURAL RIGHT JOIN","NATURAL RIGHT OUTER JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:["``","''",'""'],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:[],lineCommentTypes:["--","#"],specialWordChars:["@"],operators:[":=","<<",">>","!=","<>","<=>","&&","||"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),vc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ACCESSIBLE","ADD","ALL","ALTER","ANALYZE","AND","AS","ASC","ASENSITIVE","BEFORE","BETWEEN","BIGINT","BINARY","BLOB","BOTH","BY","CALL","CASCADE","CASE","CHANGE","CHAR","CHARACTER","CHECK","COLLATE","COLUMN","CONDITION","CONSTRAINT","CONTINUE","CONVERT","CREATE","CROSS","CUBE","CUME_DIST","CURRENT_DATE","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_USER","CURSOR","DATABASE","DATABASES","DAY_HOUR","DAY_MICROSECOND","DAY_MINUTE","DAY_SECOND","DEC","DECIMAL","DECLARE","DEFAULT","DELAYED","DELETE","DENSE_RANK","DESC","DESCRIBE","DETERMINISTIC","DISTINCT","DISTINCTROW","DIV","DOUBLE","DROP","DUAL","EACH","ELSE","ELSEIF","EMPTY","ENCLOSED","ESCAPED","EXCEPT","EXISTS","EXIT","EXPLAIN","FALSE","FETCH","FIRST_VALUE","FLOAT","FLOAT4","FLOAT8","FOR","FORCE","FOREIGN","FROM","FULLTEXT","FUNCTION","GENERATED","GET","GRANT","GROUP","GROUPING","GROUPS","HAVING","HIGH_PRIORITY","HOUR_MICROSECOND","HOUR_MINUTE","HOUR_SECOND","IF","IGNORE","IN","INDEX","INFILE","INNER","INOUT","INSENSITIVE","INSERT","INT","INT1","INT2","INT3","INT4","INT8","INTEGER","INTERVAL","INTO","IO_AFTER_GTIDS","IO_BEFORE_GTIDS","IS","ITERATE","JOIN","JSON_TABLE","KEY","KEYS","KILL","LAG","LAST_VALUE","LATERAL","LEAD","LEADING","LEAVE","LEFT","LIKE","LIMIT","LINEAR","LINES","LOAD","LOCALTIME","LOCALTIMESTAMP","LOCK","LONG","LONGBLOB","LONGTEXT","LOOP","LOW_PRIORITY","MASTER_BIND","MASTER_SSL_VERIFY_SERVER_CERT","MATCH","MAXVALUE","MEDIUMBLOB","MEDIUMINT","MEDIUMTEXT","MIDDLEINT","MINUTE_MICROSECOND","MINUTE_SECOND","MOD","MODIFIES","NATURAL","NOT","NO_WRITE_TO_BINLOG","NTH_VALUE","NTILE","NULL","NUMERIC","OF","ON","OPTIMIZE","OPTIMIZER_COSTS","OPTION","OPTIONALLY","OR","ORDER","OUT","OUTER","OUTFILE","OVER","PARTITION","PERCENT_RANK","PRECISION","PRIMARY","PROCEDURE","PURGE","RANGE","RANK","READ","READS","READ_WRITE","REAL","RECURSIVE","REFERENCES","REGEXP","RELEASE","RENAME","REPEAT","REPLACE","REQUIRE","RESIGNAL","RESTRICT","RETURN","REVOKE","RIGHT","RLIKE","ROW","ROWS","ROW_NUMBER","SCHEMA","SCHEMAS","SECOND_MICROSECOND","SELECT","SENSITIVE","SEPARATOR","SET","SHOW","SIGNAL","SMALLINT","SPATIAL","SPECIFIC","SQL","SQLEXCEPTION","SQLSTATE","SQLWARNING","SQL_BIG_RESULT","SQL_CALC_FOUND_ROWS","SQL_SMALL_RESULT","SSL","STARTING","STORED","STRAIGHT_JOIN","SYSTEM","TABLE","TERMINATED","THEN","TINYBLOB","TINYINT","TINYTEXT","TO","TRAILING","TRIGGER","TRUE","UNDO","UNION","UNIQUE","UNLOCK","UNSIGNED","UPDATE","USAGE","USE","USING","UTC_DATE","UTC_TIME","UTC_TIMESTAMP","VALUES","VARBINARY","VARCHAR","VARCHARACTER","VARYING","VIRTUAL","WHEN","WHERE","WHILE","WINDOW","WITH","WRITE","XOR","YEAR_MONTH","ZEROFILL"],f=["ADD","ALTER COLUMN","ALTER TABLE","DELETE FROM","EXCEPT","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","ORDER BY","SELECT","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","UNION","UNION ALL"],p=["AND","ELSE","OR","WHEN","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","CROSS JOIN","NATURAL JOIN","STRAIGHT_JOIN","NATURAL LEFT JOIN","NATURAL LEFT OUTER JOIN","NATURAL RIGHT JOIN","NATURAL RIGHT OUTER JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:["``","''",'""'],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:[],lineCommentTypes:["--","#"],specialWordChars:["@"],operators:[":=","<<",">>","!=","<>","<=>","&&","||","->","->>"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),bc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ALL","ALTER","ANALYZE","AND","ANY","ARRAY","AS","ASC","BEGIN","BETWEEN","BINARY","BOOLEAN","BREAK","BUCKET","BUILD","BY","CALL","CASE","CAST","CLUSTER","COLLATE","COLLECTION","COMMIT","CONNECT","CONTINUE","CORRELATE","COVER","CREATE","DATABASE","DATASET","DATASTORE","DECLARE","DECREMENT","DELETE","DERIVED","DESC","DESCRIBE","DISTINCT","DO","DROP","EACH","ELEMENT","ELSE","END","EVERY","EXCEPT","EXCLUDE","EXECUTE","EXISTS","EXPLAIN","FALSE","FETCH","FIRST","FLATTEN","FOR","FORCE","FROM","FUNCTION","GRANT","GROUP","GSI","HAVING","IF","IGNORE","ILIKE","IN","INCLUDE","INCREMENT","INDEX","INFER","INLINE","INNER","INSERT","INTERSECT","INTO","IS","JOIN","KEY","KEYS","KEYSPACE","KNOWN","LAST","LEFT","LET","LETTING","LIKE","LIMIT","LSM","MAP","MAPPING","MATCHED","MATERIALIZED","MERGE","MISSING","NAMESPACE","NEST","NOT","NULL","NUMBER","OBJECT","OFFSET","ON","OPTION","OR","ORDER","OUTER","OVER","PARSE","PARTITION","PASSWORD","PATH","POOL","PREPARE","PRIMARY","PRIVATE","PRIVILEGE","PROCEDURE","PUBLIC","RAW","REALM","REDUCE","RENAME","RETURN","RETURNING","REVOKE","RIGHT","ROLE","ROLLBACK","SATISFIES","SCHEMA","SELECT","SELF","SEMI","SET","SHOW","SOME","START","STATISTICS","STRING","SYSTEM","THEN","TO","TRANSACTION","TRIGGER","TRUE","TRUNCATE","UNDER","UNION","UNIQUE","UNKNOWN","UNNEST","UNSET","UPDATE","UPSERT","USE","USER","USING","VALIDATE","VALUE","VALUED","VALUES","VIA","VIEW","WHEN","WHERE","WHILE","WITH","WITHIN","WORK","XOR"],f=["DELETE FROM","EXCEPT ALL","EXCEPT","EXPLAIN DELETE FROM","EXPLAIN UPDATE","EXPLAIN UPSERT","FROM","GROUP BY","HAVING","INFER","INSERT INTO","LET","LIMIT","MERGE","NEST","ORDER BY","PREPARE","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","UNNEST","UPDATE","UPSERT","USE KEYS","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","MINUS","UNION","UNION ALL"],p=["AND","OR","XOR","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"''","``"],openParens:["(","[","{"],closeParens:[")","]","}"],namedPlaceholderTypes:["$"],lineCommentTypes:["#","--"],operators:["==","!="]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),Ec=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=i(fc),a=i(hc),o=i(oc);function i(e){return e&&e.__esModule?e:{default:e}}function l(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function s(e,t){return(s=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function c(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function u(e){return(u=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var f=["A","ACCESSIBLE","AGENT","AGGREGATE","ALL","ALTER","ANY","ARRAY","AS","ASC","AT","ATTRIBUTE","AUTHID","AVG","BETWEEN","BFILE_BASE","BINARY_INTEGER","BINARY","BLOB_BASE","BLOCK","BODY","BOOLEAN","BOTH","BOUND","BREADTH","BULK","BY","BYTE","C","CALL","CALLING","CASCADE","CASE","CHAR_BASE","CHAR","CHARACTER","CHARSET","CHARSETFORM","CHARSETID","CHECK","CLOB_BASE","CLONE","CLOSE","CLUSTER","CLUSTERS","COALESCE","COLAUTH","COLLECT","COLUMNS","COMMENT","COMMIT","COMMITTED","COMPILED","COMPRESS","CONNECT","CONSTANT","CONSTRUCTOR","CONTEXT","CONTINUE","CONVERT","COUNT","CRASH","CREATE","CREDENTIAL","CURRENT","CURRVAL","CURSOR","CUSTOMDATUM","DANGLING","DATA","DATE_BASE","DATE","DAY","DECIMAL","DEFAULT","DEFINE","DELETE","DEPTH","DESC","DETERMINISTIC","DIRECTORY","DISTINCT","DO","DOUBLE","DROP","DURATION","ELEMENT","ELSIF","EMPTY","END","ESCAPE","EXCEPTIONS","EXCLUSIVE","EXECUTE","EXISTS","EXIT","EXTENDS","EXTERNAL","EXTRACT","FALSE","FETCH","FINAL","FIRST","FIXED","FLOAT","FOR","FORALL","FORCE","FROM","FUNCTION","GENERAL","GOTO","GRANT","GROUP","HASH","HEAP","HIDDEN","HOUR","IDENTIFIED","IF","IMMEDIATE","IN","INCLUDING","INDEX","INDEXES","INDICATOR","INDICES","INFINITE","INSTANTIABLE","INT","INTEGER","INTERFACE","INTERVAL","INTO","INVALIDATE","IS","ISOLATION","JAVA","LANGUAGE","LARGE","LEADING","LENGTH","LEVEL","LIBRARY","LIKE","LIKE2","LIKE4","LIKEC","LIMITED","LOCAL","LOCK","LONG","MAP","MAX","MAXLEN","MEMBER","MERGE","MIN","MINUTE","MLSLABEL","MOD","MODE","MONTH","MULTISET","NAME","NAN","NATIONAL","NATIVE","NATURAL","NATURALN","NCHAR","NEW","NEXTVAL","NOCOMPRESS","NOCOPY","NOT","NOWAIT","NULL","NULLIF","NUMBER_BASE","NUMBER","OBJECT","OCICOLL","OCIDATE","OCIDATETIME","OCIDURATION","OCIINTERVAL","OCILOBLOCATOR","OCINUMBER","OCIRAW","OCIREF","OCIREFCURSOR","OCIROWID","OCISTRING","OCITYPE","OF","OLD","ON","ONLY","OPAQUE","OPEN","OPERATOR","OPTION","ORACLE","ORADATA","ORDER","ORGANIZATION","ORLANY","ORLVARY","OTHERS","OUT","OVERLAPS","OVERRIDING","PACKAGE","PARALLEL_ENABLE","PARAMETER","PARAMETERS","PARENT","PARTITION","PASCAL","PCTFREE","PIPE","PIPELINED","PLS_INTEGER","PLUGGABLE","POSITIVE","POSITIVEN","PRAGMA","PRECISION","PRIOR","PRIVATE","PROCEDURE","PUBLIC","RAISE","RANGE","RAW","READ","REAL","RECORD","REF","REFERENCE","RELEASE","RELIES_ON","REM","REMAINDER","RENAME","RESOURCE","RESULT_CACHE","RESULT","RETURN","RETURNING","REVERSE","REVOKE","ROLLBACK","ROW","ROWID","ROWNUM","ROWTYPE","SAMPLE","SAVE","SAVEPOINT","SB1","SB2","SB4","SEARCH","SECOND","SEGMENT","SELF","SEPARATE","SEQUENCE","SERIALIZABLE","SHARE","SHORT","SIZE_T","SIZE","SMALLINT","SOME","SPACE","SPARSE","SQL","SQLCODE","SQLDATA","SQLERRM","SQLNAME","SQLSTATE","STANDARD","START","STATIC","STDDEV","STORED","STRING","STRUCT","STYLE","SUBMULTISET","SUBPARTITION","SUBSTITUTABLE","SUBTYPE","SUCCESSFUL","SUM","SYNONYM","SYSDATE","TABAUTH","TABLE","TDO","THE","THEN","TIME","TIMESTAMP","TIMEZONE_ABBR","TIMEZONE_HOUR","TIMEZONE_MINUTE","TIMEZONE_REGION","TO","TRAILING","TRANSACTION","TRANSACTIONAL","TRIGGER","TRUE","TRUSTED","TYPE","UB1","UB2","UB4","UID","UNDER","UNIQUE","UNPLUG","UNSIGNED","UNTRUSTED","USE","USER","USING","VALIDATE","VALIST","VALUE","VARCHAR","VARCHAR2","VARIABLE","VARIANCE","VARRAY","VARYING","VIEW","VIEWS","VOID","WHENEVER","WHILE","WITH","WORK","WRAPPED","WRITE","YEAR","ZONE"],d=["ADD","ALTER COLUMN","ALTER TABLE","BEGIN","CONNECT BY","DECLARE","DELETE FROM","DELETE","END","EXCEPT","EXCEPTION","FETCH FIRST","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","LOOP","MODIFY","ORDER BY","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","START WITH","UPDATE","VALUES","WHERE"],p=["INTERSECT","INTERSECT ALL","MINUS","UNION","UNION ALL"],m=["AND","CROSS APPLY","ELSE","END","OR","OUTER APPLY","WHEN","XOR","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&s(e,t)}(h,e);var t,n,r,i=(n=h,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=u(n);if(r){var a=u(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return c(this,e)});function h(){return l(this,h),i.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:f,reservedTopLevelWords:d,reservedNewlineWords:m,reservedTopLevelWordsNoIndent:p,stringTypes:['""',"N''","''","``"],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:[":"],lineCommentTypes:["--"],specialWordChars:["_","$","#",".","@"],operators:["||","**","!=",":="]})}},{key:"tokenOverride",value:function(e){return(0,uc.isSet)(e)&&(0,uc.isBy)(this.previousReservedToken)?{type:o.default.RESERVED,value:e.value}:e}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(h.prototype,t),h}(r.default),e.exports=t.default}),Tc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ABORT","ABSOLUTE","ACCESS","ACTION","ADD","ADMIN","AFTER","AGGREGATE","ALL","ALSO","ALTER","ALWAYS","ANALYSE","ANALYZE","AND","ANY","ARRAY","AS","ASC","ASSERTION","ASSIGNMENT","ASYMMETRIC","AT","ATTACH","ATTRIBUTE","AUTHORIZATION","BACKWARD","BEFORE","BEGIN","BETWEEN","BIGINT","BINARY","BIT","BOOLEAN","BOTH","BY","CACHE","CALL","CALLED","CASCADE","CASCADED","CASE","CAST","CATALOG","CHAIN","CHAR","CHARACTER","CHARACTERISTICS","CHECK","CHECKPOINT","CLASS","CLOSE","CLUSTER","COALESCE","COLLATE","COLLATION","COLUMN","COLUMNS","COMMENT","COMMENTS","COMMIT","COMMITTED","CONCURRENTLY","CONFIGURATION","CONFLICT","CONNECTION","CONSTRAINT","CONSTRAINTS","CONTENT","CONTINUE","CONVERSION","COPY","COST","CREATE","CROSS","CSV","CUBE","CURRENT","CURRENT_CATALOG","CURRENT_DATE","CURRENT_ROLE","CURRENT_SCHEMA","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_USER","CURSOR","CYCLE","DATA","DATABASE","DAY","DEALLOCATE","DEC","DECIMAL","DECLARE","DEFAULT","DEFAULTS","DEFERRABLE","DEFERRED","DEFINER","DELETE","DELIMITER","DELIMITERS","DEPENDS","DESC","DETACH","DICTIONARY","DISABLE","DISCARD","DISTINCT","DO","DOCUMENT","DOMAIN","DOUBLE","DROP","EACH","ELSE","ENABLE","ENCODING","ENCRYPTED","END","ENUM","ESCAPE","EVENT","EXCEPT","EXCLUDE","EXCLUDING","EXCLUSIVE","EXECUTE","EXISTS","EXPLAIN","EXPRESSION","EXTENSION","EXTERNAL","EXTRACT","FALSE","FAMILY","FETCH","FILTER","FIRST","FLOAT","FOLLOWING","FOR","FORCE","FOREIGN","FORWARD","FREEZE","FROM","FULL","FUNCTION","FUNCTIONS","GENERATED","GLOBAL","GRANT","GRANTED","GREATEST","GROUP","GROUPING","GROUPS","HANDLER","HAVING","HEADER","HOLD","HOUR","IDENTITY","IF","ILIKE","IMMEDIATE","IMMUTABLE","IMPLICIT","IMPORT","IN","INCLUDE","INCLUDING","INCREMENT","INDEX","INDEXES","INHERIT","INHERITS","INITIALLY","INLINE","INNER","INOUT","INPUT","INSENSITIVE","INSERT","INSTEAD","INT","INTEGER","INTERSECT","INTERVAL","INTO","INVOKER","IS","ISNULL","ISOLATION","JOIN","KEY","LABEL","LANGUAGE","LARGE","LAST","LATERAL","LEADING","LEAKPROOF","LEAST","LEFT","LEVEL","LIKE","LIMIT","LISTEN","LOAD","LOCAL","LOCALTIME","LOCALTIMESTAMP","LOCATION","LOCK","LOCKED","LOGGED","MAPPING","MATCH","MATERIALIZED","MAXVALUE","METHOD","MINUTE","MINVALUE","MODE","MONTH","MOVE","NAME","NAMES","NATIONAL","NATURAL","NCHAR","NEW","NEXT","NFC","NFD","NFKC","NFKD","NO","NONE","NORMALIZE","NORMALIZED","NOT","NOTHING","NOTIFY","NOTNULL","NOWAIT","NULL","NULLIF","NULLS","NUMERIC","OBJECT","OF","OFF","OFFSET","OIDS","OLD","ON","ONLY","OPERATOR","OPTION","OPTIONS","OR","ORDER","ORDINALITY","OTHERS","OUT","OUTER","OVER","OVERLAPS","OVERLAY","OVERRIDING","OWNED","OWNER","PARALLEL","PARSER","PARTIAL","PARTITION","PASSING","PASSWORD","PLACING","PLANS","POLICY","POSITION","PRECEDING","PRECISION","PREPARE","PREPARED","PRESERVE","PRIMARY","PRIOR","PRIVILEGES","PROCEDURAL","PROCEDURE","PROCEDURES","PROGRAM","PUBLICATION","QUOTE","RANGE","READ","REAL","REASSIGN","RECHECK","RECURSIVE","REF","REFERENCES","REFERENCING","REFRESH","REINDEX","RELATIVE","RELEASE","RENAME","REPEATABLE","REPLACE","REPLICA","RESET","RESTART","RESTRICT","RETURNING","RETURNS","REVOKE","RIGHT","ROLE","ROLLBACK","ROLLUP","ROUTINE","ROUTINES","ROW","ROWS","RULE","SAVEPOINT","SCHEMA","SCHEMAS","SCROLL","SEARCH","SECOND","SECURITY","SELECT","SEQUENCE","SEQUENCES","SERIALIZABLE","SERVER","SESSION","SESSION_USER","SET","SETOF","SETS","SHARE","SHOW","SIMILAR","SIMPLE","SKIP","SMALLINT","SNAPSHOT","SOME","SQL","STABLE","STANDALONE","START","STATEMENT","STATISTICS","STDIN","STDOUT","STORAGE","STORED","STRICT","STRIP","SUBSCRIPTION","SUBSTRING","SUPPORT","SYMMETRIC","SYSID","SYSTEM","TABLE","TABLES","TABLESAMPLE","TABLESPACE","TEMP","TEMPLATE","TEMPORARY","TEXT","THEN","TIES","TIME","TIMESTAMP","TO","TRAILING","TRANSACTION","TRANSFORM","TREAT","TRIGGER","TRIM","TRUE","TRUNCATE","TRUSTED","TYPE","TYPES","UESCAPE","UNBOUNDED","UNCOMMITTED","UNENCRYPTED","UNION","UNIQUE","UNKNOWN","UNLISTEN","UNLOGGED","UNTIL","UPDATE","USER","USING","VACUUM","VALID","VALIDATE","VALIDATOR","VALUE","VALUES","VARCHAR","VARIADIC","VARYING","VERBOSE","VERSION","VIEW","VIEWS","VOLATILE","WHEN","WHERE","WHITESPACE","WINDOW","WITH","WITHIN","WITHOUT","WORK","WRAPPER","WRITE","XML","XMLATTRIBUTES","XMLCONCAT","XMLELEMENT","XMLEXISTS","XMLFOREST","XMLNAMESPACES","XMLPARSE","XMLPI","XMLROOT","XMLSERIALIZE","XMLTABLE","YEAR","YES","ZONE"],f=["ADD","AFTER","ALTER COLUMN","ALTER TABLE","CASE","DELETE FROM","END","EXCEPT","FETCH FIRST","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","ORDER BY","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","UNION","UNION ALL"],p=["AND","ELSE","OR","WHEN","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"''","U&''",'U&""',"$$"],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["$"],namedPlaceholderTypes:[":"],lineCommentTypes:["--"],operators:["!=","<<",">>","||/","|/","::","->>","->","~~*","~~","!~~*","!~~","~*","!~*","!~","!!"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),Sc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["AES128","AES256","ALLOWOVERWRITE","ANALYSE","ARRAY","AS","ASC","AUTHORIZATION","BACKUP","BINARY","BLANKSASNULL","BOTH","BYTEDICT","BZIP2","CAST","CHECK","COLLATE","COLUMN","CONSTRAINT","CREATE","CREDENTIALS","CURRENT_DATE","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_USER","CURRENT_USER_ID","DEFAULT","DEFERRABLE","DEFLATE","DEFRAG","DELTA","DELTA32K","DESC","DISABLE","DISTINCT","DO","ELSE","EMPTYASNULL","ENABLE","ENCODE","ENCRYPT","ENCRYPTION","END","EXPLICIT","FALSE","FOR","FOREIGN","FREEZE","FULL","GLOBALDICT256","GLOBALDICT64K","GRANT","GZIP","IDENTITY","IGNORE","ILIKE","INITIALLY","INTO","LEADING","LOCALTIME","LOCALTIMESTAMP","LUN","LUNS","LZO","LZOP","MINUS","MOSTLY13","MOSTLY32","MOSTLY8","NATURAL","NEW","NULLS","OFF","OFFLINE","OFFSET","OLD","ON","ONLY","OPEN","ORDER","OVERLAPS","PARALLEL","PARTITION","PERCENT","PERMISSIONS","PLACING","PRIMARY","RAW","READRATIO","RECOVER","REFERENCES","REJECTLOG","RESORT","RESTORE","SESSION_USER","SIMILAR","SYSDATE","SYSTEM","TABLE","TAG","TDES","TEXT255","TEXT32K","THEN","TIMESTAMP","TO","TOP","TRAILING","TRUE","TRUNCATECOLUMNS","UNIQUE","USER","USING","VERBOSE","WALLET","WHEN","WITH","WITHOUT","PREDICATE","COLUMNS","COMPROWS","COMPRESSION","COPY","FORMAT","DELIMITER","FIXEDWIDTH","AVRO","JSON","ENCRYPTED","BZIP2","GZIP","LZOP","PARQUET","ORC","ACCEPTANYDATE","ACCEPTINVCHARS","BLANKSASNULL","DATEFORMAT","EMPTYASNULL","ENCODING","ESCAPE","EXPLICIT_IDS","FILLRECORD","IGNOREBLANKLINES","IGNOREHEADER","NULL AS","REMOVEQUOTES","ROUNDEC","TIMEFORMAT","TRIMBLANKS","TRUNCATECOLUMNS","COMPROWS","COMPUPDATE","MAXERROR","NOLOAD","STATUPDATE","MANIFEST","REGION","IAM_ROLE","MASTER_SYMMETRIC_KEY","SSH","ACCEPTANYDATE","ACCEPTINVCHARS","ACCESS_KEY_ID","SECRET_ACCESS_KEY","AVRO","BLANKSASNULL","BZIP2","COMPROWS","COMPUPDATE","CREDENTIALS","DATEFORMAT","DELIMITER","EMPTYASNULL","ENCODING","ENCRYPTED","ESCAPE","EXPLICIT_IDS","FILLRECORD","FIXEDWIDTH","FORMAT","IAM_ROLE","GZIP","IGNOREBLANKLINES","IGNOREHEADER","JSON","LZOP","MANIFEST","MASTER_SYMMETRIC_KEY","MAXERROR","NOLOAD","NULL AS","READRATIO","REGION","REMOVEQUOTES","ROUNDEC","SSH","STATUPDATE","TIMEFORMAT","SESSION_TOKEN","TRIMBLANKS","TRUNCATECOLUMNS","EXTERNAL","DATA CATALOG","HIVE METASTORE","CATALOG_ROLE","VACUUM","COPY","UNLOAD","EVEN","ALL"],f=["ADD","AFTER","ALTER COLUMN","ALTER TABLE","DELETE FROM","EXCEPT","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","INTERSECT","TOP","LIMIT","MODIFY","ORDER BY","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","UNION ALL","UNION","UPDATE","VALUES","WHERE","VACUUM","COPY","UNLOAD","ANALYZE","ANALYSE","DISTKEY","SORTKEY","COMPOUND","INTERLEAVED","FORMAT","DELIMITER","FIXEDWIDTH","AVRO","JSON","ENCRYPTED","BZIP2","GZIP","LZOP","PARQUET","ORC","ACCEPTANYDATE","ACCEPTINVCHARS","BLANKSASNULL","DATEFORMAT","EMPTYASNULL","ENCODING","ESCAPE","EXPLICIT_IDS","FILLRECORD","IGNOREBLANKLINES","IGNOREHEADER","NULL AS","REMOVEQUOTES","ROUNDEC","TIMEFORMAT","TRIMBLANKS","TRUNCATECOLUMNS","COMPROWS","COMPUPDATE","MAXERROR","NOLOAD","STATUPDATE","MANIFEST","REGION","IAM_ROLE","MASTER_SYMMETRIC_KEY","SSH","ACCEPTANYDATE","ACCEPTINVCHARS","ACCESS_KEY_ID","SECRET_ACCESS_KEY","AVRO","BLANKSASNULL","BZIP2","COMPROWS","COMPUPDATE","CREDENTIALS","DATEFORMAT","DELIMITER","EMPTYASNULL","ENCODING","ENCRYPTED","ESCAPE","EXPLICIT_IDS","FILLRECORD","FIXEDWIDTH","FORMAT","IAM_ROLE","GZIP","IGNOREBLANKLINES","IGNOREHEADER","JSON","LZOP","MANIFEST","MASTER_SYMMETRIC_KEY","MAXERROR","NOLOAD","NULL AS","READRATIO","REGION","REMOVEQUOTES","ROUNDEC","SSH","STATUPDATE","TIMEFORMAT","SESSION_TOKEN","TRIMBLANKS","TRUNCATECOLUMNS","EXTERNAL","DATA CATALOG","HIVE METASTORE","CATALOG_ROLE"],d=[],p=["AND","ELSE","OR","OUTER APPLY","WHEN","VACUUM","COPY","UNLOAD","ANALYZE","ANALYSE","DISTKEY","SORTKEY","COMPOUND","INTERLEAVED","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"''","``"],openParens:["("],closeParens:[")"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:["@","#","$"],lineCommentTypes:["--"],operators:["|/","||/","<<",">>","!=","||"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),wc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=i(fc),a=i(hc),o=i(oc);function i(e){return e&&e.__esModule?e:{default:e}}function l(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function s(e,t){return(s=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function c(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function u(e){return(u=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var f=["ALL","ALTER","ANALYSE","ANALYZE","ARRAY_ZIP","ARRAY","AS","ASC","AVG","BETWEEN","CASCADE","CASE","CAST","COALESCE","COLLECT_LIST","COLLECT_SET","COLUMN","COLUMNS","COMMENT","CONSTRAINT","CONTAINS","CONVERT","COUNT","CUME_DIST","CURRENT ROW","CURRENT_DATE","CURRENT_TIMESTAMP","DATABASE","DATABASES","DATE_ADD","DATE_SUB","DATE_TRUNC","DAY_HOUR","DAY_MINUTE","DAY_SECOND","DAY","DAYS","DECODE","DEFAULT","DELETE","DENSE_RANK","DESC","DESCRIBE","DISTINCT","DISTINCTROW","DIV","DROP","ELSE","ENCODE","END","EXISTS","EXPLAIN","EXPLODE_OUTER","EXPLODE","FILTER","FIRST_VALUE","FIRST","FIXED","FLATTEN","FOLLOWING","FROM_UNIXTIME","FULL","GREATEST","GROUP_CONCAT","HOUR_MINUTE","HOUR_SECOND","HOUR","HOURS","IF","IFNULL","IN","INSERT","INTERVAL","INTO","IS","LAG","LAST_VALUE","LAST","LEAD","LEADING","LEAST","LEVEL","LIKE","MAX","MERGE","MIN","MINUTE_SECOND","MINUTE","MONTH","NATURAL","NOT","NOW()","NTILE","NULL","NULLIF","OFFSET","ON DELETE","ON UPDATE","ON","ONLY","OPTIMIZE","OVER","PERCENT_RANK","PRECEDING","RANGE","RANK","REGEXP","RENAME","RLIKE","ROW","ROWS","SECOND","SEPARATOR","SEQUENCE","SIZE","STRING","STRUCT","SUM","TABLE","TABLES","TEMPORARY","THEN","TO_DATE","TO_JSON","TO","TRAILING","TRANSFORM","TRUE","TRUNCATE","TYPE","TYPES","UNBOUNDED","UNIQUE","UNIX_TIMESTAMP","UNLOCK","UNSIGNED","USING","VARIABLES","VIEW","WHEN","WITH","YEAR_MONTH"],d=["ADD","AFTER","ALTER COLUMN","ALTER DATABASE","ALTER SCHEMA","ALTER TABLE","CLUSTER BY","CLUSTERED BY","DELETE FROM","DISTRIBUTE BY","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","OPTIONS","ORDER BY","PARTITION BY","PARTITIONED BY","RANGE","ROWS","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","TBLPROPERTIES","UPDATE","USING","VALUES","WHERE","WINDOW"],p=["EXCEPT ALL","EXCEPT","INTERSECT ALL","INTERSECT","UNION ALL","UNION"],m=["AND","CREATE OR","CREATE","ELSE","LATERAL VIEW","OR","OUTER APPLY","WHEN","XOR","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN","ANTI JOIN","SEMI JOIN","LEFT ANTI JOIN","LEFT SEMI JOIN","RIGHT OUTER JOIN","RIGHT SEMI JOIN","NATURAL ANTI JOIN","NATURAL FULL OUTER JOIN","NATURAL INNER JOIN","NATURAL LEFT ANTI JOIN","NATURAL LEFT OUTER JOIN","NATURAL LEFT SEMI JOIN","NATURAL OUTER JOIN","NATURAL RIGHT OUTER JOIN","NATURAL RIGHT SEMI JOIN","NATURAL SEMI JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&s(e,t)}(h,e);var t,n,r,i=(n=h,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=u(n);if(r){var a=u(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return c(this,e)});function h(){return l(this,h),i.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:f,reservedTopLevelWords:d,reservedNewlineWords:m,reservedTopLevelWordsNoIndent:p,stringTypes:['""',"''","``","{}"],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:["$"],lineCommentTypes:["--"],operators:["!=","<=>","&&","||","=="]})}},{key:"tokenOverride",value:function(e){if((0,uc.isWindow)(e)){var t=this.tokenLookAhead();if(t&&t.type===o.default.OPEN_PAREN)return{type:o.default.RESERVED,value:e.value}}if((0,uc.isEnd)(e)){var n=this.tokenLookBehind();if(n&&n.type===o.default.OPERATOR&&"."===n.value)return{type:o.default.WORD,value:e.value}}return e}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(h.prototype,t),h}(r.default),e.exports=t.default}),Nc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ABS","ALL","ALLOCATE","ALTER","AND","ANY","ARE","ARRAY","AS","ASENSITIVE","ASYMMETRIC","AT","ATOMIC","AUTHORIZATION","AVG","BEGIN","BETWEEN","BIGINT","BINARY","BLOB","BOOLEAN","BOTH","BY","CALL","CALLED","CARDINALITY","CASCADED","CASE","CAST","CEIL","CEILING","CHAR","CHAR_LENGTH","CHARACTER","CHARACTER_LENGTH","CHECK","CLOB","CLOSE","COALESCE","COLLATE","COLLECT","COLUMN","COMMIT","CONDITION","CONNECT","CONSTRAINT","CONVERT","CORR","CORRESPONDING","COUNT","COVAR_POP","COVAR_SAMP","CREATE","CROSS","CUBE","CUME_DIST","CURRENT","CURRENT_CATALOG","CURRENT_DATE","CURRENT_DEFAULT_TRANSFORM_GROUP","CURRENT_PATH","CURRENT_ROLE","CURRENT_SCHEMA","CURRENT_TIME","CURRENT_TIMESTAMP","CURRENT_TRANSFORM_GROUP_FOR_TYPE","CURRENT_USER","CURSOR","CYCLE","DATE","DAY","DEALLOCATE","DEC","DECIMAL","DECLARE","DEFAULT","DELETE","DENSE_RANK","DEREF","DESCRIBE","DETERMINISTIC","DISCONNECT","DISTINCT","DOUBLE","DROP","DYNAMIC","EACH","ELEMENT","ELSE","END","END-EXEC","ESCAPE","EVERY","EXCEPT","EXEC","EXECUTE","EXISTS","EXP","EXTERNAL","EXTRACT","FALSE","FETCH","FILTER","FLOAT","FLOOR","FOR","FOREIGN","FREE","FROM","FULL","FUNCTION","FUSION","GET","GLOBAL","GRANT","GROUP","GROUPING","HAVING","HOLD","HOUR","IDENTITY","IN","INDICATOR","INNER","INOUT","INSENSITIVE","INSERT","INT","INTEGER","INTERSECT","INTERSECTION","INTERVAL","INTO","IS","JOIN","LANGUAGE","LARGE","LATERAL","LEADING","LEFT","LIKE","LIKE_REGEX","LN","LOCAL","LOCALTIME","LOCALTIMESTAMP","LOWER","MATCH","MAX","MEMBER","MERGE","METHOD","MIN","MINUTE","MOD","MODIFIES","MODULE","MONTH","MULTISET","NATIONAL","NATURAL","NCHAR","NCLOB","NEW","NO","NONE","NORMALIZE","NOT","NULL","NULLIF","NUMERIC","OCTET_LENGTH","OCCURRENCES_REGEX","OF","OLD","ON","ONLY","OPEN","OR","ORDER","OUT","OUTER","OVER","OVERLAPS","OVERLAY","PARAMETER","PARTITION","PERCENT_RANK","PERCENTILE_CONT","PERCENTILE_DISC","POSITION","POSITION_REGEX","POWER","PRECISION","PREPARE","PRIMARY","PROCEDURE","RANGE","RANK","READS","REAL","RECURSIVE","REF","REFERENCES","REFERENCING","REGR_AVGX","REGR_AVGY","REGR_COUNT","REGR_INTERCEPT","REGR_R2","REGR_SLOPE","REGR_SXX","REGR_SXY","REGR_SYY","RELEASE","RESULT","RETURN","RETURNS","REVOKE","RIGHT","ROLLBACK","ROLLUP","ROW","ROW_NUMBER","ROWS","SAVEPOINT","SCOPE","SCROLL","SEARCH","SECOND","SELECT","SENSITIVE","SESSION_USER","SET","SIMILAR","SMALLINT","SOME","SPECIFIC","SPECIFICTYPE","SQL","SQLEXCEPTION","SQLSTATE","SQLWARNING","SQRT","START","STATIC","STDDEV_POP","STDDEV_SAMP","SUBMULTISET","SUBSTRING","SUBSTRING_REGEX","SUM","SYMMETRIC","SYSTEM","SYSTEM_USER","TABLE","TABLESAMPLE","THEN","TIME","TIMESTAMP","TIMEZONE_HOUR","TIMEZONE_MINUTE","TO","TRAILING","TRANSLATE","TRANSLATE_REGEX","TRANSLATION","TREAT","TRIGGER","TRIM","TRUE","UESCAPE","UNION","UNIQUE","UNKNOWN","UNNEST","UPDATE","UPPER","USER","USING","VALUE","VALUES","VAR_POP","VAR_SAMP","VARBINARY","VARCHAR","VARYING","WHEN","WHENEVER","WHERE","WIDTH_BUCKET","WINDOW","WITH","WITHIN","WITHOUT","YEAR"],f=["ADD","ALTER COLUMN","ALTER TABLE","CASE","DELETE FROM","END","FETCH FIRST","FETCH NEXT","FETCH PRIOR","FETCH LAST","FETCH ABSOLUTE","FETCH RELATIVE","FROM","GROUP BY","HAVING","INSERT INTO","LIMIT","ORDER BY","SELECT","SET SCHEMA","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","INTERSECT DISTINCT","UNION","UNION ALL","UNION DISTINCT","EXCEPT","EXCEPT ALL","EXCEPT DISTINCT"],p=["AND","ELSE","OR","WHEN","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN","NATURAL JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"''"],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:["?"],namedPlaceholderTypes:[],lineCommentTypes:["--"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),Rc=Le(function(e,t){function n(e){return(n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}Object.defineProperty(t,"__esModule",{value:!0}),t.default=void 0;var r=o(fc),a=o(hc);function o(e){return e&&e.__esModule?e:{default:e}}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function l(e,t){return(l=Object.setPrototypeOf||function(e,t){return e.__proto__=t,e})(e,t)}function s(e,t){return!t||"object"!==n(t)&&"function"!=typeof t?function(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}(e):t}function c(e){return(c=Object.setPrototypeOf?Object.getPrototypeOf:function(e){return e.__proto__||Object.getPrototypeOf(e)})(e)}var u=["ADD","EXTERNAL","PROCEDURE","ALL","FETCH","PUBLIC","ALTER","FILE","RAISERROR","AND","FILLFACTOR","READ","ANY","FOR","READTEXT","AS","FOREIGN","RECONFIGURE","ASC","FREETEXT","REFERENCES","AUTHORIZATION","FREETEXTTABLE","REPLICATION","BACKUP","FROM","RESTORE","BEGIN","FULL","RESTRICT","BETWEEN","FUNCTION","RETURN","BREAK","GOTO","REVERT","BROWSE","GRANT","REVOKE","BULK","GROUP","RIGHT","BY","HAVING","ROLLBACK","CASCADE","HOLDLOCK","ROWCOUNT","CASE","IDENTITY","ROWGUIDCOL","CHECK","IDENTITY_INSERT","RULE","CHECKPOINT","IDENTITYCOL","SAVE","CLOSE","IF","SCHEMA","CLUSTERED","IN","SECURITYAUDIT","COALESCE","INDEX","SELECT","COLLATE","INNER","SEMANTICKEYPHRASETABLE","COLUMN","INSERT","SEMANTICSIMILARITYDETAILSTABLE","COMMIT","INTERSECT","SEMANTICSIMILARITYTABLE","COMPUTE","INTO","SESSION_USER","CONSTRAINT","IS","SET","CONTAINS","JOIN","SETUSER","CONTAINSTABLE","KEY","SHUTDOWN","CONTINUE","KILL","SOME","CONVERT","LEFT","STATISTICS","CREATE","LIKE","SYSTEM_USER","CROSS","LINENO","TABLE","CURRENT","LOAD","TABLESAMPLE","CURRENT_DATE","MERGE","TEXTSIZE","CURRENT_TIME","NATIONAL","THEN","CURRENT_TIMESTAMP","NOCHECK","TO","CURRENT_USER","NONCLUSTERED","TOP","CURSOR","NOT","TRAN","DATABASE","NULL","TRANSACTION","DBCC","NULLIF","TRIGGER","DEALLOCATE","OF","TRUNCATE","DECLARE","OFF","TRY_CONVERT","DEFAULT","OFFSETS","TSEQUAL","DELETE","ON","UNION","DENY","OPEN","UNIQUE","DESC","OPENDATASOURCE","UNPIVOT","DISK","OPENQUERY","UPDATE","DISTINCT","OPENROWSET","UPDATETEXT","DISTRIBUTED","OPENXML","USE","DOUBLE","OPTION","USER","DROP","OR","VALUES","DUMP","ORDER","VARYING","ELSE","OUTER","VIEW","END","OVER","WAITFOR","ERRLVL","PERCENT","WHEN","ESCAPE","PIVOT","WHERE","EXCEPT","PLAN","WHILE","EXEC","PRECISION","WITH","EXECUTE","PRIMARY","WITHIN GROUP","EXISTS","PRINT","WRITETEXT","EXIT","PROC"],f=["ADD","ALTER COLUMN","ALTER TABLE","CASE","DELETE FROM","END","EXCEPT","FROM","GROUP BY","HAVING","INSERT INTO","INSERT","LIMIT","ORDER BY","SELECT","SET CURRENT SCHEMA","SET SCHEMA","SET","UPDATE","VALUES","WHERE"],d=["INTERSECT","INTERSECT ALL","MINUS","UNION","UNION ALL"],p=["AND","ELSE","OR","WHEN","JOIN","INNER JOIN","LEFT JOIN","LEFT OUTER JOIN","RIGHT JOIN","RIGHT OUTER JOIN","FULL JOIN","FULL OUTER JOIN","CROSS JOIN"];t.default=/*#__PURE__*/function(e){!function(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,writable:!0,configurable:!0}}),t&&l(e,t)}(m,e);var t,n,r,o=(n=m,r=function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],function(){})),!0}catch(e){return!1}}(),function(){var e,t=c(n);if(r){var a=c(this).constructor;e=Reflect.construct(t,arguments,a)}else e=t.apply(this,arguments);return s(this,e)});function m(){return i(this,m),o.apply(this,arguments)}return(t=[{key:"tokenizer",value:function(){return new a.default({reservedWords:u,reservedTopLevelWords:f,reservedNewlineWords:p,reservedTopLevelWordsNoIndent:d,stringTypes:['""',"N''","''","[]"],openParens:["(","CASE"],closeParens:[")","END"],indexedPlaceholderTypes:[],namedPlaceholderTypes:["@"],lineCommentTypes:["--"],specialWordChars:["#","@"],operators:[">=","<=","<>","!=","!<","!>","+=","-=","*=","/=","%=","|=","&=","^=","::"]})}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(m.prototype,t),m}(r.default),e.exports=t.default}),Oc=/*@__PURE__*/Ie(Le(function(e,t){Object.defineProperty(t,"__esModule",{value:!0}),t.supportedDialects=t.format=void 0;var n=d(gc),r=d(yc),a=d(vc),o=d(bc),i=d(Ec),l=d(Tc),s=d(Sc),c=d(wc),u=d(Nc),f=d(Rc);function d(e){return e&&e.__esModule?e:{default:e}}function p(e){return(p="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}var m={db2:n.default,mariadb:r.default,mysql:a.default,n1ql:o.default,plsql:i.default,postgresql:l.default,redshift:s.default,spark:c.default,sql:u.default,tsql:f.default};t.format=function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{};if("string"!=typeof e)throw new Error("Invalid query argument. Extected string, instead got "+p(e));var n=u.default;if(void 0!==t.language&&(n=m[t.language]),void 0===n)throw Error("Unsupported SQL dialect: ".concat(t.language));return new n(t).format(e)};var h=Object.keys(m);t.supportedDialects=h}));function Cc({children:e,language:t}){return c.createElement(Il,{language:t,customStyle:{background:"transparent"}},e)}function xc({value:e,limitHeight:t=!0,language:n=null,transparent:r=!1,overflowX:a=!0}){const[o,i]=c.useState(t),[l,s]=c.useState("sql"===n),u=c.useRef(null),f=c.useRef(null);return c.useEffect(()=>{u.current&&s(u.current.scrollHeight>u.current.clientHeight)},[u.current,o,e,t]),c.createElement("div",{ref:f,className:`\n ${l?"cursor-pointer":""}\n ${r?"":"~bg-gray-500/5"}\n group py-2 relative`,onClick:function(e){var t;3!==e.detail?l&&(!o&&null!=(t=window.getSelection())&&t.toString().length||i(!o)):function(){const e=document.createRange();e.selectNodeContents(f.current);const t=window.getSelection();t.removeAllRanges(),t.addRange(e)}()}},c.createElement("div",{className:a?"mask-fade-x":""},"sql"===n&&c.createElement(c.Fragment,null,o?c.createElement("pre",{className:"pl-4 "+(a?"overflow-x-scroll scrollbar-hidden-x pr-12":"truncate pr-8")},c.createElement("code",{className:"font-mono leading-relaxed text-sm font-normal"},c.createElement(Cc,{language:"sql"},e))):c.createElement("pre",{className:"pl-4 "+(a?"overflow-x-scroll scrollbar-hidden-x pr-12":"pr-8")},c.createElement("code",{className:"font-mono leading-relaxed text-sm font-normal"},c.createElement(Cc,{language:"sql"},Oc.format(e,{language:"mysql"}))))),"sql"!==n&&c.createElement("pre",{ref:u,className:`\n pl-4\n ${l?"mask-fade-y -mb-2":""}\n ${o?"overflow-y-hidden max-h-32":""}\n ${a?"overflow-x-scroll scrollbar-hidden-x pr-12":"pr-8"}\n `},c.createElement("code",{className:"font-mono leading-relaxed text-sm font-normal"},n?c.createElement(Cc,{language:n},e):e))),c.createElement(zo,{className:"absolute top-2 right-3",value:e}),l&&c.createElement(Fo,{onClick:()=>i(!o),className:"\n absolute -bottom-3 left-1/2 -translate-x-1/2\n opacity-0 group-hover:opacity-100 scale-80 group-hover:scale-100 delay-100\n "},c.createElement(qa,{icon:Ja,className:"transition-transform duration-300 transform "+(o?"":"rotate-180")})))}const kc=["children","className"];function Ac(e){let{children:t,className:n=""}=e,r=Ce(e,kc);return t?c.createElement("dl",Ne({className:`grid grid-cols-1 gap-2 ${n}`},r),t):null}function Ic({frame:e}){var t;return c.createElement(Ac,{className:"pb-10 px-6 @lg:px-10"},null==(t=e.arguments)?void 0:t.map((e,t)=>c.createElement(Ac.Row,{key:t,label:c.createElement("div",{className:"font-mono text-sm"},c.createElement("span",{className:"hljs-function hljs-params hljs-variable"},c.createElement("span",{title:"by reference"},e.is_variadic&&"…"),c.createElement("span",null,e.passed_by_reference&&"&"),c.createElement("span",{title:"variadic"},"$"),e.name),c.createElement("span",{className:"text-xs pl-px hljs-function hljs-keyword"},":",e.original_type,e.truncated&&" - truncated")),value:e.value,type:e.original_type,stacked:!0})))}function Lc({frames:e,openFrameIndex:t}){const n=c.useMemo(()=>{let n=1;const r=function(e,t,n){var r=null==e?0:e.length;if(!r)return-1;var a=0;return a<0&&(a=ac(r+a,0)),gt(e,rc(e=>"application"===ke(e)),a)}(e);return-1!==r&&(n=e.length-r),t&&(n=e.length-t),Nt({frames:e,expanded:[],selected:n},{type:"COLLAPSE_ALL_VENDOR_FRAMES"})},[e]),[r,a]=c.useReducer(Nt,n),o=c.useMemo(()=>function(e){return xe(e.frames).filter(e=>"vendor"===ke(e)).every(t=>e.expanded.includes(t.frame_number))}(r),[r]),i=c.useMemo(()=>function({frames:e,selected:t,expanded:n}){return e.reduce((r,a,o)=>{const i={current:a,previous:r[r.length-1]||Rt,isFirstFrame:0===o,frameNumber:e.length-o,expanded:n,selected:t};return i.expanded.includes(i.frameNumber)?r.concat(function(e){return e.current.relative_file!==e.previous.relative_file?[{type:ke(e.current),relative_file:e.current.relative_file,expanded:!0,frames:[Ne({},e.current,{frame_number:e.frameNumber,selected:e.selected===e.frameNumber})]}]:(e.previous.frames.push(Ne({},e.current,{frame_number:e.frameNumber,selected:e.selected===e.frameNumber})),[])}(i)):r.concat(function(e){const t=ke(e.current);return e.previous.expanded||t!==e.previous.type?[{type:t,relative_file:e.current.relative_file,expanded:!1,frames:[Ne({},e.current,{frame_number:e.frameNumber,selected:e.selected===e.frameNumber})]}]:(e.previous.frames.push(Ne({},e.current,{selected:!1,frame_number:e.frameNumber})),[])}(i))},[])}(r),[r]),l=c.useMemo(()=>function(e){const t=xe(e.frames);return t.find(t=>t.frame_number===e.selected)||t[0]||null}(r),[r]);return Ot("j",()=>{a({type:"SELECT_NEXT_FRAME"})}),Ot("k",()=>{a({type:"SELECT_PREVIOUS_FRAME"})}),c.createElement(c.Fragment,null,c.createElement("aside",{className:"z-30 flex flex-col border-r ~border-gray-200 relative"},c.createElement("div",{className:"max-h-[33vh] @4xl:max-h-[none] @4xl:absolute inset-0 flex flex-col overflow-hidden ~bg-white rounded-t-lg"},c.createElement("header",{className:"flex-none px-6 @lg:px-10 h-16 flex items-center justify-start ~bg-white border-b ~border-gray-200"},c.createElement(xt,{onClick:()=>a({type:o?"COLLAPSE_ALL_VENDOR_FRAMES":"EXPAND_ALL_VENDOR_FRAMES"})},c.createElement("div",{className:"flex "+(o?"flex-col-reverse":"flex-col")},c.createElement(qa,{icon:Za,className:"-my-px text-[8px] ~text-gray-500 group-hover:text-indigo-500"}),c.createElement(qa,{icon:Ja,className:"-my-px text-[8px] ~text-gray-500 group-hover:text-indigo-500"})),o?"Collapse vendor frames":" Expand vendor frames")),c.createElement("div",{id:"frames",className:"flex-grow overflow-auto scrollbar-hidden-y mask-fade-frames"},c.createElement("ol",{className:"text-sm pb-16"},i.map((e,t)=>c.createElement(_o,{key:t,frameGroup:e,onExpand:()=>a({type:"EXPAND_FRAMES",frames:e.frames.map(e=>e.frame_number)}),onSelect:e=>{a({type:"SELECT_FRAME",frame:e})}})))))),c.createElement("section",{className:"flex flex-col border-t @4xl:border-t-0 ~border-gray-200 relative"},l&&c.createElement(c.Fragment,null,c.createElement("header",{className:"~text-gray-500 flex-none z-30 h-16 px-6 @lg:px-10 flex items-center justify-end"},c.createElement(Bo,{path:l.file,lineNumber:l.line_number,className:"flex items-center text-sm"})),c.createElement(_l,{frame:l}))),(null==l?void 0:l.arguments)&&l.arguments.length>0&&c.createElement("section",{className:"border-t ~border-gray-200 @4xl:col-span-2"},c.createElement("header",{className:"font-bold text-xs ~text-gray-500 uppercase tracking-wider h-16 px-6 @lg:px-10 flex items-center"},"arguments"),c.createElement(Ic,{frame:l})))}function _c({openFrameIndex:e}){const{frames:t}=c.useContext(be);return c.createElement("div",{className:"@container bg-gray-25 dark:shadow-none dark:bg-gray-800/50 bg-gradient-to-bl from-white dark:from-gray-700/50 via-transparent dark:ring-1 dark:ring-inset dark:ring-white/5 rounded-lg shadow-2xl shadow-gray-500/20"},c.createElement("div",{className:"grid grid-cols-1 @4xl:grid-cols-[33.33%_66.66%] @4xl:grid-rows-[57rem] items-stretch overflow-hidden"},c.createElement(Lc,{frames:t,openFrameIndex:e})))}function Pc({message:e,className:t=""}){const[n,r]=c.useState(!1);return c.createElement("div",{className:`\n my-4 font-semibold leading-snug text-xl\n ${t}\n `,onClick:function(){var e;n&&null!=(e=window.getSelection())&&e.toString().length||r(!n)}},c.createElement("div",{className:n?"line-clamp-none":"line-clamp-2"},e))}function Mc({message:e,exceptionClass:t,className:n=""}){const[r,a]=c.useState(e),[o,i]=c.useState(null);return c.useEffect(()=>{if("Illuminate\\Database\\QueryException"===t||e.match(/SQLSTATE\[[\s\S]*\][\s\S]*SQL: [\s\S]*\)/)){const t=/*#__PURE__*/we(/\((?:|Connection: [\s\S]*?, )SQL: ([\s\S]*?)\)($| \(View: [\s\S]*\)$)/,{query:1}),[,n]=e.match(t)||[];i(n),a(e.replace(t,"$2"))}},[e,t]),c.createElement(c.Fragment,null,c.createElement(Pc,{message:r,className:n}),o&&c.createElement(xc,{value:o,language:"sql"}))}Ac.Row=function({value:e="",label:t="",className:n="",stacked:r=!1,type:a,small:o=!1}){let i=e;const[l,s]=c.useState(!1);let u;return c.isValidElement(e)?i=e:"boolean"==typeof e?i=c.createElement("span",{className:(e?"text-emerald-500 bg-emerald-500/5":"text-red-500 bg-red-800/5")+" text-sm px-3 py-2 inline-flex gap-2 items-center justify-center"},c.createElement(qa,{className:`${e} ? 'text-emerald-500' : 'text-red-500`,icon:e?no:Io}),c.createElement("span",{className:"font-mono"},e?"true":"false")):"string"===a||"object"==typeof e?i=c.createElement(xc,{value:Do(e),language:"json"}):"string"==typeof e?i=c.createElement(xc,{value:e}):"number"==typeof e&&(i=c.createElement(xc,{value:String(e)})),c.createElement("div",{className:`${r?"flex flex-col":"flex items-baseline "+(o?"gap-3":"gap-10")} ${n}`},c.createElement("dt",{className:`\n ${r?"self-start pt-2 pb-1.5 leading-tight":l?o?"flex-grow truncate min-w-[2rem] max-w-max":"flex-grow truncate min-w-[8rem] max-w-max":o?"flex-none truncate w-[2rem]":"flex-none truncate w-[8rem]"}\n `,onMouseOver:()=>{u=setTimeout(()=>s(!0),500)},onMouseOut:()=>{clearTimeout(u),s(!1)}},t),c.createElement("dd",{className:"flex-grow min-w-0"},i))};const Dc=["children","className","disabled"];function Uc(e){let{children:t,className:n="",disabled:r=!1}=e,a=Ce(e,Dc);return c.createElement("button",Ne({disabled:r,className:`px-4 h-8 whitespace-nowrap border-b\n text-xs uppercase tracking-wider font-bold rounded-sm\n shadow-md\n transform\n transition-animation\n hover:shadow-lg\n active:shadow-inner\n active:translate-y-px\n ${r?"opacity-50":"opacity-100"}\n ${n}\n `},a),t)}var jc=/*@__PURE__*/Ie(Le(function(e,t){e.exports=(()=>{var e=Object.create,t=Object.defineProperty,n=Object.defineProperties,r=Object.getOwnPropertyDescriptor,a=Object.getOwnPropertyDescriptors,o=Object.getOwnPropertyNames,i=Object.getOwnPropertySymbols,l=Object.getPrototypeOf,s=Object.prototype.hasOwnProperty,c=Object.prototype.propertyIsEnumerable,u=Math.pow,f=(e,n,r)=>n in e?t(e,n,{enumerable:!0,configurable:!0,writable:!0,value:r}):e[n]=r,d=(e,t)=>{for(var n in t||(t={}))s.call(t,n)&&f(e,n,t[n]);if(i)for(var n of i(t))c.call(t,n)&&f(e,n,t[n]);return e},p=(e,t)=>n(e,a(t)),m=(e,t)=>()=>(t||e((t={exports:{}}).exports,t),t.exports),h=(e,n)=>{for(var r in n)t(e,r,{get:n[r],enumerable:!0})},g=(e,n,a,i)=>{if(n&&"object"==typeof n||"function"==typeof n)for(let l of o(n))!s.call(e,l)&&l!==a&&t(e,l,{get:()=>n[l],enumerable:!(i=r(n,l))||i.enumerable});return e},y=(n,r,a)=>(a=null!=n?e(l(n)):{},g(!r&&n&&n.__esModule?a:t(a,"default",{value:n,enumerable:!0}),n)),v=m(e=>{var t=Symbol.for("react.element"),n=Symbol.for("react.portal"),r=Symbol.for("react.fragment"),a=Symbol.for("react.strict_mode"),o=Symbol.for("react.profiler"),i=Symbol.for("react.provider"),l=Symbol.for("react.context"),s=Symbol.for("react.forward_ref"),c=Symbol.for("react.suspense"),u=Symbol.for("react.memo"),f=Symbol.for("react.lazy"),d=Symbol.iterator,p={isMounted:function(){return!1},enqueueForceUpdate:function(){},enqueueReplaceState:function(){},enqueueSetState:function(){}},m=Object.assign,h={};function g(e,t,n){this.props=e,this.context=t,this.refs=h,this.updater=n||p}function y(){}function v(e,t,n){this.props=e,this.context=t,this.refs=h,this.updater=n||p}g.prototype.isReactComponent={},g.prototype.setState=function(e,t){if("object"!=typeof e&&"function"!=typeof e&&null!=e)throw Error("setState(...): takes an object of state variables to update or a function which returns an object of state variables.");this.updater.enqueueSetState(this,e,t,"setState")},g.prototype.forceUpdate=function(e){this.updater.enqueueForceUpdate(this,e,"forceUpdate")},y.prototype=g.prototype;var b=v.prototype=new y;b.constructor=v,m(b,g.prototype),b.isPureReactComponent=!0;var E=Array.isArray,T=Object.prototype.hasOwnProperty,S={current:null},w={key:!0,ref:!0,__self:!0,__source:!0};function N(e,n,r){var a,o={},i=null,l=null;if(null!=n)for(a in void 0!==n.ref&&(l=n.ref),void 0!==n.key&&(i=""+n.key),n)T.call(n,a)&&!w.hasOwnProperty(a)&&(o[a]=n[a]);var s=arguments.length-2;if(1===s)o.children=r;else if(1<s){for(var c=Array(s),u=0;u<s;u++)c[u]=arguments[u+2];o.children=c}if(e&&e.defaultProps)for(a in s=e.defaultProps)void 0===o[a]&&(o[a]=s[a]);return{$$typeof:t,type:e,key:i,ref:l,props:o,_owner:S.current}}function R(e){return"object"==typeof e&&null!==e&&e.$$typeof===t}var O=/\/+/g;function C(e,t){return"object"==typeof e&&null!==e&&null!=e.key?function(e){var t={"=":"=0",":":"=2"};return"$"+e.replace(/[=:]/g,function(e){return t[e]})}(""+e.key):t.toString(36)}function x(e,r,a,o,i){var l=typeof e;("undefined"===l||"boolean"===l)&&(e=null);var s=!1;if(null===e)s=!0;else switch(l){case"string":case"number":s=!0;break;case"object":switch(e.$$typeof){case t:case n:s=!0}}if(s)return i=i(s=e),e=""===o?"."+C(s,0):o,E(i)?(a="",null!=e&&(a=e.replace(O,"$&/")+"/"),x(i,r,a,"",function(e){return e})):null!=i&&(R(i)&&(i=function(e,n){return{$$typeof:t,type:e.type,key:n,ref:e.ref,props:e.props,_owner:e._owner}}(i,a+(!i.key||s&&s.key===i.key?"":(""+i.key).replace(O,"$&/")+"/")+e)),r.push(i)),1;if(s=0,o=""===o?".":o+":",E(e))for(var c=0;c<e.length;c++){var u=o+C(l=e[c],c);s+=x(l,r,a,u,i)}else if("function"==typeof(u=function(e){return null===e||"object"!=typeof e?null:"function"==typeof(e=d&&e[d]||e["@@iterator"])?e:null}(e)))for(e=u.call(e),c=0;!(l=e.next()).done;)s+=x(l=l.value,r,a,u=o+C(l,c++),i);else if("object"===l)throw r=String(e),Error("Objects are not valid as a React child (found: "+("[object Object]"===r?"object with keys {"+Object.keys(e).join(", ")+"}":r)+"). If you meant to render a collection of children, use an array instead.");return s}function k(e,t,n){if(null==e)return e;var r=[],a=0;return x(e,r,"","",function(e){return t.call(n,e,a++)}),r}function A(e){if(-1===e._status){var t=e._result;(t=t()).then(function(t){(0===e._status||-1===e._status)&&(e._status=1,e._result=t)},function(t){(0===e._status||-1===e._status)&&(e._status=2,e._result=t)}),-1===e._status&&(e._status=0,e._result=t)}if(1===e._status)return e._result.default;throw e._result}var I={current:null},L={transition:null},_={ReactCurrentDispatcher:I,ReactCurrentBatchConfig:L,ReactCurrentOwner:S};e.Children={map:k,forEach:function(e,t,n){k(e,function(){t.apply(this,arguments)},n)},count:function(e){var t=0;return k(e,function(){t++}),t},toArray:function(e){return k(e,function(e){return e})||[]},only:function(e){if(!R(e))throw Error("React.Children.only expected to receive a single React element child.");return e}},e.Component=g,e.Fragment=r,e.Profiler=o,e.PureComponent=v,e.StrictMode=a,e.Suspense=c,e.__SECRET_INTERNALS_DO_NOT_USE_OR_YOU_WILL_BE_FIRED=_,e.cloneElement=function(e,n,r){if(null==e)throw Error("React.cloneElement(...): The argument must be a React element, but you passed "+e+".");var a=m({},e.props),o=e.key,i=e.ref,l=e._owner;if(null!=n){if(void 0!==n.ref&&(i=n.ref,l=S.current),void 0!==n.key&&(o=""+n.key),e.type&&e.type.defaultProps)var s=e.type.defaultProps;for(c in n)T.call(n,c)&&!w.hasOwnProperty(c)&&(a[c]=void 0===n[c]&&void 0!==s?s[c]:n[c])}var c=arguments.length-2;if(1===c)a.children=r;else if(1<c){s=Array(c);for(var u=0;u<c;u++)s[u]=arguments[u+2];a.children=s}return{$$typeof:t,type:e.type,key:o,ref:i,props:a,_owner:l}},e.createContext=function(e){return(e={$$typeof:l,_currentValue:e,_currentValue2:e,_threadCount:0,Provider:null,Consumer:null,_defaultValue:null,_globalName:null}).Provider={$$typeof:i,_context:e},e.Consumer=e},e.createElement=N,e.createFactory=function(e){var t=N.bind(null,e);return t.type=e,t},e.createRef=function(){return{current:null}},e.forwardRef=function(e){return{$$typeof:s,render:e}},e.isValidElement=R,e.lazy=function(e){return{$$typeof:f,_payload:{_status:-1,_result:e},_init:A}},e.memo=function(e,t){return{$$typeof:u,type:e,compare:void 0===t?null:t}},e.startTransition=function(e){var t=L.transition;L.transition={};try{e()}finally{L.transition=t}},e.unstable_act=function(){throw Error("act(...) is not supported in production builds of React.")},e.useCallback=function(e,t){return I.current.useCallback(e,t)},e.useContext=function(e){return I.current.useContext(e)},e.useDebugValue=function(){},e.useDeferredValue=function(e){return I.current.useDeferredValue(e)},e.useEffect=function(e,t){return I.current.useEffect(e,t)},e.useId=function(){return I.current.useId()},e.useImperativeHandle=function(e,t,n){return I.current.useImperativeHandle(e,t,n)},e.useInsertionEffect=function(e,t){return I.current.useInsertionEffect(e,t)},e.useLayoutEffect=function(e,t){return I.current.useLayoutEffect(e,t)},e.useMemo=function(e,t){return I.current.useMemo(e,t)},e.useReducer=function(e,t,n){return I.current.useReducer(e,t,n)},e.useRef=function(e){return I.current.useRef(e)},e.useState=function(e){return I.current.useState(e)},e.useSyncExternalStore=function(e,t,n){return I.current.useSyncExternalStore(e,t,n)},e.useTransition=function(){return I.current.useTransition()},e.version="18.2.0"}),b=m((e,t)=>{t.exports=v()}),E=m((e,t)=>{t.exports=function(e){return null!=e&&null!=e.constructor&&"function"==typeof e.constructor.isBuffer&&e.constructor.isBuffer(e)}}),T=m((e,t)=>{var n=Object.prototype.hasOwnProperty,r=Object.prototype.toString,a=Object.defineProperty,o=Object.getOwnPropertyDescriptor,i=function(e){return"function"==typeof Array.isArray?Array.isArray(e):"[object Array]"===r.call(e)},l=function(e){if(!e||"[object Object]"!==r.call(e))return!1;var t,a=n.call(e,"constructor"),o=e.constructor&&e.constructor.prototype&&n.call(e.constructor.prototype,"isPrototypeOf");if(e.constructor&&!a&&!o)return!1;for(t in e);return void 0===t||n.call(e,t)},s=function(e,t){a&&"__proto__"===t.name?a(e,t.name,{enumerable:!0,configurable:!0,value:t.newValue,writable:!0}):e[t.name]=t.newValue},c=function(e,t){if("__proto__"===t){if(!n.call(e,t))return;if(o)return o(e,t).value}return e[t]};t.exports=function e(){var t,n,r,a,o,u,f=arguments[0],d=1,p=arguments.length,m=!1;for("boolean"==typeof f&&(m=f,f=arguments[1]||{},d=2),(null==f||"object"!=typeof f&&"function"!=typeof f)&&(f={});d<p;++d)if(null!=(t=arguments[d]))for(n in t)r=c(f,n),f!==(a=c(t,n))&&(m&&a&&(l(a)||(o=i(a)))?(o?(o=!1,u=r&&i(r)?r:[]):u=r&&l(r)?r:{},s(f,{name:n,newValue:e(m,u,a)})):void 0!==a&&s(f,{name:n,newValue:a}));return f}}),S=m((e,t)=>{t.exports="SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED"}),w=m((e,t)=>{var n=S();function r(){}function a(){}a.resetWarningCache=r,t.exports=function(){function e(e,t,r,a,o,i){if(i!==n){var l=new Error("Calling PropTypes validators directly is not supported by the `prop-types` package. Use PropTypes.checkPropTypes() to call them. Read more at http://fb.me/use-check-prop-types");throw l.name="Invariant Violation",l}}function t(){return e}e.isRequired=e;var o={array:e,bigint:e,bool:e,func:e,number:e,object:e,string:e,symbol:e,any:e,arrayOf:t,element:e,elementType:e,instanceOf:t,node:e,objectOf:t,oneOf:t,oneOfType:t,shape:t,exact:t,checkPropTypes:a,resetWarningCache:r};return o.PropTypes=o,o}}),N=m((e,t)=>{t.exports=w()()}),R=m(e=>{var t,n=Symbol.for("react.element"),r=Symbol.for("react.portal"),a=Symbol.for("react.fragment"),o=Symbol.for("react.strict_mode"),i=Symbol.for("react.profiler"),l=Symbol.for("react.provider"),s=Symbol.for("react.context"),c=Symbol.for("react.server_context"),u=Symbol.for("react.forward_ref"),f=Symbol.for("react.suspense"),d=Symbol.for("react.suspense_list"),p=Symbol.for("react.memo"),m=Symbol.for("react.lazy"),h=Symbol.for("react.offscreen");function g(e){if("object"==typeof e&&null!==e){var t=e.$$typeof;switch(t){case n:switch(e=e.type){case a:case i:case o:case f:case d:return e;default:switch(e=e&&e.$$typeof){case c:case s:case u:case m:case p:case l:return e;default:return t}}case r:return t}}}t=Symbol.for("react.module.reference"),e.ContextConsumer=s,e.ContextProvider=l,e.Element=n,e.ForwardRef=u,e.Fragment=a,e.Lazy=m,e.Memo=p,e.Portal=r,e.Profiler=i,e.StrictMode=o,e.Suspense=f,e.SuspenseList=d,e.isAsyncMode=function(){return!1},e.isConcurrentMode=function(){return!1},e.isContextConsumer=function(e){return g(e)===s},e.isContextProvider=function(e){return g(e)===l},e.isElement=function(e){return"object"==typeof e&&null!==e&&e.$$typeof===n},e.isForwardRef=function(e){return g(e)===u},e.isFragment=function(e){return g(e)===a},e.isLazy=function(e){return g(e)===m},e.isMemo=function(e){return g(e)===p},e.isPortal=function(e){return g(e)===r},e.isProfiler=function(e){return g(e)===i},e.isStrictMode=function(e){return g(e)===o},e.isSuspense=function(e){return g(e)===f},e.isSuspenseList=function(e){return g(e)===d},e.isValidElementType=function(e){return"string"==typeof e||"function"==typeof e||e===a||e===i||e===o||e===f||e===d||e===h||"object"==typeof e&&null!==e&&(e.$$typeof===m||e.$$typeof===p||e.$$typeof===l||e.$$typeof===s||e.$$typeof===u||e.$$typeof===t||void 0!==e.getModuleId)},e.typeOf=g}),O=m((e,t)=>{t.exports=R()}),C=m((e,t)=>{var n=/\/\*[^*]*\*+([^/*][^*]*\*+)*\//g,r=/\n/g,a=/^\s*/,o=/^(\*?[-#/*\\\w]+(\[[0-9a-z_-]+\])?)\s*/,i=/^:\s*/,l=/^((?:'(?:\\'|.)*?'|"(?:\\"|.)*?"|\([^)]*?\)|[^};])+)/,s=/^[;\s]*/,c=/^\s+|\s+$/g,u="";function f(e){return e?e.replace(c,u):u}t.exports=function(e,t){if("string"!=typeof e)throw new TypeError("First argument must be a string");if(!e)return[];t=t||{};var c=1,d=1;function p(e){var t=e.match(r);t&&(c+=t.length);var n=e.lastIndexOf("\n");d=~n?e.length-n:d+e.length}function m(){var e={line:c,column:d};return function(t){return t.position=new h(e),v(),t}}function h(e){this.start=e,this.end={line:c,column:d},this.source=t.source}function g(n){var r=new Error(t.source+":"+c+":"+d+": "+n);if(r.reason=n,r.filename=t.source,r.line=c,r.column=d,r.source=e,!t.silent)throw r}function y(t){var n=t.exec(e);if(n){var r=n[0];return p(r),e=e.slice(r.length),n}}function v(){y(a)}function b(e){var t;for(e=e||[];t=E();)!1!==t&&e.push(t);return e}function E(){var t=m();if("/"==e.charAt(0)&&"*"==e.charAt(1)){for(var n=2;u!=e.charAt(n)&&("*"!=e.charAt(n)||"/"!=e.charAt(n+1));)++n;if(u===e.charAt((n+=2)-1))return g("End of comment missing");var r=e.slice(2,n-2);return d+=2,p(r),e=e.slice(n),d+=2,t({type:"comment",comment:r})}}function T(){var e=m(),t=y(o);if(t){if(E(),!y(i))return g("property missing ':'");var r=y(l),a=e({type:"declaration",property:f(t[0].replace(n,u)),value:r?f(r[0].replace(n,u)):u});return y(s),a}}return h.prototype.content=e,v(),function(){var e,t=[];for(b(t);e=T();)!1!==e&&(t.push(e),b(t));return t}()}}),x=m((e,t)=>{var n=C();function r(e,t){var r=null;if(!e||"string"!=typeof e)return r;for(var a,o,i,l=n(e),s="function"==typeof t,c=0,u=l.length;c<u;c++)o=(a=l[c]).property,i=a.value,s?t(o,i,a):i&&(r||(r={}),r[o]=i);return r}t.exports=r,t.exports.default=r}),k={};h(k,{default:()=>Er,uriTransformer:()=>I});var A=["http","https","mailto","tel"];function I(e){let t=(e||"").trim(),n=t.charAt(0);if("#"===n||"/"===n)return t;let r=t.indexOf(":");if(-1===r)return t;let a=-1;for(;++a<A.length;){let e=A[a];if(r===e.length&&t.slice(0,e.length).toLowerCase()===e)return t}return a=t.indexOf("?"),-1!==a&&r>a||(a=t.indexOf("#"),-1!==a&&r>a)?t:"javascript:void(0)"}var L=y(b(),1),_=y(E(),1);function P(e){return e&&"object"==typeof e?"position"in e||"type"in e?D(e.position):"start"in e||"end"in e?D(e):"line"in e||"column"in e?M(e):"":""}function M(e){return U(e&&e.line)+":"+U(e&&e.column)}function D(e){return M(e&&e.start)+"-"+M(e&&e.end)}function U(e){return e&&"number"==typeof e?e:1}var j=class extends Error{constructor(e,t,n){let r=[null,null],a={start:{line:null,column:null},end:{line:null,column:null}};if(super(),"string"==typeof t&&(n=t,t=void 0),"string"==typeof n){let e=n.indexOf(":");-1===e?r[1]=n:(r[0]=n.slice(0,e),r[1]=n.slice(e+1))}t&&("type"in t||"position"in t?t.position&&(a=t.position):"start"in t||"end"in t?a=t:("line"in t||"column"in t)&&(a.start=t)),this.name=P(t)||"1:1",this.message="object"==typeof e?e.message:e,this.stack="","object"==typeof e&&e.stack&&(this.stack=e.stack),this.reason=this.message,this.line=a.start.line,this.column=a.start.column,this.position=a,this.source=r[0],this.ruleId=r[1]}};j.prototype.file="",j.prototype.name="",j.prototype.reason="",j.prototype.message="",j.prototype.stack="",j.prototype.fatal=null,j.prototype.column=null,j.prototype.line=null,j.prototype.source=null,j.prototype.ruleId=null,j.prototype.position=null;var F=function(e,t){if(void 0!==t&&"string"!=typeof t)throw new TypeError('"ext" argument must be a string');B(e);let n,r=0,a=-1,o=e.length;if(void 0===t||0===t.length||t.length>e.length){for(;o--;)if(47===e.charCodeAt(o)){if(n){r=o+1;break}}else a<0&&(n=!0,a=o+1);return a<0?"":e.slice(r,a)}if(t===e)return"";let i=-1,l=t.length-1;for(;o--;)if(47===e.charCodeAt(o)){if(n){r=o+1;break}}else i<0&&(n=!0,i=o+1),l>-1&&(e.charCodeAt(o)===t.charCodeAt(l--)?l<0&&(a=o):(l=-1,a=i));return r===a?a=i:a<0&&(a=e.length),e.slice(r,a)},z=function(...e){let t,n=-1;for(;++n<e.length;)B(e[n]),e[n]&&(t=void 0===t?e[n]:t+"/"+e[n]);return void 0===t?".":function(e){B(e);let t=47===e.charCodeAt(0),n=function(e,t){let n,r,a="",o=0,i=-1,l=0,s=-1;for(;++s<=e.length;){if(s<e.length)n=e.charCodeAt(s);else{if(47===n)break;n=47}if(47===n){if(i!==s-1&&1!==l)if(i!==s-1&&2===l){if(a.length<2||2!==o||46!==a.charCodeAt(a.length-1)||46!==a.charCodeAt(a.length-2))if(a.length>2){if(r=a.lastIndexOf("/"),r!==a.length-1){r<0?(a="",o=0):(a=a.slice(0,r),o=a.length-1-a.lastIndexOf("/")),i=s,l=0;continue}}else if(a.length>0){a="",o=0,i=s,l=0;continue}t&&(a=a.length>0?a+"/..":"..",o=2)}else a.length>0?a+="/"+e.slice(i+1,s):a=e.slice(i+1,s),o=s-i-1;i=s,l=0}else 46===n&&l>-1?l++:l=-1}return a}(e,!t);return 0===n.length&&!t&&(n="."),n.length>0&&47===e.charCodeAt(e.length-1)&&(n+="/"),t?"/"+n:n}(t)};function B(e){if("string"!=typeof e)throw new TypeError("Path must be a string. Received "+JSON.stringify(e))}function H(e){return null!==e&&"object"==typeof e&&e.href&&e.origin}var V=["history","path","basename","stem","extname","dirname"],W=class{constructor(e){let t;t=e?"string"==typeof e||function(e){return(0,_.default)(e)}(e)?{value:e}:H(e)?{path:e}:e:{},this.data={},this.messages=[],this.history=[],this.cwd="/";let n,r=-1;for(;++r<V.length;){let e=V[r];e in t&&null!=t[e]&&(this[e]="history"===e?[...t[e]]:t[e])}for(n in t)V.includes(n)||(this[n]=t[n])}get path(){return this.history[this.history.length-1]}set path(e){H(e)&&(e=function(e){if("string"==typeof e)e=new URL(e);else if(!H(e)){let t=new TypeError('The "path" argument must be of type string or an instance of URL. Received `'+e+"`");throw t.code="ERR_INVALID_ARG_TYPE",t}if("file:"!==e.protocol){let e=new TypeError("The URL must be of scheme file");throw e.code="ERR_INVALID_URL_SCHEME",e}return function(e){if(""!==e.hostname){let e=new TypeError('File URL host must be "localhost" or empty on darwin');throw e.code="ERR_INVALID_FILE_URL_HOST",e}let t=e.pathname,n=-1;for(;++n<t.length;)if(37===t.charCodeAt(n)&&50===t.charCodeAt(n+1)){let e=t.charCodeAt(n+2);if(70===e||102===e){let e=new TypeError("File URL path must not include encoded / characters");throw e.code="ERR_INVALID_FILE_URL_PATH",e}}return decodeURIComponent(t)}(e)}(e)),Y(e,"path"),this.path!==e&&this.history.push(e)}get dirname(){return"string"==typeof this.path?function(e){if(B(e),0===e.length)return".";let t,n=-1,r=e.length;for(;--r;)if(47===e.charCodeAt(r)){if(t){n=r;break}}else t||(t=!0);return n<0?47===e.charCodeAt(0)?"/":".":1===n&&47===e.charCodeAt(0)?"//":e.slice(0,n)}(this.path):void 0}set dirname(e){$(this.basename,"dirname"),this.path=z(e||"",this.basename)}get basename(){return"string"==typeof this.path?F(this.path):void 0}set basename(e){Y(e,"basename"),G(e,"basename"),this.path=z(this.dirname||"",e)}get extname(){return"string"==typeof this.path?function(e){B(e);let t,n=e.length,r=-1,a=0,o=-1,i=0;for(;n--;){let l=e.charCodeAt(n);if(47!==l)r<0&&(t=!0,r=n+1),46===l?o<0?o=n:1!==i&&(i=1):o>-1&&(i=-1);else if(t){a=n+1;break}}return o<0||r<0||0===i||1===i&&o===r-1&&o===a+1?"":e.slice(o,r)}(this.path):void 0}set extname(e){if(G(e,"extname"),$(this.dirname,"extname"),e){if(46!==e.charCodeAt(0))throw new Error("`extname` must start with `.`");if(e.includes(".",1))throw new Error("`extname` cannot contain multiple dots")}this.path=z(this.dirname,this.stem+(e||""))}get stem(){return"string"==typeof this.path?F(this.path,this.extname):void 0}set stem(e){Y(e,"stem"),G(e,"stem"),this.path=z(this.dirname||"",e+(this.extname||""))}toString(e){return(this.value||"").toString(e||void 0)}message(e,t,n){let r=new j(e,t,n);return this.path&&(r.name=this.path+":"+r.name,r.file=this.path),r.fatal=!1,this.messages.push(r),r}info(e,t,n){let r=this.message(e,t,n);return r.fatal=null,r}fail(e,t,n){let r=this.message(e,t,n);throw r.fatal=!0,r}};function G(e,t){if(e&&e.includes("/"))throw new Error("`"+t+"` cannot be a path: did not expect `/`")}function Y(e,t){if(!e)throw new Error("`"+t+"` cannot be empty")}function $(e,t){if(!e)throw new Error("Setting `"+t+"` requires `path` to be set too")}function X(e){if(e)throw e}var q=y(E(),1),K=y(T(),1);function J(e){if("object"!=typeof e||null===e)return!1;let t=Object.getPrototypeOf(e);return!(null!==t&&t!==Object.prototype&&null!==Object.getPrototypeOf(t)||Symbol.toStringTag in e||Symbol.iterator in e)}var Q=function e(){let t,n=function(){let e=[],t={run:function(...t){let n=-1,r=t.pop();if("function"!=typeof r)throw new TypeError("Expected function as last argument, not "+r);!function a(o,...i){let l=e[++n],s=-1;if(o)r(o);else{for(;++s<t.length;)null==i[s]&&(i[s]=t[s]);t=i,l?function(e,t){let n;return function(...t){let o,i=e.length>t.length;i&&t.push(r);try{o=e.apply(this,t)}catch(e){let t=e;if(i&&n)throw t;return r(t)}i||(o instanceof Promise?o.then(a,r):o instanceof Error?r(o):a(o))};function r(e,...r){n||(n=!0,t(e,...r))}function a(e){r(null,e)}}(l,a)(...i):r(null,...i)}}(null,...t)},use:function(n){if("function"!=typeof n)throw new TypeError("Expected `middelware` to be a function, not "+n);return e.push(n),t}};return t}(),r=[],a={},o=-1;return i.data=function(e,n){return"string"==typeof e?2===arguments.length?(re("data",t),a[e]=n,i):Z.call(a,e)&&a[e]||null:e?(re("data",t),a=e,i):a},i.Parser=void 0,i.Compiler=void 0,i.freeze=function(){if(t)return i;for(;++o<r.length;){let[e,...t]=r[o];if(!1===t[0])continue;!0===t[0]&&(t[0]=void 0);let a=e.call(i,...t);"function"==typeof a&&n.use(a)}return t=!0,o=Number.POSITIVE_INFINITY,i},i.attachers=r,i.use=function(e,...n){let o;if(re("use",t),null!=e)if("function"==typeof e)u(e,...n);else{if("object"!=typeof e)throw new TypeError("Expected usable value, not `"+e+"`");Array.isArray(e)?c(e):s(e)}return o&&(a.settings=Object.assign(a.settings||{},o)),i;function l(e){if("function"==typeof e)u(e);else{if("object"!=typeof e)throw new TypeError("Expected usable value, not `"+e+"`");if(Array.isArray(e)){let[t,...n]=e;u(t,...n)}else s(e)}}function s(e){c(e.plugins),e.settings&&(o=Object.assign(o||{},e.settings))}function c(e){let t=-1;if(null!=e){if(!Array.isArray(e))throw new TypeError("Expected a list of plugins, not `"+e+"`");for(;++t<e.length;)l(e[t])}}function u(e,t){let n,a=-1;for(;++a<r.length;)if(r[a][0]===e){n=r[a];break}n?(J(n[1])&&J(t)&&(t=(0,K.default)(!0,n[1],t)),n[1]=t):r.push([...arguments])}},i.parse=function(e){i.freeze();let t=ie(e),n=i.Parser;return te("parse",n),ee(n,"parse")?new n(String(t),t).parse():n(String(t),t)},i.stringify=function(e,t){i.freeze();let n=ie(t),r=i.Compiler;return ne("stringify",r),ae(e),ee(r,"compile")?new r(e,n).compile():r(e,n)},i.run=function(e,t,r){if(ae(e),i.freeze(),!r&&"function"==typeof t&&(r=t,t=void 0),!r)return new Promise(a);function a(a,o){n.run(e,ie(t),function(t,n,i){n=n||e,t?o(t):a?a(n):r(null,n,i)})}a(null,r)},i.runSync=function(e,t){let n,r;return i.run(e,t,function(e,t){X(e),n=t,r=!0}),oe("runSync","run",r),n},i.process=function(e,t){if(i.freeze(),te("process",i.Parser),ne("process",i.Compiler),!t)return new Promise(n);function n(n,r){let a=ie(e);function o(e,a){e||!a?r(e):n?n(a):t(null,a)}i.run(i.parse(a),a,(e,t,n)=>{if(!e&&t&&n){let r=i.stringify(t,n);null==r||(function(e){return"string"==typeof e||(0,q.default)(e)}(r)?n.value=r:n.result=r),o(e,n)}else o(e)})}n(null,t)},i.processSync=function(e){let t;i.freeze(),te("processSync",i.Parser),ne("processSync",i.Compiler);let n=ie(e);return i.process(n,function(e){t=!0,X(e)}),oe("processSync","process",t),n},i;function i(){let t=e(),n=-1;for(;++n<r.length;)t.use(...r[n]);return t.data((0,K.default)(!0,{},a)),t}}().freeze(),Z={}.hasOwnProperty;function ee(e,t){return"function"==typeof e&&e.prototype&&(function(e){let t;for(t in e)if(Z.call(e,t))return!0;return!1}(e.prototype)||t in e.prototype)}function te(e,t){if("function"!=typeof t)throw new TypeError("Cannot `"+e+"` without `Parser`")}function ne(e,t){if("function"!=typeof t)throw new TypeError("Cannot `"+e+"` without `Compiler`")}function re(e,t){if(t)throw new Error("Cannot call `"+e+"` on a frozen processor.\nCreate a new processor first, by calling it: use `processor()` instead of `processor`.")}function ae(e){if(!J(e)||"string"!=typeof e.type)throw new TypeError("Expected node, got `"+e+"`")}function oe(e,t,n){if(!n)throw new Error("`"+e+"` finished async. Use `"+t+"` instead")}function ie(e){return function(e){return!!(e&&"object"==typeof e&&"message"in e&&"messages"in e)}(e)?e:new W(e)}var le={};function se(e,t,n){if(function(e){return!(!e||"object"!=typeof e)}(e)){if("value"in e)return"html"!==e.type||n?e.value:"";if(t&&"alt"in e&&e.alt)return e.alt;if("children"in e)return ce(e.children,t,n)}return Array.isArray(e)?ce(e,t,n):""}function ce(e,t,n){let r=[],a=-1;for(;++a<e.length;)r[a]=se(e[a],t,n);return r.join("")}function ue(e,t,n,r){let a,o=e.length,i=0;if(t=t<0?-t>o?0:o+t:t>o?o:t,n=n>0?n:0,r.length<1e4)a=Array.from(r),a.unshift(t,n),[].splice.apply(e,a);else for(n&&[].splice.apply(e,[t,n]);i<r.length;)a=r.slice(i,i+1e4),a.unshift(t,0),[].splice.apply(e,a),i+=1e4,t+=1e4}function fe(e,t){return e.length>0?(ue(e,e.length,0,t),e):t}var de={}.hasOwnProperty;function pe(e){let t={},n=-1;for(;++n<e.length;)me(t,e[n]);return t}function me(e,t){let n;for(n in t){let r,a=(de.call(e,n)?e[n]:void 0)||(e[n]={}),o=t[n];for(r in o){de.call(a,r)||(a[r]=[]);let e=o[r];he(a[r],Array.isArray(e)?e:e?[e]:[])}}}function he(e,t){let n=-1,r=[];for(;++n<t.length;)("after"===t[n].add?e:r).push(t[n]);ue(e,0,0,r)}var ge=xe(/[A-Za-z]/),ye=xe(/\d/),ve=xe(/[\dA-Fa-f]/),be=xe(/[\dA-Za-z]/),Ee=xe(/[!-/:-@[-`{-~]/),Te=xe(/[#-'*+\--9=?A-Z^-~]/);function Se(e){return null!==e&&(e<32||127===e)}function we(e){return null!==e&&(e<0||32===e)}function Ne(e){return null!==e&&e<-2}function Re(e){return-2===e||-1===e||32===e}var Oe=xe(/\s/),Ce=xe(/[!-/:-@[-`{-~\u00A1\u00A7\u00AB\u00B6\u00B7\u00BB\u00BF\u037E\u0387\u055A-\u055F\u0589\u058A\u05BE\u05C0\u05C3\u05C6\u05F3\u05F4\u0609\u060A\u060C\u060D\u061B\u061E\u061F\u066A-\u066D\u06D4\u0700-\u070D\u07F7-\u07F9\u0830-\u083E\u085E\u0964\u0965\u0970\u09FD\u0A76\u0AF0\u0C77\u0C84\u0DF4\u0E4F\u0E5A\u0E5B\u0F04-\u0F12\u0F14\u0F3A-\u0F3D\u0F85\u0FD0-\u0FD4\u0FD9\u0FDA\u104A-\u104F\u10FB\u1360-\u1368\u1400\u166E\u169B\u169C\u16EB-\u16ED\u1735\u1736\u17D4-\u17D6\u17D8-\u17DA\u1800-\u180A\u1944\u1945\u1A1E\u1A1F\u1AA0-\u1AA6\u1AA8-\u1AAD\u1B5A-\u1B60\u1BFC-\u1BFF\u1C3B-\u1C3F\u1C7E\u1C7F\u1CC0-\u1CC7\u1CD3\u2010-\u2027\u2030-\u2043\u2045-\u2051\u2053-\u205E\u207D\u207E\u208D\u208E\u2308-\u230B\u2329\u232A\u2768-\u2775\u27C5\u27C6\u27E6-\u27EF\u2983-\u2998\u29D8-\u29DB\u29FC\u29FD\u2CF9-\u2CFC\u2CFE\u2CFF\u2D70\u2E00-\u2E2E\u2E30-\u2E4F\u2E52\u3001-\u3003\u3008-\u3011\u3014-\u301F\u3030\u303D\u30A0\u30FB\uA4FE\uA4FF\uA60D-\uA60F\uA673\uA67E\uA6F2-\uA6F7\uA874-\uA877\uA8CE\uA8CF\uA8F8-\uA8FA\uA8FC\uA92E\uA92F\uA95F\uA9C1-\uA9CD\uA9DE\uA9DF\uAA5C-\uAA5F\uAADE\uAADF\uAAF0\uAAF1\uABEB\uFD3E\uFD3F\uFE10-\uFE19\uFE30-\uFE52\uFE54-\uFE61\uFE63\uFE68\uFE6A\uFE6B\uFF01-\uFF03\uFF05-\uFF0A\uFF0C-\uFF0F\uFF1A\uFF1B\uFF1F\uFF20\uFF3B-\uFF3D\uFF3F\uFF5B\uFF5D\uFF5F-\uFF65]/);function xe(e){return function(t){return null!==t&&e.test(String.fromCharCode(t))}}function ke(e,t,n,r){let a=r?r-1:Number.POSITIVE_INFINITY,o=0;return function(r){return Re(r)?(e.enter(n),i(r)):t(r)};function i(r){return Re(r)&&o++<a?(e.consume(r),i):(e.exit(n),t(r))}}var Ae={tokenize:function(e){let t,n=e.attempt(this.parser.constructs.contentInitial,function(t){if(null!==t)return e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),ke(e,n,"linePrefix");e.consume(t)},function(t){return e.enter("paragraph"),r(t)});return n;function r(n){let r=e.enter("chunkText",{contentType:"text",previous:t});return t&&(t.next=r),t=r,a(n)}function a(t){return null===t?(e.exit("chunkText"),e.exit("paragraph"),void e.consume(t)):Ne(t)?(e.consume(t),e.exit("chunkText"),r):(e.consume(t),a)}}},Ie={tokenize:function(e){let t,n,r,a=this,o=[],i=0;return l;function l(t){if(i<o.length){let n=o[i];return a.containerState=n[1],e.attempt(n[0].continuation,s,c)(t)}return c(t)}function s(e){if(i++,a.containerState._closeFlow){a.containerState._closeFlow=void 0,t&&v();let n,r=a.events.length,o=r;for(;o--;)if("exit"===a.events[o][0]&&"chunkFlow"===a.events[o][1].type){n=a.events[o][1].end;break}y(i);let l=r;for(;l<a.events.length;)a.events[l][1].end=Object.assign({},n),l++;return ue(a.events,o+1,0,a.events.slice(r)),a.events.length=l,c(e)}return l(e)}function c(n){if(i===o.length){if(!t)return d(n);if(t.currentConstruct&&t.currentConstruct.concrete)return m(n);a.interrupt=!(!t.currentConstruct||t._gfmTableDynamicInterruptHack)}return a.containerState={},e.check(Le,u,f)(n)}function u(e){return t&&v(),y(i),d(e)}function f(e){return a.parser.lazy[a.now().line]=i!==o.length,r=a.now().offset,m(e)}function d(t){return a.containerState={},e.attempt(Le,p,m)(t)}function p(e){return i++,o.push([a.currentConstruct,a.containerState]),d(e)}function m(r){return null===r?(t&&v(),y(0),void e.consume(r)):(t=t||a.parser.flow(a.now()),e.enter("chunkFlow",{contentType:"flow",previous:n,_tokenizer:t}),h(r))}function h(t){return null===t?(g(e.exit("chunkFlow"),!0),y(0),void e.consume(t)):Ne(t)?(e.consume(t),g(e.exit("chunkFlow")),i=0,a.interrupt=void 0,l):(e.consume(t),h)}function g(e,o){let l=a.sliceStream(e);if(o&&l.push(null),e.previous=n,n&&(n.next=e),n=e,t.defineSkip(e.start),t.write(l),a.parser.lazy[e.start.line]){let e=t.events.length;for(;e--;)if(t.events[e][1].start.offset<r&&(!t.events[e][1].end||t.events[e][1].end.offset>r))return;let n,o,l=a.events.length,s=l;for(;s--;)if("exit"===a.events[s][0]&&"chunkFlow"===a.events[s][1].type){if(n){o=a.events[s][1].end;break}n=!0}for(y(i),e=l;e<a.events.length;)a.events[e][1].end=Object.assign({},o),e++;ue(a.events,s+1,0,a.events.slice(l)),a.events.length=e}}function y(t){let n=o.length;for(;n-- >t;){let t=o[n];a.containerState=t[1],t[0].exit.call(a,e)}o.length=t}function v(){t.write([null]),n=void 0,t=void 0,a.containerState._closeFlow=void 0}}},Le={tokenize:function(e,t,n){return ke(e,e.attempt(this.parser.constructs.document,t,n),"linePrefix",this.parser.constructs.disable.null.includes("codeIndented")?void 0:4)}};function _e(e){return null===e||we(e)||Oe(e)?1:Ce(e)?2:void 0}function Pe(e,t,n){let r=[],a=-1;for(;++a<e.length;){let o=e[a].resolveAll;o&&!r.includes(o)&&(t=o(t,n),r.push(o))}return t}var Me={name:"attention",tokenize:function(e,t){let n,r=this.parser.constructs.attentionMarkers.null,a=this.previous,o=_e(a);return function(t){return e.enter("attentionSequence"),n=t,i(t)};function i(l){if(l===n)return e.consume(l),i;let s=e.exit("attentionSequence"),c=_e(l),u=!c||2===c&&o||r.includes(l),f=!o||2===o&&c||r.includes(a);return s._open=!!(42===n?u:u&&(o||!f)),s._close=!!(42===n?f:f&&(c||!u)),t(l)}},resolveAll:function(e,t){let n,r,a,o,i,l,s,c,u=-1;for(;++u<e.length;)if("enter"===e[u][0]&&"attentionSequence"===e[u][1].type&&e[u][1]._close)for(n=u;n--;)if("exit"===e[n][0]&&"attentionSequence"===e[n][1].type&&e[n][1]._open&&t.sliceSerialize(e[n][1]).charCodeAt(0)===t.sliceSerialize(e[u][1]).charCodeAt(0)){if((e[n][1]._close||e[u][1]._open)&&(e[u][1].end.offset-e[u][1].start.offset)%3&&!((e[n][1].end.offset-e[n][1].start.offset+e[u][1].end.offset-e[u][1].start.offset)%3))continue;l=e[n][1].end.offset-e[n][1].start.offset>1&&e[u][1].end.offset-e[u][1].start.offset>1?2:1;let f=Object.assign({},e[n][1].end),d=Object.assign({},e[u][1].start);De(f,-l),De(d,l),o={type:l>1?"strongSequence":"emphasisSequence",start:f,end:Object.assign({},e[n][1].end)},i={type:l>1?"strongSequence":"emphasisSequence",start:Object.assign({},e[u][1].start),end:d},a={type:l>1?"strongText":"emphasisText",start:Object.assign({},e[n][1].end),end:Object.assign({},e[u][1].start)},r={type:l>1?"strong":"emphasis",start:Object.assign({},o.start),end:Object.assign({},i.end)},e[n][1].end=Object.assign({},o.start),e[u][1].start=Object.assign({},i.end),s=[],e[n][1].end.offset-e[n][1].start.offset&&(s=fe(s,[["enter",e[n][1],t],["exit",e[n][1],t]])),s=fe(s,[["enter",r,t],["enter",o,t],["exit",o,t],["enter",a,t]]),s=fe(s,Pe(t.parser.constructs.insideSpan.null,e.slice(n+1,u),t)),s=fe(s,[["exit",a,t],["enter",i,t],["exit",i,t],["exit",r,t]]),e[u][1].end.offset-e[u][1].start.offset?(c=2,s=fe(s,[["enter",e[u][1],t],["exit",e[u][1],t]])):c=0,ue(e,n-1,u-n+3,s),u=n+s.length-c-2;break}for(u=-1;++u<e.length;)"attentionSequence"===e[u][1].type&&(e[u][1].type="data");return e}};function De(e,t){e.column+=t,e.offset+=t,e._bufferIndex+=t}var Ue={name:"autolink",tokenize:function(e,t,n){let r=1;return function(t){return e.enter("autolink"),e.enter("autolinkMarker"),e.consume(t),e.exit("autolinkMarker"),e.enter("autolinkProtocol"),a};function a(t){return ge(t)?(e.consume(t),o):Te(t)?s(t):n(t)}function o(e){return 43===e||45===e||46===e||be(e)?i(e):s(e)}function i(t){return 58===t?(e.consume(t),l):(43===t||45===t||46===t||be(t))&&r++<32?(e.consume(t),i):s(t)}function l(t){return 62===t?(e.exit("autolinkProtocol"),d(t)):null===t||32===t||60===t||Se(t)?n(t):(e.consume(t),l)}function s(t){return 64===t?(e.consume(t),r=0,c):Te(t)?(e.consume(t),s):n(t)}function c(e){return be(e)?u(e):n(e)}function u(t){return 46===t?(e.consume(t),r=0,c):62===t?(e.exit("autolinkProtocol").type="autolinkEmail",d(t)):f(t)}function f(t){return(45===t||be(t))&&r++<63?(e.consume(t),45===t?f:u):n(t)}function d(n){return e.enter("autolinkMarker"),e.consume(n),e.exit("autolinkMarker"),e.exit("autolink"),t}}},je={tokenize:function(e,t,n){return ke(e,function(e){return null===e||Ne(e)?t(e):n(e)},"linePrefix")},partial:!0},Fe={name:"blockQuote",tokenize:function(e,t,n){let r=this;return function(t){if(62===t){let n=r.containerState;return n.open||(e.enter("blockQuote",{_container:!0}),n.open=!0),e.enter("blockQuotePrefix"),e.enter("blockQuoteMarker"),e.consume(t),e.exit("blockQuoteMarker"),a}return n(t)};function a(n){return Re(n)?(e.enter("blockQuotePrefixWhitespace"),e.consume(n),e.exit("blockQuotePrefixWhitespace"),e.exit("blockQuotePrefix"),t):(e.exit("blockQuotePrefix"),t(n))}},continuation:{tokenize:function(e,t,n){return ke(e,e.attempt(Fe,t,n),"linePrefix",this.parser.constructs.disable.null.includes("codeIndented")?void 0:4)}},exit:function(e){e.exit("blockQuote")}},ze={name:"characterEscape",tokenize:function(e,t,n){return function(t){return e.enter("characterEscape"),e.enter("escapeMarker"),e.consume(t),e.exit("escapeMarker"),r};function r(r){return Ee(r)?(e.enter("characterEscapeValue"),e.consume(r),e.exit("characterEscapeValue"),e.exit("characterEscape"),t):n(r)}}},Be=document.createElement("i");function He(e){let t="&"+e+";";Be.innerHTML=t;let n=Be.textContent;return(59!==n.charCodeAt(n.length-1)||"semi"===e)&&n!==t&&n}var Ve={name:"characterReference",tokenize:function(e,t,n){let r,a,o=this,i=0;return function(t){return e.enter("characterReference"),e.enter("characterReferenceMarker"),e.consume(t),e.exit("characterReferenceMarker"),l};function l(t){return 35===t?(e.enter("characterReferenceMarkerNumeric"),e.consume(t),e.exit("characterReferenceMarkerNumeric"),s):(e.enter("characterReferenceValue"),r=31,a=be,c(t))}function s(t){return 88===t||120===t?(e.enter("characterReferenceMarkerHexadecimal"),e.consume(t),e.exit("characterReferenceMarkerHexadecimal"),e.enter("characterReferenceValue"),r=6,a=ve,c):(e.enter("characterReferenceValue"),r=7,a=ye,c(t))}function c(l){let s;return 59===l&&i?(s=e.exit("characterReferenceValue"),a!==be||He(o.sliceSerialize(s))?(e.enter("characterReferenceMarker"),e.consume(l),e.exit("characterReferenceMarker"),e.exit("characterReference"),t):n(l)):a(l)&&i++<r?(e.consume(l),c):n(l)}}},We={name:"codeFenced",tokenize:function(e,t,n){let r,a=this,o={tokenize:function(e,t,n){let a=0;return ke(e,function(t){return e.enter("codeFencedFence"),e.enter("codeFencedFenceSequence"),o(t)},"linePrefix",this.parser.constructs.disable.null.includes("codeIndented")?void 0:4);function o(t){return t===r?(e.consume(t),a++,o):a<c?n(t):(e.exit("codeFencedFenceSequence"),ke(e,i,"whitespace")(t))}function i(r){return null===r||Ne(r)?(e.exit("codeFencedFence"),t(r)):n(r)}},partial:!0},i={tokenize:function(e,t,n){let r=this;return function(t){return e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),a};function a(e){return r.parser.lazy[r.now().line]?n(e):t(e)}},partial:!0},l=this.events[this.events.length-1],s=l&&"linePrefix"===l[1].type?l[2].sliceSerialize(l[1],!0).length:0,c=0;return function(t){return e.enter("codeFenced"),e.enter("codeFencedFence"),e.enter("codeFencedFenceSequence"),r=t,u(t)};function u(t){return t===r?(e.consume(t),c++,u):(e.exit("codeFencedFenceSequence"),c<3?n(t):ke(e,f,"whitespace")(t))}function f(t){return null===t||Ne(t)?h(t):(e.enter("codeFencedFenceInfo"),e.enter("chunkString",{contentType:"string"}),d(t))}function d(t){return null===t||we(t)?(e.exit("chunkString"),e.exit("codeFencedFenceInfo"),ke(e,p,"whitespace")(t)):96===t&&t===r?n(t):(e.consume(t),d)}function p(t){return null===t||Ne(t)?h(t):(e.enter("codeFencedFenceMeta"),e.enter("chunkString",{contentType:"string"}),m(t))}function m(t){return null===t||Ne(t)?(e.exit("chunkString"),e.exit("codeFencedFenceMeta"),h(t)):96===t&&t===r?n(t):(e.consume(t),m)}function h(n){return e.exit("codeFencedFence"),a.interrupt?t(n):g(n)}function g(t){return null===t?v(t):Ne(t)?e.attempt(i,e.attempt(o,v,s?ke(e,g,"linePrefix",s+1):g),v)(t):(e.enter("codeFlowValue"),y(t))}function y(t){return null===t||Ne(t)?(e.exit("codeFlowValue"),g(t)):(e.consume(t),y)}function v(n){return e.exit("codeFenced"),t(n)}},concrete:!0},Ge={name:"codeIndented",tokenize:function(e,t,n){let r=this;return function(t){return e.enter("codeIndented"),ke(e,a,"linePrefix",5)(t)};function a(e){let t=r.events[r.events.length-1];return t&&"linePrefix"===t[1].type&&t[2].sliceSerialize(t[1],!0).length>=4?o(e):n(e)}function o(t){return null===t?l(t):Ne(t)?e.attempt(Ye,o,l)(t):(e.enter("codeFlowValue"),i(t))}function i(t){return null===t||Ne(t)?(e.exit("codeFlowValue"),o(t)):(e.consume(t),i)}function l(n){return e.exit("codeIndented"),t(n)}}},Ye={tokenize:function(e,t,n){let r=this;return a;function a(t){return r.parser.lazy[r.now().line]?n(t):Ne(t)?(e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),a):ke(e,o,"linePrefix",5)(t)}function o(e){let o=r.events[r.events.length-1];return o&&"linePrefix"===o[1].type&&o[2].sliceSerialize(o[1],!0).length>=4?t(e):Ne(e)?a(e):n(e)}},partial:!0},$e={name:"codeText",tokenize:function(e,t,n){let r,a,o=0;return function(t){return e.enter("codeText"),e.enter("codeTextSequence"),i(t)};function i(t){return 96===t?(e.consume(t),o++,i):(e.exit("codeTextSequence"),l(t))}function l(t){return null===t?n(t):96===t?(a=e.enter("codeTextSequence"),r=0,c(t)):32===t?(e.enter("space"),e.consume(t),e.exit("space"),l):Ne(t)?(e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),l):(e.enter("codeTextData"),s(t))}function s(t){return null===t||32===t||96===t||Ne(t)?(e.exit("codeTextData"),l(t)):(e.consume(t),s)}function c(n){return 96===n?(e.consume(n),r++,c):r===o?(e.exit("codeTextSequence"),e.exit("codeText"),t(n)):(a.type="codeTextData",s(n))}},resolve:function(e){let t,n,r=e.length-4,a=3;if(!("lineEnding"!==e[a][1].type&&"space"!==e[a][1].type||"lineEnding"!==e[r][1].type&&"space"!==e[r][1].type))for(t=a;++t<r;)if("codeTextData"===e[t][1].type){e[a][1].type="codeTextPadding",e[r][1].type="codeTextPadding",a+=2,r-=2;break}for(t=a-1,r++;++t<=r;)void 0===n?t!==r&&"lineEnding"!==e[t][1].type&&(n=t):(t===r||"lineEnding"===e[t][1].type)&&(e[n][1].type="codeTextData",t!==n+2&&(e[n][1].end=e[t-1][1].end,e.splice(n+2,t-n-2),r-=t-n-2,t=n+2),n=void 0);return e},previous:function(e){return 96!==e||"characterEscape"===this.events[this.events.length-1][1].type}};function Xe(e){let t,n,r,a,o,i,l,s={},c=-1;for(;++c<e.length;){for(;c in s;)c=s[c];if(t=e[c],c&&"chunkFlow"===t[1].type&&"listItemPrefix"===e[c-1][1].type&&(i=t[1]._tokenizer.events,r=0,r<i.length&&"lineEndingBlank"===i[r][1].type&&(r+=2),r<i.length&&"content"===i[r][1].type))for(;++r<i.length&&"content"!==i[r][1].type;)"chunkText"===i[r][1].type&&(i[r][1]._isInFirstContentOfListItem=!0,r++);if("enter"===t[0])t[1].contentType&&(Object.assign(s,qe(e,c)),c=s[c],l=!0);else if(t[1]._container){for(r=c,n=void 0;r--&&(a=e[r],"lineEnding"===a[1].type||"lineEndingBlank"===a[1].type);)"enter"===a[0]&&(n&&(e[n][1].type="lineEndingBlank"),a[1].type="lineEnding",n=r);n&&(t[1].end=Object.assign({},e[n][1].start),o=e.slice(n,c),o.unshift(t),ue(e,n,c-n+1,o))}}return!l}function qe(e,t){let n,r,a=e[t][1],o=e[t][2],i=t-1,l=[],s=a._tokenizer||o.parser[a.contentType](a.start),c=s.events,u=[],f={},d=-1,p=a,m=0,h=0,g=[h];for(;p;){for(;e[++i][1]!==p;);l.push(i),p._tokenizer||(n=o.sliceStream(p),p.next||n.push(null),r&&s.defineSkip(p.start),p._isInFirstContentOfListItem&&(s._gfmTasklistFirstContentOfListItem=!0),s.write(n),p._isInFirstContentOfListItem&&(s._gfmTasklistFirstContentOfListItem=void 0)),r=p,p=p.next}for(p=a;++d<c.length;)"exit"===c[d][0]&&"enter"===c[d-1][0]&&c[d][1].type===c[d-1][1].type&&c[d][1].start.line!==c[d][1].end.line&&(h=d+1,g.push(h),p._tokenizer=void 0,p.previous=void 0,p=p.next);for(s.events=[],p?(p._tokenizer=void 0,p.previous=void 0):g.pop(),d=g.length;d--;){let t=c.slice(g[d],g[d+1]),n=l.pop();u.unshift([n,n+t.length-1]),ue(e,n,2,t)}for(d=-1;++d<u.length;)f[m+u[d][0]]=m+u[d][1],m+=u[d][1]-u[d][0]-1;return f}var Ke={tokenize:function(e,t){let n;return function(t){return e.enter("content"),n=e.enter("chunkContent",{contentType:"content"}),r(t)};function r(t){return null===t?a(t):Ne(t)?e.check(Je,o,a)(t):(e.consume(t),r)}function a(n){return e.exit("chunkContent"),e.exit("content"),t(n)}function o(t){return e.consume(t),e.exit("chunkContent"),n.next=e.enter("chunkContent",{contentType:"content",previous:n}),n=n.next,r}},resolve:function(e){return Xe(e),e}},Je={tokenize:function(e,t,n){let r=this;return function(t){return e.exit("chunkContent"),e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),ke(e,a,"linePrefix")};function a(a){if(null===a||Ne(a))return n(a);let o=r.events[r.events.length-1];return!r.parser.constructs.disable.null.includes("codeIndented")&&o&&"linePrefix"===o[1].type&&o[2].sliceSerialize(o[1],!0).length>=4?t(a):e.interrupt(r.parser.constructs.flow,n,t)(a)}},partial:!0};function Qe(e,t,n,r,a,o,i,l,s){let c=s||Number.POSITIVE_INFINITY,u=0;return function(t){return 60===t?(e.enter(r),e.enter(a),e.enter(o),e.consume(t),e.exit(o),f):null===t||41===t||Se(t)?n(t):(e.enter(r),e.enter(i),e.enter(l),e.enter("chunkString",{contentType:"string"}),m(t))};function f(n){return 62===n?(e.enter(o),e.consume(n),e.exit(o),e.exit(a),e.exit(r),t):(e.enter(l),e.enter("chunkString",{contentType:"string"}),d(n))}function d(t){return 62===t?(e.exit("chunkString"),e.exit(l),f(t)):null===t||60===t||Ne(t)?n(t):(e.consume(t),92===t?p:d)}function p(t){return 60===t||62===t||92===t?(e.consume(t),d):d(t)}function m(a){return 40===a?++u>c?n(a):(e.consume(a),m):41===a?u--?(e.consume(a),m):(e.exit("chunkString"),e.exit(l),e.exit(i),e.exit(r),t(a)):null===a||we(a)?u?n(a):(e.exit("chunkString"),e.exit(l),e.exit(i),e.exit(r),t(a)):Se(a)?n(a):(e.consume(a),92===a?h:m)}function h(t){return 40===t||41===t||92===t?(e.consume(t),m):m(t)}}function Ze(e,t,n,r,a,o){let i,l=this,s=0;return function(t){return e.enter(r),e.enter(a),e.consume(t),e.exit(a),e.enter(o),c};function c(f){return null===f||91===f||93===f&&!i||94===f&&!s&&"_hiddenFootnoteSupport"in l.parser.constructs||s>999?n(f):93===f?(e.exit(o),e.enter(a),e.consume(f),e.exit(a),e.exit(r),t):Ne(f)?(e.enter("lineEnding"),e.consume(f),e.exit("lineEnding"),c):(e.enter("chunkString",{contentType:"string"}),u(f))}function u(t){return null===t||91===t||93===t||Ne(t)||s++>999?(e.exit("chunkString"),c(t)):(e.consume(t),i=i||!Re(t),92===t?f:u)}function f(t){return 91===t||92===t||93===t?(e.consume(t),s++,u):u(t)}}function et(e,t,n,r,a,o){let i;return function(t){return e.enter(r),e.enter(a),e.consume(t),e.exit(a),i=40===t?41:t,l};function l(n){return n===i?(e.enter(a),e.consume(n),e.exit(a),e.exit(r),t):(e.enter(o),s(n))}function s(t){return t===i?(e.exit(o),l(i)):null===t?n(t):Ne(t)?(e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),ke(e,s,"linePrefix")):(e.enter("chunkString",{contentType:"string"}),c(t))}function c(t){return t===i||null===t||Ne(t)?(e.exit("chunkString"),s(t)):(e.consume(t),92===t?u:c)}function u(t){return t===i||92===t?(e.consume(t),c):c(t)}}function tt(e,t){let n;return function r(a){return Ne(a)?(e.enter("lineEnding"),e.consume(a),e.exit("lineEnding"),n=!0,r):Re(a)?ke(e,r,n?"linePrefix":"lineSuffix")(a):t(a)}}function nt(e){return e.replace(/[\t\n\r ]+/g," ").replace(/^ | $/g,"").toLowerCase().toUpperCase()}var rt={name:"definition",tokenize:function(e,t,n){let r,a=this;return function(t){return e.enter("definition"),Ze.call(a,e,o,n,"definitionLabel","definitionLabelMarker","definitionLabelString")(t)};function o(t){return r=nt(a.sliceSerialize(a.events[a.events.length-1][1]).slice(1,-1)),58===t?(e.enter("definitionMarker"),e.consume(t),e.exit("definitionMarker"),tt(e,Qe(e,e.attempt(at,ke(e,i,"whitespace"),ke(e,i,"whitespace")),n,"definitionDestination","definitionDestinationLiteral","definitionDestinationLiteralMarker","definitionDestinationRaw","definitionDestinationString"))):n(t)}function i(o){return null===o||Ne(o)?(e.exit("definition"),a.parser.defined.includes(r)||a.parser.defined.push(r),t(o)):n(o)}}},at={tokenize:function(e,t,n){return function(t){return we(t)?tt(e,r)(t):n(t)};function r(t){return 34===t||39===t||40===t?et(e,ke(e,a,"whitespace"),n,"definitionTitle","definitionTitleMarker","definitionTitleString")(t):n(t)}function a(e){return null===e||Ne(e)?t(e):n(e)}},partial:!0},ot={name:"hardBreakEscape",tokenize:function(e,t,n){return function(t){return e.enter("hardBreakEscape"),e.enter("escapeMarker"),e.consume(t),r};function r(r){return Ne(r)?(e.exit("escapeMarker"),e.exit("hardBreakEscape"),t(r)):n(r)}}},it={name:"headingAtx",tokenize:function(e,t,n){let r=this,a=0;return function(t){return e.enter("atxHeading"),e.enter("atxHeadingSequence"),o(t)};function o(l){return 35===l&&a++<6?(e.consume(l),o):null===l||we(l)?(e.exit("atxHeadingSequence"),r.interrupt?t(l):i(l)):n(l)}function i(n){return 35===n?(e.enter("atxHeadingSequence"),l(n)):null===n||Ne(n)?(e.exit("atxHeading"),t(n)):Re(n)?ke(e,i,"whitespace")(n):(e.enter("atxHeadingText"),s(n))}function l(t){return 35===t?(e.consume(t),l):(e.exit("atxHeadingSequence"),i(t))}function s(t){return null===t||35===t||we(t)?(e.exit("atxHeadingText"),i(t)):(e.consume(t),s)}},resolve:function(e,t){let n,r,a=e.length-2,o=3;return"whitespace"===e[o][1].type&&(o+=2),a-2>o&&"whitespace"===e[a][1].type&&(a-=2),"atxHeadingSequence"===e[a][1].type&&(o===a-1||a-4>o&&"whitespace"===e[a-2][1].type)&&(a-=o+1===a?2:4),a>o&&(n={type:"atxHeadingText",start:e[o][1].start,end:e[a][1].end},r={type:"chunkText",start:e[o][1].start,end:e[a][1].end,contentType:"text"},ue(e,o,a-o+1,[["enter",n,t],["enter",r,t],["exit",r,t],["exit",n,t]])),e}},lt=["address","article","aside","base","basefont","blockquote","body","caption","center","col","colgroup","dd","details","dialog","dir","div","dl","dt","fieldset","figcaption","figure","footer","form","frame","frameset","h1","h2","h3","h4","h5","h6","head","header","hr","html","iframe","legend","li","link","main","menu","menuitem","nav","noframes","ol","optgroup","option","p","param","section","summary","table","tbody","td","tfoot","th","thead","title","tr","track","ul"],st=["pre","script","style","textarea"],ct={name:"htmlFlow",tokenize:function(e,t,n){let r,a,o,i,l,s=this;return function(t){return e.enter("htmlFlow"),e.enter("htmlFlowData"),e.consume(t),c};function c(i){return 33===i?(e.consume(i),u):47===i?(e.consume(i),p):63===i?(e.consume(i),r=3,s.interrupt?t:P):ge(i)?(e.consume(i),o=String.fromCharCode(i),a=!0,m):n(i)}function u(a){return 45===a?(e.consume(a),r=2,f):91===a?(e.consume(a),r=5,o="CDATA[",i=0,d):ge(a)?(e.consume(a),r=4,s.interrupt?t:P):n(a)}function f(r){return 45===r?(e.consume(r),s.interrupt?t:P):n(r)}function d(r){return r===o.charCodeAt(i++)?(e.consume(r),i===o.length?s.interrupt?t:O:d):n(r)}function p(t){return ge(t)?(e.consume(t),o=String.fromCharCode(t),m):n(t)}function m(i){return null===i||47===i||62===i||we(i)?47!==i&&a&&st.includes(o.toLowerCase())?(r=1,s.interrupt?t(i):O(i)):lt.includes(o.toLowerCase())?(r=6,47===i?(e.consume(i),h):s.interrupt?t(i):O(i)):(r=7,s.interrupt&&!s.parser.lazy[s.now().line]?n(i):a?y(i):g(i)):45===i||be(i)?(e.consume(i),o+=String.fromCharCode(i),m):n(i)}function h(r){return 62===r?(e.consume(r),s.interrupt?t:O):n(r)}function g(t){return Re(t)?(e.consume(t),g):N(t)}function y(t){return 47===t?(e.consume(t),N):58===t||95===t||ge(t)?(e.consume(t),v):Re(t)?(e.consume(t),y):N(t)}function v(t){return 45===t||46===t||58===t||95===t||be(t)?(e.consume(t),v):b(t)}function b(t){return 61===t?(e.consume(t),E):Re(t)?(e.consume(t),b):y(t)}function E(t){return null===t||60===t||61===t||62===t||96===t?n(t):34===t||39===t?(e.consume(t),l=t,T):Re(t)?(e.consume(t),E):(l=null,S(t))}function T(t){return null===t||Ne(t)?n(t):t===l?(e.consume(t),w):(e.consume(t),T)}function S(t){return null===t||34===t||39===t||60===t||61===t||62===t||96===t||we(t)?b(t):(e.consume(t),S)}function w(e){return 47===e||62===e||Re(e)?y(e):n(e)}function N(t){return 62===t?(e.consume(t),R):n(t)}function R(t){return Re(t)?(e.consume(t),R):null===t||Ne(t)?O(t):n(t)}function O(t){return 45===t&&2===r?(e.consume(t),A):60===t&&1===r?(e.consume(t),I):62===t&&4===r?(e.consume(t),M):63===t&&3===r?(e.consume(t),P):93===t&&5===r?(e.consume(t),_):!Ne(t)||6!==r&&7!==r?null===t||Ne(t)?C(t):(e.consume(t),O):e.check(ut,M,C)(t)}function C(t){return e.exit("htmlFlowData"),x(t)}function x(t){return null===t?D(t):Ne(t)?e.attempt({tokenize:k,partial:!0},x,D)(t):(e.enter("htmlFlowData"),O(t))}function k(e,t,n){return function(t){return e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),r};function r(e){return s.parser.lazy[s.now().line]?n(e):t(e)}}function A(t){return 45===t?(e.consume(t),P):O(t)}function I(t){return 47===t?(e.consume(t),o="",L):O(t)}function L(t){return 62===t&&st.includes(o.toLowerCase())?(e.consume(t),M):ge(t)&&o.length<8?(e.consume(t),o+=String.fromCharCode(t),L):O(t)}function _(t){return 93===t?(e.consume(t),P):O(t)}function P(t){return 62===t?(e.consume(t),M):45===t&&2===r?(e.consume(t),P):O(t)}function M(t){return null===t||Ne(t)?(e.exit("htmlFlowData"),D(t)):(e.consume(t),M)}function D(n){return e.exit("htmlFlow"),t(n)}},resolveTo:function(e){let t=e.length;for(;t--&&("enter"!==e[t][0]||"htmlFlow"!==e[t][1].type););return t>1&&"linePrefix"===e[t-2][1].type&&(e[t][1].start=e[t-2][1].start,e[t+1][1].start=e[t-2][1].start,e.splice(t-2,2)),e},concrete:!0},ut={tokenize:function(e,t,n){return function(r){return e.exit("htmlFlowData"),e.enter("lineEndingBlank"),e.consume(r),e.exit("lineEndingBlank"),e.attempt(je,t,n)}},partial:!0},ft={name:"htmlText",tokenize:function(e,t,n){let r,a,o,i,l=this;return function(t){return e.enter("htmlText"),e.enter("htmlTextData"),e.consume(t),s};function s(t){return 33===t?(e.consume(t),c):47===t?(e.consume(t),S):63===t?(e.consume(t),E):ge(t)?(e.consume(t),R):n(t)}function c(t){return 45===t?(e.consume(t),u):91===t?(e.consume(t),a="CDATA[",o=0,h):ge(t)?(e.consume(t),b):n(t)}function u(t){return 45===t?(e.consume(t),f):n(t)}function f(t){return null===t||62===t?n(t):45===t?(e.consume(t),d):p(t)}function d(e){return null===e||62===e?n(e):p(e)}function p(t){return null===t?n(t):45===t?(e.consume(t),m):Ne(t)?(i=p,_(t)):(e.consume(t),p)}function m(t){return 45===t?(e.consume(t),M):p(t)}function h(t){return t===a.charCodeAt(o++)?(e.consume(t),o===a.length?g:h):n(t)}function g(t){return null===t?n(t):93===t?(e.consume(t),y):Ne(t)?(i=g,_(t)):(e.consume(t),g)}function y(t){return 93===t?(e.consume(t),v):g(t)}function v(t){return 62===t?M(t):93===t?(e.consume(t),v):g(t)}function b(t){return null===t||62===t?M(t):Ne(t)?(i=b,_(t)):(e.consume(t),b)}function E(t){return null===t?n(t):63===t?(e.consume(t),T):Ne(t)?(i=E,_(t)):(e.consume(t),E)}function T(e){return 62===e?M(e):E(e)}function S(t){return ge(t)?(e.consume(t),w):n(t)}function w(t){return 45===t||be(t)?(e.consume(t),w):N(t)}function N(t){return Ne(t)?(i=N,_(t)):Re(t)?(e.consume(t),N):M(t)}function R(t){return 45===t||be(t)?(e.consume(t),R):47===t||62===t||we(t)?O(t):n(t)}function O(t){return 47===t?(e.consume(t),M):58===t||95===t||ge(t)?(e.consume(t),C):Ne(t)?(i=O,_(t)):Re(t)?(e.consume(t),O):M(t)}function C(t){return 45===t||46===t||58===t||95===t||be(t)?(e.consume(t),C):x(t)}function x(t){return 61===t?(e.consume(t),k):Ne(t)?(i=x,_(t)):Re(t)?(e.consume(t),x):O(t)}function k(t){return null===t||60===t||61===t||62===t||96===t?n(t):34===t||39===t?(e.consume(t),r=t,A):Ne(t)?(i=k,_(t)):Re(t)?(e.consume(t),k):(e.consume(t),r=void 0,L)}function A(t){return t===r?(e.consume(t),I):null===t?n(t):Ne(t)?(i=A,_(t)):(e.consume(t),A)}function I(e){return 62===e||47===e||we(e)?O(e):n(e)}function L(t){return null===t||34===t||39===t||60===t||61===t||96===t?n(t):62===t||we(t)?O(t):(e.consume(t),L)}function _(t){return e.exit("htmlTextData"),e.enter("lineEnding"),e.consume(t),e.exit("lineEnding"),ke(e,P,"linePrefix",l.parser.constructs.disable.null.includes("codeIndented")?void 0:4)}function P(t){return e.enter("htmlTextData"),i(t)}function M(r){return 62===r?(e.consume(r),e.exit("htmlTextData"),e.exit("htmlText"),t):n(r)}}},dt={name:"labelEnd",tokenize:function(e,t,n){let r,a,o=this,i=o.events.length;for(;i--;)if(("labelImage"===o.events[i][1].type||"labelLink"===o.events[i][1].type)&&!o.events[i][1]._balanced){r=o.events[i][1];break}return function(t){return r?r._inactive?s(t):(a=o.parser.defined.includes(nt(o.sliceSerialize({start:r.end,end:o.now()}))),e.enter("labelEnd"),e.enter("labelMarker"),e.consume(t),e.exit("labelMarker"),e.exit("labelEnd"),l):n(t)};function l(n){return 40===n?e.attempt(pt,t,a?t:s)(n):91===n?e.attempt(mt,t,a?e.attempt(ht,t,s):s)(n):a?t(n):s(n)}function s(e){return r._balanced=!0,n(e)}},resolveTo:function(e,t){let n,r,a,o,i=e.length,l=0;for(;i--;)if(n=e[i][1],r){if("link"===n.type||"labelLink"===n.type&&n._inactive)break;"enter"===e[i][0]&&"labelLink"===n.type&&(n._inactive=!0)}else if(a){if("enter"===e[i][0]&&("labelImage"===n.type||"labelLink"===n.type)&&!n._balanced&&(r=i,"labelLink"!==n.type)){l=2;break}}else"labelEnd"===n.type&&(a=i);let s={type:"labelLink"===e[r][1].type?"link":"image",start:Object.assign({},e[r][1].start),end:Object.assign({},e[e.length-1][1].end)},c={type:"label",start:Object.assign({},e[r][1].start),end:Object.assign({},e[a][1].end)},u={type:"labelText",start:Object.assign({},e[r+l+2][1].end),end:Object.assign({},e[a-2][1].start)};return o=[["enter",s,t],["enter",c,t]],o=fe(o,e.slice(r+1,r+l+3)),o=fe(o,[["enter",u,t]]),o=fe(o,Pe(t.parser.constructs.insideSpan.null,e.slice(r+l+4,a-3),t)),o=fe(o,[["exit",u,t],e[a-2],e[a-1],["exit",c,t]]),o=fe(o,e.slice(a+1)),o=fe(o,[["exit",s,t]]),ue(e,r,e.length,o),e},resolveAll:function(e){let t,n=-1;for(;++n<e.length;)t=e[n][1],("labelImage"===t.type||"labelLink"===t.type||"labelEnd"===t.type)&&(e.splice(n+1,"labelImage"===t.type?4:2),t.type="data",n++);return e}},pt={tokenize:function(e,t,n){return function(t){return e.enter("resource"),e.enter("resourceMarker"),e.consume(t),e.exit("resourceMarker"),tt(e,r)};function r(t){return 41===t?i(t):Qe(e,a,n,"resourceDestination","resourceDestinationLiteral","resourceDestinationLiteralMarker","resourceDestinationRaw","resourceDestinationString",32)(t)}function a(t){return we(t)?tt(e,o)(t):i(t)}function o(t){return 34===t||39===t||40===t?et(e,tt(e,i),n,"resourceTitle","resourceTitleMarker","resourceTitleString")(t):i(t)}function i(r){return 41===r?(e.enter("resourceMarker"),e.consume(r),e.exit("resourceMarker"),e.exit("resource"),t):n(r)}}},mt={tokenize:function(e,t,n){let r=this;return function(t){return Ze.call(r,e,a,n,"reference","referenceMarker","referenceString")(t)};function a(e){return r.parser.defined.includes(nt(r.sliceSerialize(r.events[r.events.length-1][1]).slice(1,-1)))?t(e):n(e)}}},ht={tokenize:function(e,t,n){return function(t){return e.enter("reference"),e.enter("referenceMarker"),e.consume(t),e.exit("referenceMarker"),r};function r(r){return 93===r?(e.enter("referenceMarker"),e.consume(r),e.exit("referenceMarker"),e.exit("reference"),t):n(r)}}},gt={name:"labelStartImage",tokenize:function(e,t,n){let r=this;return function(t){return e.enter("labelImage"),e.enter("labelImageMarker"),e.consume(t),e.exit("labelImageMarker"),a};function a(t){return 91===t?(e.enter("labelMarker"),e.consume(t),e.exit("labelMarker"),e.exit("labelImage"),o):n(t)}function o(e){return 94===e&&"_hiddenFootnoteSupport"in r.parser.constructs?n(e):t(e)}},resolveAll:dt.resolveAll},yt={name:"labelStartLink",tokenize:function(e,t,n){let r=this;return function(t){return e.enter("labelLink"),e.enter("labelMarker"),e.consume(t),e.exit("labelMarker"),e.exit("labelLink"),a};function a(e){return 94===e&&"_hiddenFootnoteSupport"in r.parser.constructs?n(e):t(e)}},resolveAll:dt.resolveAll},vt={name:"lineEnding",tokenize:function(e,t){return function(n){return e.enter("lineEnding"),e.consume(n),e.exit("lineEnding"),ke(e,t,"linePrefix")}}},bt={name:"thematicBreak",tokenize:function(e,t,n){let r,a=0;return function(t){return e.enter("thematicBreak"),r=t,o(t)};function o(l){return l===r?(e.enter("thematicBreakSequence"),i(l)):Re(l)?ke(e,o,"whitespace")(l):a<3||null!==l&&!Ne(l)?n(l):(e.exit("thematicBreak"),t(l))}function i(t){return t===r?(e.consume(t),a++,i):(e.exit("thematicBreakSequence"),o(t))}}},Et={name:"list",tokenize:function(e,t,n){let r=this,a=r.events[r.events.length-1],o=a&&"linePrefix"===a[1].type?a[2].sliceSerialize(a[1],!0).length:0,i=0;return function(t){let a=r.containerState.type||(42===t||43===t||45===t?"listUnordered":"listOrdered");if("listUnordered"===a?!r.containerState.marker||t===r.containerState.marker:ye(t)){if(r.containerState.type||(r.containerState.type=a,e.enter(a,{_container:!0})),"listUnordered"===a)return e.enter("listItemPrefix"),42===t||45===t?e.check(bt,n,s)(t):s(t);if(!r.interrupt||49===t)return e.enter("listItemPrefix"),e.enter("listItemValue"),l(t)}return n(t)};function l(t){return ye(t)&&++i<10?(e.consume(t),l):(!r.interrupt||i<2)&&(r.containerState.marker?t===r.containerState.marker:41===t||46===t)?(e.exit("listItemValue"),s(t)):n(t)}function s(t){return e.enter("listItemMarker"),e.consume(t),e.exit("listItemMarker"),r.containerState.marker=r.containerState.marker||t,e.check(je,r.interrupt?n:c,e.attempt(Tt,f,u))}function c(e){return r.containerState.initialBlankLine=!0,o++,f(e)}function u(t){return Re(t)?(e.enter("listItemPrefixWhitespace"),e.consume(t),e.exit("listItemPrefixWhitespace"),f):n(t)}function f(n){return r.containerState.size=o+r.sliceSerialize(e.exit("listItemPrefix"),!0).length,t(n)}},continuation:{tokenize:function(e,t,n){let r=this;return r.containerState._closeFlow=void 0,e.check(je,function(n){return r.containerState.furtherBlankLines=r.containerState.furtherBlankLines||r.containerState.initialBlankLine,ke(e,t,"listItemIndent",r.containerState.size+1)(n)},function(n){return r.containerState.furtherBlankLines||!Re(n)?(r.containerState.furtherBlankLines=void 0,r.containerState.initialBlankLine=void 0,a(n)):(r.containerState.furtherBlankLines=void 0,r.containerState.initialBlankLine=void 0,e.attempt(St,t,a)(n))});function a(a){return r.containerState._closeFlow=!0,r.interrupt=void 0,ke(e,e.attempt(Et,t,n),"linePrefix",r.parser.constructs.disable.null.includes("codeIndented")?void 0:4)(a)}}},exit:function(e){e.exit(this.containerState.type)}},Tt={tokenize:function(e,t,n){let r=this;return ke(e,function(e){let a=r.events[r.events.length-1];return!Re(e)&&a&&"listItemPrefixWhitespace"===a[1].type?t(e):n(e)},"listItemPrefixWhitespace",r.parser.constructs.disable.null.includes("codeIndented")?void 0:5)},partial:!0},St={tokenize:function(e,t,n){let r=this;return ke(e,function(e){let a=r.events[r.events.length-1];return a&&"listItemIndent"===a[1].type&&a[2].sliceSerialize(a[1],!0).length===r.containerState.size?t(e):n(e)},"listItemIndent",r.containerState.size+1)},partial:!0},wt={name:"setextUnderline",tokenize:function(e,t,n){let r,a,o=this,i=o.events.length;for(;i--;)if("lineEnding"!==o.events[i][1].type&&"linePrefix"!==o.events[i][1].type&&"content"!==o.events[i][1].type){a="paragraph"===o.events[i][1].type;break}return function(t){return o.parser.lazy[o.now().line]||!o.interrupt&&!a?n(t):(e.enter("setextHeadingLine"),e.enter("setextHeadingLineSequence"),r=t,l(t))};function l(t){return t===r?(e.consume(t),l):(e.exit("setextHeadingLineSequence"),ke(e,s,"lineSuffix")(t))}function s(r){return null===r||Ne(r)?(e.exit("setextHeadingLine"),t(r)):n(r)}},resolveTo:function(e,t){let n,r,a,o=e.length;for(;o--;)if("enter"===e[o][0]){if("content"===e[o][1].type){n=o;break}"paragraph"===e[o][1].type&&(r=o)}else"content"===e[o][1].type&&e.splice(o,1),!a&&"definition"===e[o][1].type&&(a=o);let i={type:"setextHeading",start:Object.assign({},e[r][1].start),end:Object.assign({},e[e.length-1][1].end)};return e[r][1].type="setextHeadingText",a?(e.splice(r,0,["enter",i,t]),e.splice(a+1,0,["exit",e[n][1],t]),e[n][1].end=Object.assign({},e[a][1].end)):e[n][1]=i,e.push(["exit",i,t]),e}},Nt={tokenize:function(e){let t=this,n=e.attempt(je,function(r){if(null!==r)return e.enter("lineEndingBlank"),e.consume(r),e.exit("lineEndingBlank"),t.currentConstruct=void 0,n;e.consume(r)},e.attempt(this.parser.constructs.flowInitial,r,ke(e,e.attempt(this.parser.constructs.flow,r,e.attempt(Ke,r)),"linePrefix")));return n;function r(r){if(null!==r)return e.enter("lineEnding"),e.consume(r),e.exit("lineEnding"),t.currentConstruct=void 0,n;e.consume(r)}}},Rt={resolveAll:kt()},Ot=xt("string"),Ct=xt("text");function xt(e){return{tokenize:function(t){let n=this,r=this.parser.constructs[e],a=t.attempt(r,o,i);return o;function o(e){return s(e)?a(e):i(e)}function i(e){if(null!==e)return t.enter("data"),t.consume(e),l;t.consume(e)}function l(e){return s(e)?(t.exit("data"),a(e)):(t.consume(e),l)}function s(e){if(null===e)return!0;let t=r[e],a=-1;if(t)for(;++a<t.length;){let e=t[a];if(!e.previous||e.previous.call(n,n.previous))return!0}return!1}},resolveAll:kt("text"===e?At:void 0)}}function kt(e){return function(t,n){let r,a=-1;for(;++a<=t.length;)void 0===r?t[a]&&"data"===t[a][1].type&&(r=a,a++):(!t[a]||"data"!==t[a][1].type)&&(a!==r+2&&(t[r][1].end=t[a-1][1].end,t.splice(r+2,a-r-2),a=r+2),r=void 0);return e?e(t,n):t}}function At(e,t){let n=0;for(;++n<=e.length;)if((n===e.length||"lineEnding"===e[n][1].type)&&"data"===e[n-1][1].type){let r,a=e[n-1][1],o=t.sliceStream(a),i=o.length,l=-1,s=0;for(;i--;){let e=o[i];if("string"==typeof e){for(l=e.length;32===e.charCodeAt(l-1);)s++,l--;if(l)break;l=-1}else if(-2===e)r=!0,s++;else if(-1!==e){i++;break}}if(s){let o={type:n===e.length||r||s<2?"lineSuffix":"hardBreakTrailing",start:{line:a.end.line,column:a.end.column-s,offset:a.end.offset-s,_index:a.start._index+i,_bufferIndex:i?l:a.start._bufferIndex+l},end:Object.assign({},a.end)};a.end=Object.assign({},o.start),a.start.offset===a.end.offset?Object.assign(a,o):(e.splice(n,0,["enter",o,t],["exit",o,t]),n+=2)}n++}return e}var It={};h(It,{attentionMarkers:()=>Ft,contentInitial:()=>_t,disable:()=>zt,document:()=>Lt,flow:()=>Mt,flowInitial:()=>Pt,insideSpan:()=>jt,string:()=>Dt,text:()=>Ut});var Lt={42:Et,43:Et,45:Et,48:Et,49:Et,50:Et,51:Et,52:Et,53:Et,54:Et,55:Et,56:Et,57:Et,62:Fe},_t={91:rt},Pt={[-2]:Ge,[-1]:Ge,32:Ge},Mt={35:it,42:bt,45:[wt,bt],60:ct,61:wt,95:bt,96:We,126:We},Dt={38:Ve,92:ze},Ut={[-5]:vt,[-4]:vt,[-3]:vt,33:gt,38:Ve,42:Me,60:[Ue,ft],91:yt,92:[ot,ze],93:dt,95:Me,96:$e},jt={null:[Me,Rt]},Ft={null:[42,95]},zt={null:[]},Bt=/[\0\t\n\r]/g;function Ht(e,t){let n=Number.parseInt(e,t);return n<9||11===n||n>13&&n<32||n>126&&n<160||n>55295&&n<57344||n>64975&&n<65008||65535==(65535&n)||65534==(65535&n)||n>1114111?"�":String.fromCharCode(n)}var Vt=/\\([!-/:-@[-`{-~])|&(#(?:\d{1,7}|x[\da-f]{1,6})|[\da-z]{1,31});/gi;function Wt(e,t,n){if(t)return t;if(35===n.charCodeAt(0)){let e=n.charCodeAt(1),t=120===e||88===e;return Ht(n.slice(t?2:1),t?16:10)}return He(n)||e}var Gt={}.hasOwnProperty,Yt=function(e,t,n){return"string"!=typeof t&&(n=t,t=void 0),function(e){let t={transforms:[],canContainEols:["emphasis","fragment","heading","paragraph","strong"],enter:{autolink:i(E),autolinkProtocol:d,autolinkEmail:d,atxHeading:i(y),blockQuote:i(function(){return{type:"blockquote",children:[]}}),characterEscape:d,characterReference:d,codeFenced:i(g),codeFencedFenceInfo:l,codeFencedFenceMeta:l,codeIndented:i(g,l),codeText:i(function(){return{type:"inlineCode",value:""}},l),codeTextData:d,data:d,codeFlowValue:d,definition:i(function(){return{type:"definition",identifier:"",label:null,title:null,url:""}}),definitionDestinationString:l,definitionLabelString:l,definitionTitleString:l,emphasis:i(function(){return{type:"emphasis",children:[]}}),hardBreakEscape:i(v),hardBreakTrailing:i(v),htmlFlow:i(b,l),htmlFlowData:d,htmlText:i(b,l),htmlTextData:d,image:i(function(){return{type:"image",title:null,url:"",alt:null}}),label:l,link:i(E),listItem:i(function(e){return{type:"listItem",spread:e._spread,checked:null,children:[]}}),listItemValue:function(e){o("expectingFirstListItemValue")&&(this.stack[this.stack.length-2].start=Number.parseInt(this.sliceSerialize(e),10),a("expectingFirstListItemValue"))},listOrdered:i(T,function(){a("expectingFirstListItemValue",!0)}),listUnordered:i(T),paragraph:i(function(){return{type:"paragraph",children:[]}}),reference:function(){a("referenceType","collapsed")},referenceString:l,resourceDestinationString:l,resourceTitleString:l,setextHeading:i(y),strong:i(function(){return{type:"strong",children:[]}}),thematicBreak:i(function(){return{type:"thematicBreak"}})},exit:{atxHeading:c(),atxHeadingSequence:function(e){let t=this.stack[this.stack.length-1];if(!t.depth){let n=this.sliceSerialize(e).length;t.depth=n}},autolink:c(),autolinkEmail:function(e){p.call(this,e),this.stack[this.stack.length-1].url="mailto:"+this.sliceSerialize(e)},autolinkProtocol:function(e){p.call(this,e),this.stack[this.stack.length-1].url=this.sliceSerialize(e)},blockQuote:c(),characterEscapeValue:p,characterReferenceMarkerHexadecimal:h,characterReferenceMarkerNumeric:h,characterReferenceValue:function(e){let t,n=this.sliceSerialize(e),r=o("characterReferenceType");r?(t=Ht(n,"characterReferenceMarkerNumeric"===r?10:16),a("characterReferenceType")):t=He(n);let i=this.stack.pop();i.value+=t,i.position.end=$t(e.end)},codeFenced:c(function(){let e=this.resume();this.stack[this.stack.length-1].value=e.replace(/^(\r?\n|\r)|(\r?\n|\r)$/g,""),a("flowCodeInside")}),codeFencedFence:function(){o("flowCodeInside")||(this.buffer(),a("flowCodeInside",!0))},codeFencedFenceInfo:function(){let e=this.resume();this.stack[this.stack.length-1].lang=e},codeFencedFenceMeta:function(){let e=this.resume();this.stack[this.stack.length-1].meta=e},codeFlowValue:p,codeIndented:c(function(){let e=this.resume();this.stack[this.stack.length-1].value=e.replace(/(\r?\n|\r)$/g,"")}),codeText:c(function(){let e=this.resume();this.stack[this.stack.length-1].value=e}),codeTextData:p,data:p,definition:c(),definitionDestinationString:function(){let e=this.resume();this.stack[this.stack.length-1].url=e},definitionLabelString:function(e){let t=this.resume(),n=this.stack[this.stack.length-1];n.label=t,n.identifier=nt(this.sliceSerialize(e)).toLowerCase()},definitionTitleString:function(){let e=this.resume();this.stack[this.stack.length-1].title=e},emphasis:c(),hardBreakEscape:c(m),hardBreakTrailing:c(m),htmlFlow:c(function(){let e=this.resume();this.stack[this.stack.length-1].value=e}),htmlFlowData:p,htmlText:c(function(){let e=this.resume();this.stack[this.stack.length-1].value=e}),htmlTextData:p,image:c(function(){let e=this.stack[this.stack.length-1];if(o("inReference")){let t=o("referenceType")||"shortcut";e.type+="Reference",e.referenceType=t,delete e.url,delete e.title}else delete e.identifier,delete e.label;a("referenceType")}),label:function(){let e=this.stack[this.stack.length-1],t=this.resume(),n=this.stack[this.stack.length-1];a("inReference",!0),"link"===n.type?n.children=e.children:n.alt=t},labelText:function(e){let t=this.sliceSerialize(e),n=this.stack[this.stack.length-2];n.label=function(e){return e.replace(Vt,Wt)}(t),n.identifier=nt(t).toLowerCase()},lineEnding:function(e){let n=this.stack[this.stack.length-1];if(o("atHardBreak"))return n.children[n.children.length-1].position.end=$t(e.end),void a("atHardBreak");!o("setextHeadingSlurpLineEnding")&&t.canContainEols.includes(n.type)&&(d.call(this,e),p.call(this,e))},link:c(function(){let e=this.stack[this.stack.length-1];if(o("inReference")){let t=o("referenceType")||"shortcut";e.type+="Reference",e.referenceType=t,delete e.url,delete e.title}else delete e.identifier,delete e.label;a("referenceType")}),listItem:c(),listOrdered:c(),listUnordered:c(),paragraph:c(),referenceString:function(e){let t=this.resume(),n=this.stack[this.stack.length-1];n.label=t,n.identifier=nt(this.sliceSerialize(e)).toLowerCase(),a("referenceType","full")},resourceDestinationString:function(){let e=this.resume();this.stack[this.stack.length-1].url=e},resourceTitleString:function(){let e=this.resume();this.stack[this.stack.length-1].title=e},resource:function(){a("inReference")},setextHeading:c(function(){a("setextHeadingSlurpLineEnding")}),setextHeadingLineSequence:function(e){this.stack[this.stack.length-1].depth=61===this.sliceSerialize(e).charCodeAt(0)?1:2},setextHeadingText:function(){a("setextHeadingSlurpLineEnding",!0)},strong:c(),thematicBreak:c()}};Xt(t,(e||{}).mdastExtensions||[]);let n={};return function(e){let n={type:"root",children:[]},i={stack:[n],tokenStack:[],config:t,enter:s,exit:u,buffer:l,resume:f,setData:a,getData:o},c=[],d=-1;for(;++d<e.length;)"listOrdered"!==e[d][1].type&&"listUnordered"!==e[d][1].type||("enter"===e[d][0]?c.push(d):d=r(e,c.pop(),d));for(d=-1;++d<e.length;){let n=t[e[d][0]];Gt.call(n,e[d][1].type)&&n[e[d][1].type].call(Object.assign({sliceSerialize:e[d][2].sliceSerialize},i),e[d][1])}if(i.tokenStack.length>0){let e=i.tokenStack[i.tokenStack.length-1];(e[1]||Kt).call(i,void 0,e[0])}for(n.position={start:$t(e.length>0?e[0][1].start:{line:1,column:1,offset:0}),end:$t(e.length>0?e[e.length-2][1].end:{line:1,column:1,offset:0})},d=-1;++d<t.transforms.length;)n=t.transforms[d](n)||n;return n};function r(e,t,n){let r,a,o,i,l=t-1,s=-1,c=!1;for(;++l<=n;){let t=e[l];if("listUnordered"===t[1].type||"listOrdered"===t[1].type||"blockQuote"===t[1].type?("enter"===t[0]?s++:s--,i=void 0):"lineEndingBlank"===t[1].type?"enter"===t[0]&&(r&&!i&&!s&&!o&&(o=l),i=void 0):"linePrefix"===t[1].type||"listItemValue"===t[1].type||"listItemMarker"===t[1].type||"listItemPrefix"===t[1].type||"listItemPrefixWhitespace"===t[1].type||(i=void 0),!s&&"enter"===t[0]&&"listItemPrefix"===t[1].type||-1===s&&"exit"===t[0]&&("listUnordered"===t[1].type||"listOrdered"===t[1].type)){if(r){let i=l;for(a=void 0;i--;){let t=e[i];if("lineEnding"===t[1].type||"lineEndingBlank"===t[1].type){if("exit"===t[0])continue;a&&(e[a][1].type="lineEndingBlank",c=!0),t[1].type="lineEnding",a=i}else if("linePrefix"!==t[1].type&&"blockQuotePrefix"!==t[1].type&&"blockQuotePrefixWhitespace"!==t[1].type&&"blockQuoteMarker"!==t[1].type&&"listItemIndent"!==t[1].type)break}o&&(!a||o<a)&&(r._spread=!0),r.end=Object.assign({},a?e[a][1].start:t[1].end),e.splice(a||l,0,["exit",r,t[2]]),l++,n++}"listItemPrefix"===t[1].type&&(r={type:"listItem",_spread:!1,start:Object.assign({},t[1].start)},e.splice(l,0,["enter",r,t[2]]),l++,n++,o=void 0,i=!0)}}return e[t][1]._spread=c,n}function a(e,t){n[e]=t}function o(e){return n[e]}function i(e,t){return function(n){s.call(this,e(n),n),t&&t.call(this,n)}}function l(){this.stack.push({type:"fragment",children:[]})}function s(e,t,n){return this.stack[this.stack.length-1].children.push(e),this.stack.push(e),this.tokenStack.push([t,n]),e.position={start:$t(t.start)},e}function c(e){return function(t){e&&e.call(this,t),u.call(this,t)}}function u(e,t){let n=this.stack.pop(),r=this.tokenStack.pop();if(!r)throw new Error("Cannot close `"+e.type+"` ("+P({start:e.start,end:e.end})+"): it’s not open");return r[0].type!==e.type&&(t?t.call(this,e,r[0]):(r[1]||Kt).call(this,e,r[0])),n.position.end=$t(e.end),n}function f(){return function(e,t){return se(e,"boolean"!=typeof le.includeImageAlt||le.includeImageAlt,"boolean"!=typeof le.includeHtml||le.includeHtml)}(this.stack.pop())}function d(e){let t=this.stack[this.stack.length-1],n=t.children[t.children.length-1];(!n||"text"!==n.type)&&(n={type:"text",value:""},n.position={start:$t(e.start)},t.children.push(n)),this.stack.push(n)}function p(e){let t=this.stack.pop();t.value+=this.sliceSerialize(e),t.position.end=$t(e.end)}function m(){a("atHardBreak",!0)}function h(e){a("characterReferenceType",e.type)}function g(){return{type:"code",lang:null,meta:null,value:""}}function y(){return{type:"heading",depth:void 0,children:[]}}function v(){return{type:"break"}}function b(){return{type:"html",value:""}}function E(){return{type:"link",title:null,url:"",children:[]}}function T(e){return{type:"list",ordered:"listOrdered"===e.type,start:null,spread:e._spread,children:[]}}}(n)(function(e){for(;!Xe(e););return e}(function(e={}){let t={defined:[],lazy:{},constructs:pe([It].concat(e.extensions||[])),content:n(Ae),document:n(Ie),flow:n(Nt),string:n(Ot),text:n(Ct)};return t;function n(e){return function(n){return function(e,t,n){let r=Object.assign(n?Object.assign({},n):{line:1,column:1,offset:0},{_index:0,_bufferIndex:-1}),a={},o=[],i=[],l=[],s={consume:function(e){Ne(e)?(r.line++,r.column=1,r.offset+=-3===e?2:1,y()):-1!==e&&(r.column++,r.offset++),r._bufferIndex<0?r._index++:(r._bufferIndex++,r._bufferIndex===i[r._index].length&&(r._bufferIndex=-1,r._index++)),c.previous=e},enter:function(e,t){let n=t||{};return n.type=e,n.start=d(),c.events.push(["enter",n,c]),l.push(n),n},exit:function(e){let t=l.pop();return t.end=d(),c.events.push(["exit",t,c]),t},attempt:h(function(e,t){g(e,t.from)}),check:h(m),interrupt:h(m,{interrupt:!0})},c={previous:null,code:null,containerState:{},events:[],parser:e,sliceStream:f,sliceSerialize:function(e,t){return function(e,t){let n,r=-1,a=[];for(;++r<e.length;){let o,i=e[r];if("string"==typeof i)o=i;else switch(i){case-5:o="\r";break;case-4:o="\n";break;case-3:o="\r\n";break;case-2:o=t?" ":"\t";break;case-1:if(!t&&n)continue;o=" ";break;default:o=String.fromCharCode(i)}n=-2===i,a.push(o)}return a.join("")}(f(e),t)},now:d,defineSkip:function(e){a[e.line]=e.column,y()},write:function(e){return i=fe(i,e),function(){let e;for(;r._index<i.length;){let t=i[r._index];if("string"==typeof t)for(e=r._index,r._bufferIndex<0&&(r._bufferIndex=0);r._index===e&&r._bufferIndex<t.length;)p(t.charCodeAt(r._bufferIndex));else p(t)}}(),null!==i[i.length-1]?[]:(g(t,0),c.events=Pe(o,c.events,c),c.events)}},u=t.tokenize.call(c,s);return t.resolveAll&&o.push(t),c;function f(e){return function(e,t){let n,r=t.start._index,a=t.start._bufferIndex,o=t.end._index,i=t.end._bufferIndex;return r===o?n=[e[r].slice(a,i)]:(n=e.slice(r,o),a>-1&&(n[0]=n[0].slice(a)),i>0&&n.push(e[o].slice(0,i))),n}(i,e)}function d(){return Object.assign({},r)}function p(e){u=u(e)}function m(e,t){t.restore()}function h(e,t){return function(n,a,o){let i,u,f,p;return Array.isArray(n)?h(n):"tokenize"in n?h([n]):(m=n,function(e){let t=null!==e&&m[e],n=null!==e&&m.null;return h([...Array.isArray(t)?t:t?[t]:[],...Array.isArray(n)?n:n?[n]:[]])(e)});var m;function h(e){return i=e,u=0,0===e.length?o:g(e[u])}function g(e){return function(n){return p=function(){let e=d(),t=c.previous,n=c.currentConstruct,a=c.events.length,o=Array.from(l);return{restore:function(){r=e,c.previous=t,c.currentConstruct=n,c.events.length=a,l=o,y()},from:a}}(),f=e,e.partial||(c.currentConstruct=e),e.name&&c.parser.constructs.disable.null.includes(e.name)?b():e.tokenize.call(t?Object.assign(Object.create(c),t):c,s,v,b)(n)}}function v(t){return e(f,p),a}function b(e){return p.restore(),++u<i.length?g(i[u]):o}}}function g(e,t){e.resolveAll&&!o.includes(e)&&o.push(e),e.resolve&&ue(c.events,t,c.events.length-t,e.resolve(c.events.slice(t),c)),e.resolveTo&&(c.events=e.resolveTo(c.events,c))}function y(){r.line in a&&r.column<2&&(r.column=a[r.line],r.offset+=a[r.line]-1)}}(t,e,n)}}}(n).document().write(function(){let e,t=1,n="",r=!0;return function(a,o,i){let l,s,c,u,f,d=[];for(a=n+a.toString(o),c=0,n="",r&&(65279===a.charCodeAt(0)&&c++,r=void 0);c<a.length;){if(Bt.lastIndex=c,l=Bt.exec(a),u=l&&void 0!==l.index?l.index:a.length,f=a.charCodeAt(u),!l){n=a.slice(c);break}if(10===f&&c===u&&e)d.push(-3),e=void 0;else switch(e&&(d.push(-5),e=void 0),c<u&&(d.push(a.slice(c,u)),t+=u-c),f){case 0:d.push(65533),t++;break;case 9:for(s=4*Math.ceil(t/4),d.push(-2);t++<s;)d.push(-1);break;case 10:d.push(-4),t=1;break;default:e=!0,t=1}c=u+1}return i&&(e&&d.push(-5),n&&d.push(n),d.push(null)),d}}()(e,t,!0))))};function $t(e){return{line:e.line,column:e.column,offset:e.offset}}function Xt(e,t){let n=-1;for(;++n<t.length;){let r=t[n];Array.isArray(r)?Xt(e,r):qt(e,r)}}function qt(e,t){let n;for(n in t)if(Gt.call(t,n))if("canContainEols"===n){let r=t[n];r&&e[n].push(...r)}else if("transforms"===n){let r=t[n];r&&e[n].push(...r)}else if("enter"===n||"exit"===n){let r=t[n];r&&Object.assign(e[n],r)}}function Kt(e,t){throw e?new Error("Cannot close `"+e.type+"` ("+P({start:e.start,end:e.end})+"): a different token (`"+t.type+"`, "+P({start:t.start,end:t.end})+") is open"):new Error("Cannot close document, a token (`"+t.type+"`, "+P({start:t.start,end:t.end})+") is still open")}var Jt=function(e){Object.assign(this,{Parser:t=>{let n=this.data("settings");return Yt(t,Object.assign({},n,e,{extensions:this.data("micromarkExtensions")||[],mdastExtensions:this.data("fromMarkdownExtensions")||[]}))}})};function Qt(e){let t=[],n=-1,r=0,a=0;for(;++n<e.length;){let o=e.charCodeAt(n),i="";if(37===o&&be(e.charCodeAt(n+1))&&be(e.charCodeAt(n+2)))a=2;else if(o<128)/[!#$&-;=?-Z_a-z~]/.test(String.fromCharCode(o))||(i=String.fromCharCode(o));else if(o>55295&&o<57344){let t=e.charCodeAt(n+1);o<56320&&t>56319&&t<57344?(i=String.fromCharCode(o,t),a=1):i="�"}else i=String.fromCharCode(o);i&&(t.push(e.slice(r,n),encodeURIComponent(i)),r=n+a+1,i=""),a&&(n+=a,a=0)}return t.join("")+e.slice(r)}function Zt(e,t){let n,r=String(t.identifier).toUpperCase(),a=Qt(r.toLowerCase()),o=e.footnoteOrder.indexOf(r);-1===o?(e.footnoteOrder.push(r),e.footnoteCounts[r]=1,n=e.footnoteOrder.length):(e.footnoteCounts[r]++,n=o+1);let i=e.footnoteCounts[r],l={type:"element",tagName:"a",properties:{href:"#"+e.clobberPrefix+"fn-"+a,id:e.clobberPrefix+"fnref-"+a+(i>1?"-"+i:""),dataFootnoteRef:!0,ariaDescribedBy:["footnote-label"]},children:[{type:"text",value:String(n)}]};e.patch(t,l);let s={type:"element",tagName:"sup",properties:{},children:[l]};return e.patch(t,s),e.applyData(t,s)}function en(e,t){let n=t.referenceType,r="]";if("collapsed"===n?r+="[]":"full"===n&&(r+="["+(t.label||t.identifier)+"]"),"imageReference"===t.type)return{type:"text",value:"!["+t.alt+r};let a=e.all(t),o=a[0];o&&"text"===o.type?o.value="["+o.value:a.unshift({type:"text",value:"["});let i=a[a.length-1];return i&&"text"===i.type?i.value+=r:a.push({type:"text",value:r}),a}function tn(e){let t=e.spread;return null==t?e.children.length>1:t}var nn=an("start"),rn=an("end");function an(e){return function(t){let n=t&&t.position&&t.position[e]||{};return{line:n.line||null,column:n.column||null,offset:n.offset>-1?n.offset:null}}}function on(e){let t=String(e),n=/\r?\n|\r/g,r=n.exec(t),a=0,o=[];for(;r;)o.push(ln(t.slice(a,r.index),a>0,!0),r[0]),a=r.index+r[0].length,r=n.exec(t);return o.push(ln(t.slice(a),a>0,!1)),o.join("")}function ln(e,t,n){let r=0,a=e.length;if(t){let t=e.codePointAt(r);for(;9===t||32===t;)r++,t=e.codePointAt(r)}if(n){let t=e.codePointAt(a-1);for(;9===t||32===t;)a--,t=e.codePointAt(a-1)}return a>r?e.slice(r,a):""}var sn={blockquote:function(e,t){let n={type:"element",tagName:"blockquote",properties:{},children:e.wrap(e.all(t),!0)};return e.patch(t,n),e.applyData(t,n)},break:function(e,t){let n={type:"element",tagName:"br",properties:{},children:[]};return e.patch(t,n),[e.applyData(t,n),{type:"text",value:"\n"}]},code:function(e,t){let n=t.value?t.value+"\n":"",r=t.lang?t.lang.match(/^[^ \t]+(?=[ \t]|$)/):null,a={};r&&(a.className=["language-"+r]);let o={type:"element",tagName:"code",properties:a,children:[{type:"text",value:n}]};return t.meta&&(o.data={meta:t.meta}),e.patch(t,o),o=e.applyData(t,o),o={type:"element",tagName:"pre",properties:{},children:[o]},e.patch(t,o),o},delete:function(e,t){let n={type:"element",tagName:"del",properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},emphasis:function(e,t){let n={type:"element",tagName:"em",properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},footnoteReference:Zt,footnote:function(e,t){let n=e.footnoteById,r=1;for(;r in n;)r++;let a=String(r);return n[a]={type:"footnoteDefinition",identifier:a,children:[{type:"paragraph",children:t.children}],position:t.position},Zt(e,{type:"footnoteReference",identifier:a,position:t.position})},heading:function(e,t){let n={type:"element",tagName:"h"+t.depth,properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},html:function(e,t){if(e.dangerous){let n={type:"raw",value:t.value};return e.patch(t,n),e.applyData(t,n)}return null},imageReference:function(e,t){let n=e.definition(t.identifier);if(!n)return en(e,t);let r={src:Qt(n.url||""),alt:t.alt};null!=n.title&&(r.title=n.title);let a={type:"element",tagName:"img",properties:r,children:[]};return e.patch(t,a),e.applyData(t,a)},image:function(e,t){let n={src:Qt(t.url)};null!=t.alt&&(n.alt=t.alt),null!=t.title&&(n.title=t.title);let r={type:"element",tagName:"img",properties:n,children:[]};return e.patch(t,r),e.applyData(t,r)},inlineCode:function(e,t){let n={type:"text",value:t.value.replace(/\r?\n|\r/g," ")};e.patch(t,n);let r={type:"element",tagName:"code",properties:{},children:[n]};return e.patch(t,r),e.applyData(t,r)},linkReference:function(e,t){let n=e.definition(t.identifier);if(!n)return en(e,t);let r={href:Qt(n.url||"")};null!=n.title&&(r.title=n.title);let a={type:"element",tagName:"a",properties:r,children:e.all(t)};return e.patch(t,a),e.applyData(t,a)},link:function(e,t){let n={href:Qt(t.url)};null!=t.title&&(n.title=t.title);let r={type:"element",tagName:"a",properties:n,children:e.all(t)};return e.patch(t,r),e.applyData(t,r)},listItem:function(e,t,n){let r=e.all(t),a=n?function(e){let t=!1;if("list"===e.type){t=e.spread||!1;let n=e.children,r=-1;for(;!t&&++r<n.length;)t=tn(n[r])}return t}(n):tn(t),o={},i=[];if("boolean"==typeof t.checked){let e,n=r[0];n&&"element"===n.type&&"p"===n.tagName?e=n:(e={type:"element",tagName:"p",properties:{},children:[]},r.unshift(e)),e.children.length>0&&e.children.unshift({type:"text",value:" "}),e.children.unshift({type:"element",tagName:"input",properties:{type:"checkbox",checked:t.checked,disabled:!0},children:[]}),o.className=["task-list-item"]}let l=-1;for(;++l<r.length;){let e=r[l];(a||0!==l||"element"!==e.type||"p"!==e.tagName)&&i.push({type:"text",value:"\n"}),"element"!==e.type||"p"!==e.tagName||a?i.push(e):i.push(...e.children)}let s=r[r.length-1];s&&(a||"element"!==s.type||"p"!==s.tagName)&&i.push({type:"text",value:"\n"});let c={type:"element",tagName:"li",properties:o,children:i};return e.patch(t,c),e.applyData(t,c)},list:function(e,t){let n={},r=e.all(t),a=-1;for("number"==typeof t.start&&1!==t.start&&(n.start=t.start);++a<r.length;){let e=r[a];if("element"===e.type&&"li"===e.tagName&&e.properties&&Array.isArray(e.properties.className)&&e.properties.className.includes("task-list-item")){n.className=["contains-task-list"];break}}let o={type:"element",tagName:t.ordered?"ol":"ul",properties:n,children:e.wrap(r,!0)};return e.patch(t,o),e.applyData(t,o)},paragraph:function(e,t){let n={type:"element",tagName:"p",properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},root:function(e,t){let n={type:"root",children:e.wrap(e.all(t))};return e.patch(t,n),e.applyData(t,n)},strong:function(e,t){let n={type:"element",tagName:"strong",properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},table:function(e,t){let n=e.all(t),r=n.shift(),a=[];if(r){let n={type:"element",tagName:"thead",properties:{},children:e.wrap([r],!0)};e.patch(t.children[0],n),a.push(n)}if(n.length>0){let r={type:"element",tagName:"tbody",properties:{},children:e.wrap(n,!0)},o=nn(t.children[1]),i=rn(t.children[t.children.length-1]);o.line&&i.line&&(r.position={start:o,end:i}),a.push(r)}let o={type:"element",tagName:"table",properties:{},children:e.wrap(a,!0)};return e.patch(t,o),e.applyData(t,o)},tableCell:function(e,t){let n={type:"element",tagName:"td",properties:{},children:e.all(t)};return e.patch(t,n),e.applyData(t,n)},tableRow:function(e,t,n){let r=n?n.children:void 0,a=0===(r?r.indexOf(t):1)?"th":"td",o=n&&"table"===n.type?n.align:void 0,i=o?o.length:t.children.length,l=-1,s=[];for(;++l<i;){let n=t.children[l],r={},i=o?o[l]:void 0;i&&(r.align=i);let c={type:"element",tagName:a,properties:r,children:[]};n&&(c.children=e.all(n),e.patch(n,c),c=e.applyData(t,c)),s.push(c)}let c={type:"element",tagName:"tr",properties:{},children:e.wrap(s,!0)};return e.patch(t,c),e.applyData(t,c)},text:function(e,t){let n={type:"text",value:on(String(t.value))};return e.patch(t,n),e.applyData(t,n)},thematicBreak:function(e,t){let n={type:"element",tagName:"hr",properties:{},children:[]};return e.patch(t,n),e.applyData(t,n)},toml:cn,yaml:cn,definition:cn,footnoteDefinition:cn};function cn(){return null}var un=function(e){if(null==e)return dn;if("string"==typeof e)return function(e){return fn(function(t){return t&&t.type===e})}(e);if("object"==typeof e)return Array.isArray(e)?function(e){let t=[],n=-1;for(;++n<e.length;)t[n]=un(e[n]);return fn(function(...e){let n=-1;for(;++n<t.length;)if(t[n].call(this,...e))return!0;return!1})}(e):function(e){return fn(function(t){let n;for(n in e)if(t[n]!==e[n])return!1;return!0})}(e);if("function"==typeof e)return fn(e);throw new Error("Expected function, string, or object as test")};function fn(e){return function(t,...n){return!!(t&&"object"==typeof t&&"type"in t&&e.call(this,t,...n))}}function dn(){return!0}var pn=function(e,t,n,r){"function"==typeof t&&"function"!=typeof n&&(r=n,n=t,t=null),function(e,t,n,r){"function"==typeof t&&"function"!=typeof n&&(r=n,n=t,t=null);let a=un(t),o=r?-1:1;!function e(i,l,s){let c=i&&"object"==typeof i?i:{};if("string"==typeof c.type){let e="string"==typeof c.tagName?c.tagName:"string"==typeof c.name?c.name:void 0;Object.defineProperty(u,"name",{value:"node ("+i.type+(e?"<"+e+">":"")+")"})}return u;function u(){let c,u,f,d=[];if((!t||a(i,l,s[s.length-1]||null))&&(d=function(e){return Array.isArray(e)?e:"number"==typeof e?[!0,e]:[e]}(n(i,s)),!1===d[0]))return d;if(i.children&&"skip"!==d[0])for(u=(r?i.children.length:-1)+o,f=s.concat(i);u>-1&&u<i.children.length;){if(c=e(i.children[u],u,f)(),!1===c[0])return c;u="number"==typeof c[1]?c[1]:u+o}return d}}(e,void 0,[])()}(e,t,function(e,t){let r=t[t.length-1];return n(e,r?r.children.indexOf(e):null,r)},r)},mn={}.hasOwnProperty;function hn(e){return String(e||"").toUpperCase()}var gn={}.hasOwnProperty;function yn(e,t){e.position&&(t.position=function(e){return{start:nn(e),end:rn(e)}}(e))}function vn(e,t){let n=t;if(e&&e.data){let t=e.data.hName,r=e.data.hChildren,a=e.data.hProperties;"string"==typeof t&&("element"===n.type?n.tagName=t:n={type:"element",tagName:t,properties:{},children:[]}),"element"===n.type&&a&&(n.properties=d(d({},n.properties),a)),"children"in n&&n.children&&null!=r&&(n.children=r)}return n}function bn(e,t,n){let r=t&&t.type;if(!r)throw new Error("Expected node, got `"+t+"`");return gn.call(e.handlers,r)?e.handlers[r](e,t,n):e.passThrough&&e.passThrough.includes(r)?"children"in t?p(d({},t),{children:En(e,t)}):t:e.unknownHandler?e.unknownHandler(e,t,n):function(e,t){let n=t.data||{},r=!("value"in t)||gn.call(n,"hProperties")||gn.call(n,"hChildren")?{type:"element",tagName:"div",properties:{},children:En(e,t)}:{type:"text",value:t.value};return e.patch(t,r),e.applyData(t,r)}(e,t)}function En(e,t){let n=[];if("children"in t){let r=t.children,a=-1;for(;++a<r.length;){let o=bn(e,r[a],t);if(o){if(a&&"break"===r[a-1].type&&(!Array.isArray(o)&&"text"===o.type&&(o.value=o.value.replace(/^\s+/,"")),!Array.isArray(o)&&"element"===o.type)){let e=o.children[0];e&&"text"===e.type&&(e.value=e.value.replace(/^\s+/,""))}Array.isArray(o)?n.push(...o):n.push(o)}}}return n}function Tn(e,t){let n=[],r=-1;for(t&&n.push({type:"text",value:"\n"});++r<e.length;)r&&n.push({type:"text",value:"\n"}),n.push(e[r]);return t&&e.length>0&&n.push({type:"text",value:"\n"}),n}function Sn(e,t){let n=function(e,t){let n=t||{},r={};return o.dangerous=n.allowDangerousHtml||!1,o.clobberPrefix=null==n.clobberPrefix?"user-content-":n.clobberPrefix,o.footnoteLabel=n.footnoteLabel||"Footnotes",o.footnoteLabelTagName=n.footnoteLabelTagName||"h2",o.footnoteLabelProperties=n.footnoteLabelProperties||{className:["sr-only"]},o.footnoteBackLabel=n.footnoteBackLabel||"Back to content",o.unknownHandler=n.unknownHandler,o.passThrough=n.passThrough,o.handlers=d(d({},sn),n.handlers),o.definition=function(e){let t=Object.create(null);if(!e||!e.type)throw new Error("mdast-util-definitions expected node");return pn(e,"definition",e=>{let n=hn(e.identifier);n&&!mn.call(t,n)&&(t[n]=e)}),function(e){let n=hn(e);return n&&mn.call(t,n)?t[n]:null}}(e),o.footnoteById=r,o.footnoteOrder=[],o.footnoteCounts={},o.patch=yn,o.applyData=vn,o.one=function(e,t){return bn(o,e,t)},o.all=function(e){return En(o,e)},o.wrap=Tn,o.augment=a,pn(e,"footnoteDefinition",e=>{let t=String(e.identifier).toUpperCase();gn.call(r,t)||(r[t]=e)}),o;function a(e,t){if(e&&"data"in e&&e.data){let n=e.data;n.hName&&("element"!==t.type&&(t={type:"element",tagName:"",properties:{},children:[]}),t.tagName=n.hName),"element"===t.type&&n.hProperties&&(t.properties=d(d({},t.properties),n.hProperties)),"children"in t&&t.children&&n.hChildren&&(t.children=n.hChildren)}if(e){let n="type"in e?e:{position:e};(function(e){return!(e&&e.position&&e.position.start&&e.position.start.line&&e.position.start.column&&e.position.end&&e.position.end.line&&e.position.end.column)})(n)||(t.position={start:nn(n),end:rn(n)})}return t}function o(e,t,n,r){return Array.isArray(n)&&(r=n,n={}),a(e,{type:"element",tagName:t,properties:n||{},children:r||[]})}}(e,t),r=n.one(e,null),a=function(e){let t=[],n=-1;for(;++n<e.footnoteOrder.length;){let r=e.footnoteById[e.footnoteOrder[n]];if(!r)continue;let a=e.all(r),o=String(r.identifier).toUpperCase(),i=Qt(o.toLowerCase()),l=0,s=[];for(;++l<=e.footnoteCounts[o];){let t={type:"element",tagName:"a",properties:{href:"#"+e.clobberPrefix+"fnref-"+i+(l>1?"-"+l:""),dataFootnoteBackref:!0,className:["data-footnote-backref"],ariaLabel:e.footnoteBackLabel},children:[{type:"text",value:"↩"}]};l>1&&t.children.push({type:"element",tagName:"sup",children:[{type:"text",value:String(l)}]}),s.length>0&&s.push({type:"text",value:" "}),s.push(t)}let c=a[a.length-1];if(c&&"element"===c.type&&"p"===c.tagName){let e=c.children[c.children.length-1];e&&"text"===e.type?e.value+=" ":c.children.push({type:"text",value:" "}),c.children.push(...s)}else a.push(...s);let u={type:"element",tagName:"li",properties:{id:e.clobberPrefix+"fn-"+i},children:e.wrap(a,!0)};e.patch(r,u),t.push(u)}if(0!==t.length)return{type:"element",tagName:"section",properties:{dataFootnotes:!0,className:["footnotes"]},children:[{type:"element",tagName:e.footnoteLabelTagName,properties:p(d({},JSON.parse(JSON.stringify(e.footnoteLabelProperties))),{id:"footnote-label"}),children:[{type:"text",value:e.footnoteLabel}]},{type:"text",value:"\n"},{type:"element",tagName:"ol",properties:{},children:e.wrap(t,!0)},{type:"text",value:"\n"}]}}(n);return a&&r.children.push({type:"text",value:"\n"},a),Array.isArray(r)?{type:"root",children:r}:r}var wn=function(e,t){return e&&"run"in e?function(e,t){return(n,r,a)=>{e.run(Sn(n,t),r,e=>{a(e)})}}(e,t):function(e){return t=>Sn(t,e)}(e||t)},Nn=y(N(),1),Rn=class{constructor(e,t,n){this.property=e,this.normal=t,n&&(this.space=n)}};function On(e,t){let n={},r={},a=-1;for(;++a<e.length;)Object.assign(n,e[a].property),Object.assign(r,e[a].normal);return new Rn(n,r,t)}function Cn(e){return e.toLowerCase()}Rn.prototype.property={},Rn.prototype.normal={},Rn.prototype.space=null;var xn=class{constructor(e,t){this.property=e,this.attribute=t}};xn.prototype.space=null,xn.prototype.boolean=!1,xn.prototype.booleanish=!1,xn.prototype.overloadedBoolean=!1,xn.prototype.number=!1,xn.prototype.commaSeparated=!1,xn.prototype.spaceSeparated=!1,xn.prototype.commaOrSpaceSeparated=!1,xn.prototype.mustUseProperty=!1,xn.prototype.defined=!1;var kn={};h(kn,{boolean:()=>In,booleanish:()=>Ln,commaOrSpaceSeparated:()=>Un,commaSeparated:()=>Dn,number:()=>Pn,overloadedBoolean:()=>_n,spaceSeparated:()=>Mn});var An=0,In=jn(),Ln=jn(),_n=jn(),Pn=jn(),Mn=jn(),Dn=jn(),Un=jn();function jn(){return u(2,++An)}var Fn=Object.keys(kn),zn=class extends xn{constructor(e,t,n,r){let a=-1;if(super(e,t),Bn(this,"space",r),"number"==typeof n)for(;++a<Fn.length;){let e=Fn[a];Bn(this,Fn[a],(n&kn[e])===kn[e])}}};function Bn(e,t,n){n&&(e[t]=n)}zn.prototype.defined=!0;var Hn={}.hasOwnProperty;function Vn(e){let t,n={},r={};for(t in e.properties)if(Hn.call(e.properties,t)){let a=e.properties[t],o=new zn(t,e.transform(e.attributes||{},t),a,e.space);e.mustUseProperty&&e.mustUseProperty.includes(t)&&(o.mustUseProperty=!0),n[t]=o,r[Cn(t)]=t,r[Cn(o.attribute)]=t}return new Rn(n,r,e.space)}var Wn=Vn({space:"xlink",transform:(e,t)=>"xlink:"+t.slice(5).toLowerCase(),properties:{xLinkActuate:null,xLinkArcRole:null,xLinkHref:null,xLinkRole:null,xLinkShow:null,xLinkTitle:null,xLinkType:null}}),Gn=Vn({space:"xml",transform:(e,t)=>"xml:"+t.slice(3).toLowerCase(),properties:{xmlLang:null,xmlBase:null,xmlSpace:null}});function Yn(e,t){return t in e?e[t]:t}function $n(e,t){return Yn(e,t.toLowerCase())}var Xn=Vn({space:"xmlns",attributes:{xmlnsxlink:"xmlns:xlink"},transform:$n,properties:{xmlns:null,xmlnsXLink:null}}),qn=Vn({transform:(e,t)=>"role"===t?t:"aria-"+t.slice(4).toLowerCase(),properties:{ariaActiveDescendant:null,ariaAtomic:Ln,ariaAutoComplete:null,ariaBusy:Ln,ariaChecked:Ln,ariaColCount:Pn,ariaColIndex:Pn,ariaColSpan:Pn,ariaControls:Mn,ariaCurrent:null,ariaDescribedBy:Mn,ariaDetails:null,ariaDisabled:Ln,ariaDropEffect:Mn,ariaErrorMessage:null,ariaExpanded:Ln,ariaFlowTo:Mn,ariaGrabbed:Ln,ariaHasPopup:null,ariaHidden:Ln,ariaInvalid:null,ariaKeyShortcuts:null,ariaLabel:null,ariaLabelledBy:Mn,ariaLevel:Pn,ariaLive:null,ariaModal:Ln,ariaMultiLine:Ln,ariaMultiSelectable:Ln,ariaOrientation:null,ariaOwns:Mn,ariaPlaceholder:null,ariaPosInSet:Pn,ariaPressed:Ln,ariaReadOnly:Ln,ariaRelevant:null,ariaRequired:Ln,ariaRoleDescription:Mn,ariaRowCount:Pn,ariaRowIndex:Pn,ariaRowSpan:Pn,ariaSelected:Ln,ariaSetSize:Pn,ariaSort:null,ariaValueMax:Pn,ariaValueMin:Pn,ariaValueNow:Pn,ariaValueText:null,role:null}}),Kn=Vn({space:"html",attributes:{acceptcharset:"accept-charset",classname:"class",htmlfor:"for",httpequiv:"http-equiv"},transform:$n,mustUseProperty:["checked","multiple","muted","selected"],properties:{abbr:null,accept:Dn,acceptCharset:Mn,accessKey:Mn,action:null,allow:null,allowFullScreen:In,allowPaymentRequest:In,allowUserMedia:In,alt:null,as:null,async:In,autoCapitalize:null,autoComplete:Mn,autoFocus:In,autoPlay:In,capture:In,charSet:null,checked:In,cite:null,className:Mn,cols:Pn,colSpan:null,content:null,contentEditable:Ln,controls:In,controlsList:Mn,coords:Pn|Dn,crossOrigin:null,data:null,dateTime:null,decoding:null,default:In,defer:In,dir:null,dirName:null,disabled:In,download:_n,draggable:Ln,encType:null,enterKeyHint:null,form:null,formAction:null,formEncType:null,formMethod:null,formNoValidate:In,formTarget:null,headers:Mn,height:Pn,hidden:In,high:Pn,href:null,hrefLang:null,htmlFor:Mn,httpEquiv:Mn,id:null,imageSizes:null,imageSrcSet:null,inputMode:null,integrity:null,is:null,isMap:In,itemId:null,itemProp:Mn,itemRef:Mn,itemScope:In,itemType:Mn,kind:null,label:null,lang:null,language:null,list:null,loading:null,loop:In,low:Pn,manifest:null,max:null,maxLength:Pn,media:null,method:null,min:null,minLength:Pn,multiple:In,muted:In,name:null,nonce:null,noModule:In,noValidate:In,onAbort:null,onAfterPrint:null,onAuxClick:null,onBeforeMatch:null,onBeforePrint:null,onBeforeUnload:null,onBlur:null,onCancel:null,onCanPlay:null,onCanPlayThrough:null,onChange:null,onClick:null,onClose:null,onContextLost:null,onContextMenu:null,onContextRestored:null,onCopy:null,onCueChange:null,onCut:null,onDblClick:null,onDrag:null,onDragEnd:null,onDragEnter:null,onDragExit:null,onDragLeave:null,onDragOver:null,onDragStart:null,onDrop:null,onDurationChange:null,onEmptied:null,onEnded:null,onError:null,onFocus:null,onFormData:null,onHashChange:null,onInput:null,onInvalid:null,onKeyDown:null,onKeyPress:null,onKeyUp:null,onLanguageChange:null,onLoad:null,onLoadedData:null,onLoadedMetadata:null,onLoadEnd:null,onLoadStart:null,onMessage:null,onMessageError:null,onMouseDown:null,onMouseEnter:null,onMouseLeave:null,onMouseMove:null,onMouseOut:null,onMouseOver:null,onMouseUp:null,onOffline:null,onOnline:null,onPageHide:null,onPageShow:null,onPaste:null,onPause:null,onPlay:null,onPlaying:null,onPopState:null,onProgress:null,onRateChange:null,onRejectionHandled:null,onReset:null,onResize:null,onScroll:null,onScrollEnd:null,onSecurityPolicyViolation:null,onSeeked:null,onSeeking:null,onSelect:null,onSlotChange:null,onStalled:null,onStorage:null,onSubmit:null,onSuspend:null,onTimeUpdate:null,onToggle:null,onUnhandledRejection:null,onUnload:null,onVolumeChange:null,onWaiting:null,onWheel:null,open:In,optimum:Pn,pattern:null,ping:Mn,placeholder:null,playsInline:In,poster:null,preload:null,readOnly:In,referrerPolicy:null,rel:Mn,required:In,reversed:In,rows:Pn,rowSpan:Pn,sandbox:Mn,scope:null,scoped:In,seamless:In,selected:In,shape:null,size:Pn,sizes:null,slot:null,span:Pn,spellCheck:Ln,src:null,srcDoc:null,srcLang:null,srcSet:null,start:Pn,step:null,style:null,tabIndex:Pn,target:null,title:null,translate:null,type:null,typeMustMatch:In,useMap:null,value:Ln,width:Pn,wrap:null,align:null,aLink:null,archive:Mn,axis:null,background:null,bgColor:null,border:Pn,borderColor:null,bottomMargin:Pn,cellPadding:null,cellSpacing:null,char:null,charOff:null,classId:null,clear:null,code:null,codeBase:null,codeType:null,color:null,compact:In,declare:In,event:null,face:null,frame:null,frameBorder:null,hSpace:Pn,leftMargin:Pn,link:null,longDesc:null,lowSrc:null,marginHeight:Pn,marginWidth:Pn,noResize:In,noHref:In,noShade:In,noWrap:In,object:null,profile:null,prompt:null,rev:null,rightMargin:Pn,rules:null,scheme:null,scrolling:Ln,standby:null,summary:null,text:null,topMargin:Pn,valueType:null,version:null,vAlign:null,vLink:null,vSpace:Pn,allowTransparency:null,autoCorrect:null,autoSave:null,disablePictureInPicture:In,disableRemotePlayback:In,prefix:null,property:null,results:Pn,security:null,unselectable:null}}),Jn=Vn({space:"svg",attributes:{accentHeight:"accent-height",alignmentBaseline:"alignment-baseline",arabicForm:"arabic-form",baselineShift:"baseline-shift",capHeight:"cap-height",className:"class",clipPath:"clip-path",clipRule:"clip-rule",colorInterpolation:"color-interpolation",colorInterpolationFilters:"color-interpolation-filters",colorProfile:"color-profile",colorRendering:"color-rendering",crossOrigin:"crossorigin",dataType:"datatype",dominantBaseline:"dominant-baseline",enableBackground:"enable-background",fillOpacity:"fill-opacity",fillRule:"fill-rule",floodColor:"flood-color",floodOpacity:"flood-opacity",fontFamily:"font-family",fontSize:"font-size",fontSizeAdjust:"font-size-adjust",fontStretch:"font-stretch",fontStyle:"font-style",fontVariant:"font-variant",fontWeight:"font-weight",glyphName:"glyph-name",glyphOrientationHorizontal:"glyph-orientation-horizontal",glyphOrientationVertical:"glyph-orientation-vertical",hrefLang:"hreflang",horizAdvX:"horiz-adv-x",horizOriginX:"horiz-origin-x",horizOriginY:"horiz-origin-y",imageRendering:"image-rendering",letterSpacing:"letter-spacing",lightingColor:"lighting-color",markerEnd:"marker-end",markerMid:"marker-mid",markerStart:"marker-start",navDown:"nav-down",navDownLeft:"nav-down-left",navDownRight:"nav-down-right",navLeft:"nav-left",navNext:"nav-next",navPrev:"nav-prev",navRight:"nav-right",navUp:"nav-up",navUpLeft:"nav-up-left",navUpRight:"nav-up-right",onAbort:"onabort",onActivate:"onactivate",onAfterPrint:"onafterprint",onBeforePrint:"onbeforeprint",onBegin:"onbegin",onCancel:"oncancel",onCanPlay:"oncanplay",onCanPlayThrough:"oncanplaythrough",onChange:"onchange",onClick:"onclick",onClose:"onclose",onCopy:"oncopy",onCueChange:"oncuechange",onCut:"oncut",onDblClick:"ondblclick",onDrag:"ondrag",onDragEnd:"ondragend",onDragEnter:"ondragenter",onDragExit:"ondragexit",onDragLeave:"ondragleave",onDragOver:"ondragover",onDragStart:"ondragstart",onDrop:"ondrop",onDurationChange:"ondurationchange",onEmptied:"onemptied",onEnd:"onend",onEnded:"onended",onError:"onerror",onFocus:"onfocus",onFocusIn:"onfocusin",onFocusOut:"onfocusout",onHashChange:"onhashchange",onInput:"oninput",onInvalid:"oninvalid",onKeyDown:"onkeydown",onKeyPress:"onkeypress",onKeyUp:"onkeyup",onLoad:"onload",onLoadedData:"onloadeddata",onLoadedMetadata:"onloadedmetadata",onLoadStart:"onloadstart",onMessage:"onmessage",onMouseDown:"onmousedown",onMouseEnter:"onmouseenter",onMouseLeave:"onmouseleave",onMouseMove:"onmousemove",onMouseOut:"onmouseout",onMouseOver:"onmouseover",onMouseUp:"onmouseup",onMouseWheel:"onmousewheel",onOffline:"onoffline",onOnline:"ononline",onPageHide:"onpagehide",onPageShow:"onpageshow",onPaste:"onpaste",onPause:"onpause",onPlay:"onplay",onPlaying:"onplaying",onPopState:"onpopstate",onProgress:"onprogress",onRateChange:"onratechange",onRepeat:"onrepeat",onReset:"onreset",onResize:"onresize",onScroll:"onscroll",onSeeked:"onseeked",onSeeking:"onseeking",onSelect:"onselect",onShow:"onshow",onStalled:"onstalled",onStorage:"onstorage",onSubmit:"onsubmit",onSuspend:"onsuspend",onTimeUpdate:"ontimeupdate",onToggle:"ontoggle",onUnload:"onunload",onVolumeChange:"onvolumechange",onWaiting:"onwaiting",onZoom:"onzoom",overlinePosition:"overline-position",overlineThickness:"overline-thickness",paintOrder:"paint-order",panose1:"panose-1",pointerEvents:"pointer-events",referrerPolicy:"referrerpolicy",renderingIntent:"rendering-intent",shapeRendering:"shape-rendering",stopColor:"stop-color",stopOpacity:"stop-opacity",strikethroughPosition:"strikethrough-position",strikethroughThickness:"strikethrough-thickness",strokeDashArray:"stroke-dasharray",strokeDashOffset:"stroke-dashoffset",strokeLineCap:"stroke-linecap",strokeLineJoin:"stroke-linejoin",strokeMiterLimit:"stroke-miterlimit",strokeOpacity:"stroke-opacity",strokeWidth:"stroke-width",tabIndex:"tabindex",textAnchor:"text-anchor",textDecoration:"text-decoration",textRendering:"text-rendering",typeOf:"typeof",underlinePosition:"underline-position",underlineThickness:"underline-thickness",unicodeBidi:"unicode-bidi",unicodeRange:"unicode-range",unitsPerEm:"units-per-em",vAlphabetic:"v-alphabetic",vHanging:"v-hanging",vIdeographic:"v-ideographic",vMathematical:"v-mathematical",vectorEffect:"vector-effect",vertAdvY:"vert-adv-y",vertOriginX:"vert-origin-x",vertOriginY:"vert-origin-y",wordSpacing:"word-spacing",writingMode:"writing-mode",xHeight:"x-height",playbackOrder:"playbackorder",timelineBegin:"timelinebegin"},transform:Yn,properties:{about:Un,accentHeight:Pn,accumulate:null,additive:null,alignmentBaseline:null,alphabetic:Pn,amplitude:Pn,arabicForm:null,ascent:Pn,attributeName:null,attributeType:null,azimuth:Pn,bandwidth:null,baselineShift:null,baseFrequency:null,baseProfile:null,bbox:null,begin:null,bias:Pn,by:null,calcMode:null,capHeight:Pn,className:Mn,clip:null,clipPath:null,clipPathUnits:null,clipRule:null,color:null,colorInterpolation:null,colorInterpolationFilters:null,colorProfile:null,colorRendering:null,content:null,contentScriptType:null,contentStyleType:null,crossOrigin:null,cursor:null,cx:null,cy:null,d:null,dataType:null,defaultAction:null,descent:Pn,diffuseConstant:Pn,direction:null,display:null,dur:null,divisor:Pn,dominantBaseline:null,download:In,dx:null,dy:null,edgeMode:null,editable:null,elevation:Pn,enableBackground:null,end:null,event:null,exponent:Pn,externalResourcesRequired:null,fill:null,fillOpacity:Pn,fillRule:null,filter:null,filterRes:null,filterUnits:null,floodColor:null,floodOpacity:null,focusable:null,focusHighlight:null,fontFamily:null,fontSize:null,fontSizeAdjust:null,fontStretch:null,fontStyle:null,fontVariant:null,fontWeight:null,format:null,fr:null,from:null,fx:null,fy:null,g1:Dn,g2:Dn,glyphName:Dn,glyphOrientationHorizontal:null,glyphOrientationVertical:null,glyphRef:null,gradientTransform:null,gradientUnits:null,handler:null,hanging:Pn,hatchContentUnits:null,hatchUnits:null,height:null,href:null,hrefLang:null,horizAdvX:Pn,horizOriginX:Pn,horizOriginY:Pn,id:null,ideographic:Pn,imageRendering:null,initialVisibility:null,in:null,in2:null,intercept:Pn,k:Pn,k1:Pn,k2:Pn,k3:Pn,k4:Pn,kernelMatrix:Un,kernelUnitLength:null,keyPoints:null,keySplines:null,keyTimes:null,kerning:null,lang:null,lengthAdjust:null,letterSpacing:null,lightingColor:null,limitingConeAngle:Pn,local:null,markerEnd:null,markerMid:null,markerStart:null,markerHeight:null,markerUnits:null,markerWidth:null,mask:null,maskContentUnits:null,maskUnits:null,mathematical:null,max:null,media:null,mediaCharacterEncoding:null,mediaContentEncodings:null,mediaSize:Pn,mediaTime:null,method:null,min:null,mode:null,name:null,navDown:null,navDownLeft:null,navDownRight:null,navLeft:null,navNext:null,navPrev:null,navRight:null,navUp:null,navUpLeft:null,navUpRight:null,numOctaves:null,observer:null,offset:null,onAbort:null,onActivate:null,onAfterPrint:null,onBeforePrint:null,onBegin:null,onCancel:null,onCanPlay:null,onCanPlayThrough:null,onChange:null,onClick:null,onClose:null,onCopy:null,onCueChange:null,onCut:null,onDblClick:null,onDrag:null,onDragEnd:null,onDragEnter:null,onDragExit:null,onDragLeave:null,onDragOver:null,onDragStart:null,onDrop:null,onDurationChange:null,onEmptied:null,onEnd:null,onEnded:null,onError:null,onFocus:null,onFocusIn:null,onFocusOut:null,onHashChange:null,onInput:null,onInvalid:null,onKeyDown:null,onKeyPress:null,onKeyUp:null,onLoad:null,onLoadedData:null,onLoadedMetadata:null,onLoadStart:null,onMessage:null,onMouseDown:null,onMouseEnter:null,onMouseLeave:null,onMouseMove:null,onMouseOut:null,onMouseOver:null,onMouseUp:null,onMouseWheel:null,onOffline:null,onOnline:null,onPageHide:null,onPageShow:null,onPaste:null,onPause:null,onPlay:null,onPlaying:null,onPopState:null,onProgress:null,onRateChange:null,onRepeat:null,onReset:null,onResize:null,onScroll:null,onSeeked:null,onSeeking:null,onSelect:null,onShow:null,onStalled:null,onStorage:null,onSubmit:null,onSuspend:null,onTimeUpdate:null,onToggle:null,onUnload:null,onVolumeChange:null,onWaiting:null,onZoom:null,opacity:null,operator:null,order:null,orient:null,orientation:null,origin:null,overflow:null,overlay:null,overlinePosition:Pn,overlineThickness:Pn,paintOrder:null,panose1:null,path:null,pathLength:Pn,patternContentUnits:null,patternTransform:null,patternUnits:null,phase:null,ping:Mn,pitch:null,playbackOrder:null,pointerEvents:null,points:null,pointsAtX:Pn,pointsAtY:Pn,pointsAtZ:Pn,preserveAlpha:null,preserveAspectRatio:null,primitiveUnits:null,propagate:null,property:Un,r:null,radius:null,referrerPolicy:null,refX:null,refY:null,rel:Un,rev:Un,renderingIntent:null,repeatCount:null,repeatDur:null,requiredExtensions:Un,requiredFeatures:Un,requiredFonts:Un,requiredFormats:Un,resource:null,restart:null,result:null,rotate:null,rx:null,ry:null,scale:null,seed:null,shapeRendering:null,side:null,slope:null,snapshotTime:null,specularConstant:Pn,specularExponent:Pn,spreadMethod:null,spacing:null,startOffset:null,stdDeviation:null,stemh:null,stemv:null,stitchTiles:null,stopColor:null,stopOpacity:null,strikethroughPosition:Pn,strikethroughThickness:Pn,string:null,stroke:null,strokeDashArray:Un,strokeDashOffset:null,strokeLineCap:null,strokeLineJoin:null,strokeMiterLimit:Pn,strokeOpacity:Pn,strokeWidth:null,style:null,surfaceScale:Pn,syncBehavior:null,syncBehaviorDefault:null,syncMaster:null,syncTolerance:null,syncToleranceDefault:null,systemLanguage:Un,tabIndex:Pn,tableValues:null,target:null,targetX:Pn,targetY:Pn,textAnchor:null,textDecoration:null,textRendering:null,textLength:null,timelineBegin:null,title:null,transformBehavior:null,type:null,typeOf:Un,to:null,transform:null,u1:null,u2:null,underlinePosition:Pn,underlineThickness:Pn,unicode:null,unicodeBidi:null,unicodeRange:null,unitsPerEm:Pn,values:null,vAlphabetic:Pn,vMathematical:Pn,vectorEffect:null,vHanging:Pn,vIdeographic:Pn,version:null,vertAdvY:Pn,vertOriginX:Pn,vertOriginY:Pn,viewBox:null,viewTarget:null,visibility:null,width:null,widths:null,wordSpacing:null,writingMode:null,x:null,x1:null,x2:null,xChannelSelector:null,xHeight:Pn,y:null,y1:null,y2:null,yChannelSelector:null,z:null,zoomAndPan:null}}),Qn=/^data[-\w.:]+$/i,Zn=/-[a-z]/g,er=/[A-Z]/g;function tr(e){return"-"+e.toLowerCase()}function nr(e){return e.charAt(1).toUpperCase()}var rr={classId:"classID",dataType:"datatype",itemId:"itemID",strokeDashArray:"strokeDasharray",strokeDashOffset:"strokeDashoffset",strokeLineCap:"strokeLinecap",strokeLineJoin:"strokeLinejoin",strokeMiterLimit:"strokeMiterlimit",typeOf:"typeof",xLinkActuate:"xlinkActuate",xLinkArcRole:"xlinkArcrole",xLinkHref:"xlinkHref",xLinkRole:"xlinkRole",xLinkShow:"xlinkShow",xLinkTitle:"xlinkTitle",xLinkType:"xlinkType",xmlnsXLink:"xmlnsXlink"},ar=On([Gn,Wn,Xn,qn,Kn],"html"),or=On([Gn,Wn,Xn,qn,Jn],"svg");function ir(e){if(e.allowedElements&&e.disallowedElements)throw new TypeError("Only one of `allowedElements` and `disallowedElements` should be defined");if(e.allowedElements||e.disallowedElements||e.allowElement)return t=>{pn(t,"element",(t,n,r)=>{let a,o=r;if(e.allowedElements?a=!e.allowedElements.includes(t.tagName):e.disallowedElements&&(a=e.disallowedElements.includes(t.tagName)),!a&&e.allowElement&&"number"==typeof n&&(a=!e.allowElement(t,n,o)),a&&"number"==typeof n)return e.unwrapDisallowed&&t.children?o.children.splice(n,1,...t.children):o.children.splice(n,1),n})}}var lr=y(b(),1),sr=y(O(),1);function cr(e){let t=e&&"object"==typeof e&&"text"===e.type?e.value||"":e;return"string"==typeof t&&""===t.replace(/[ \t\n\f\r]/g,"")}var ur=y(x(),1).default,fr={}.hasOwnProperty,dr=new Set(["table","thead","tbody","tfoot","tr"]);function pr(e,t){let n,r=[],a=-1;for(;++a<t.children.length;)n=t.children[a],"element"===n.type?r.push(mr(e,n,a,t)):"text"===n.type?("element"!==t.type||!dr.has(t.tagName)||!cr(n))&&r.push(n.value):"raw"===n.type&&!e.options.skipHtml&&r.push(n.value);return r}function mr(e,t,n,r){let a,o=e.options,i=void 0===o.transformLinkUri?I:o.transformLinkUri,l=e.schema,s=t.tagName,c={},u=l;if("html"===l.space&&"svg"===s&&(u=or,e.schema=u),t.properties)for(a in t.properties)fr.call(t.properties,a)&&gr(c,a,t.properties[a],e);("ol"===s||"ul"===s)&&e.listDepth++;let f=pr(e,t);("ol"===s||"ul"===s)&&e.listDepth--,e.schema=l;let d=t.position||{start:{line:null,column:null,offset:null},end:{line:null,column:null,offset:null}},p=o.components&&fr.call(o.components,s)?o.components[s]:s,m="string"==typeof p||p===lr.default.Fragment;if(!sr.default.isValidElementType(p))throw new TypeError(`Component for name \`${s}\` not defined or is not renderable`);if(c.key=n,"a"===s&&o.linkTarget&&(c.target="function"==typeof o.linkTarget?o.linkTarget(String(c.href||""),t.children,"string"==typeof c.title?c.title:null):o.linkTarget),"a"===s&&i&&(c.href=i(String(c.href||""),t.children,"string"==typeof c.title?c.title:null)),!m&&"code"===s&&"element"===r.type&&"pre"!==r.tagName&&(c.inline=!0),!m&&("h1"===s||"h2"===s||"h3"===s||"h4"===s||"h5"===s||"h6"===s)&&(c.level=Number.parseInt(s.charAt(1),10)),"img"===s&&o.transformImageUri&&(c.src=o.transformImageUri(String(c.src||""),String(c.alt||""),"string"==typeof c.title?c.title:null)),!m&&"li"===s&&"element"===r.type){let e=function(e){let t=-1;for(;++t<e.children.length;){let n=e.children[t];if("element"===n.type&&"input"===n.tagName)return n}return null}(t);c.checked=e&&e.properties?!!e.properties.checked:null,c.index=hr(r,t),c.ordered="ol"===r.tagName}return!m&&("ol"===s||"ul"===s)&&(c.ordered="ol"===s,c.depth=e.listDepth),("td"===s||"th"===s)&&(c.align&&(c.style||(c.style={}),c.style.textAlign=c.align,delete c.align),m||(c.isHeader="th"===s)),!m&&"tr"===s&&"element"===r.type&&(c.isHeader="thead"===r.tagName),o.sourcePos&&(c["data-sourcepos"]=function(e){return[e.start.line,":",e.start.column,"-",e.end.line,":",e.end.column].map(String).join("")}(d)),!m&&o.rawSourcePos&&(c.sourcePosition=t.position),!m&&o.includeElementIndex&&(c.index=hr(r,t),c.siblingCount=hr(r)),m||(c.node=t),f.length>0?lr.default.createElement(p,c,f):lr.default.createElement(p,c)}function hr(e,t){let n=-1,r=0;for(;++n<e.children.length&&e.children[n]!==t;)"element"===e.children[n].type&&r++;return r}function gr(e,t,n,r){let a=function(e,t){let n=Cn(t),r=t,a=xn;if(n in e.normal)return e.property[e.normal[n]];if(n.length>4&&"data"===n.slice(0,4)&&Qn.test(t)){if("-"===t.charAt(4)){let e=t.slice(5).replace(Zn,nr);r="data"+e.charAt(0).toUpperCase()+e.slice(1)}else{let e=t.slice(4);if(!Zn.test(e)){let n=e.replace(er,tr);"-"!==n.charAt(0)&&(n="-"+n),t="data"+n}}a=zn}return new a(r,t)}(r.schema,t),o=n;null==o||o!=o||(Array.isArray(o)&&(o=a.commaSeparated?function(e,t){let n={};return(""===e[e.length-1]?[...e,""]:e).join((n.padRight?" ":"")+","+(!1===n.padLeft?"":" ")).trim()}(o):function(e){return e.join(" ").trim()}(o)),"style"===a.property&&"string"==typeof o&&(o=function(e){let t={};try{ur(e,function(e,n){let r="-ms-"===e.slice(0,4)?`ms-${e.slice(4)}`:e;t[r.replace(/-([a-z])/g,yr)]=n})}catch(e){}return t}(o)),a.space&&a.property?e[fr.call(rr,a.property)?rr[a.property]:a.property]=o:a.attribute&&(e[a.attribute]=o))}function yr(e,t){return t.toUpperCase()}var vr={}.hasOwnProperty,br={plugins:{to:"remarkPlugins",id:"change-plugins-to-remarkplugins"},renderers:{to:"components",id:"change-renderers-to-components"},astPlugins:{id:"remove-buggy-html-in-markdown-parser"},allowDangerousHtml:{id:"remove-buggy-html-in-markdown-parser"},escapeHtml:{id:"remove-buggy-html-in-markdown-parser"},source:{to:"children",id:"change-source-to-children"},allowNode:{to:"allowElement",id:"replace-allownode-allowedtypes-and-disallowedtypes"},allowedTypes:{to:"allowedElements",id:"replace-allownode-allowedtypes-and-disallowedtypes"},disallowedTypes:{to:"disallowedElements",id:"replace-allownode-allowedtypes-and-disallowedtypes"},includeNodeIndex:{to:"includeElementIndex",id:"change-includenodeindex-to-includeelementindex"}};function Er(e){for(let t in br)if(vr.call(br,t)&&vr.call(e,t)){let e=br[t];console.warn(`[react-markdown] Warning: please ${e.to?`use \`${e.to}\` instead of`:"remove"} \`${t}\` (see <https://github.com/remarkjs/react-markdown/blob/main/changelog.md#${e.id}> for more info)`),delete br[t]}let t=Q().use(Jt).use(e.remarkPlugins||[]).use(wn,p(d({},e.remarkRehypeOptions),{allowDangerousHtml:!0})).use(e.rehypePlugins||[]).use(ir,e),n=new W;"string"==typeof e.children?n.value=e.children:null!=e.children&&console.warn(`[react-markdown] Warning: please pass a string as \`children\` (not: \`${e.children}\`)`);let r=t.runSync(t.parse(n),n);if("root"!==r.type)throw new TypeError("Expected a `root` node");let a=L.default.createElement(L.default.Fragment,{},pr({options:e,schema:ar,listDepth:0},r));return e.className&&(a=L.default.createElement("div",{className:e.className},a)),a}return Er.propTypes={children:Nn.default.string,className:Nn.default.string,allowElement:Nn.default.func,allowedElements:Nn.default.arrayOf(Nn.default.string),disallowedElements:Nn.default.arrayOf(Nn.default.string),unwrapDisallowed:Nn.default.bool,remarkPlugins:Nn.default.arrayOf(Nn.default.oneOfType([Nn.default.object,Nn.default.func,Nn.default.arrayOf(Nn.default.oneOfType([Nn.default.bool,Nn.default.string,Nn.default.object,Nn.default.func,Nn.default.arrayOf(Nn.default.any)]))])),rehypePlugins:Nn.default.arrayOf(Nn.default.oneOfType([Nn.default.object,Nn.default.func,Nn.default.arrayOf(Nn.default.oneOfType([Nn.default.bool,Nn.default.string,Nn.default.object,Nn.default.func,Nn.default.arrayOf(Nn.default.any)]))])),sourcePos:Nn.default.bool,rawSourcePos:Nn.default.bool,skipHtml:Nn.default.bool,includeElementIndex:Nn.default.bool,transformLinkUri:Nn.default.oneOfType([Nn.default.func,Nn.default.bool]),linkTarget:Nn.default.oneOfType([Nn.default.func,Nn.default.string]),transformImageUri:Nn.default.func,components:Nn.default.object},(e=>g(t({},"__esModule",{value:!0}),e))(k)})()}));const Fc=["node","inline","className","children"];function zc({solution:e}){return c.createElement("div",{className:"grid grid-cols-1 gap-2"},c.createElement(jc,{children:e.description,components:{code(e){let{inline:t,className:n,children:r}=e,a=Ce(e,Fc);const o=/language-(\w+)/.exec(n||"");return t?c.createElement("code",Ne({},a,{className:n,style:{background:"rgba(255,255,255,0.75)",padding:"0.15rem 0.25rem"}}),r):c.createElement(Il,Ne({},a,{language:o?o[1]:null,children:String(r).replace(/\n$/,""),customStyle:{margin:"0.5rem 0",background:"rgba(255,255,255,0.75)",padding:"0.25rem 0.5rem",overflowX:"scroll"}}))}}}),e.action_description&&c.createElement("p",null,e.action_description),c.createElement("ul",{className:"grid grid-cols-1 gap-1 text-sm"},Object.entries(e.links).map(([e,t],n)=>c.createElement("li",{key:n},c.createElement("a",{href:t,target:"_blank",className:"underline text-emerald-700 hover:text-emerald-800"},e)))))}function Bc({solution:e}){const[t,n]=c.useState(!1),[r,a]=c.useState(null);function o(e){e.preventDefault(),location.reload()}return c.createElement(c.Fragment,null,null===r&&c.createElement(c.Fragment,null,c.createElement(Uc,{onClick:async function(){if(!t)try{if(n(!0),!e.execute_endpoint)return;const t=await fetch(e.execute_endpoint,{method:"POST",headers:{"Content-Type":"application/json",Accept:"application/json"},body:JSON.stringify({solution:e.class,parameters:e.run_parameters})});a(t.status>=200&&t.status<300)}catch(e){console.error(e),a(!1)}finally{n(!1)}},disabled:t,className:"mb-4 inline-flex items-center gap-2 bg-emerald-600 border-emerald-500/25 text-white"},t?c.createElement("span",null,"Running..."):c.createElement(c.Fragment,null,c.createElement(qa,{className:"opacity-50",icon:Ao}),e.run_button_text||"Run")),c.createElement(zc,{solution:e})),!0===r&&c.createElement("p",{className:""},"The solution was executed ",c.createElement("strong",null,"successfully"),".",c.createElement("br",null),c.createElement("a",{href:"#",className:"mt-2 inline-flex items-center gap-2 underline text-emerald-700 hover:text-emerald-800",onClick:o},c.createElement(qa,{icon:bo,className:"text-sm opacity-50"}),"Refresh now")),!1===r&&c.createElement(c.Fragment,null,c.createElement("p",{className:"bg-red-200 px-4 py-2"},"Something ",c.createElement("strong",null,"went wrong"),". Please try refreshing the page and try again.",c.createElement("br",null),c.createElement("a",{href:"#",className:"mt-2 inline-flex items-center gap-2 underline text-red-700 hover:text-red-800",onClick:o},c.createElement(qa,{icon:bo,className:"text-sm opacity-50"}),"Refresh now"))))}function Hc({solution:e,isOpen:t=!1,isCollapsible:n=!0,canExecute:r=!1}){const[a,o]=c.useState(t);return c.createElement("section",null,c.createElement("header",{className:"group mb-4"},n?c.createElement("button",{className:"flex items-center justify-start",onClick:()=>{o(!a)}},c.createElement("span",{className:"w-6 -ml-6"},c.createElement(qa,{icon:Ja,className:"group-hover:opacity-50 opacity-0 text-sm transform transition "+(a?"":"-rotate-90")})),c.createElement("h2",{className:"min-w-0 truncate font-semibold leading-snug"},e.title)):c.createElement("h2",{className:"truncate font-semibold leading-snug"},e.title)),c.createElement("div",{className:a?"":"hidden"},c.createElement(e.is_runnable&&r?Bc:zc,{solution:e}),e.ai_generated&&c.createElement(c.Fragment,null,c.createElement("hr",{className:"border-emerald-500/50 my-4"}),c.createElement("div",{className:"flex items-center gap-x-2 text-green-700"},c.createElement(qa,{fixedWidth:!0,icon:vo}),c.createElement("span",{id:"ai-message",className:"text-xs"},"This solution was ",c.createElement("a",{className:"underline",target:"_blank",href:"https://flareapp.io/docs/ignition/solutions/ai-powered-solutions"},"generated by AI")," and might not be 100% accurate.")))))}function Vc(){const{solutions:e}=c.useContext(be),[t,n]=c.useState(!1),[r,a]=c.useState(!0);return c.useEffect(()=>{try{(async()=>{var t;if(null==(t=e[0])||!t.execute_endpoint)return;const r=await(await fetch(e[0].execute_endpoint.replace("execute-solution","health-check"))).json();n(r.can_execute_commands)})()}catch(e){console.error(e),n(!1)}},[]),c.createElement(c.Fragment,null,r?c.createElement("aside",{id:"solution",className:"relative flex flex-col lg:w-2/5 flex-none"},c.createElement("div",{className:"flex-grow px-6 sm:px-10 py-8 bg-emerald-300 text-gray-800 rounded-bl-lg rounded-br-lg @4xl:rounded-bl-none @4xl:rounded-r-lg"},c.createElement("button",{onClick:()=>a(!1),className:"absolute top-3 right-4 leading-none text-emerald-500 hover:text-emerald-700 text-sm"},c.createElement(qa,{icon:Io})),e.map((n,r)=>c.createElement("div",{key:r},c.createElement(Hc,{solution:n,canExecute:t,isOpen:0===r,isCollapsible:e.length>1}),r!==e.length-1&&c.createElement("hr",{className:"my-4 border-t border-gray-800/20"}))))):c.createElement("button",{onClick:()=>a(!0),className:"\n absolute -top-3 -right-3 z-20\n w-6 h-6 rounded-full flex items-center justify-center\n text-xs bg-emerald-500 text-white hover:shadow-lg\n shadow-md\n active:shadow-sm active:translate-y-px"},c.createElement(qa,{icon:po})))}var Wc={prefix:"fab",iconName:"laravel",icon:[512,512,[],"f3bd","M504.4 115.8a5.72 5.72 0 0 0 -.28-.68 8.52 8.52 0 0 0 -.53-1.25 6 6 0 0 0 -.54-.71 9.36 9.36 0 0 0 -.72-.94c-.23-.22-.52-.4-.77-.6a8.84 8.84 0 0 0 -.9-.68L404.4 55.55a8 8 0 0 0 -8 0L300.1 111h0a8.07 8.07 0 0 0 -.88 .69 7.68 7.68 0 0 0 -.78 .6 8.23 8.23 0 0 0 -.72 .93c-.17 .24-.39 .45-.54 .71a9.7 9.7 0 0 0 -.52 1.25c-.08 .23-.21 .44-.28 .68a8.08 8.08 0 0 0 -.28 2.08V223.2l-80.22 46.19V63.44a7.8 7.8 0 0 0 -.28-2.09c-.06-.24-.2-.45-.28-.68a8.35 8.35 0 0 0 -.52-1.24c-.14-.26-.37-.47-.54-.72a9.36 9.36 0 0 0 -.72-.94 9.46 9.46 0 0 0 -.78-.6 9.8 9.8 0 0 0 -.88-.68h0L115.6 1.07a8 8 0 0 0 -8 0L11.34 56.49h0a6.52 6.52 0 0 0 -.88 .69 7.81 7.81 0 0 0 -.79 .6 8.15 8.15 0 0 0 -.71 .93c-.18 .25-.4 .46-.55 .72a7.88 7.88 0 0 0 -.51 1.24 6.46 6.46 0 0 0 -.29 .67 8.18 8.18 0 0 0 -.28 2.1v329.7a8 8 0 0 0 4 6.95l192.5 110.8a8.83 8.83 0 0 0 1.33 .54c.21 .08 .41 .2 .63 .26a7.92 7.92 0 0 0 4.1 0c.2-.05 .37-.16 .55-.22a8.6 8.6 0 0 0 1.4-.58L404.4 400.1a8 8 0 0 0 4-6.95V287.9l92.24-53.11a8 8 0 0 0 4-7V117.9A8.63 8.63 0 0 0 504.4 115.8zM111.6 17.28h0l80.19 46.15-80.2 46.18L31.41 63.44zm88.25 60V278.6l-46.53 26.79-33.69 19.4V123.5l46.53-26.79zm0 412.8L23.37 388.5V77.32L57.06 96.7l46.52 26.8V338.7a6.94 6.94 0 0 0 .12 .9 8 8 0 0 0 .16 1.18h0a5.92 5.92 0 0 0 .38 .9 6.38 6.38 0 0 0 .42 1v0a8.54 8.54 0 0 0 .6 .78 7.62 7.62 0 0 0 .66 .84l0 0c.23 .22 .52 .38 .77 .58a8.93 8.93 0 0 0 .86 .66l0 0 0 0 92.19 52.18zm8-106.2-80.06-45.32 84.09-48.41 92.26-53.11 80.13 46.13-58.8 33.56zm184.5 4.57L215.9 490.1V397.8L346.6 323.2l45.77-26.15zm0-119.1L358.7 250l-46.53-26.79V131.8l33.69 19.4L392.4 178zm8-105.3-80.2-46.17 80.2-46.16 80.18 46.15zm8 105.3V178L455 151.2l33.68-19.4v91.39h0z"]};function Gc(){const e=c.useContext(be);return c.createElement("span",{className:"py-1 px-4 items-center flex gap-3 rounded-sm ~bg-gray-500/5"},c.createElement(kt,{path:e.exception_class}))}function Yc({githubLink:e}){return c.createElement("section",{className:"flex flex-col flex-grow px-6 sm:px-10 py-8 bg-red-600 text-red-100 shadow-lg gap-3"},c.createElement("h2",{className:"text-xl font-semibold leading-snug"},"Something went wrong in Ignition!"),c.createElement("p",{className:"text-base"},"An error occurred in Ignition's UI. Please open an issue on"," ",c.createElement("a",{href:e,target:"_blank",className:"underline"},"the Ignition GitHub repo")," ","and make sure to include any errors or warnings in the developer console."))}class $c extends c.Component{constructor(e){super(e),this.state={error:null}}static getDerivedStateFromError(e){return{error:e}}render(){const{error:e}=this.state;if(e){var t,n;let r="https://github.com/spatie/ignition/issues";if(e instanceof Error){const t=`\n**Please include some context and the contents of the console in your browser's developer tools.**\n\n## JavaScript Error\n\`\`\`\n${e.stack}\n\`\`\`\n\n## Reproduction Steps\nPlease tell us what you were doing when this error occurred, so we can more easily debug it and find a solution.\n\n1. …\n\n## User Agent\n\`\`\`\n${navigator.userAgent}\n\`\`\`\n`;r=`https://github.com/spatie/ignition/issues/new?title=${e.name}: ${e.message}&labels=bug&body=${encodeURIComponent(t)}`}return(null==(t=(n=this.props).fallbackComponent)?void 0:t.call(n,r))||c.createElement(Yc,{githubLink:r})}return this.props.children}}function Xc(){var e;const t=c.useContext(be),n=t.solutions.length>0,r=!(null==(e=t.context_items.env)||!e.laravel_version);return c.createElement($c,null,c.createElement("section",{className:"@container | bg-white dark:shadow-none dark:bg-gray-800/50 dark:bg-gradient-to-bl from-gray-700/50 via-transparent dark:ring-1 dark:ring-inset dark:ring-white/5 rounded-lg shadow-2xl shadow-gray-500/20"},c.createElement("div",{className:"@4xl:flex items-stretch"},c.createElement("main",{id:"exception",className:"z-10 flex-grow min-w-0"},c.createElement("div",{className:"overflow-hidden"},c.createElement("div",{className:"px-6 @lg:px-10 py-8 overflow-x-auto"},c.createElement("header",{className:"flex items-center justify-between gap-2"},c.createElement(Gc,null),c.createElement("div",{className:"grid grid-flow-col justify-end gap-4 text-sm ~text-gray-500"},c.createElement("span",null,c.createElement("span",{className:"tracking-wider"},"PHP")," ",t.language_version),t.framework_version&&c.createElement("span",{className:"inline-flex items-center gap-1"},c.createElement(qa,{icon:r?Wc:oo}),t.framework_version))),c.createElement(Mc,{exceptionClass:t.exception_class,message:t.exception_message})))),n&&c.createElement(Vc,null))))}const qc=(e,t)=>e.join(t).toLowerCase(),Kc=/[\u0300-\u036F\u1AB0-\u1AFF\u1DC0-\u1DFF]+/g,Jc=/[A-Za-z\d]+/g,Qc=new RegExp("[A-Za-z\\d]*?(?:[a-z](?=[A-Z])|[A-Z](?=[A-Z][a-z]))|[A-Za-z\\d]+","g");function Zc(e,{camelCase:t=!0,dictionary:n,separator:r="-",transformer:a=qc}={}){const o=(n?function(e,t){for(let n=0,r=e.length;n<r;n++){const a=e[n],o=t[a]&&String(t[a]);if(void 0!==o){e=e.slice(0,n)+o+e.slice(n+1);const t=o.length-1;n+=t,r+=t}}return e}(String(e),n):String(e)).normalize("NFKD").replace(Kc,"").match(t?Qc:Jc);return o?a?a(o,String(r)):o.join(String(r)):""}function eu({className:e="",githubLink:t}){return c.createElement("div",{className:`${e} flex flex-col gap-2 bg-red-50 dark:bg-red-500/10 px-6 py-4`},c.createElement("h2",{className:"font-semibold leading-snug"},"Something went wrong in Ignition!"),c.createElement("p",{className:"text-base"},"An error occurred in Ignition's UI. Please open an issue on"," ",c.createElement("a",{href:t,target:"_blank",className:"underline"},"the Ignition GitHub repo")," ","and make sure to include any errors or warnings in the developer console."))}function tu({title:e,children:t,anchor:n}){return c.createElement("section",{className:"py-10 ~bg-white px-6 @lg:px-10 min-w-0"},c.createElement("a",{id:`context-${n}`,className:"scroll-target"}),c.createElement("h2",{className:"font-bold text-xs ~text-gray-500 uppercase tracking-wider"},e),c.createElement("div",{className:"mt-3 grid grid-cols-1 gap-10"},c.createElement($c,{fallbackComponent:e=>c.createElement(eu,{githubLink:e})},t)))}var nu=/* @__PURE__ */new Map,ru=/* @__PURE__ */new WeakMap,au=0,ou=c.createContext({inView:[],setInView:Ee});function iu({icon:e,title:t,children:n,anchor:r}){const a=function(e){const{setInView:t}=c.useContext(ou),{ref:n,inView:r}=function({threshold:e,delay:t,trackVisibility:n,rootMargin:r,root:a,triggerOnce:o,skip:i,initialInView:l,fallbackInView:s,onChange:u}={}){var f;const[d,p]=c.useState(null),m=c.useRef(),[h,g]=c.useState({inView:!!l,entry:void 0});m.current=u,c.useEffect(()=>{if(i||!d)return;let l;return l=function(e,t,n={},r){if(void 0===window.IntersectionObserver&&void 0!==r){const a=e.getBoundingClientRect();return t(r,{isIntersecting:r,target:e,intersectionRatio:"number"==typeof n.threshold?n.threshold:0,time:0,boundingClientRect:a,intersectionRect:a,rootBounds:a}),()=>{}}const{id:a,observer:o,elements:i}=function(e){let t=function(e){return Object.keys(e).sort().filter(t=>void 0!==e[t]).map(t=>{return`${t}_${"root"===t?(n=e.root,n?(ru.has(n)||ru.set(n,(au+=1).toString()),ru.get(n)):"0"):e[t]}`;var n}).toString()}(e),n=nu.get(t);if(!n){const r=/* @__PURE__ */new Map;let a;const o=new IntersectionObserver(t=>{t.forEach(t=>{var n;const o=t.isIntersecting&&a.some(e=>t.intersectionRatio>=e);e.trackVisibility&&void 0===t.isVisible&&(t.isVisible=o),null==(n=r.get(t.target))||n.forEach(e=>{e(o,t)})})},e);a=o.thresholds||(Array.isArray(e.threshold)?e.threshold:[e.threshold||0]),n={id:t,observer:o,elements:r},nu.set(t,n)}return n}(n);let l=i.get(e)||[];return i.has(e)||i.set(e,l),l.push(t),o.observe(e),function(){l.splice(l.indexOf(t),1),0===l.length&&(i.delete(e),o.unobserve(e)),0===i.size&&(o.disconnect(),nu.delete(a))}}(d,(e,t)=>{g({inView:e,entry:t}),m.current&&m.current(e,t),t.isIntersecting&&o&&l&&(l(),l=void 0)},{root:a,rootMargin:r,threshold:e,trackVisibility:n,delay:t},s),()=>{l&&l()}},[Array.isArray(e)?e.toString():e,d,a,r,o,i,n,s,t]);const y=null==(f=h.entry)?void 0:f.target,v=c.useRef();d||!y||o||i||v.current===y||(v.current=y,g({inView:!!l,entry:void 0}));const b=[p,h.inView,h.entry];return b.ref=b[0],b.inView=b[1],b.entry=b[2],b}({rootMargin:"-45% 0px -45%"});return c.useEffect(()=>{t(r?t=>[...t,e]:t=>t.filter(t=>t!==e))},[r]),n}(t);return c.createElement("div",{ref:a},c.createElement("a",{id:`context-${r}`,className:"scroll-target"}),c.createElement("h1",{className:"mb-2 flex items-center gap-2 font-semibold text-lg ~text-indigo-600"},t,c.createElement("span",{className:"opacity-50 ~text-indigo-600 text-sm"},e)),c.createElement($c,{fallbackComponent:e=>c.createElement(eu,{githubLink:e})},n))}function lu({children:e,className:t="",color:n="gray"}){return c.createElement("div",{className:`${t} ${{red:"~text-red-600 border-red-500/50",orange:"~text-orange-600 border-orange-500/50",green:"~text-emerald-600 border-emerald-500/50",blue:"~text-indigo-600 border-indigo-500/50",purple:"~text-violet-600 border-violet-600/50",gray:"~text-gray-500 border-gray-500/50"}[n]} px-1.5 py-0.5 rounded-sm bg-opacity-20 border text-xs font-medium uppercase tracking-wider`},e)}function su({request:e,requestData:t,headers:n}){const r=c.useMemo(()=>function(e,t,n){if(!e.url||!e.method)return null;const r=[`curl "${e.url}"`];r.push(` -X ${e.method}`),Object.entries(n||{}).map(function([e,t]){r.push(` -H '${e}: ${t}'`)});const a=function(e,t){var n,r;return e.body?null!=(n=t["content-type"])&&null!=(r=n[0])&&r.includes("application/json")?` -d ${JSON.stringify(e.body)}`:` ${Object.entries(e.body||{}).map(function([e,t]){return`-F '${e}=${t}'`}).join(" ")}`:null}(t,n);return a&&r.push(a),r.join(" \\\n").trimEnd().replace(/\s\\$/g,";")}(e,t,n),[e,t,n]);return c.createElement("div",null,c.createElement("div",{className:"text-lg font-semibold flex items-center gap-2"},c.createElement("span",{className:"~text-indigo-600"},e.url),e.method&&c.createElement(lu,{color:"DELETE"==e.method.toUpperCase()?"red":"blue"},e.method.toUpperCase())),r&&c.createElement("div",{className:"mt-2"},c.createElement(xc,{value:r,language:"curl"})))}function cu({items:e}){return c.createElement(Ac,null,Object.entries(e||{}).map(([e,t])=>c.createElement(Ac.Row,{key:e,label:e,value:t})))}var uu=function(e){return null==e},fu=Object.prototype.hasOwnProperty,du=function(e){if(null==e)return!0;if(bs(e)&&(Vl(e)||"string"==typeof e||"function"==typeof e.splice||ns(e)||us(e)||es(e)))return!e.length;var t=Ls(e);if("[object Map]"==t||"[object Set]"==t)return!e.size;if(ms(e))return!vs(e).length;for(var n in e)if(fu.call(e,n))return!1;return!0},pu=function(){try{var e=Ze(Object,"defineProperty");return e({},"",{}),e}catch(e){}}(),mu=Object.prototype.hasOwnProperty,hu=function(e,t,n){var r=e[t];mu.call(e,t)&&ot(r,n)&&(void 0!==n||t in e)||function(e,t,n){"__proto__"==t&&pu?pu(e,t,{configurable:!0,enumerable:!0,value:n,writable:!0}):e[t]=n}(e,t,n)},gu=function(e,t,n,r){if(!Ge(e))return e;for(var a=-1,o=(t=Qs(t,e)).length,i=o-1,l=e;null!=l&&++a<o;){var s=Zs(t[a]),c=n;if("__proto__"===s||"constructor"===s||"prototype"===s)return e;if(a!=i){var u=l[s];void 0===(c=r?r(u,s,l):void 0)&&(c=Ge(u)?u:as(t[a+1])?[]:{})}hu(l,s,c),l=l[s]}return e},yu=hs(Object.getPrototypeOf,Object),vu=Object.getOwnPropertySymbols?function(e){for(var t=[];e;)Hl(t,Xl(e)),e=yu(e);return t}:Gl,bu=Object.prototype.hasOwnProperty,Eu=function(e){return bs(e)?ds(e,!0):function(e){if(!Ge(e))return function(e){var t=[];if(null!=e)for(var n in Object(e))t.push(n);return t}(e);var t=ms(e),n=[];for(var r in e)("constructor"!=r||!t&&bu.call(e,r))&&n.push(r);return n}(e)},Tu=function(e,t){return function(e,t){if(null==e)return{};var n=$s(function(e){return Wl(e,Eu,vu)}(e),function(e){return[e]});return t=rc(t),function(e,t,n){for(var r=-1,a=t.length,o={};++r<a;){var i=t[r],l=ec(e,i);n(l,i)&&gu(o,Qs(i,e),l)}return o}(e,n,function(e,n){return t(e,n[0])})}(e,function(e){if("function"!=typeof e)throw new TypeError("Expected a function");return function(){var t=arguments;switch(t.length){case 0:return!e.call(this);case 1:return!e.call(this,t[0]);case 2:return!e.call(this,t[0],t[1]);case 3:return!e.call(this,t[0],t[1],t[2])}return!e.apply(this,t)}}(rc(t)))};function Su({headers:e}){let t=Tu(e,uu);return t=Tu(t,du),c.createElement(cu,{items:t})}function wu({requestData:e}){return c.createElement(cu,{items:e.queryString||{}})}function Nu(){var e,t;const n=null==(e=c.useContext(be).context_items)||null==(t=e.request_data)?void 0:t.body;return n?c.createElement(xc,{value:Do(n)}):null}function Ru(){var e,t;const n=null==(e=c.useContext(be).context_items)||null==(t=e.request_data)?void 0:t.files;return n?c.createElement("div",{className:"col-span-2"},c.createElement(xc,{value:Do(n)})):null}function Ou({session:e}){return c.createElement(cu,{items:e})}function Cu({cookies:e}){return c.createElement(cu,{items:e})}function xu(){const e=c.useContext(be).context_items.livewire;return e?c.createElement(cu,{items:e.data}):null}function ku(){const e=c.useContext(be).context_items.livewire;return e?c.createElement(cu,{items:{Component:e.component_class,Alias:e.component_alias,ID:e.component_id}}):null}function Au(){const e=c.useContext(be).context_items.livewire;return e?c.createElement(Ac,null,e.updates.map(({payload:e,type:t},n)=>c.createElement(Ac.Row,{key:n,label:t,value:e}))):null}const Iu=["children","className"];function Lu(e){let{children:t,className:n=""}=e,r=Ce(e,Iu);return c.createElement(c.Fragment,null,t&&c.createElement("ul",Ne({className:`gap-y-2 flex flex-col ${n}`},r),t))}function _u({route:e}){var t;return c.createElement(Ac,null,c.createElement(Ac.Row,{value:e.controllerAction,label:"Controller"}),e.route&&c.createElement(Ac.Row,{value:e.route,label:"Route name"}),!(null==(t=e.routeParameters)||!t.length)&&c.createElement(Ac.Row,{value:c.createElement(Ac,null,Object.entries(e.routeParameters).map(([e,t])=>c.createElement(Ac.Row,{stacked:!0,key:e,label:e,value:t}))),label:"Route parameters"}),e.middleware&&c.createElement(Ac.Row,{value:c.createElement(Lu,null,(e.middleware||[]).map((e,t)=>c.createElement(Lu.Item,{key:t,value:e}))),label:"Middleware"}))}Lu.Item=function({value:e=""}){let t=e;return c.isValidElement(e)?t=e:"object"==typeof e?t=c.createElement(xc,{value:Do(e),language:"json"}):"string"==typeof e&&(t=c.createElement(xc,{value:e})),c.createElement("li",null,t)};const Pu=["value"];function Mu(e){let{value:t}=e,n=Ce(e,Pu);return c.useEffect(()=>{const e=t.match(/sf-dump-\d+/);e&&window.Sfdump(e[0])},[t]),c.createElement("div",Ne({className:"~bg-gray-500/5 px-4 py-2",dangerouslySetInnerHTML:{__html:t}},n))}function Du(){const e=c.useContext(be).context_items.view;return e?c.createElement(Ac,null,c.createElement(Ac.Row,{value:c.createElement(Bo,{path:e.view}),label:"View"}),e.data&&c.createElement(Ac.Row,{value:c.createElement(Ac,null,Object.entries(e.data).map(([e,t])=>c.createElement(Ac.Row,{stacked:!0,key:e,label:e,value:c.createElement(Mu,{value:t})}))),label:"Data"})):null}var Uu=Le(function(e){!function(){var t="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/",n={rotl:function(e,t){return e<<t|e>>>32-t},rotr:function(e,t){return e<<32-t|e>>>t},endian:function(e){if(e.constructor==Number)return 16711935&n.rotl(e,8)|4278255360&n.rotl(e,24);for(var t=0;t<e.length;t++)e[t]=n.endian(e[t]);return e},randomBytes:function(e){for(var t=[];e>0;e--)t.push(Math.floor(256*Math.random()));return t},bytesToWords:function(e){for(var t=[],n=0,r=0;n<e.length;n++,r+=8)t[r>>>5]|=e[n]<<24-r%32;return t},wordsToBytes:function(e){for(var t=[],n=0;n<32*e.length;n+=8)t.push(e[n>>>5]>>>24-n%32&255);return t},bytesToHex:function(e){for(var t=[],n=0;n<e.length;n++)t.push((e[n]>>>4).toString(16)),t.push((15&e[n]).toString(16));return t.join("")},hexToBytes:function(e){for(var t=[],n=0;n<e.length;n+=2)t.push(parseInt(e.substr(n,2),16));return t},bytesToBase64:function(e){for(var n=[],r=0;r<e.length;r+=3)for(var a=e[r]<<16|e[r+1]<<8|e[r+2],o=0;o<4;o++)n.push(8*r+6*o<=8*e.length?t.charAt(a>>>6*(3-o)&63):"=");return n.join("")},base64ToBytes:function(e){e=e.replace(/[^A-Z0-9+\/]/gi,"");for(var n=[],r=0,a=0;r<e.length;a=++r%4)0!=a&&n.push((t.indexOf(e.charAt(r-1))&Math.pow(2,-2*a+8)-1)<<2*a|t.indexOf(e.charAt(r))>>>6-2*a);return n}};e.exports=n}()}),ju={utf8:{stringToBytes:function(e){return ju.bin.stringToBytes(unescape(encodeURIComponent(e)))},bytesToString:function(e){return decodeURIComponent(escape(ju.bin.bytesToString(e)))}},bin:{stringToBytes:function(e){for(var t=[],n=0;n<e.length;n++)t.push(255&e.charCodeAt(n));return t},bytesToString:function(e){for(var t=[],n=0;n<e.length;n++)t.push(String.fromCharCode(e[n]));return t.join("")}}},Fu=ju,zu=function(e){return null!=e&&(Bu(e)||function(e){return"function"==typeof e.readFloatLE&&"function"==typeof e.slice&&Bu(e.slice(0,0))}(e)||!!e._isBuffer)};function Bu(e){return!!e.constructor&&"function"==typeof e.constructor.isBuffer&&e.constructor.isBuffer(e)}var Hu=Le(function(e){!function(){var t=Uu,n=Fu.utf8,r=zu,a=Fu.bin,o=function e(o,i){o.constructor==String?o=i&&"binary"===i.encoding?a.stringToBytes(o):n.stringToBytes(o):r(o)?o=Array.prototype.slice.call(o,0):Array.isArray(o)||o.constructor===Uint8Array||(o=o.toString());for(var l=t.bytesToWords(o),s=8*o.length,c=1732584193,u=-271733879,f=-1732584194,d=271733878,p=0;p<l.length;p++)l[p]=16711935&(l[p]<<8|l[p]>>>24)|4278255360&(l[p]<<24|l[p]>>>8);l[s>>>5]|=128<<s%32,l[14+(s+64>>>9<<4)]=s;var m=e._ff,h=e._gg,g=e._hh,y=e._ii;for(p=0;p<l.length;p+=16){var v=c,b=u,E=f,T=d;c=m(c,u,f,d,l[p+0],7,-680876936),d=m(d,c,u,f,l[p+1],12,-389564586),f=m(f,d,c,u,l[p+2],17,606105819),u=m(u,f,d,c,l[p+3],22,-1044525330),c=m(c,u,f,d,l[p+4],7,-176418897),d=m(d,c,u,f,l[p+5],12,1200080426),f=m(f,d,c,u,l[p+6],17,-1473231341),u=m(u,f,d,c,l[p+7],22,-45705983),c=m(c,u,f,d,l[p+8],7,1770035416),d=m(d,c,u,f,l[p+9],12,-1958414417),f=m(f,d,c,u,l[p+10],17,-42063),u=m(u,f,d,c,l[p+11],22,-1990404162),c=m(c,u,f,d,l[p+12],7,1804603682),d=m(d,c,u,f,l[p+13],12,-40341101),f=m(f,d,c,u,l[p+14],17,-1502002290),c=h(c,u=m(u,f,d,c,l[p+15],22,1236535329),f,d,l[p+1],5,-165796510),d=h(d,c,u,f,l[p+6],9,-1069501632),f=h(f,d,c,u,l[p+11],14,643717713),u=h(u,f,d,c,l[p+0],20,-373897302),c=h(c,u,f,d,l[p+5],5,-701558691),d=h(d,c,u,f,l[p+10],9,38016083),f=h(f,d,c,u,l[p+15],14,-660478335),u=h(u,f,d,c,l[p+4],20,-405537848),c=h(c,u,f,d,l[p+9],5,568446438),d=h(d,c,u,f,l[p+14],9,-1019803690),f=h(f,d,c,u,l[p+3],14,-187363961),u=h(u,f,d,c,l[p+8],20,1163531501),c=h(c,u,f,d,l[p+13],5,-1444681467),d=h(d,c,u,f,l[p+2],9,-51403784),f=h(f,d,c,u,l[p+7],14,1735328473),c=g(c,u=h(u,f,d,c,l[p+12],20,-1926607734),f,d,l[p+5],4,-378558),d=g(d,c,u,f,l[p+8],11,-2022574463),f=g(f,d,c,u,l[p+11],16,1839030562),u=g(u,f,d,c,l[p+14],23,-35309556),c=g(c,u,f,d,l[p+1],4,-1530992060),d=g(d,c,u,f,l[p+4],11,1272893353),f=g(f,d,c,u,l[p+7],16,-155497632),u=g(u,f,d,c,l[p+10],23,-1094730640),c=g(c,u,f,d,l[p+13],4,681279174),d=g(d,c,u,f,l[p+0],11,-358537222),f=g(f,d,c,u,l[p+3],16,-722521979),u=g(u,f,d,c,l[p+6],23,76029189),c=g(c,u,f,d,l[p+9],4,-640364487),d=g(d,c,u,f,l[p+12],11,-421815835),f=g(f,d,c,u,l[p+15],16,530742520),c=y(c,u=g(u,f,d,c,l[p+2],23,-995338651),f,d,l[p+0],6,-198630844),d=y(d,c,u,f,l[p+7],10,1126891415),f=y(f,d,c,u,l[p+14],15,-1416354905),u=y(u,f,d,c,l[p+5],21,-57434055),c=y(c,u,f,d,l[p+12],6,1700485571),d=y(d,c,u,f,l[p+3],10,-1894986606),f=y(f,d,c,u,l[p+10],15,-1051523),u=y(u,f,d,c,l[p+1],21,-2054922799),c=y(c,u,f,d,l[p+8],6,1873313359),d=y(d,c,u,f,l[p+15],10,-30611744),f=y(f,d,c,u,l[p+6],15,-1560198380),u=y(u,f,d,c,l[p+13],21,1309151649),c=y(c,u,f,d,l[p+4],6,-145523070),d=y(d,c,u,f,l[p+11],10,-1120210379),f=y(f,d,c,u,l[p+2],15,718787259),u=y(u,f,d,c,l[p+9],21,-343485551),c=c+v>>>0,u=u+b>>>0,f=f+E>>>0,d=d+T>>>0}return t.endian([c,u,f,d])};o._ff=function(e,t,n,r,a,o,i){var l=e+(t&n|~t&r)+(a>>>0)+i;return(l<<o|l>>>32-o)+t},o._gg=function(e,t,n,r,a,o,i){var l=e+(t&r|n&~r)+(a>>>0)+i;return(l<<o|l>>>32-o)+t},o._hh=function(e,t,n,r,a,o,i){var l=e+(t^n^r)+(a>>>0)+i;return(l<<o|l>>>32-o)+t},o._ii=function(e,t,n,r,a,o,i){var l=e+(n^(t|~r))+(a>>>0)+i;return(l<<o|l>>>32-o)+t},o._blocksize=16,o._digestsize=16,e.exports=function(e,n){if(null==e)throw new Error("Illegal argument "+e);var r=t.wordsToBytes(o(e,n));return n&&n.asBytes?r:n&&n.asString?a.bytesToString(r):t.bytesToHex(r)}}()});function Vu({user:e}){return c.createElement(c.Fragment,null,e.email&&c.createElement("div",{className:"mb-2 flex items-center gap-3"},c.createElement("div",null,c.createElement("img",{className:"inline-block h-9 w-9 rounded-full",alt:e.email,src:`https://gravatar.com/avatar/${Hu(e.email)}/?s=240`})),c.createElement("div",{className:"leading-tight"},e.name&&c.createElement("p",{className:"font-semibold"},e.name),c.createElement("p",{className:"text-sm"},e.email))),c.createElement(xc,{value:Do(e),language:"json"}))}function Wu({children:e,className:t=""}){return c.createElement("div",{className:`${t}`},c.createElement("div",{className:"flex items-center gap-2 bg-yellow-50 dark:bg-yellow-500/10 px-4 py-2"},c.createElement("div",{className:"flex-shrink-0","aria-hidden":"true"},c.createElement(qa,{className:"text-yellow-500 ",icon:Oo})),c.createElement("p",{className:"text-sm"},e)))}var Gu=function(e,t){!0===t&&(t=0);var n=e.indexOf("://"),r=e.substring(0,n).split("+").filter(Boolean);return"number"==typeof t?r[t]:r},Yu=function e(t){if(Array.isArray(t))return-1!==t.indexOf("ssh")||-1!==t.indexOf("rsync");if("string"!=typeof t)return!1;var n=Gu(t);if(t=t.substring(t.indexOf("://")+3),e(n))return!0;var r=new RegExp(".([a-zA-Z\\d]+):(\\d+)/");return!t.match(r)&&t.indexOf("@")<t.indexOf(":")},$u=new RegExp("%[a-f0-9]{2}","gi"),Xu=new RegExp("(%[a-f0-9]{2})+","gi");function qu(e,t){try{return decodeURIComponent(e.join(""))}catch(e){}if(1===e.length)return e;var n=e.slice(0,t=t||1),r=e.slice(t);return Array.prototype.concat.call([],qu(n),qu(r))}function Ku(e){try{return decodeURIComponent(e)}catch(r){for(var t=e.match($u),n=1;n<t.length;n++)t=(e=qu(t,n).join("")).match($u);return e}}var Ju=function(e){if("string"!=typeof e)throw new TypeError("Expected `encodedURI` to be of type `string`, got `"+typeof e+"`");try{return e=e.replace(/\+/g," "),decodeURIComponent(e)}catch(t){return function(e){for(var t={"%FE%FF":"��","%FF%FE":"��"},n=Xu.exec(e);n;){try{t[n[0]]=decodeURIComponent(n[0])}catch(e){var r=Ku(n[0]);r!==n[0]&&(t[n[0]]=r)}n=Xu.exec(e)}t["%C2"]="�";for(var a=Object.keys(t),o=0;o<a.length;o++){var i=a[o];e=e.replace(new RegExp(i,"g"),t[i])}return e}(e)}},Qu=(e,t)=>{if("string"!=typeof e||"string"!=typeof t)throw new TypeError("Expected the arguments to be of type `string`");if(""===t)return[e];const n=e.indexOf(t);return-1===n?[e]:[e.slice(0,n),e.slice(n+t.length)]},Zu=function(e,t){for(var n={},r=Object.keys(e),a=Array.isArray(t),o=0;o<r.length;o++){var i=r[o],l=e[i];(a?-1!==t.indexOf(i):t(i,l,e))&&(n[i]=l)}return n},ef=Le(function(e,t){function n(e){if("string"!=typeof e||1!==e.length)throw new TypeError("arrayFormatSeparator must be single character string")}function r(e,t){return t.encode?t.strict?encodeURIComponent(e).replace(/[!'()*]/g,e=>`%${e.charCodeAt(0).toString(16).toUpperCase()}`):encodeURIComponent(e):e}function a(e,t){return t.decode?Ju(e):e}function o(e){return Array.isArray(e)?e.sort():"object"==typeof e?o(Object.keys(e)).sort((e,t)=>Number(e)-Number(t)).map(t=>e[t]):e}function i(e){const t=e.indexOf("#");return-1!==t&&(e=e.slice(0,t)),e}function l(e){const t=(e=i(e)).indexOf("?");return-1===t?"":e.slice(t+1)}function s(e,t){return t.parseNumbers&&!Number.isNaN(Number(e))&&"string"==typeof e&&""!==e.trim()?e=Number(e):!t.parseBooleans||null===e||"true"!==e.toLowerCase()&&"false"!==e.toLowerCase()||(e="true"===e.toLowerCase()),e}function c(e,t){n((t=Object.assign({decode:!0,sort:!0,arrayFormat:"none",arrayFormatSeparator:",",parseNumbers:!1,parseBooleans:!1},t)).arrayFormatSeparator);const r=function(e){let t;switch(e.arrayFormat){case"index":return(e,n,r)=>{t=/\[(\d*)\]$/.exec(e),e=e.replace(/\[\d*\]$/,""),t?(void 0===r[e]&&(r[e]={}),r[e][t[1]]=n):r[e]=n};case"bracket":return(e,n,r)=>{t=/(\[\])$/.exec(e),r[e=e.replace(/\[\]$/,"")]=t?void 0!==r[e]?[].concat(r[e],n):[n]:n};case"comma":case"separator":return(t,n,r)=>{const o="string"==typeof n&&n.includes(e.arrayFormatSeparator),i="string"==typeof n&&!o&&a(n,e).includes(e.arrayFormatSeparator);n=i?a(n,e):n;const l=o||i?n.split(e.arrayFormatSeparator).map(t=>a(t,e)):null===n?n:a(n,e);r[t]=l};default:return(e,t,n)=>{n[e]=void 0!==n[e]?[].concat(n[e],t):t}}}(t),i=Object.create(null);if("string"!=typeof e)return i;if(!(e=e.trim().replace(/^[?#&]/,"")))return i;for(const n of e.split("&")){if(""===n)continue;let[e,o]=Qu(t.decode?n.replace(/\+/g," "):n,"=");o=void 0===o?null:["comma","separator"].includes(t.arrayFormat)?o:a(o,t),r(a(e,t),o,i)}for(const e of Object.keys(i)){const n=i[e];if("object"==typeof n&&null!==n)for(const e of Object.keys(n))n[e]=s(n[e],t);else i[e]=s(n,t)}return!1===t.sort?i:(!0===t.sort?Object.keys(i).sort():Object.keys(i).sort(t.sort)).reduce((e,t)=>{const n=i[t];return e[t]=Boolean(n)&&"object"==typeof n&&!Array.isArray(n)?o(n):n,e},Object.create(null))}t.extract=l,t.parse=c,t.stringify=(e,t)=>{if(!e)return"";n((t=Object.assign({encode:!0,strict:!0,arrayFormat:"none",arrayFormatSeparator:","},t)).arrayFormatSeparator);const a=n=>t.skipNull&&null==e[n]||t.skipEmptyString&&""===e[n],o=function(e){switch(e.arrayFormat){case"index":return t=>(n,a)=>{const o=n.length;return void 0===a||e.skipNull&&null===a||e.skipEmptyString&&""===a?n:null===a?[...n,[r(t,e),"[",o,"]"].join("")]:[...n,[r(t,e),"[",r(o,e),"]=",r(a,e)].join("")]};case"bracket":return t=>(n,a)=>void 0===a||e.skipNull&&null===a||e.skipEmptyString&&""===a?n:null===a?[...n,[r(t,e),"[]"].join("")]:[...n,[r(t,e),"[]=",r(a,e)].join("")];case"comma":case"separator":return t=>(n,a)=>null==a||0===a.length?n:0===n.length?[[r(t,e),"=",r(a,e)].join("")]:[[n,r(a,e)].join(e.arrayFormatSeparator)];default:return t=>(n,a)=>void 0===a||e.skipNull&&null===a||e.skipEmptyString&&""===a?n:null===a?[...n,r(t,e)]:[...n,[r(t,e),"=",r(a,e)].join("")]}}(t),i={};for(const t of Object.keys(e))a(t)||(i[t]=e[t]);const l=Object.keys(i);return!1!==t.sort&&l.sort(t.sort),l.map(n=>{const a=e[n];return void 0===a?"":null===a?r(n,t):Array.isArray(a)?a.reduce(o(n),[]).join("&"):r(n,t)+"="+r(a,t)}).filter(e=>e.length>0).join("&")},t.parseUrl=(e,t)=>{t=Object.assign({decode:!0},t);const[n,r]=Qu(e,"#");return Object.assign({url:n.split("?")[0]||"",query:c(l(e),t)},t&&t.parseFragmentIdentifier&&r?{fragmentIdentifier:a(r,t)}:{})},t.stringifyUrl=(e,n)=>{n=Object.assign({encode:!0,strict:!0},n);const a=i(e.url).split("?")[0]||"",o=t.extract(e.url),l=t.parse(o,{sort:!1}),s=Object.assign(l,e.query);let c=t.stringify(s,n);c&&(c=`?${c}`);let u=function(e){let t="";const n=e.indexOf("#");return-1!==n&&(t=e.slice(n)),t}(e.url);return e.fragmentIdentifier&&(u=`#${r(e.fragmentIdentifier,n)}`),`${a}${c}${u}`},t.pick=(e,n,r)=>{r=Object.assign({parseFragmentIdentifier:!0},r);const{url:a,query:o,fragmentIdentifier:i}=t.parseUrl(e,r);return t.stringifyUrl({url:a,query:Zu(o,n),fragmentIdentifier:i},r)},t.exclude=(e,n,r)=>{const a=Array.isArray(n)?e=>!n.includes(e):(e,t)=>!n(e,t);return t.pick(e,a,r)}});function tf(e){if("string"!=typeof e)throw new Error("The url must be a string.");let t=function(e){let t=function(e){if("string"!=typeof e||!e.trim())throw new Error("Invalid url.");return function(e){e=(e||"").trim();var t={protocols:Gu(e),protocol:null,port:null,resource:"",user:"",pathname:"",hash:"",search:"",href:e,query:Object.create(null)},n=e.indexOf("://"),r=null,a=null;e.startsWith(".")&&(e.startsWith("./")&&(e=e.substring(2)),t.pathname=e,t.protocol="file");var o=e.charAt(1);return t.protocol||(t.protocol=t.protocols[0],t.protocol||(Yu(e)?t.protocol="ssh":"/"===o||"~"===o?(e=e.substring(2),t.protocol="file"):t.protocol="file")),-1!==n&&(e=e.substring(n+3)),a=e.split(/\/|\\/),t.resource="file"!==t.protocol?a.shift():"",2===(r=t.resource.split("@")).length&&(t.user=r[0],t.resource=r[1]),2===(r=t.resource.split(":")).length&&(t.resource=r[0],r[1]?(t.port=Number(r[1]),isNaN(t.port)&&(t.port=null,a.unshift(r[1]))):t.port=null),a=a.filter(Boolean),t.pathname="file"===t.protocol?t.href:t.pathname||("file"!==t.protocol||"/"===t.href[0]?"/":"")+a.join("/"),2===(r=t.pathname.split("#")).length&&(t.pathname=r[0],t.hash=r[1]),2===(r=t.pathname.split("?")).length&&(t.pathname=r[0],t.search=r[1]),t.query=ef.parse(t.search),t.href=t.href.replace(/\/$/,""),t.pathname=t.pathname.replace(/\/$/,""),t}(e)}(e);t.token="";let n=t.user.split(":");return 2===n.length&&("x-oauth-basic"===n[1]?t.token=n[0]:"x-token-auth"===n[0]&&(t.token=n[1])),t.protocol=Yu(t.protocols)||Yu(e)?"ssh":t.protocols.length?t.protocols[0]:"file",t.href=t.href.replace(/\/$/,""),t}(e),n=t.resource.split("."),r=null;switch(t.toString=function(e){return tf.stringify(this,e)},t.source=n.length>2?n.slice(1-n.length).join("."):t.source=t.resource,t.git_suffix=/\.git$/.test(t.pathname),t.name=decodeURIComponent(t.pathname.replace(/^\//,"").replace(/\.git$/,"")),t.owner=decodeURIComponent(t.user),t.source){case"git.cloudforge.com":t.owner=t.user,t.organization=n[0],t.source="cloudforge.com";break;case"visualstudio.com":if("vs-ssh.visualstudio.com"===t.resource){r=t.name.split("/"),4===r.length&&(t.organization=r[1],t.owner=r[2],t.name=r[3],t.full_name=r[2]+"/"+r[3]);break}r=t.name.split("/"),2===r.length?(t.owner=r[1],t.name=r[1],t.full_name="_git/"+t.name):3===r.length?(t.name=r[2],"DefaultCollection"===r[0]?(t.owner=r[2],t.organization=r[0],t.full_name=t.organization+"/_git/"+t.name):(t.owner=r[0],t.full_name=t.owner+"/_git/"+t.name)):4===r.length&&(t.organization=r[0],t.owner=r[1],t.name=r[3],t.full_name=t.organization+"/"+t.owner+"/_git/"+t.name);break;case"dev.azure.com":case"azure.com":if("ssh.dev.azure.com"===t.resource){r=t.name.split("/"),4===r.length&&(t.organization=r[1],t.owner=r[2],t.name=r[3]);break}r=t.name.split("/"),5===r.length?(t.organization=r[0],t.owner=r[1],t.name=r[4],t.full_name="_git/"+t.name):3===r.length?(t.name=r[2],"DefaultCollection"===r[0]?(t.owner=r[2],t.organization=r[0],t.full_name=t.organization+"/_git/"+t.name):(t.owner=r[0],t.full_name=t.owner+"/_git/"+t.name)):4===r.length&&(t.organization=r[0],t.owner=r[1],t.name=r[3],t.full_name=t.organization+"/"+t.owner+"/_git/"+t.name),t.query&&t.query.path&&(t.filepath=t.query.path.replace(/^\/+/g,"")),t.query&&t.query.version&&(t.ref=t.query.version.replace(/^GB/,""));break;default:r=t.name.split("/");let e=r.length-1;if(r.length>=2){const n=r.indexOf("-",2),a=r.indexOf("blob",2),o=r.indexOf("tree",2),i=r.indexOf("commit",2),l=r.indexOf("src",2),s=r.indexOf("raw",2);e=n>0?n-1:a>0?a-1:o>0?o-1:i>0?i-1:l>0?l-1:s>0?s-1:e,t.owner=r.slice(0,e).join("/"),t.name=r[e],i&&(t.commit=r[e+2])}t.ref="",t.filepathtype="",t.filepath="";const a=r.length>e&&"-"===r[e+1]?e+1:e;r.length>a+2&&["raw","src","blob","tree"].indexOf(r[a+1])>=0&&(t.filepathtype=r[a+1],t.ref=r[a+2],r.length>a+3&&(t.filepath=r.slice(a+3).join("/"))),t.organization=t.owner}t.full_name||(t.full_name=t.owner,t.name&&(t.full_name&&(t.full_name+="/"),t.full_name+=t.name)),t.owner.startsWith("scm/")&&(t.source="bitbucket-server",t.owner=t.owner.replace("scm/",""),t.organization=t.owner,t.full_name=`${t.owner}/${t.name}`);const a=/(projects|users)\/(.*?)\/repos\/(.*?)((\/.*$)|$)/.exec(t.pathname);return null!=a&&(t.source="bitbucket-server",t.owner="users"===a[1]?"~"+a[2]:a[2],t.organization=t.owner,t.name=a[3],r=a[4].split("/"),r.length>1&&(["raw","browse"].indexOf(r[1])>=0?(t.filepathtype=r[1],r.length>2&&(t.filepath=r[2])):"commits"===r[1]&&r.length>2&&(t.commit=r[2])),t.full_name=`${t.owner}/${t.name}`,t.ref=t.query.at?t.query.at:""),t}function nf({git:e}){const{commitUrl:t}=function(e,t){if(!e)return{resource:null,repoUrl:null,commitUrl:null};const n=tf(e),r=tf.stringify(Ne({},n,{git_suffix:!1}),"https");return{repoUrl:r,resource:n.resource,commitUrl:`${r}/commit/${t}`}}(e.remote,e.hash);return c.createElement(c.Fragment,null,e.hash&&e.message&&c.createElement("div",{className:"flex items-center gap-4"},c.createElement("div",{className:"flex-grow font-semibold"},e.message),c.createElement("div",{className:"~bg-gray-500/5 flex items-center"},c.createElement(xc,{transparent:!0,overflowX:!1,value:e.hash}),t&&c.createElement("a",{href:t,target:"_blank",className:"mr-4"},c.createElement(xt,null,c.createElement(qa,{className:"group-hover:text-indigo-500",icon:Co}),"View commit ",e.hash.substr(0,7))))),e.isDirty&&c.createElement("div",null,c.createElement(Wu,{className:"mt-4"},"Last commit is dirty. (Un)staged changes have been made since this commit.")),e.tag&&c.createElement(Ac,null,c.createElement(Ac.Row,{label:"Latest tag",value:e.tag})))}tf.stringify=function(e,t){t=t||(e.protocols&&e.protocols.length?e.protocols.join("+"):e.protocol);const n=e.port?`:${e.port}`:"",r=e.user||"git",a=e.git_suffix?".git":"";switch(t){case"ssh":return n?`ssh://${r}@${e.resource}${n}/${e.full_name}${a}`:`${r}@${e.resource}:${e.full_name}${a}`;case"git+ssh":case"ssh+git":case"ftp":case"ftps":return`${t}://${r}@${e.resource}${n}/${e.full_name}${a}`;case"http":case"https":return`${t}://${e.token?function(e){switch(e.source){case"bitbucket.org":return`x-token-auth:${e.token}@`;default:return`${e.token}@`}}(e):e.user&&(e.protocols.includes("http")||e.protocols.includes("https"))?`${e.user}@`:""}${e.resource}${n}/${function(e){switch(e.source){case"bitbucket-server":return`scm/${e.full_name}`;default:return`${e.full_name}`}}(e)}${a}`;default:return e.href}};var rf,af,of=(rf={"À":"A","Á":"A","Â":"A","Ã":"A","Ä":"A","Å":"A","à":"a","á":"a","â":"a","ã":"a","ä":"a","å":"a","Ç":"C","ç":"c","Ð":"D","ð":"d","È":"E","É":"E","Ê":"E","Ë":"E","è":"e","é":"e","ê":"e","ë":"e","Ì":"I","Í":"I","Î":"I","Ï":"I","ì":"i","í":"i","î":"i","ï":"i","Ñ":"N","ñ":"n","Ò":"O","Ó":"O","Ô":"O","Õ":"O","Ö":"O","Ø":"O","ò":"o","ó":"o","ô":"o","õ":"o","ö":"o","ø":"o","Ù":"U","Ú":"U","Û":"U","Ü":"U","ù":"u","ú":"u","û":"u","ü":"u","Ý":"Y","ý":"y","ÿ":"y","Æ":"Ae","æ":"ae","Þ":"Th","þ":"th","ß":"ss","Ā":"A","Ă":"A","Ą":"A","ā":"a","ă":"a","ą":"a","Ć":"C","Ĉ":"C","Ċ":"C","Č":"C","ć":"c","ĉ":"c","ċ":"c","č":"c","Ď":"D","Đ":"D","ď":"d","đ":"d","Ē":"E","Ĕ":"E","Ė":"E","Ę":"E","Ě":"E","ē":"e","ĕ":"e","ė":"e","ę":"e","ě":"e","Ĝ":"G","Ğ":"G","Ġ":"G","Ģ":"G","ĝ":"g","ğ":"g","ġ":"g","ģ":"g","Ĥ":"H","Ħ":"H","ĥ":"h","ħ":"h","Ĩ":"I","Ī":"I","Ĭ":"I","Į":"I","İ":"I","ĩ":"i","ī":"i","ĭ":"i","į":"i","ı":"i","Ĵ":"J","ĵ":"j","Ķ":"K","ķ":"k","ĸ":"k","Ĺ":"L","Ļ":"L","Ľ":"L","Ŀ":"L","Ł":"L","ĺ":"l","ļ":"l","ľ":"l","ŀ":"l","ł":"l","Ń":"N","Ņ":"N","Ň":"N","Ŋ":"N","ń":"n","ņ":"n","ň":"n","ŋ":"n","Ō":"O","Ŏ":"O","Ő":"O","ō":"o","ŏ":"o","ő":"o","Ŕ":"R","Ŗ":"R","Ř":"R","ŕ":"r","ŗ":"r","ř":"r","Ś":"S","Ŝ":"S","Ş":"S","Š":"S","ś":"s","ŝ":"s","ş":"s","š":"s","Ţ":"T","Ť":"T","Ŧ":"T","ţ":"t","ť":"t","ŧ":"t","Ũ":"U","Ū":"U","Ŭ":"U","Ů":"U","Ű":"U","Ų":"U","ũ":"u","ū":"u","ŭ":"u","ů":"u","ű":"u","ų":"u","Ŵ":"W","ŵ":"w","Ŷ":"Y","ŷ":"y","Ÿ":"Y","Ź":"Z","Ż":"Z","Ž":"Z","ź":"z","ż":"z","ž":"z","IJ":"IJ","ij":"ij","Œ":"Oe","œ":"oe","ʼn":"'n","ſ":"s"},function(e){return null==rf?void 0:rf[e]}),lf=/[\xc0-\xd6\xd8-\xf6\xf8-\xff\u0100-\u017f]/g,sf=RegExp("[\\u0300-\\u036f\\ufe20-\\ufe2f\\u20d0-\\u20ff]","g"),cf=/[^\x00-\x2f\x3a-\x40\x5b-\x60\x7b-\x7f]+/g,uf=/[a-z][A-Z]|[A-Z]{2}[a-z]|[0-9][a-zA-Z]|[a-zA-Z][0-9]|[^a-zA-Z0-9 ]/,ff="\\xac\\xb1\\xd7\\xf7\\x00-\\x2f\\x3a-\\x40\\x5b-\\x60\\x7b-\\xbf\\u2000-\\u206f \\t\\x0b\\f\\xa0\\ufeff\\n\\r\\u2028\\u2029\\u1680\\u180e\\u2000\\u2001\\u2002\\u2003\\u2004\\u2005\\u2006\\u2007\\u2008\\u2009\\u200a\\u202f\\u205f\\u3000",df="["+ff+"]",pf="\\d+",mf="[a-z\\xdf-\\xf6\\xf8-\\xff]",hf="[^\\ud800-\\udfff"+ff+pf+"\\u2700-\\u27bfa-z\\xdf-\\xf6\\xf8-\\xffA-Z\\xc0-\\xd6\\xd8-\\xde]",gf="(?:\\ud83c[\\udde6-\\uddff]){2}",yf="[\\ud800-\\udbff][\\udc00-\\udfff]",vf="[A-Z\\xc0-\\xd6\\xd8-\\xde]",bf="(?:"+mf+"|"+hf+")",Ef="(?:"+vf+"|"+hf+")",Tf="(?:[\\u0300-\\u036f\\ufe20-\\ufe2f\\u20d0-\\u20ff]|\\ud83c[\\udffb-\\udfff])?",Sf="[\\ufe0e\\ufe0f]?"+Tf+"(?:\\u200d(?:"+["[^\\ud800-\\udfff]",gf,yf].join("|")+")[\\ufe0e\\ufe0f]?"+Tf+")*",wf="(?:"+["[\\u2700-\\u27bf]",gf,yf].join("|")+")"+Sf,Nf=RegExp([vf+"?"+mf+"+(?:['’](?:d|ll|m|re|s|t|ve))?(?="+[df,vf,"$"].join("|")+")",Ef+"+(?:['’](?:D|LL|M|RE|S|T|VE))?(?="+[df,vf+bf,"$"].join("|")+")",vf+"?"+bf+"+(?:['’](?:d|ll|m|re|s|t|ve))?",vf+"+(?:['’](?:D|LL|M|RE|S|T|VE))?","\\d*(?:1ST|2ND|3RD|(?![123])\\dTH)(?=\\b|[a-z_])","\\d*(?:1st|2nd|3rd|(?![123])\\dth)(?=\\b|[A-Z_])",pf,wf].join("|"),"g"),Rf=RegExp("['’]","g"),Of=RegExp("[\\u200d\\ud800-\\udfff\\u0300-\\u036f\\ufe20-\\ufe2f\\u20d0-\\u20ff\\ufe0e\\ufe0f]"),Cf=function(e){return Of.test(e)},xf="[\\u0300-\\u036f\\ufe20-\\ufe2f\\u20d0-\\u20ff]",kf="\\ud83c[\\udffb-\\udfff]",Af="[^\\ud800-\\udfff]",If="(?:\\ud83c[\\udde6-\\uddff]){2}",Lf="[\\ud800-\\udbff][\\udc00-\\udfff]",_f="(?:"+xf+"|"+kf+")?",Pf="[\\ufe0e\\ufe0f]?"+_f+"(?:\\u200d(?:"+[Af,If,Lf].join("|")+")[\\ufe0e\\ufe0f]?"+_f+")*",Mf="(?:"+[Af+xf+"?",xf,If,Lf,"[\\ud800-\\udfff]"].join("|")+")",Df=RegExp(kf+"(?="+kf+")|"+Mf+Pf,"g"),Uf=(af=function(e,t,n){return e+(n?" ":"")+function(e){e=Js(e);var t,n,r,a=Cf(e)?function(e){return Cf(e)?function(e){return e.match(Df)||[]}(e):function(e){return e.split("")}(e)}(e):void 0,o=a?a[0]:e.charAt(0),i=a?(t=a,r=t.length,n=void 0===n?r:n,function(e,t,n){var r=-1,a=e.length;t<0&&(t=-t>a?0:a+t),(n=n>a?a:n)<0&&(n+=a),a=t>n?0:n-t>>>0,t>>>=0;for(var o=Array(a);++r<a;)o[r]=e[r+t];return o}(t,1,n)).join(""):e.slice(1);return o.toUpperCase()+i}(t)},function(e){return function(e,t,n,r){for(var a=-1,o=null==e?0:e.length;++a<o;)n=t(n,e[a],a,e);return n}(function(e,t,n){return e=Js(e),void 0===(t=t)?function(e){return uf.test(e)}(e)?function(e){return e.match(Nf)||[]}(e):function(e){return e.match(cf)||[]}(e):e.match(t)||[]}(function(e){return(e=Js(e))&&e.replace(lf,of).replace(sf,"")}(e).replace(Rf,"")),af,"")});function jf({env:e}){const t=c.useContext(be);return c.createElement(Ac,null,t.application_version&&c.createElement(Ac.Row,{key:"app_version",value:t.application_version,label:"App Version"}),Object.entries(e).map(([e,t])=>c.createElement(Ac.Row,{key:e,value:t,label:Uf(e)})))}function Ff({children:e}){return c.createElement("ul",{className:"grid grid-cols-1 gap-10"},e)}function zf({title:e,children:t,anchor:n}){return c.createElement("li",null,c.createElement("a",{href:`#context-${n}`,className:"uppercase tracking-wider ~text-gray-500 text-xs font-bold"},e),c.createElement("ul",{className:"mt-3 grid grid-cols-1 gap-3"},t))}function Bf({icon:e,title:t,anchor:n,active:r=!1}){return c.createElement("li",null,c.createElement("a",{href:`#context-${n}`,className:`\n flex items-center gap-3\n group text-base hover:text-indigo-500\n ${r?"~text-indigo-600":""}\n `},c.createElement("span",{className:"opacity-50"},e),c.createElement("span",null,t)))}function Hf({children:e}){const{inView:t}=c.useContext(ou);return c.createElement(c.Fragment,null,c.createElement("nav",{className:"hidden @2xl:block min-w-[8rem] flex-none mr-10 @4xl:mr-20"},c.createElement("div",{className:"sticky top-[7.5rem]"},c.createElement(Ff,null,c.Children.map(e,e=>c.createElement(c.Fragment,null,e&&c.createElement(zf,{title:e.props.title,anchor:e.props.anchor},c.Children.map(e.props.children,e=>c.createElement(c.Fragment,null,e&&e.type===iu&&c.createElement(Bf,{icon:e.props.icon,active:t[t.length-1]===e.props.title,title:e.props.title,anchor:e.props.anchor}))))))))),c.createElement("div",{className:"overflow-hidden grid grid-cols-1 gap-px bg-white dark:shadow-none dark:bg-gray-800/50 dark:bg-gradient-to-bl from-gray-700/50 via-transparent dark:ring-1 dark:ring-inset dark:ring-white/5 rounded-lg shadow-2xl shadow-gray-500/20 flex-grow"},e))}function Vf({children:e}){const[t,n]=c.useState([]);return c.createElement(ou.Provider,{value:{inView:t,setInView:n}},e)}function Wf({className:e=""}){return c.createElement("svg",{version:"1.1",xmlns:"http://www.w3.org/2000/svg",x:"0px",y:"0px",viewBox:"0 0 512 512",enableBackground:"new 0 0 512 512",className:`${e}`},c.createElement("path",{fill:"currentcolor",d:"M381.6,334.8c-24.7,0-27.7,33.6-45.2,44.6v52c0,17.6,14.2,31.8,31.8,31.8c17.6,0,31.8-14.2,31.8-31.8v-88.6\n C395,338.1,389.2,334.8,381.6,334.8z"}),c.createElement("path",{fill:"currentcolor",d:"M263.2,334.8c-25.5,0-27.8,35.8-46.9,45.7v96.2c0,19.5,15.8,35.3,35.3,35.3s35.3-15.8,35.3-35.3V349.1\n C280.9,341.1,273.9,334.8,263.2,334.8z"}),c.createElement("path",{fill:"currentcolor",d:"M144.8,334.8c-22.9,0-27.1,28.9-41.6,41.9l0,38c0,17.6,14.2,31.8,31.8,31.8c17.6,0,31.8-14.2,31.8-31.8v-67.9\n C161.2,339.9,154.5,334.8,144.8,334.8z"}),c.createElement("path",{id:"Body-Copy-4",fill:"currentcolor",fillRule:"evenodd",clipRule:"evenodd",d:"M458.9,340.2c-8.3,12.6-14.7,28.2-31.7,28.2\n\t\tc-28.6,0-30.1-44-58.7-44c-28.6,0-27,44-55.6,44c-28.6,0-30.1-44-58.7-44s-27,44-55.6,44s-30.1-44-58.7-44s-27,44-55.6,44\n\t\tc-9,0-15.3-4.4-20.6-10.3c-20.4-35.6-32.2-77.2-32.2-121.8C31.6,105.8,132.4,0,256.7,0s225.1,105.8,225.1,236.2\n\t\tC481.8,273.5,473.6,308.8,458.9,340.2z"}),c.createElement("path",{id:"Oval",fillRule:"evenodd",clipRule:"evenodd",fill:"#FFFFFF",d:"M244.6,295.1c78.3,0,111.2-45.4,111.2-109.9\n\t\tS306.1,61.4,244.6,61.4s-111.2,59.4-111.2,123.9S166.4,295.1,244.6,295.1z"}),c.createElement("ellipse",{id:"Oval_1_",fill:"currentcolor",fillRule:"evenodd",clipRule:"evenodd",cx:"214.7",cy:"142.9",rx:"41.7",ry:"46"}),c.createElement("ellipse",{id:"Oval_2_",fillRule:"evenodd",clipRule:"evenodd",fill:"#FFFFFF",cx:"207.8",cy:"132.2",rx:"20.9",ry:"21.3"}))}function Gf({items:e}){return c.createElement(Ac,null,Object.entries(e).map(([e,t])=>c.createElement(Ac.Row,{key:e,value:t,label:Uf(e)})))}function Yf({commandArguments:e}){return c.createElement("div",{className:"col-span-2"},c.createElement(xc,{value:e.join(" ")}))}function $f({request:e}){return e.useragent?c.createElement(xc,{value:e.useragent}):null}function Xf(){var e,t,n,r,a;const o=c.useContext(be),i=o.context_items,l=i.request_data;return c.createElement($c,null,c.createElement("div",{className:"@container flex items-stretch"},c.createElement(Vf,null,c.createElement(Hf,null,i.request&&c.createElement(tu,{title:"Request",anchor:"request"},c.createElement(su,{request:i.request,requestData:i.request_data,headers:i.headers}),!!i.request.useragent&&c.createElement(iu,{title:"Browser",anchor:"request-browser",icon:c.createElement(qa,{fixedWidth:!0,icon:ko}),children:c.createElement($f,{request:i.request})}),i.headers&&c.createElement(iu,{title:"Headers",anchor:"request-headers",icon:c.createElement(qa,{fixedWidth:!0,icon:yo}),children:c.createElement(Su,{headers:i.headers})}),i.request_data&&!!Object.values(i.request_data.queryString||[]).length&&c.createElement(iu,{title:"Query String",anchor:"request-query-string",icon:c.createElement(qa,{fixedWidth:!0,icon:ro}),children:c.createElement(wu,{requestData:i.request_data})}),!(null==(e=i.request_data)||!e.body)&&c.createElement(iu,{title:"Body",anchor:"request-body",icon:c.createElement(qa,{fixedWidth:!0,icon:ao}),children:c.createElement(Nu,null)}),!(null==l||null==(t=l.files)||!t.length)&&c.createElement(iu,{title:"Files",anchor:"request-files",icon:c.createElement(qa,{fixedWidth:!0,icon:co}),children:c.createElement(Ru,null)}),!(null==(n=i.session)||!n.length)&&c.createElement(iu,{title:"Session",anchor:"request-session",icon:c.createElement(qa,{fixedWidth:!0,icon:uo}),children:c.createElement(Ou,{session:i.session})}),!(null==(r=i.cookies)||!r.length)&&c.createElement(iu,{title:"Cookies",anchor:"request-cookies",icon:c.createElement(qa,{fixedWidth:!0,icon:io}),children:c.createElement(Cu,{cookies:i.cookies})})),(i.route||i.view||i.arguments||i.job)&&c.createElement(tu,{title:"App",anchor:"app"},i.route&&c.createElement(iu,{title:"Routing",anchor:"app-routing",icon:c.createElement(qa,{fixedWidth:!0,icon:To}),children:c.createElement(_u,{route:i.route})}),i.view&&c.createElement(iu,{title:"Views",anchor:"app-views",icon:c.createElement(qa,{fixedWidth:!0,icon:ho}),children:c.createElement(Du,null)}),i.arguments&&c.createElement(iu,{title:"Command",anchor:"context-command",icon:c.createElement(qa,{fixedWidth:!0,icon:Ro}),children:c.createElement(Yf,{commandArguments:i.arguments})}),i.job&&c.createElement(iu,{title:"Job",anchor:"context-job",icon:c.createElement(qa,{fixedWidth:!0,icon:fo}),children:c.createElement(Gf,{items:i.job||{}})})),i.livewire&&c.createElement(tu,{title:"Livewire",anchor:"livewire"},c.createElement(iu,{title:"Component",anchor:"livewire-component",icon:c.createElement(Wf,{className:"svg-inline--fa fa-w-16 fa-fw"}),children:c.createElement(ku,null)}),c.createElement(iu,{title:"Updates",anchor:"livewire-updates",icon:c.createElement(qa,{fixedWidth:!0,icon:Eo}),children:c.createElement(Au,null)}),c.createElement(iu,{title:"Data",anchor:"livewire-data",icon:c.createElement(qa,{fixedWidth:!0,icon:No}),children:c.createElement(xu,null)})),!!(i.user||i.git||i.env||o.application_version||i.exception)&&c.createElement(tu,{title:"Context",anchor:"context"},i.user&&c.createElement(iu,{title:"User",anchor:"user-user",icon:c.createElement(qa,{fixedWidth:!0,icon:xo}),children:c.createElement(Vu,{user:i.user})}),i.git&&c.createElement(iu,{title:"Git",anchor:"context-git",icon:c.createElement(qa,{fixedWidth:!0,icon:oo}),children:c.createElement(nf,{git:i.git})}),!(!i.env&&!o.application_version)&&c.createElement(iu,{title:"Versions",anchor:"context-versions",icon:c.createElement(qa,{fixedWidth:!0,icon:So}),children:c.createElement(jf,{env:i.env||{}})}),i.exception&&c.createElement(iu,{title:"Exception",anchor:"context-exception",icon:c.createElement(qa,{fixedWidth:!0,icon:to}),children:c.createElement(Gf,{items:i.exception||{}})})),(null==(a=o.custom_context_items)?void 0:a.length)>0&&c.createElement(tu,{title:"Custom",anchor:"custom-context"},o.custom_context_items.map(e=>c.createElement(iu,{key:e.name,title:Uf(e.name),anchor:`custom-context-${Zc(e.name)}`,icon:c.createElement(qa,{fixedWidth:!0,icon:eo}),children:c.createElement(Gf,{items:e.items})})))))))}function qf({children:e,className:t}){const[n,r]=c.useState(0),a=e.filter(e=>!1!==e),o=c.Children.map(a,e=>({name:e.props.name,component:e.props.component,count:e.props.count,checked:e.props.checked,onChange:e.props.onChange})).filter(e=>e.count),i=o[n].component;return c.createElement("div",{className:`${t} | bg-gray-300/50 dark:bg-black/10 shadow-inner rounded-lg`},c.createElement("nav",{className:"z-10 flex justify-center items-center"},c.createElement("ul",{className:"-my-5 flex justify-start items-center rounded-full shadow-lg bg-indigo-500 text-white space-x-px"},o.map((e,t)=>c.createElement("li",{key:t,className:`\n ${t===n?"bg-indigo-600":"bg-indigo-500 text-indigo-100"}\n ${0===t?"rounded-l-full":""}\n ${t===o.length-1?"rounded-r-full":""}\n hover:text-white\n `},c.createElement("button",{onClick:()=>r(t),className:"group flex items-center px-3 sm:px-5 h-10 uppercase tracking-wider text-xs font-medium "},c.createElement("span",{className:"mr-1.5 inline-flex items-center justify-center px-1 min-w-[1rem] h-4 bg-gray-900/30 text-white rounded-full text-xs"},e.count),c.createElement("span",null,e.name)))))),c.createElement($c,{fallbackComponent:e=>c.createElement(eu,{githubLink:e,className:"pt-10"})},c.createElement("div",{className:"grid grid-cols-1 gap-10 py-10 px-6 @lg:px-10"},c.createElement(i,null))))}function Kf({children:e,context:t=null,level:n=null,meta:r=null,time:a}){const[o,i]=c.useState(!1),l={error:"red",warn:"orange",warning:"orange",info:"blue",debug:"green",trace:"gray",notice:"purple",critical:"red",alert:"red",emergency:"red"};return c.createElement("div",{className:"min-w-0 grid grid-cols-1 gap-2"},c.createElement("div",{className:"flex items-center gap-1"},c.createElement(lu,{color:n?l[n]:"gray",className:"font-mono"},a.toLocaleTimeString()),n&&c.createElement(lu,{color:l[n]},n),r&&Object.entries(r).map(([e,t])=>c.createElement(c.Fragment,{key:e},"runtime"===e&&c.createElement(lu,{className:"inline-flex items-center gap-2"},c.createElement(qa,{title:"Runtime",className:"opacity-50",icon:wo})," ",t),"connection"===e&&c.createElement(lu,{className:"inline-flex items-center gap-2"},c.createElement(qa,{title:"Connection",className:"opacity-50",icon:so})," ",t),"runtime"!==e&&"connection"!==e&&c.createElement(lu,null,e,": ",t))),t&&c.createElement(c.Fragment,null,c.createElement("div",{className:"ml-auto"},c.createElement(xt,{onClick:()=>i(!o)},c.createElement(qa,{icon:o?mo:ao,className:"text-[8px] ~text-gray-500 group-hover:text-indigo-500"}),o?"As list":"Raw")))),c.createElement("div",null,e),t&&c.createElement(c.Fragment,null,o?c.createElement(xc,{value:Do(t),language:"json"}):c.createElement("div",{className:"pl-4"},c.createElement(cu,{items:t}))))}function Jf(){const e=c.useContext(be),t=Object.values(e.context_items.logs);return c.createElement(c.Fragment,null,t.map((e,t)=>c.createElement(Kf,{key:t,context:e.context,level:e.level,time:Mo(e.microtime)},c.createElement(xc,{value:e.message}))))}function Qf(){const e=c.useContext(be),t=Object.values(e.context_items.dumps);return c.createElement(c.Fragment,null,t.map((e,t)=>c.createElement(Kf,{key:t,time:Mo(e.microtime)},c.createElement("div",{className:"mb-2"},c.createElement(Bo,{path:e.file,lineNumber:e.line_number,className:"text-sm"})),c.createElement(Mu,{value:e.html_dump}))))}function Zf({bindings:e,hidden:t=!1}){const[n,r]=c.useState(t);return c.createElement("div",null,c.createElement("button",{type:"button",className:"font-bold text-xs ~text-gray-500 uppercase tracking-wider flex flex-row items-center gap-2 mb-2",onClick:()=>r(!n)},c.createElement(qa,{icon:Qa,className:"transition-transform duration-300 transform "+(n?"":"rotate-90")}),e.length," query ",e.length>1?"parameters":"parameter"),!n&&c.createElement(Ac,{className:"ml-4"},e.map((e,t)=>c.createElement(Ac.Row,{small:!0,key:t,value:e,label:c.createElement("code",{className:"text-sm text-gray-500"},t+1)}))))}function ed(){const e=c.useContext(be);let t=Object.values(e.context_items.queries);function n(e){return null!==e.bindings&&e.sql.split("?").length-1===e.bindings.length}function r(e){var t;let n=e.sql;return null==(t=e.bindings)||t.forEach(e=>{n=n.replace("?",e)}),n}return c.createElement(c.Fragment,null,t.map((e,t)=>c.createElement(Kf,{key:t,time:Mo(e.microtime),meta:{runtime:`${e.time}ms`,connection:e.connection_name}},e.bindings&&e.bindings.length>0?c.createElement("div",{className:"grid gap-4 grid-cols-1"},c.createElement(xc,{value:n(e)?r(e):e.sql,language:"sql"}),c.createElement(Zf,{bindings:e.bindings,hidden:n(e)})):c.createElement(xc,{value:e.sql,language:"sql"}))))}function td(){const e=c.useContext(be);return c.createElement(c.Fragment,null,e.glows.map((e,t)=>c.createElement(Kf,{key:t,level:e.message_level,context:e.meta_data,time:Mo(e.microtime)},c.createElement(xc,{value:e.name}))))}function nd(){const e=c.useContext(be),t=e.context_items.queries,n=e.context_items.logs,r=e.glows;return c.createElement($c,null,c.createElement(qf,{className:"@container"},c.createElement(qf.Tab,{component:Qf,name:"Dumps",count:Object.keys(e.context_items.dumps||[]).length}),c.createElement(qf.Tab,{component:td,name:"Glows",count:r.length}),c.createElement(qf.Tab,{component:ed,name:"Queries",count:Object.keys(t||[]).length}),c.createElement(qf.Tab,{component:Jf,name:"Logs",count:Object.keys(n||[]).length})))}function rd({children:e,className:t=""}){return c.createElement("code",{className:`font-mono leading-relaxed font-normal ~bg-gray-500/5 px-1 py-1 ${t}`},e)}function ad({className:e=""}){return c.createElement("svg",{height:"58",viewBox:"0 0 38 58",width:"38",xmlns:"http://www.w3.org/2000/svg",className:`w-4 h-5 ml-1.5 ${e}`},c.createElement("linearGradient",{id:"a",x1:"50%",x2:"50%",y1:"100%",y2:"0%"},c.createElement("stop",{offset:"0",stopColor:"#48b987"}),c.createElement("stop",{offset:"1",stopColor:"#137449"})),c.createElement("linearGradient",{id:"b",x1:"50%",x2:"50%",y1:"0%",y2:"100%"},c.createElement("stop",{offset:"0",stopColor:"#66ffbc"}),c.createElement("stop",{offset:"1",stopColor:"#218e5e"})),c.createElement("linearGradient",{id:"c",x1:"81.686741%",x2:"17.119683%",y1:"50%",y2:"46.893103%"},c.createElement("stop",{offset:"0",stopColor:"#ccffe7",stopOpacity:".492379"}),c.createElement("stop",{offset:".37576486",stopColor:"#fff",stopOpacity:".30736"}),c.createElement("stop",{offset:"1",stopColor:"#00ff85",stopOpacity:"0"})),c.createElement("linearGradient",{id:"d",x1:"50%",x2:"50%",y1:"100%",y2:"0%"},c.createElement("stop",{offset:"0",stopColor:"#a189f2"}),c.createElement("stop",{offset:"1",stopColor:"#3f00f5"})),c.createElement("linearGradient",{id:"e",x1:"50%",x2:"50%",y1:"0%",y2:"100%"},c.createElement("stop",{offset:"0",stopColor:"#bbadfa"}),c.createElement("stop",{offset:"1",stopColor:"#9275f4"})),c.createElement("g",{fill:"none"},c.createElement("g",{transform:"translate(1 1)"},c.createElement("path",{d:"m11.9943899 27.9858314-11.9943899-6.9992916v-13.98724823l12.0617111 7.02196133z",fill:"url(#a)"}),c.createElement("path",{d:"m23.9775596 20.9808724-23.9775596-13.98158083 11.9943899-6.99929157 24.0056101 13.9815808z",fill:"url(#b)",stroke:"url(#c)"})),c.createElement("g",{transform:"translate(1 29.014169)"},c.createElement("path",{d:"m11.9943899 27.9858314-11.9943899-6.9936241v-13.99291573l11.9663394 6.99362413z",fill:"url(#d)"}),c.createElement("path",{d:"m11.9663394 13.9929157-11.9663394-6.99362413 11.9943899-6.99929157 11.9943899 6.99929157z",fill:"url(#e)"}))))}function od(){return c.createElement("svg",{id:"ignition",className:"w-8 h-8 -ml-1",viewBox:"0 0 500 500"},c.createElement("g",null,c.createElement("polygon",{style:{fill:"transparent"},points:"466.5,375 466.5,125 250,0 33.5,125 33.5,375 250,500 \t"}),c.createElement("g",null,c.createElement("polygon",{style:{fill:"#ff4590"},points:"314.2,176 314.2,250 250,287 250,212.6 \t\t"}),c.createElement("polygon",{style:{fill:"#ffd000"},points:"185.9,398.1 185.9,324.1 250,287 249.9,360.9 \t\t"}),c.createElement("polygon",{style:{fill:"#de075d"},points:"250,139.1 250,287 185.9,250 185.8,101.9 \t\t"}),c.createElement("polygon",{style:{fill:"#e0b800"},points:"249.9,360.9 250,287 314.1,324 314.1,398.1 \t\t"}))))}function id(e){var t=e.label,n=e.onChange;return c.createElement("label",{className:"flex items-center"},c.createElement("input",{type:"checkbox",checked:e.checked,onChange:function(e){return n(e.target.checked)},className:"sr-only peer"}),c.createElement("span",{className:"mr-2 flex items-center w-6 h-4 ~bg-gray-100 peer-checked:bg-emerald-300 rounded-full shadow-inner transition-colors"}),c.createElement("span",{className:"absolute left-0.5 top-0.5 w-3 h-3 ~bg-dropdown rounded-full shadow-md transform peer-checked:translate-x-2 transition-transform"}),c.createElement("span",{className:"uppercase tracking-wider text-xs font-medium"},t))}qf.Tab=e=>null;var ld=c.createContext();function sd(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function cd(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?sd(Object(n),!0).forEach(function(t){fd(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):sd(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}function ud(e){return(ud="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function fd(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function dd(e,t){return function(e){if(Array.isArray(e))return e}(e)||function(e,t){var n=null==e?null:"undefined"!=typeof Symbol&&e[Symbol.iterator]||e["@@iterator"];if(null!=n){var r,a,o=[],i=!0,l=!1;try{for(n=n.call(e);!(i=(r=n.next()).done)&&(o.push(r.value),!t||o.length!==t);i=!0);}catch(e){l=!0,a=e}finally{try{i||null==n.return||n.return()}finally{if(l)throw a}}return o}}(e,t)||md(e,t)||function(){throw new TypeError("Invalid attempt to destructure non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function pd(e){return function(e){if(Array.isArray(e))return hd(e)}(e)||function(e){if("undefined"!=typeof Symbol&&null!=e[Symbol.iterator]||null!=e["@@iterator"])return Array.from(e)}(e)||md(e)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function md(e,t){if(e){if("string"==typeof e)return hd(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?hd(e,t):void 0}}function hd(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}var gd=function(){},yd={},vd={},bd=null,Ed={mark:gd,measure:gd};try{"undefined"!=typeof window&&(yd=window),"undefined"!=typeof document&&(vd=document),"undefined"!=typeof MutationObserver&&(bd=MutationObserver),"undefined"!=typeof performance&&(Ed=performance)}catch(pa){}var Td=(yd.navigator||{}).userAgent,Sd=void 0===Td?"":Td,wd=yd,Nd=vd,Rd=bd,Od=Ed,Cd=!!Nd.documentElement&&!!Nd.head&&"function"==typeof Nd.addEventListener&&"function"==typeof Nd.createElement,xd=~Sd.indexOf("MSIE")||~Sd.indexOf("Trident/"),kd=["HTML","HEAD","STYLE","SCRIPT"],Ad=function(){try{return!1}catch(e){return!1}}(),Id={fas:"solid","fa-solid":"solid",far:"regular","fa-regular":"regular",fal:"light","fa-light":"light",fat:"thin","fa-thin":"thin",fad:"duotone","fa-duotone":"duotone",fab:"brands","fa-brands":"brands",fak:"kit","fa-kit":"kit",fa:"solid"},Ld={solid:"fas",regular:"far",light:"fal",thin:"fat",duotone:"fad",brands:"fab",kit:"fak"},_d={fab:"fa-brands",fad:"fa-duotone",fak:"fa-kit",fal:"fa-light",far:"fa-regular",fas:"fa-solid",fat:"fa-thin"},Pd={"fa-brands":"fab","fa-duotone":"fad","fa-kit":"fak","fa-light":"fal","fa-regular":"far","fa-solid":"fas","fa-thin":"fat"},Md=/fa[srltdbk\-\ ]/,Dd=/Font ?Awesome ?([56 ]*)(Solid|Regular|Light|Thin|Duotone|Brands|Free|Pro|Kit)?.*/i,Ud={900:"fas",400:"far",normal:"far",300:"fal",100:"fat"},jd=[1,2,3,4,5,6,7,8,9,10],Fd=jd.concat([11,12,13,14,15,16,17,18,19,20]),zd=["class","data-prefix","data-icon","data-fa-transform","data-fa-mask"],Bd=[].concat(pd(Object.keys(Ld)),["2xs","xs","sm","lg","xl","2xl","beat","border","fade","beat-fade","bounce","flip-both","flip-horizontal","flip-vertical","flip","fw","inverse","layers-counter","layers-text","layers","li","pull-left","pull-right","pulse","rotate-180","rotate-270","rotate-90","rotate-by","shake","spin-pulse","spin-reverse","spin","stack-1x","stack-2x","stack","ul","duotone-group","swap-opacity","primary","secondary"]).concat(jd.map(function(e){return"".concat(e,"x")})).concat(Fd.map(function(e){return"w-".concat(e)})),Hd=wd.FontAwesomeConfig||{};Nd&&"function"==typeof Nd.querySelector&&[["data-family-prefix","familyPrefix"],["data-style-default","styleDefault"],["data-replacement-class","replacementClass"],["data-auto-replace-svg","autoReplaceSvg"],["data-auto-add-css","autoAddCss"],["data-auto-a11y","autoA11y"],["data-search-pseudo-elements","searchPseudoElements"],["data-observe-mutations","observeMutations"],["data-mutate-approach","mutateApproach"],["data-keep-original-source","keepOriginalSource"],["data-measure-performance","measurePerformance"],["data-show-missing-icons","showMissingIcons"]].forEach(function(e){var t=dd(e,2),n=t[1],r=function(e){return""===e||"false"!==e&&("true"===e||e)}(function(e){var t=Nd.querySelector("script["+e+"]");if(t)return t.getAttribute(e)}(t[0]));null!=r&&(Hd[n]=r)});var Vd=cd(cd({},{familyPrefix:"fa",styleDefault:"solid",replacementClass:"svg-inline--fa",autoReplaceSvg:!0,autoAddCss:!0,autoA11y:!0,searchPseudoElements:!1,observeMutations:!0,mutateApproach:"async",keepOriginalSource:!0,measurePerformance:!1,showMissingIcons:!0}),Hd);Vd.autoReplaceSvg||(Vd.observeMutations=!1);var Wd={};Object.keys(Vd).forEach(function(e){Object.defineProperty(Wd,e,{enumerable:!0,set:function(t){Vd[e]=t,Gd.forEach(function(e){return e(Wd)})},get:function(){return Vd[e]}})}),wd.FontAwesomeConfig=Wd;var Gd=[],Yd=16,$d={size:16,x:0,y:0,rotate:0,flipX:!1,flipY:!1};function Xd(){for(var e=12,t="";e-- >0;)t+="0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"[62*Math.random()|0];return t}function qd(e){for(var t=[],n=(e||[]).length>>>0;n--;)t[n]=e[n];return t}function Kd(e){return e.classList?qd(e.classList):(e.getAttribute("class")||"").split(" ").filter(function(e){return e})}function Jd(e){return"".concat(e).replace(/&/g,"&").replace(/"/g,""").replace(/'/g,"'").replace(/</g,"<").replace(/>/g,">")}function Qd(e){return Object.keys(e||{}).reduce(function(t,n){return t+"".concat(n,": ").concat(e[n].trim(),";")},"")}function Zd(e){return e.size!==$d.size||e.x!==$d.x||e.y!==$d.y||e.rotate!==$d.rotate||e.flipX||e.flipY}function ep(){var e="fa",t="svg-inline--fa",n=Wd.familyPrefix,r=Wd.replacementClass,a=':root, :host {\n --fa-font-solid: normal 900 1em/1 "Font Awesome 6 Solid";\n --fa-font-regular: normal 400 1em/1 "Font Awesome 6 Regular";\n --fa-font-light: normal 300 1em/1 "Font Awesome 6 Light";\n --fa-font-thin: normal 100 1em/1 "Font Awesome 6 Thin";\n --fa-font-duotone: normal 900 1em/1 "Font Awesome 6 Duotone";\n --fa-font-brands: normal 400 1em/1 "Font Awesome 6 Brands";\n}\n\nsvg:not(:root).svg-inline--fa, svg:not(:host).svg-inline--fa {\n overflow: visible;\n box-sizing: content-box;\n}\n\n.svg-inline--fa {\n display: var(--fa-display, inline-block);\n height: 1em;\n overflow: visible;\n vertical-align: -0.125em;\n}\n.svg-inline--fa.fa-2xs {\n vertical-align: 0.1em;\n}\n.svg-inline--fa.fa-xs {\n vertical-align: 0em;\n}\n.svg-inline--fa.fa-sm {\n vertical-align: -0.0714285705em;\n}\n.svg-inline--fa.fa-lg {\n vertical-align: -0.2em;\n}\n.svg-inline--fa.fa-xl {\n vertical-align: -0.25em;\n}\n.svg-inline--fa.fa-2xl {\n vertical-align: -0.3125em;\n}\n.svg-inline--fa.fa-pull-left {\n margin-right: var(--fa-pull-margin, 0.3em);\n width: auto;\n}\n.svg-inline--fa.fa-pull-right {\n margin-left: var(--fa-pull-margin, 0.3em);\n width: auto;\n}\n.svg-inline--fa.fa-li {\n width: var(--fa-li-width, 2em);\n top: 0.25em;\n}\n.svg-inline--fa.fa-fw {\n width: var(--fa-fw-width, 1.25em);\n}\n\n.fa-layers svg.svg-inline--fa {\n bottom: 0;\n left: 0;\n margin: auto;\n position: absolute;\n right: 0;\n top: 0;\n}\n\n.fa-layers-counter, .fa-layers-text {\n display: inline-block;\n position: absolute;\n text-align: center;\n}\n\n.fa-layers {\n display: inline-block;\n height: 1em;\n position: relative;\n text-align: center;\n vertical-align: -0.125em;\n width: 1em;\n}\n.fa-layers svg.svg-inline--fa {\n -webkit-transform-origin: center center;\n transform-origin: center center;\n}\n\n.fa-layers-text {\n left: 50%;\n top: 50%;\n -webkit-transform: translate(-50%, -50%);\n transform: translate(-50%, -50%);\n -webkit-transform-origin: center center;\n transform-origin: center center;\n}\n\n.fa-layers-counter {\n background-color: var(--fa-counter-background-color, #ff253a);\n border-radius: var(--fa-counter-border-radius, 1em);\n box-sizing: border-box;\n color: var(--fa-inverse, #fff);\n line-height: var(--fa-counter-line-height, 1);\n max-width: var(--fa-counter-max-width, 5em);\n min-width: var(--fa-counter-min-width, 1.5em);\n overflow: hidden;\n padding: var(--fa-counter-padding, 0.25em 0.5em);\n right: var(--fa-right, 0);\n text-overflow: ellipsis;\n top: var(--fa-top, 0);\n -webkit-transform: scale(var(--fa-counter-scale, 0.25));\n transform: scale(var(--fa-counter-scale, 0.25));\n -webkit-transform-origin: top right;\n transform-origin: top right;\n}\n\n.fa-layers-bottom-right {\n bottom: var(--fa-bottom, 0);\n right: var(--fa-right, 0);\n top: auto;\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: bottom right;\n transform-origin: bottom right;\n}\n\n.fa-layers-bottom-left {\n bottom: var(--fa-bottom, 0);\n left: var(--fa-left, 0);\n right: auto;\n top: auto;\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: bottom left;\n transform-origin: bottom left;\n}\n\n.fa-layers-top-right {\n top: var(--fa-top, 0);\n right: var(--fa-right, 0);\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: top right;\n transform-origin: top right;\n}\n\n.fa-layers-top-left {\n left: var(--fa-left, 0);\n right: auto;\n top: var(--fa-top, 0);\n -webkit-transform: scale(var(--fa-layers-scale, 0.25));\n transform: scale(var(--fa-layers-scale, 0.25));\n -webkit-transform-origin: top left;\n transform-origin: top left;\n}\n\n.fa-1x {\n font-size: 1em;\n}\n\n.fa-2x {\n font-size: 2em;\n}\n\n.fa-3x {\n font-size: 3em;\n}\n\n.fa-4x {\n font-size: 4em;\n}\n\n.fa-5x {\n font-size: 5em;\n}\n\n.fa-6x {\n font-size: 6em;\n}\n\n.fa-7x {\n font-size: 7em;\n}\n\n.fa-8x {\n font-size: 8em;\n}\n\n.fa-9x {\n font-size: 9em;\n}\n\n.fa-10x {\n font-size: 10em;\n}\n\n.fa-2xs {\n font-size: 0.625em;\n line-height: 0.1em;\n vertical-align: 0.225em;\n}\n\n.fa-xs {\n font-size: 0.75em;\n line-height: 0.0833333337em;\n vertical-align: 0.125em;\n}\n\n.fa-sm {\n font-size: 0.875em;\n line-height: 0.0714285718em;\n vertical-align: 0.0535714295em;\n}\n\n.fa-lg {\n font-size: 1.25em;\n line-height: 0.05em;\n vertical-align: -0.075em;\n}\n\n.fa-xl {\n font-size: 1.5em;\n line-height: 0.0416666682em;\n vertical-align: -0.125em;\n}\n\n.fa-2xl {\n font-size: 2em;\n line-height: 0.03125em;\n vertical-align: -0.1875em;\n}\n\n.fa-fw {\n text-align: center;\n width: 1.25em;\n}\n\n.fa-ul {\n list-style-type: none;\n margin-left: var(--fa-li-margin, 2.5em);\n padding-left: 0;\n}\n.fa-ul > li {\n position: relative;\n}\n\n.fa-li {\n left: calc(var(--fa-li-width, 2em) * -1);\n position: absolute;\n text-align: center;\n width: var(--fa-li-width, 2em);\n line-height: inherit;\n}\n\n.fa-border {\n border-color: var(--fa-border-color, #eee);\n border-radius: var(--fa-border-radius, 0.1em);\n border-style: var(--fa-border-style, solid);\n border-width: var(--fa-border-width, 0.08em);\n padding: var(--fa-border-padding, 0.2em 0.25em 0.15em);\n}\n\n.fa-pull-left {\n float: left;\n margin-right: var(--fa-pull-margin, 0.3em);\n}\n\n.fa-pull-right {\n float: right;\n margin-left: var(--fa-pull-margin, 0.3em);\n}\n\n.fa-beat {\n -webkit-animation-name: fa-beat;\n animation-name: fa-beat;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, ease-in-out);\n animation-timing-function: var(--fa-animation-timing, ease-in-out);\n}\n\n.fa-bounce {\n -webkit-animation-name: fa-bounce;\n animation-name: fa-bounce;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.28, 0.84, 0.42, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.28, 0.84, 0.42, 1));\n}\n\n.fa-fade {\n -webkit-animation-name: fa-fade;\n animation-name: fa-fade;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n}\n\n.fa-beat-fade {\n -webkit-animation-name: fa-beat-fade;\n animation-name: fa-beat-fade;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n animation-timing-function: var(--fa-animation-timing, cubic-bezier(0.4, 0, 0.6, 1));\n}\n\n.fa-flip {\n -webkit-animation-name: fa-flip;\n animation-name: fa-flip;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, ease-in-out);\n animation-timing-function: var(--fa-animation-timing, ease-in-out);\n}\n\n.fa-shake {\n -webkit-animation-name: fa-shake;\n animation-name: fa-shake;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, linear);\n animation-timing-function: var(--fa-animation-timing, linear);\n}\n\n.fa-spin {\n -webkit-animation-name: fa-spin;\n animation-name: fa-spin;\n -webkit-animation-delay: var(--fa-animation-delay, 0);\n animation-delay: var(--fa-animation-delay, 0);\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 2s);\n animation-duration: var(--fa-animation-duration, 2s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, linear);\n animation-timing-function: var(--fa-animation-timing, linear);\n}\n\n.fa-spin-reverse {\n --fa-animation-direction: reverse;\n}\n\n.fa-pulse,\n.fa-spin-pulse {\n -webkit-animation-name: fa-spin;\n animation-name: fa-spin;\n -webkit-animation-direction: var(--fa-animation-direction, normal);\n animation-direction: var(--fa-animation-direction, normal);\n -webkit-animation-duration: var(--fa-animation-duration, 1s);\n animation-duration: var(--fa-animation-duration, 1s);\n -webkit-animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n animation-iteration-count: var(--fa-animation-iteration-count, infinite);\n -webkit-animation-timing-function: var(--fa-animation-timing, steps(8));\n animation-timing-function: var(--fa-animation-timing, steps(8));\n}\n\n@media (prefers-reduced-motion: reduce) {\n .fa-beat,\n.fa-bounce,\n.fa-fade,\n.fa-beat-fade,\n.fa-flip,\n.fa-pulse,\n.fa-shake,\n.fa-spin,\n.fa-spin-pulse {\n -webkit-animation-delay: -1ms;\n animation-delay: -1ms;\n -webkit-animation-duration: 1ms;\n animation-duration: 1ms;\n -webkit-animation-iteration-count: 1;\n animation-iteration-count: 1;\n transition-delay: 0s;\n transition-duration: 0s;\n }\n}\n@-webkit-keyframes fa-beat {\n 0%, 90% {\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 45% {\n -webkit-transform: scale(var(--fa-beat-scale, 1.25));\n transform: scale(var(--fa-beat-scale, 1.25));\n }\n}\n@keyframes fa-beat {\n 0%, 90% {\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 45% {\n -webkit-transform: scale(var(--fa-beat-scale, 1.25));\n transform: scale(var(--fa-beat-scale, 1.25));\n }\n}\n@-webkit-keyframes fa-bounce {\n 0% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 10% {\n -webkit-transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n }\n 30% {\n -webkit-transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n }\n 50% {\n -webkit-transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n }\n 57% {\n -webkit-transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n }\n 64% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 100% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n}\n@keyframes fa-bounce {\n 0% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 10% {\n -webkit-transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n transform: scale(var(--fa-bounce-start-scale-x, 1.1), var(--fa-bounce-start-scale-y, 0.9)) translateY(0);\n }\n 30% {\n -webkit-transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n transform: scale(var(--fa-bounce-jump-scale-x, 0.9), var(--fa-bounce-jump-scale-y, 1.1)) translateY(var(--fa-bounce-height, -0.5em));\n }\n 50% {\n -webkit-transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n transform: scale(var(--fa-bounce-land-scale-x, 1.05), var(--fa-bounce-land-scale-y, 0.95)) translateY(0);\n }\n 57% {\n -webkit-transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n transform: scale(1, 1) translateY(var(--fa-bounce-rebound, -0.125em));\n }\n 64% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n 100% {\n -webkit-transform: scale(1, 1) translateY(0);\n transform: scale(1, 1) translateY(0);\n }\n}\n@-webkit-keyframes fa-fade {\n 50% {\n opacity: var(--fa-fade-opacity, 0.4);\n }\n}\n@keyframes fa-fade {\n 50% {\n opacity: var(--fa-fade-opacity, 0.4);\n }\n}\n@-webkit-keyframes fa-beat-fade {\n 0%, 100% {\n opacity: var(--fa-beat-fade-opacity, 0.4);\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 50% {\n opacity: 1;\n -webkit-transform: scale(var(--fa-beat-fade-scale, 1.125));\n transform: scale(var(--fa-beat-fade-scale, 1.125));\n }\n}\n@keyframes fa-beat-fade {\n 0%, 100% {\n opacity: var(--fa-beat-fade-opacity, 0.4);\n -webkit-transform: scale(1);\n transform: scale(1);\n }\n 50% {\n opacity: 1;\n -webkit-transform: scale(var(--fa-beat-fade-scale, 1.125));\n transform: scale(var(--fa-beat-fade-scale, 1.125));\n }\n}\n@-webkit-keyframes fa-flip {\n 50% {\n -webkit-transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n }\n}\n@keyframes fa-flip {\n 50% {\n -webkit-transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n transform: rotate3d(var(--fa-flip-x, 0), var(--fa-flip-y, 1), var(--fa-flip-z, 0), var(--fa-flip-angle, -180deg));\n }\n}\n@-webkit-keyframes fa-shake {\n 0% {\n -webkit-transform: rotate(-15deg);\n transform: rotate(-15deg);\n }\n 4% {\n -webkit-transform: rotate(15deg);\n transform: rotate(15deg);\n }\n 8%, 24% {\n -webkit-transform: rotate(-18deg);\n transform: rotate(-18deg);\n }\n 12%, 28% {\n -webkit-transform: rotate(18deg);\n transform: rotate(18deg);\n }\n 16% {\n -webkit-transform: rotate(-22deg);\n transform: rotate(-22deg);\n }\n 20% {\n -webkit-transform: rotate(22deg);\n transform: rotate(22deg);\n }\n 32% {\n -webkit-transform: rotate(-12deg);\n transform: rotate(-12deg);\n }\n 36% {\n -webkit-transform: rotate(12deg);\n transform: rotate(12deg);\n }\n 40%, 100% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n}\n@keyframes fa-shake {\n 0% {\n -webkit-transform: rotate(-15deg);\n transform: rotate(-15deg);\n }\n 4% {\n -webkit-transform: rotate(15deg);\n transform: rotate(15deg);\n }\n 8%, 24% {\n -webkit-transform: rotate(-18deg);\n transform: rotate(-18deg);\n }\n 12%, 28% {\n -webkit-transform: rotate(18deg);\n transform: rotate(18deg);\n }\n 16% {\n -webkit-transform: rotate(-22deg);\n transform: rotate(-22deg);\n }\n 20% {\n -webkit-transform: rotate(22deg);\n transform: rotate(22deg);\n }\n 32% {\n -webkit-transform: rotate(-12deg);\n transform: rotate(-12deg);\n }\n 36% {\n -webkit-transform: rotate(12deg);\n transform: rotate(12deg);\n }\n 40%, 100% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n}\n@-webkit-keyframes fa-spin {\n 0% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n 100% {\n -webkit-transform: rotate(360deg);\n transform: rotate(360deg);\n }\n}\n@keyframes fa-spin {\n 0% {\n -webkit-transform: rotate(0deg);\n transform: rotate(0deg);\n }\n 100% {\n -webkit-transform: rotate(360deg);\n transform: rotate(360deg);\n }\n}\n.fa-rotate-90 {\n -webkit-transform: rotate(90deg);\n transform: rotate(90deg);\n}\n\n.fa-rotate-180 {\n -webkit-transform: rotate(180deg);\n transform: rotate(180deg);\n}\n\n.fa-rotate-270 {\n -webkit-transform: rotate(270deg);\n transform: rotate(270deg);\n}\n\n.fa-flip-horizontal {\n -webkit-transform: scale(-1, 1);\n transform: scale(-1, 1);\n}\n\n.fa-flip-vertical {\n -webkit-transform: scale(1, -1);\n transform: scale(1, -1);\n}\n\n.fa-flip-both,\n.fa-flip-horizontal.fa-flip-vertical {\n -webkit-transform: scale(-1, -1);\n transform: scale(-1, -1);\n}\n\n.fa-rotate-by {\n -webkit-transform: rotate(var(--fa-rotate-angle, none));\n transform: rotate(var(--fa-rotate-angle, none));\n}\n\n.fa-stack {\n display: inline-block;\n vertical-align: middle;\n height: 2em;\n position: relative;\n width: 2.5em;\n}\n\n.fa-stack-1x,\n.fa-stack-2x {\n bottom: 0;\n left: 0;\n margin: auto;\n position: absolute;\n right: 0;\n top: 0;\n z-index: var(--fa-stack-z-index, auto);\n}\n\n.svg-inline--fa.fa-stack-1x {\n height: 1em;\n width: 1.25em;\n}\n.svg-inline--fa.fa-stack-2x {\n height: 2em;\n width: 2.5em;\n}\n\n.fa-inverse {\n color: var(--fa-inverse, #fff);\n}\n\n.sr-only,\n.fa-sr-only {\n position: absolute;\n width: 1px;\n height: 1px;\n padding: 0;\n margin: -1px;\n overflow: hidden;\n clip: rect(0, 0, 0, 0);\n white-space: nowrap;\n border-width: 0;\n}\n\n.sr-only-focusable:not(:focus),\n.fa-sr-only-focusable:not(:focus) {\n position: absolute;\n width: 1px;\n height: 1px;\n padding: 0;\n margin: -1px;\n overflow: hidden;\n clip: rect(0, 0, 0, 0);\n white-space: nowrap;\n border-width: 0;\n}\n\n.svg-inline--fa .fa-primary {\n fill: var(--fa-primary-color, currentColor);\n opacity: var(--fa-primary-opacity, 1);\n}\n\n.svg-inline--fa .fa-secondary {\n fill: var(--fa-secondary-color, currentColor);\n opacity: var(--fa-secondary-opacity, 0.4);\n}\n\n.svg-inline--fa.fa-swap-opacity .fa-primary {\n opacity: var(--fa-secondary-opacity, 0.4);\n}\n\n.svg-inline--fa.fa-swap-opacity .fa-secondary {\n opacity: var(--fa-primary-opacity, 1);\n}\n\n.svg-inline--fa mask .fa-primary,\n.svg-inline--fa mask .fa-secondary {\n fill: black;\n}\n\n.fad.fa-inverse,\n.fa-duotone.fa-inverse {\n color: var(--fa-inverse, #fff);\n}';if(n!==e||r!==t){var o=new RegExp("\\.".concat(e,"\\-"),"g"),i=new RegExp("\\--".concat(e,"\\-"),"g"),l=new RegExp("\\.".concat(t),"g");a=a.replace(o,".".concat(n,"-")).replace(i,"--".concat(n,"-")).replace(l,".".concat(r))}return a}var tp=!1;function np(){Wd.autoAddCss&&!tp&&(function(e){if(e&&Cd){var t=Nd.createElement("style");t.setAttribute("type","text/css"),t.innerHTML=e;for(var n=Nd.head.childNodes,r=null,a=n.length-1;a>-1;a--){var o=n[a],i=(o.tagName||"").toUpperCase();["STYLE","LINK"].indexOf(i)>-1&&(r=o)}Nd.head.insertBefore(t,r)}}(ep()),tp=!0)}var rp={mixout:function(){return{dom:{css:ep,insertCss:np}}},hooks:function(){return{beforeDOMElementCreation:function(){np()},beforeI2svg:function(){np()}}}},ap=wd||{};ap.___FONT_AWESOME___||(ap.___FONT_AWESOME___={}),ap.___FONT_AWESOME___.styles||(ap.___FONT_AWESOME___.styles={}),ap.___FONT_AWESOME___.hooks||(ap.___FONT_AWESOME___.hooks={}),ap.___FONT_AWESOME___.shims||(ap.___FONT_AWESOME___.shims=[]);var op=ap.___FONT_AWESOME___,ip=[],lp=!1;function sp(e){Cd&&(lp?setTimeout(e,0):ip.push(e))}function cp(e){var t=e.tag,n=e.attributes,r=void 0===n?{}:n,a=e.children,o=void 0===a?[]:a;return"string"==typeof e?Jd(e):"<".concat(t," ").concat(function(e){return Object.keys(e||{}).reduce(function(t,n){return t+"".concat(n,'="').concat(Jd(e[n]),'" ')},"").trim()}(r),">").concat(o.map(cp).join(""),"</").concat(t,">")}function up(e,t,n){if(e&&e[t]&&e[t][n])return{prefix:t,iconName:n,icon:e[t][n]}}Cd&&((lp=(Nd.documentElement.doScroll?/^loaded|^c/:/^loaded|^i|^c/).test(Nd.readyState))||Nd.addEventListener("DOMContentLoaded",function e(){Nd.removeEventListener("DOMContentLoaded",e),lp=1,ip.map(function(e){return e()})}));var fp=function(e,t,n,r){var a,o,i,l=Object.keys(e),s=l.length,c=void 0!==r?function(e,t){return function(n,r,a,o){return e.call(t,n,r,a,o)}}(t,r):t;for(void 0===n?(a=1,i=e[l[0]]):(a=0,i=n);a<s;a++)i=c(i,e[o=l[a]],o,e);return i};function dp(e){var t=function(e){for(var t=[],n=0,r=e.length;n<r;){var a=e.charCodeAt(n++);if(a>=55296&&a<=56319&&n<r){var o=e.charCodeAt(n++);56320==(64512&o)?t.push(((1023&a)<<10)+(1023&o)+65536):(t.push(a),n--)}else t.push(a)}return t}(e);return 1===t.length?t[0].toString(16):null}function pp(e){return Object.keys(e).reduce(function(t,n){var r=e[n];return r.icon?t[r.iconName]=r.icon:t[n]=r,t},{})}function mp(e,t){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{},r=n.skipHooks,a=void 0!==r&&r,o=pp(t);"function"!=typeof op.hooks.addPack||a?op.styles[e]=cd(cd({},op.styles[e]||{}),o):op.hooks.addPack(e,pp(t)),"fas"===e&&mp("fa",t)}var hp=op.styles,gp=op.shims,yp=Object.values(_d),vp=null,bp={},Ep={},Tp={},Sp={},wp={},Np=Object.keys(Id);function Rp(e,t){var n=t.split("-"),r=n[0],a=n.slice(1).join("-");return r!==e||""===a||~Bd.indexOf(a)?null:a}var Op=function(){var e=function(e){return fp(hp,function(t,n,r){return t[r]=fp(n,e,{}),t},{})};bp=e(function(e,t,n){return t[3]&&(e[t[3]]=n),t[2]&&t[2].filter(function(e){return"number"==typeof e}).forEach(function(t){e[t.toString(16)]=n}),e}),Ep=e(function(e,t,n){return e[n]=n,t[2]&&t[2].filter(function(e){return"string"==typeof e}).forEach(function(t){e[t]=n}),e}),wp=e(function(e,t,n){var r=t[2];return e[n]=n,r.forEach(function(t){e[t]=n}),e});var t="far"in hp||Wd.autoFetchSvg,n=fp(gp,function(e,n){var r=n[0],a=n[1],o=n[2];return"far"!==a||t||(a="fas"),"string"==typeof r&&(e.names[r]={prefix:a,iconName:o}),"number"==typeof r&&(e.unicodes[r.toString(16)]={prefix:a,iconName:o}),e},{names:{},unicodes:{}});Tp=n.names,Sp=n.unicodes,vp=Ip(Wd.styleDefault)};function Cp(e,t){return(bp[e]||{})[t]}function xp(e,t){return(wp[e]||{})[t]}function kp(e){return Tp[e]||{prefix:null,iconName:null}}function Ap(){return vp}function Ip(e){return Ld[e]||Ld[Id[e]]||(e in op.styles?e:null)||null}function Lp(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.skipLookups,r=void 0!==n&&n,a=null,o=e.reduce(function(e,t){var n=Rp(Wd.familyPrefix,t);if(hp[t]?(t=yp.includes(t)?Pd[t]:t,a=t,e.prefix=t):Np.indexOf(t)>-1?(a=t,e.prefix=Ip(t)):n?e.iconName=n:t!==Wd.replacementClass&&e.rest.push(t),!r&&e.prefix&&e.iconName){var o="fa"===a?kp(e.iconName):{},i=xp(e.prefix,e.iconName);o.prefix&&(a=null),e.iconName=o.iconName||i||e.iconName,e.prefix=o.prefix||e.prefix,"far"!==e.prefix||hp.far||!hp.fas||Wd.autoFetchSvg||(e.prefix="fas")}return e},{prefix:null,iconName:null,rest:[]});return"fa"!==o.prefix&&"fa"!==a||(o.prefix=Ap()||"fas"),o}Gd.push(function(e){vp=Ip(e.styleDefault)}),Op();var _p=/*#__PURE__*/function(){function e(){!function(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}(this,e),this.definitions={}}var t,n;return t=e,(n=[{key:"add",value:function(){for(var e=this,t=arguments.length,n=new Array(t),r=0;r<t;r++)n[r]=arguments[r];var a=n.reduce(this._pullDefinitions,{});Object.keys(a).forEach(function(t){e.definitions[t]=cd(cd({},e.definitions[t]||{}),a[t]),mp(t,a[t]);var n=_d[t];n&&mp(n,a[t]),Op()})}},{key:"reset",value:function(){this.definitions={}}},{key:"_pullDefinitions",value:function(e,t){var n=t.prefix&&t.iconName&&t.icon?{0:t}:t;return Object.keys(n).map(function(t){var r=n[t],a=r.prefix,o=r.iconName,i=r.icon,l=i[2];e[a]||(e[a]={}),l.length>0&&l.forEach(function(t){"string"==typeof t&&(e[a][t]=i)}),e[a][o]=i}),e}}])&&function(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}(t.prototype,n),Object.defineProperty(t,"prototype",{writable:!1}),e}(),Pp=[],Mp={},Dp={},Up=Object.keys(Dp);function jp(e,t){for(var n=arguments.length,r=new Array(n>2?n-2:0),a=2;a<n;a++)r[a-2]=arguments[a];var o=Mp[e]||[];return o.forEach(function(e){t=e.apply(null,[t].concat(r))}),t}function Fp(e){for(var t=arguments.length,n=new Array(t>1?t-1:0),r=1;r<t;r++)n[r-1]=arguments[r];var a=Mp[e]||[];a.forEach(function(e){e.apply(null,n)})}function zp(){var e=arguments[0],t=Array.prototype.slice.call(arguments,1);return Dp[e]?Dp[e].apply(null,t):void 0}function Bp(e){"fa"===e.prefix&&(e.prefix="fas");var t=e.iconName,n=e.prefix||Ap();if(t)return t=xp(n,t)||t,up(Hp.definitions,n,t)||up(op.styles,n,t)}var Hp=new _p,Vp={i2svg:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};return Cd?(Fp("beforeI2svg",e),zp("pseudoElements2svg",e),zp("i2svg",e)):Promise.reject("Operation requires a DOM of some kind.")},watch:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=e.autoReplaceSvgRoot;!1===Wd.autoReplaceSvg&&(Wd.autoReplaceSvg=!0),Wd.observeMutations=!0,sp(function(){Gp({autoReplaceSvgRoot:t}),Fp("watch",e)})}},Wp={noAuto:function(){Wd.autoReplaceSvg=!1,Wd.observeMutations=!1,Fp("noAuto")},config:Wd,dom:Vp,parse:{icon:function(e){if(null===e)return null;if("object"===ud(e)&&e.prefix&&e.iconName)return{prefix:e.prefix,iconName:xp(e.prefix,e.iconName)||e.iconName};if(Array.isArray(e)&&2===e.length){var t=0===e[1].indexOf("fa-")?e[1].slice(3):e[1],n=Ip(e[0]);return{prefix:n,iconName:xp(n,t)||t}}if("string"==typeof e&&(e.indexOf("".concat(Wd.familyPrefix,"-"))>-1||e.match(Md))){var r=Lp(e.split(" "),{skipLookups:!0});return{prefix:r.prefix||Ap(),iconName:xp(r.prefix,r.iconName)||r.iconName}}if("string"==typeof e){var a=Ap();return{prefix:a,iconName:xp(a,e)||e}}}},library:Hp,findIconDefinition:Bp,toHtml:cp},Gp=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=e.autoReplaceSvgRoot,n=void 0===t?Nd:t;(Object.keys(op.styles).length>0||Wd.autoFetchSvg)&&Cd&&Wd.autoReplaceSvg&&Wp.dom.i2svg({node:n})};function Yp(e,t){return Object.defineProperty(e,"abstract",{get:t}),Object.defineProperty(e,"html",{get:function(){return e.abstract.map(function(e){return cp(e)})}}),Object.defineProperty(e,"node",{get:function(){if(Cd){var t=Nd.createElement("div");return t.innerHTML=e.html,t.children}}}),e}function $p(e){var t=e.icons,n=t.main,r=t.mask,a=e.prefix,o=e.iconName,i=e.transform,l=e.symbol,s=e.title,c=e.maskId,u=e.titleId,f=e.extra,d=e.watchable,p=void 0!==d&&d,m=r.found?r:n,h=m.width,g=m.height,y="fak"===a,v=[Wd.replacementClass,o?"".concat(Wd.familyPrefix,"-").concat(o):""].filter(function(e){return-1===f.classes.indexOf(e)}).filter(function(e){return""!==e||!!e}).concat(f.classes).join(" "),b={children:[],attributes:cd(cd({},f.attributes),{},{"data-prefix":a,"data-icon":o,class:v,role:f.attributes.role||"img",xmlns:"http://www.w3.org/2000/svg",viewBox:"0 0 ".concat(h," ").concat(g)})},E=y&&!~f.classes.indexOf("fa-fw")?{width:"".concat(h/g*16*.0625,"em")}:{};p&&(b.attributes["data-fa-i2svg"]=""),s&&(b.children.push({tag:"title",attributes:{id:b.attributes["aria-labelledby"]||"title-".concat(u||Xd())},children:[s]}),delete b.attributes.title);var T=cd(cd({},b),{},{prefix:a,iconName:o,main:n,mask:r,maskId:c,transform:i,symbol:l,styles:cd(cd({},E),f.styles)}),S=r.found&&n.found?zp("generateAbstractMask",T)||{children:[],attributes:{}}:zp("generateAbstractIcon",T)||{children:[],attributes:{}},w=S.attributes;return T.children=S.children,T.attributes=w,l?function(e){var t=e.iconName,n=e.children,r=e.attributes,a=e.symbol,o=!0===a?"".concat(e.prefix,"-").concat(Wd.familyPrefix,"-").concat(t):a;return[{tag:"svg",attributes:{style:"display: none;"},children:[{tag:"symbol",attributes:cd(cd({},r),{},{id:o}),children:n}]}]}(T):function(e){var t=e.children,n=e.main,r=e.mask,a=e.attributes,o=e.styles,i=e.transform;if(Zd(i)&&n.found&&!r.found){var l={x:n.width/n.height/2,y:.5};a.style=Qd(cd(cd({},o),{},{"transform-origin":"".concat(l.x+i.x/16,"em ").concat(l.y+i.y/16,"em")}))}return[{tag:"svg",attributes:a,children:t}]}(T)}function Xp(e){var t=e.content,n=e.width,r=e.height,a=e.transform,o=e.title,i=e.extra,l=e.watchable,s=void 0!==l&&l,c=cd(cd(cd({},i.attributes),o?{title:o}:{}),{},{class:i.classes.join(" ")});s&&(c["data-fa-i2svg"]="");var u=cd({},i.styles);Zd(a)&&(u.transform=function(e){var t=e.transform,n=e.width,r=e.height,a=void 0===r?16:r,o=e.startCentered,i=void 0!==o&&o,l="";return l+=i&&xd?"translate(".concat(t.x/Yd-(void 0===n?16:n)/2,"em, ").concat(t.y/Yd-a/2,"em) "):i?"translate(calc(-50% + ".concat(t.x/Yd,"em), calc(-50% + ").concat(t.y/Yd,"em)) "):"translate(".concat(t.x/Yd,"em, ").concat(t.y/Yd,"em) "),(l+="scale(".concat(t.size/Yd*(t.flipX?-1:1),", ").concat(t.size/Yd*(t.flipY?-1:1),") "))+"rotate(".concat(t.rotate,"deg) ")}({transform:a,startCentered:!0,width:n,height:r}),u["-webkit-transform"]=u.transform);var f=Qd(u);f.length>0&&(c.style=f);var d=[];return d.push({tag:"span",attributes:c,children:[t]}),o&&d.push({tag:"span",attributes:{class:"sr-only"},children:[o]}),d}function qp(e){var t=e.content,n=e.title,r=e.extra,a=cd(cd(cd({},r.attributes),n?{title:n}:{}),{},{class:r.classes.join(" ")}),o=Qd(r.styles);o.length>0&&(a.style=o);var i=[];return i.push({tag:"span",attributes:a,children:[t]}),n&&i.push({tag:"span",attributes:{class:"sr-only"},children:[n]}),i}var Kp=op.styles;function Jp(e){var t=e[0],n=e[1],r=dd(e.slice(4),1)[0];return{found:!0,width:t,height:n,icon:Array.isArray(r)?{tag:"g",attributes:{class:"".concat(Wd.familyPrefix,"-").concat("duotone-group")},children:[{tag:"path",attributes:{class:"".concat(Wd.familyPrefix,"-").concat("secondary"),fill:"currentColor",d:r[0]}},{tag:"path",attributes:{class:"".concat(Wd.familyPrefix,"-").concat("primary"),fill:"currentColor",d:r[1]}}]}:{tag:"path",attributes:{fill:"currentColor",d:r}}}}var Qp={found:!1,width:512,height:512};function Zp(e,t){var n=t;return"fa"===t&&null!==Wd.styleDefault&&(t=Ap()),new Promise(function(r,a){if(zp("missingIconAbstract"),"fa"===n){var o=kp(e)||{};e=o.iconName||e,t=o.prefix||t}if(e&&t&&Kp[t]&&Kp[t][e])return r(Jp(Kp[t][e]));!function(e,t){Ad||Wd.showMissingIcons||!e||console.error('Icon with name "'.concat(e,'" and prefix "').concat(t,'" is missing.'))}(e,t),r(cd(cd({},Qp),{},{icon:Wd.showMissingIcons&&e&&zp("missingIconAbstract")||{}}))})}var em=function(){},tm=Wd.measurePerformance&&Od&&Od.mark&&Od.measure?Od:{mark:em,measure:em},nm=function(e){return tm.mark("".concat('FA "6.1.1"'," ").concat(e," begins")),function(){return function(e){tm.mark("".concat('FA "6.1.1"'," ").concat(e," ends")),tm.measure("".concat('FA "6.1.1"'," ").concat(e),"".concat('FA "6.1.1"'," ").concat(e," begins"),"".concat('FA "6.1.1"'," ").concat(e," ends"))}(e)}},rm=function(){};function am(e){return"string"==typeof(e.getAttribute?e.getAttribute("data-fa-i2svg"):null)}function om(e){return Nd.createElementNS("http://www.w3.org/2000/svg",e)}function im(e){return Nd.createElement(e)}function lm(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.ceFn,r=void 0===n?"svg"===e.tag?om:im:n;if("string"==typeof e)return Nd.createTextNode(e);var a=r(e.tag);Object.keys(e.attributes||[]).forEach(function(t){a.setAttribute(t,e.attributes[t])});var o=e.children||[];return o.forEach(function(e){a.appendChild(lm(e,{ceFn:r}))}),a}var sm={replace:function(e){var t=e[0];if(t.parentNode)if(e[1].forEach(function(e){t.parentNode.insertBefore(lm(e),t)}),null===t.getAttribute("data-fa-i2svg")&&Wd.keepOriginalSource){var n=Nd.createComment(function(e){var t=" ".concat(e.outerHTML," ");return"".concat(t,"Font Awesome fontawesome.com ")}(t));t.parentNode.replaceChild(n,t)}else t.remove()},nest:function(e){var t=e[0],n=e[1];if(~Kd(t).indexOf(Wd.replacementClass))return sm.replace(e);var r=new RegExp("".concat(Wd.familyPrefix,"-.*"));if(delete n[0].attributes.id,n[0].attributes.class){var a=n[0].attributes.class.split(" ").reduce(function(e,t){return t===Wd.replacementClass||t.match(r)?e.toSvg.push(t):e.toNode.push(t),e},{toNode:[],toSvg:[]});n[0].attributes.class=a.toSvg.join(" "),0===a.toNode.length?t.removeAttribute("class"):t.setAttribute("class",a.toNode.join(" "))}var o=n.map(function(e){return cp(e)}).join("\n");t.setAttribute("data-fa-i2svg",""),t.innerHTML=o}};function cm(e){e()}function um(e,t){var n="function"==typeof t?t:rm;if(0===e.length)n();else{var r=cm;"async"===Wd.mutateApproach&&(r=wd.requestAnimationFrame||cm),r(function(){var t=!0===Wd.autoReplaceSvg?sm.replace:sm[Wd.autoReplaceSvg]||sm.replace,r=nm("mutate");e.map(t),r(),n()})}}var fm=!1;function dm(){fm=!0}function pm(){fm=!1}var mm=null;function hm(e){if(Rd&&Wd.observeMutations){var t=e.treeCallback,n=void 0===t?rm:t,r=e.nodeCallback,a=void 0===r?rm:r,o=e.pseudoElementsCallback,i=void 0===o?rm:o,l=e.observeMutationsRoot,s=void 0===l?Nd:l;mm=new Rd(function(e){if(!fm){var t=Ap();qd(e).forEach(function(e){if("childList"===e.type&&e.addedNodes.length>0&&!am(e.addedNodes[0])&&(Wd.searchPseudoElements&&i(e.target),n(e.target)),"attributes"===e.type&&e.target.parentNode&&Wd.searchPseudoElements&&i(e.target.parentNode),"attributes"===e.type&&am(e.target)&&~zd.indexOf(e.attributeName))if("class"===e.attributeName&&function(e){var t=e.getAttribute?e.getAttribute("data-prefix"):null,n=e.getAttribute?e.getAttribute("data-icon"):null;return t&&n}(e.target)){var r=Lp(Kd(e.target)),o=r.iconName;e.target.setAttribute("data-prefix",r.prefix||t),o&&e.target.setAttribute("data-icon",o)}else(function(e){return e&&e.classList&&e.classList.contains&&e.classList.contains(Wd.replacementClass)})(e.target)&&a(e.target)})}}),Cd&&mm.observe(s,{childList:!0,attributes:!0,characterData:!0,subtree:!0})}}function gm(e){var t=e.getAttribute("style"),n=[];return t&&(n=t.split(";").reduce(function(e,t){var n=t.split(":"),r=n[0],a=n.slice(1);return r&&a.length>0&&(e[r]=a.join(":").trim()),e},{})),n}function ym(e){var t=e.getAttribute("data-prefix"),n=e.getAttribute("data-icon"),r=void 0!==e.innerText?e.innerText.trim():"",a=Lp(Kd(e));return a.prefix||(a.prefix=Ap()),t&&n&&(a.prefix=t,a.iconName=n),a.iconName&&a.prefix||a.prefix&&r.length>0&&(a.iconName=(Ep[a.prefix]||{})[e.innerText]||Cp(a.prefix,dp(e.innerText))),a}function vm(e){var t=qd(e.attributes).reduce(function(e,t){return"class"!==e.name&&"style"!==e.name&&(e[t.name]=t.value),e},{}),n=e.getAttribute("title"),r=e.getAttribute("data-fa-title-id");return Wd.autoA11y&&(n?t["aria-labelledby"]="".concat(Wd.replacementClass,"-title-").concat(r||Xd()):(t["aria-hidden"]="true",t.focusable="false")),t}function bm(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{styleParser:!0},n=ym(e),r=n.iconName,a=n.prefix,o=n.rest,i=vm(e),l=jp("parseNodeAttributes",{},e),s=t.styleParser?gm(e):[];return cd({iconName:r,title:e.getAttribute("title"),titleId:e.getAttribute("data-fa-title-id"),prefix:a,transform:$d,mask:{iconName:null,prefix:null,rest:[]},maskId:null,symbol:!1,extra:{classes:o,styles:s,attributes:i}},l)}var Em=op.styles;function Tm(e){var t="nest"===Wd.autoReplaceSvg?bm(e,{styleParser:!1}):bm(e);return~t.extra.classes.indexOf("fa-layers-text")?zp("generateLayersText",e,t):zp("generateSvgReplacementMutation",e,t)}function Sm(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:null;if(!Cd)return Promise.resolve();var n=Nd.documentElement.classList,r=function(e){return n.add("".concat("fontawesome-i2svg","-").concat(e))},a=function(e){return n.remove("".concat("fontawesome-i2svg","-").concat(e))},o=Object.keys(Wd.autoFetchSvg?Id:Em),i=[".".concat("fa-layers-text",":not([").concat("data-fa-i2svg","])")].concat(o.map(function(e){return".".concat(e,":not([").concat("data-fa-i2svg","])")})).join(", ");if(0===i.length)return Promise.resolve();var l=[];try{l=qd(e.querySelectorAll(i))}catch(e){}if(!(l.length>0))return Promise.resolve();r("pending"),a("complete");var s=nm("onTree"),c=l.reduce(function(e,t){try{var n=Tm(t);n&&e.push(n)}catch(e){Ad||"MissingIcon"===e.name&&console.error(e)}return e},[]);return new Promise(function(e,n){Promise.all(c).then(function(n){um(n,function(){r("active"),r("complete"),a("pending"),"function"==typeof t&&t(),s(),e()})}).catch(function(e){s(),n(e)})})}function wm(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:null;Tm(e).then(function(e){e&&um([e],t)})}var Nm=function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.transform,r=void 0===n?$d:n,a=t.symbol,o=void 0!==a&&a,i=t.mask,l=void 0===i?null:i,s=t.maskId,c=void 0===s?null:s,u=t.title,f=void 0===u?null:u,d=t.titleId,p=void 0===d?null:d,m=t.classes,h=void 0===m?[]:m,g=t.attributes,y=void 0===g?{}:g,v=t.styles,b=void 0===v?{}:v;if(e){var E=e.prefix,T=e.iconName,S=e.icon;return Yp(cd({type:"icon"},e),function(){return Fp("beforeDOMElementCreation",{iconDefinition:e,params:t}),Wd.autoA11y&&(f?y["aria-labelledby"]="".concat(Wd.replacementClass,"-title-").concat(p||Xd()):(y["aria-hidden"]="true",y.focusable="false")),$p({icons:{main:Jp(S),mask:l?Jp(l.icon):{found:!1,width:null,height:null,icon:{}}},prefix:E,iconName:T,transform:cd(cd({},$d),r),symbol:o,title:f,maskId:c,titleId:p,extra:{attributes:y,styles:b,classes:h}})})}},Rm={mixout:function(){return{icon:(e=Nm,function(t){var n=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},r=(t||{}).icon?t:Bp(t||{}),a=n.mask;return a&&(a=(a||{}).icon?a:Bp(a||{})),e(r,cd(cd({},n),{},{mask:a}))})};var e},hooks:function(){return{mutationObserverCallbacks:function(e){return e.treeCallback=Sm,e.nodeCallback=wm,e}}},provides:function(e){e.i2svg=function(e){var t=e.node,n=e.callback;return Sm(void 0===t?Nd:t,void 0===n?function(){}:n)},e.generateSvgReplacementMutation=function(e,t){var n=t.iconName,r=t.title,a=t.titleId,o=t.prefix,i=t.transform,l=t.symbol,s=t.mask,c=t.maskId,u=t.extra;return new Promise(function(t,f){Promise.all([Zp(n,o),s.iconName?Zp(s.iconName,s.prefix):Promise.resolve({found:!1,width:512,height:512,icon:{}})]).then(function(s){var f=dd(s,2);t([e,$p({icons:{main:f[0],mask:f[1]},prefix:o,iconName:n,transform:i,symbol:l,maskId:c,title:r,titleId:a,extra:u,watchable:!0})])}).catch(f)})},e.generateAbstractIcon=function(e){var t,n=e.children,r=e.attributes,a=e.main,o=e.transform,i=Qd(e.styles);return i.length>0&&(r.style=i),Zd(o)&&(t=zp("generateAbstractTransformGrouping",{main:a,transform:o,containerWidth:a.width,iconWidth:a.width})),n.push(t||a.icon),{children:n,attributes:r}}}},Om={mixout:function(){return{layer:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.classes,r=void 0===n?[]:n;return Yp({type:"layer"},function(){Fp("beforeDOMElementCreation",{assembler:e,params:t});var n=[];return e(function(e){Array.isArray(e)?e.map(function(e){n=n.concat(e.abstract)}):n=n.concat(e.abstract)}),[{tag:"span",attributes:{class:["".concat(Wd.familyPrefix,"-layers")].concat(pd(r)).join(" ")},children:n}]})}}}},Cm={mixout:function(){return{counter:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.title,r=void 0===n?null:n,a=t.classes,o=void 0===a?[]:a,i=t.attributes,l=void 0===i?{}:i,s=t.styles,c=void 0===s?{}:s;return Yp({type:"counter",content:e},function(){return Fp("beforeDOMElementCreation",{content:e,params:t}),qp({content:e.toString(),title:r,extra:{attributes:l,styles:c,classes:["".concat(Wd.familyPrefix,"-layers-counter")].concat(pd(o))}})})}}}},xm={mixout:function(){return{text:function(e){var t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},n=t.transform,r=void 0===n?$d:n,a=t.title,o=void 0===a?null:a,i=t.classes,l=void 0===i?[]:i,s=t.attributes,c=void 0===s?{}:s,u=t.styles,f=void 0===u?{}:u;return Yp({type:"text",content:e},function(){return Fp("beforeDOMElementCreation",{content:e,params:t}),Xp({content:e,transform:cd(cd({},$d),r),title:o,extra:{attributes:c,styles:f,classes:["".concat(Wd.familyPrefix,"-layers-text")].concat(pd(l))}})})}}},provides:function(e){e.generateLayersText=function(e,t){var n=t.title,r=t.transform,a=t.extra,o=null,i=null;if(xd){var l=parseInt(getComputedStyle(e).fontSize,10),s=e.getBoundingClientRect();o=s.width/l,i=s.height/l}return Wd.autoA11y&&!n&&(a.attributes["aria-hidden"]="true"),Promise.resolve([e,Xp({content:e.innerHTML,width:o,height:i,transform:r,title:n,extra:a,watchable:!0})])}}},km=new RegExp('"',"ug"),Am=[1105920,1112319];function Im(e,t){var n="".concat("data-fa-pseudo-element-pending").concat(t.replace(":","-"));return new Promise(function(r,a){if(null!==e.getAttribute(n))return r();var o,i,l,s=qd(e.children).filter(function(e){return e.getAttribute("data-fa-pseudo-element")===t})[0],c=wd.getComputedStyle(e,t),u=c.getPropertyValue("font-family").match(Dd),f=c.getPropertyValue("font-weight"),d=c.getPropertyValue("content");if(s&&!u)return e.removeChild(s),r();if(u&&"none"!==d&&""!==d){var p=c.getPropertyValue("content"),m=~["Solid","Regular","Light","Thin","Duotone","Brands","Kit"].indexOf(u[2])?Ld[u[2].toLowerCase()]:Ud[f],h=function(e){var t,n,r,a,o=e.replace(km,""),i=(r=(t=o).length,(a=t.charCodeAt(0))>=55296&&a<=56319&&r>1&&(n=t.charCodeAt(1))>=56320&&n<=57343?1024*(a-55296)+n-56320+65536:a),l=i>=Am[0]&&i<=Am[1],s=2===o.length&&o[0]===o[1];return{value:dp(s?o[0]:o),isSecondary:l||s}}(p),g=h.value,y=h.isSecondary,v=u[0].startsWith("FontAwesome"),b=Cp(m,g),E=b;if(v){var T=(i=Sp[o=g],l=Cp("fas",o),i||(l?{prefix:"fas",iconName:l}:null)||{prefix:null,iconName:null});T.iconName&&T.prefix&&(b=T.iconName,m=T.prefix)}if(!b||y||s&&s.getAttribute("data-prefix")===m&&s.getAttribute("data-icon")===E)r();else{e.setAttribute(n,E),s&&e.removeChild(s);var S={iconName:null,title:null,titleId:null,prefix:null,transform:$d,symbol:!1,mask:{iconName:null,prefix:null,rest:[]},maskId:null,extra:{classes:[],styles:{},attributes:{}}},w=S.extra;w.attributes["data-fa-pseudo-element"]=t,Zp(b,m).then(function(a){var o=$p(cd(cd({},S),{},{icons:{main:a,mask:{prefix:null,iconName:null,rest:[]}},prefix:m,iconName:E,extra:w,watchable:!0})),i=Nd.createElement("svg");"::before"===t?e.insertBefore(i,e.firstChild):e.appendChild(i),i.outerHTML=o.map(function(e){return cp(e)}).join("\n"),e.removeAttribute(n),r()}).catch(a)}}else r()})}function Lm(e){return Promise.all([Im(e,"::before"),Im(e,"::after")])}function _m(e){return!(e.parentNode===document.head||~kd.indexOf(e.tagName.toUpperCase())||e.getAttribute("data-fa-pseudo-element")||e.parentNode&&"svg"===e.parentNode.tagName)}function Pm(e){if(Cd)return new Promise(function(t,n){var r=qd(e.querySelectorAll("*")).filter(_m).map(Lm),a=nm("searchPseudoElements");dm(),Promise.all(r).then(function(){a(),pm(),t()}).catch(function(){a(),pm(),n()})})}var Mm=!1,Dm=function(e){return e.toLowerCase().split(" ").reduce(function(e,t){var n=t.toLowerCase().split("-"),r=n[0],a=n.slice(1).join("-");if(r&&"h"===a)return e.flipX=!0,e;if(r&&"v"===a)return e.flipY=!0,e;if(a=parseFloat(a),isNaN(a))return e;switch(r){case"grow":e.size=e.size+a;break;case"shrink":e.size=e.size-a;break;case"left":e.x=e.x-a;break;case"right":e.x=e.x+a;break;case"up":e.y=e.y-a;break;case"down":e.y=e.y+a;break;case"rotate":e.rotate=e.rotate+a}return e},{size:16,x:0,y:0,flipX:!1,flipY:!1,rotate:0})},Um={x:0,y:0,width:"100%",height:"100%"};function jm(e){var t=!(arguments.length>1&&void 0!==arguments[1])||arguments[1];return e.attributes&&(e.attributes.fill||t)&&(e.attributes.fill="black"),e}!function(e,t){var n=Wp;Pp=e,Mp={},Object.keys(Dp).forEach(function(e){-1===Up.indexOf(e)&&delete Dp[e]}),Pp.forEach(function(e){var t=e.mixout?e.mixout():{};if(Object.keys(t).forEach(function(e){"function"==typeof t[e]&&(n[e]=t[e]),"object"===ud(t[e])&&Object.keys(t[e]).forEach(function(r){n[e]||(n[e]={}),n[e][r]=t[e][r]})}),e.hooks){var r=e.hooks();Object.keys(r).forEach(function(e){Mp[e]||(Mp[e]=[]),Mp[e].push(r[e])})}e.provides&&e.provides(Dp)})}([rp,Rm,Om,Cm,xm,{hooks:function(){return{mutationObserverCallbacks:function(e){return e.pseudoElementsCallback=Pm,e}}},provides:function(e){e.pseudoElements2svg=function(e){var t=e.node;Wd.searchPseudoElements&&Pm(void 0===t?Nd:t)}}},{mixout:function(){return{dom:{unwatch:function(){dm(),Mm=!0}}}},hooks:function(){return{bootstrap:function(){hm(jp("mutationObserverCallbacks",{}))},noAuto:function(){mm&&mm.disconnect()},watch:function(e){var t=e.observeMutationsRoot;Mm?pm():hm(jp("mutationObserverCallbacks",{observeMutationsRoot:t}))}}}},{mixout:function(){return{parse:{transform:function(e){return Dm(e)}}}},hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-transform");return n&&(e.transform=Dm(n)),e}}},provides:function(e){e.generateAbstractTransformGrouping=function(e){var t=e.main,n=e.transform,r=e.iconWidth,a={transform:"translate(".concat(e.containerWidth/2," 256)")},o="translate(".concat(32*n.x,", ").concat(32*n.y,") "),i="scale(".concat(n.size/16*(n.flipX?-1:1),", ").concat(n.size/16*(n.flipY?-1:1),") "),l="rotate(".concat(n.rotate," 0 0)"),s={outer:a,inner:{transform:"".concat(o," ").concat(i," ").concat(l)},path:{transform:"translate(".concat(r/2*-1," -256)")}};return{tag:"g",attributes:cd({},s.outer),children:[{tag:"g",attributes:cd({},s.inner),children:[{tag:t.icon.tag,children:t.icon.children,attributes:cd(cd({},t.icon.attributes),s.path)}]}]}}}},{hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-mask"),r=n?Lp(n.split(" ").map(function(e){return e.trim()})):{prefix:null,iconName:null,rest:[]};return r.prefix||(r.prefix=Ap()),e.mask=r,e.maskId=t.getAttribute("data-fa-mask-id"),e}}},provides:function(e){e.generateAbstractMask=function(e){var t,n=e.children,r=e.attributes,a=e.main,o=e.mask,i=e.maskId,l=a.icon,s=o.icon,c=function(e){var t=e.transform,n=e.iconWidth,r={transform:"translate(".concat(e.containerWidth/2," 256)")},a="translate(".concat(32*t.x,", ").concat(32*t.y,") "),o="scale(".concat(t.size/16*(t.flipX?-1:1),", ").concat(t.size/16*(t.flipY?-1:1),") "),i="rotate(".concat(t.rotate," 0 0)");return{outer:r,inner:{transform:"".concat(a," ").concat(o," ").concat(i)},path:{transform:"translate(".concat(n/2*-1," -256)")}}}({transform:e.transform,containerWidth:o.width,iconWidth:a.width}),u={tag:"rect",attributes:cd(cd({},Um),{},{fill:"white"})},f=l.children?{children:l.children.map(jm)}:{},d={tag:"g",attributes:cd({},c.inner),children:[jm(cd({tag:l.tag,attributes:cd(cd({},l.attributes),c.path)},f))]},p={tag:"g",attributes:cd({},c.outer),children:[d]},m="mask-".concat(i||Xd()),h="clip-".concat(i||Xd()),g={tag:"mask",attributes:cd(cd({},Um),{},{id:m,maskUnits:"userSpaceOnUse",maskContentUnits:"userSpaceOnUse"}),children:[u,p]},y={tag:"defs",children:[{tag:"clipPath",attributes:{id:h},children:(t=s,"g"===t.tag?t.children:[t])},g]};return n.push(y,{tag:"rect",attributes:cd({fill:"currentColor","clip-path":"url(#".concat(h,")"),mask:"url(#".concat(m,")")},Um)}),{children:n,attributes:r}}}},{provides:function(e){var t=!1;wd.matchMedia&&(t=wd.matchMedia("(prefers-reduced-motion: reduce)").matches),e.missingIconAbstract=function(){var e=[],n={fill:"currentColor"},r={attributeType:"XML",repeatCount:"indefinite",dur:"2s"};e.push({tag:"path",attributes:cd(cd({},n),{},{d:"M156.5,447.7l-12.6,29.5c-18.7-9.5-35.9-21.2-51.5-34.9l22.7-22.7C127.6,430.5,141.5,440,156.5,447.7z M40.6,272H8.5 c1.4,21.2,5.4,41.7,11.7,61.1L50,321.2C45.1,305.5,41.8,289,40.6,272z M40.6,240c1.4-18.8,5.2-37,11.1-54.1l-29.5-12.6 C14.7,194.3,10,216.7,8.5,240H40.6z M64.3,156.5c7.8-14.9,17.2-28.8,28.1-41.5L69.7,92.3c-13.7,15.6-25.5,32.8-34.9,51.5 L64.3,156.5z M397,419.6c-13.9,12-29.4,22.3-46.1,30.4l11.9,29.8c20.7-9.9,39.8-22.6,56.9-37.6L397,419.6z M115,92.4 c13.9-12,29.4-22.3,46.1-30.4l-11.9-29.8c-20.7,9.9-39.8,22.6-56.8,37.6L115,92.4z M447.7,355.5c-7.8,14.9-17.2,28.8-28.1,41.5 l22.7,22.7c13.7-15.6,25.5-32.9,34.9-51.5L447.7,355.5z M471.4,272c-1.4,18.8-5.2,37-11.1,54.1l29.5,12.6 c7.5-21.1,12.2-43.5,13.6-66.8H471.4z M321.2,462c-15.7,5-32.2,8.2-49.2,9.4v32.1c21.2-1.4,41.7-5.4,61.1-11.7L321.2,462z M240,471.4c-18.8-1.4-37-5.2-54.1-11.1l-12.6,29.5c21.1,7.5,43.5,12.2,66.8,13.6V471.4z M462,190.8c5,15.7,8.2,32.2,9.4,49.2h32.1 c-1.4-21.2-5.4-41.7-11.7-61.1L462,190.8z M92.4,397c-12-13.9-22.3-29.4-30.4-46.1l-29.8,11.9c9.9,20.7,22.6,39.8,37.6,56.9 L92.4,397z M272,40.6c18.8,1.4,36.9,5.2,54.1,11.1l12.6-29.5C317.7,14.7,295.3,10,272,8.5V40.6z M190.8,50 c15.7-5,32.2-8.2,49.2-9.4V8.5c-21.2,1.4-41.7,5.4-61.1,11.7L190.8,50z M442.3,92.3L419.6,115c12,13.9,22.3,29.4,30.5,46.1 l29.8-11.9C470,128.5,457.3,109.4,442.3,92.3z M397,92.4l22.7-22.7c-15.6-13.7-32.8-25.5-51.5-34.9l-12.6,29.5 C370.4,72.1,384.4,81.5,397,92.4z"})});var a=cd(cd({},r),{},{attributeName:"opacity"}),o={tag:"circle",attributes:cd(cd({},n),{},{cx:"256",cy:"364",r:"28"}),children:[]};return t||o.children.push({tag:"animate",attributes:cd(cd({},r),{},{attributeName:"r",values:"28;14;28;28;14;28;"})},{tag:"animate",attributes:cd(cd({},a),{},{values:"1;0;1;1;0;1;"})}),e.push(o),e.push({tag:"path",attributes:cd(cd({},n),{},{opacity:"1",d:"M263.7,312h-16c-6.6,0-12-5.4-12-12c0-71,77.4-63.9,77.4-107.8c0-20-17.8-40.2-57.4-40.2c-29.1,0-44.3,9.6-59.2,28.7 c-3.9,5-11.1,6-16.2,2.4l-13.1-9.2c-5.6-3.9-6.9-11.8-2.6-17.2c21.2-27.2,46.4-44.7,91.2-44.7c52.3,0,97.4,29.8,97.4,80.2 c0,67.6-77.4,63.5-77.4,107.8C275.7,306.6,270.3,312,263.7,312z"}),children:t?[]:[{tag:"animate",attributes:cd(cd({},a),{},{values:"1;0;0;0;0;1;"})}]}),t||e.push({tag:"path",attributes:cd(cd({},n),{},{opacity:"0",d:"M232.5,134.5l7,168c0.3,6.4,5.6,11.5,12,11.5h9c6.4,0,11.7-5.1,12-11.5l7-168c0.3-6.8-5.2-12.5-12-12.5h-23 C237.7,122,232.2,127.7,232.5,134.5z"}),children:[{tag:"animate",attributes:cd(cd({},a),{},{values:"0;0;1;1;0;0;"})}]}),{tag:"g",attributes:{class:"missing"},children:e}}}},{hooks:function(){return{parseNodeAttributes:function(e,t){var n=t.getAttribute("data-fa-symbol");return e.symbol=null!==n&&(""===n||n),e}}}}]);var Fm,zm=Wp.parse,Bm=Wp.icon,Hm=n(function(e,t){!function(){var e="function"==typeof Symbol&&Symbol.for,n=e?Symbol.for("react.element"):60103,r=e?Symbol.for("react.portal"):60106,a=e?Symbol.for("react.fragment"):60107,o=e?Symbol.for("react.strict_mode"):60108,i=e?Symbol.for("react.profiler"):60114,l=e?Symbol.for("react.provider"):60109,s=e?Symbol.for("react.context"):60110,c=e?Symbol.for("react.async_mode"):60111,u=e?Symbol.for("react.concurrent_mode"):60111,f=e?Symbol.for("react.forward_ref"):60112,d=e?Symbol.for("react.suspense"):60113,p=e?Symbol.for("react.suspense_list"):60120,m=e?Symbol.for("react.memo"):60115,h=e?Symbol.for("react.lazy"):60116,g=e?Symbol.for("react.block"):60121,y=e?Symbol.for("react.fundamental"):60117,v=e?Symbol.for("react.responder"):60118,b=e?Symbol.for("react.scope"):60119;function E(e){if("object"==typeof e&&null!==e){var t=e.$$typeof;switch(t){case n:var p=e.type;switch(p){case c:case u:case a:case i:case o:case d:return p;default:var g=p&&p.$$typeof;switch(g){case s:case f:case h:case m:case l:return g;default:return t}}case r:return t}}}var T=u,S=s,w=l,N=n,R=f,O=a,C=h,x=m,k=r,A=i,I=o,L=d,_=!1;function P(e){return E(e)===u}t.AsyncMode=c,t.ConcurrentMode=T,t.ContextConsumer=S,t.ContextProvider=w,t.Element=N,t.ForwardRef=R,t.Fragment=O,t.Lazy=C,t.Memo=x,t.Portal=k,t.Profiler=A,t.StrictMode=I,t.Suspense=L,t.isAsyncMode=function(e){return _||(_=!0,console.warn("The ReactIs.isAsyncMode() alias has been deprecated, and will be removed in React 17+. Update your code to use ReactIs.isConcurrentMode() instead. It has the exact same API.")),P(e)||E(e)===c},t.isConcurrentMode=P,t.isContextConsumer=function(e){return E(e)===s},t.isContextProvider=function(e){return E(e)===l},t.isElement=function(e){return"object"==typeof e&&null!==e&&e.$$typeof===n},t.isForwardRef=function(e){return E(e)===f},t.isFragment=function(e){return E(e)===a},t.isLazy=function(e){return E(e)===h},t.isMemo=function(e){return E(e)===m},t.isPortal=function(e){return E(e)===r},t.isProfiler=function(e){return E(e)===i},t.isStrictMode=function(e){return E(e)===o},t.isSuspense=function(e){return E(e)===d},t.isValidElementType=function(e){return"string"==typeof e||"function"==typeof e||e===a||e===u||e===i||e===o||e===d||e===p||"object"==typeof e&&null!==e&&(e.$$typeof===h||e.$$typeof===m||e.$$typeof===l||e.$$typeof===s||e.$$typeof===f||e.$$typeof===y||e.$$typeof===v||e.$$typeof===b||e.$$typeof===g)},t.typeOf=E}()}),Vm=n(function(e){e.exports=Hm}),Wm="SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED",Gm=Function.call.bind(Object.prototype.hasOwnProperty),Ym={},$m=Gm;function Xm(e,t,n,r,a){for(var o in e)if($m(e,o)){var i;try{if("function"!=typeof e[o]){var l=Error((r||"React class")+": "+n+" type `"+o+"` is invalid; it must be a function, usually from the `prop-types` package, but received `"+typeof e[o]+"`.This often happens because of typos such as `PropTypes.function` instead of `PropTypes.func`.");throw l.name="Invariant Violation",l}i=e[o](t,o,r,n,null,"SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED")}catch(e){i=e}if(!i||i instanceof Error||Fm((r||"React class")+": type specification of "+n+" `"+o+"` is invalid; the type checker function must return `null` or an `Error` but returned a "+typeof i+". You may have forgotten to pass an argument to the type checker creator (arrayOf, instanceOf, objectOf, oneOf, oneOfType, and shape all require an argument)."),i instanceof Error&&!(i.message in Ym)){Ym[i.message]=!0;var s=a?a():"";Fm("Failed "+n+" type: "+i.message+(null!=s?s:""))}}}Fm=function(e){var t="Warning: "+e;"undefined"!=typeof console&&console.error(t);try{throw new Error(t)}catch(e){}},Xm.resetWarningCache=function(){Ym={}};var qm,Km=Xm;function Jm(){return null}qm=function(e){var t="Warning: "+e;"undefined"!=typeof console&&console.error(t);try{throw new Error(t)}catch(e){}};var Qm=n(function(e){e.exports=function(e,t){var n="function"==typeof Symbol&&Symbol.iterator,r="<<anonymous>>",a={array:c("array"),bigint:c("bigint"),bool:c("boolean"),func:c("function"),number:c("number"),object:c("object"),string:c("string"),symbol:c("symbol"),any:s(Jm),arrayOf:function(e){return s(function(t,n,r,a,o){if("function"!=typeof e)return new i("Property `"+o+"` of component `"+r+"` has invalid PropType notation inside arrayOf.");var l=t[n];if(!Array.isArray(l))return new i("Invalid "+a+" `"+o+"` of type `"+d(l)+"` supplied to `"+r+"`, expected an array.");for(var s=0;s<l.length;s++){var c=e(l,s,r,a,o+"["+s+"]",Wm);if(c instanceof Error)return c}return null})},element:s(function(t,n,r,a,o){var l=t[n];return e(l)?null:new i("Invalid "+a+" `"+o+"` of type `"+d(l)+"` supplied to `"+r+"`, expected a single ReactElement.")}),elementType:s(function(e,t,n,r,a){var o=e[t];return Vm.isValidElementType(o)?null:new i("Invalid "+r+" `"+a+"` of type `"+d(o)+"` supplied to `"+n+"`, expected a single ReactElement type.")}),instanceOf:function(e){return s(function(t,n,a,o,l){return t[n]instanceof e?null:new i("Invalid "+o+" `"+l+"` of type `"+((s=t[n]).constructor&&s.constructor.name?s.constructor.name:r)+"` supplied to `"+a+"`, expected instance of `"+(e.name||r)+"`.");var s})},node:s(function(e,t,n,r,a){return f(e[t])?null:new i("Invalid "+r+" `"+a+"` supplied to `"+n+"`, expected a ReactNode.")}),objectOf:function(e){return s(function(t,n,r,a,o){if("function"!=typeof e)return new i("Property `"+o+"` of component `"+r+"` has invalid PropType notation inside objectOf.");var l=t[n],s=d(l);if("object"!==s)return new i("Invalid "+a+" `"+o+"` of type `"+s+"` supplied to `"+r+"`, expected an object.");for(var c in l)if(Gm(l,c)){var u=e(l,c,r,a,o+"."+c,Wm);if(u instanceof Error)return u}return null})},oneOf:function(e){if(!Array.isArray(e))return qm(arguments.length>1?"Invalid arguments supplied to oneOf, expected an array, got "+arguments.length+" arguments. A common mistake is to write oneOf(x, y, z) instead of oneOf([x, y, z]).":"Invalid argument supplied to oneOf, expected an array."),Jm;function t(t,n,r,a,l){for(var s=t[n],c=0;c<e.length;c++)if(o(s,e[c]))return null;var u=JSON.stringify(e,function(e,t){return"symbol"===p(t)?String(t):t});return new i("Invalid "+a+" `"+l+"` of value `"+String(s)+"` supplied to `"+r+"`, expected one of "+u+".")}return s(t)},oneOfType:function(e){if(!Array.isArray(e))return qm("Invalid argument supplied to oneOfType, expected an instance of array."),Jm;for(var t=0;t<e.length;t++){var n=e[t];if("function"!=typeof n)return qm("Invalid argument supplied to oneOfType. Expected an array of check functions, but received "+m(n)+" at index "+t+"."),Jm}return s(function(t,n,r,a,o){for(var l=[],s=0;s<e.length;s++){var c=(0,e[s])(t,n,r,a,o,Wm);if(null==c)return null;c.data&&Gm(c.data,"expectedType")&&l.push(c.data.expectedType)}return new i("Invalid "+a+" `"+o+"` supplied to `"+r+"`"+(l.length>0?", expected one of type ["+l.join(", ")+"]":"")+".")})},shape:function(e){return s(function(t,n,r,a,o){var l=t[n],s=d(l);if("object"!==s)return new i("Invalid "+a+" `"+o+"` of type `"+s+"` supplied to `"+r+"`, expected `object`.");for(var c in e){var f=e[c];if("function"!=typeof f)return u(r,a,o,c,p(f));var m=f(l,c,r,a,o+"."+c,Wm);if(m)return m}return null})},exact:function(e){return s(function(t,n,r,a,o){var s=t[n],c=d(s);if("object"!==c)return new i("Invalid "+a+" `"+o+"` of type `"+c+"` supplied to `"+r+"`, expected `object`.");var f=l({},t[n],e);for(var m in f){var h=e[m];if(Gm(e,m)&&"function"!=typeof h)return u(r,a,o,m,p(h));if(!h)return new i("Invalid "+a+" `"+o+"` key `"+m+"` supplied to `"+r+"`.\nBad object: "+JSON.stringify(t[n],null," ")+"\nValid keys: "+JSON.stringify(Object.keys(e),null," "));var g=h(s,m,r,a,o+"."+m,Wm);if(g)return g}return null})}};function o(e,t){return e===t?0!==e||1/e==1/t:e!=e&&t!=t}function i(e,t){this.message=e,this.data=t&&"object"==typeof t?t:{},this.stack=""}function s(e){function t(t,n,a,o,l,s,c){if(o=o||r,s=s||a,c!==Wm){var u=new Error("Calling PropTypes validators directly is not supported by the `prop-types` package. Use `PropTypes.checkPropTypes()` to call them. Read more at http://fb.me/use-check-prop-types");throw u.name="Invariant Violation",u}return null==n[a]?t?new i(null===n[a]?"The "+l+" `"+s+"` is marked as required in `"+o+"`, but its value is `null`.":"The "+l+" `"+s+"` is marked as required in `"+o+"`, but its value is `undefined`."):null:e(n,a,o,l,s)}var n=t.bind(null,!1);return n.isRequired=t.bind(null,!0),n}function c(e){return s(function(t,n,r,a,o,l){var s=t[n];return d(s)!==e?new i("Invalid "+a+" `"+o+"` of type `"+p(s)+"` supplied to `"+r+"`, expected `"+e+"`.",{expectedType:e}):null})}function u(e,t,n,r,a){return new i((e||"React class")+": "+t+" type `"+n+"."+r+"` is invalid; it must be a function, usually from the `prop-types` package, but received `"+a+"`.")}function f(t){switch(typeof t){case"number":case"string":case"undefined":return!0;case"boolean":return!t;case"object":if(Array.isArray(t))return t.every(f);if(null===t||e(t))return!0;var r=function(e){var t=e&&(n&&e[n]||e["@@iterator"]);if("function"==typeof t)return t}(t);if(!r)return!1;var a,o=r.call(t);if(r!==t.entries){for(;!(a=o.next()).done;)if(!f(a.value))return!1}else for(;!(a=o.next()).done;){var i=a.value;if(i&&!f(i[1]))return!1}return!0;default:return!1}}function d(e){var t=typeof e;return Array.isArray(e)?"array":e instanceof RegExp?"object":function(e,t){return"symbol"===e||!!t&&("Symbol"===t["@@toStringTag"]||"function"==typeof Symbol&&t instanceof Symbol)}(t,e)?"symbol":t}function p(e){if(null==e)return""+e;var t=d(e);if("object"===t){if(e instanceof Date)return"date";if(e instanceof RegExp)return"regexp"}return t}function m(e){var t=p(e);switch(t){case"array":case"object":return"an "+t;case"boolean":case"date":case"regexp":return"a "+t;default:return t}}return i.prototype=Error.prototype,a.checkPropTypes=Km,a.resetWarningCache=Km.resetWarningCache,a.PropTypes=a,a}(Vm.isElement)});function Zm(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var r=Object.getOwnPropertySymbols(e);t&&(r=r.filter(function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable})),n.push.apply(n,r)}return n}function eh(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?Zm(Object(n),!0).forEach(function(t){nh(e,t,n[t])}):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):Zm(Object(n)).forEach(function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))})}return e}function th(e){return(th="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function nh(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function rh(e,t){if(null==e)return{};var n,r,a=function(e,t){if(null==e)return{};var n,r,a={},o=Object.keys(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||(a[n]=e[n]);return a}(e,t);if(Object.getOwnPropertySymbols){var o=Object.getOwnPropertySymbols(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||Object.prototype.propertyIsEnumerable.call(e,n)&&(a[n]=e[n])}return a}function ah(e){return function(e){if(Array.isArray(e))return oh(e)}(e)||function(e){if("undefined"!=typeof Symbol&&null!=e[Symbol.iterator]||null!=e["@@iterator"])return Array.from(e)}(e)||function(e,t){if(e){if("string"==typeof e)return oh(e,t);var n=Object.prototype.toString.call(e).slice(8,-1);return"Object"===n&&e.constructor&&(n=e.constructor.name),"Map"===n||"Set"===n?Array.from(e):"Arguments"===n||/^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)?oh(e,t):void 0}}(e)||function(){throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")}()}function oh(e,t){(null==t||t>e.length)&&(t=e.length);for(var n=0,r=new Array(t);n<t;n++)r[n]=e[n];return r}function ih(e){return t=e,(t-=0)==t?e:(e=e.replace(/[\-_\s]+(.)?/g,function(e,t){return t?t.toUpperCase():""})).substr(0,1).toLowerCase()+e.substr(1);var t}var lh=["style"];function sh(e){return e.split(";").map(function(e){return e.trim()}).filter(function(e){return e}).reduce(function(e,t){var n,r=t.indexOf(":"),a=ih(t.slice(0,r)),o=t.slice(r+1).trim();return a.startsWith("webkit")?e[(n=a,n.charAt(0).toUpperCase()+n.slice(1))]=o:e[a]=o,e},{})}var ch=!1;try{ch=!1}catch(pa){}function uh(e){return e&&"object"===th(e)&&e.prefix&&e.iconName&&e.icon?e:zm.icon?zm.icon(e):null===e?null:e&&"object"===th(e)&&e.prefix&&e.iconName?e:Array.isArray(e)&&2===e.length?{prefix:e[0],iconName:e[1]}:"string"==typeof e?{prefix:"fas",iconName:e}:void 0}function fh(e,t){return Array.isArray(t)&&t.length>0||!Array.isArray(t)&&t?nh({},e,t):{}}var dh=["forwardedRef"];function ph(e){var t=e.forwardedRef,n=rh(e,dh),r=n.mask,a=n.symbol,o=n.className,i=n.title,l=n.titleId,s=n.maskId,c=uh(n.icon),u=fh("classes",[].concat(ah(function(e){var t,n=e.flip,r=e.size,a=e.rotation,o=e.pull,i=(nh(t={"fa-beat":e.beat,"fa-fade":e.fade,"fa-beat-fade":e.beatFade,"fa-bounce":e.bounce,"fa-shake":e.shake,"fa-flash":e.flash,"fa-spin":e.spin,"fa-spin-reverse":e.spinReverse,"fa-spin-pulse":e.spinPulse,"fa-pulse":e.pulse,"fa-fw":e.fixedWidth,"fa-inverse":e.inverse,"fa-border":e.border,"fa-li":e.listItem,"fa-flip-horizontal":"horizontal"===n||"both"===n,"fa-flip-vertical":"vertical"===n||"both"===n},"fa-".concat(r),null!=r),nh(t,"fa-rotate-".concat(a),null!=a&&0!==a),nh(t,"fa-pull-".concat(o),null!=o),nh(t,"fa-swap-opacity",e.swapOpacity),t);return Object.keys(i).map(function(e){return i[e]?e:null}).filter(function(e){return e})}(n)),ah(o.split(" ")))),f=fh("transform","string"==typeof n.transform?zm.transform(n.transform):n.transform),d=fh("mask",uh(r)),p=Bm(c,eh(eh(eh(eh({},u),f),d),{},{symbol:a,title:i,titleId:l,maskId:s}));if(!p)return function(){var e;!ch&&console&&"function"==typeof console.error&&(e=console).error.apply(e,arguments)}("Could not find icon",c),null;var m=p.abstract,h={ref:t};return Object.keys(n).forEach(function(e){ph.defaultProps.hasOwnProperty(e)||(h[e]=n[e])}),mh(m[0],h)}ph.displayName="FontAwesomeIcon",ph.propTypes={beat:Qm.bool,border:Qm.bool,bounce:Qm.bool,className:Qm.string,fade:Qm.bool,flash:Qm.bool,mask:Qm.oneOfType([Qm.object,Qm.array,Qm.string]),maskId:Qm.string,fixedWidth:Qm.bool,inverse:Qm.bool,flip:Qm.oneOf(["horizontal","vertical","both"]),icon:Qm.oneOfType([Qm.object,Qm.array,Qm.string]),listItem:Qm.bool,pull:Qm.oneOf(["right","left"]),pulse:Qm.bool,rotation:Qm.oneOf([0,90,180,270]),shake:Qm.bool,size:Qm.oneOf(["2xs","xs","sm","lg","xl","2xl","1x","2x","3x","4x","5x","6x","7x","8x","9x","10x"]),spin:Qm.bool,spinPulse:Qm.bool,spinReverse:Qm.bool,symbol:Qm.oneOfType([Qm.bool,Qm.string]),title:Qm.string,titleId:Qm.string,transform:Qm.oneOfType([Qm.string,Qm.object]),swapOpacity:Qm.bool},ph.defaultProps={border:!1,className:"",mask:null,maskId:null,fixedWidth:!1,inverse:!1,flip:null,icon:null,listItem:!1,pull:null,pulse:!1,rotation:null,size:null,spin:!1,beat:!1,fade:!1,beatFade:!1,bounce:!1,shake:!1,symbol:!1,title:"",titleId:null,transform:null,swapOpacity:!1};var mh=function e(t,n){var r=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{};if("string"==typeof n)return n;var a=(n.children||[]).map(function(n){return e(t,n)}),o=Object.keys(n.attributes||{}).reduce(function(e,t){var r=n.attributes[t];switch(t){case"class":e.attrs.className=r,delete n.attributes.class;break;case"style":e.attrs.style=sh(r);break;default:0===t.indexOf("aria-")||0===t.indexOf("data-")?e.attrs[t.toLowerCase()]=r:e.attrs[ih(t)]=r}return e},{attrs:{}}),i=r.style,l=void 0===i?{}:i,s=rh(r,lh);return o.attrs.style=eh(eh({},o.attrs.style),l),t.apply(void 0,[n.tag,eh(eh({},o.attrs),s)].concat(ah(a)))}.bind(null,c.createElement),hh={prefix:"fas",iconName:"align-left",icon:[448,512,[],"f036","M256 96H32C14.33 96 0 81.67 0 64C0 46.33 14.33 32 32 32H256C273.7 32 288 46.33 288 64C288 81.67 273.7 96 256 96zM256 352H32C14.33 352 0 337.7 0 320C0 302.3 14.33 288 32 288H256C273.7 288 288 302.3 288 320C288 337.7 273.7 352 256 352zM0 192C0 174.3 14.33 160 32 160H416C433.7 160 448 174.3 448 192C448 209.7 433.7 224 416 224H32C14.33 224 0 209.7 0 192zM416 480H32C14.33 480 0 465.7 0 448C0 430.3 14.33 416 32 416H416C433.7 416 448 430.3 448 448C448 465.7 433.7 480 416 480z"]},gh={prefix:"fas",iconName:"angle-down",icon:[384,512,[8964],"f107","M192 384c-8.188 0-16.38-3.125-22.62-9.375l-160-160c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0L192 306.8l137.4-137.4c12.5-12.5 32.75-12.5 45.25 0s12.5 32.75 0 45.25l-160 160C208.4 380.9 200.2 384 192 384z"]},yh={prefix:"fas",iconName:"bug",icon:[512,512,[],"f188","M352 96V99.56C352 115.3 339.3 128 323.6 128H188.4C172.7 128 159.1 115.3 159.1 99.56V96C159.1 42.98 202.1 0 255.1 0C309 0 352 42.98 352 96zM41.37 105.4C53.87 92.88 74.13 92.88 86.63 105.4L150.6 169.4C151.3 170 151.9 170.7 152.5 171.4C166.8 164.1 182.9 160 199.1 160H312C329.1 160 345.2 164.1 359.5 171.4C360.1 170.7 360.7 170 361.4 169.4L425.4 105.4C437.9 92.88 458.1 92.88 470.6 105.4C483.1 117.9 483.1 138.1 470.6 150.6L406.6 214.6C405.1 215.3 405.3 215.9 404.6 216.5C410.7 228.5 414.6 241.9 415.7 256H480C497.7 256 512 270.3 512 288C512 305.7 497.7 320 480 320H416C416 344.6 410.5 367.8 400.6 388.6C402.7 389.9 404.8 391.5 406.6 393.4L470.6 457.4C483.1 469.9 483.1 490.1 470.6 502.6C458.1 515.1 437.9 515.1 425.4 502.6L362.3 439.6C337.8 461.4 306.5 475.8 272 479.2V240C272 231.2 264.8 224 255.1 224C247.2 224 239.1 231.2 239.1 240V479.2C205.5 475.8 174.2 461.4 149.7 439.6L86.63 502.6C74.13 515.1 53.87 515.1 41.37 502.6C28.88 490.1 28.88 469.9 41.37 457.4L105.4 393.4C107.2 391.5 109.3 389.9 111.4 388.6C101.5 367.8 96 344.6 96 320H32C14.33 320 0 305.7 0 288C0 270.3 14.33 256 32 256H96.3C97.38 241.9 101.3 228.5 107.4 216.5C106.7 215.9 106 215.3 105.4 214.6L41.37 150.6C28.88 138.1 28.88 117.9 41.37 105.4H41.37z"]},vh={prefix:"fas",iconName:"circle-half-stroke",icon:[512,512,[9680,"adjust"],"f042","M512 256C512 397.4 397.4 512 256 512C114.6 512 0 397.4 0 256C0 114.6 114.6 0 256 0C397.4 0 512 114.6 512 256zM256 64V448C362 448 448 362 448 256C448 149.1 362 64 256 64z"]},bh={prefix:"fas",iconName:"expand",icon:[448,512,[],"f065","M128 32H32C14.31 32 0 46.31 0 64v96c0 17.69 14.31 32 32 32s32-14.31 32-32V96h64c17.69 0 32-14.31 32-32S145.7 32 128 32zM416 32h-96c-17.69 0-32 14.31-32 32s14.31 32 32 32h64v64c0 17.69 14.31 32 32 32s32-14.31 32-32V64C448 46.31 433.7 32 416 32zM128 416H64v-64c0-17.69-14.31-32-32-32s-32 14.31-32 32v96c0 17.69 14.31 32 32 32h96c17.69 0 32-14.31 32-32S145.7 416 128 416zM416 320c-17.69 0-32 14.31-32 32v64h-64c-17.69 0-32 14.31-32 32s14.31 32 32 32h96c17.69 0 32-14.31 32-32v-96C448 334.3 433.7 320 416 320z"]},Eh={prefix:"fas",iconName:"gear",icon:[512,512,[9881,"cog"],"f013","M495.9 166.6C499.2 175.2 496.4 184.9 489.6 191.2L446.3 230.6C447.4 238.9 448 247.4 448 256C448 264.6 447.4 273.1 446.3 281.4L489.6 320.8C496.4 327.1 499.2 336.8 495.9 345.4C491.5 357.3 486.2 368.8 480.2 379.7L475.5 387.8C468.9 398.8 461.5 409.2 453.4 419.1C447.4 426.2 437.7 428.7 428.9 425.9L373.2 408.1C359.8 418.4 344.1 427 329.2 433.6L316.7 490.7C314.7 499.7 307.7 506.1 298.5 508.5C284.7 510.8 270.5 512 255.1 512C241.5 512 227.3 510.8 213.5 508.5C204.3 506.1 197.3 499.7 195.3 490.7L182.8 433.6C167 427 152.2 418.4 138.8 408.1L83.14 425.9C74.3 428.7 64.55 426.2 58.63 419.1C50.52 409.2 43.12 398.8 36.52 387.8L31.84 379.7C25.77 368.8 20.49 357.3 16.06 345.4C12.82 336.8 15.55 327.1 22.41 320.8L65.67 281.4C64.57 273.1 64 264.6 64 256C64 247.4 64.57 238.9 65.67 230.6L22.41 191.2C15.55 184.9 12.82 175.3 16.06 166.6C20.49 154.7 25.78 143.2 31.84 132.3L36.51 124.2C43.12 113.2 50.52 102.8 58.63 92.95C64.55 85.8 74.3 83.32 83.14 86.14L138.8 103.9C152.2 93.56 167 84.96 182.8 78.43L195.3 21.33C197.3 12.25 204.3 5.04 213.5 3.51C227.3 1.201 241.5 0 256 0C270.5 0 284.7 1.201 298.5 3.51C307.7 5.04 314.7 12.25 316.7 21.33L329.2 78.43C344.1 84.96 359.8 93.56 373.2 103.9L428.9 86.14C437.7 83.32 447.4 85.8 453.4 92.95C461.5 102.8 468.9 113.2 475.5 124.2L480.2 132.3C486.2 143.2 491.5 154.7 495.9 166.6V166.6zM256 336C300.2 336 336 300.2 336 255.1C336 211.8 300.2 175.1 256 175.1C211.8 175.1 176 211.8 176 255.1C176 300.2 211.8 336 256 336z"]},Th={prefix:"fas",iconName:"moon",icon:[512,512,[127769,9214],"f186","M32 256c0-123.8 100.3-224 223.8-224c11.36 0 29.7 1.668 40.9 3.746c9.616 1.777 11.75 14.63 3.279 19.44C245 86.5 211.2 144.6 211.2 207.8c0 109.7 99.71 193 208.3 172.3c9.561-1.805 16.28 9.324 10.11 16.95C387.9 448.6 324.8 480 255.8 480C132.1 480 32 379.6 32 256z"]},Sh={prefix:"fas",iconName:"share",icon:[512,512,["arrow-turn-right","mail-forward"],"f064","M503.7 226.2l-176 151.1c-15.38 13.3-39.69 2.545-39.69-18.16V272.1C132.9 274.3 66.06 312.8 111.4 457.8c5.031 16.09-14.41 28.56-28.06 18.62C39.59 444.6 0 383.8 0 322.3c0-152.2 127.4-184.4 288-186.3V56.02c0-20.67 24.28-31.46 39.69-18.16l176 151.1C514.8 199.4 514.8 216.6 503.7 226.2z"]},wh={prefix:"fas",iconName:"shield-halved",icon:[512,512,["shield-alt"],"f3ed","M256-.0078C260.7-.0081 265.2 1.008 269.4 2.913L457.7 82.79C479.7 92.12 496.2 113.8 496 139.1C495.5 239.2 454.7 420.7 282.4 503.2C265.7 511.1 246.3 511.1 229.6 503.2C57.25 420.7 16.49 239.2 15.1 139.1C15.87 113.8 32.32 92.12 54.3 82.79L242.7 2.913C246.8 1.008 251.4-.0081 256-.0078V-.0078zM256 444.8C393.1 378 431.1 230.1 432 141.4L256 66.77L256 444.8z"]},Nh={prefix:"fas",iconName:"sun",icon:[512,512,[9728],"f185","M256 159.1c-53.02 0-95.1 42.98-95.1 95.1S202.1 351.1 256 351.1s95.1-42.98 95.1-95.1S309 159.1 256 159.1zM509.3 347L446.1 255.1l63.15-91.01c6.332-9.125 1.104-21.74-9.826-23.72l-109-19.7l-19.7-109c-1.975-10.93-14.59-16.16-23.72-9.824L256 65.89L164.1 2.736c-9.125-6.332-21.74-1.107-23.72 9.824L121.6 121.6L12.56 141.3C1.633 143.2-3.596 155.9 2.736 164.1L65.89 256l-63.15 91.01c-6.332 9.125-1.105 21.74 9.824 23.72l109 19.7l19.7 109c1.975 10.93 14.59 16.16 23.72 9.824L256 446.1l91.01 63.15c9.127 6.334 21.75 1.107 23.72-9.822l19.7-109l109-19.7C510.4 368.8 515.6 356.1 509.3 347zM256 383.1c-70.69 0-127.1-57.31-127.1-127.1c0-70.69 57.31-127.1 127.1-127.1s127.1 57.3 127.1 127.1C383.1 326.7 326.7 383.1 256 383.1z"]},Rh={prefix:"fas",iconName:"up-right-from-square",icon:[512,512,["external-link-alt"],"f35d","M384 320c-17.67 0-32 14.33-32 32v96H64V160h96c17.67 0 32-14.32 32-32s-14.33-32-32-32L64 96c-35.35 0-64 28.65-64 64V448c0 35.34 28.65 64 64 64h288c35.35 0 64-28.66 64-64v-96C416 334.3 401.7 320 384 320zM488 0H352c-12.94 0-24.62 7.797-29.56 19.75c-4.969 11.97-2.219 25.72 6.938 34.88L370.8 96L169.4 297.4c-12.5 12.5-12.5 32.75 0 45.25C175.6 348.9 183.8 352 192 352s16.38-3.125 22.62-9.375L416 141.3l41.38 41.38c9.156 9.141 22.88 11.84 34.88 6.938C504.2 184.6 512 172.9 512 160V24C512 10.74 501.3 0 488 0z"]};function Oh(e){var t=e.url,n=e.openText;return c.createElement("div",null,c.createElement("p",{className:"text-sm mb-1"},e.helpText),c.createElement("div",{className:"flex gap-3"},c.createElement("a",{href:t,target:"_blank",className:"underline ~text-violet-500 hover:~text-violet-600"},c.createElement(ph,{icon:Rh,className:"opacity-50 text-xs mr-1"}),n),c.createElement(zo,{alwaysVisible:!0,direction:"left",value:t})))}function Ch(e){var t=[];return e.includes("stackTrace")&&t.push("stackTraceTab"),e.includes("context")&&t.push("requestTab","appTab","userTab","contextTab"),e.includes("debug")&&t.push("debugTab"),t}function xh(e,t){return t.includes("stackTrace")||(e.stacktrace=e.stacktrace.slice(0,1)),t.includes("debug")||(e.glows=[],e.context.dumps=[],e.context.queries=[],e.context.logs=[]),t.includes("context")||(e.context.request_data={queryString:{},body:{},files:[]},e.context.headers={},e.context.cookies={},e.context.session={},e.context.route=null,e.context.user=null,delete e.context.git,delete e.context.livewire,e.context.view=null),e}function kh(t){var n=t.isOpen,r=c.useContext(ld),a=c.useState(null),o=a[0],i=a[1],l=c.useState(null),s=l[0],u=l[1],f=c.useState(!1),d=f[0],p=f[1],m=c.useState([{name:"stackTrace",label:"Stack",selected:!0},{name:"context",label:"Context",selected:!0},{name:"debug",label:"Debug",selected:!0}]),h=m[0],g=m[1];return c.createElement("div",{className:"block absolute mt-2 top-10 right-1/2 transform translate-x-8 transition-all duration-150 origin-top-right\n "+(n?"":"opacity-0 pointer-events-none scale-90")},c.createElement("div",{className:"flex px-4 justify-end"},c.createElement("div",{className:"w-0 h-0 border-[10px] border-t-0 border-transparent ~border-b-dropdown"})),c.createElement("div",{className:"flex flex-col gap-6 ~bg-dropdown px-10 py-8 shadow-2xl"},c.createElement("div",{className:"flex items-center justify-between gap-6"},c.createElement("h4",{className:"whitespace-nowrap font-semibold"},"Share with Flare"),c.createElement("a",{className:"text-xs ~text-gray-500 hover:text-violet-500 flex items-center underline transition-colors",href:"https://flareapp.io/docs/ignition/introducing-ignition/sharing-errors?utm_campaign=ignition&utm_source=ignition",target:"_blank"},"Docs",c.createElement(ad,null))),!o&&c.createElement(c.Fragment,null,c.createElement("ul",{className:"grid justify-start gap-3"},h.map(function(t){var n=t.name;return c.createElement("li",{key:n},c.createElement(id,{onChange:function(){return t=n,void(h.find(function(e){return e.name===t})&&g(h.map(function(n){return n.name===t?e({},n,{selected:!n.selected}):n})));var t},checked:t.selected,label:t.label}))})),c.createElement("div",{className:"flex items-center gap-4"},c.createElement(Uc,{disabled:d||!h.some(function(e){return e.selected}),className:"bg-violet-500 border-violet-500/25 CopyButton text-white",onClick:function(){try{var e=function(){p(!1)};if(!r.config.shareEndpoint)return Promise.resolve();u(null),p(!0);var t=h.filter(function(e){return e.selected}).map(function(e){return e.name}),n=function(e,n){try{var a=Promise.resolve(function(e,t){var n={tabs:Ch(t),lineSelection:window.location.hash,report:xh(e.shareableReport,t)};return new Promise(function(t,r){try{var a=function(){r()},o=function(r,a){try{var o=Promise.resolve(fetch(e.config.shareEndpoint,{method:"POST",body:JSON.stringify(n),headers:{"Content-Type":"application/json",Accept:"application/json"}})).then(function(e){return Promise.resolve(e.json()).then(function(e){e&&e.owner_url&&e.public_url&&t(e)})})}catch(e){return a(e)}return o&&o.then?o.then(void 0,a):o}(0,function(e){r(e)});return Promise.resolve(o&&o.then?o.then(a):a())}catch(e){return Promise.reject(e)}})}(r,t)).then(function(e){window.open(e.owner_url),i(e.public_url)})}catch(e){return n(e)}return a&&a.then?a.then(void 0,n):a}(0,function(e){console.error(e),u("Something went wrong while sharing, please try again.")});return Promise.resolve(n&&n.then?n.then(e):e())}catch(e){return Promise.reject(e)}}},c.createElement(ph,{icon:Rh,className:"opacity-50 text-xs mr-1"}),"Create Share"))),o&&c.createElement("div",{className:"grid grid-cols-1 gap-4"},c.createElement(Oh,{url:o,helpText:"Share your error with others",openText:"Visit public share"})),s&&c.createElement("p",{className:"text-red-500"},s)))}function Ah(t){var n,r=t.isOpen,a=c.useContext(ld),o=c.useContext(Te),i=o.ignitionConfig,l=o.setIgnitionConfig,s=c.useState(i.editor||""),u=s[0],f=s[1],d=c.useState(null),p=d[0],m=d[1],h=c.useState(!1),g=h[0],y=h[1],v=c.useState(!1),b=v[0],E=v[1],T=c.useState([{value:"light",icon:c.createElement(ph,{icon:Nh,className:"group-hover:text-amber-400"}),selected:"light"===i.theme},{value:"dark",icon:c.createElement(ph,{icon:Th,className:"group-hover:text-amber-300"}),selected:"dark"===i.theme},{value:"auto",icon:c.createElement(ph,{icon:vh,className:"group-hover:text-indigo-500"}),selected:"auto"===i.theme}]),S=T[0],w=T[1];return c.createElement("div",{className:"\n absolute mt-2 top-10 right-1/2 translate-x-6 transition-all duration-150 origin-top-right\n "+(r?"":"opacity-0 pointer-events-none scale-90")+"\n "},c.createElement("div",{className:"flex px-4 justify-end"},c.createElement("div",{className:"w-0 h-0 border-[10px] border-t-0 border-transparent ~border-b-dropdown"})),c.createElement("div",{className:"flex flex-col gap-6 ~bg-dropdown px-10 py-8 shadow-2xl"},c.createElement("div",{className:"flex items-center justify-between gap-6"},c.createElement("h4",{className:"whitespace-nowrap font-semibold"},"Ignition Settings"),c.createElement("a",{className:"text-xs ~text-gray-500 hover:text-red-500 flex items-center underline transition-colors",href:"https://flareapp.io/ignition?utm_campaign=ignition&utm_source=ignition",target:"_blank"},"Docs",c.createElement(od,null))),c.createElement("label",{htmlFor:"editor-select"},c.createElement("span",{className:"uppercase tracking-wider ~text-gray-500 text-xs font-bold"},"Editor"),c.createElement("div",{className:"group mt-2"},c.createElement("select",{id:"editor-select",className:"block appearance-none w-full ~bg-gray-500/5 h-12 px-4 pr-8 rounded-none leading-tight",value:u,onChange:function(t){return function(t){f(t),l(e({},i,{editor:t}))}(t.target.value)}},Object.entries((null==i?void 0:i.editorOptions)||[]).map(function(e){var t=e[0];return c.createElement("option",{className:"text-gray-800",key:t,value:t},e[1].label)})),c.createElement("div",{className:"pointer-events-none absolute inset-y-0 right-0 flex items-center px-4"},c.createElement(ph,{icon:gh,className:"group-hover:text-indigo-500 text-sm"})))),c.createElement("div",null,c.createElement("span",{className:"uppercase tracking-wider ~text-gray-500 text-xs font-bold"},"Theme"),c.createElement("button",{className:"mt-2 w-full ~bg-gray-500/5 rounded-none leading-tight",onClick:function(){var t=S.findIndex(function(e){return e.selected}),n=-1===t||t===S.length-1?0:t+1;m(S[t].value),w([].concat(S.map(function(e,t){return e.selected=t===n,e}))),l(e({},i,{theme:S[n].value}))}},c.createElement("div",{className:"group flex items-center",style:{WebkitMaskImage:"linear-gradient(to bottom, rgba(0,0,0,1) 60%, rgba(0,0,0,0) 100%)"}},c.createElement("div",{className:"px-4"},S.map(function(e){var t=e.value,n=e.selected;return c.createElement("div",{key:t,className:"\n h-12 flex items-center origin-bottom\n "+(n?"transition-transform duration-1000":"")+"\n "+(t===p?"transition-transform duration-1000 absolute top-0 left-4 rotate-180":"")+"\n "+(n||t===p?"":"absolute top-0 left-4 -rotate-180")+"\n "},c.createElement("span",{className:"text-sm ~text-gray-500 transition-colors duration-500"},e.icon))})),c.createElement("div",{id:"theme-name",className:"-ml-1 first-letter:uppercase"},null==(n=S.find(function(e){return e.selected}))?void 0:n.value)))),c.createElement("div",{className:"flex items-center gap-4"},c.createElement(Uc,{onClick:function(){try{return g?Promise.resolve():Promise.resolve(function(e,t){try{var n=function(e,t){try{var n=function(){var e;if(E(!1),y(!0),a.updateConfigEndpoint)return Promise.resolve(fetch(a.updateConfigEndpoint,{method:"POST",headers:{"Content-Type":"application/json",Accept:"application/json"},body:JSON.stringify({editor:u,theme:null==(e=S.find(function(e){return e.selected}))?void 0:e.value,hide_solutions:!1})})).then(function(e){E(e.status>=200&&e.status<300),setTimeout(function(){E(!1)},3e3)})}()}catch(e){return t(e)}return n&&n.then?n.then(void 0,t):n}(0,function(e){console.error(e),E(!1)})}catch(e){return t(!0,e)}return n&&n.then?n.then(t.bind(null,!1),t.bind(null,!0)):t(!1,n)}(0,function(e,t){if(y(!1),e)throw t;return t}))}catch(e){return Promise.reject(e)}},disabled:g,className:"bg-red-500 border-red-500/25 text-white"},"Save settings"),b&&c.createElement("p",{className:"text-emerald-500 text-sm"},"Saved!")),c.createElement("p",{className:"text-xs"},"Settings will be saved locally in ",c.createElement(rd,null,"~/.ignition.json"),".")))}var Ih={prefix:"fab",iconName:"laravel",icon:[512,512,[],"f3bd","M504.4 115.8a5.72 5.72 0 0 0 -.28-.68 8.52 8.52 0 0 0 -.53-1.25 6 6 0 0 0 -.54-.71 9.36 9.36 0 0 0 -.72-.94c-.23-.22-.52-.4-.77-.6a8.84 8.84 0 0 0 -.9-.68L404.4 55.55a8 8 0 0 0 -8 0L300.1 111h0a8.07 8.07 0 0 0 -.88 .69 7.68 7.68 0 0 0 -.78 .6 8.23 8.23 0 0 0 -.72 .93c-.17 .24-.39 .45-.54 .71a9.7 9.7 0 0 0 -.52 1.25c-.08 .23-.21 .44-.28 .68a8.08 8.08 0 0 0 -.28 2.08V223.2l-80.22 46.19V63.44a7.8 7.8 0 0 0 -.28-2.09c-.06-.24-.2-.45-.28-.68a8.35 8.35 0 0 0 -.52-1.24c-.14-.26-.37-.47-.54-.72a9.36 9.36 0 0 0 -.72-.94 9.46 9.46 0 0 0 -.78-.6 9.8 9.8 0 0 0 -.88-.68h0L115.6 1.07a8 8 0 0 0 -8 0L11.34 56.49h0a6.52 6.52 0 0 0 -.88 .69 7.81 7.81 0 0 0 -.79 .6 8.15 8.15 0 0 0 -.71 .93c-.18 .25-.4 .46-.55 .72a7.88 7.88 0 0 0 -.51 1.24 6.46 6.46 0 0 0 -.29 .67 8.18 8.18 0 0 0 -.28 2.1v329.7a8 8 0 0 0 4 6.95l192.5 110.8a8.83 8.83 0 0 0 1.33 .54c.21 .08 .41 .2 .63 .26a7.92 7.92 0 0 0 4.1 0c.2-.05 .37-.16 .55-.22a8.6 8.6 0 0 0 1.4-.58L404.4 400.1a8 8 0 0 0 4-6.95V287.9l92.24-53.11a8 8 0 0 0 4-7V117.9A8.63 8.63 0 0 0 504.4 115.8zM111.6 17.28h0l80.19 46.15-80.2 46.18L31.41 63.44zm88.25 60V278.6l-46.53 26.79-33.69 19.4V123.5l46.53-26.79zm0 412.8L23.37 388.5V77.32L57.06 96.7l46.52 26.8V338.7a6.94 6.94 0 0 0 .12 .9 8 8 0 0 0 .16 1.18h0a5.92 5.92 0 0 0 .38 .9 6.38 6.38 0 0 0 .42 1v0a8.54 8.54 0 0 0 .6 .78 7.62 7.62 0 0 0 .66 .84l0 0c.23 .22 .52 .38 .77 .58a8.93 8.93 0 0 0 .86 .66l0 0 0 0 92.19 52.18zm8-106.2-80.06-45.32 84.09-48.41 92.26-53.11 80.13 46.13-58.8 33.56zm184.5 4.57L215.9 490.1V397.8L346.6 323.2l45.77-26.15zm0-119.1L358.7 250l-46.53-26.79V131.8l33.69 19.4L392.4 178zm8-105.3-80.2-46.17 80.2-46.16 80.18 46.15zm8 105.3V178L455 151.2l33.68-19.4v91.39h0z"]},Lh={prefix:"fab",iconName:"php",icon:[640,512,[],"f457","M320 104.5c171.4 0 303.2 72.2 303.2 151.5S491.3 407.5 320 407.5c-171.4 0-303.2-72.2-303.2-151.5S148.7 104.5 320 104.5m0-16.8C143.3 87.7 0 163 0 256s143.3 168.3 320 168.3S640 349 640 256 496.7 87.7 320 87.7zM218.2 242.5c-7.9 40.5-35.8 36.3-70.1 36.3l13.7-70.6c38 0 63.8-4.1 56.4 34.3zM97.4 350.3h36.7l8.7-44.8c41.1 0 66.6 3 90.2-19.1 26.1-24 32.9-66.7 14.3-88.1-9.7-11.2-25.3-16.7-46.5-16.7h-70.7L97.4 350.3zm185.7-213.6h36.5l-8.7 44.8c31.5 0 60.7-2.3 74.8 10.7 14.8 13.6 7.7 31-8.3 113.1h-37c15.4-79.4 18.3-86 12.7-92-5.4-5.8-17.7-4.6-47.4-4.6l-18.8 96.6h-36.5l32.7-168.6zM505 242.5c-8 41.1-36.7 36.3-70.1 36.3l13.7-70.6c38.2 0 63.8-4.1 56.4 34.3zM384.2 350.3H421l8.7-44.8c43.2 0 67.1 2.5 90.2-19.1 26.1-24 32.9-66.7 14.3-88.1-9.7-11.2-25.3-16.7-46.5-16.7H417l-32.8 168.7z"]},_h="object"==typeof t&&t&&t.Object===Object&&t,Ph="object"==typeof self&&self&&self.Object===Object&&self,Mh=_h||Ph||Function("return this")(),Dh=Mh.Symbol,Uh=Object.prototype,jh=Uh.hasOwnProperty,Fh=Uh.toString,zh=Dh?Dh.toStringTag:void 0,Bh=Object.prototype.toString,Hh=Dh?Dh.toStringTag:void 0,Vh=function(e){return null==e?void 0===e?"[object Undefined]":"[object Null]":Hh&&Hh in Object(e)?function(e){var t=jh.call(e,zh),n=e[zh];try{e[zh]=void 0;var r=!0}catch(e){}var a=Fh.call(e);return r&&(t?e[zh]=n:delete e[zh]),a}(e):function(e){return Bh.call(e)}(e)},Wh=function(e){var t=typeof e;return null!=e&&("object"==t||"function"==t)},Gh=function(e){if(!Wh(e))return!1;var t=Vh(e);return"[object Function]"==t||"[object GeneratorFunction]"==t||"[object AsyncFunction]"==t||"[object Proxy]"==t},Yh=Mh["__core-js_shared__"],$h=function(){var e=/[^.]+$/.exec(Yh&&Yh.keys&&Yh.keys.IE_PROTO||"");return e?"Symbol(src)_1."+e:""}(),Xh=Function.prototype.toString,qh=function(e){if(null!=e){try{return Xh.call(e)}catch(e){}try{return e+""}catch(e){}}return""},Kh=/^\[object .+?Constructor\]$/,Jh=RegExp("^"+Function.prototype.toString.call(Object.prototype.hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g,"\\$&").replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g,"$1.*?")+"$"),Qh=function(e,t){var n=function(e,t){return null==e?void 0:e[t]}(e,t);return function(e){return!(!Wh(e)||function(e){return!!$h&&$h in e}(e))&&(Gh(e)?Jh:Kh).test(qh(e))}(n)?n:void 0},Zh=function(){try{var e=Qh(Object,"defineProperty");return e({},"",{}),e}catch(e){}}(),eg=function(e,t,n){"__proto__"==t&&Zh?Zh(e,t,{configurable:!0,enumerable:!0,value:n,writable:!0}):e[t]=n},tg=function(e){return null!=e&&"object"==typeof e},ng=function(e){return tg(e)&&"[object Arguments]"==Vh(e)},rg=Object.prototype,ag=rg.hasOwnProperty,og=rg.propertyIsEnumerable,ig=ng(function(){return arguments}())?ng:function(e){return tg(e)&&ag.call(e,"callee")&&!og.call(e,"callee")},lg=Array.isArray,sg=function(){return!1},cg=n(function(e,t){var n=t&&!t.nodeType&&t,r=n&&e&&!e.nodeType&&e,a=r&&r.exports===n?Mh.Buffer:void 0;e.exports=(a?a.isBuffer:void 0)||sg}),ug=/^(?:0|[1-9]\d*)$/,fg=function(e,t){var n=typeof e;return!!(t=null==t?9007199254740991:t)&&("number"==n||"symbol"!=n&&ug.test(e))&&e>-1&&e%1==0&&e<t},dg=function(e){return"number"==typeof e&&e>-1&&e%1==0&&e<=9007199254740991},pg={};pg["[object Float32Array]"]=pg["[object Float64Array]"]=pg["[object Int8Array]"]=pg["[object Int16Array]"]=pg["[object Int32Array]"]=pg["[object Uint8Array]"]=pg["[object Uint8ClampedArray]"]=pg["[object Uint16Array]"]=pg["[object Uint32Array]"]=!0,pg["[object Arguments]"]=pg["[object Array]"]=pg["[object ArrayBuffer]"]=pg["[object Boolean]"]=pg["[object DataView]"]=pg["[object Date]"]=pg["[object Error]"]=pg["[object Function]"]=pg["[object Map]"]=pg["[object Number]"]=pg["[object Object]"]=pg["[object RegExp]"]=pg["[object Set]"]=pg["[object String]"]=pg["[object WeakMap]"]=!1;var mg=n(function(e,t){var n=t&&!t.nodeType&&t,r=n&&e&&!e.nodeType&&e,a=r&&r.exports===n&&_h.process,o=function(){try{return r&&r.require&&r.require("util").types||a&&a.binding&&a.binding("util")}catch(e){}}();e.exports=o}),hg=mg&&mg.isTypedArray,gg=hg?function(e){return function(t){return e(t)}}(hg):function(e){return tg(e)&&dg(e.length)&&!!pg[Vh(e)]},yg=Object.prototype.hasOwnProperty,vg=Object.prototype,bg=function(e,t){return function(n){return e(t(n))}}(Object.keys,Object),Eg=Object.prototype.hasOwnProperty,Tg=function(e){return null!=e&&dg(e.length)&&!Gh(e)},Sg=function(e){return Tg(e)?function(e,t){var n=lg(e),r=!n&&ig(e),a=!n&&!r&&cg(e),o=!n&&!r&&!a&&gg(e),i=n||r||a||o,l=i?function(e,t){for(var n=-1,r=Array(e);++n<e;)r[n]=t(n);return r}(e.length,String):[],s=l.length;for(var c in e)!yg.call(e,c)||i&&("length"==c||a&&("offset"==c||"parent"==c)||o&&("buffer"==c||"byteLength"==c||"byteOffset"==c)||fg(c,s))||l.push(c);return l}(e):function(e){if((t=e)!==("function"==typeof(n=t&&t.constructor)&&n.prototype||vg))return bg(e);var t,n,r=[];for(var a in Object(e))Eg.call(e,a)&&"constructor"!=a&&r.push(a);return r}(e)},wg=function(e,t){return e&&function(e,t,n){for(var r=-1,a=Object(e),o=n(e),i=o.length;i--;){var l=o[++r];if(!1===t(a[l],l,a))break}return e}(e,t,Sg)},Ng=function(e,t){return e===t||e!=e&&t!=t},Rg=function(e,t){for(var n=e.length;n--;)if(Ng(e[n][0],t))return n;return-1},Og=Array.prototype.splice;function Cg(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}Cg.prototype.clear=function(){this.__data__=[],this.size=0},Cg.prototype.delete=function(e){var t=this.__data__,n=Rg(t,e);return!(n<0||(n==t.length-1?t.pop():Og.call(t,n,1),--this.size,0))},Cg.prototype.get=function(e){var t=this.__data__,n=Rg(t,e);return n<0?void 0:t[n][1]},Cg.prototype.has=function(e){return Rg(this.__data__,e)>-1},Cg.prototype.set=function(e,t){var n=this.__data__,r=Rg(n,e);return r<0?(++this.size,n.push([e,t])):n[r][1]=t,this};var xg=Cg,kg=Qh(Mh,"Map"),Ag=Qh(Object,"create"),Ig=Object.prototype.hasOwnProperty,Lg=Object.prototype.hasOwnProperty;function _g(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}_g.prototype.clear=function(){this.__data__=Ag?Ag(null):{},this.size=0},_g.prototype.delete=function(e){var t=this.has(e)&&delete this.__data__[e];return this.size-=t?1:0,t},_g.prototype.get=function(e){var t=this.__data__;if(Ag){var n=t[e];return"__lodash_hash_undefined__"===n?void 0:n}return Ig.call(t,e)?t[e]:void 0},_g.prototype.has=function(e){var t=this.__data__;return Ag?void 0!==t[e]:Lg.call(t,e)},_g.prototype.set=function(e,t){var n=this.__data__;return this.size+=this.has(e)?0:1,n[e]=Ag&&void 0===t?"__lodash_hash_undefined__":t,this};var Pg=_g,Mg=function(e,t){var n,r,a=e.__data__;return("string"==(r=typeof(n=t))||"number"==r||"symbol"==r||"boolean"==r?"__proto__"!==n:null===n)?a["string"==typeof t?"string":"hash"]:a.map};function Dg(e){var t=-1,n=null==e?0:e.length;for(this.clear();++t<n;){var r=e[t];this.set(r[0],r[1])}}Dg.prototype.clear=function(){this.size=0,this.__data__={hash:new Pg,map:new(kg||xg),string:new Pg}},Dg.prototype.delete=function(e){var t=Mg(this,e).delete(e);return this.size-=t?1:0,t},Dg.prototype.get=function(e){return Mg(this,e).get(e)},Dg.prototype.has=function(e){return Mg(this,e).has(e)},Dg.prototype.set=function(e,t){var n=Mg(this,e),r=n.size;return n.set(e,t),this.size+=n.size==r?0:1,this};var Ug=Dg;function jg(e){var t=this.__data__=new xg(e);this.size=t.size}jg.prototype.clear=function(){this.__data__=new xg,this.size=0},jg.prototype.delete=function(e){var t=this.__data__,n=t.delete(e);return this.size=t.size,n},jg.prototype.get=function(e){return this.__data__.get(e)},jg.prototype.has=function(e){return this.__data__.has(e)},jg.prototype.set=function(e,t){var n=this.__data__;if(n instanceof xg){var r=n.__data__;if(!kg||r.length<199)return r.push([e,t]),this.size=++n.size,this;n=this.__data__=new Ug(r)}return n.set(e,t),this.size=n.size,this};var Fg=jg;function zg(e){var t=-1,n=null==e?0:e.length;for(this.__data__=new Ug;++t<n;)this.add(e[t])}zg.prototype.add=zg.prototype.push=function(e){return this.__data__.set(e,"__lodash_hash_undefined__"),this},zg.prototype.has=function(e){return this.__data__.has(e)};var Bg=zg,Hg=function(e,t){for(var n=-1,r=null==e?0:e.length;++n<r;)if(t(e[n],n,e))return!0;return!1},Vg=function(e,t){return e.has(t)},Wg=function(e,t,n,r,a,o){var i=1&n,l=e.length,s=t.length;if(l!=s&&!(i&&s>l))return!1;var c=o.get(e),u=o.get(t);if(c&&u)return c==t&&u==e;var f=-1,d=!0,p=2&n?new Bg:void 0;for(o.set(e,t),o.set(t,e);++f<l;){var m=e[f],h=t[f];if(r)var g=i?r(h,m,f,t,e,o):r(m,h,f,e,t,o);if(void 0!==g){if(g)continue;d=!1;break}if(p){if(!Hg(t,function(e,t){if(!Vg(p,t)&&(m===e||a(m,e,n,r,o)))return p.push(t)})){d=!1;break}}else if(m!==h&&!a(m,h,n,r,o)){d=!1;break}}return o.delete(e),o.delete(t),d},Gg=Mh.Uint8Array,Yg=function(e){var t=-1,n=Array(e.size);return e.forEach(function(e,r){n[++t]=[r,e]}),n},$g=function(e){var t=-1,n=Array(e.size);return e.forEach(function(e){n[++t]=e}),n},Xg=Dh?Dh.prototype:void 0,qg=Xg?Xg.valueOf:void 0,Kg=Object.prototype.propertyIsEnumerable,Jg=Object.getOwnPropertySymbols,Qg=Jg?function(e){return null==e?[]:(e=Object(e),function(t,n){for(var r=-1,a=null==t?0:t.length,o=0,i=[];++r<a;){var l=t[r];Kg.call(e,l)&&(i[o++]=l)}return i}(Jg(e)))}:function(){return[]},Zg=function(e){return function(e,t,n){var r=Sg(e);return lg(e)?r:function(e,t){for(var n=-1,r=t.length,a=e.length;++n<r;)e[a+n]=t[n];return e}(r,n(e))}(e,0,Qg)},ey=Object.prototype.hasOwnProperty,ty=Qh(Mh,"DataView"),ny=Qh(Mh,"Promise"),ry=Qh(Mh,"Set"),ay=Qh(Mh,"WeakMap"),oy=qh(ty),iy=qh(kg),ly=qh(ny),sy=qh(ry),cy=qh(ay),uy=Vh;(ty&&"[object DataView]"!=uy(new ty(new ArrayBuffer(1)))||kg&&"[object Map]"!=uy(new kg)||ny&&"[object Promise]"!=uy(ny.resolve())||ry&&"[object Set]"!=uy(new ry)||ay&&"[object WeakMap]"!=uy(new ay))&&(uy=function(e){var t=Vh(e),n="[object Object]"==t?e.constructor:void 0,r=n?qh(n):"";if(r)switch(r){case oy:return"[object DataView]";case iy:return"[object Map]";case ly:return"[object Promise]";case sy:return"[object Set]";case cy:return"[object WeakMap]"}return t});var fy=uy,dy="[object Object]",py=Object.prototype.hasOwnProperty,my=function e(t,n,r,a,o){return t===n||(null==t||null==n||!tg(t)&&!tg(n)?t!=t&&n!=n:function(e,t,n,r,a,o){var i=lg(e),l=lg(t),s=i?"[object Array]":fy(e),c=l?"[object Array]":fy(t),u=(s="[object Arguments]"==s?dy:s)==dy,f=(c="[object Arguments]"==c?dy:c)==dy,d=s==c;if(d&&cg(e)){if(!cg(t))return!1;i=!0,u=!1}if(d&&!u)return o||(o=new Fg),i||gg(e)?Wg(e,t,n,r,a,o):function(e,t,n,r,a,o,i){switch(n){case"[object DataView]":if(e.byteLength!=t.byteLength||e.byteOffset!=t.byteOffset)return!1;e=e.buffer,t=t.buffer;case"[object ArrayBuffer]":return!(e.byteLength!=t.byteLength||!o(new Gg(e),new Gg(t)));case"[object Boolean]":case"[object Date]":case"[object Number]":return Ng(+e,+t);case"[object Error]":return e.name==t.name&&e.message==t.message;case"[object RegExp]":case"[object String]":return e==t+"";case"[object Map]":var l=Yg;case"[object Set]":if(l||(l=$g),e.size!=t.size&&!(1&r))return!1;var s=i.get(e);if(s)return s==t;r|=2,i.set(e,t);var c=Wg(l(e),l(t),r,a,o,i);return i.delete(e),c;case"[object Symbol]":if(qg)return qg.call(e)==qg.call(t)}return!1}(e,t,s,n,r,a,o);if(!(1&n)){var p=u&&py.call(e,"__wrapped__"),m=f&&py.call(t,"__wrapped__");if(p||m){var h=p?e.value():e,g=m?t.value():t;return o||(o=new Fg),a(h,g,n,r,o)}}return!!d&&(o||(o=new Fg),function(e,t,n,r,a,o){var i=1&n,l=Zg(e),s=l.length;if(s!=Zg(t).length&&!i)return!1;for(var c=s;c--;){var u=l[c];if(!(i?u in t:ey.call(t,u)))return!1}var f=o.get(e),d=o.get(t);if(f&&d)return f==t&&d==e;var p=!0;o.set(e,t),o.set(t,e);for(var m=i;++c<s;){var h=e[u=l[c]],g=t[u];if(r)var y=i?r(g,h,u,t,e,o):r(h,g,u,e,t,o);if(!(void 0===y?h===g||a(h,g,n,r,o):y)){p=!1;break}m||(m="constructor"==u)}if(p&&!m){var v=e.constructor,b=t.constructor;v==b||!("constructor"in e)||!("constructor"in t)||"function"==typeof v&&v instanceof v&&"function"==typeof b&&b instanceof b||(p=!1)}return o.delete(e),o.delete(t),p}(e,t,n,r,a,o))}(t,n,r,a,e,o))},hy=function(e){return e==e&&!Wh(e)},gy=function(e,t){return function(n){return null!=n&&n[e]===t&&(void 0!==t||e in Object(n))}},yy=function(e){return"symbol"==typeof e||tg(e)&&"[object Symbol]"==Vh(e)},vy=/\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,by=/^\w*$/,Ey=function(e,t){if(lg(e))return!1;var n=typeof e;return!("number"!=n&&"symbol"!=n&&"boolean"!=n&&null!=e&&!yy(e))||by.test(e)||!vy.test(e)||null!=t&&e in Object(t)};function Ty(e,t){if("function"!=typeof e||null!=t&&"function"!=typeof t)throw new TypeError("Expected a function");var n=function(){var r=arguments,a=t?t.apply(this,r):r[0],o=n.cache;if(o.has(a))return o.get(a);var i=e.apply(this,r);return n.cache=o.set(a,i)||o,i};return n.cache=new(Ty.Cache||Ug),n}Ty.Cache=Ug;var Sy,wy,Ny=Ty,Ry=/[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g,Oy=/\\(\\)?/g,Cy=function(e){var t=Ny(function(e){var t=[];return 46===e.charCodeAt(0)&&t.push(""),e.replace(Ry,function(e,n,r,a){t.push(r?a.replace(Oy,"$1"):n||e)}),t},function(e){return 500===n.size&&n.clear(),e}),n=t.cache;return t}(),xy=Dh?Dh.prototype:void 0,ky=xy?xy.toString:void 0,Ay=function e(t){if("string"==typeof t)return t;if(lg(t))return function(e,t){for(var n=-1,r=null==e?0:e.length,a=Array(r);++n<r;)a[n]=t(e[n],n,e);return a}(t,e)+"";if(yy(t))return ky?ky.call(t):"";var n=t+"";return"0"==n&&1/t==-Infinity?"-0":n},Iy=function(e,t){return lg(e)?e:Ey(e,t)?[e]:Cy(function(e){return null==e?"":Ay(e)}(e))},Ly=function(e){if("string"==typeof e||yy(e))return e;var t=e+"";return"0"==t&&1/e==-Infinity?"-0":t},_y=function(e,t){for(var n=0,r=(t=Iy(t,e)).length;null!=e&&n<r;)e=e[Ly(t[n++])];return n&&n==r?e:void 0},Py=function(e,t){return null!=e&&t in Object(e)},My=function(e){return e},Dy=function(e){return"function"==typeof e?e:null==e?My:"object"==typeof e?lg(e)?function(e,t){return Ey(e)&&hy(t)?gy(Ly(e),t):function(n){var r=function(e,t,n){var r=null==e?void 0:_y(e,t);return void 0===r?void 0:r}(n,e);return void 0===r&&r===t?function(e,t){return null!=e&&function(e,t,n){for(var r=-1,a=(t=Iy(t,e)).length,o=!1;++r<a;){var i=Ly(t[r]);if(!(o=null!=e&&n(e,i)))break;e=e[i]}return o||++r!=a?o:!!(a=null==e?0:e.length)&&dg(a)&&fg(i,a)&&(lg(e)||ig(e))}(e,t,Py)}(n,e):my(t,r,3)}}(e[0],e[1]):function(e){var t=function(e){for(var t=Sg(e),n=t.length;n--;){var r=t[n],a=e[r];t[n]=[r,a,hy(a)]}return t}(e);return 1==t.length&&t[0][2]?gy(t[0][0],t[0][1]):function(n){return n===e||function(e,t,n,r){var a=n.length,o=a;if(null==e)return!o;for(e=Object(e);a--;){var i=n[a];if(i[2]?i[1]!==e[i[0]]:!(i[0]in e))return!1}for(;++a<o;){var l=(i=n[a])[0],s=e[l],c=i[1];if(i[2]){if(void 0===s&&!(l in e))return!1}else{var u=new Fg;if(!my(c,s,3,void 0,u))return!1}}return!0}(n,0,t)}}(e):Ey(t=e)?(n=Ly(t),function(e){return null==e?void 0:e[n]}):function(e){return function(t){return _y(t,e)}}(t);var t,n},Uy=function(e,t){var n={};return t=Dy(t),wg(e,function(e,r,a){eg(n,r,t(e,r,a))}),n},jy=function(e,t,n,r){for(var a=-1,o=null==e?0:e.length;++a<o;){var i=e[a];t(r,i,n(i),e)}return r},Fy=(Sy=wg,function(e,t){if(null==e)return e;if(!Tg(e))return Sy(e,t);for(var n=e.length,r=-1,a=Object(e);++r<n&&!1!==t(a[r],r,a););return e}),zy=function(e,t,n,r){return Fy(e,function(e,a,o){t(r,e,n(e),o)}),r},By=(wy=function(e,t,n){eg(e,n,t)},function(e,t){return(lg(e)?jy:zy)(e,wy,Dy(t),{})});function Hy(e,t){c.useEffect(function(){function n(n){e.current&&!e.current.contains(n.target)&&t()}return document.addEventListener("click",n),function(){document.removeEventListener("click",n)}},[])}function Vy(e){var t,n=e.showException,r=c.useContext(be),a=c.useState(!1),o=a[0],i=a[1],l=c.useState(!1),s=l[0],u=l[1],f=function(e){var t=c.useState(window.pageYOffset),n=t[0],r=t[1];return c.useEffect(function(){var e=function(){r(window.pageYOffset)};return window.addEventListener("scroll",e),function(){return window.removeEventListener("scroll",e)}},[]),n>=10}(),d=c.useContext(Te).ignitionConfig,p=c.useRef(null),m=c.useRef(null);Hy(p,function(){return i(!1)}),Hy(m,function(){return u(!1)});var h=Uy(By(r.context_items.env||[],"name"),"value"),g="local"!==h.app_env&&h.app_debug,y=function(e){var t;if(null!=(t=e.context_items.env)&&t.laravel_version){var n=e.documentation_links.find(function(e){return e.startsWith("https://laravel.com/")});return n?{type:"laravel",url:n,tailored:!0}:{type:"laravel",url:"https://laravel.com/docs/",tailored:!1}}var r=e.documentation_links.find(function(e){return e.startsWith("https://php.net/")});return r?{type:"php",url:r,tailored:!0}:{type:"generic",url:"https://php.net/docs",tailored:!1}}(r);return c.createElement("nav",{className:"z-50 fixed top-0 h-20 w-full"},c.createElement("div",null,c.createElement("div",{className:"\n "+(f?"~bg-gray-100":"~bg-body")+"\n z-10 transform translate-x-0 transition-color duration-100\n "},c.createElement("div",{className:"h-10 flex justify-between px-6 lg:px-10 mx-auto max-w-4xl lg:max-w-[90rem]"},c.createElement("ul",{className:"-ml-3 sm:-ml-5 grid grid-flow-col justify-start items-center"},c.createElement(ve,{name:"stack",icon:c.createElement(ph,{icon:hh})}),c.createElement(ve,{name:"context",icon:c.createElement(ph,{icon:bh})}),Uo(r)&&c.createElement(ve,{name:"debug",icon:c.createElement(ph,{icon:yh}),important:!(null==(t=r.context_items.dumps)||!t.length)}),c.createElement(ve,{name:"flare",href:"https://flareapp.io/?utm_campaign=ignition&utm_source=ignition",icon:c.createElement("svg",{viewBox:"0 0 36 56",fill:"currentColor",className:"h-[.9rem] -top-[.1rem] inline-block"},c.createElement("path",{d:"M 11.995 55.987 L 0 48.993 L 0 35 L 11.967 41.994 L 11.995 55.987 Z"}),c.createElement("path",{d:"M 11.967 41.993 L 0 34.999 L 11.995 28 L 23.989 34.999 L 11.967 41.993 Z"}),c.createElement("path",{d:"M 11.995 27.987 L 0 20.987 L 0 7 L 12.062 14.022 L 11.995 27.987 Z"}),c.createElement("path",{d:"M 23.978 20.981 L 0 6.999 L 11.995 0 L 36 13.981 L 23.978 20.981 Z"}))})),c.createElement("ul",{className:"-mr-3 sm:-mr-5 grid grid-flow-col justify-end items-center"},d.enableShareButton&&c.createElement(ve,{navRef:p,name:"share",icon:c.createElement(ph,{icon:Sh}),onClick:function(){i(!o)}},c.createElement(kh,{isOpen:o})),y&&c.createElement(ve,{name:"docs",href:y.url,icon:c.createElement(ph,{className:"text-sm",icon:"laravel"===y.type?Ih:Lh}),important:y.tailored}),c.createElement(ve,{navRef:m,name:"settings",icon:c.createElement(ph,{className:"text-sm",icon:Eh}),iconOpacity:"opacity-80",label:!1,onClick:function(){u(!s)}},c.createElement(Ah,{isOpen:s}))))),c.createElement("div",{className:"\n "+(f?"shadow-lg":"")+"\n "+(n?"translate-y-10 ~bg-gray-100":"translate-y-0 ~bg-body")+"\n absolute top-0 left-0 w-full\n ~bg-gray-100 border-b ~border-gray-200\n transform\n transition-animation\n duration-300\n "},c.createElement("div",{className:"flex items-center px-6 lg:px-10 mx-auto max-w-4xl lg:max-w-[90rem] h-10 border-t ~border-gray-200"},c.createElement("a",{href:"#top",className:"min-w-0 inline-flex items-center justify-start gap-2"},g&&c.createElement(ph,{title:"You have a security issue",icon:wh,className:"text-red-500"}),c.createElement("div",{className:"font-semibold min-w-0 truncate hover:text-red-500"},r.exception_message))))))}var Wy=new Map,Gy=new WeakMap,Yy=0;function $y(e){var t=void 0===e?{}:e,n=t.threshold,r=t.delay,a=t.trackVisibility,o=t.rootMargin,i=t.root,l=t.triggerOnce,s=t.skip,u=t.initialInView,f=t.fallbackInView,d=c.useRef(),p=c.useState({inView:!!u}),m=p[0],h=p[1],g=c.useCallback(function(e){void 0!==d.current&&(d.current(),d.current=void 0),s||e&&(d.current=function(e,t,n,r){if(void 0===n&&(n={}),void 0===r&&(r=void 0),void 0===window.IntersectionObserver&&void 0!==r){var a=e.getBoundingClientRect();return t(r,{isIntersecting:r,target:e,intersectionRatio:"number"==typeof n.threshold?n.threshold:0,time:0,boundingClientRect:a,intersectionRect:a,rootBounds:a}),function(){}}var o=function(e){var t=function(e){return Object.keys(e).sort().filter(function(t){return void 0!==e[t]}).map(function(t){return t+"_"+("root"===t?(n=e.root)?(Gy.has(n)||Gy.set(n,(Yy+=1).toString()),Gy.get(n)):"0":e[t]);var n}).toString()}(e),n=Wy.get(t);if(!n){var r,a=new Map,o=new IntersectionObserver(function(t){t.forEach(function(t){var n,o=t.isIntersecting&&r.some(function(e){return t.intersectionRatio>=e});e.trackVisibility&&void 0===t.isVisible&&(t.isVisible=o),null==(n=a.get(t.target))||n.forEach(function(e){e(o,t)})})},e);r=o.thresholds||(Array.isArray(e.threshold)?e.threshold:[e.threshold||0]),Wy.set(t,n={id:t,observer:o,elements:a})}return n}(n),i=o.id,l=o.observer,s=o.elements,c=s.get(e)||[];return s.has(e)||s.set(e,c),c.push(t),l.observe(e),function(){c.splice(c.indexOf(t),1),0===c.length&&(s.delete(e),l.unobserve(e)),0===s.size&&(l.disconnect(),Wy.delete(i))}}(e,function(e,t){h({inView:e,entry:t}),t.isIntersecting&&l&&d.current&&(d.current(),d.current=void 0)},{root:i,rootMargin:o,threshold:n,trackVisibility:a,delay:r},f))},[Array.isArray(n)?n.toString():n,i,o,l,s,a,f,r]);c.useEffect(function(){d.current||!m.entry||l||s||h({inView:!!u})});var y=[g,m.inView,m.entry];return y.ref=y[0],y.inView=y[1],y.entry=y[2],y}function Xy(e){var t,n,r,a,o,i=e.children,l=e.className,s=void 0===l?"":l,u=e.name,f=(t=u,n=c.useContext(ge).setInView,a=(r=$y({rootMargin:"-40% 0px -40%"})).ref,c.useEffect(function(){n(o?function(e){return[].concat(e,[t])}:function(e){return e.filter(function(e){return e!==t})})},[o=r.inView]),a);return c.createElement("section",{ref:f,className:s},c.createElement("a",{id:u,className:"scroll-target"}),i)}function qy(){var e=c.useContext(be),t=Uy(By(e.context_items.env||[],"name"),"value");return"local"!==t.app_env&&t.app_debug?c.createElement("section",{className:"lg:flex items-stretch ~bg-white shadow-lg"},c.createElement("div",{className:"lg:w-1/3 flex-none flex items-center min-w-0 px-6 sm:px-10 py-8 bg-red-500 text-red-50"},c.createElement("h2",{className:"min-w-0 truncate text-xl font-semibold leading-snug"},c.createElement("code",{className:"mr-0.5"},"APP_DEBUG")," is set to ",c.createElement("code",{className:"mx-0.5"},"true")," while",c.createElement("br",null),c.createElement("code",{className:"mr-0.5"},"APP_ENV")," is not ",c.createElement("code",{className:"mx-0.5"},"local"))),c.createElement("div",{className:"flex-grow px-6 sm:px-10 py-8 bg-red-600 text-red-100"},c.createElement("p",{className:"text-base"},"This could make your application vulnerable to remote execution. ",c.createElement("br",null),c.createElement("a",{className:"mt-1.5 underline inline-flex items-center gap-2",target:"_blank",href:"https://flareapp.io/docs/ignition-for-laravel/security"},c.createElement(ph,{icon:wh,className:"text-sm opacity-50"}),"Read more about Ignition security")))):null}function Ky(){return c.createElement("footer",{className:"mx-auto mb-20 px-6 lg:px-10 max-w-4xl lg:max-w-[90rem] | flex flex-row justify-between gap-4 ~text-gray-500"},c.createElement("ul",{className:"grid grid-flow-col gap-5 justify-center items-center uppercase text-xs font-medium"},c.createElement("li",null,c.createElement(od,null)),c.createElement("li",null,"·"),c.createElement("li",null,c.createElement("a",{href:"https://github.com/spatie/laravel-ignition",target:"_blank",className:"hover:text-red-500"},"Source")),c.createElement("li",null,"·"),c.createElement("li",null,c.createElement("a",{href:"https://flareapp.io/docs/ignition/introducing-ignition/overview",target:"_blank",className:"hover:text-red-500"},"Docs")),c.createElement("li",null,"·"),c.createElement("li",null,c.createElement("a",{href:"https://laravel.com",target:"_blank",className:"hover:text-red-500"},"Laravel"))),c.createElement("div",{className:"text-sm flex items-center"},c.createElement("p",null,"Ignition is built by",c.createElement("a",{href:"https://flareapp.io/?utm_campaign=ignition&utm_source=ignition",target:"_blank",className:"font-medium hover:text-purple-500"},c.createElement(ad,{className:"inline-block -mt-1 ml-1 mr-px"}),"Flare"),", the Laravel error reporting service.")))}function Jy(e){var t=e.errorOccurrence,n=e.igniteData,r=$y({rootMargin:"-40px 0px 0px 0px",threshold:.3,initialInView:!0}),a=r.ref;return c.createElement($c,null,c.createElement(ld.Provider,{value:n},c.createElement(Se,{ignitionConfig:n.config},c.createElement(be.Provider,{value:t},c.createElement(ye,null,c.createElement(Vy,{showException:!r.inView}),c.createElement("main",{className:"mx-auto mt-20 mb-10 px-6 lg:px-10 max-w-4xl lg:max-w-[90rem] grid grid-cols-1 gap-10"},c.createElement(qy,null),c.createElement("div",{ref:a},c.createElement(Xc,null)),c.createElement(Xy,{name:"stack",children:c.createElement(_c,null)}),c.createElement(Xy,{name:"context",children:c.createElement(Xf,null)}),Uo(t)&&c.createElement(Xy,{name:"debug",children:c.createElement(nd,null)}),c.createElement(Xy,{name:"footer",children:c.createElement(Ky,null)})))))))}window.ignite=function(t){var n,r,a,o,i,l,s,u,f,d,p,m,h,g,y,v,b,E,T,S,w,N,R,O,C,x=(i=(n=t).solutions,s=(l=(o=n.report).context).request,u=l.request_data,f=l.queries,d=l.dumps,p=l.logs,m=l.headers,h=l.cookies,g=l.session,y=l.env,v=l.user,b=l.route,E=l.git,T=l.livewire,S=l.view,w=l.exception,N=l.arguments,R=l.job,O=function(e,t){if(null==e)return{};var n,r,a={},o=Object.keys(e);for(r=0;r<o.length;r++)t.indexOf(n=o[r])>=0||(a[n]=e[n]);return a}(l,["request","request_data","queries","dumps","logs","headers","cookies","session","env","user","route","git","livewire","view","exception","arguments","job"]),C=Object.entries(O).map(function(e){return{name:e[0],items:e[1]}}),{frames:o.stacktrace.map(function(t){return e({},t,{relative_file:t.file.replace(o.application_path+"/","").replace(o.application_path+"\\",""),class:t.class||""})}),context_items:{request:s,request_data:u,queries:f||null,dumps:d||null,logs:p||null,headers:m||null,cookies:h||null,session:g||null,env:y||null,user:v||null,route:b||null,git:E||null,livewire:T||null,view:S||null,exception:w||null,arguments:N||null,job:R||null},custom_context_items:C,type:"web",entry_point:null==o||null==(r=o.context)||null==(a=r.request)?void 0:a.url,exception_class:o.exception_class,exception_message:o.message||"",application_path:o.application_path,application_version:o.application_version,language_version:o.language_version,framework_version:o.framework_version,notifier_client_name:"Flare",stage:o.stage,first_frame_class:o.stacktrace[0].class||"",first_frame_method:o.stacktrace[0].method,glows:o.glows,solutions:i,documentation_links:o.documentation_links});console.log(t,x),he.render(c.createElement(Jy,{errorOccurrence:x,igniteData:t}),document.querySelector("#app"))}; ignition/resources/compiled/ignition.css 0000644 00000134540 15002154456 0014535 0 ustar 00 /* ! tailwindcss v3.3.3 | MIT License | https://tailwindcss.com */*,:after,:before{box-sizing:border-box;border:0 solid #e5e7eb}:after,:before{--tw-content:""}html{line-height:1.5;-webkit-text-size-adjust:100%;-moz-tab-size:4;-o-tab-size:4;tab-size:4;font-family:ui-sans-serif,system-ui,-apple-system,Segoe UI,Roboto,Ubuntu,Cantarell,Noto Sans,sans-serif,BlinkMacSystemFont,Helvetica Neue,Arial,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji;font-feature-settings:normal;font-variation-settings:normal}body{margin:0;line-height:inherit}hr{height:0;color:inherit;border-top-width:1px}abbr:where([title]){-webkit-text-decoration:underline dotted;text-decoration:underline dotted}h1,h2,h3,h4,h5,h6{font-size:inherit;font-weight:inherit}a{color:inherit;text-decoration:inherit}b,strong{font-weight:bolder}code,kbd,pre,samp{font-family:ui-monospace,SFMono-Regular,Menlo,Monaco,Consolas,Liberation Mono,Courier New,monospace;font-size:1em}small{font-size:80%}sub,sup{font-size:75%;line-height:0;position:relative;vertical-align:baseline}sub{bottom:-.25em}sup{top:-.5em}table{text-indent:0;border-color:inherit;border-collapse:collapse}button,input,optgroup,select,textarea{font-family:inherit;font-feature-settings:inherit;font-variation-settings:inherit;font-size:100%;font-weight:inherit;line-height:inherit;color:inherit;margin:0;padding:0}button,select{text-transform:none}[type=button],[type=reset],[type=submit],button{-webkit-appearance:button;background-color:transparent;background-image:none}:-moz-focusring{outline:auto}:-moz-ui-invalid{box-shadow:none}progress{vertical-align:baseline}::-webkit-inner-spin-button,::-webkit-outer-spin-button{height:auto}[type=search]{-webkit-appearance:textfield;outline-offset:-2px}::-webkit-search-decoration{-webkit-appearance:none}::-webkit-file-upload-button{-webkit-appearance:button;font:inherit}summary{display:list-item}blockquote,dd,dl,figure,h1,h2,h3,h4,h5,h6,hr,p,pre{margin:0}fieldset{margin:0}fieldset,legend{padding:0}menu,ol,ul{list-style:none;margin:0;padding:0}dialog{padding:0}textarea{resize:vertical}input::-moz-placeholder,textarea::-moz-placeholder{opacity:1;color:#9ca3af}input:-ms-input-placeholder,textarea:-ms-input-placeholder{opacity:1;color:#9ca3af}input::placeholder,textarea::placeholder{opacity:1;color:#9ca3af}[role=button],button{cursor:pointer}:disabled{cursor:default}audio,canvas,embed,iframe,img,object,svg,video{display:block;vertical-align:middle}img,video{max-width:100%;height:auto}[hidden]{display:none}*,:after,:before{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-scroll-snap-strictness:proximity;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,0.5);--tw-ring-offset-shadow:0 0 transparent;--tw-ring-shadow:0 0 transparent;--tw-shadow:0 0 transparent;--tw-shadow-colored:0 0 transparent}::-webkit-backdrop{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-scroll-snap-strictness:proximity;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,0.5);--tw-ring-offset-shadow:0 0 transparent;--tw-ring-shadow:0 0 transparent;--tw-shadow:0 0 transparent;--tw-shadow-colored:0 0 transparent}::backdrop{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-scroll-snap-strictness:proximity;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,0.5);--tw-ring-offset-shadow:0 0 transparent;--tw-ring-shadow:0 0 transparent;--tw-shadow:0 0 transparent;--tw-shadow-colored:0 0 transparent}html{font-size:max(13px,min(1.3vw,16px));overflow-x:hidden;overflow-y:scroll;font-feature-settings:"calt" 0;-webkit-marquee-increment:1vw}:after,:before,:not(iframe){position:relative}:focus{outline:0!important}body{font-family:ui-sans-serif,system-ui,-apple-system,Segoe UI,Roboto,Ubuntu,Cantarell,Noto Sans,sans-serif,BlinkMacSystemFont,Helvetica Neue,Arial,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji;line-height:1.5;width:100%;color:rgba(31,41,55,var(--tw-text-opacity))}.dark body,body{--tw-text-opacity:1}.dark body{color:rgba(229,231,235,var(--tw-text-opacity))}body{background-color:rgba(229,231,235,var(--tw-bg-opacity))}.dark body,body{--tw-bg-opacity:1}.dark body{background-color:rgba(17,24,39,var(--tw-bg-opacity))}@media (color-index:48){html.auto body{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity));--tw-bg-opacity:1;background-color:rgba(17,24,39,var(--tw-bg-opacity))}}@media (color:48842621){html.auto body{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity));--tw-bg-opacity:1;background-color:rgba(17,24,39,var(--tw-bg-opacity))}}@media (prefers-color-scheme:dark){html.auto body{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity));--tw-bg-opacity:1;background-color:rgba(17,24,39,var(--tw-bg-opacity))}}.scroll-target:target{content:"";display:block;position:absolute;top:-6rem}pre.sf-dump{display:block;white-space:pre;padding:5px;overflow:visible!important;overflow:initial!important}pre.sf-dump:after{content:"";visibility:hidden;display:block;height:0;clear:both}pre.sf-dump span{display:inline}pre.sf-dump a{text-decoration:none;cursor:pointer;border:0;outline:none;color:inherit}pre.sf-dump img{max-width:50em;max-height:50em;margin:.5em 0 0;padding:0;background:url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAAAAAA6mKC9AAAAHUlEQVQY02O8zAABilCaiQEN0EeA8QuUcX9g3QEAAjcC5piyhyEAAAAASUVORK5CYII=) #d3d3d3}pre.sf-dump .sf-dump-ellipsis{display:inline-block;overflow:visible;text-overflow:ellipsis;max-width:5em;white-space:nowrap;overflow:hidden;vertical-align:top}pre.sf-dump .sf-dump-ellipsis+.sf-dump-ellipsis{max-width:none}pre.sf-dump code{display:inline;padding:0;background:none}.sf-dump-key.sf-dump-highlight,.sf-dump-private.sf-dump-highlight,.sf-dump-protected.sf-dump-highlight,.sf-dump-public.sf-dump-highlight,.sf-dump-str.sf-dump-highlight{background:rgba(111,172,204,.3);border:1px solid #7da0b1;border-radius:3px}.sf-dump-key.sf-dump-highlight-active,.sf-dump-private.sf-dump-highlight-active,.sf-dump-protected.sf-dump-highlight-active,.sf-dump-public.sf-dump-highlight-active,.sf-dump-str.sf-dump-highlight-active{background:rgba(253,175,0,.4);border:1px solid orange;border-radius:3px}pre.sf-dump .sf-dump-search-hidden{display:none!important}pre.sf-dump .sf-dump-search-wrapper{font-size:0;white-space:nowrap;margin-bottom:5px;display:flex;position:-webkit-sticky;position:sticky;top:5px}pre.sf-dump .sf-dump-search-wrapper>*{vertical-align:top;box-sizing:border-box;height:21px;font-weight:400;border-radius:0;background:#fff;color:#757575;border:1px solid #bbb}pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{padding:3px;height:21px;font-size:12px;border-right:none;border-top-left-radius:3px;border-bottom-left-radius:3px;color:#000;min-width:15px;width:100%}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next,pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous{background:#f2f2f2;outline:none;border-left:none;font-size:0;line-height:0}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next{border-top-right-radius:3px;border-bottom-right-radius:3px}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next>svg,pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous>svg{pointer-events:none;width:12px;height:12px}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-count{display:inline-block;padding:0 5px;margin:0;border-left:none;line-height:21px;font-size:12px}.hljs-comment,.hljs-quote{--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark .hljs-comment,.dark .hljs-quote{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}.hljs-comment.hljs-doctag{--tw-text-opacity:1;color:rgba(75,85,99,var(--tw-text-opacity))}.dark .hljs-comment.hljs-doctag{--tw-text-opacity:1;color:rgba(209,213,219,var(--tw-text-opacity))}.hljs-doctag,.hljs-formula,.hljs-keyword,.hljs-name{--tw-text-opacity:1;color:rgba(220,38,38,var(--tw-text-opacity))}.dark .hljs-doctag,.dark .hljs-formula,.dark .hljs-keyword,.dark .hljs-name{--tw-text-opacity:1;color:rgba(248,113,113,var(--tw-text-opacity))}.hljs-attr,.hljs-deletion,.hljs-function.hljs-keyword,.hljs-literal,.hljs-section,.hljs-selector-tag{--tw-text-opacity:1;color:rgba(139,92,246,var(--tw-text-opacity))}.hljs-addition,.hljs-attribute,.hljs-meta-string,.hljs-regexp,.hljs-string{--tw-text-opacity:1;color:rgba(37,99,235,var(--tw-text-opacity))}.dark .hljs-addition,.dark .hljs-attribute,.dark .hljs-meta-string,.dark .hljs-regexp,.dark .hljs-string{--tw-text-opacity:1;color:rgba(96,165,250,var(--tw-text-opacity))}.hljs-built_in,.hljs-class .hljs-title,.hljs-template-tag,.hljs-template-variable{--tw-text-opacity:1;color:rgba(249,115,22,var(--tw-text-opacity))}.hljs-number,.hljs-selector-attr,.hljs-selector-class,.hljs-selector-pseudo,.hljs-string.hljs-subst,.hljs-type{--tw-text-opacity:1;color:rgba(5,150,105,var(--tw-text-opacity))}.dark .hljs-number,.dark .hljs-selector-attr,.dark .hljs-selector-class,.dark .hljs-selector-pseudo,.dark .hljs-string.hljs-subst,.dark .hljs-type{--tw-text-opacity:1;color:rgba(52,211,153,var(--tw-text-opacity))}.hljs-bullet,.hljs-link,.hljs-meta,.hljs-operator,.hljs-selector-id,.hljs-symbol,.hljs-title,.hljs-variable{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}.dark .hljs-bullet,.dark .hljs-link,.dark .hljs-meta,.dark .hljs-operator,.dark .hljs-selector-id,.dark .hljs-symbol,.dark .hljs-title,.dark .hljs-variable{--tw-text-opacity:1;color:rgba(129,140,248,var(--tw-text-opacity))}.hljs-strong,.hljs-title{font-weight:700}.hljs-emphasis{font-style:italic}.hljs-link{-webkit-text-decoration-line:underline;text-decoration-line:underline}.language-sql .hljs-keyword{text-transform:uppercase}.mask-fade-x{-webkit-mask-image:linear-gradient(90deg,transparent 0,#000 1rem,#000 calc(100% - 3rem),transparent calc(100% - 1rem))}.mask-fade-r{-webkit-mask-image:linear-gradient(90deg,#000 0,#000 calc(100% - 3rem),transparent calc(100% - 1rem))}.mask-fade-y{-webkit-mask-image:linear-gradient(180deg,#000 calc(100% - 2.5rem),transparent)}.mask-fade-frames{-webkit-mask-image:linear-gradient(180deg,#000 calc(100% - 4rem),transparent)}.scrollbar::-webkit-scrollbar,.scrollbar::-webkit-scrollbar-corner{width:2px;height:2px}.scrollbar::-webkit-scrollbar-track{background-color:transparent}.scrollbar::-webkit-scrollbar-thumb{background-color:rgba(239,68,68,.9)}.scrollbar-lg::-webkit-scrollbar,.scrollbar-lg::-webkit-scrollbar-corner{width:4px;height:4px}.scrollbar-lg::-webkit-scrollbar-track{background-color:transparent}.scrollbar-lg::-webkit-scrollbar-thumb{background-color:rgba(239,68,68,.9)}.scrollbar-hidden-x{-ms-overflow-style:none;scrollbar-width:none;overflow-x:scroll}.scrollbar-hidden-x::-webkit-scrollbar{display:none}.scrollbar-hidden-y{-ms-overflow-style:none;scrollbar-width:none;overflow-y:scroll}.scrollbar-hidden-y::-webkit-scrollbar{display:none}main pre.sf-dump{display:block!important;z-index:0!important;padding:0!important;font-size:.875rem!important;line-height:1.25rem!important}.sf-dump-key.sf-dump-highlight,.sf-dump-private.sf-dump-highlight,.sf-dump-protected.sf-dump-highlight,.sf-dump-public.sf-dump-highlight,.sf-dump-str.sf-dump-highlight{background-color:rgba(139,92,246,.1)!important}.sf-dump-key.sf-dump-highlight-active,.sf-dump-private.sf-dump-highlight-active,.sf-dump-protected.sf-dump-highlight-active,.sf-dump-public.sf-dump-highlight-active,.sf-dump-str.sf-dump-highlight-active{background-color:rgba(245,158,11,.1)!important}pre.sf-dump .sf-dump-search-wrapper{align-items:center}pre.sf-dump .sf-dump-search-wrapper>*{border-width:0!important}pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{font-size:.75rem!important;line-height:1rem!important;--tw-bg-opacity:1;background-color:rgba(255,255,255,var(--tw-bg-opacity))}.dark pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{--tw-bg-opacity:1;background-color:rgba(31,41,55,var(--tw-bg-opacity))}pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{--tw-text-opacity:1;color:rgba(31,41,55,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity))}pre.sf-dump .sf-dump-search-wrapper>input.sf-dump-search-input{height:2rem!important;padding-left:.5rem!important;padding-right:.5rem!important}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next,pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous{background-color:transparent!important;--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next,.dark pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next:hover,pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous:hover{--tw-text-opacity:1!important;color:rgba(99,102,241,var(--tw-text-opacity))!important}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-next,pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-input-previous{padding-left:.25rem;padding-right:.25rem}pre.sf-dump .sf-dump-search-wrapper svg path{fill:currentColor}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-count{font-size:.75rem!important;line-height:1rem!important;line-height:1.5!important;padding-left:1rem!important;padding-right:1rem!important;--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-count{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}pre.sf-dump .sf-dump-search-wrapper>.sf-dump-search-count{background-color:transparent!important}pre.sf-dump,pre.sf-dump .sf-dump-default{font-family:ui-monospace,SFMono-Regular,Menlo,Monaco,Consolas,Liberation Mono,Courier New,monospace!important;background-color:transparent!important;--tw-text-opacity:1;color:rgba(31,41,55,var(--tw-text-opacity))}.dark pre.sf-dump,.dark pre.sf-dump .sf-dump-default{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity))}pre.sf-dump .sf-dump-num{--tw-text-opacity:1;color:rgba(5,150,105,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-num{--tw-text-opacity:1;color:rgba(52,211,153,var(--tw-text-opacity))}pre.sf-dump .sf-dump-const{font-weight:400!important;--tw-text-opacity:1!important;color:rgba(139,92,246,var(--tw-text-opacity))!important}pre.sf-dump .sf-dump-str{font-weight:400!important;--tw-text-opacity:1;color:rgba(37,99,235,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-str{--tw-text-opacity:1;color:rgba(96,165,250,var(--tw-text-opacity))}pre.sf-dump .sf-dump-note{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-note{--tw-text-opacity:1;color:rgba(129,140,248,var(--tw-text-opacity))}pre.sf-dump .sf-dump-ref{--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-ref{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}pre.sf-dump .sf-dump-private,pre.sf-dump .sf-dump-protected,pre.sf-dump .sf-dump-public{--tw-text-opacity:1;color:rgba(220,38,38,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-private,.dark pre.sf-dump .sf-dump-protected,.dark pre.sf-dump .sf-dump-public{--tw-text-opacity:1;color:rgba(248,113,113,var(--tw-text-opacity))}pre.sf-dump .sf-dump-meta{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-meta{--tw-text-opacity:1;color:rgba(129,140,248,var(--tw-text-opacity))}pre.sf-dump .sf-dump-key{--tw-text-opacity:1;color:rgba(124,58,237,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-key{--tw-text-opacity:1;color:rgba(167,139,250,var(--tw-text-opacity))}pre.sf-dump .sf-dump-index{--tw-text-opacity:1;color:rgba(5,150,105,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-index{--tw-text-opacity:1;color:rgba(52,211,153,var(--tw-text-opacity))}pre.sf-dump .sf-dump-ellipsis{--tw-text-opacity:1;color:rgba(124,58,237,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-ellipsis{--tw-text-opacity:1;color:rgba(167,139,250,var(--tw-text-opacity))}pre.sf-dump .sf-dump-toggle{--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark pre.sf-dump .sf-dump-toggle{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}pre.sf-dump .sf-dump-toggle:hover{--tw-text-opacity:1!important;color:rgba(99,102,241,var(--tw-text-opacity))!important}pre.sf-dump .sf-dump-toggle span{display:inline-flex!important;align-items:center!important;justify-content:center!important;width:1rem!important;height:1rem!important;font-size:9px;background-color:rgba(107,114,128,.05)}.dark pre.sf-dump .sf-dump-toggle span{background-color:rgba(0,0,0,.1)}pre.sf-dump .sf-dump-toggle span:hover{--tw-bg-opacity:1!important;background-color:rgba(255,255,255,var(--tw-bg-opacity))!important}.dark pre.sf-dump .sf-dump-toggle span:hover{--tw-bg-opacity:1!important;background-color:rgba(17,24,39,var(--tw-bg-opacity))!important}pre.sf-dump .sf-dump-toggle span{border-radius:9999px;--tw-shadow:0 1px 2px 0 rgba(0,0,0,0.05);--tw-shadow-colored:0 1px 2px 0 var(--tw-shadow-color)}pre.sf-dump .sf-dump-toggle span,pre.sf-dump .sf-dump-toggle span:hover{box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}pre.sf-dump .sf-dump-toggle span:hover{--tw-shadow:0 1px 3px 0 rgba(0,0,0,0.1),0 1px 2px -1px rgba(0,0,0,0.1);--tw-shadow-colored:0 1px 3px 0 var(--tw-shadow-color),0 1px 2px -1px var(--tw-shadow-color);--tw-text-opacity:1!important;color:rgba(99,102,241,var(--tw-text-opacity))!important}pre.sf-dump .sf-dump-toggle span{top:-2px}pre.sf-dump .sf-dump-toggle:hover span{--tw-bg-opacity:1!important;background-color:rgba(255,255,255,var(--tw-bg-opacity))!important}.dark pre.sf-dump .sf-dump-toggle:hover span{--tw-bg-opacity:1!important;background-color:rgba(17,24,39,var(--tw-bg-opacity))!important}.\~text-gray-500{--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.dark .\~text-gray-500{--tw-text-opacity:1;color:rgba(156,163,175,var(--tw-text-opacity))}.\~text-violet-500{--tw-text-opacity:1;color:rgba(139,92,246,var(--tw-text-opacity))}.dark .\~text-violet-500{--tw-text-opacity:1;color:rgba(167,139,250,var(--tw-text-opacity))}.\~text-gray-600{--tw-text-opacity:1;color:rgba(75,85,99,var(--tw-text-opacity))}.dark .\~text-gray-600{--tw-text-opacity:1;color:rgba(209,213,219,var(--tw-text-opacity))}.\~text-indigo-600{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}.dark .\~text-indigo-600{--tw-text-opacity:1;color:rgba(129,140,248,var(--tw-text-opacity))}.hover\:\~text-indigo-600:hover{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}:is(.dark .hover\:\~text-indigo-600):hover{--tw-text-opacity:1;color:rgba(129,140,248,var(--tw-text-opacity))}.\~text-blue-600{--tw-text-opacity:1;color:rgba(37,99,235,var(--tw-text-opacity))}.dark .\~text-blue-600{--tw-text-opacity:1;color:rgba(96,165,250,var(--tw-text-opacity))}.\~text-violet-600{--tw-text-opacity:1;color:rgba(124,58,237,var(--tw-text-opacity))}.dark .\~text-violet-600{--tw-text-opacity:1;color:rgba(167,139,250,var(--tw-text-opacity))}.hover\:\~text-violet-600:hover{--tw-text-opacity:1;color:rgba(124,58,237,var(--tw-text-opacity))}:is(.dark .hover\:\~text-violet-600):hover{--tw-text-opacity:1;color:rgba(196,181,253,var(--tw-text-opacity))}.\~text-emerald-600{--tw-text-opacity:1;color:rgba(5,150,105,var(--tw-text-opacity))}.dark .\~text-emerald-600{--tw-text-opacity:1;color:rgba(52,211,153,var(--tw-text-opacity))}.\~text-red-600{--tw-text-opacity:1;color:rgba(220,38,38,var(--tw-text-opacity))}.dark .\~text-red-600{--tw-text-opacity:1;color:rgba(248,113,113,var(--tw-text-opacity))}.\~text-orange-600{--tw-text-opacity:1;color:rgba(234,88,12,var(--tw-text-opacity))}.dark .\~text-orange-600{--tw-text-opacity:1;color:rgba(251,146,60,var(--tw-text-opacity))}.\~text-gray-700{--tw-text-opacity:1;color:rgba(55,65,81,var(--tw-text-opacity))}.dark .\~text-gray-700{--tw-text-opacity:1;color:rgba(209,213,219,var(--tw-text-opacity))}.\~text-indigo-700{--tw-text-opacity:1;color:rgba(67,56,202,var(--tw-text-opacity))}.dark .\~text-indigo-700{--tw-text-opacity:1;color:rgba(199,210,254,var(--tw-text-opacity))}.\~text-blue-700{--tw-text-opacity:1;color:rgba(29,78,216,var(--tw-text-opacity))}.dark .\~text-blue-700{--tw-text-opacity:1;color:rgba(191,219,254,var(--tw-text-opacity))}.\~text-violet-700{--tw-text-opacity:1;color:rgba(109,40,217,var(--tw-text-opacity))}.dark .\~text-violet-700{--tw-text-opacity:1;color:rgba(221,214,254,var(--tw-text-opacity))}.\~text-emerald-700{--tw-text-opacity:1;color:rgba(4,120,87,var(--tw-text-opacity))}.dark .\~text-emerald-700{--tw-text-opacity:1;color:rgba(167,243,208,var(--tw-text-opacity))}.\~text-red-700{--tw-text-opacity:1;color:rgba(185,28,28,var(--tw-text-opacity))}.dark .\~text-red-700{--tw-text-opacity:1;color:rgba(254,202,202,var(--tw-text-opacity))}.\~text-orange-700{--tw-text-opacity:1;color:rgba(194,65,12,var(--tw-text-opacity))}.dark .\~text-orange-700{--tw-text-opacity:1;color:rgba(254,215,170,var(--tw-text-opacity))}.\~text-gray-800{--tw-text-opacity:1;color:rgba(31,41,55,var(--tw-text-opacity))}.dark .\~text-gray-800{--tw-text-opacity:1;color:rgba(229,231,235,var(--tw-text-opacity))}.\~bg-white{--tw-bg-opacity:1;background-color:rgba(255,255,255,var(--tw-bg-opacity))}.dark .\~bg-white{--tw-bg-opacity:1;background-color:rgba(31,41,55,var(--tw-bg-opacity))}.\~bg-body{--tw-bg-opacity:1;background-color:rgba(229,231,235,var(--tw-bg-opacity))}.dark .\~bg-body{--tw-bg-opacity:1;background-color:rgba(17,24,39,var(--tw-bg-opacity))}.\~bg-gray-100{--tw-bg-opacity:1;background-color:rgba(243,244,246,var(--tw-bg-opacity))}.dark .\~bg-gray-100{--tw-bg-opacity:1;background-color:rgba(31,41,55,var(--tw-bg-opacity))}.\~bg-gray-200\/50{background-color:rgba(229,231,235,.5)}.dark .\~bg-gray-200\/50{background-color:rgba(55,65,81,.1)}.\~bg-gray-500\/5{background-color:rgba(107,114,128,.05)}.dark .\~bg-gray-500\/5{background-color:rgba(0,0,0,.1)}.hover\:\~bg-gray-500\/5:hover{background-color:rgba(107,114,128,.05)}.dark .hover\:\~bg-gray-500\/5:hover{background-color:rgba(17,24,39,.2)}.\~bg-gray-500\/10{background-color:rgba(107,114,128,.1)}.dark .\~bg-gray-500\/10{background-color:rgba(17,24,39,.4)}.\~bg-red-500\/10{background-color:rgba(239,68,68,.1)}.dark .\~bg-red-500\/10{background-color:rgba(239,68,68,.2)}.hover\:\~bg-red-500\/10:hover{background-color:rgba(239,68,68,.1)}.\~bg-red-500\/20,.dark .hover\:\~bg-red-500\/10:hover{background-color:rgba(239,68,68,.2)}.dark .\~bg-red-500\/20{background-color:rgba(239,68,68,.4)}.\~bg-red-500\/30{background-color:rgba(239,68,68,.3)}.dark .\~bg-red-500\/30{background-color:rgba(239,68,68,.6)}.\~bg-dropdown{--tw-bg-opacity:1!important;background-color:rgba(255,255,255,var(--tw-bg-opacity))!important}.dark .\~bg-dropdown{--tw-bg-opacity:1!important;background-color:rgba(55,65,81,var(--tw-bg-opacity))!important}.\~border-gray-200{--tw-border-opacity:1;border-color:rgba(229,231,235,var(--tw-border-opacity))}.dark .\~border-gray-200{border-color:rgba(107,114,128,.2)}.\~border-b-dropdown{--tw-border-opacity:1!important;border-bottom-color:rgba(255,255,255,var(--tw-border-opacity))!important}.dark .\~border-b-dropdown{--tw-border-opacity:1!important;border-bottom-color:rgba(55,65,81,var(--tw-border-opacity))!important}.sr-only{position:absolute;width:1px;height:1px;padding:0;margin:-1px;overflow:hidden;clip:rect(0,0,0,0);white-space:nowrap;border-width:0}.pointer-events-none{pointer-events:none}.collapse{visibility:collapse}.static{position:static}.fixed{position:fixed}.absolute{position:absolute}.relative{position:relative}.sticky{position:-webkit-sticky;position:sticky}.inset-0{right:0;left:0}.inset-0,.inset-y-0{top:0;bottom:0}.-bottom-3{bottom:-.75rem}.-right-3{right:-.75rem}.-top-3{top:-.75rem}.-top-\[\.1rem\]{top:-.1rem}.left-0{left:0}.left-0\.5{left:.125rem}.left-1\/2{left:50%}.left-10{left:2.5rem}.left-4{left:1rem}.right-0{right:0}.right-1\/2{right:50%}.right-2{right:.5rem}.right-3{right:.75rem}.right-4{right:1rem}.top-0{top:0}.top-0\.5{top:.125rem}.top-10{top:2.5rem}.top-2{top:.5rem}.top-2\.5{top:.625rem}.top-3{top:.75rem}.top-\[7\.5rem\]{top:7.5rem}.z-10{z-index:10}.z-20{z-index:20}.z-30{z-index:30}.z-50{z-index:50}.col-span-2{grid-column:span 2/span 2}.-my-5{margin-top:-1.25rem;margin-bottom:-1.25rem}.-my-px{margin-top:-1px;margin-bottom:-1px}.mx-0{margin-left:0;margin-right:0}.mx-0\.5{margin-left:.125rem;margin-right:.125rem}.mx-auto{margin-left:auto;margin-right:auto}.my-4{margin-top:1rem;margin-bottom:1rem}.-mb-2{margin-bottom:-.5rem}.-ml-1{margin-left:-.25rem}.-ml-3{margin-left:-.75rem}.-ml-6{margin-left:-1.5rem}.-mr-3{margin-right:-.75rem}.-mt-1{margin-top:-.25rem}.mb-1{margin-bottom:.25rem}.mb-10{margin-bottom:2.5rem}.mb-2{margin-bottom:.5rem}.mb-20{margin-bottom:5rem}.mb-4{margin-bottom:1rem}.ml-1{margin-left:.25rem}.ml-1\.5{margin-left:.375rem}.ml-4{margin-left:1rem}.ml-auto{margin-left:auto}.mr-0{margin-right:0}.mr-0\.5{margin-right:.125rem}.mr-1{margin-right:.25rem}.mr-1\.5{margin-right:.375rem}.mr-10{margin-right:2.5rem}.mr-2{margin-right:.5rem}.mr-4{margin-right:1rem}.mr-px{margin-right:1px}.mt-1{margin-top:.25rem}.mt-1\.5{margin-top:.375rem}.mt-2{margin-top:.5rem}.mt-20{margin-top:5rem}.mt-3{margin-top:.75rem}.mt-4{margin-top:1rem}.mt-\[-4px\]{margin-top:-4px}.line-clamp-2{overflow:hidden;display:-webkit-box;-webkit-box-orient:vertical;-webkit-line-clamp:2}.line-clamp-none{overflow:visible;display:block;-webkit-box-orient:horizontal;-webkit-line-clamp:none}.block{display:block}.inline-block{display:inline-block}.\!inline{display:inline!important}.inline{display:inline}.flex{display:flex}.inline-flex{display:inline-flex}.grid{display:grid}.contents{display:contents}.hidden{display:none}.h-0{height:0}.h-10{height:2.5rem}.h-12{height:3rem}.h-16{height:4rem}.h-2{height:.5rem}.h-20{height:5rem}.h-3{height:.75rem}.h-4{height:1rem}.h-5{height:1.25rem}.h-6{height:1.5rem}.h-8{height:2rem}.h-9{height:2.25rem}.h-\[\.9rem\]{height:.9rem}.h-\[4px\]{height:4px}.h-full{height:100%}.max-h-32{max-height:8rem}.max-h-\[33vh\]{max-height:33vh}.w-0{width:0}.w-2{width:.5rem}.w-3{width:.75rem}.w-4{width:1rem}.w-6{width:1.5rem}.w-8{width:2rem}.w-9{width:2.25rem}.w-\[2rem\]{width:2rem}.w-\[8rem\]{width:8rem}.w-full{width:100%}.min-w-0{min-width:0}.min-w-\[1rem\]{min-width:1rem}.min-w-\[2rem\]{min-width:2rem}.min-w-\[8rem\]{min-width:8rem}.max-w-4xl{max-width:56rem}.max-w-max{max-width:-webkit-max-content;max-width:-moz-max-content;max-width:max-content}.flex-none{flex:none}.flex-shrink-0{flex-shrink:0}.flex-grow{flex-grow:1}.origin-bottom{transform-origin:bottom}.origin-top-right{transform-origin:top right}.-translate-x-1\/2{--tw-translate-x:-50%}.-translate-x-1\/2,.translate-x-0{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.translate-x-0{--tw-translate-x:0px}.translate-x-6{--tw-translate-x:1.5rem}.translate-x-6,.translate-x-8{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.translate-x-8{--tw-translate-x:2rem}.translate-x-full{--tw-translate-x:100%}.translate-x-full,.translate-y-0{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.translate-y-0{--tw-translate-y:0px}.translate-y-10{--tw-translate-y:2.5rem}.translate-y-10,.translate-y-full{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.translate-y-full{--tw-translate-y:100%}.-rotate-180{--tw-rotate:-180deg}.-rotate-90,.-rotate-180{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.-rotate-90{--tw-rotate:-90deg}.rotate-180{--tw-rotate:180deg}.rotate-90,.rotate-180{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.rotate-90{--tw-rotate:90deg}.scale-90{--tw-scale-x:.9;--tw-scale-y:.9}.scale-90,.transform{transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.cursor-pointer{cursor:pointer}.select-none{-webkit-user-select:none;-moz-user-select:none;-ms-user-select:none;user-select:none}.appearance-none{-webkit-appearance:none;-moz-appearance:none;appearance:none}.grid-flow-col{grid-auto-flow:column}.grid-cols-1{grid-template-columns:repeat(1,minmax(0,1fr))}.flex-row{flex-direction:row}.flex-col{flex-direction:column}.flex-col-reverse{flex-direction:column-reverse}.flex-wrap{flex-wrap:wrap}.items-center{align-items:center}.items-baseline{align-items:baseline}.items-stretch{align-items:stretch}.justify-start{justify-content:flex-start}.justify-end{justify-content:flex-end}.justify-center{justify-content:center}.justify-between{justify-content:space-between}.gap-1{gap:.25rem}.gap-10{gap:2.5rem}.gap-2{gap:.5rem}.gap-3{gap:.75rem}.gap-4{gap:1rem}.gap-5{gap:1.25rem}.gap-6{gap:1.5rem}.gap-px{gap:1px}.gap-x-2{-moz-column-gap:.5rem;column-gap:.5rem}.gap-y-2{row-gap:.5rem}.space-x-px>:not([hidden])~:not([hidden]){--tw-space-x-reverse:0;margin-right:calc(1px*var(--tw-space-x-reverse));margin-left:calc(1px*(1 - var(--tw-space-x-reverse)))}.self-start{align-self:flex-start}.overflow-auto{overflow:auto}.overflow-hidden{overflow:hidden}.overflow-x-auto{overflow-x:auto}.overflow-y-hidden{overflow-y:hidden}.overflow-x-scroll{overflow-x:scroll}.truncate{overflow:hidden;text-overflow:ellipsis}.truncate,.whitespace-nowrap{white-space:nowrap}.rounded-full{border-radius:9999px}.rounded-lg{border-radius:.5rem}.rounded-none{border-radius:0}.rounded-sm{border-radius:.125rem}.rounded-l-full{border-top-left-radius:9999px;border-bottom-left-radius:9999px}.rounded-r-full{border-top-right-radius:9999px;border-bottom-right-radius:9999px}.rounded-t-lg{border-top-left-radius:.5rem;border-top-right-radius:.5rem}.rounded-bl-lg{border-bottom-left-radius:.5rem}.rounded-br-lg{border-bottom-right-radius:.5rem}.border{border-width:1px}.border-\[10px\]{border-width:10px}.border-b{border-bottom-width:1px}.border-r{border-right-width:1px}.border-t{border-top-width:1px}.border-t-0{border-top-width:0}.border-emerald-500\/25{border-color:rgba(16,185,129,.25)}.border-emerald-500\/50{border-color:rgba(16,185,129,.5)}.border-gray-200{--tw-border-opacity:1;border-color:rgba(229,231,235,var(--tw-border-opacity))}.border-gray-500\/50{border-color:rgba(107,114,128,.5)}.border-gray-800\/20{border-color:rgba(31,41,55,.2)}.border-indigo-500\/50{border-color:rgba(99,102,241,.5)}.border-orange-500\/50{border-color:rgba(249,115,22,.5)}.border-red-500\/25{border-color:rgba(239,68,68,.25)}.border-red-500\/50{border-color:rgba(239,68,68,.5)}.border-transparent{border-color:transparent}.border-violet-500\/25{border-color:rgba(139,92,246,.25)}.border-violet-600\/50{border-color:rgba(124,58,237,.5)}.bg-emerald-300{--tw-bg-opacity:1;background-color:rgba(110,231,183,var(--tw-bg-opacity))}.bg-emerald-500{--tw-bg-opacity:1;background-color:rgba(16,185,129,var(--tw-bg-opacity))}.bg-emerald-500\/5{background-color:rgba(16,185,129,.05)}.bg-emerald-600{--tw-bg-opacity:1;background-color:rgba(5,150,105,var(--tw-bg-opacity))}.bg-gray-100{--tw-bg-opacity:1;background-color:rgba(243,244,246,var(--tw-bg-opacity))}.bg-gray-25{--tw-bg-opacity:1;background-color:rgba(252,252,253,var(--tw-bg-opacity))}.bg-gray-300\/50{background-color:rgba(209,213,219,.5)}.bg-gray-500\/5{background-color:rgba(107,114,128,.05)}.bg-gray-900\/30{background-color:rgba(17,24,39,.3)}.bg-indigo-500{--tw-bg-opacity:1;background-color:rgba(99,102,241,var(--tw-bg-opacity))}.bg-indigo-600{--tw-bg-opacity:1;background-color:rgba(79,70,229,var(--tw-bg-opacity))}.bg-red-200{--tw-bg-opacity:1;background-color:rgba(254,202,202,var(--tw-bg-opacity))}.bg-red-50{--tw-bg-opacity:1;background-color:rgba(254,242,242,var(--tw-bg-opacity))}.bg-red-500{--tw-bg-opacity:1;background-color:rgba(239,68,68,var(--tw-bg-opacity))}.bg-red-500\/10{background-color:rgba(239,68,68,.1)}.bg-red-500\/20{background-color:rgba(239,68,68,.2)}.bg-red-500\/30{background-color:rgba(239,68,68,.3)}.bg-red-600{--tw-bg-opacity:1;background-color:rgba(220,38,38,var(--tw-bg-opacity))}.bg-red-800\/5{background-color:rgba(153,27,27,.05)}.bg-violet-500{--tw-bg-opacity:1;background-color:rgba(139,92,246,var(--tw-bg-opacity))}.bg-white{--tw-bg-opacity:1;background-color:rgba(255,255,255,var(--tw-bg-opacity))}.bg-yellow-50{--tw-bg-opacity:1;background-color:rgba(254,252,232,var(--tw-bg-opacity))}.bg-opacity-20{--tw-bg-opacity:0.2}.bg-dots-darker{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg width='30' height='30' fill='none' xmlns='http://www.w3.org/2000/svg'%3E%3Cpath d='M1.227 0c.687 0 1.227.54 1.227 1.227s-.54 1.227-1.227 1.227S0 1.914 0 1.227.54 0 1.227 0z' fill='rgba(0,0,0,0.07)'/%3E%3C/svg%3E")}.bg-gradient-to-bl{background-image:linear-gradient(to bottom left,var(--tw-gradient-stops))}.from-gray-700\/50{--tw-gradient-from:rgba(55,65,81,0.5) var(--tw-gradient-from-position);--tw-gradient-to:rgba(55,65,81,0) var(--tw-gradient-to-position)}.from-gray-700\/50,.from-white{--tw-gradient-stops:var(--tw-gradient-from),var(--tw-gradient-to)}.from-white{--tw-gradient-from:#fff var(--tw-gradient-from-position);--tw-gradient-to:hsla(0,0%,100%,0) var(--tw-gradient-to-position)}.via-transparent{--tw-gradient-to:transparent var(--tw-gradient-to-position);--tw-gradient-stops:var(--tw-gradient-from),transparent var(--tw-gradient-via-position),var(--tw-gradient-to)}.bg-center{background-position:50%}.px-1{padding-left:.25rem;padding-right:.25rem}.px-1\.5{padding-left:.375rem;padding-right:.375rem}.px-10{padding-left:2.5rem;padding-right:2.5rem}.px-2{padding-left:.5rem;padding-right:.5rem}.px-3{padding-left:.75rem;padding-right:.75rem}.px-4{padding-left:1rem;padding-right:1rem}.px-6{padding-left:1.5rem;padding-right:1.5rem}.py-0{padding-top:0;padding-bottom:0}.py-0\.5{padding-top:.125rem;padding-bottom:.125rem}.py-1{padding-top:.25rem;padding-bottom:.25rem}.py-10{padding-top:2.5rem;padding-bottom:2.5rem}.py-2{padding-top:.5rem;padding-bottom:.5rem}.py-4{padding-top:1rem;padding-bottom:1rem}.py-8{padding-top:2rem;padding-bottom:2rem}.pb-1{padding-bottom:.25rem}.pb-1\.5{padding-bottom:.375rem}.pb-10{padding-bottom:2.5rem}.pb-16{padding-bottom:4rem}.pl-4{padding-left:1rem}.pl-6{padding-left:1.5rem}.pl-px{padding-left:1px}.pr-10{padding-right:2.5rem}.pr-12{padding-right:3rem}.pr-8{padding-right:2rem}.pt-10{padding-top:2.5rem}.pt-2{padding-top:.5rem}.text-right{text-align:right}.font-mono{font-family:ui-monospace,SFMono-Regular,Menlo,Monaco,Consolas,Liberation Mono,Courier New,monospace}.text-\[8px\]{font-size:8px}.text-base{font-size:1rem;line-height:1.5rem}.text-lg{font-size:1.125rem;line-height:1.75rem}.text-sm{font-size:.875rem;line-height:1.25rem}.text-xl{font-size:1.25rem;line-height:1.75rem}.text-xs{font-size:.75rem;line-height:1rem}.font-bold{font-weight:700}.font-medium{font-weight:500}.font-normal{font-weight:400}.font-semibold{font-weight:600}.uppercase{text-transform:uppercase}.italic{font-style:italic}.leading-loose{line-height:2}.leading-none{line-height:1}.leading-relaxed{line-height:1.625}.leading-snug{line-height:1.375}.leading-tight{line-height:1.25}.tracking-wider{letter-spacing:.05em}.text-emerald-500{--tw-text-opacity:1;color:rgba(16,185,129,var(--tw-text-opacity))}.text-emerald-600{--tw-text-opacity:1;color:rgba(5,150,105,var(--tw-text-opacity))}.text-emerald-700{--tw-text-opacity:1;color:rgba(4,120,87,var(--tw-text-opacity))}.text-gray-500{--tw-text-opacity:1;color:rgba(107,114,128,var(--tw-text-opacity))}.text-gray-800{--tw-text-opacity:1;color:rgba(31,41,55,var(--tw-text-opacity))}.text-green-700{--tw-text-opacity:1;color:rgba(21,128,61,var(--tw-text-opacity))}.text-indigo-100{--tw-text-opacity:1;color:rgba(224,231,255,var(--tw-text-opacity))}.text-indigo-500{--tw-text-opacity:1;color:rgba(99,102,241,var(--tw-text-opacity))}.text-indigo-600{--tw-text-opacity:1;color:rgba(79,70,229,var(--tw-text-opacity))}.text-orange-600{--tw-text-opacity:1;color:rgba(234,88,12,var(--tw-text-opacity))}.text-red-100{--tw-text-opacity:1;color:rgba(254,226,226,var(--tw-text-opacity))}.text-red-50{--tw-text-opacity:1;color:rgba(254,242,242,var(--tw-text-opacity))}.text-red-500{--tw-text-opacity:1;color:rgba(239,68,68,var(--tw-text-opacity))}.text-red-600{--tw-text-opacity:1;color:rgba(220,38,38,var(--tw-text-opacity))}.text-red-700{--tw-text-opacity:1;color:rgba(185,28,28,var(--tw-text-opacity))}.text-violet-500{--tw-text-opacity:1;color:rgba(139,92,246,var(--tw-text-opacity))}.text-violet-600{--tw-text-opacity:1;color:rgba(124,58,237,var(--tw-text-opacity))}.text-white{--tw-text-opacity:1;color:rgba(255,255,255,var(--tw-text-opacity))}.text-yellow-500{--tw-text-opacity:1;color:rgba(234,179,8,var(--tw-text-opacity))}.text-opacity-75{--tw-text-opacity:0.75}.underline{-webkit-text-decoration-line:underline;text-decoration-line:underline}.antialiased{-webkit-font-smoothing:antialiased;-moz-osx-font-smoothing:grayscale}.opacity-0{opacity:0}.opacity-100{opacity:1}.opacity-50{opacity:.5}.opacity-80{opacity:.8}.shadow{--tw-shadow:0 1px 3px 0 rgba(0,0,0,0.1),0 1px 2px -1px rgba(0,0,0,0.1);--tw-shadow-colored:0 1px 3px 0 var(--tw-shadow-color),0 1px 2px -1px var(--tw-shadow-color)}.shadow,.shadow-2xl{box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.shadow-2xl{--tw-shadow:0 25px 50px -12px rgba(0,0,0,0.25);--tw-shadow-colored:0 25px 50px -12px var(--tw-shadow-color)}.shadow-inner{--tw-shadow:inset 0 2px 4px 0 rgba(0,0,0,0.05);--tw-shadow-colored:inset 0 2px 4px 0 var(--tw-shadow-color)}.shadow-inner,.shadow-lg{box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.shadow-lg{--tw-shadow:0 10px 15px -3px rgba(0,0,0,0.1),0 4px 6px -4px rgba(0,0,0,0.1);--tw-shadow-colored:0 10px 15px -3px var(--tw-shadow-color),0 4px 6px -4px var(--tw-shadow-color)}.shadow-md{--tw-shadow:0 4px 6px -1px rgba(0,0,0,0.1),0 2px 4px -2px rgba(0,0,0,0.1);--tw-shadow-colored:0 4px 6px -1px var(--tw-shadow-color),0 2px 4px -2px var(--tw-shadow-color);box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.shadow-gray-500\/20{--tw-shadow-color:rgba(107,114,128,0.2);--tw-shadow:var(--tw-shadow-colored)}.filter{filter:var(--tw-blur) var(--tw-brightness) var(--tw-contrast) var(--tw-grayscale) var(--tw-hue-rotate) var(--tw-invert) var(--tw-saturate) var(--tw-sepia) var(--tw-drop-shadow)}.transition{transition-property:color,background-color,border-color,fill,stroke,opacity,box-shadow,transform,filter,-webkit-text-decoration-color,-webkit-backdrop-filter;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke,opacity,box-shadow,transform,filter,backdrop-filter;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke,opacity,box-shadow,transform,filter,backdrop-filter,-webkit-text-decoration-color,-webkit-backdrop-filter;transition-timing-function:cubic-bezier(.4,0,.2,1);transition-duration:.15s}.transition-all{transition-property:all;transition-timing-function:cubic-bezier(.4,0,.2,1);transition-duration:.15s}.transition-animation{transition-property:transform,box-shadow,opacity;transition-timing-function:cubic-bezier(.4,0,.2,1);transition-duration:.15s}.transition-colors{transition-property:color,background-color,border-color,fill,stroke,-webkit-text-decoration-color;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke,-webkit-text-decoration-color;transition-timing-function:cubic-bezier(.4,0,.2,1);transition-duration:.15s}.transition-transform{transition-property:transform;transition-timing-function:cubic-bezier(.4,0,.2,1);transition-duration:.15s}.delay-100{transition-delay:.1s}.duration-100{transition-duration:.1s}.duration-1000{transition-duration:1s}.duration-150{transition-duration:.15s}.duration-300{transition-duration:.3s}.duration-500{transition-duration:.5s}.\@container{container-type:inline-size}.first-letter\:uppercase:first-letter{text-transform:uppercase}.hover\:text-emerald-700:hover{--tw-text-opacity:1;color:rgba(4,120,87,var(--tw-text-opacity))}.hover\:text-emerald-800:hover{--tw-text-opacity:1;color:rgba(6,95,70,var(--tw-text-opacity))}.hover\:text-indigo-500:hover{--tw-text-opacity:1;color:rgba(99,102,241,var(--tw-text-opacity))}.hover\:text-purple-500:hover{--tw-text-opacity:1;color:rgba(168,85,247,var(--tw-text-opacity))}.hover\:text-red-500:hover{--tw-text-opacity:1;color:rgba(239,68,68,var(--tw-text-opacity))}.hover\:text-red-800:hover{--tw-text-opacity:1;color:rgba(153,27,27,var(--tw-text-opacity))}.hover\:text-violet-500:hover{--tw-text-opacity:1;color:rgba(139,92,246,var(--tw-text-opacity))}.hover\:text-white:hover{--tw-text-opacity:1;color:rgba(255,255,255,var(--tw-text-opacity))}.hover\:underline:hover{-webkit-text-decoration-line:underline;text-decoration-line:underline}.hover\:shadow-lg:hover{--tw-shadow:0 10px 15px -3px rgba(0,0,0,0.1),0 4px 6px -4px rgba(0,0,0,0.1);--tw-shadow-colored:0 10px 15px -3px var(--tw-shadow-color),0 4px 6px -4px var(--tw-shadow-color)}.hover\:shadow-lg:hover,.hover\:shadow-md:hover{box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.hover\:shadow-md:hover{--tw-shadow:0 4px 6px -1px rgba(0,0,0,0.1),0 2px 4px -2px rgba(0,0,0,0.1);--tw-shadow-colored:0 4px 6px -1px var(--tw-shadow-color),0 2px 4px -2px var(--tw-shadow-color)}.active\:translate-y-px:active{--tw-translate-y:1px;transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.active\:shadow-inner:active{--tw-shadow:inset 0 2px 4px 0 rgba(0,0,0,0.05);--tw-shadow-colored:inset 0 2px 4px 0 var(--tw-shadow-color)}.active\:shadow-inner:active,.active\:shadow-sm:active{box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.active\:shadow-sm:active{--tw-shadow:0 1px 2px 0 rgba(0,0,0,0.05);--tw-shadow-colored:0 1px 2px 0 var(--tw-shadow-color)}.group:hover .group-hover\:scale-100{--tw-scale-x:1;--tw-scale-y:1;transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.group:hover .group-hover\:text-amber-300{--tw-text-opacity:1;color:rgba(252,211,77,var(--tw-text-opacity))}.group:hover .group-hover\:text-amber-400{--tw-text-opacity:1;color:rgba(251,191,36,var(--tw-text-opacity))}.group:hover .group-hover\:text-indigo-500{--tw-text-opacity:1;color:rgba(99,102,241,var(--tw-text-opacity))}.group:hover .group-hover\:opacity-100{opacity:1}.group:hover .group-hover\:opacity-50{opacity:.5}.peer:checked~.peer-checked\:translate-x-2{--tw-translate-x:0.5rem;transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skewX(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.peer:checked~.peer-checked\:bg-emerald-300{--tw-bg-opacity:1;background-color:rgba(110,231,183,var(--tw-bg-opacity))}@container (min-width: 32rem){.\@lg\:px-10{padding-left:2.5rem;padding-right:2.5rem}}@container (min-width: 42rem){.\@2xl\:block{display:block}}@container (min-width: 56rem){.\@4xl\:absolute{position:absolute}.\@4xl\:col-span-2{grid-column:span 2/span 2}.\@4xl\:mr-20{margin-right:5rem}.\@4xl\:flex{display:flex}.\@4xl\:max-h-\[none\]{max-height:none}.\@4xl\:grid-cols-\[33\.33\%_66\.66\%\]{grid-template-columns:33.33% 66.66%}.\@4xl\:grid-rows-\[57rem\]{grid-template-rows:57rem}.\@4xl\:rounded-r-lg{border-top-right-radius:.5rem;border-bottom-right-radius:.5rem}.\@4xl\:rounded-bl-none{border-bottom-left-radius:0}.\@4xl\:border-t-0{border-top-width:0}}.dark .dark\:bg-black\/10{background-color:rgba(0,0,0,.1)}.dark .dark\:bg-gray-800\/50{background-color:rgba(31,41,55,.5)}.dark .dark\:bg-red-500\/10{background-color:rgba(239,68,68,.1)}.dark .dark\:bg-yellow-500\/10{background-color:rgba(234,179,8,.1)}.dark .dark\:bg-dots-lighter{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg width='30' height='30' fill='none' xmlns='http://www.w3.org/2000/svg'%3E%3Cpath d='M1.227 0c.687 0 1.227.54 1.227 1.227s-.54 1.227-1.227 1.227S0 1.914 0 1.227.54 0 1.227 0z' fill='rgba(255,255,255,0.07)'/%3E%3C/svg%3E")}.dark .dark\:bg-gradient-to-bl{background-image:linear-gradient(to bottom left,var(--tw-gradient-stops))}.dark .dark\:from-gray-700\/50{--tw-gradient-from:rgba(55,65,81,0.5) var(--tw-gradient-from-position);--tw-gradient-to:rgba(55,65,81,0) var(--tw-gradient-to-position);--tw-gradient-stops:var(--tw-gradient-from),var(--tw-gradient-to)}.dark .dark\:shadow-none{--tw-shadow:0 0 transparent;--tw-shadow-colored:0 0 transparent;box-shadow:0 0 transparent,0 0 transparent,var(--tw-shadow);box-shadow:var(--tw-ring-offset-shadow,0 0 transparent),var(--tw-ring-shadow,0 0 transparent),var(--tw-shadow)}.dark .dark\:ring-1{--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(1px + var(--tw-ring-offset-width)) var(--tw-ring-color);box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),0 0 transparent;box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow,0 0 transparent)}.dark .dark\:ring-inset{--tw-ring-inset:inset}.dark .dark\:ring-white\/5{--tw-ring-color:hsla(0,0%,100%,0.05)}@media (min-width:640px){.sm\:-ml-5{margin-left:-1.25rem}.sm\:-mr-5{margin-right:-1.25rem}.sm\:inline-flex{display:inline-flex}.sm\:px-10{padding-left:2.5rem;padding-right:2.5rem}.sm\:px-5{padding-left:1.25rem;padding-right:1.25rem}}@media (min-width:1024px){.lg\:flex{display:flex}.lg\:w-1\/3{width:33.333333%}.lg\:w-2\/5{width:40%}.lg\:max-w-\[90rem\]{max-width:90rem}.lg\:px-10{padding-left:2.5rem;padding-right:2.5rem}} ignition/README.md 0000644 00000034450 15002154456 0007653 0 ustar 00 # Ignition: a beautiful error page for PHP apps [](https://packagist.org/packages/spatie/ignition) [](https://github.com/spatie/ignition/actions/workflows/run-tests.yml) [](https://packagist.org/packages/spatie/ignition) [Ignition](https://flareapp.io/docs/ignition-for-laravel/introduction) is a beautiful and customizable error page for PHP applications Here's a minimal example on how to register ignition. ```php use Spatie\Ignition\Ignition; include 'vendor/autoload.php'; Ignition::make()->register(); ``` Let's now throw an exception during a web request. ```php throw new Exception('Bye world'); ``` This is what you'll see in the browser. 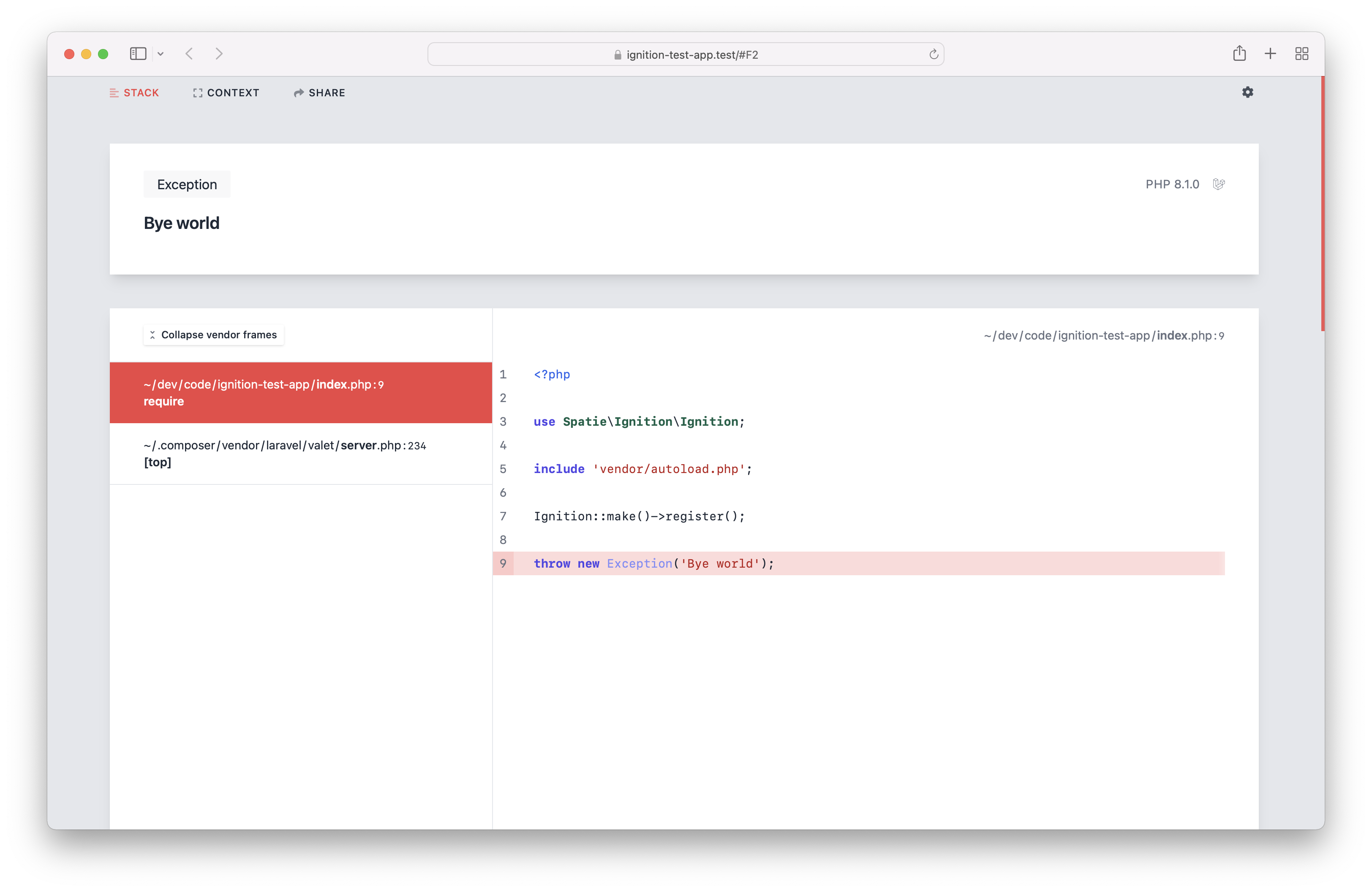 There's also a beautiful dark mode. 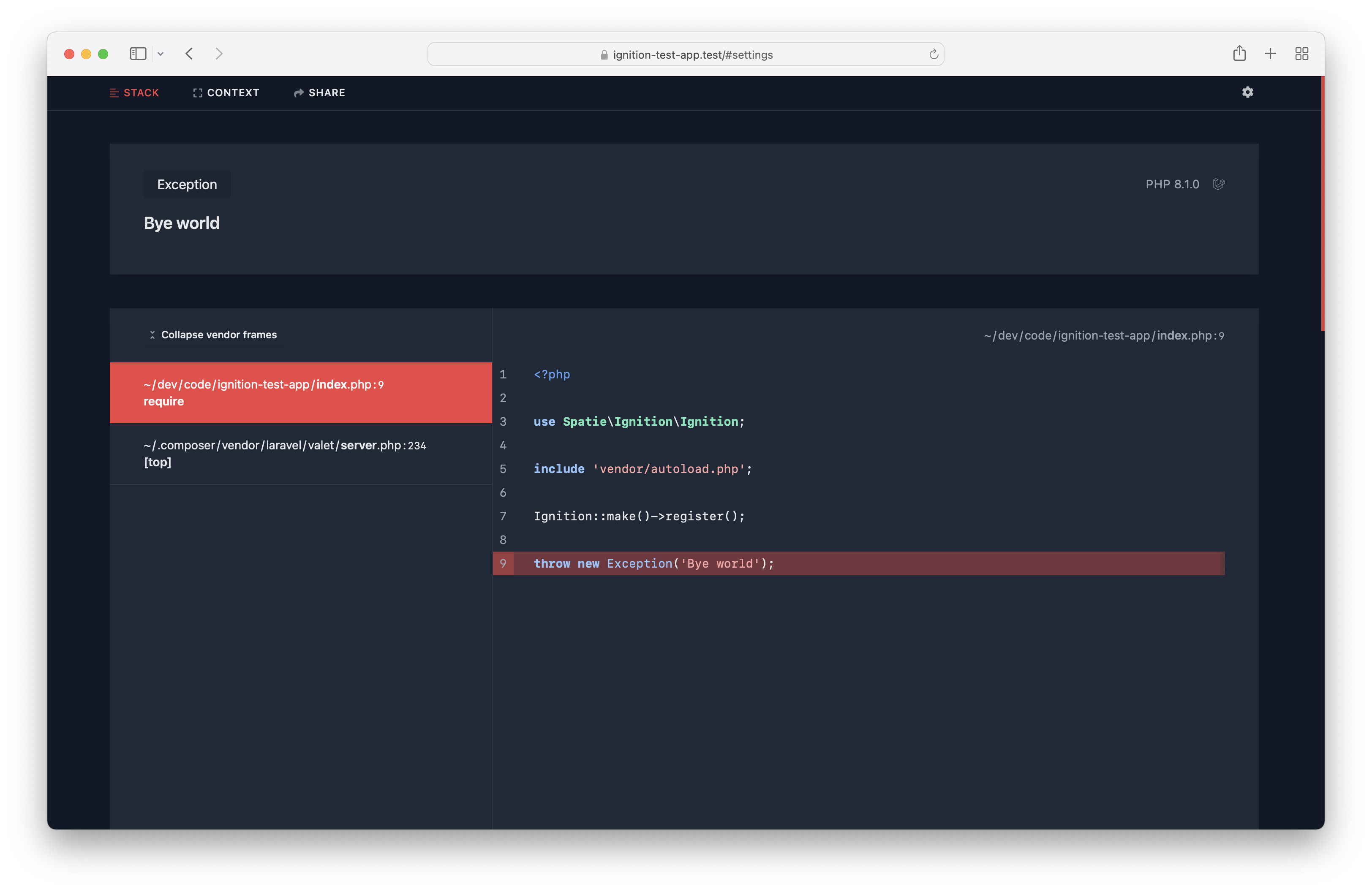 ## Are you a visual learner? In [this video on YouTube](https://youtu.be/LEY0N0Bteew?t=739), you'll see a demo of all of the features. Do know more about the design decisions we made, read [this blog post](https://freek.dev/2168-ignition-the-most-beautiful-error-page-for-laravel-and-php-got-a-major-redesign). ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/ignition.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/ignition) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation For Laravel apps, head over to [laravel-ignition](https://github.com/spatie/laravel-ignition). For Symfony apps, go to [symfony-ignition-bundle](https://github.com/spatie/symfony-ignition-bundle). For Drupal 10+ websites, use the [Ignition module](https://www.drupal.org/project/ignition). For all other PHP projects, install the package via composer: ```bash composer require spatie/ignition ``` ## Usage In order to display the Ignition error page when an error occurs in your project, you must add this code. Typically, this would be done in the bootstrap part of your application. ```php \Spatie\Ignition\Ignition::make()->register(); ``` ### Setting the application path When setting the application path, Ignition will trim the given value from all paths. This will make the error page look more cleaner. ```php \Spatie\Ignition\Ignition::make() ->applicationPath($basePathOfYourApplication) ->register(); ``` ### Using dark mode By default, Ignition uses a nice white based theme. If this is too bright for your eyes, you can use dark mode. ```php \Spatie\Ignition\Ignition::make() ->useDarkMode() ->register(); ``` ### Avoid rendering Ignition in a production environment You don't want to render the Ignition error page in a production environment, as it potentially can display sensitive information. To avoid rendering Ignition, you can call `shouldDisplayException` and pass it a falsy value. ```php \Spatie\Ignition\Ignition::make() ->shouldDisplayException($inLocalEnvironment) ->register(); ``` ### Displaying solutions In addition to displaying an exception, Ignition can display a solution as well. Out of the box, Ignition will display solutions for common errors such as bad methods calls, or using undefined properties. #### Adding a solution directly to an exception To add a solution text to your exception, let the exception implement the `Spatie\Ignition\Contracts\ProvidesSolution` interface. This interface requires you to implement one method, which is going to return the `Solution` that users will see when the exception gets thrown. ```php use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\Contracts\ProvidesSolution; class CustomException extends Exception implements ProvidesSolution { public function getSolution(): Solution { return new CustomSolution(); } } ``` ```php use Spatie\Ignition\Contracts\Solution; class CustomSolution implements Solution { public function getSolutionTitle(): string { return 'The solution title goes here'; } public function getSolutionDescription(): string { return 'This is a longer description of the solution that you want to show.'; } public function getDocumentationLinks(): array { return [ 'Your documentation' => 'https://your-project.com/relevant-docs-page', ]; } } ``` This is how the exception would be displayed if you were to throw it. 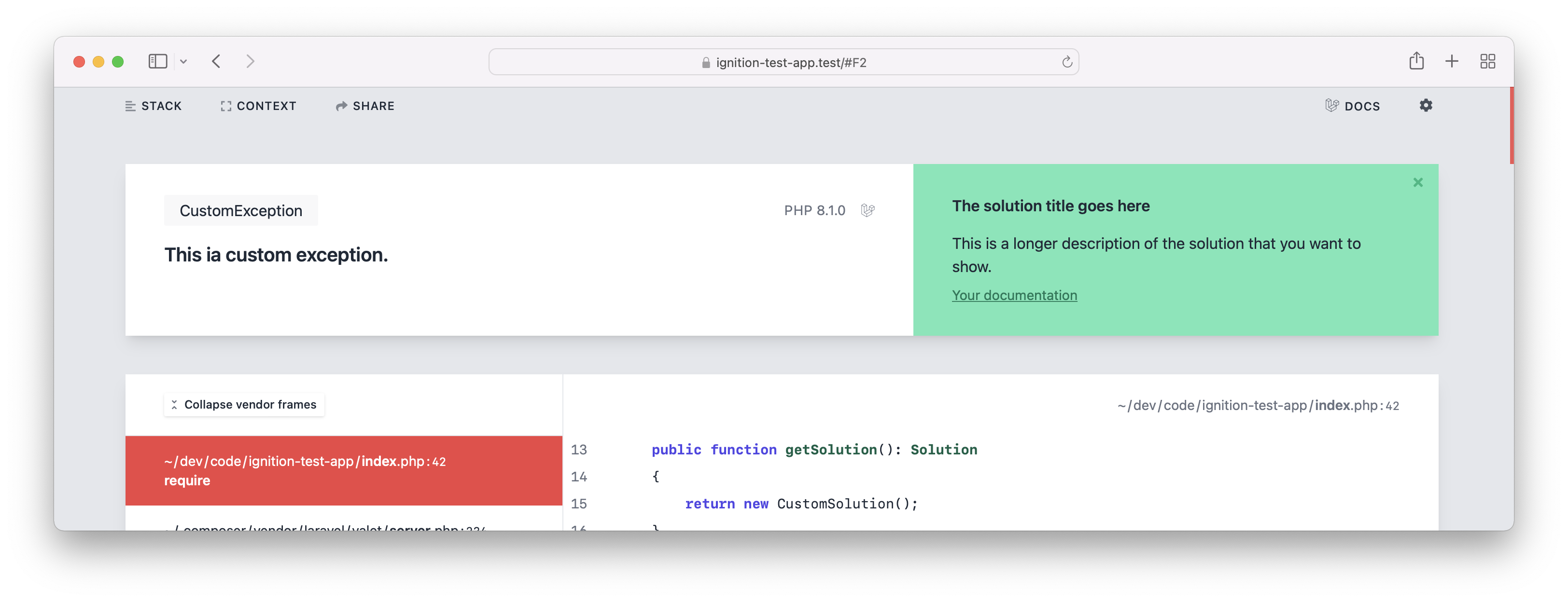 #### Using solution providers Instead of adding solutions to exceptions directly, you can also create a solution provider. While exceptions that return a solution, provide the solution directly to Ignition, a solution provider allows you to figure out if an exception can be solved. For example, you could create a custom "Stack Overflow solution provider", that will look up if a solution can be found for a given throwable. Solution providers can be added by third party packages or within your own application. A solution provider is any class that implements the \Spatie\Ignition\Contracts\HasSolutionsForThrowable interface. This is how the interface looks like: ```php interface HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool; /** @return \Spatie\Ignition\Contracts\Solution[] */ public function getSolutions(Throwable $throwable): array; } ``` When an error occurs in your app, the class will receive the `Throwable` in the `canSolve` method. In that method you can decide if your solution provider is applicable to the `Throwable` passed. If you return `true`, `getSolutions` will get called. To register a solution provider to Ignition you must call the `addSolutionProviders` method. ```php \Spatie\Ignition\Ignition::make() ->addSolutionProviders([ YourSolutionProvider::class, AnotherSolutionProvider::class, ]) ->register(); ``` ### AI powered solutions Ignition can send your exception to Open AI that will attempt to automatically suggest a solution. In many cases, the suggested solutions is quite useful, but keep in mind that the solution may not be 100% correct for your context. To generate AI powered solutions, you must first install this optional dependency. ```bash composer require openai-php/client ``` To start sending your errors to OpenAI, you must instanciate the `OpenAiSolutionProvider`. The constructor expects a OpenAI API key to be passed, you should generate this key [at OpenAI](https://platform.openai.com). ```php use \Spatie\Ignition\Solutions\OpenAi\OpenAiSolutionProvider; $aiSolutionProvider = new OpenAiSolutionProvider($openAiKey); ``` To use the solution provider, you should pass it to `addSolutionProviders` when registering Ignition. ```php \Spatie\Ignition\Ignition::make() ->addSolutionProviders([ $aiSolutionProvider, // other solution providers... ]) ->register(); ``` By default, the solution provider will send these bits of info to Open AI: - the error message - the error class - the stack frame - other small bits of info of context surrounding your error It will not send the request payload or any environment variables to avoid sending sensitive data to OpenAI. #### Caching requests to AI By default, all errors will be sent to OpenAI. Optionally, you can add caching so similar errors will only get sent to OpenAI once. To cache errors, you can call `useCache` on `$aiSolutionProvider`. You should pass [a simple-cache-implementation](https://packagist.org/providers/psr/simple-cache-implementation). Here's the signature of the `useCache` method. ```php public function useCache(CacheInterface $cache, int $cacheTtlInSeconds = 60 * 60) ``` #### Hinting the application type To increase the quality of the suggested solutions, you can send along the application type (Symfony, Drupal, WordPress, ...) to the AI. To send the application type call `applicationType` on the solution provider. ```php $aiSolutionProvider->applicationType('WordPress 6.2') ``` ### Sending exceptions to Flare Ignition comes with the ability to send exceptions to [Flare](https://flareapp.io), an exception monitoring service. Flare can notify you when new exceptions are occurring in your production environment. To send exceptions to Flare, simply call the `sendToFlareMethod` and pass it the API key you got when creating a project on Flare. You probably want to combine this with calling `runningInProductionEnvironment`. That method will, when passed a truthy value, not display the Ignition error page, but only send the exception to Flare. ```php \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->register(); ``` When you pass a falsy value to `runningInProductionEnvironment`, the Ignition error page will get shown, but no exceptions will be sent to Flare. ### Sending custom context to Flare When you send an error to Flare, you can add custom information that will be sent along with every exception that happens in your application. This can be very useful if you want to provide key-value related information that furthermore helps you to debug a possible exception. ```php use Spatie\FlareClient\Flare; \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->configureFlare(function(Flare $flare) { $flare->context('Tenant', 'My-Tenant-Identifier'); }) ->register(); ``` Sometimes you may want to group your context items by a key that you provide to have an easier visual differentiation when you look at your custom context items. The Flare client allows you to also provide your own custom context groups like this: ```php use Spatie\FlareClient\Flare; \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->configureFlare(function(Flare $flare) { $flare->group('Custom information', [ 'key' => 'value', 'another key' => 'another value', ]); }) ->register(); ``` ### Anonymize request to Flare By default, the Ignition collects information about the IP address of your application users. If you don't want to send this information to Flare, call `anonymizeIp()`. ```php use Spatie\FlareClient\Flare; \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->configureFlare(function(Flare $flare) { $flare->anonymizeIp(); }) ->register(); ``` ### Censoring request body fields When an exception occurs in a web request, the Flare client will pass on any request fields that are present in the body. In some cases, such as a login page, these request fields may contain a password that you don't want to send to Flare. To censor out values of certain fields, you can use `censorRequestBodyFields`. You should pass it the names of the fields you wish to censor. ```php use Spatie\FlareClient\Flare; \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->configureFlare(function(Flare $flare) { $flare->censorRequestBodyFields(['password']); }) ->register(); ``` This will replace the value of any sent fields named "password" with the value "<CENSORED>". ### Using middleware to modify data sent to Flare Before Flare receives the data that was collected from your local exception, we give you the ability to call custom middleware methods. These methods retrieve the report that should be sent to Flare and allow you to add custom information to that report. A valid middleware is any class that implements `FlareMiddleware`. ```php use Spatie\FlareClient\Report; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; class MyMiddleware implements FlareMiddleware { public function handle(Report $report, Closure $next) { $report->message("{$report->getMessage()}, now modified"); return $next($report); } } ``` ```php use Spatie\FlareClient\Flare; \Spatie\Ignition\Ignition::make() ->runningInProductionEnvironment($boolean) ->sendToFlare($yourApiKey) ->configureFlare(function(Flare $flare) { $flare->registerMiddleware([ MyMiddleware::class, ]) }) ->register(); ``` ### Changelog Please see [CHANGELOG](CHANGELOG.md) for more information about what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Dev setup Here are the steps you'll need to perform if you want to work on the UI of Ignition. - clone (or move) `spatie/ignition`, `spatie/ignition-ui`, `spatie/laravel-ignition`, `spatie/flare-client-php` and `spatie/ignition-test` into the same directory (e.g. `~/code/flare`) - create a new `package.json` file in `~/code/flare` directory: ```json { "private": true, "workspaces": [ "ignition-ui", "ignition" ] } ``` - run `yarn install` in the `~/code/flare` directory - in the `~/code/flare/ignition-test` directory - run `composer update` - run `cp .env.example .env` - run `php artisan key:generate` - run `yarn dev` in both the `ignition` and `ignition-ui` project - http://ignition-test.test/ should now work (= show the new UI). If you use valet, you might want to run `valet park` inside the `~/code/flare` directory. - http://ignition-test.test/ has a bit of everything - http://ignition-test.test/sql-error has a solution and SQL exception ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Spatie](https://spatie.be) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. ignition/src/Config/IgnitionConfig.php 0000644 00000015312 15002154456 0014003 0 ustar 00 <?php namespace Spatie\Ignition\Config; use Illuminate\Contracts\Support\Arrayable; use Spatie\Ignition\Contracts\ConfigManager; use Throwable; /** @implements Arrayable<string, string|null|bool|array<string, mixed>> */ class IgnitionConfig implements Arrayable { private ConfigManager $manager; public static function loadFromConfigFile(): self { return (new self())->loadConfigFile(); } /** * @param array<string, mixed> $options */ public function __construct(protected array $options = []) { $defaultOptions = $this->getDefaultOptions(); $this->options = array_merge($defaultOptions, $options); $this->manager = $this->initConfigManager(); } public function setOption(string $name, string $value): self { $this->options[$name] = $value; return $this; } private function initConfigManager(): ConfigManager { try { /** @phpstan-ignore-next-line */ return app(ConfigManager::class); } catch (Throwable) { return new FileConfigManager(); } } /** @param array<string, string> $options */ public function merge(array $options): self { $this->options = array_merge($this->options, $options); return $this; } public function loadConfigFile(): self { $this->merge($this->getConfigOptions()); return $this; } /** @return array<string, mixed> */ public function getConfigOptions(): array { return $this->manager->load(); } /** * @param array<string, mixed> $options * @return bool */ public function saveValues(array $options): bool { return $this->manager->save($options); } public function hideSolutions(): bool { return $this->options['hide_solutions'] ?? false; } public function editor(): ?string { return $this->options['editor'] ?? null; } /** * @return array<string, mixed> $options */ public function editorOptions(): array { return $this->options['editor_options'] ?? []; } public function remoteSitesPath(): ?string { return $this->options['remote_sites_path'] ?? null; } public function localSitesPath(): ?string { return $this->options['local_sites_path'] ?? null; } public function theme(): ?string { return $this->options['theme'] ?? null; } public function shareButtonEnabled(): bool { return (bool)($this->options['enable_share_button'] ?? false); } public function shareEndpoint(): string { return $this->options['share_endpoint'] ?? $this->getDefaultOptions()['share_endpoint']; } public function runnableSolutionsEnabled(): bool { return (bool)($this->options['enable_runnable_solutions'] ?? false); } /** @return array<string, string|null|bool|array<string, mixed>> */ public function toArray(): array { return [ 'editor' => $this->editor(), 'theme' => $this->theme(), 'hideSolutions' => $this->hideSolutions(), 'remoteSitesPath' => $this->remoteSitesPath(), 'localSitesPath' => $this->localSitesPath(), 'enableShareButton' => $this->shareButtonEnabled(), 'enableRunnableSolutions' => $this->runnableSolutionsEnabled(), 'directorySeparator' => DIRECTORY_SEPARATOR, 'editorOptions' => $this->editorOptions(), 'shareEndpoint' => $this->shareEndpoint(), ]; } /** * @return array<string, mixed> $options */ protected function getDefaultOptions(): array { return [ 'share_endpoint' => 'https://flareapp.io/api/public-reports', 'theme' => 'light', 'editor' => 'vscode', 'editor_options' => [ 'clipboard' => [ 'label' => 'Clipboard', 'url' => '%path:%line', 'clipboard' => true, ], 'sublime' => [ 'label' => 'Sublime', 'url' => 'subl://open?url=file://%path&line=%line', ], 'textmate' => [ 'label' => 'TextMate', 'url' => 'txmt://open?url=file://%path&line=%line', ], 'emacs' => [ 'label' => 'Emacs', 'url' => 'emacs://open?url=file://%path&line=%line', ], 'macvim' => [ 'label' => 'MacVim', 'url' => 'mvim://open/?url=file://%path&line=%line', ], 'phpstorm' => [ 'label' => 'PhpStorm', 'url' => 'phpstorm://open?file=%path&line=%line', ], 'phpstorm-remote' => [ 'label' => 'PHPStorm Remote', 'url' => 'javascript:r = new XMLHttpRequest;r.open("get", "http://localhost:63342/api/file/%path:%line");r.send()', ], 'idea' => [ 'label' => 'Idea', 'url' => 'idea://open?file=%path&line=%line', ], 'vscode' => [ 'label' => 'VS Code', 'url' => 'vscode://file/%path:%line', ], 'vscode-insiders' => [ 'label' => 'VS Code Insiders', 'url' => 'vscode-insiders://file/%path:%line', ], 'vscode-remote' => [ 'label' => 'VS Code Remote', 'url' => 'vscode://vscode-remote/%path:%line', ], 'vscode-insiders-remote' => [ 'label' => 'VS Code Insiders Remote', 'url' => 'vscode-insiders://vscode-remote/%path:%line', ], 'vscodium' => [ 'label' => 'VS Codium', 'url' => 'vscodium://file/%path:%line', ], 'atom' => [ 'label' => 'Atom', 'url' => 'atom://core/open/file?filename=%path&line=%line', ], 'nova' => [ 'label' => 'Nova', 'url' => 'nova://open?path=%path&line=%line', ], 'netbeans' => [ 'label' => 'NetBeans', 'url' => 'netbeans://open/?f=%path:%line', ], 'xdebug' => [ 'label' => 'Xdebug', 'url' => 'xdebug://%path@%line', ], ], ]; } } ignition/src/Config/FileConfigManager.php 0000644 00000006677 15002154456 0014413 0 ustar 00 <?php namespace Spatie\Ignition\Config; use Spatie\Ignition\Contracts\ConfigManager; use Throwable; class FileConfigManager implements ConfigManager { private const SETTINGS_FILE_NAME = '.ignition.json'; private string $path; private string $file; public function __construct(string $path = '') { $this->path = $this->initPath($path); $this->file = $this->initFile(); } protected function initPath(string $path): string { $path = $this->retrievePath($path); if (! $this->isValidWritablePath($path)) { return ''; } return $this->preparePath($path); } protected function retrievePath(string $path): string { if ($path !== '') { return $path; } return $this->initPathFromEnvironment(); } protected function isValidWritablePath(string $path): bool { return @file_exists($path) && @is_writable($path); } protected function preparePath(string $path): string { return rtrim($path, DIRECTORY_SEPARATOR); } protected function initPathFromEnvironment(): string { if (! empty($_SERVER['HOMEDRIVE']) && ! empty($_SERVER['HOMEPATH'])) { return $_SERVER['HOMEDRIVE'] . $_SERVER['HOMEPATH']; } if (! empty(getenv('HOME'))) { return getenv('HOME'); } return ''; } protected function initFile(): string { return $this->path . DIRECTORY_SEPARATOR . self::SETTINGS_FILE_NAME; } /** {@inheritDoc} */ public function load(): array { return $this->readFromFile(); } /** @return array<string, mixed> */ protected function readFromFile(): array { if (! $this->isValidFile()) { return []; } $content = (string)file_get_contents($this->file); $settings = json_decode($content, true) ?? []; return $settings; } protected function isValidFile(): bool { return $this->isValidPath() && @file_exists($this->file) && @is_writable($this->file); } protected function isValidPath(): bool { return trim($this->path) !== ''; } /** {@inheritDoc} */ public function save(array $options): bool { if (! $this->createFile()) { return false; } return $this->saveToFile($options); } protected function createFile(): bool { if (! $this->isValidPath()) { return false; } if (@file_exists($this->file)) { return true; } return (file_put_contents($this->file, '') !== false); } /** * @param array<string, mixed> $options * * @return bool */ protected function saveToFile(array $options): bool { try { $content = json_encode($options, JSON_THROW_ON_ERROR); } catch (Throwable) { return false; } return $this->writeToFile($content); } protected function writeToFile(string $content): bool { if (! $this->isValidFile()) { return false; } return (file_put_contents($this->file, $content) !== false); } /** {@inheritDoc} */ public function getPersistentInfo(): array { return [ 'name' => self::SETTINGS_FILE_NAME, 'path' => $this->path, 'file' => $this->file, ]; } } ignition/src/Solutions/SuggestCorrectVariableNameSolution.php 0000644 00000001576 15002154456 0020645 0 ustar 00 <?php namespace Spatie\Ignition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestCorrectVariableNameSolution implements Solution { protected ?string $variableName; protected ?string $viewFile; protected ?string $suggested; public function __construct(string $variableName = null, string $viewFile = null, string $suggested = null) { $this->variableName = $variableName; $this->viewFile = $viewFile; $this->suggested = $suggested; } public function getSolutionTitle(): string { return 'Possible typo $'.$this->variableName; } public function getDocumentationLinks(): array { return []; } public function getSolutionDescription(): string { return "Did you mean `$$this->suggested`?"; } public function isRunnable(): bool { return false; } } ignition/src/Solutions/SuggestImportSolution.php 0000644 00000001140 15002154456 0016232 0 ustar 00 <?php namespace Spatie\Ignition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestImportSolution implements Solution { protected string $class; public function __construct(string $class) { $this->class = $class; } public function getSolutionTitle(): string { return 'A class import is missing'; } public function getSolutionDescription(): string { return 'You have a missing class import. Try importing this class: `'.$this->class.'`.'; } public function getDocumentationLinks(): array { return []; } } ignition/src/Solutions/SolutionProviders/BadMethodCallSolutionProvider.php 0000644 00000005233 15002154456 0023275 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\SolutionProviders; use BadMethodCallException; use Illuminate\Support\Collection; use ReflectionClass; use ReflectionMethod; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class BadMethodCallSolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/([a-zA-Z\\\\]+)::([a-zA-Z]+)/m'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof BadMethodCallException) { return false; } if (is_null($this->getClassAndMethodFromExceptionMessage($throwable->getMessage()))) { return false; } return true; } public function getSolutions(Throwable $throwable): array { return [ BaseSolution::create('Bad Method Call') ->setSolutionDescription($this->getSolutionDescription($throwable)), ]; } public function getSolutionDescription(Throwable $throwable): string { if (! $this->canSolve($throwable)) { return ''; } /** @phpstan-ignore-next-line */ extract($this->getClassAndMethodFromExceptionMessage($throwable->getMessage()), EXTR_OVERWRITE); $possibleMethod = $this->findPossibleMethod($class ?? '', $method ?? ''); $class ??= 'UnknownClass'; return "Did you mean {$class}::{$possibleMethod?->name}() ?"; } /** * @param string $message * * @return null|array<string, mixed> */ protected function getClassAndMethodFromExceptionMessage(string $message): ?array { if (! preg_match(self::REGEX, $message, $matches)) { return null; } return [ 'class' => $matches[1], 'method' => $matches[2], ]; } /** * @param class-string $class * @param string $invalidMethodName * * @return \ReflectionMethod|null */ protected function findPossibleMethod(string $class, string $invalidMethodName): ?ReflectionMethod { return $this->getAvailableMethods($class) ->sortByDesc(function (ReflectionMethod $method) use ($invalidMethodName) { similar_text($invalidMethodName, $method->name, $percentage); return $percentage; })->first(); } /** * @param class-string $class * * @return \Illuminate\Support\Collection<int, ReflectionMethod> */ protected function getAvailableMethods(string $class): Collection { $class = new ReflectionClass($class); return Collection::make($class->getMethods()); } } ignition/src/Solutions/SolutionProviders/SolutionProviderRepository.php 0000644 00000006547 15002154456 0023042 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\SolutionProviders; use Illuminate\Support\Collection; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\Contracts\SolutionProviderRepository as SolutionProviderRepositoryContract; use Throwable; class SolutionProviderRepository implements SolutionProviderRepositoryContract { /** @var Collection<int, class-string<HasSolutionsForThrowable>|HasSolutionsForThrowable> */ protected Collection $solutionProviders; /** @param array<int, class-string<HasSolutionsForThrowable>|HasSolutionsForThrowable> $solutionProviders */ public function __construct(array $solutionProviders = []) { $this->solutionProviders = Collection::make($solutionProviders); } public function registerSolutionProvider(string|HasSolutionsForThrowable $solutionProvider): SolutionProviderRepositoryContract { $this->solutionProviders->push($solutionProvider); return $this; } public function registerSolutionProviders(array $solutionProviderClasses): SolutionProviderRepositoryContract { $this->solutionProviders = $this->solutionProviders->merge($solutionProviderClasses); return $this; } public function getSolutionsForThrowable(Throwable $throwable): array { $solutions = []; if ($throwable instanceof Solution) { $solutions[] = $throwable; } if ($throwable instanceof ProvidesSolution) { $solutions[] = $throwable->getSolution(); } $providedSolutions = $this ->initialiseSolutionProviderRepositories() ->filter(function (HasSolutionsForThrowable $solutionProvider) use ($throwable) { try { return $solutionProvider->canSolve($throwable); } catch (Throwable $exception) { return false; } }) ->map(function (HasSolutionsForThrowable $solutionProvider) use ($throwable) { try { return $solutionProvider->getSolutions($throwable); } catch (Throwable $exception) { return []; } }) ->flatten() ->toArray(); return array_merge($solutions, $providedSolutions); } public function getSolutionForClass(string $solutionClass): ?Solution { if (! class_exists($solutionClass)) { return null; } if (! in_array(Solution::class, class_implements($solutionClass) ?: [])) { return null; } if (! function_exists('app')) { return null; } return app($solutionClass); } /** @return Collection<int, HasSolutionsForThrowable> */ protected function initialiseSolutionProviderRepositories(): Collection { return $this->solutionProviders ->filter(fn (HasSolutionsForThrowable|string $provider) => in_array(HasSolutionsForThrowable::class, class_implements($provider) ?: [])) ->map(function (string|HasSolutionsForThrowable $provider): HasSolutionsForThrowable { if (is_string($provider)) { return new $provider; } return $provider; }); } } ignition/src/Solutions/SolutionProviders/UndefinedPropertySolutionProvider.php 0000644 00000006726 15002154456 0024330 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\SolutionProviders; use ErrorException; use Illuminate\Support\Collection; use ReflectionClass; use ReflectionProperty; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class UndefinedPropertySolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/([a-zA-Z\\\\]+)::\$([a-zA-Z]+)/m'; protected const MINIMUM_SIMILARITY = 80; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof ErrorException) { return false; } if (is_null($this->getClassAndPropertyFromExceptionMessage($throwable->getMessage()))) { return false; } if (! $this->similarPropertyExists($throwable)) { return false; } return true; } public function getSolutions(Throwable $throwable): array { return [ BaseSolution::create('Unknown Property') ->setSolutionDescription($this->getSolutionDescription($throwable)), ]; } public function getSolutionDescription(Throwable $throwable): string { if (! $this->canSolve($throwable) || ! $this->similarPropertyExists($throwable)) { return ''; } extract( /** @phpstan-ignore-next-line */ $this->getClassAndPropertyFromExceptionMessage($throwable->getMessage()), EXTR_OVERWRITE, ); $possibleProperty = $this->findPossibleProperty($class ?? '', $property ?? ''); $class = $class ?? ''; return "Did you mean {$class}::\${$possibleProperty->name} ?"; } protected function similarPropertyExists(Throwable $throwable): bool { /** @phpstan-ignore-next-line */ extract($this->getClassAndPropertyFromExceptionMessage($throwable->getMessage()), EXTR_OVERWRITE); $possibleProperty = $this->findPossibleProperty($class ?? '', $property ?? ''); return $possibleProperty !== null; } /** * @param string $message * * @return null|array<string, string> */ protected function getClassAndPropertyFromExceptionMessage(string $message): ?array { if (! preg_match(self::REGEX, $message, $matches)) { return null; } return [ 'class' => $matches[1], 'property' => $matches[2], ]; } /** * @param class-string $class * @param string $invalidPropertyName * * @return mixed */ protected function findPossibleProperty(string $class, string $invalidPropertyName): mixed { return $this->getAvailableProperties($class) ->sortByDesc(function (ReflectionProperty $property) use ($invalidPropertyName) { similar_text($invalidPropertyName, $property->name, $percentage); return $percentage; }) ->filter(function (ReflectionProperty $property) use ($invalidPropertyName) { similar_text($invalidPropertyName, $property->name, $percentage); return $percentage >= self::MINIMUM_SIMILARITY; })->first(); } /** * @param class-string $class * * @return Collection<int, ReflectionProperty> */ protected function getAvailableProperties(string $class): Collection { $class = new ReflectionClass($class); return Collection::make($class->getProperties()); } } ignition/src/Solutions/SolutionProviders/MergeConflictSolutionProvider.php 0000644 00000004070 15002154456 0023371 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\SolutionProviders; use Illuminate\Support\Str; use ParseError; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class MergeConflictSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { if (! ($throwable instanceof ParseError)) { return false; } if (! $this->hasMergeConflictExceptionMessage($throwable)) { return false; } $file = (string)file_get_contents($throwable->getFile()); if (! str_contains($file, '=======')) { return false; } if (! str_contains($file, '>>>>>>>')) { return false; } return true; } public function getSolutions(Throwable $throwable): array { $file = (string)file_get_contents($throwable->getFile()); preg_match('/\>\>\>\>\>\>\> (.*?)\n/', $file, $matches); $source = $matches[1]; $target = $this->getCurrentBranch(basename($throwable->getFile())); return [ BaseSolution::create("Merge conflict from branch '$source' into $target") ->setSolutionDescription('You have a Git merge conflict. To undo your merge do `git reset --hard HEAD`'), ]; } protected function getCurrentBranch(string $directory): string { $branch = "'".trim((string)shell_exec("cd {$directory}; git branch | grep \\* | cut -d ' ' -f2"))."'"; if ($branch === "''") { $branch = 'current branch'; } return $branch; } protected function hasMergeConflictExceptionMessage(Throwable $throwable): bool { // For PHP 7.x and below if (Str::startsWith($throwable->getMessage(), 'syntax error, unexpected \'<<\'')) { return true; } // For PHP 8+ if (Str::startsWith($throwable->getMessage(), 'syntax error, unexpected token "<<"')) { return true; } return false; } } ignition/src/Solutions/SolutionTransformer.php 0000644 00000001570 15002154456 0015727 0 ustar 00 <?php namespace Spatie\Ignition\Solutions; use Illuminate\Contracts\Support\Arrayable; use Spatie\Ignition\Contracts\Solution; /** @implements Arrayable<string, array<string,string>|string|false> */ class SolutionTransformer implements Arrayable { protected Solution $solution; public function __construct(Solution $solution) { $this->solution = $solution; } /** @return array<string, array<string,string>|string|false> */ public function toArray(): array { return [ 'class' => get_class($this->solution), 'title' => $this->solution->getSolutionTitle(), 'links' => $this->solution->getDocumentationLinks(), 'description' => $this->solution->getSolutionDescription(), 'is_runnable' => false, 'ai_generated' => $this->solution->aiGenerated ?? false, ]; } } ignition/src/Solutions/OpenAi/OpenAiSolutionResponse.php 0000644 00000002635 15002154456 0017475 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\OpenAi; class OpenAiSolutionResponse { protected string $rawText; public function __construct(string $rawText) { $this->rawText = trim($rawText); } public function description(): string { return $this->between('FIX', 'ENDFIX', $this->rawText); } public function links(): array { $textLinks = $this->between('LINKS', 'ENDLINKS', $this->rawText); $textLinks = explode(PHP_EOL, $textLinks); $textLinks = array_map(function ($textLink) { $textLink = str_replace('\\', '\\\\', $textLink); $textLink = str_replace('\\\\\\', '\\\\', $textLink); return json_decode($textLink, true); }, $textLinks); array_filter($textLinks); $links = []; foreach ($textLinks as $textLink) { $links[$textLink['title']] = $textLink['url']; } return $links; } protected function between(string $start, string $end, string $text): string { $startPosition = strpos($text, $start); if ($startPosition === false) { return ""; } $startPosition += strlen($start); $endPosition = strpos($text, $end, $startPosition); if ($endPosition === false) { return ""; } return trim(substr($text, $startPosition, $endPosition - $startPosition)); } } ignition/src/Solutions/OpenAi/OpenAiPromptViewModel.php 0000644 00000001610 15002154456 0017227 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\OpenAi; class OpenAiPromptViewModel { public function __construct( protected string $file, protected string $exceptionMessage, protected string $exceptionClass, protected string $snippet, protected string $line, protected string|null $applicationType = null, ) { } public function file(): string { return $this->file; } public function line(): string { return $this->line; } public function snippet(): string { return $this->snippet; } public function exceptionMessage(): string { return $this->exceptionMessage; } public function exceptionClass(): string { return $this->exceptionClass; } public function applicationType(): string|null { return $this->applicationType; } } ignition/src/Solutions/OpenAi/OpenAiSolutionProvider.php 0000644 00000003011 15002154456 0017456 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\OpenAi; use Psr\SimpleCache\CacheInterface; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class OpenAiSolutionProvider implements HasSolutionsForThrowable { public function __construct( protected string $openAiKey, protected ?CacheInterface $cache = null, protected int $cacheTtlInSeconds = 60 * 60, protected string|null $applicationType = null, protected string|null $applicationPath = null, ) { $this->cache ??= new DummyCache(); } public function canSolve(Throwable $throwable): bool { return true; } public function getSolutions(Throwable $throwable): array { return [ new OpenAiSolution( $throwable, $this->openAiKey, $this->cache, $this->cacheTtlInSeconds, $this->applicationType, $this->applicationPath, ), ]; } public function applicationType(string $applicationType): self { $this->applicationType = $applicationType; return $this; } public function applicationPath(string $applicationPath): self { $this->applicationPath = $applicationPath; return $this; } public function useCache(CacheInterface $cache, int $cacheTtlInSeconds = 60 * 60): self { $this->cache = $cache; $this->cacheTtlInSeconds = $cacheTtlInSeconds; return $this; } } ignition/src/Solutions/OpenAi/OpenAiSolution.php 0000644 00000006345 15002154456 0015760 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\OpenAi; use OpenAI; use Psr\SimpleCache\CacheInterface; use Spatie\Backtrace\Backtrace; use Spatie\Backtrace\Frame; use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\ErrorPage\Renderer; use Spatie\Ignition\Ignition; use Throwable; class OpenAiSolution implements Solution { public bool $aiGenerated = true; protected string $prompt; protected OpenAiSolutionResponse $openAiSolutionResponse; public function __construct( protected Throwable $throwable, protected string $openAiKey, protected CacheInterface|null $cache = null, protected int|null $cacheTtlInSeconds = 60, protected string|null $applicationType = null, protected string|null $applicationPath = null, ) { $this->prompt = $this->generatePrompt(); $this->openAiSolutionResponse = $this->getAiSolution(); } public function getSolutionTitle(): string { return 'AI Generated Solution'; } public function getSolutionDescription(): string { return $this->openAiSolutionResponse->description(); } public function getDocumentationLinks(): array { return $this->openAiSolutionResponse->links(); } public function getAiSolution(): ?OpenAiSolutionResponse { $solution = $this->cache->get($this->getCacheKey()); if ($solution) { return new OpenAiSolutionResponse($solution); } $solutionText = OpenAI::client($this->openAiKey) ->chat() ->create([ 'model' => $this->getModel(), 'messages' => [['role' => 'user', 'content' => $this->prompt]], 'max_tokens' => 1000, 'temperature' => 0, ])->choices[0]->message->content; $this->cache->set($this->getCacheKey(), $solutionText, $this->cacheTtlInSeconds); return new OpenAiSolutionResponse($solutionText); } protected function getCacheKey(): string { $hash = sha1($this->prompt); return "ignition-solution-{$hash}"; } protected function generatePrompt(): string { $viewPath = Ignition::viewPath('aiPrompt'); $viewModel = new OpenAiPromptViewModel( file: $this->throwable->getFile(), exceptionMessage: $this->throwable->getMessage(), exceptionClass: get_class($this->throwable), snippet: $this->getApplicationFrame($this->throwable)->getSnippetAsString(15), line: $this->throwable->getLine(), applicationType: $this->applicationType, ); return (new Renderer())->renderAsString( ['viewModel' => $viewModel], $viewPath, ); } protected function getModel(): string { return 'gpt-3.5-turbo'; } protected function getApplicationFrame(Throwable $throwable): ?Frame { $backtrace = Backtrace::createForThrowable($throwable); if ($this->applicationPath) { $backtrace->applicationPath($this->applicationPath); } $frames = $backtrace->frames(); return $frames[$backtrace->firstApplicationFrameIndex()] ?? null; } } ignition/src/Solutions/OpenAi/DummyCache.php 0000644 00000001627 15002154456 0015065 0 ustar 00 <?php namespace Spatie\Ignition\Solutions\OpenAi; use Psr\SimpleCache\CacheInterface; class DummyCache implements CacheInterface { public function get(string $key, mixed $default = null): mixed { return null; } public function set(string $key, mixed $value, \DateInterval|int|null $ttl = null): bool { return true; } public function delete(string $key): bool { return true; } public function clear(): bool { return true; } public function getMultiple(iterable $keys, mixed $default = null): iterable { return []; } public function setMultiple(iterable $values, \DateInterval|int|null $ttl = null): bool { return true; } public function deleteMultiple(iterable $keys): bool { return true; } public function has(string $key): bool { return false; } } ignition/src/Contracts/HasSolutionsForThrowable.php 0000644 00000000522 15002154456 0016577 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; use Throwable; /** * Interface used for SolutionProviders. */ interface HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool; /** @return array<int, \Spatie\Ignition\Contracts\Solution> */ public function getSolutions(Throwable $throwable): array; } ignition/src/Contracts/ProvidesSolution.php 0000644 00000000310 15002154456 0015150 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; /** * Interface to be used on exceptions that provide their own solution. */ interface ProvidesSolution { public function getSolution(): Solution; } ignition/src/Contracts/ConfigManager.php 0000644 00000000516 15002154456 0014330 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; interface ConfigManager { /** @return array<string, mixed> */ public function load(): array; /** @param array<string, mixed> $options */ public function save(array $options): bool; /** @return array<string, mixed> */ public function getPersistentInfo(): array; } ignition/src/Contracts/RunnableSolution.php 0000644 00000000615 15002154456 0015133 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; interface RunnableSolution extends Solution { public function getSolutionActionDescription(): string; public function getRunButtonText(): string; /** @param array<string, mixed> $parameters */ public function run(array $parameters = []): void; /** @return array<string, mixed> */ public function getRunParameters(): array; } ignition/src/Contracts/BaseSolution.php 0000644 00000002556 15002154456 0014245 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; class BaseSolution implements Solution { protected string $title; protected string $description = ''; /** @var array<string, string> */ protected array $links = []; public static function create(string $title = ''): static { // It's important to keep the return type as static because // the old Facade Ignition contracts extend from this method. /** @phpstan-ignore-next-line */ return new static($title); } public function __construct(string $title = '') { $this->title = $title; } public function getSolutionTitle(): string { return $this->title; } public function setSolutionTitle(string $title): self { $this->title = $title; return $this; } public function getSolutionDescription(): string { return $this->description; } public function setSolutionDescription(string $description): self { $this->description = $description; return $this; } /** @return array<string, string> */ public function getDocumentationLinks(): array { return $this->links; } /** @param array<string, string> $links */ public function setDocumentationLinks(array $links): self { $this->links = $links; return $this; } } ignition/src/Contracts/SolutionProviderRepository.php 0000644 00000001602 15002154456 0017254 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; use Throwable; interface SolutionProviderRepository { /** * @param class-string<HasSolutionsForThrowable>|HasSolutionsForThrowable $solutionProvider * * @return $this */ public function registerSolutionProvider(string $solutionProvider): self; /** * @param array<class-string<HasSolutionsForThrowable>|HasSolutionsForThrowable> $solutionProviders * * @return $this */ public function registerSolutionProviders(array $solutionProviders): self; /** * @param Throwable $throwable * * @return array<int, Solution> */ public function getSolutionsForThrowable(Throwable $throwable): array; /** * @param class-string<Solution> $solutionClass * * @return null|Solution */ public function getSolutionForClass(string $solutionClass): ?Solution; } ignition/src/Contracts/Solution.php 0000644 00000000411 15002154456 0013436 0 ustar 00 <?php namespace Spatie\Ignition\Contracts; interface Solution { public function getSolutionTitle(): string; public function getSolutionDescription(): string; /** @return array<string, string> */ public function getDocumentationLinks(): array; } ignition/src/ErrorPage/ErrorPageViewModel.php 0000644 00000006600 15002154456 0015260 0 ustar 00 <?php namespace Spatie\Ignition\ErrorPage; use Spatie\FlareClient\Report; use Spatie\FlareClient\Truncation\ReportTrimmer; use Spatie\Ignition\Config\IgnitionConfig; use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\Solutions\SolutionTransformer; use Throwable; class ErrorPageViewModel { /** * @param \Throwable|null $throwable * @param \Spatie\Ignition\Config\IgnitionConfig $ignitionConfig * @param \Spatie\FlareClient\Report $report * @param array<int, Solution> $solutions * @param string|null $solutionTransformerClass */ public function __construct( protected ?Throwable $throwable, protected IgnitionConfig $ignitionConfig, protected Report $report, protected array $solutions, protected ?string $solutionTransformerClass = null, protected string $customHtmlHead = '', protected string $customHtmlBody = '' ) { $this->solutionTransformerClass ??= SolutionTransformer::class; } public function throwableString(): string { if (! $this->throwable) { return ''; } $throwableString = sprintf( "%s: %s in file %s on line %d\n\n%s\n", get_class($this->throwable), $this->throwable->getMessage(), $this->throwable->getFile(), $this->throwable->getLine(), $this->report->getThrowable()?->getTraceAsString() ); return htmlspecialchars($throwableString); } public function title(): string { return htmlspecialchars($this->report->getMessage()); } /** * @return array<string, mixed> */ public function config(): array { return $this->ignitionConfig->toArray(); } public function theme(): string { return $this->config()['theme'] ?? 'auto'; } /** * @return array<int, mixed> */ public function solutions(): array { return array_map(function (Solution $solution) { /** @var class-string $transformerClass */ $transformerClass = $this->solutionTransformerClass; /** @var SolutionTransformer $transformer */ $transformer = new $transformerClass($solution); return ($transformer)->toArray(); }, $this->solutions); } /** * @return array<string, mixed> */ public function report(): array { return $this->report->toArray(); } public function jsonEncode(mixed $data): string { $jsonOptions = JSON_PARTIAL_OUTPUT_ON_ERROR | JSON_HEX_TAG | JSON_HEX_APOS | JSON_HEX_AMP | JSON_HEX_QUOT; return (string) json_encode($data, $jsonOptions); } public function getAssetContents(string $asset): string { $assetPath = __DIR__."/../../resources/compiled/{$asset}"; return (string) file_get_contents($assetPath); } /** * @return array<int|string, mixed> */ public function shareableReport(): array { return (new ReportTrimmer())->trim($this->report()); } public function updateConfigEndpoint(): string { // TODO: Should be based on Ignition config return '/_ignition/update-config'; } public function customHtmlHead(): string { return $this->customHtmlHead; } public function customHtmlBody(): string { return $this->customHtmlBody; } } ignition/src/ErrorPage/Renderer.php 0000644 00000000771 15002154456 0013327 0 ustar 00 <?php namespace Spatie\Ignition\ErrorPage; class Renderer { /** * @param array<string, mixed> $data * * @return void */ public function render(array $data, string $viewPath): void { $viewFile = $viewPath; extract($data, EXTR_OVERWRITE); include $viewFile; } public function renderAsString(array $date, string $viewPath): string { ob_start(); $this->render($date, $viewPath); return ob_get_clean(); } } ignition/src/Ignition.php 0000644 00000023742 15002154456 0011456 0 ustar 00 <?php namespace Spatie\Ignition; use ArrayObject; use ErrorException; use Spatie\FlareClient\Context\BaseContextProviderDetector; use Spatie\FlareClient\Context\ContextProviderDetector; use Spatie\FlareClient\Enums\MessageLevels; use Spatie\FlareClient\Flare; use Spatie\FlareClient\FlareMiddleware\AddDocumentationLinks; use Spatie\FlareClient\FlareMiddleware\AddSolutions; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; use Spatie\Ignition\Config\IgnitionConfig; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\Ignition\Contracts\SolutionProviderRepository as SolutionProviderRepositoryContract; use Spatie\Ignition\ErrorPage\ErrorPageViewModel; use Spatie\Ignition\ErrorPage\Renderer; use Spatie\Ignition\Solutions\SolutionProviders\BadMethodCallSolutionProvider; use Spatie\Ignition\Solutions\SolutionProviders\MergeConflictSolutionProvider; use Spatie\Ignition\Solutions\SolutionProviders\SolutionProviderRepository; use Spatie\Ignition\Solutions\SolutionProviders\UndefinedPropertySolutionProvider; use Throwable; class Ignition { protected Flare $flare; protected bool $shouldDisplayException = true; protected string $flareApiKey = ''; protected string $applicationPath = ''; /** @var array<int, FlareMiddleware> */ protected array $middleware = []; protected IgnitionConfig $ignitionConfig; protected ContextProviderDetector $contextProviderDetector; protected SolutionProviderRepositoryContract $solutionProviderRepository; protected ?bool $inProductionEnvironment = null; protected ?string $solutionTransformerClass = null; /** @var ArrayObject<int, callable(Throwable): mixed> */ protected ArrayObject $documentationLinkResolvers; protected string $customHtmlHead = ''; protected string $customHtmlBody = ''; public static function make(): self { return new self(); } public function __construct() { $this->flare = Flare::make(); $this->ignitionConfig = IgnitionConfig::loadFromConfigFile(); $this->solutionProviderRepository = new SolutionProviderRepository($this->getDefaultSolutionProviders()); $this->documentationLinkResolvers = new ArrayObject(); $this->contextProviderDetector = new BaseContextProviderDetector(); $this->middleware[] = new AddSolutions($this->solutionProviderRepository); $this->middleware[] = new AddDocumentationLinks($this->documentationLinkResolvers); } public function setSolutionTransformerClass(string $solutionTransformerClass): self { $this->solutionTransformerClass = $solutionTransformerClass; return $this; } /** @param callable(Throwable): mixed $callable */ public function resolveDocumentationLink(callable $callable): self { $this->documentationLinkResolvers[] = $callable; return $this; } public function setConfig(IgnitionConfig $ignitionConfig): self { $this->ignitionConfig = $ignitionConfig; return $this; } public function runningInProductionEnvironment(bool $boolean = true): self { $this->inProductionEnvironment = $boolean; return $this; } public function getFlare(): Flare { return $this->flare; } public function setFlare(Flare $flare): self { $this->flare = $flare; return $this; } public function setSolutionProviderRepository(SolutionProviderRepositoryContract $solutionProviderRepository): self { $this->solutionProviderRepository = $solutionProviderRepository; return $this; } public function shouldDisplayException(bool $shouldDisplayException): self { $this->shouldDisplayException = $shouldDisplayException; return $this; } public function applicationPath(string $applicationPath): self { $this->applicationPath = $applicationPath; return $this; } /** * @param string $name * @param string $messageLevel * @param array<int, mixed> $metaData * * @return $this */ public function glow( string $name, string $messageLevel = MessageLevels::INFO, array $metaData = [] ): self { $this->flare->glow($name, $messageLevel, $metaData); return $this; } /** * @param array<int, HasSolutionsForThrowable|class-string<HasSolutionsForThrowable>> $solutionProviders * * @return $this */ public function addSolutionProviders(array $solutionProviders): self { $this->solutionProviderRepository->registerSolutionProviders($solutionProviders); return $this; } /** @deprecated Use `setTheme('dark')` instead */ public function useDarkMode(): self { return $this->setTheme('dark'); } /** @deprecated Use `setTheme($theme)` instead */ public function theme(string $theme): self { return $this->setTheme($theme); } public function setTheme(string $theme): self { $this->ignitionConfig->setOption('theme', $theme); return $this; } public function setEditor(string $editor): self { $this->ignitionConfig->setOption('editor', $editor); return $this; } public function sendToFlare(?string $apiKey): self { $this->flareApiKey = $apiKey ?? ''; return $this; } public function configureFlare(callable $callable): self { ($callable)($this->flare); return $this; } /** * @param FlareMiddleware|array<int, FlareMiddleware> $middleware * * @return $this */ public function registerMiddleware(array|FlareMiddleware $middleware): self { if (! is_array($middleware)) { $middleware = [$middleware]; } foreach ($middleware as $singleMiddleware) { $this->middleware = array_merge($this->middleware, $middleware); } return $this; } public function setContextProviderDetector(ContextProviderDetector $contextProviderDetector): self { $this->contextProviderDetector = $contextProviderDetector; return $this; } public function reset(): self { $this->flare->reset(); return $this; } public function register(): self { error_reporting(-1); /** @phpstan-ignore-next-line */ set_error_handler([$this, 'renderError']); /** @phpstan-ignore-next-line */ set_exception_handler([$this, 'handleException']); return $this; } /** * @param int $level * @param string $message * @param string $file * @param int $line * @param array<int, mixed> $context * * @return void * @throws \ErrorException */ public function renderError( int $level, string $message, string $file = '', int $line = 0, array $context = [] ): void { throw new ErrorException($message, 0, $level, $file, $line); } /** * This is the main entry point for the framework agnostic Ignition package. * Displays the Ignition page and optionally sends a report to Flare. */ public function handleException(Throwable $throwable): Report { $this->setUpFlare(); $report = $this->createReport($throwable); if ($this->shouldDisplayException && $this->inProductionEnvironment !== true) { $this->renderException($throwable, $report); } if ($this->flare->apiTokenSet() && $this->inProductionEnvironment !== false) { $this->flare->report($throwable, report: $report); } return $report; } /** * This is the main entrypoint for laravel-ignition. It only renders the exception. * Sending the report to Flare is handled in the laravel-ignition log handler. */ public function renderException(Throwable $throwable, ?Report $report = null): void { $this->setUpFlare(); $report ??= $this->createReport($throwable); $viewModel = new ErrorPageViewModel( $throwable, $this->ignitionConfig, $report, $this->solutionProviderRepository->getSolutionsForThrowable($throwable), $this->solutionTransformerClass, $this->customHtmlHead, $this->customHtmlBody, ); (new Renderer())->render(['viewModel' => $viewModel], self::viewPath('errorPage')); } public static function viewPath(string $viewName): string { return __DIR__ . "/../resources/views/{$viewName}.php"; } /** * Add custom HTML which will be added to the head tag of the error page. */ public function addCustomHtmlToHead(string $html): self { $this->customHtmlHead .= $html; return $this; } /** * Add custom HTML which will be added to the body tag of the error page. */ public function addCustomHtmlToBody(string $html): self { $this->customHtmlBody .= $html; return $this; } protected function setUpFlare(): self { if (! $this->flare->apiTokenSet()) { $this->flare->setApiToken($this->flareApiKey ?? ''); } $this->flare->setContextProviderDetector($this->contextProviderDetector); foreach ($this->middleware as $singleMiddleware) { $this->flare->registerMiddleware($singleMiddleware); } if ($this->applicationPath !== '') { $this->flare->applicationPath($this->applicationPath); } return $this; } /** @return array<class-string<HasSolutionsForThrowable>> */ protected function getDefaultSolutionProviders(): array { return [ BadMethodCallSolutionProvider::class, MergeConflictSolutionProvider::class, UndefinedPropertySolutionProvider::class, ]; } protected function createReport(Throwable $throwable): Report { return $this->flare->createReport($throwable); } } ignition/LICENSE.md 0000644 00000002075 15002154456 0007776 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-backup/composer.json 0000644 00000005407 15002154456 0012167 0 ustar 00 { "name": "spatie/laravel-backup", "description": "A Laravel package to backup your application", "keywords": [ "spatie", "backup", "database", "laravel-backup" ], "homepage": "https://github.com/spatie/laravel-backup", "license": "MIT", "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^8.0", "ext-zip": "^1.14.0", "illuminate/console": "^9.0|^10.0", "illuminate/contracts": "^9.0|^10.0", "illuminate/events": "^9.0|^10.0", "illuminate/filesystem": "^9.0|^10.0", "illuminate/notifications": "^9.0|^10.0", "illuminate/support": "^9.0|^10.0", "league/flysystem": "^3.0", "spatie/db-dumper": "^3.0", "spatie/laravel-package-tools": "^1.6.2", "spatie/laravel-signal-aware-command": "^1.2", "spatie/temporary-directory": "^2.0", "symfony/console": "^6.0", "symfony/finder": "^6.0" }, "require-dev": { "ext-pcntl": "*", "composer-runtime-api": "^2.0", "laravel/slack-notification-channel": "^2.5", "league/flysystem-aws-s3-v3": "^2.0|^3.0", "mockery/mockery": "^1.4", "nunomaduro/larastan": "^2.1", "orchestra/testbench": "^7.0|^8.0", "pestphp/pest": "^1.20", "phpstan/extension-installer": "^1.1", "phpstan/phpstan-deprecation-rules": "^1.0", "phpstan/phpstan-phpunit": "^1.1" }, "autoload": { "psr-4": { "Spatie\\Backup\\": "src" }, "files": [ "src/Helpers/functions.php" ] }, "autoload-dev": { "psr-4": { "Spatie\\Backup\\Tests\\": "tests" } }, "scripts": { "test": "vendor/bin/pest", "format": "vendor/bin/php-cs-fixer fix --allow-risky=yes", "analyse": "vendor/bin/phpstan analyse" }, "suggest": { "laravel/slack-notification-channel": "Required for sending notifications via Slack" }, "config": { "sort-packages": true, "allow-plugins": { "pestphp/pest-plugin": true, "phpstan/extension-installer": true } }, "extra": { "laravel": { "providers": [ "Spatie\\Backup\\BackupServiceProvider" ] } }, "minimum-stability": "dev", "prefer-stable": true, "funding": [ { "type": "github", "url": "https://github.com/sponsors/spatie" }, { "type": "other", "url": "https://spatie.be/open-source/support-us" } ] } laravel-backup/resources/lang/bg/notifications.php 0000644 00000010141 15002154456 0016341 0 ustar 00 <?php return [ 'exception_message' => 'Съобщение за изключение: :message', 'exception_trace' => 'Проследяване на изключение: :trace', 'exception_message_title' => 'Съобщение за изключение', 'exception_trace_title' => 'Проследяване на изключение', 'backup_failed_subject' => 'Неуспешно резервно копие на :application_name', 'backup_failed_body' => 'Важно: Възникна грешка при архивиране на :application_name', 'backup_successful_subject' => 'Успешно ново резервно копие на :application_name', 'backup_successful_subject_title' => 'Успешно ново резервно копие!', 'backup_successful_body' => 'Чудесни новини, ново резервно копие на :application_name беше успешно създадено на диска с име :disk_name.', 'cleanup_failed_subject' => 'Почистването на резервните копия на :application_name не бе успешно.', 'cleanup_failed_body' => 'Възникна грешка при почистването на резервните копия на :application_name', 'cleanup_successful_subject' => 'Почистването на архивите на :application_name е успешно', 'cleanup_successful_subject_title' => 'Почистването на резервните копия е успешно!', 'cleanup_successful_body' => 'Почистването на резервни копия на :application_name на диска с име :disk_name беше успешно.', 'healthy_backup_found_subject' => 'Резервните копия за :application_name на диск :disk_name са здрави', 'healthy_backup_found_subject_title' => 'Резервните копия за :application_name са здрави', 'healthy_backup_found_body' => 'Резервните копия за :application_name се считат за здрави. Добра работа!', 'unhealthy_backup_found_subject' => 'Важно: Резервните копия за :application_name не са здрави', 'unhealthy_backup_found_subject_title' => 'Важно: Резервните копия за :application_name не са здрави. :проблем', 'unhealthy_backup_found_body' => 'Резервните копия за :application_name на диск :disk_name не са здрави.', 'unhealthy_backup_found_not_reachable' => 'Дестинацията за резервни копия не може да бъде достигната. :грешка', 'unhealthy_backup_found_empty' => 'Изобщо няма резервни копия на това приложение.', 'unhealthy_backup_found_old' => 'Последното резервно копие, направено на :date, се счита за твърде старо.', 'unhealthy_backup_found_unknown' => 'За съжаление не може да се определи точна причина.', 'unhealthy_backup_found_full' => 'Резервните копия използват твърде много място за съхранение. Текущото използване е :disk_usage, което е по-високо от разрешеното ограничение на :disk_limit.', 'no_backups_info' => 'Все още не са правени резервни копия', 'application_name' => 'Име на приложението', 'backup_name' => 'Име на резервно копие', 'disk' => 'Диск', 'newest_backup_size' => 'Най-новият размер на резервно копие', 'number_of_backups' => 'Брой резервни копия', 'total_storage_used' => 'Общо използвано дисково пространство', 'newest_backup_date' => 'Най-нова дата на резервно копие', 'oldest_backup_date' => 'Най-старата дата на резервно копие', ]; laravel-backup/resources/lang/es/notifications.php 0000644 00000006417 15002154456 0016373 0 ustar 00 <?php return [ 'exception_message' => 'Mensaje de la excepción: :message', 'exception_trace' => 'Traza de la excepción: :trace', 'exception_message_title' => 'Mensaje de la excepción', 'exception_trace_title' => 'Traza de la excepción', 'backup_failed_subject' => 'Copia de seguridad de :application_name fallida', 'backup_failed_body' => 'Importante: Ocurrió un error al realizar la copia de seguridad de :application_name', 'backup_successful_subject' => 'Se completó con éxito la copia de seguridad de :application_name', 'backup_successful_subject_title' => '¡Nueva copia de seguridad creada con éxito!', 'backup_successful_body' => 'Buenas noticias, una nueva copia de seguridad de :application_name fue creada con éxito en el disco llamado :disk_name.', 'cleanup_failed_subject' => 'La limpieza de copias de seguridad de :application_name falló.', 'cleanup_failed_body' => 'Ocurrió un error mientras se realizaba la limpieza de copias de seguridad de :application_name', 'cleanup_successful_subject' => 'La limpieza de copias de seguridad de :application_name se completó con éxito', 'cleanup_successful_subject_title' => '!Limpieza de copias de seguridad completada con éxito!', 'cleanup_successful_body' => 'La limpieza de copias de seguridad de :application_name en el disco llamado :disk_name se completo con éxito.', 'healthy_backup_found_subject' => 'Las copias de seguridad de :application_name en el disco :disk_name están en buen estado', 'healthy_backup_found_subject_title' => 'Las copias de seguridad de :application_name están en buen estado', 'healthy_backup_found_body' => 'Las copias de seguridad de :application_name se consideran en buen estado. ¡Buen trabajo!', 'unhealthy_backup_found_subject' => 'Importante: Las copias de seguridad de :application_name están en mal estado', 'unhealthy_backup_found_subject_title' => 'Importante: Las copias de seguridad de :application_name están en mal estado. :problem', 'unhealthy_backup_found_body' => 'Las copias de seguridad de :application_name en el disco :disk_name están en mal estado.', 'unhealthy_backup_found_not_reachable' => 'No se puede acceder al destino de la copia de seguridad. :error', 'unhealthy_backup_found_empty' => 'No existe ninguna copia de seguridad de esta aplicación.', 'unhealthy_backup_found_old' => 'La última copia de seguriad hecha en :date es demasiado antigua.', 'unhealthy_backup_found_unknown' => 'Lo siento, no es posible determinar la razón exacta.', 'unhealthy_backup_found_full' => 'Las copias de seguridad están ocupando demasiado espacio. El espacio utilizado actualmente es :disk_usage el cual es mayor que el límite permitido de :disk_limit.', 'no_backups_info' => 'Aún no se hicieron copias de seguridad', 'application_name' => 'Nombre de la aplicación', 'backup_name' => 'Nombre de la copia de seguridad', 'disk' => 'Disco', 'newest_backup_size' => 'Tamaño de copia de seguridad más reciente', 'number_of_backups' => 'Número de copias de seguridad', 'total_storage_used' => 'Almacenamiento total utilizado', 'newest_backup_date' => 'Fecha de la copia de seguridad más reciente', 'oldest_backup_date' => 'Fecha de la copia de seguridad más antigua', ]; laravel-backup/resources/lang/pt/notifications.php 0000644 00000005671 15002154456 0016410 0 ustar 00 <?php return [ 'exception_message' => 'Exception message: :message', 'exception_trace' => 'Exception trace: :trace', 'exception_message_title' => 'Exception message', 'exception_trace_title' => 'Exception trace', 'backup_failed_subject' => 'Falha no backup da aplicação :application_name', 'backup_failed_body' => 'Importante: Ocorreu um erro ao executar o backup da aplicação :application_name', 'backup_successful_subject' => 'Backup realizado com sucesso: :application_name', 'backup_successful_subject_title' => 'Backup Realizado com Sucesso!', 'backup_successful_body' => 'Boas notícias, foi criado um novo backup no disco :disk_name referente à aplicação :application_name.', 'cleanup_failed_subject' => 'Falha na limpeza dos backups da aplicação :application_name.', 'cleanup_failed_body' => 'Ocorreu um erro ao executar a limpeza dos backups da aplicação :application_name', 'cleanup_successful_subject' => 'Limpeza dos backups da aplicação :application_name concluída!', 'cleanup_successful_subject_title' => 'Limpeza dos backups concluída!', 'cleanup_successful_body' => 'Concluída a limpeza dos backups da aplicação :application_name no disco :disk_name.', 'healthy_backup_found_subject' => 'Os backups da aplicação :application_name no disco :disk_name estão em dia', 'healthy_backup_found_subject_title' => 'Os backups da aplicação :application_name estão em dia', 'healthy_backup_found_body' => 'Os backups da aplicação :application_name estão em dia. Bom trabalho!', 'unhealthy_backup_found_subject' => 'Importante: Os backups da aplicação :application_name não estão em dia', 'unhealthy_backup_found_subject_title' => 'Importante: Os backups da aplicação :application_name não estão em dia. :problem', 'unhealthy_backup_found_body' => 'Os backups da aplicação :application_name no disco :disk_name não estão em dia.', 'unhealthy_backup_found_not_reachable' => 'O destino dos backups não pode ser alcançado. :error', 'unhealthy_backup_found_empty' => 'Não existem backups para essa aplicação.', 'unhealthy_backup_found_old' => 'O último backup realizado em :date é demasiado antigo.', 'unhealthy_backup_found_unknown' => 'Desculpe, impossível determinar a razão exata.', 'unhealthy_backup_found_full' => 'Os backups estão a utilizar demasiado espaço de armazenamento. A utilização atual é de :disk_usage, o que é maior que o limite permitido de :disk_limit.', 'no_backups_info' => 'Nenhum backup foi feito ainda', 'application_name' => 'Nome da Aplicação', 'backup_name' => 'Nome de backup', 'disk' => 'Disco', 'newest_backup_size' => 'Tamanho de backup mais recente', 'number_of_backups' => 'Número de backups', 'total_storage_used' => 'Armazenamento total usado', 'newest_backup_date' => 'Tamanho de backup mais recente', 'oldest_backup_date' => 'Tamanho de backup mais antigo', ]; laravel-backup/resources/lang/hr/notifications.php 0000644 00000005536 15002154456 0016376 0 ustar 00 <?php return [ 'exception_message' => 'Greška: :message', 'exception_trace' => 'Praćenje greške: :trace', 'exception_message_title' => 'Greška', 'exception_trace_title' => 'Praćenje greške', 'backup_failed_subject' => 'Neuspješno sigurnosno kopiranje za :application_name', 'backup_failed_body' => 'Važno: Došlo je do greške prilikom sigurnosnog kopiranja za :application_name', 'backup_successful_subject' => 'Uspješno sigurnosno kopiranje za :application_name', 'backup_successful_subject_title' => 'Uspješno sigurnosno kopiranje!', 'backup_successful_body' => 'Nova sigurnosna kopija za :application_name je uspješno spremljena na disk :disk_name.', 'cleanup_failed_subject' => 'Neuspješno čišćenje sigurnosnih kopija za :application_name', 'cleanup_failed_body' => 'Došlo je do greške prilikom čišćenja sigurnosnih kopija za :application_name', 'cleanup_successful_subject' => 'Uspješno čišćenje sigurnosnih kopija za :application_name', 'cleanup_successful_subject_title' => 'Uspješno čišćenje sigurnosnih kopija!', 'cleanup_successful_body' => 'Sigurnosne kopije za :application_name su uspješno očišćene s diska :disk_name.', 'healthy_backup_found_subject' => 'Sigurnosne kopije za :application_name na disku :disk_name su zdrave', 'healthy_backup_found_subject_title' => 'Sigurnosne kopije za :application_name su zdrave', 'healthy_backup_found_body' => 'Sigurnosne kopije za :application_name se smatraju zdravima. Svaka čast!', 'unhealthy_backup_found_subject' => 'Važno: Sigurnosne kopije za :application_name su nezdrave', 'unhealthy_backup_found_subject_title' => 'Važno: Sigurnosne kopije za :application_name su nezdrave. :problem', 'unhealthy_backup_found_body' => 'Sigurnosne kopije za :application_name na disku :disk_name su nezdrave.', 'unhealthy_backup_found_not_reachable' => 'Destinacija sigurnosne kopije nije dohvatljiva. :error', 'unhealthy_backup_found_empty' => 'Nijedna sigurnosna kopija ove aplikacije ne postoji.', 'unhealthy_backup_found_old' => 'Zadnja sigurnosna kopija generirana na datum :date smatra se prestarom.', 'unhealthy_backup_found_unknown' => 'Isprike, ali nije moguće odrediti razlog.', 'unhealthy_backup_found_full' => 'Sigurnosne kopije zauzimaju previše prostora. Trenutno zauzeće je :disk_usage što je više od dozvoljenog ograničenja od :disk_limit.', 'no_backups_info' => 'Nema sigurnosnih kopija', 'application_name' => 'Naziv aplikacije', 'backup_name' => 'Naziv sigurnosne kopije', 'disk' => 'Disk', 'newest_backup_size' => 'Veličina najnovije sigurnosne kopije', 'number_of_backups' => 'Broj sigurnosnih kopija', 'total_storage_used' => 'Ukupno zauzeće', 'newest_backup_date' => 'Najnovija kopija na datum', 'oldest_backup_date' => 'Najstarija kopija na datum', ]; laravel-backup/resources/lang/ro/notifications.php 0000644 00000006357 15002154456 0016407 0 ustar 00 <?php return [ 'exception_message' => 'Cu excepția mesajului: :message', 'exception_trace' => 'Urmă excepţie: :trace', 'exception_message_title' => 'Mesaj de excepție', 'exception_trace_title' => 'Urmă excepţie', 'backup_failed_subject' => 'Nu s-a putut face copie de rezervă pentru :application_name', 'backup_failed_body' => 'Important: A apărut o eroare în timpul generării copiei de rezervă pentru :application_name', 'backup_successful_subject' => 'Copie de rezervă efectuată cu succes pentru :application_name', 'backup_successful_subject_title' => 'O nouă copie de rezervă a fost efectuată cu succes!', 'backup_successful_body' => 'Vești bune, o nouă copie de rezervă pentru :application_name a fost creată cu succes pe discul cu numele :disk_name.', 'cleanup_failed_subject' => 'Curățarea copiilor de rezervă pentru :application_name nu a reușit.', 'cleanup_failed_body' => 'A apărut o eroare în timpul curățirii copiilor de rezervă pentru :application_name', 'cleanup_successful_subject' => 'Curățarea copiilor de rezervă pentru :application_name a fost făcută cu succes', 'cleanup_successful_subject_title' => 'Curățarea copiilor de rezervă a fost făcută cu succes!', 'cleanup_successful_body' => 'Curățarea copiilor de rezervă pentru :application_name de pe discul cu numele :disk_name a fost făcută cu succes.', 'healthy_backup_found_subject' => 'Copiile de rezervă pentru :application_name de pe discul :disk_name sunt în regulă', 'healthy_backup_found_subject_title' => 'Copiile de rezervă pentru :application_name sunt în regulă', 'healthy_backup_found_body' => 'Copiile de rezervă pentru :application_name sunt considerate în regulă. Bună treabă!', 'unhealthy_backup_found_subject' => 'Important: Copiile de rezervă pentru :application_name nu sunt în regulă', 'unhealthy_backup_found_subject_title' => 'Important: Copiile de rezervă pentru :application_name nu sunt în regulă. :problem', 'unhealthy_backup_found_body' => 'Copiile de rezervă pentru :application_name de pe discul :disk_name nu sunt în regulă.', 'unhealthy_backup_found_not_reachable' => 'Nu se poate ajunge la destinația copiilor de rezervă. :error', 'unhealthy_backup_found_empty' => 'Nu există copii de rezervă ale acestei aplicații.', 'unhealthy_backup_found_old' => 'Cea mai recentă copie de rezervă făcută la :date este considerată prea veche.', 'unhealthy_backup_found_unknown' => 'Ne pare rău, un motiv exact nu poate fi determinat.', 'unhealthy_backup_found_full' => 'Copiile de rezervă folosesc prea mult spațiu de stocare. Utilizarea curentă este de :disk_usage care este mai mare decât limita permisă de :disk_limit.', 'no_backups_info' => 'Nu s-au făcut încă copii de rezervă', 'application_name' => 'Numele aplicatiei', 'backup_name' => 'Numele de rezervă', 'disk' => 'Disc', 'newest_backup_size' => 'Cea mai nouă dimensiune de rezervă', 'number_of_backups' => 'Număr de copii de rezervă', 'total_storage_used' => 'Spațiu total de stocare utilizat', 'newest_backup_date' => 'Cea mai nouă dimensiune de rezervă', 'oldest_backup_date' => 'Cea mai veche dimensiune de rezervă', ]; laravel-backup/resources/lang/fi/notifications.php 0000644 00000005356 15002154456 0016363 0 ustar 00 <?php return [ 'exception_message' => 'Virheilmoitus: :message', 'exception_trace' => 'Virhe, jäljitys: :trace', 'exception_message_title' => 'Virheilmoitus', 'exception_trace_title' => 'Virheen jäljitys', 'backup_failed_subject' => ':application_name varmuuskopiointi epäonnistui', 'backup_failed_body' => 'HUOM!: :application_name varmuuskoipionnissa tapahtui virhe', 'backup_successful_subject' => ':application_name varmuuskopioitu onnistuneesti', 'backup_successful_subject_title' => 'Uusi varmuuskopio!', 'backup_successful_body' => 'Hyviä uutisia! :application_name on varmuuskopioitu levylle :disk_name.', 'cleanup_failed_subject' => ':application_name varmuuskopioiden poistaminen epäonnistui.', 'cleanup_failed_body' => ':application_name varmuuskopioiden poistamisessa tapahtui virhe.', 'cleanup_successful_subject' => ':application_name varmuuskopiot poistettu onnistuneesti', 'cleanup_successful_subject_title' => 'Varmuuskopiot poistettu onnistuneesti!', 'cleanup_successful_body' => ':application_name varmuuskopiot poistettu onnistuneesti levyltä :disk_name.', 'healthy_backup_found_subject' => ':application_name varmuuskopiot levyllä :disk_name ovat kunnossa', 'healthy_backup_found_subject_title' => ':application_name varmuuskopiot ovat kunnossa', 'healthy_backup_found_body' => ':application_name varmuuskopiot ovat kunnossa. Hieno homma!', 'unhealthy_backup_found_subject' => 'HUOM!: :application_name varmuuskopiot ovat vialliset', 'unhealthy_backup_found_subject_title' => 'HUOM!: :application_name varmuuskopiot ovat vialliset. :problem', 'unhealthy_backup_found_body' => ':application_name varmuuskopiot levyllä :disk_name ovat vialliset.', 'unhealthy_backup_found_not_reachable' => 'Varmuuskopioiden kohdekansio ei ole saatavilla. :error', 'unhealthy_backup_found_empty' => 'Tästä sovelluksesta ei ole varmuuskopioita.', 'unhealthy_backup_found_old' => 'Viimeisin varmuuskopio, luotu :date, on liian vanha.', 'unhealthy_backup_found_unknown' => 'Virhe, tarkempaa tietoa syystä ei valitettavasti ole saatavilla.', 'unhealthy_backup_found_full' => 'Varmuuskopiot vievät liikaa levytilaa. Tällä hetkellä käytössä :disk_usage, mikä on suurempi kuin sallittu tilavuus (:disk_limit).', 'no_backups_info' => 'Varmuuskopioita ei vielä tehty', 'application_name' => 'Sovelluksen nimi', 'backup_name' => 'Varmuuskopion nimi', 'disk' => 'Levy', 'newest_backup_size' => 'Uusin varmuuskopion koko', 'number_of_backups' => 'Varmuuskopioiden määrä', 'total_storage_used' => 'Käytetty tallennustila yhteensä', 'newest_backup_date' => 'Uusin varmuuskopion koko', 'oldest_backup_date' => 'Vanhin varmuuskopion koko', ]; laravel-backup/resources/lang/cs/notifications.php 0000644 00000005423 15002154456 0016365 0 ustar 00 <?php return [ 'exception_message' => 'Zpráva výjimky: :message', 'exception_trace' => 'Stopa výjimky: :trace', 'exception_message_title' => 'Zpráva výjimky', 'exception_trace_title' => 'Stopa výjimky', 'backup_failed_subject' => 'Záloha :application_name neuspěla', 'backup_failed_body' => 'Důležité: Při záloze :application_name se vyskytla chyba', 'backup_successful_subject' => 'Úspěšná nová záloha :application_name', 'backup_successful_subject_title' => 'Úspěšná nová záloha!', 'backup_successful_body' => 'Dobrá zpráva, na disku jménem :disk_name byla úspěšně vytvořena nová záloha :application_name.', 'cleanup_failed_subject' => 'Vyčištění záloh :application_name neuspělo.', 'cleanup_failed_body' => 'Při čištění záloh :application_name se vyskytla chyba', 'cleanup_successful_subject' => 'Vyčištění záloh :application_name úspěšné', 'cleanup_successful_subject_title' => 'Vyčištění záloh bylo úspěšné!', 'cleanup_successful_body' => 'Vyčištění záloh :application_name na disku jménem :disk_name bylo úspěšné.', 'healthy_backup_found_subject' => 'Zálohy pro :application_name na disku :disk_name jsou zdravé', 'healthy_backup_found_subject_title' => 'Zálohy pro :application_name jsou zdravé', 'healthy_backup_found_body' => 'Zálohy pro :application_name jsou považovány za zdravé. Dobrá práce!', 'unhealthy_backup_found_subject' => 'Důležité: Zálohy pro :application_name jsou nezdravé', 'unhealthy_backup_found_subject_title' => 'Důležité: Zálohy pro :application_name jsou nezdravé. :problem', 'unhealthy_backup_found_body' => 'Zálohy pro :application_name na disku :disk_name jsou nezdravé.', 'unhealthy_backup_found_not_reachable' => 'Nelze se dostat k cíli zálohy. :error', 'unhealthy_backup_found_empty' => 'Tato aplikace nemá vůbec žádné zálohy.', 'unhealthy_backup_found_old' => 'Poslední záloha vytvořená dne :date je považována za příliš starou.', 'unhealthy_backup_found_unknown' => 'Omlouváme se, nemůžeme určit přesný důvod.', 'unhealthy_backup_found_full' => 'Zálohy zabírají příliš mnoho místa na disku. Aktuální využití disku je :disk_usage, což je vyšší než povolený limit :disk_limit.', 'no_backups_info' => 'Zatím nebyly vytvořeny žádné zálohy', 'application_name' => 'Název aplikace', 'backup_name' => 'Název zálohy', 'disk' => 'Disk', 'newest_backup_size' => 'Velikost nejnovější zálohy', 'number_of_backups' => 'Počet záloh', 'total_storage_used' => 'Celková využitá kapacita úložiště', 'newest_backup_date' => 'Datum nejnovější zálohy', 'oldest_backup_date' => 'Datum nejstarší zálohy', ]; laravel-backup/resources/lang/he/notifications.php 0000644 00000006206 15002154456 0016354 0 ustar 00 <?php return [ 'exception_message' => 'הודעת חריגה: :message', 'exception_trace' => 'מעקב חריגה: :trace', 'exception_message_title' => 'הודעת חריגה', 'exception_trace_title' => 'מעקב חריגה', 'backup_failed_subject' => 'כשל בגיבוי של :application_name', 'backup_failed_body' => 'חשוב: אירעה שגיאה במהלך גיבוי היישום :application_name', 'backup_successful_subject' => 'גיבוי חדש מוצלח של :application_name', 'backup_successful_subject_title' => 'גיבוי חדש מוצלח!', 'backup_successful_body' => 'חדשות טובות, גיבוי חדש של :application_name נוצר בהצלחה על הדיסק בשם :disk_name.', 'cleanup_failed_subject' => 'נכשל בניקוי הגיבויים של :application_name', 'cleanup_failed_body' => 'אירעה שגיאה במהלך ניקוי הגיבויים של :application_name', 'cleanup_successful_subject' => 'ניקוי הגיבויים של :application_name בוצע בהצלחה', 'cleanup_successful_subject_title' => 'ניקוי הגיבויים בוצע בהצלחה!', 'cleanup_successful_body' => 'ניקוי הגיבויים של :application_name על הדיסק בשם :disk_name בוצע בהצלחה.', 'healthy_backup_found_subject' => 'הגיבויים של :application_name על הדיסק :disk_name תקינים', 'healthy_backup_found_subject_title' => 'הגיבויים של :application_name תקינים', 'healthy_backup_found_body' => 'הגיבויים של :application_name נחשבים לתקינים. עבודה טובה!', 'unhealthy_backup_found_subject' => 'חשוב: הגיבויים של :application_name אינם תקינים', 'unhealthy_backup_found_subject_title' => 'חשוב: הגיבויים של :application_name אינם תקינים. :problem', 'unhealthy_backup_found_body' => 'הגיבויים של :application_name על הדיסק :disk_name אינם תקינים.', 'unhealthy_backup_found_not_reachable' => 'לא ניתן להגיע ליעד הגיבוי. :error', 'unhealthy_backup_found_empty' => 'אין גיבויים של היישום הזה בכלל.', 'unhealthy_backup_found_old' => 'הגיבוי האחרון שנעשה בתאריך :date נחשב כישן מדי.', 'unhealthy_backup_found_unknown' => 'מצטערים, לא ניתן לקבוע סיבה מדויקת.', 'unhealthy_backup_found_full' => 'הגיבויים משתמשים בשטח אחסון רב מידי. שימוש הנוכחי הוא :disk_usage, שגבול המותר הוא :disk_limit.', 'no_backups_info' => 'לא נעשו עדיין גיבויים', 'application_name' => 'שם היישום', 'backup_name' => 'שם הגיבוי', 'disk' => 'דיסק', 'newest_backup_size' => 'גודל הגיבוי החדש ביותר', 'number_of_backups' => 'מספר הגיבויים', 'total_storage_used' => 'סך האחסון המופעל', 'newest_backup_date' => 'תאריך הגיבוי החדש ביותר', 'oldest_backup_date' => 'תאריך הגיבוי הישן ביותר', ]; laravel-backup/resources/lang/id/notifications.php 0000644 00000005335 15002154456 0016356 0 ustar 00 <?php return [ 'exception_message' => 'Pesan pengecualian: :message', 'exception_trace' => 'Jejak pengecualian: :trace', 'exception_message_title' => 'Pesan pengecualian', 'exception_trace_title' => 'Jejak pengecualian', 'backup_failed_subject' => 'Gagal backup :application_name', 'backup_failed_body' => 'Penting: Sebuah error terjadi ketika membackup :application_name', 'backup_successful_subject' => 'Backup baru sukses dari :application_name', 'backup_successful_subject_title' => 'Backup baru sukses!', 'backup_successful_body' => 'Kabar baik, sebuah backup baru dari :application_name sukses dibuat pada disk bernama :disk_name.', 'cleanup_failed_subject' => 'Membersihkan backup dari :application_name yang gagal.', 'cleanup_failed_body' => 'Sebuah error teradi ketika membersihkan backup dari :application_name', 'cleanup_successful_subject' => 'Sukses membersihkan backup :application_name', 'cleanup_successful_subject_title' => 'Sukses membersihkan backup!', 'cleanup_successful_body' => 'Pembersihan backup :application_name pada disk bernama :disk_name telah sukses.', 'healthy_backup_found_subject' => 'Backup untuk :application_name pada disk :disk_name sehat', 'healthy_backup_found_subject_title' => 'Backup untuk :application_name sehat', 'healthy_backup_found_body' => 'Backup untuk :application_name dipertimbangkan sehat. Kerja bagus!', 'unhealthy_backup_found_subject' => 'Penting: Backup untuk :application_name tidak sehat', 'unhealthy_backup_found_subject_title' => 'Penting: Backup untuk :application_name tidak sehat. :problem', 'unhealthy_backup_found_body' => 'Backup untuk :application_name pada disk :disk_name tidak sehat.', 'unhealthy_backup_found_not_reachable' => 'Tujuan backup tidak dapat terjangkau. :error', 'unhealthy_backup_found_empty' => 'Tidak ada backup pada aplikasi ini sama sekali.', 'unhealthy_backup_found_old' => 'Backup terakhir dibuat pada :date dimana dipertimbahkan sudah sangat lama.', 'unhealthy_backup_found_unknown' => 'Maaf, sebuah alasan persisnya tidak dapat ditentukan.', 'unhealthy_backup_found_full' => 'Backup menggunakan terlalu banyak kapasitas penyimpanan. Penggunaan terkini adalah :disk_usage dimana lebih besar dari batas yang diperbolehkan yaitu :disk_limit.', 'no_backups_info' => 'Belum ada backup yang dibuat', 'application_name' => 'Nama aplikasi', 'backup_name' => 'Nama cadangan', 'disk' => 'Disk', 'newest_backup_size' => 'Ukuran cadangan terbaru', 'number_of_backups' => 'Jumlah cadangan', 'total_storage_used' => 'Total penyimpanan yang digunakan', 'newest_backup_date' => 'Ukuran cadangan terbaru', 'oldest_backup_date' => 'Ukuran cadangan tertua', ]; laravel-backup/resources/lang/it/notifications.php 0000644 00000005543 15002154456 0016377 0 ustar 00 <?php return [ 'exception_message' => 'Messaggio dell\'eccezione: :message', 'exception_trace' => 'Traccia dell\'eccezione: :trace', 'exception_message_title' => 'Messaggio dell\'eccezione', 'exception_trace_title' => 'Traccia dell\'eccezione', 'backup_failed_subject' => 'Fallito il backup di :application_name', 'backup_failed_body' => 'Importante: Si è verificato un errore durante il backup di :application_name', 'backup_successful_subject' => 'Creato nuovo backup di :application_name', 'backup_successful_subject_title' => 'Nuovo backup creato!', 'backup_successful_body' => 'Grande notizia, un nuovo backup di :application_name è stato creato con successo sul disco :disk_name.', 'cleanup_failed_subject' => 'Pulizia dei backup di :application_name fallita.', 'cleanup_failed_body' => 'Si è verificato un errore durante la pulizia dei backup di :application_name', 'cleanup_successful_subject' => 'Pulizia dei backup di :application_name avvenuta con successo', 'cleanup_successful_subject_title' => 'Pulizia dei backup avvenuta con successo!', 'cleanup_successful_body' => 'La pulizia dei backup di :application_name sul disco :disk_name è avvenuta con successo.', 'healthy_backup_found_subject' => 'I backup per :application_name sul disco :disk_name sono sani', 'healthy_backup_found_subject_title' => 'I backup per :application_name sono sani', 'healthy_backup_found_body' => 'I backup per :application_name sono considerati sani. Bel Lavoro!', 'unhealthy_backup_found_subject' => 'Importante: i backup per :application_name sono corrotti', 'unhealthy_backup_found_subject_title' => 'Importante: i backup per :application_name sono corrotti. :problem', 'unhealthy_backup_found_body' => 'I backup per :application_name sul disco :disk_name sono corrotti.', 'unhealthy_backup_found_not_reachable' => 'Impossibile raggiungere la destinazione di backup. :error', 'unhealthy_backup_found_empty' => 'Non esiste alcun backup di questa applicazione.', 'unhealthy_backup_found_old' => 'L\'ultimo backup fatto il :date è considerato troppo vecchio.', 'unhealthy_backup_found_unknown' => 'Spiacenti, non è possibile determinare una ragione esatta.', 'unhealthy_backup_found_full' => 'I backup utilizzano troppa memoria. L\'utilizzo corrente è :disk_usage che è superiore al limite consentito di :disk_limit.', 'no_backups_info' => 'Non sono stati ancora effettuati backup', 'application_name' => 'Nome dell\'applicazione', 'backup_name' => 'Nome di backup', 'disk' => 'Disco', 'newest_backup_size' => 'Dimensione backup più recente', 'number_of_backups' => 'Numero di backup', 'total_storage_used' => 'Spazio di archiviazione totale utilizzato', 'newest_backup_date' => 'Data del backup più recente', 'oldest_backup_date' => 'Data del backup più vecchio', ]; laravel-backup/resources/lang/en/notifications.php 0000644 00000005265 15002154456 0016366 0 ustar 00 <?php return [ 'exception_message' => 'Exception message: :message', 'exception_trace' => 'Exception trace: :trace', 'exception_message_title' => 'Exception message', 'exception_trace_title' => 'Exception trace', 'backup_failed_subject' => 'Failed backup of :application_name', 'backup_failed_body' => 'Important: An error occurred while backing up :application_name', 'backup_successful_subject' => 'Successful new backup of :application_name', 'backup_successful_subject_title' => 'Successful new backup!', 'backup_successful_body' => 'Great news, a new backup of :application_name was successfully created on the disk named :disk_name.', 'cleanup_failed_subject' => 'Cleaning up the backups of :application_name failed.', 'cleanup_failed_body' => 'An error occurred while cleaning up the backups of :application_name', 'cleanup_successful_subject' => 'Clean up of :application_name backups successful', 'cleanup_successful_subject_title' => 'Clean up of backups successful!', 'cleanup_successful_body' => 'The clean up of the :application_name backups on the disk named :disk_name was successful.', 'healthy_backup_found_subject' => 'The backups for :application_name on disk :disk_name are healthy', 'healthy_backup_found_subject_title' => 'The backups for :application_name are healthy', 'healthy_backup_found_body' => 'The backups for :application_name are considered healthy. Good job!', 'unhealthy_backup_found_subject' => 'Important: The backups for :application_name are unhealthy', 'unhealthy_backup_found_subject_title' => 'Important: The backups for :application_name are unhealthy. :problem', 'unhealthy_backup_found_body' => 'The backups for :application_name on disk :disk_name are unhealthy.', 'unhealthy_backup_found_not_reachable' => 'The backup destination cannot be reached. :error', 'unhealthy_backup_found_empty' => 'There are no backups of this application at all.', 'unhealthy_backup_found_old' => 'The latest backup made on :date is considered too old.', 'unhealthy_backup_found_unknown' => 'Sorry, an exact reason cannot be determined.', 'unhealthy_backup_found_full' => 'The backups are using too much storage. Current usage is :disk_usage which is higher than the allowed limit of :disk_limit.', 'no_backups_info' => 'No backups were made yet', 'application_name' => 'Application name', 'backup_name' => 'Backup name', 'disk' => 'Disk', 'newest_backup_size' => 'Newest backup size', 'number_of_backups' => 'Number of backups', 'total_storage_used' => 'Total storage used', 'newest_backup_date' => 'Newest backup date', 'oldest_backup_date' => 'Oldest backup date', ]; laravel-backup/resources/lang/ja/notifications.php 0000644 00000006374 15002154456 0016360 0 ustar 00 <?php return [ 'exception_message' => '例外のメッセージ: :message', 'exception_trace' => '例外の追跡: :trace', 'exception_message_title' => '例外のメッセージ', 'exception_trace_title' => '例外の追跡', 'backup_failed_subject' => ':application_name のバックアップに失敗しました。', 'backup_failed_body' => '重要: :application_name のバックアップ中にエラーが発生しました。', 'backup_successful_subject' => ':application_name のバックアップに成功しました。', 'backup_successful_subject_title' => 'バックアップに成功しました!', 'backup_successful_body' => '朗報です。ディスク :disk_name へ :application_name のバックアップが成功しました。', 'cleanup_failed_subject' => ':application_name のバックアップ削除に失敗しました。', 'cleanup_failed_body' => ':application_name のバックアップ削除中にエラーが発生しました。', 'cleanup_successful_subject' => ':application_name のバックアップ削除に成功しました。', 'cleanup_successful_subject_title' => 'バックアップ削除に成功しました!', 'cleanup_successful_body' => 'ディスク :disk_name に保存された :application_name のバックアップ削除に成功しました。', 'healthy_backup_found_subject' => 'ディスク :disk_name への :application_name のバックアップは正常です。', 'healthy_backup_found_subject_title' => ':application_name のバックアップは正常です。', 'healthy_backup_found_body' => ':application_name へのバックアップは正常です。いい仕事してますね!', 'unhealthy_backup_found_subject' => '重要: :application_name のバックアップに異常があります。', 'unhealthy_backup_found_subject_title' => '重要: :application_name のバックアップに異常があります。 :problem', 'unhealthy_backup_found_body' => ':disk_name への :application_name のバックアップに異常があります。', 'unhealthy_backup_found_not_reachable' => 'バックアップ先にアクセスできませんでした。 :error', 'unhealthy_backup_found_empty' => 'このアプリケーションのバックアップは見つかりませんでした。', 'unhealthy_backup_found_old' => ':date に保存された直近のバックアップが古すぎます。', 'unhealthy_backup_found_unknown' => '申し訳ございません。予期せぬエラーです。', 'unhealthy_backup_found_full' => 'バックアップがディスク容量を圧迫しています。現在の使用量 :disk_usage は、許可された限界値 :disk_limit を超えています。', 'no_backups_info' => 'バックアップはまだ作成されていません', 'application_name' => 'アプリケーション名', 'backup_name' => 'バックアップ名', 'disk' => 'ディスク', 'newest_backup_size' => '最新のバックアップサイズ', 'number_of_backups' => 'バックアップ数', 'total_storage_used' => '使用された合計ストレージ', 'newest_backup_date' => '最新のバックアップ日時', 'oldest_backup_date' => '最も古いバックアップ日時', ]; laravel-backup/resources/lang/no/notifications.php 0000644 00000005200 15002154456 0016365 0 ustar 00 <?php return [ 'exception_message' => 'Exception: :message', 'exception_trace' => 'Exception trace: :trace', 'exception_message_title' => 'Exception', 'exception_trace_title' => 'Exception trace', 'backup_failed_subject' => 'Backup feilet for :application_name', 'backup_failed_body' => 'Viktg: En feil oppstod under backing av :application_name', 'backup_successful_subject' => 'Gjennomført backup av :application_name', 'backup_successful_subject_title' => 'Gjennomført backup!', 'backup_successful_body' => 'Gode nyheter, en ny backup av :application_name ble opprettet på disken :disk_name.', 'cleanup_failed_subject' => 'Opprydding av backup for :application_name feilet.', 'cleanup_failed_body' => 'En feil oppstod under opprydding av backups for :application_name', 'cleanup_successful_subject' => 'Opprydding av backup for :application_name gjennomført', 'cleanup_successful_subject_title' => 'Opprydding av backup gjennomført!', 'cleanup_successful_body' => 'Oppryddingen av backup for :application_name på disken :disk_name har blitt gjennomført.', 'healthy_backup_found_subject' => 'Alle backups for :application_name på disken :disk_name er OK', 'healthy_backup_found_subject_title' => 'Alle backups for :application_name er OK', 'healthy_backup_found_body' => 'Alle backups for :application_name er ok. Godt jobba!', 'unhealthy_backup_found_subject' => 'Viktig: Backups for :application_name ikke OK', 'unhealthy_backup_found_subject_title' => 'Viktig: Backups for :application_name er ikke OK. :problem', 'unhealthy_backup_found_body' => 'Backups for :application_name på disken :disk_name er ikke OK.', 'unhealthy_backup_found_not_reachable' => 'Kunne ikke finne backup-destinasjonen. :error', 'unhealthy_backup_found_empty' => 'Denne applikasjonen mangler backups.', 'unhealthy_backup_found_old' => 'Den siste backupem fra :date er for gammel.', 'unhealthy_backup_found_unknown' => 'Beklager, kunne ikke finne nøyaktig årsak.', 'unhealthy_backup_found_full' => 'Backups bruker for mye lagringsplass. Nåværende diskbruk er :disk_usage, som er mer enn den tillatte grensen på :disk_limit.', 'no_backups_info' => 'Ingen sikkerhetskopier ble gjort ennå', 'application_name' => 'Programnavn', 'backup_name' => 'Navn på sikkerhetskopi', 'disk' => 'Disk', 'newest_backup_size' => 'Nyeste backup-størrelse', 'number_of_backups' => 'Antall sikkerhetskopier', 'total_storage_used' => 'Total lagring brukt', 'newest_backup_date' => 'Nyeste backup-størrelse', 'oldest_backup_date' => 'Eldste sikkerhetskopistørrelse', ]; laravel-backup/resources/lang/pl/notifications.php 0000644 00000006026 15002154456 0016373 0 ustar 00 <?php return [ 'exception_message' => 'Błąd: :message', 'exception_trace' => 'Zrzut błędu: :trace', 'exception_message_title' => 'Błąd', 'exception_trace_title' => 'Zrzut błędu', 'backup_failed_subject' => 'Tworzenie kopii zapasowej aplikacji :application_name nie powiodło się', 'backup_failed_body' => 'Ważne: Wystąpił błąd podczas tworzenia kopii zapasowej aplikacji :application_name', 'backup_successful_subject' => 'Pomyślnie utworzono kopię zapasową aplikacji :application_name', 'backup_successful_subject_title' => 'Nowa kopia zapasowa!', 'backup_successful_body' => 'Wspaniała wiadomość, nowa kopia zapasowa aplikacji :application_name została pomyślnie utworzona na dysku o nazwie :disk_name.', 'cleanup_failed_subject' => 'Czyszczenie kopii zapasowych aplikacji :application_name nie powiodło się.', 'cleanup_failed_body' => 'Wystąpił błąd podczas czyszczenia kopii zapasowej aplikacji :application_name', 'cleanup_successful_subject' => 'Kopie zapasowe aplikacji :application_name zostały pomyślnie wyczyszczone', 'cleanup_successful_subject_title' => 'Kopie zapasowe zostały pomyślnie wyczyszczone!', 'cleanup_successful_body' => 'Czyszczenie kopii zapasowych aplikacji :application_name na dysku :disk_name zakończone sukcesem.', 'healthy_backup_found_subject' => 'Kopie zapasowe aplikacji :application_name na dysku :disk_name są poprawne', 'healthy_backup_found_subject_title' => 'Kopie zapasowe aplikacji :application_name są poprawne', 'healthy_backup_found_body' => 'Kopie zapasowe aplikacji :application_name są poprawne. Dobra robota!', 'unhealthy_backup_found_subject' => 'Ważne: Kopie zapasowe aplikacji :application_name są niepoprawne', 'unhealthy_backup_found_subject_title' => 'Ważne: Kopie zapasowe aplikacji :application_name są niepoprawne. :problem', 'unhealthy_backup_found_body' => 'Kopie zapasowe aplikacji :application_name na dysku :disk_name są niepoprawne.', 'unhealthy_backup_found_not_reachable' => 'Miejsce docelowe kopii zapasowej nie jest osiągalne. :error', 'unhealthy_backup_found_empty' => 'W aplikacji nie ma żadnej kopii zapasowych tej aplikacji.', 'unhealthy_backup_found_old' => 'Ostatnia kopia zapasowa wykonania dnia :date jest zbyt stara.', 'unhealthy_backup_found_unknown' => 'Niestety, nie można ustalić dokładnego błędu.', 'unhealthy_backup_found_full' => 'Kopie zapasowe zajmują zbyt dużo miejsca. Obecne użycie dysku :disk_usage jest większe od ustalonego limitu :disk_limit.', 'no_backups_info' => 'Nie utworzono jeszcze kopii zapasowych', 'application_name' => 'Nazwa aplikacji', 'backup_name' => 'Nazwa kopii zapasowej', 'disk' => 'Dysk', 'newest_backup_size' => 'Najnowszy rozmiar kopii zapasowej', 'number_of_backups' => 'Liczba kopii zapasowych', 'total_storage_used' => 'Całkowite wykorzystane miejsce', 'newest_backup_date' => 'Najnowszy rozmiar kopii zapasowej', 'oldest_backup_date' => 'Najstarszy rozmiar kopii zapasowej', ]; laravel-backup/resources/lang/de/notifications.php 0000644 00000005427 15002154456 0016354 0 ustar 00 <?php return [ 'exception_message' => 'Fehlermeldung: :message', 'exception_trace' => 'Fehlerverfolgung: :trace', 'exception_message_title' => 'Fehlermeldung', 'exception_trace_title' => 'Fehlerverfolgung', 'backup_failed_subject' => 'Backup von :application_name konnte nicht erstellt werden', 'backup_failed_body' => 'Wichtig: Beim Backup von :application_name ist ein Fehler aufgetreten', 'backup_successful_subject' => 'Erfolgreiches neues Backup von :application_name', 'backup_successful_subject_title' => 'Erfolgreiches neues Backup!', 'backup_successful_body' => 'Gute Nachrichten, ein neues Backup von :application_name wurde erfolgreich erstellt und in :disk_name gepeichert.', 'cleanup_failed_subject' => 'Aufräumen der Backups von :application_name schlug fehl.', 'cleanup_failed_body' => 'Beim aufräumen der Backups von :application_name ist ein Fehler aufgetreten', 'cleanup_successful_subject' => 'Aufräumen der Backups von :application_name backups erfolgreich', 'cleanup_successful_subject_title' => 'Aufräumen der Backups erfolgreich!', 'cleanup_successful_body' => 'Aufräumen der Backups von :application_name in :disk_name war erfolgreich.', 'healthy_backup_found_subject' => 'Die Backups von :application_name in :disk_name sind gesund', 'healthy_backup_found_subject_title' => 'Die Backups von :application_name sind Gesund', 'healthy_backup_found_body' => 'Die Backups von :application_name wurden als gesund eingestuft. Gute Arbeit!', 'unhealthy_backup_found_subject' => 'Wichtig: Die Backups für :application_name sind nicht gesund', 'unhealthy_backup_found_subject_title' => 'Wichtig: Die Backups für :application_name sind ungesund. :problem', 'unhealthy_backup_found_body' => 'Die Backups für :application_name in :disk_name sind ungesund.', 'unhealthy_backup_found_not_reachable' => 'Das Backup Ziel konnte nicht erreicht werden. :error', 'unhealthy_backup_found_empty' => 'Es gibt für die Anwendung noch gar keine Backups.', 'unhealthy_backup_found_old' => 'Das letzte Backup am :date ist zu lange her.', 'unhealthy_backup_found_unknown' => 'Sorry, ein genauer Grund konnte nicht gefunden werden.', 'unhealthy_backup_found_full' => 'Die Backups verbrauchen zu viel Platz. Aktuell wird :disk_usage belegt, dass ist höher als das erlaubte Limit von :disk_limit.', 'no_backups_info' => 'Bisher keine Backups vorhanden', 'application_name' => 'Applikationsname', 'backup_name' => 'Backup Name', 'disk' => 'Speicherort', 'newest_backup_size' => 'Neuste Backup-Größe', 'number_of_backups' => 'Anzahl Backups', 'total_storage_used' => 'Gesamter genutzter Speicherplatz', 'newest_backup_date' => 'Neustes Backup', 'oldest_backup_date' => 'Ältestes Backup', ]; laravel-backup/resources/lang/ru/notifications.php 0000644 00000007722 15002154456 0016412 0 ustar 00 <?php return [ 'exception_message' => 'Сообщение об ошибке: :message', 'exception_trace' => 'Сведения об ошибке: :trace', 'exception_message_title' => 'Сообщение об ошибке', 'exception_trace_title' => 'Сведения об ошибке', 'backup_failed_subject' => 'Не удалось сделать резервную копию :application_name', 'backup_failed_body' => 'Внимание: Произошла ошибка во время резервного копирования :application_name', 'backup_successful_subject' => 'Успешно создана новая резервная копия :application_name', 'backup_successful_subject_title' => 'Успешно создана новая резервная копия!', 'backup_successful_body' => 'Отличная новость, новая резервная копия :application_name успешно создана и сохранена на диск :disk_name.', 'cleanup_failed_subject' => 'Не удалось очистить резервные копии :application_name', 'cleanup_failed_body' => 'Произошла ошибка при очистке резервных копий :application_name', 'cleanup_successful_subject' => 'Очистка от резервных копий :application_name прошла успешно', 'cleanup_successful_subject_title' => 'Очистка резервных копий прошла успешно!', 'cleanup_successful_body' => 'Очистка от старых резервных копий :application_name на диске :disk_name прошла успешно.', 'healthy_backup_found_subject' => 'Резервные копии :application_name с диска :disk_name исправны', 'healthy_backup_found_subject_title' => 'Резервные копии :application_name исправны', 'healthy_backup_found_body' => 'Резервные копии :application_name считаются исправными. Хорошая работа!', 'unhealthy_backup_found_subject' => 'Внимание: резервные копии :application_name неисправны', 'unhealthy_backup_found_subject_title' => 'Внимание: резервные копии для :application_name неисправны. :problem', 'unhealthy_backup_found_body' => 'Резервные копии для :application_name на диске :disk_name неисправны.', 'unhealthy_backup_found_not_reachable' => 'Не удается достичь места назначения резервной копии. :error', 'unhealthy_backup_found_empty' => 'Резервные копии для этого приложения отсутствуют.', 'unhealthy_backup_found_old' => 'Последнее резервное копирование созданное :date является устаревшим.', 'unhealthy_backup_found_unknown' => 'Извините, точная причина не может быть определена.', 'unhealthy_backup_found_full' => 'Резервные копии используют слишком много памяти. Используется :disk_usage что выше допустимого предела: :disk_limit.', 'no_backups_info' => 'Резервных копий еще не было', 'application_name' => 'Имя приложения', 'backup_name' => 'Имя резервной копии', 'disk' => 'Диск', 'newest_backup_size' => 'Размер последней резервной копии', 'number_of_backups' => 'Количество резервных копий', 'total_storage_used' => 'Общий объем используемого хранилища', 'newest_backup_date' => 'Дата последней резервной копии', 'oldest_backup_date' => 'Дата самой старой резервной копии', ]; laravel-backup/resources/lang/zh-TW/notifications.php 0000644 00000004674 15002154456 0016740 0 ustar 00 <?php return [ 'exception_message' => '異常訊息: :message', 'exception_trace' => '異常追蹤: :trace', 'exception_message_title' => '異常訊息', 'exception_trace_title' => '異常追蹤', 'backup_failed_subject' => ':application_name 備份失敗', 'backup_failed_body' => '重要說明:備份 :application_name 時發生錯誤', 'backup_successful_subject' => ':application_name 備份成功', 'backup_successful_subject_title' => '備份成功!', 'backup_successful_body' => '好消息, :application_name 備份成功,位於磁碟 :disk_name 中。', 'cleanup_failed_subject' => '清除 :application_name 的備份失敗。', 'cleanup_failed_body' => '清除備份 :application_name 時發生錯誤', 'cleanup_successful_subject' => '成功清除 :application_name 的備份', 'cleanup_successful_subject_title' => '成功清除備份!', 'cleanup_successful_body' => '成功清除 :disk_name 磁碟上 :application_name 的備份。', 'healthy_backup_found_subject' => ':disk_name 磁碟上 :application_name 的備份是健康的', 'healthy_backup_found_subject_title' => ':application_name 的備份是健康的', 'healthy_backup_found_body' => ':application_name 的備份是健康的。幹的好!', 'unhealthy_backup_found_subject' => '重要說明::application_name 的備份不健康', 'unhealthy_backup_found_subject_title' => '重要說明::application_name 備份不健康。 :problem', 'unhealthy_backup_found_body' => ':disk_name 磁碟上 :application_name 的備份不健康。', 'unhealthy_backup_found_not_reachable' => '無法訪問備份目標。 :error', 'unhealthy_backup_found_empty' => '根本沒有此應用程序的備份。', 'unhealthy_backup_found_old' => '最近的備份創建於 :date ,太舊了。', 'unhealthy_backup_found_unknown' => '對不起,確切原因無法確定。', 'unhealthy_backup_found_full' => '備份佔用了太多存儲空間。當前佔用了 :disk_usage ,高於允許的限制 :disk_limit。', 'no_backups_info' => '尚未進行任何備份', 'application_name' => '應用名稱', 'backup_name' => '備份名稱', 'disk' => '磁碟', 'newest_backup_size' => '最新備份大小', 'number_of_backups' => '備份數量', 'total_storage_used' => '使用的總存儲量', 'newest_backup_date' => '最新備份大小', 'oldest_backup_date' => '最早的備份大小', ]; laravel-backup/resources/lang/zh-CN/notifications.php 0000644 00000004674 15002154456 0016706 0 ustar 00 <?php return [ 'exception_message' => '异常信息: :message', 'exception_trace' => '异常跟踪: :trace', 'exception_message_title' => '异常信息', 'exception_trace_title' => '异常跟踪', 'backup_failed_subject' => ':application_name 备份失败', 'backup_failed_body' => '重要说明:备份 :application_name 时发生错误', 'backup_successful_subject' => ':application_name 备份成功', 'backup_successful_subject_title' => '备份成功!', 'backup_successful_body' => '好消息, :application_name 备份成功,位于磁盘 :disk_name 中。', 'cleanup_failed_subject' => '清除 :application_name 的备份失败。', 'cleanup_failed_body' => '清除备份 :application_name 时发生错误', 'cleanup_successful_subject' => '成功清除 :application_name 的备份', 'cleanup_successful_subject_title' => '成功清除备份!', 'cleanup_successful_body' => '成功清除 :disk_name 磁盘上 :application_name 的备份。', 'healthy_backup_found_subject' => ':disk_name 磁盘上 :application_name 的备份是健康的', 'healthy_backup_found_subject_title' => ':application_name 的备份是健康的', 'healthy_backup_found_body' => ':application_name 的备份是健康的。干的好!', 'unhealthy_backup_found_subject' => '重要说明::application_name 的备份不健康', 'unhealthy_backup_found_subject_title' => '重要说明::application_name 备份不健康。 :problem', 'unhealthy_backup_found_body' => ':disk_name 磁盘上 :application_name 的备份不健康。', 'unhealthy_backup_found_not_reachable' => '无法访问备份目标。 :error', 'unhealthy_backup_found_empty' => '根本没有此应用程序的备份。', 'unhealthy_backup_found_old' => '最近的备份创建于 :date ,太旧了。', 'unhealthy_backup_found_unknown' => '对不起,确切原因无法确定。', 'unhealthy_backup_found_full' => '备份占用了太多存储空间。当前占用了 :disk_usage ,高于允许的限制 :disk_limit。', 'no_backups_info' => '尚未进行任何备份', 'application_name' => '应用名称', 'backup_name' => '备份名称', 'disk' => '磁盘', 'newest_backup_size' => '最新备份大小', 'number_of_backups' => '备份数量', 'total_storage_used' => '使用的总存储量', 'newest_backup_date' => '最新备份大小', 'oldest_backup_date' => '最旧的备份大小', ]; laravel-backup/resources/lang/ar/notifications.php 0000644 00000007025 15002154456 0016362 0 ustar 00 <?php return [ 'exception_message' => 'رسالة استثناء: :message', 'exception_trace' => 'تتبع الإستثناء: :trace', 'exception_message_title' => 'رسالة استثناء', 'exception_trace_title' => 'تتبع الإستثناء', 'backup_failed_subject' => 'أخفق النسخ الاحتياطي لل :application_name', 'backup_failed_body' => 'مهم: حدث خطأ أثناء النسخ الاحتياطي :application_name', 'backup_successful_subject' => 'نسخ احتياطي جديد ناجح ل :application_name', 'backup_successful_subject_title' => 'نجاح النسخ الاحتياطي الجديد!', 'backup_successful_body' => 'أخبار عظيمة، نسخة احتياطية جديدة ل :application_name تم إنشاؤها بنجاح على القرص المسمى :disk_name.', 'cleanup_failed_subject' => 'فشل تنظيف النسخ الاحتياطي للتطبيق :application_name .', 'cleanup_failed_body' => 'حدث خطأ أثناء تنظيف النسخ الاحتياطية ل :application_name', 'cleanup_successful_subject' => 'تنظيف النسخ الاحتياطية ل :application_name تمت بنجاح', 'cleanup_successful_subject_title' => 'تنظيف النسخ الاحتياطية تم بنجاح!', 'cleanup_successful_body' => 'تنظيف النسخ الاحتياطية ل :application_name على القرص المسمى :disk_name تم بنجاح.', 'healthy_backup_found_subject' => 'النسخ الاحتياطية ل :application_name على القرص :disk_name صحية', 'healthy_backup_found_subject_title' => 'النسخ الاحتياطية ل :application_name صحية', 'healthy_backup_found_body' => 'تعتبر النسخ الاحتياطية ل :application_name صحية. عمل جيد!', 'unhealthy_backup_found_subject' => 'مهم: النسخ الاحتياطية ل :application_name غير صحية', 'unhealthy_backup_found_subject_title' => 'مهم: النسخ الاحتياطية ل :application_name غير صحية. :problem', 'unhealthy_backup_found_body' => 'النسخ الاحتياطية ل :application_name على القرص :disk_name غير صحية.', 'unhealthy_backup_found_not_reachable' => 'لا يمكن الوصول إلى وجهة النسخ الاحتياطي. :error', 'unhealthy_backup_found_empty' => 'لا توجد نسخ احتياطية لهذا التطبيق على الإطلاق.', 'unhealthy_backup_found_old' => 'تم إنشاء أحدث النسخ الاحتياطية في :date وتعتبر قديمة جدا.', 'unhealthy_backup_found_unknown' => 'عذرا، لا يمكن تحديد سبب دقيق.', 'unhealthy_backup_found_full' => 'النسخ الاحتياطية تستخدم الكثير من التخزين. الاستخدام الحالي هو :disk_usage وهو أعلى من الحد المسموح به من :disk_limit.', 'no_backups_info' => 'لم يتم عمل نسخ احتياطية حتى الآن', 'application_name' => 'اسم التطبيق', 'backup_name' => 'اسم النسخ الاحتياطي', 'disk' => 'القرص', 'newest_backup_size' => 'أحدث حجم للنسخ الاحتياطي', 'number_of_backups' => 'عدد النسخ الاحتياطية', 'total_storage_used' => 'إجمالي مساحة التخزين المستخدمة', 'newest_backup_date' => 'أحدث تاريخ النسخ الاحتياطي', 'oldest_backup_date' => 'أقدم تاريخ نسخ احتياطي', ]; laravel-backup/resources/lang/pt-BR/notifications.php 0000644 00000005651 15002154456 0016707 0 ustar 00 <?php return [ 'exception_message' => 'Exception message: :message', 'exception_trace' => 'Exception trace: :trace', 'exception_message_title' => 'Exception message', 'exception_trace_title' => 'Exception trace', 'backup_failed_subject' => 'Falha no backup da aplicação :application_name', 'backup_failed_body' => 'Importante: Ocorreu um erro ao fazer o backup da aplicação :application_name', 'backup_successful_subject' => 'Backup realizado com sucesso: :application_name', 'backup_successful_subject_title' => 'Backup Realizado com sucesso!', 'backup_successful_body' => 'Boas notícias, um novo backup da aplicação :application_name foi criado no disco :disk_name.', 'cleanup_failed_subject' => 'Falha na limpeza dos backups da aplicação :application_name.', 'cleanup_failed_body' => 'Um erro ocorreu ao fazer a limpeza dos backups da aplicação :application_name', 'cleanup_successful_subject' => 'Limpeza dos backups da aplicação :application_name concluída!', 'cleanup_successful_subject_title' => 'Limpeza dos backups concluída!', 'cleanup_successful_body' => 'A limpeza dos backups da aplicação :application_name no disco :disk_name foi concluída.', 'healthy_backup_found_subject' => 'Os backups da aplicação :application_name no disco :disk_name estão em dia', 'healthy_backup_found_subject_title' => 'Os backups da aplicação :application_name estão em dia', 'healthy_backup_found_body' => 'Os backups da aplicação :application_name estão em dia. Bom trabalho!', 'unhealthy_backup_found_subject' => 'Importante: Os backups da aplicação :application_name não estão em dia', 'unhealthy_backup_found_subject_title' => 'Importante: Os backups da aplicação :application_name não estão em dia. :problem', 'unhealthy_backup_found_body' => 'Os backups da aplicação :application_name no disco :disk_name não estão em dia.', 'unhealthy_backup_found_not_reachable' => 'O destino dos backups não pode ser alcançado. :error', 'unhealthy_backup_found_empty' => 'Não existem backups para essa aplicação.', 'unhealthy_backup_found_old' => 'O último backup realizado em :date é considerado muito antigo.', 'unhealthy_backup_found_unknown' => 'Desculpe, a exata razão não pode ser encontrada.', 'unhealthy_backup_found_full' => 'Os backups estão usando muito espaço de armazenamento. A utilização atual é de :disk_usage, o que é maior que o limite permitido de :disk_limit.', 'no_backups_info' => 'Nenhum backup foi feito ainda', 'application_name' => 'Nome da Aplicação', 'backup_name' => 'Nome de backup', 'disk' => 'Disco', 'newest_backup_size' => 'Tamanho do backup mais recente', 'number_of_backups' => 'Número de backups', 'total_storage_used' => 'Armazenamento total usado', 'newest_backup_date' => 'Data do backup mais recente', 'oldest_backup_date' => 'Data do backup mais antigo', ]; laravel-backup/resources/lang/uk/notifications.php 0000644 00000007607 15002154456 0016405 0 ustar 00 <?php return [ 'exception_message' => 'Повідомлення про помилку: :message', 'exception_trace' => 'Деталі помилки: :trace', 'exception_message_title' => 'Повідомлення помилки', 'exception_trace_title' => 'Деталі помилки', 'backup_failed_subject' => 'Не вдалось зробити резервну копію :application_name', 'backup_failed_body' => 'Увага: Трапилась помилка під час резервного копіювання :application_name', 'backup_successful_subject' => 'Успішне резервне копіювання :application_name', 'backup_successful_subject_title' => 'Успішно створена резервна копія!', 'backup_successful_body' => 'Чудова новина, нова резервна копія :application_name успішно створена і збережена на диск :disk_name.', 'cleanup_failed_subject' => 'Не вдалось очистити резервні копії :application_name', 'cleanup_failed_body' => 'Сталася помилка під час очищення резервних копій :application_name', 'cleanup_successful_subject' => 'Успішне очищення від резервних копій :application_name', 'cleanup_successful_subject_title' => 'Очищення резервних копій пройшло вдало!', 'cleanup_successful_body' => 'Очищенно від старих резервних копій :application_name на диску :disk_name пойшло успішно.', 'healthy_backup_found_subject' => 'Резервна копія :application_name з диску :disk_name установлена', 'healthy_backup_found_subject_title' => 'Резервна копія :application_name установлена', 'healthy_backup_found_body' => 'Резервна копія :application_name успішно установлена. Хороша робота!', 'unhealthy_backup_found_subject' => 'Увага: резервна копія :application_name не установилась', 'unhealthy_backup_found_subject_title' => 'Увага: резервна копія для :application_name не установилась. :problem', 'unhealthy_backup_found_body' => 'Резервна копія для :application_name на диску :disk_name не установилась.', 'unhealthy_backup_found_not_reachable' => 'Резервна копія не змогла установитись. :error', 'unhealthy_backup_found_empty' => 'Резервні копії для цього додатку відсутні.', 'unhealthy_backup_found_old' => 'Останнє резервне копіювання створено :date є застарілим.', 'unhealthy_backup_found_unknown' => 'Вибачте, але ми не змогли визначити точну причину.', 'unhealthy_backup_found_full' => 'Резервні копії використовують занадто багато пам`яті. Використовується :disk_usage що вище за допустиму межу :disk_limit.', 'no_backups_info' => 'Резервних копій ще не було зроблено', 'application_name' => 'Назва програми', 'backup_name' => 'Резервне ім’я', 'disk' => 'Диск', 'newest_backup_size' => 'Найновіший розмір резервної копії', 'number_of_backups' => 'Кількість резервних копій', 'total_storage_used' => 'Загальний обсяг використаного сховища', 'newest_backup_date' => 'Найновіший розмір резервної копії', 'oldest_backup_date' => 'Найстаріший розмір резервної копії', ]; laravel-backup/resources/lang/hi/notifications.php 0000644 00000010262 15002154456 0016355 0 ustar 00 <?php return [ 'exception_message' => 'अपवाद संदेश: :message', 'exception_trace' => 'अपवाद निशान: :trace', 'exception_message_title' => 'अपवादी संदेश', 'exception_trace_title' => 'अपवाद निशान', 'backup_failed_subject' => ':application_name का बैकअप असफल रहा', 'backup_failed_body' => 'जरूरी सुचना: :application_name का बैकअप लेते समय असफल रहे', 'backup_successful_subject' => ':application_name का बैकअप सफल रहा', 'backup_successful_subject_title' => 'बैकअप सफल रहा!', 'backup_successful_body' => 'खुशखबर, :application_name का बैकअप :disk_name पर संग्रहित करने मे सफल रहे.', 'cleanup_failed_subject' => ':application_name के बैकअप की सफाई असफल रही.', 'cleanup_failed_body' => ':application_name के बैकअप की सफाई करते समय कुछ बाधा आयी है.', 'cleanup_successful_subject' => ':application_name के बैकअप की सफाई सफल रही', 'cleanup_successful_subject_title' => 'बैकअप की सफाई सफल रही!', 'cleanup_successful_body' => ':application_name का बैकअप जो :disk_name नाम की डिस्क पर संग्रहित है, उसकी सफाई सफल रही.', 'healthy_backup_found_subject' => ':disk_name नाम की डिस्क पर संग्रहित :application_name के बैकअप स्वस्थ है', 'healthy_backup_found_subject_title' => ':application_name के सभी बैकअप स्वस्थ है', 'healthy_backup_found_body' => 'बहुत बढ़िया! :application_name के सभी बैकअप स्वस्थ है.', 'unhealthy_backup_found_subject' => 'जरूरी सुचना : :application_name के बैकअप अस्वस्थ है', 'unhealthy_backup_found_subject_title' => 'जरूरी सुचना : :application_name के बैकअप :problem के बजेसे अस्वस्थ है', 'unhealthy_backup_found_body' => ':disk_name नाम की डिस्क पर संग्रहित :application_name के बैकअप अस्वस्थ है', 'unhealthy_backup_found_not_reachable' => ':error के बजेसे बैकअप की मंजिल तक पोहोच नहीं सकते.', 'unhealthy_backup_found_empty' => 'इस एप्लीकेशन का कोई भी बैकअप नहीं है.', 'unhealthy_backup_found_old' => 'हालहीमें :date को लिया हुआ बैकअप बहुत पुराना है.', 'unhealthy_backup_found_unknown' => 'माफ़ कीजिये, सही कारण निर्धारित नहीं कर सकते.', 'unhealthy_backup_found_full' => 'सभी बैकअप बहुत ज्यादा जगह का उपयोग कर रहे है. फ़िलहाल सभी बैकअप :disk_usage जगह का उपयोग कर रहे है, जो की :disk_limit अनुमति सीमा से अधिक का है.', 'no_backups_info' => 'अभी तक कोई बैकअप नहीं बनाया गया था', 'application_name' => 'आवेदन का नाम', 'backup_name' => 'बैकअप नाम', 'disk' => 'डिस्क', 'newest_backup_size' => 'नवीनतम बैकअप आकार', 'number_of_backups' => 'बैकअप की संख्या', 'total_storage_used' => 'उपयोग किया गया कुल संग्रहण', 'newest_backup_date' => 'नवीनतम बैकअप आकार', 'oldest_backup_date' => 'सबसे पुराना बैकअप आकार', ]; laravel-backup/resources/lang/fr/notifications.php 0000644 00000006041 15002154456 0016364 0 ustar 00 <?php return [ 'exception_message' => 'Message de l\'exception : :message', 'exception_trace' => 'Trace de l\'exception : :trace', 'exception_message_title' => 'Message de l\'exception', 'exception_trace_title' => 'Trace de l\'exception', 'backup_failed_subject' => 'Échec de la sauvegarde de :application_name', 'backup_failed_body' => 'Important : Une erreur est survenue lors de la sauvegarde de :application_name', 'backup_successful_subject' => 'Succès de la sauvegarde de :application_name', 'backup_successful_subject_title' => 'Sauvegarde créée avec succès !', 'backup_successful_body' => 'Bonne nouvelle, une nouvelle sauvegarde de :application_name a été créée avec succès sur le disque nommé :disk_name.', 'cleanup_failed_subject' => 'Le nettoyage des sauvegardes de :application_name a echoué.', 'cleanup_failed_body' => 'Une erreur est survenue lors du nettoyage des sauvegardes de :application_name', 'cleanup_successful_subject' => 'Succès du nettoyage des sauvegardes de :application_name', 'cleanup_successful_subject_title' => 'Sauvegardes nettoyées avec succès !', 'cleanup_successful_body' => 'Le nettoyage des sauvegardes de :application_name sur le disque nommé :disk_name a été effectué avec succès.', 'healthy_backup_found_subject' => 'Les sauvegardes pour :application_name sur le disque :disk_name sont saines', 'healthy_backup_found_subject_title' => 'Les sauvegardes pour :application_name sont saines', 'healthy_backup_found_body' => 'Les sauvegardes pour :application_name sont considérées saines. Bon travail !', 'unhealthy_backup_found_subject' => 'Important : Les sauvegardes pour :application_name sont corrompues', 'unhealthy_backup_found_subject_title' => 'Important : Les sauvegardes pour :application_name sont corrompues. :problem', 'unhealthy_backup_found_body' => 'Les sauvegardes pour :application_name sur le disque :disk_name sont corrompues.', 'unhealthy_backup_found_not_reachable' => 'La destination de la sauvegarde n\'est pas accessible. :error', 'unhealthy_backup_found_empty' => 'Il n\'y a aucune sauvegarde pour cette application.', 'unhealthy_backup_found_old' => 'La dernière sauvegarde du :date est considérée trop vieille.', 'unhealthy_backup_found_unknown' => 'Désolé, une raison exacte ne peut être déterminée.', 'unhealthy_backup_found_full' => 'Les sauvegardes utilisent trop d\'espace disque. L\'utilisation actuelle est de :disk_usage alors que la limite autorisée est de :disk_limit.', 'no_backups_info' => 'Aucune sauvegarde n\'a encore été effectuée', 'application_name' => 'Nom de l\'application', 'backup_name' => 'Nom de la sauvegarde', 'disk' => 'Disque', 'newest_backup_size' => 'Taille de la sauvegarde la plus récente', 'number_of_backups' => 'Nombre de sauvegardes', 'total_storage_used' => 'Stockage total utilisé', 'newest_backup_date' => 'Date de la sauvegarde la plus récente', 'oldest_backup_date' => 'Date de la sauvegarde la plus ancienne', ]; laravel-backup/resources/lang/da/notifications.php 0000644 00000005227 15002154456 0016346 0 ustar 00 <?php return [ 'exception_message' => 'Fejlbesked: :message', 'exception_trace' => 'Fejl trace: :trace', 'exception_message_title' => 'Fejlbesked', 'exception_trace_title' => 'Fejl trace', 'backup_failed_subject' => 'Backup af :application_name fejlede', 'backup_failed_body' => 'Vigtigt: Der skete en fejl under backup af :application_name', 'backup_successful_subject' => 'Ny backup af :application_name oprettet', 'backup_successful_subject_title' => 'Ny backup!', 'backup_successful_body' => 'Gode nyheder - der blev oprettet en ny backup af :application_name på disken :disk_name.', 'cleanup_failed_subject' => 'Oprydning af backups for :application_name fejlede.', 'cleanup_failed_body' => 'Der skete en fejl under oprydning af backups for :application_name', 'cleanup_successful_subject' => 'Oprydning af backups for :application_name gennemført', 'cleanup_successful_subject_title' => 'Backup oprydning gennemført!', 'cleanup_successful_body' => 'Oprydningen af backups for :application_name på disken :disk_name er gennemført.', 'healthy_backup_found_subject' => 'Alle backups for :application_name på disken :disk_name er OK', 'healthy_backup_found_subject_title' => 'Alle backups for :application_name er OK', 'healthy_backup_found_body' => 'Alle backups for :application_name er ok. Godt gået!', 'unhealthy_backup_found_subject' => 'Vigtigt: Backups for :application_name fejlbehæftede', 'unhealthy_backup_found_subject_title' => 'Vigtigt: Backups for :application_name er fejlbehæftede. :problem', 'unhealthy_backup_found_body' => 'Backups for :application_name på disken :disk_name er fejlbehæftede.', 'unhealthy_backup_found_not_reachable' => 'Backup destinationen kunne ikke findes. :error', 'unhealthy_backup_found_empty' => 'Denne applikation har ingen backups overhovedet.', 'unhealthy_backup_found_old' => 'Den seneste backup fra :date er for gammel.', 'unhealthy_backup_found_unknown' => 'Beklager, en præcis årsag kunne ikke findes.', 'unhealthy_backup_found_full' => 'Backups bruger for meget plads. Nuværende disk forbrug er :disk_usage, hvilket er mere end den tilladte grænse på :disk_limit.', 'no_backups_info' => 'Der blev ikke foretaget nogen sikkerhedskopier endnu', 'application_name' => 'Ansøgningens navn', 'backup_name' => 'Backup navn', 'disk' => 'Disk', 'newest_backup_size' => 'Nyeste backup-størrelse', 'number_of_backups' => 'Antal sikkerhedskopier', 'total_storage_used' => 'Samlet lagerplads brugt', 'newest_backup_date' => 'Nyeste backup-størrelse', 'oldest_backup_date' => 'Ældste backup-størrelse', ]; laravel-backup/resources/lang/bn/notifications.php 0000644 00000011244 15002154456 0016355 0 ustar 00 <?php return [ 'exception_message' => 'এক্সসেপশন বার্তা: :message', 'exception_trace' => 'এক্সসেপশন ট্রেস: :trace', 'exception_message_title' => 'এক্সসেপশন message', 'exception_trace_title' => 'এক্সসেপশন ট্রেস', 'backup_failed_subject' => ':application_name এর ব্যাকআপ ব্যর্থ হয়েছে।', 'backup_failed_body' => 'গুরুত্বপূর্ণঃ :application_name ব্যাক আপ করার সময় একটি ত্রুটি ঘটেছে।', 'backup_successful_subject' => ':application_name এর নতুন ব্যাকআপ সফল হয়েছে।', 'backup_successful_subject_title' => 'নতুন ব্যাকআপ সফল হয়েছে!', 'backup_successful_body' => 'খুশির খবর, :application_name এর নতুন ব্যাকআপ :disk_name ডিস্কে সফলভাবে তৈরি হয়েছে।', 'cleanup_failed_subject' => ':application_name ব্যাকআপগুলি সাফ করতে ব্যর্থ হয়েছে।', 'cleanup_failed_body' => ':application_name ব্যাকআপগুলি সাফ করার সময় একটি ত্রুটি ঘটেছে।', 'cleanup_successful_subject' => ':application_name এর ব্যাকআপগুলি সফলভাবে সাফ করা হয়েছে।', 'cleanup_successful_subject_title' => 'ব্যাকআপগুলি সফলভাবে সাফ করা হয়েছে!', 'cleanup_successful_body' => ':application_name এর ব্যাকআপগুলি :disk_name ডিস্ক থেকে সফলভাবে সাফ করা হয়েছে।', 'healthy_backup_found_subject' => ':application_name এর ব্যাকআপগুলি :disk_name ডিস্কে স্বাস্থ্যকর অবস্থায় আছে।', 'healthy_backup_found_subject_title' => ':application_name এর ব্যাকআপগুলি স্বাস্থ্যকর অবস্থায় আছে।', 'healthy_backup_found_body' => ':application_name এর ব্যাকআপগুলি স্বাস্থ্যকর বিবেচনা করা হচ্ছে। Good job!', 'unhealthy_backup_found_subject' => 'গুরুত্বপূর্ণঃ :application_name এর ব্যাকআপগুলি অস্বাস্থ্যকর অবস্থায় আছে।', 'unhealthy_backup_found_subject_title' => 'গুরুত্বপূর্ণঃ :application_name এর ব্যাকআপগুলি অস্বাস্থ্যকর অবস্থায় আছে। :problem', 'unhealthy_backup_found_body' => ':disk_name ডিস্কের :application_name এর ব্যাকআপগুলি অস্বাস্থ্যকর অবস্থায় আছে।', 'unhealthy_backup_found_not_reachable' => 'ব্যাকআপ গন্তব্যে পৌঁছানো যায় নি। :error', 'unhealthy_backup_found_empty' => 'এই অ্যাপ্লিকেশনটির কোনও ব্যাকআপ নেই।', 'unhealthy_backup_found_old' => 'সর্বশেষ ব্যাকআপ যেটি :date এই তারিখে করা হয়েছে, সেটি খুব পুরানো।', 'unhealthy_backup_found_unknown' => 'দুঃখিত, সঠিক কারণ নির্ধারণ করা সম্ভব হয়নি।', 'unhealthy_backup_found_full' => 'ব্যাকআপগুলি অতিরিক্ত স্টোরেজ ব্যবহার করছে। বর্তমান ব্যবহারের পরিমান :disk_usage যা অনুমোদিত সীমা :disk_limit এর বেশি।', 'no_backups_info' => 'কোনো ব্যাকআপ এখনও তৈরি হয়নি', 'application_name' => 'আবেদনের নাম', 'backup_name' => 'ব্যাকআপের নাম', 'disk' => 'ডিস্ক', 'newest_backup_size' => 'নতুন ব্যাকআপ আকার', 'number_of_backups' => 'ব্যাকআপের সংখ্যা', 'total_storage_used' => 'ব্যবহৃত মোট সঞ্চয়স্থান', 'newest_backup_date' => 'নতুন ব্যাকআপের তারিখ', 'oldest_backup_date' => 'পুরানো ব্যাকআপের তারিখ', ]; laravel-backup/resources/lang/tr/notifications.php 0000644 00000005316 15002154456 0016406 0 ustar 00 <?php return [ 'exception_message' => 'Hata mesajı: :message', 'exception_trace' => 'Hata izleri: :trace', 'exception_message_title' => 'Hata mesajı', 'exception_trace_title' => 'Hata izleri', 'backup_failed_subject' => 'Yedeklenemedi :application_name', 'backup_failed_body' => 'Önemli: Yedeklenirken bir hata oluştu :application_name', 'backup_successful_subject' => 'Başarılı :application_name yeni yedeklemesi', 'backup_successful_subject_title' => 'Başarılı bir yeni yedekleme!', 'backup_successful_body' => 'Harika bir haber, :application_name âit yeni bir yedekleme :disk_name adlı diskte başarıyla oluşturuldu.', 'cleanup_failed_subject' => ':application_name yedeklemeleri temizlenmesi başarısız.', 'cleanup_failed_body' => ':application_name yedeklerini temizlerken bir hata oluştu ', 'cleanup_successful_subject' => ':application_name yedeklemeleri temizlenmesi başarılı.', 'cleanup_successful_subject_title' => 'Yedeklerin temizlenmesi başarılı!', 'cleanup_successful_body' => ':application_name yedeklemeleri temizlenmesi ,:disk_name diskinden silindi', 'healthy_backup_found_subject' => ':application_name yedeklenmesi ,:disk_name adlı diskte sağlıklı', 'healthy_backup_found_subject_title' => ':application_name yedeklenmesi sağlıklı', 'healthy_backup_found_body' => ':application_name için yapılan yedeklemeler sağlıklı sayılır. Aferin!', 'unhealthy_backup_found_subject' => 'Önemli: :application_name için yedeklemeler sağlıksız', 'unhealthy_backup_found_subject_title' => 'Önemli: :application_name için yedeklemeler sağlıksız. :problem', 'unhealthy_backup_found_body' => 'Yedeklemeler: :application_name disk: :disk_name sağlıksız.', 'unhealthy_backup_found_not_reachable' => 'Yedekleme hedefine ulaşılamıyor. :error', 'unhealthy_backup_found_empty' => 'Bu uygulamanın yedekleri yok.', 'unhealthy_backup_found_old' => ':date tarihinde yapılan en son yedekleme çok eski kabul ediliyor.', 'unhealthy_backup_found_unknown' => 'Üzgünüm, kesin bir sebep belirlenemiyor.', 'unhealthy_backup_found_full' => 'Yedeklemeler çok fazla depolama alanı kullanıyor. Şu anki kullanım: :disk_usage, izin verilen sınırdan yüksek: :disk_limit.', 'no_backups_info' => 'Henüz yedekleme yapılmadı', 'application_name' => 'Uygulama Adı', 'backup_name' => 'Yedek adı', 'disk' => 'Disk', 'newest_backup_size' => 'En yeni yedekleme boyutu', 'number_of_backups' => 'Yedekleme sayısı', 'total_storage_used' => 'Kullanılan toplam depolama alanı', 'newest_backup_date' => 'En yeni yedekleme boyutu', 'oldest_backup_date' => 'En eski yedekleme boyutu', ]; laravel-backup/resources/lang/nl/notifications.php 0000644 00000005521 15002154456 0016370 0 ustar 00 <?php return [ 'exception_message' => 'Fout bericht: :message', 'exception_trace' => 'Fout trace: :trace', 'exception_message_title' => 'Fout bericht', 'exception_trace_title' => 'Fout trace', 'backup_failed_subject' => 'Back-up van :application_name mislukt', 'backup_failed_body' => 'Belangrijk: Er ging iets fout tijdens het maken van een back-up van :application_name', 'backup_successful_subject' => 'Succesvolle nieuwe back-up van :application_name', 'backup_successful_subject_title' => 'Succesvolle nieuwe back-up!', 'backup_successful_body' => 'Goed nieuws, een nieuwe back-up van :application_name was succesvol aangemaakt op de schijf genaamd :disk_name.', 'cleanup_failed_subject' => 'Het opschonen van de back-ups van :application_name is mislukt.', 'cleanup_failed_body' => 'Er ging iets fout tijdens het opschonen van de back-ups van :application_name', 'cleanup_successful_subject' => 'Opschonen van :application_name back-ups was succesvol.', 'cleanup_successful_subject_title' => 'Opschonen van back-ups was succesvol!', 'cleanup_successful_body' => 'Het opschonen van de :application_name back-ups op de schijf genaamd :disk_name was succesvol.', 'healthy_backup_found_subject' => 'De back-ups voor :application_name op schijf :disk_name zijn gezond', 'healthy_backup_found_subject_title' => 'De back-ups voor :application_name zijn gezond', 'healthy_backup_found_body' => 'De back-ups voor :application_name worden als gezond beschouwd. Goed gedaan!', 'unhealthy_backup_found_subject' => 'Belangrijk: De back-ups voor :application_name zijn niet meer gezond', 'unhealthy_backup_found_subject_title' => 'Belangrijk: De back-ups voor :application_name zijn niet gezond. :problem', 'unhealthy_backup_found_body' => 'De back-ups voor :application_name op schijf :disk_name zijn niet gezond.', 'unhealthy_backup_found_not_reachable' => 'De back-upbestemming kon niet worden bereikt. :error', 'unhealthy_backup_found_empty' => 'Er zijn geen back-ups van deze applicatie beschikbaar.', 'unhealthy_backup_found_old' => 'De laatste back-up gemaakt op :date is te oud.', 'unhealthy_backup_found_unknown' => 'Sorry, een exacte reden kon niet worden bepaald.', 'unhealthy_backup_found_full' => 'De back-ups gebruiken te veel opslagruimte. Momenteel wordt er :disk_usage gebruikt wat hoger is dan de toegestane limiet van :disk_limit.', 'no_backups_info' => 'Er zijn nog geen back-ups gemaakt', 'application_name' => 'Naam van de toepassing', 'backup_name' => 'Back-upnaam', 'disk' => 'Schijf', 'newest_backup_size' => 'Nieuwste back-upgrootte', 'number_of_backups' => 'Aantal back-ups', 'total_storage_used' => 'Totale gebruikte opslagruimte', 'newest_backup_date' => 'Datum nieuwste back-up', 'oldest_backup_date' => 'Datum oudste back-up', ]; laravel-backup/resources/lang/fa/notifications.php 0000644 00000007107 15002154456 0016347 0 ustar 00 <?php return [ 'exception_message' => 'پیغام خطا: :message', 'exception_trace' => 'جزییات خطا: :trace', 'exception_message_title' => 'پیغام خطا', 'exception_trace_title' => 'جزییات خطا', 'backup_failed_subject' => 'پشتیبانگیری :application_name با خطا مواجه شد.', 'backup_failed_body' => 'پیغام مهم: هنگام پشتیبانگیری از :application_name خطایی رخ داده است. ', 'backup_successful_subject' => 'نسخه پشتیبان جدید :application_name با موفقیت ساخته شد.', 'backup_successful_subject_title' => 'پشتیبانگیری موفق!', 'backup_successful_body' => 'خبر خوب، به تازگی نسخه پشتیبان :application_name روی دیسک :disk_name با موفقیت ساخته شد. ', 'cleanup_failed_subject' => 'پاکسازی نسخه پشتیبان :application_name انجام نشد.', 'cleanup_failed_body' => 'هنگام پاکسازی نسخه پشتیبان :application_name خطایی رخ داده است.', 'cleanup_successful_subject' => 'پاکسازی نسخه پشتیبان :application_name با موفقیت انجام شد.', 'cleanup_successful_subject_title' => 'پاکسازی نسخه پشتیبان!', 'cleanup_successful_body' => 'پاکسازی نسخه پشتیبان :application_name روی دیسک :disk_name با موفقیت انجام شد.', 'healthy_backup_found_subject' => 'نسخه پشتیبان :application_name روی دیسک :disk_name سالم بود.', 'healthy_backup_found_subject_title' => 'نسخه پشتیبان :application_name سالم بود.', 'healthy_backup_found_body' => 'نسخه پشتیبان :application_name به نظر سالم میاد. دمت گرم!', 'unhealthy_backup_found_subject' => 'خبر مهم: نسخه پشتیبان :application_name سالم نبود.', 'unhealthy_backup_found_subject_title' => 'خبر مهم: نسخه پشتیبان :application_name سالم نبود. :problem', 'unhealthy_backup_found_body' => 'نسخه پشتیبان :application_name روی دیسک :disk_name سالم نبود.', 'unhealthy_backup_found_not_reachable' => 'مقصد پشتیبانگیری در دسترس نبود. :error', 'unhealthy_backup_found_empty' => 'برای این برنامه هیچ نسخه پشتیبانی وجود ندارد.', 'unhealthy_backup_found_old' => 'آخرین نسخه پشتیبان برای تاریخ :date است، که به نظر خیلی قدیمی میاد. ', 'unhealthy_backup_found_unknown' => 'متاسفانه دلیل دقیقی قابل تعیین نیست.', 'unhealthy_backup_found_full' => 'نسخههای پشتیبان حجم زیادی اشغال کردهاند. میزان دیسک استفادهشده :disk_usage است که از میزان مجاز :disk_limit فراتر رفته است. ', 'no_backups_info' => 'هنوز نسخه پشتیبان تهیه نشده است', 'application_name' => 'نام نرمافزار', 'backup_name' => 'نام نسخه پشتیبان', 'disk' => 'دیسک', 'newest_backup_size' => 'اندازه جدیدترین نسخه پشتیبان', 'number_of_backups' => 'تعداد نسخههای پشتیبان', 'total_storage_used' => 'کل فضای ذخیرهسازی استفادهشده', 'newest_backup_date' => 'تاریخ جدیدترین نسخه پشتیبان', 'oldest_backup_date' => 'تاریخ قدیمیترین نسخه پشتیبان', ]; laravel-backup/README.md 0000644 00000010546 15002154456 0010724 0 ustar 00 <p align="center"><img src="/art/socialcard.png" alt="Social Card of Laravel Activity Log"></p> # A modern backup solution for Laravel apps [](https://packagist.org/packages/spatie/laravel-backup) [](LICENSE.md)  [](https://scrutinizer-ci.com/g/spatie/laravel-backup) [](https://packagist.org/packages/spatie/laravel-backup) This Laravel package [creates a backup of your application](https://spatie.be/docs/laravel-backup/v8/taking-backups/overview). The backup is a zip file that contains all files in the directories you specify along with a dump of your database. The backup can be stored on [any of the filesystems you have configured in Laravel](https://laravel.com/docs/filesystem). Feeling paranoid about backups? No problem! You can backup your application to multiple filesystems at once. Once installed taking a backup of your files and databases is very easy. Just issue this artisan command: ``` bash php artisan backup:run ``` But we didn't stop there. The package also provides [a backup monitor to check the health of your backups](https://spatie.be/docs/laravel-backup/v8/monitoring-the-health-of-all-backups/overview). You can be [notified via several channels](https://spatie.be/docs/laravel-backup/v8/sending-notifications/overview) when a problem with one of your backups is found. To avoid using excessive disk space, the package can also [clean up old backups](https://spatie.be/docs/laravel-backup/v8/cleaning-up-old-backups/overview). ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/laravel-backup.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/laravel-backup) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation and usage This package requires PHP 8.0 and Laravel 8.0 or higher. You'll find installation instructions and full documentation on https://spatie.be/docs/laravel-backup. ## Using an older version of PHP / Laravel? If you are on a PHP version below 8.0 or a Laravel version below 8.0 just use an older version of this package. Read the extensive [documentation on version 3](https://spatie.be/docs/laravel-backup/v3), [on version 4](https://spatie.be/docs/laravel-backup/v4), [on version 5](https://spatie.be/docs/laravel-backup/v5) and [on version 6](https://spatie.be/docs/laravel-backup/v6). We won't introduce new features to v6 and below anymore but we will still fix bugs. ## Testing Run the tests with: ``` bash composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security If you discover any security-related issues, please email security@spatie.be instead of using the issue tracker. ## Postcardware You're free to use this package, but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/open-source/postcards). ## Credits - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) And a special thanks to [Caneco](https://twitter.com/caneco) for the logo ✨ ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. laravel-backup/phpstan-baseline.neon 0000644 00000000445 15002154456 0013560 0 ustar 00 parameters: ignoreErrors: - message: "#^Illuminate\\\\Support\\\\Collection\\<\\(int\\|string\\), Spatie\\\\Backup\\\\BackupDestination\\\\BackupCollection\\> does not accept Illuminate\\\\Support\\\\Collection\\.$#" count: 4 path: src/Tasks/Cleanup/Strategies/DefaultStrategy.php laravel-backup/src/Exceptions/NotificationCouldNotBeSent.php 0000644 00000000516 15002154456 0020261 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class NotificationCouldNotBeSent extends Exception { public static function noNotificationClassForEvent($event): self { $eventClass = $event::class; return new static("There is no notification class that can handle event `{$eventClass}`."); } } laravel-backup/src/Exceptions/InvalidCommand.php 0000644 00000000317 15002154456 0015746 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class InvalidCommand extends Exception { public static function create(string $reason): self { return new static($reason); } } laravel-backup/src/Exceptions/InvalidBackupDestination.php 0000644 00000001175 15002154456 0020002 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class InvalidBackupDestination extends Exception { public static function diskNotSet(string $backupName): self { return new static("There is no disk set for the backup named `{$backupName}`."); } public static function connectionError(string $diskName): self { return new static ("There is a connection error when trying to connect to disk named `{$diskName}`"); } public static function writeError(string $diskName): self { return new static ("There was an error trying to write to disk named `{$diskName}`"); } } laravel-backup/src/Exceptions/CannotCreateDbDumper.php 0000644 00000001057 15002154456 0017054 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class CannotCreateDbDumper extends Exception { public static function unsupportedDriver(string $driver): self { $supportedDrivers = collect(config("database.connections"))->keys(); $formattedSupportedDrivers = $supportedDrivers ->map(fn (string $supportedDriver) => "`$supportedDriver`") ->join(glue: ', ', finalGlue: ' or '); return new static("Cannot create a dumper for db driver `{$driver}`. Use {$formattedSupportedDrivers}."); } } laravel-backup/src/Exceptions/InvalidBackupJob.php 0000644 00000001106 15002154456 0016225 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class InvalidBackupJob extends Exception { public static function noDestinationsSpecified(): self { return new static('A backup job cannot run without a destination to backup to!'); } public static function destinationDoesNotExist(string $diskName): self { return new static("There is no backup destination with a disk named `{$diskName}`."); } public static function noFilesToBeBackedUp(): self { return new static('There are no files to be backed up.'); } } laravel-backup/src/Exceptions/InvalidHealthCheck.php 0000644 00000000326 15002154456 0016533 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; class InvalidHealthCheck extends Exception { public static function because(string $message): self { return new static($message); } } laravel-backup/src/Exceptions/InvalidBackupFile.php 0000644 00000001010 15002154456 0016364 0 ustar 00 <?php namespace Spatie\Backup\Exceptions; use Exception; use Spatie\Backup\BackupDestination\Backup; class InvalidBackupFile extends Exception { public static function writeError(string $backupName): self { return new static("There has been an error writing file for the backup named `{$backupName}`."); } public static function readError(Backup $backup): self { $path = $backup->path(); return new static("There has been an error reading the backup `{$path}`"); } } laravel-backup/src/Notifications/Notifications/CleanupHasFailedNotification.php 0000644 00000006124 15002154456 0024063 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\CleanupHasFailed; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; class CleanupHasFailedNotification extends BaseNotification { public function __construct( public CleanupHasFailed $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->error() ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.cleanup_failed_subject', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.cleanup_failed_body', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.exception_message', ['message' => $this->event->exception->getMessage()])) ->line(trans('backup::notifications.exception_trace', ['trace' => $this->event->exception->getTraceAsString()])); $this->backupDestinationProperties()->each(function ($value, $name) use ($mailMessage) { $mailMessage->line("{$name}: $value"); }); return $mailMessage; } public function toSlack(): SlackMessage { return (new SlackMessage()) ->error() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.cleanup_failed_subject', ['application_name' => $this->applicationName()])) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_message_title')) ->content($this->event->exception->getMessage()); }) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_message_trace')) ->content($this->event->exception->getTraceAsString()); }) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); } public function toDiscord(): DiscordMessage { return (new DiscordMessage()) ->error() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title( trans('backup::notifications.cleanup_failed_subject', ['application_name' => $this->applicationName()]) )->fields([ trans('backup::notifications.exception_message_title') => $this->event->exception->getMessage(), ]); } } laravel-backup/src/Notifications/Notifications/BackupHasFailedNotification.php 0000644 00000006013 15002154456 0023676 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\BackupHasFailed; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; class BackupHasFailedNotification extends BaseNotification { public function __construct( public BackupHasFailed $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->error() ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.backup_failed_subject', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.backup_failed_body', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.exception_message', ['message' => $this->event->exception->getMessage()])) ->line(trans('backup::notifications.exception_trace', ['trace' => $this->event->exception->getTraceAsString()])); $this->backupDestinationProperties()->each(fn ($value, $name) => $mailMessage->line("{$name}: $value")); return $mailMessage; } public function toSlack(): SlackMessage { return (new SlackMessage()) ->error() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.backup_failed_subject', ['application_name' => $this->applicationName()])) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_message_title')) ->content($this->event->exception->getMessage()); }) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_trace_title')) ->content($this->event->exception->getTraceAsString()); }) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); } public function toDiscord(): DiscordMessage { return (new DiscordMessage()) ->error() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title(trans('backup::notifications.backup_failed_subject', ['application_name' => $this->applicationName()])) ->fields([ trans('backup::notifications.exception_message_title') => $this->event->exception->getMessage(), ]); } } laravel-backup/src/Notifications/Notifications/HealthyBackupWasFoundNotification.php 0000644 00000004535 15002154456 0025132 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\HealthyBackupWasFound; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; class HealthyBackupWasFoundNotification extends BaseNotification { public function __construct( public HealthyBackupWasFound $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.healthy_backup_found_subject', ['application_name' => $this->applicationName(), 'disk_name' => $this->diskName()])) ->line(trans('backup::notifications.healthy_backup_found_body', ['application_name' => $this->applicationName()])); $this->backupDestinationProperties()->each(function ($value, $name) use ($mailMessage) { $mailMessage->line("{$name}: $value"); }); return $mailMessage; } public function toSlack(): SlackMessage { return (new SlackMessage()) ->success() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.healthy_backup_found_subject_title', ['application_name' => $this->applicationName()])) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); } public function toDiscord(): DiscordMessage { return (new DiscordMessage()) ->success() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title( trans('backup::notifications.healthy_backup_found_subject_title', [ 'application_name' => $this->applicationName(), ]) )->fields($this->backupDestinationProperties()->toArray()); } } laravel-backup/src/Notifications/Notifications/CleanupWasSuccessfulNotification.php 0000644 00000004272 15002154456 0025037 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\CleanupWasSuccessful; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; class CleanupWasSuccessfulNotification extends BaseNotification { public function __construct( public CleanupWasSuccessful $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.cleanup_successful_subject', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.cleanup_successful_body', ['application_name' => $this->applicationName(), 'disk_name' => $this->diskName()])); $this->backupDestinationProperties()->each(function ($value, $name) use ($mailMessage) { $mailMessage->line("{$name}: $value"); }); return $mailMessage; } public function toSlack(): SlackMessage { return (new SlackMessage()) ->success() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.cleanup_successful_subject_title')) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); } public function toDiscord(): DiscordMessage { return (new DiscordMessage()) ->success() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title(trans('backup::notifications.cleanup_successful_subject_title')) ->fields($this->backupDestinationProperties()->toArray()); } } laravel-backup/src/Notifications/Notifications/BackupWasSuccessfulNotification.php 0000644 00000004263 15002154456 0024655 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\BackupWasSuccessful; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; class BackupWasSuccessfulNotification extends BaseNotification { public function __construct( public BackupWasSuccessful $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.backup_successful_subject', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.backup_successful_body', ['application_name' => $this->applicationName(), 'disk_name' => $this->diskName()])); $this->backupDestinationProperties()->each(function ($value, $name) use ($mailMessage) { $mailMessage->line("{$name}: $value"); }); return $mailMessage; } public function toSlack(): SlackMessage { return (new SlackMessage()) ->success() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.backup_successful_subject_title')) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); } public function toDiscord(): DiscordMessage { return (new DiscordMessage()) ->success() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title(trans('backup::notifications.backup_successful_subject_title')) ->fields($this->backupDestinationProperties()->toArray()); } } laravel-backup/src/Notifications/Notifications/UnhealthyBackupWasFoundNotification.php 0000644 00000011332 15002154456 0025466 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Notifications; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Messages\SlackAttachment; use Illuminate\Notifications\Messages\SlackMessage; use Spatie\Backup\Events\UnhealthyBackupWasFound; use Spatie\Backup\Notifications\BaseNotification; use Spatie\Backup\Notifications\Channels\Discord\DiscordMessage; use Spatie\Backup\Tasks\Monitor\HealthCheckFailure; class UnhealthyBackupWasFoundNotification extends BaseNotification { public function __construct( public UnhealthyBackupWasFound $event, ) { } public function toMail(): MailMessage { $mailMessage = (new MailMessage()) ->error() ->from(config('backup.notifications.mail.from.address', config('mail.from.address')), config('backup.notifications.mail.from.name', config('mail.from.name'))) ->subject(trans('backup::notifications.unhealthy_backup_found_subject', ['application_name' => $this->applicationName()])) ->line(trans('backup::notifications.unhealthy_backup_found_body', ['application_name' => $this->applicationName(), 'disk_name' => $this->diskName()])) ->line($this->problemDescription()); $this->backupDestinationProperties()->each(function ($value, $name) use ($mailMessage) { $mailMessage->line("{$name}: $value"); }); if ($this->failure()->wasUnexpected()) { $mailMessage ->line('Health check: '.$this->failure()->healthCheck()->name()) ->line(trans('backup::notifications.exception_message', ['message' => $this->failure()->exception()->getMessage()])) ->line(trans('backup::notifications.exception_trace', ['trace' => $this->failure()->exception()->getTraceAsString()])); } return $mailMessage; } public function toSlack(): SlackMessage { $slackMessage = (new SlackMessage()) ->error() ->from(config('backup.notifications.slack.username'), config('backup.notifications.slack.icon')) ->to(config('backup.notifications.slack.channel')) ->content(trans('backup::notifications.unhealthy_backup_found_subject_title', ['application_name' => $this->applicationName(), 'problem' => $this->problemDescription()])) ->attachment(function (SlackAttachment $attachment) { $attachment->fields($this->backupDestinationProperties()->toArray()); }); if ($this->failure()->wasUnexpected()) { $slackMessage ->attachment(function (SlackAttachment $attachment) { $attachment ->title('Health check') ->content($this->failure()->healthCheck()->name()); }) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_message_title')) ->content($this->failure()->exception()->getMessage()); }) ->attachment(function (SlackAttachment $attachment) { $attachment ->title(trans('backup::notifications.exception_trace_title')) ->content($this->failure()->exception()->getTraceAsString()); }); } return $slackMessage; } public function toDiscord(): DiscordMessage { $discordMessage = (new DiscordMessage()) ->error() ->from(config('backup.notifications.discord.username'), config('backup.notifications.discord.avatar_url')) ->title( trans('backup::notifications.unhealthy_backup_found_subject_title', [ 'application_name' => $this->applicationName(), 'problem' => $this->problemDescription(), ]) )->fields($this->backupDestinationProperties()->toArray()); if ($this->failure()->wasUnexpected()) { $discordMessage ->fields(['Health Check' => $this->failure()->healthCheck()->name()]) ->fields([ trans('backup::notifications.exception_message_title') => $this->failure()->exception()->getMessage(), ]); } return $discordMessage; } protected function problemDescription(): string { if ($this->failure()->wasUnexpected()) { return trans('backup::notifications.unhealthy_backup_found_unknown'); } return $this->failure()->exception()->getMessage(); } protected function failure(): HealthCheckFailure { return $this->event->backupDestinationStatus->getHealthCheckFailure(); } } laravel-backup/src/Notifications/Notifiable.php 0000644 00000001156 15002154456 0015627 0 ustar 00 <?php namespace Spatie\Backup\Notifications; use Illuminate\Notifications\Notifiable as NotifiableTrait; class Notifiable { use NotifiableTrait; public function routeNotificationForMail(): string | array { return config('backup.notifications.mail.to'); } public function routeNotificationForSlack(): string { return config('backup.notifications.slack.webhook_url'); } public function routeNotificationForDiscord(): string { return config('backup.notifications.discord.webhook_url'); } public function getKey(): int { return 1; } } laravel-backup/src/Notifications/BaseNotification.php 0000644 00000005703 15002154456 0016776 0 ustar 00 <?php namespace Spatie\Backup\Notifications; use Illuminate\Notifications\Notification; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Helpers\Format; abstract class BaseNotification extends Notification { public function via(): array { $notificationChannels = config('backup.notifications.notifications.'.static::class); return array_filter($notificationChannels); } public function applicationName(): string { $name = config('app.name') ?? config('app.url') ?? 'Laravel'; $env = app()->environment(); return "{$name} ({$env})"; } public function backupName(): string { return $this->backupDestination()->backupName(); } public function diskName(): string { return $this->backupDestination()->diskName(); } protected function backupDestinationProperties(): Collection { $backupDestination = $this->backupDestination(); if (! $backupDestination) { return collect(); } $backupDestination->fresh(); $newestBackup = $backupDestination->newestBackup(); $oldestBackup = $backupDestination->oldestBackup(); $noBackupsText = trans('backup::notifications.no_backups_info'); $applicationName = trans('backup::notifications.application_name'); $backupName = trans('backup::notifications.backup_name'); $disk = trans('backup::notifications.disk'); $newestBackupSize = trans('backup::notifications.newest_backup_size'); $numberOfBackups = trans('backup::notifications.number_of_backups'); $totalStorageUsed = trans('backup::notifications.total_storage_used'); $newestBackupDate = trans('backup::notifications.newest_backup_date'); $oldestBackupDate = trans('backup::notifications.oldest_backup_date'); return collect([ $applicationName => $this->applicationName(), $backupName => $this->backupName(), $disk => $backupDestination->diskName(), $newestBackupSize => $newestBackup ? Format::humanReadableSize($newestBackup->sizeInBytes()) : $noBackupsText, $numberOfBackups => (string) $backupDestination->backups()->count(), $totalStorageUsed => Format::humanReadableSize($backupDestination->backups()->size()), $newestBackupDate => $newestBackup ? $newestBackup->date()->format('Y/m/d H:i:s') : $noBackupsText, $oldestBackupDate => $oldestBackup ? $oldestBackup->date()->format('Y/m/d H:i:s') : $noBackupsText, ])->filter(); } public function backupDestination(): ?BackupDestination { if (isset($this->event->backupDestination)) { return $this->event->backupDestination; } if (isset($this->event->backupDestinationStatus)) { return $this->event->backupDestinationStatus->backupDestination(); } return null; } } laravel-backup/src/Notifications/EventHandler.php 0000644 00000003771 15002154456 0016137 0 ustar 00 <?php namespace Spatie\Backup\Notifications; use Illuminate\Contracts\Config\Repository; use Illuminate\Contracts\Events\Dispatcher; use Illuminate\Notifications\Notification; use Spatie\Backup\Events\BackupHasFailed; use Spatie\Backup\Events\BackupWasSuccessful; use Spatie\Backup\Events\CleanupHasFailed; use Spatie\Backup\Events\CleanupWasSuccessful; use Spatie\Backup\Events\HealthyBackupWasFound; use Spatie\Backup\Events\UnhealthyBackupWasFound; use Spatie\Backup\Exceptions\NotificationCouldNotBeSent; class EventHandler { public function __construct( protected Repository $config ) { } public function subscribe(Dispatcher $events): void { $events->listen($this->allBackupEventClasses(), function ($event) { $notifiable = $this->determineNotifiable(); $notification = $this->determineNotification($event); $notifiable->notify($notification); }); } protected function determineNotifiable() { $notifiableClass = $this->config->get('backup.notifications.notifiable'); return app($notifiableClass); } protected function determineNotification($event): Notification { $lookingForNotificationClass = class_basename($event) . "Notification"; $notificationClass = collect($this->config->get('backup.notifications.notifications')) ->keys() ->first(fn (string $notificationClass) => class_basename($notificationClass) === $lookingForNotificationClass); if (! $notificationClass) { throw NotificationCouldNotBeSent::noNotificationClassForEvent($event); } return new $notificationClass($event); } protected function allBackupEventClasses(): array { return [ BackupHasFailed::class, BackupWasSuccessful::class, CleanupHasFailed::class, CleanupWasSuccessful::class, HealthyBackupWasFound::class, UnhealthyBackupWasFound::class, ]; } } laravel-backup/src/Notifications/Channels/Discord/DiscordMessage.php 0000644 00000005416 15002154456 0021574 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Channels\Discord; use Carbon\Carbon; class DiscordMessage { public const COLOR_SUCCESS = '0b6623'; public const COLOR_WARNING = 'fD6a02'; public const COLOR_ERROR = 'e32929'; protected string $username = 'Laravel Backup'; protected ?string $avatarUrl = null; protected string $title = ''; protected string $description = ''; protected array $fields = []; protected ?string $timestamp = null; protected ?string $footer = null; protected ?string $color = null; protected string $url = ''; public function from(string $username, string $avatarUrl = null): self { $this->username = $username; if (! is_null($avatarUrl)) { $this->avatarUrl = $avatarUrl; } return $this; } public function url(string $url): self { $this->url = $url; return $this; } public function title($title): self { $this->title = $title; return $this; } public function description(string $description): self { $this->description = $description; return $this; } public function timestamp(Carbon $carbon): self { $this->timestamp = $carbon->toIso8601String(); return $this; } public function footer(string $footer): self { $this->footer = $footer; return $this; } public function success(): self { $this->color = static::COLOR_SUCCESS; return $this; } public function warning(): self { $this->color = static::COLOR_WARNING; return $this; } public function error(): self { $this->color = static::COLOR_ERROR; return $this; } public function fields(array $fields, bool $inline = true): self { foreach ($fields as $label => $value) { $this->fields[] = [ 'name' => $label, 'value' => $value, 'inline' => $inline, ]; } return $this; } public function toArray(): array { return [ 'username' => $this->username ?? 'Laravel Backup', 'avatar_url' => $this->avatarUrl, 'embeds' => [ [ 'title' => $this->title, 'url' => $this->url, 'type' => 'rich', 'description' => $this->description, 'fields' => $this->fields, 'color' => hexdec($this->color), 'footer' => [ 'text' => $this->footer ?? '', ], 'timestamp' => $this->timestamp ?? now(), ], ], ]; } } laravel-backup/src/Notifications/Channels/Discord/DiscordChannel.php 0000644 00000000725 15002154456 0021556 0 ustar 00 <?php namespace Spatie\Backup\Notifications\Channels\Discord; use Illuminate\Notifications\Notification; use Illuminate\Support\Facades\Http; class DiscordChannel { public function send($notifiable, Notification $notification): void { $discordMessage = $notification->toDiscord(); // @phpstan-ignore-line $discordWebhook = $notifiable->routeNotificationForDiscord(); Http::post($discordWebhook, $discordMessage->toArray()); } } laravel-backup/src/BackupServiceProvider.php 0000644 00000003603 15002154456 0015202 0 ustar 00 <?php namespace Spatie\Backup; use Illuminate\Notifications\ChannelManager; use Illuminate\Support\Facades\Event; use Illuminate\Support\Facades\Notification; use Spatie\Backup\Commands\BackupCommand; use Spatie\Backup\Commands\CleanupCommand; use Spatie\Backup\Commands\ListCommand; use Spatie\Backup\Commands\MonitorCommand; use Spatie\Backup\Events\BackupZipWasCreated; use Spatie\Backup\Helpers\ConsoleOutput; use Spatie\Backup\Listeners\EncryptBackupArchive; use Spatie\Backup\Notifications\Channels\Discord\DiscordChannel; use Spatie\Backup\Notifications\EventHandler; use Spatie\Backup\Tasks\Cleanup\CleanupStrategy; use Spatie\LaravelPackageTools\Package; use Spatie\LaravelPackageTools\PackageServiceProvider; class BackupServiceProvider extends PackageServiceProvider { public function configurePackage(Package $package): void { $package ->name('laravel-backup') ->hasConfigFile() ->hasTranslations() ->hasCommands([ BackupCommand::class, CleanupCommand::class, ListCommand::class, MonitorCommand::class, ]); } public function packageBooted() { if (EncryptBackupArchive::shouldEncrypt()) { Event::listen(BackupZipWasCreated::class, EncryptBackupArchive::class); } } public function packageRegistered() { $this->app['events']->subscribe(EventHandler::class); $this->app->singleton(ConsoleOutput::class); $this->app->bind(CleanupStrategy::class, config('backup.cleanup.strategy')); $this->registerDiscordChannel(); } protected function registerDiscordChannel() { Notification::resolved(function (ChannelManager $service) { $service->extend('discord', function ($app) { return new DiscordChannel(); }); }); } } laravel-backup/src/Events/BackupWasSuccessful.php 0000644 00000000342 15002154456 0016122 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\Backup\BackupDestination\BackupDestination; class BackupWasSuccessful { public function __construct( public BackupDestination $backupDestination, ) { } } laravel-backup/src/Events/BackupHasFailed.php 0000644 00000000432 15002154456 0015150 0 ustar 00 <?php namespace Spatie\Backup\Events; use Exception; use Spatie\Backup\BackupDestination\BackupDestination; class BackupHasFailed { public function __construct( public Exception $exception, public ?BackupDestination $backupDestination = null, ) { } } laravel-backup/src/Events/BackupManifestWasCreated.php 0000644 00000000307 15002154456 0017042 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\Backup\Tasks\Backup\Manifest; class BackupManifestWasCreated { public function __construct( public Manifest $manifest, ) { } } laravel-backup/src/Events/UnhealthyBackupWasFound.php 0000644 00000000363 15002154456 0016743 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\Backup\Tasks\Monitor\BackupDestinationStatus; class UnhealthyBackupWasFound { public function __construct( public BackupDestinationStatus $backupDestinationStatus ) { } } laravel-backup/src/Events/HealthyBackupWasFound.php 0000644 00000000362 15002154456 0016377 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\Backup\Tasks\Monitor\BackupDestinationStatus; class HealthyBackupWasFound { public function __construct( public BackupDestinationStatus $backupDestinationStatus, ) { } } laravel-backup/src/Events/CleanupHasFailed.php 0000644 00000000433 15002154456 0015333 0 ustar 00 <?php namespace Spatie\Backup\Events; use Exception; use Spatie\Backup\BackupDestination\BackupDestination; class CleanupHasFailed { public function __construct( public Exception $exception, public ?BackupDestination $backupDestination = null, ) { } } laravel-backup/src/Events/BackupZipWasCreated.php 0000644 00000000227 15002154456 0016037 0 ustar 00 <?php namespace Spatie\Backup\Events; class BackupZipWasCreated { public function __construct( public string $pathToZip, ) { } } laravel-backup/src/Events/DumpingDatabase.php 0000644 00000000262 15002154456 0015233 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\DbDumper\DbDumper; class DumpingDatabase { public function __construct( public DbDumper $dbDumper ) { } } laravel-backup/src/Events/CleanupWasSuccessful.php 0000644 00000000343 15002154456 0016305 0 ustar 00 <?php namespace Spatie\Backup\Events; use Spatie\Backup\BackupDestination\BackupDestination; class CleanupWasSuccessful { public function __construct( public BackupDestination $backupDestination, ) { } } laravel-backup/src/BackupDestination/BackupDestinationFactory.php 0000644 00000000554 15002154456 0021311 0 ustar 00 <?php namespace Spatie\Backup\BackupDestination; use Illuminate\Support\Collection; class BackupDestinationFactory { public static function createFromArray(array $config): Collection { return collect($config['destination']['disks']) ->map(fn ($filesystemName) => BackupDestination::create($filesystemName, $config['name'])); } } laravel-backup/src/BackupDestination/BackupDestination.php 0000644 00000010241 15002154456 0017753 0 ustar 00 <?php namespace Spatie\Backup\BackupDestination; use Carbon\Carbon; use Exception; use Illuminate\Contracts\Filesystem\Factory; use Illuminate\Contracts\Filesystem\Filesystem; use Spatie\Backup\Exceptions\InvalidBackupDestination; class BackupDestination { protected ?Filesystem $disk; protected string $diskName; protected string $backupName; public ?Exception $connectionError = null; protected ?BackupCollection $backupCollectionCache = null; public function __construct(Filesystem $disk = null, string $backupName, string $diskName) { $this->disk = $disk; $this->diskName = $diskName; $this->backupName = $backupName; } public function disk(): Filesystem { return $this->disk; } public function diskName(): string { return $this->diskName; } public function filesystemType(): string { if (is_null($this->disk)) { return 'unknown'; } $adapterClass = $this->disk->getAdapter()::class; $filesystemType = last(explode('\\', $adapterClass)); return strtolower($filesystemType); } public static function create(string $diskName, string $backupName): self { try { $disk = app(Factory::class)->disk($diskName); return new static($disk, $backupName, $diskName); } catch (Exception $exception) { $backupDestination = new static(null, $backupName, $diskName); $backupDestination->connectionError = $exception; return $backupDestination; } } public function write(string $file): void { if (! is_null($this->connectionError)) { throw InvalidBackupDestination::connectionError($this->diskName); } if (is_null($this->disk)) { throw InvalidBackupDestination::diskNotSet($this->backupName); } $destination = $this->backupName.'/'.pathinfo($file, PATHINFO_BASENAME); $handle = fopen($file, 'r+'); $this->disk->getDriver()->writeStream( $destination, $handle, $this->getDiskOptions(), ); if (is_resource($handle)) { fclose($handle); } } public function backupName(): string { return $this->backupName; } public function backups(): BackupCollection { if ($this->backupCollectionCache) { return $this->backupCollectionCache; } $files = []; if (! is_null($this->disk)) { // $this->disk->allFiles() may fail when $this->disk is not reachable // in that case we still want to send the notification try { $files = $this->disk->allFiles($this->backupName); } catch (Exception) { } } return $this->backupCollectionCache = BackupCollection::createFromFiles( $this->disk, $files ); } public function connectionError(): Exception { return $this->connectionError; } public function getDiskOptions(): array { return config("filesystems.disks.{$this->diskName()}.backup_options") ?? []; } public function isReachable(): bool { if (is_null($this->disk)) { return false; } try { $this->disk->files($this->backupName); return true; } catch (Exception $exception) { $this->connectionError = $exception; return false; } } public function usedStorage(): float { return $this->backups()->size(); } public function newestBackup(): ?Backup { return $this->backups()->newest(); } public function oldestBackup(): ?Backup { return $this->backups()->oldest(); } public function newestBackupIsOlderThan(Carbon $date): bool { $newestBackup = $this->newestBackup(); if (is_null($newestBackup)) { return true; } return $newestBackup->date()->gt($date); } public function fresh(): self { $this->backupCollectionCache = null; return $this; } } laravel-backup/src/BackupDestination/Backup.php 0000644 00000003665 15002154456 0015565 0 ustar 00 <?php namespace Spatie\Backup\BackupDestination; use Carbon\Carbon; use Illuminate\Contracts\Filesystem\Filesystem; use InvalidArgumentException; use Spatie\Backup\Exceptions\InvalidBackupFile; use Spatie\Backup\Tasks\Backup\BackupJob; class Backup { protected bool $exists = true; protected ?Carbon $date = null; protected ?int $size = null; public function __construct( protected Filesystem $disk, protected string $path, ) { $this->exists = $this->disk->exists($this->path); } public function disk(): Filesystem { return $this->disk; } public function path(): string { return $this->path; } public function exists(): bool { return $this->exists; } public function date(): Carbon { if ($this->date === null) { try { $basename = basename($this->path); $this->date = Carbon::createFromFormat(BackupJob::FILENAME_FORMAT, $basename); } catch (InvalidArgumentException) { $this->date = Carbon::createFromTimestamp($this->disk->lastModified($this->path)); } } return $this->date; } public function sizeInBytes(): float { if ($this->size === null) { if (! $this->exists()) { return 0; } $this->size = $this->disk->size($this->path); } return $this->size; } public function stream() { return throw_unless( $this->disk->readStream($this->path), InvalidBackupFile::readError($this) ); } public function delete(): void { if (! $this->disk->delete($this->path)) { consoleOutput()->error("Failed to delete backup `{$this->path}`."); return; } $this->exists = false; consoleOutput()->info("Deleted backup `{$this->path}`."); } } laravel-backup/src/BackupDestination/BackupCollection.php 0000644 00000002112 15002154456 0017563 0 ustar 00 <?php namespace Spatie\Backup\BackupDestination; use Illuminate\Contracts\Filesystem\Filesystem; use Illuminate\Support\Collection; use Spatie\Backup\Helpers\File; class BackupCollection extends Collection { protected ?float $sizeCache = null; public static function createFromFiles(?FileSystem $disk, array $files): self { return (new static($files)) ->filter(fn (string $path) => (new File())->isZipFile($disk, $path)) ->map(fn (string $path) => new Backup($disk, $path)) ->sortByDesc(fn (Backup $backup) => $backup->date()->timestamp) ->values(); } public function newest(): ?Backup { return $this->first(); } public function oldest(): ?Backup { return $this ->filter(fn (Backup $backup) => $backup->exists()) ->last(); } public function size(): float { if ($this->sizeCache !== null) { return $this->sizeCache; } return $this->sizeCache = $this->sum(fn (Backup $backup) => $backup->sizeInBytes()); } } laravel-backup/src/Commands/BackupCommand.php 0000644 00000004613 15002154456 0015210 0 ustar 00 <?php namespace Spatie\Backup\Commands; use Exception; use Spatie\Backup\Events\BackupHasFailed; use Spatie\Backup\Exceptions\InvalidCommand; use Spatie\Backup\Tasks\Backup\BackupJobFactory; class BackupCommand extends BaseCommand { protected $signature = 'backup:run {--filename=} {--only-db} {--db-name=*} {--only-files} {--only-to-disk=} {--disable-notifications} {--timeout=}'; protected $description = 'Run the backup.'; public function handle() { consoleOutput()->comment('Starting backup...'); $disableNotifications = $this->option('disable-notifications'); if ($this->option('timeout') && is_numeric($this->option('timeout'))) { set_time_limit((int) $this->option('timeout')); } try { $this->guardAgainstInvalidOptions(); $backupJob = BackupJobFactory::createFromArray(config('backup')); if ($this->option('only-db')) { $backupJob->dontBackupFilesystem(); } if ($this->option('db-name')) { $backupJob->onlyDbName($this->option('db-name')); } if ($this->option('only-files')) { $backupJob->dontBackupDatabases(); } if ($this->option('only-to-disk')) { $backupJob->onlyBackupTo($this->option('only-to-disk')); } if ($this->option('filename')) { $backupJob->setFilename($this->option('filename')); } if ($disableNotifications) { $backupJob->disableNotifications(); } if (! $this->getSubscribedSignals()) { $backupJob->disableSignals(); } $backupJob->run(); consoleOutput()->comment('Backup completed!'); } catch (Exception $exception) { consoleOutput()->error("Backup failed because: {$exception->getMessage()}."); report($exception); if (! $disableNotifications) { event(new BackupHasFailed($exception)); } return 1; } } protected function guardAgainstInvalidOptions() { if (! $this->option('only-db')) { return; } if (! $this->option('only-files')) { return; } throw InvalidCommand::create('Cannot use `only-db` and `only-files` together'); } } laravel-backup/src/Commands/BaseCommand.php 0000644 00000001777 15002154456 0014665 0 ustar 00 <?php namespace Spatie\Backup\Commands; use Spatie\Backup\Helpers\ConsoleOutput; use Spatie\SignalAwareCommand\SignalAwareCommand; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; use Symfony\Component\Console\SignalRegistry\SignalRegistry; abstract class BaseCommand extends SignalAwareCommand { protected array $handlesSignals = []; public function __construct() { if ($this->runningInConsole() && SignalRegistry::isSupported()) { $this->handlesSignals[] = SIGINT; } parent::__construct(); } public function run(InputInterface $input, OutputInterface $output): int { app(ConsoleOutput::class)->setCommand($this); return parent::run($input, $output); } protected function runningInConsole(): bool { return in_array(php_sapi_name(), ['cli', 'phpdbg']); } public function getSubscribedSignals(): array { return $this->handlesSignals; } } laravel-backup/src/Commands/MonitorCommand.php 0000644 00000003042 15002154456 0015425 0 ustar 00 <?php namespace Spatie\Backup\Commands; use Spatie\Backup\Events\HealthyBackupWasFound; use Spatie\Backup\Events\UnhealthyBackupWasFound; use Spatie\Backup\Tasks\Monitor\BackupDestinationStatusFactory; class MonitorCommand extends BaseCommand { /** @var string */ protected $signature = 'backup:monitor'; /** @var string */ protected $description = 'Monitor the health of all backups.'; public function handle() { if (config()->has('backup.monitorBackups')) { $this->warn("Warning! Your config file still uses the old monitorBackups key. Update it to monitor_backups."); } $hasError = false; $statuses = BackupDestinationStatusFactory::createForMonitorConfig(config('backup.monitor_backups')); foreach ($statuses as $backupDestinationStatus) { $backupName = $backupDestinationStatus->backupDestination()->backupName(); $diskName = $backupDestinationStatus->backupDestination()->diskName(); if ($backupDestinationStatus->isHealthy()) { $this->info("The {$backupName} backups on the {$diskName} disk are considered healthy."); event(new HealthyBackupWasFound($backupDestinationStatus)); } else { $hasError = true; $this->error("The {$backupName} backups on the {$diskName} disk are considered unhealthy!"); event(new UnhealthyBackupWasFound($backupDestinationStatus)); } } if ($hasError) { return 1; } } } laravel-backup/src/Commands/CleanupCommand.php 0000644 00000002600 15002154456 0015364 0 ustar 00 <?php namespace Spatie\Backup\Commands; use Exception; use Spatie\Backup\BackupDestination\BackupDestinationFactory; use Spatie\Backup\Events\CleanupHasFailed; use Spatie\Backup\Tasks\Cleanup\CleanupJob; use Spatie\Backup\Tasks\Cleanup\CleanupStrategy; class CleanupCommand extends BaseCommand { /** @var string */ protected $signature = 'backup:clean {--disable-notifications}'; /** @var string */ protected $description = 'Remove all backups older than specified number of days in config.'; protected CleanupStrategy $strategy; public function __construct(CleanupStrategy $strategy) { parent::__construct(); $this->strategy = $strategy; } public function handle() { consoleOutput()->comment('Starting cleanup...'); $disableNotifications = $this->option('disable-notifications'); try { $config = config('backup'); $backupDestinations = BackupDestinationFactory::createFromArray($config['backup']); $cleanupJob = new CleanupJob($backupDestinations, $this->strategy, $disableNotifications); $cleanupJob->run(); consoleOutput()->comment('Cleanup completed!'); } catch (Exception $exception) { if (! $disableNotifications) { event(new CleanupHasFailed($exception)); } return 1; } } } laravel-backup/src/Commands/ListCommand.php 0000644 00000007352 15002154456 0014721 0 ustar 00 <?php namespace Spatie\Backup\Commands; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\Backup; use Spatie\Backup\Helpers\Format; use Spatie\Backup\Helpers\RightAlignedTableStyle; use Spatie\Backup\Tasks\Monitor\BackupDestinationStatus; use Spatie\Backup\Tasks\Monitor\BackupDestinationStatusFactory; class ListCommand extends BaseCommand { /** @var string */ protected $signature = 'backup:list'; /** @var string */ protected $description = 'Display a list of all backups.'; public function handle() { if (config()->has('backup.monitorBackups')) { $this->warn("Warning! Your config file still uses the old monitorBackups key. Update it to monitor_backups."); } $statuses = BackupDestinationStatusFactory::createForMonitorConfig(config('backup.monitor_backups')); $this->displayOverview($statuses)->displayFailures($statuses); } protected function displayOverview(Collection $backupDestinationStatuses) { $headers = ['Name', 'Disk', 'Reachable', 'Healthy', '# of backups', 'Newest backup', 'Used storage']; $rows = $backupDestinationStatuses->map(function (BackupDestinationStatus $backupDestinationStatus) { return $this->convertToRow($backupDestinationStatus); }); $this->table($headers, $rows, 'default', [ 4 => new RightAlignedTableStyle(), 6 => new RightAlignedTableStyle(), ]); return $this; } public function convertToRow(BackupDestinationStatus $backupDestinationStatus): array { $destination = $backupDestinationStatus->backupDestination(); $row = [ $destination->backupName(), 'disk' => $destination->diskName(), Format::emoji($destination->isReachable()), Format::emoji($backupDestinationStatus->isHealthy()), 'amount' => $destination->backups()->count(), 'newest' => $this->getFormattedBackupDate($destination->newestBackup()), 'usedStorage' => Format::humanReadableSize($destination->usedStorage()), ]; if (! $destination->isReachable()) { foreach (['amount', 'newest', 'usedStorage'] as $propertyName) { $row[$propertyName] = '/'; } } if ($backupDestinationStatus->getHealthCheckFailure() !== null) { $row['disk'] = '<error>'.$row['disk'].'</error>'; } return $row; } protected function displayFailures(Collection $backupDestinationStatuses) { $failed = $backupDestinationStatuses ->filter(function (BackupDestinationStatus $backupDestinationStatus) { return $backupDestinationStatus->getHealthCheckFailure() !== null; }) ->map(function (BackupDestinationStatus $backupDestinationStatus) { return [ $backupDestinationStatus->backupDestination()->backupName(), $backupDestinationStatus->backupDestination()->diskName(), $backupDestinationStatus->getHealthCheckFailure()->healthCheck()->name(), $backupDestinationStatus->getHealthCheckFailure()->exception()->getMessage(), ]; }); if ($failed->isNotEmpty()) { $this->warn(''); $this->warn('Unhealthy backup destinations'); $this->warn('-----------------------------'); $this->table(['Name', 'Disk', 'Failed check', 'Description'], $failed->all()); } return $this; } protected function getFormattedBackupDate(Backup $backup = null) { return is_null($backup) ? 'No backups present' : Format::ageInDays($backup->date()); } } laravel-backup/src/Tasks/Monitor/BackupDestinationStatus.php 0000644 00000002765 15002154456 0020300 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor; use Exception; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Tasks\Monitor\HealthChecks\IsReachable; class BackupDestinationStatus { protected ?HealthCheckFailure $healthCheckFailure = null; public function __construct( protected BackupDestination $backupDestination, protected array $healthChecks = [] ) { } public function backupDestination(): BackupDestination { return $this->backupDestination; } public function check(HealthCheck $check): bool | HealthCheckFailure { try { $check->checkHealth($this->backupDestination()); } catch (Exception $exception) { return new HealthCheckFailure($check, $exception); } return true; } public function getHealthChecks(): Collection { return collect($this->healthChecks)->prepend(new IsReachable()); } public function getHealthCheckFailure(): ?HealthCheckFailure { return $this->healthCheckFailure; } public function isHealthy(): bool { $healthChecks = $this->getHealthChecks(); foreach ($healthChecks as $healthCheck) { $checkResult = $this->check($healthCheck); if ($checkResult instanceof HealthCheckFailure) { $this->healthCheckFailure = $checkResult; return false; } } return true; } } laravel-backup/src/Tasks/Monitor/HealthCheckFailure.php 0000644 00000001077 15002154456 0017133 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor; use Exception; use Spatie\Backup\Exceptions\InvalidHealthCheck; class HealthCheckFailure { public function __construct( protected HealthCheck $healthCheck, protected Exception $exception ) { } public function healthCheck(): HealthCheck { return $this->healthCheck; } public function exception(): Exception { return $this->exception; } public function wasUnexpected(): bool { return ! $this->exception instanceof InvalidHealthCheck; } } laravel-backup/src/Tasks/Monitor/HealthCheck.php 0000644 00000001464 15002154456 0015623 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor; use Illuminate\Support\Str; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Exceptions\InvalidHealthCheck; abstract class HealthCheck { abstract public function checkHealth(BackupDestination $backupDestination); public function name(): string { return Str::title(class_basename($this)); } protected function fail(string $message): void { throw InvalidHealthCheck::because($message); } protected function failIf(bool $condition, string $message): void { if ($condition) { $this->fail($message); } } protected function failUnless(bool $condition, string $message): void { if (! $condition) { $this->fail($message); } } } laravel-backup/src/Tasks/Monitor/BackupDestinationStatusFactory.php 0000644 00000003403 15002154456 0021616 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestination; class BackupDestinationStatusFactory { public static function createForMonitorConfig(array $monitorConfiguration): Collection { return collect($monitorConfiguration) ->flatMap(fn (array $monitorProperties) => self::createForSingleMonitor($monitorProperties)) ->sortBy(fn (BackupDestinationStatus $backupDestinationStatus) => $backupDestinationStatus->backupDestination()->backupName() . '-' . $backupDestinationStatus->backupDestination()->diskName()); } public static function createForSingleMonitor(array $monitorConfig): Collection { return collect($monitorConfig['disks']) ->map(function ($diskName) use ($monitorConfig) { $backupDestination = BackupDestination::create($diskName, $monitorConfig['name']); return new BackupDestinationStatus($backupDestination, static::buildHealthChecks($monitorConfig)); }); } protected static function buildHealthChecks($monitorConfig): array { return collect(Arr::get($monitorConfig, 'health_checks')) ->map(function ($options, $class) { if (is_int($class)) { $class = $options; $options = []; } return static::buildHealthCheck($class, $options); })->toArray(); } protected static function buildHealthCheck(string $class, string | array $options): HealthCheck { if (! is_array($options)) { return new $class($options); } return app()->makeWith($class, $options); } } laravel-backup/src/Tasks/Monitor/HealthChecks/MaximumStorageInMegabytes.php 0000644 00000002340 15002154456 0023072 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor\HealthChecks; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Helpers\Format; use Spatie\Backup\Tasks\Monitor\HealthCheck; class MaximumStorageInMegabytes extends HealthCheck { public function __construct( protected int $maximumSizeInMegaBytes = 5000 ) { } public function checkHealth(BackupDestination $backupDestination): void { $usageInBytes = $backupDestination->usedStorage(); $this->failIf( $this->exceedsAllowance($usageInBytes), trans('backup::notifications.unhealthy_backup_found_full', [ 'disk_usage' => $this->humanReadableSize($usageInBytes), 'disk_limit' => $this->humanReadableSize($this->bytes($this->maximumSizeInMegaBytes)), ]) ); } protected function exceedsAllowance(float $usageInBytes): bool { return $usageInBytes > $this->bytes($this->maximumSizeInMegaBytes); } protected function bytes(int $megaBytes): int { return $megaBytes * 1024 * 1024; } protected function humanReadableSize(float $sizeInBytes): string { return Format::humanReadableSize($sizeInBytes); } } laravel-backup/src/Tasks/Monitor/HealthChecks/MaximumAgeInDays.php 0000644 00000002247 15002154456 0021150 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor\HealthChecks; use Spatie\Backup\BackupDestination\Backup; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Tasks\Monitor\HealthCheck; class MaximumAgeInDays extends HealthCheck { public function __construct( protected int $days = 1 ) { } public function checkHealth(BackupDestination $backupDestination): void { $this->failIf( $this->hasNoBackups($backupDestination), trans('backup::notifications.unhealthy_backup_found_empty') ); $newestBackup = $backupDestination->backups()->newest(); $this->failIf( $this->isTooOld($newestBackup), trans('backup::notifications.unhealthy_backup_found_old', ['date' => $newestBackup->date()->format('Y/m/d h:i:s')]) ); } protected function hasNoBackups(BackupDestination $backupDestination): bool { return $backupDestination->backups()->isEmpty(); } protected function isTooOld(Backup $backup): bool { if ($backup->date()->gt(now()->subDays($this->days))) { return false; } return true; } } laravel-backup/src/Tasks/Monitor/HealthChecks/IsReachable.php 0000644 00000001031 15002154456 0020136 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Monitor\HealthChecks; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Tasks\Monitor\HealthCheck; class IsReachable extends HealthCheck { public function checkHealth(BackupDestination $backupDestination): void { $this->failUnless( $backupDestination->isReachable(), trans('backup::notifications.unhealthy_backup_found_not_reachable', [ 'error' => $backupDestination->connectionError, ]) ); } } laravel-backup/src/Tasks/Cleanup/CleanupStrategy.php 0000644 00000001342 15002154456 0016525 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Cleanup; use Illuminate\Contracts\Config\Repository; use Spatie\Backup\BackupDestination\BackupCollection; use Spatie\Backup\BackupDestination\BackupDestination; abstract class CleanupStrategy { protected BackupDestination $backupDestination; public function __construct( protected Repository $config, ) { } abstract public function deleteOldBackups(BackupCollection $backups); public function setBackupDestination(BackupDestination $backupDestination): self { $this->backupDestination = $backupDestination; return $this; } public function backupDestination(): BackupDestination { return $this->backupDestination; } } laravel-backup/src/Tasks/Cleanup/Strategies/DefaultStrategy.php 0000644 00000007732 15002154456 0020645 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Cleanup\Strategies; use Carbon\Carbon; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\Backup; use Spatie\Backup\BackupDestination\BackupCollection; use Spatie\Backup\Tasks\Cleanup\CleanupStrategy; use Spatie\Backup\Tasks\Cleanup\Period; class DefaultStrategy extends CleanupStrategy { protected ?Backup $newestBackup = null; public function deleteOldBackups(BackupCollection $backups) { // Don't ever delete the newest backup. $this->newestBackup = $backups->shift(); $dateRanges = $this->calculateDateRanges(); $backupsPerPeriod = $dateRanges->map(function (Period $period) use ($backups) { return $backups ->filter(fn (Backup $backup) => $backup->date()->between($period->startDate(), $period->endDate())); }); $backupsPerPeriod['daily'] = $this->groupByDateFormat($backupsPerPeriod['daily'], 'Ymd'); $backupsPerPeriod['weekly'] = $this->groupByDateFormat($backupsPerPeriod['weekly'], 'YW'); $backupsPerPeriod['monthly'] = $this->groupByDateFormat($backupsPerPeriod['monthly'], 'Ym'); $backupsPerPeriod['yearly'] = $this->groupByDateFormat($backupsPerPeriod['yearly'], 'Y'); $this->removeBackupsForAllPeriodsExceptOne($backupsPerPeriod); $this->removeBackupsOlderThan($dateRanges['yearly']->endDate(), $backups); $this->removeOldBackupsUntilUsingLessThanMaximumStorage($backups); } protected function calculateDateRanges(): Collection { $config = $this->config->get('backup.cleanup.default_strategy'); $daily = new Period( Carbon::now()->subDays($config['keep_all_backups_for_days']), Carbon::now() ->subDays($config['keep_all_backups_for_days']) ->subDays($config['keep_daily_backups_for_days']) ); $weekly = new Period( $daily->endDate(), $daily->endDate() ->subWeeks($config['keep_weekly_backups_for_weeks']) ); $monthly = new Period( $weekly->endDate(), $weekly->endDate() ->subMonths($config['keep_monthly_backups_for_months']) ); $yearly = new Period( $monthly->endDate(), $monthly->endDate() ->subYears($config['keep_yearly_backups_for_years']) ); return collect(compact('daily', 'weekly', 'monthly', 'yearly')); } protected function groupByDateFormat(Collection $backups, string $dateFormat): Collection { return $backups->groupBy(fn (Backup $backup) => $backup->date()->format($dateFormat)); } protected function removeBackupsForAllPeriodsExceptOne(Collection $backupsPerPeriod) { $backupsPerPeriod->each(function (Collection $groupedBackupsByDateProperty, string $periodName) { $groupedBackupsByDateProperty->each(function (Collection $group) { $group->shift(); $group->each(fn (Backup $backup) => $backup->delete()); }); }); } protected function removeBackupsOlderThan(Carbon $endDate, BackupCollection $backups) { $backups ->filter(fn (Backup $backup) => $backup->exists() && $backup->date()->lt($endDate)) ->each(fn (Backup $backup) => $backup->delete()); } protected function removeOldBackupsUntilUsingLessThanMaximumStorage(BackupCollection $backups) { if (! $oldest = $backups->oldest()) { return; } $maximumSize = $this->config->get('backup.cleanup.default_strategy.delete_oldest_backups_when_using_more_megabytes_than') * 1024 * 1024; if (($backups->size() + $this->newestBackup->sizeInBytes()) <= $maximumSize) { return; } $oldest->delete(); $backups = $backups->filter(fn (Backup $backup) => $backup->exists()); $this->removeOldBackupsUntilUsingLessThanMaximumStorage($backups); } } laravel-backup/src/Tasks/Cleanup/Period.php 0000644 00000000602 15002154456 0014633 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Cleanup; use Carbon\Carbon; class Period { public function __construct( protected Carbon $startDate, protected Carbon $endDate ) { } public function startDate(): Carbon { return $this->startDate->copy(); } public function endDate(): Carbon { return $this->endDate->copy(); } } laravel-backup/src/Tasks/Cleanup/CleanupJob.php 0000644 00000004157 15002154456 0015444 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Cleanup; use Exception; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Events\CleanupHasFailed; use Spatie\Backup\Events\CleanupWasSuccessful; use Spatie\Backup\Helpers\Format; class CleanupJob { protected Collection $backupDestinations; protected CleanupStrategy $strategy; protected bool $sendNotifications = true; public function __construct( Collection $backupDestinations, CleanupStrategy $strategy, bool $disableNotifications = false, ) { $this->backupDestinations = $backupDestinations; $this->strategy = $strategy; $this->sendNotifications = ! $disableNotifications; } public function run(): void { $this->backupDestinations->each(function (BackupDestination $backupDestination) { try { if (! $backupDestination->isReachable()) { throw new Exception("Could not connect to disk {$backupDestination->diskName()} because: {$backupDestination->connectionError()}"); } consoleOutput()->info("Cleaning backups of {$backupDestination->backupName()} on disk {$backupDestination->diskName()}..."); $this->strategy ->setBackupDestination($backupDestination) ->deleteOldBackups($backupDestination->backups()); $this->sendNotification(new CleanupWasSuccessful($backupDestination)); $usedStorage = Format::humanReadableSize($backupDestination->fresh()->usedStorage()); consoleOutput()->info("Used storage after cleanup: {$usedStorage}."); } catch (Exception $exception) { consoleOutput()->error("Cleanup failed because: {$exception->getMessage()}."); $this->sendNotification(new CleanupHasFailed($exception)); throw $exception; } }); } protected function sendNotification($notification): void { if ($this->sendNotifications) { event($notification); } } } laravel-backup/src/Tasks/Backup/Manifest.php 0000644 00000002525 15002154456 0015003 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Countable; use Generator; use SplFileObject; class Manifest implements Countable { protected string $manifestPath; public static function create(string $manifestPath): self { return new static($manifestPath); } public function __construct(string $manifestPath) { $this->manifestPath = $manifestPath; touch($manifestPath); } public function path(): string { return $this->manifestPath; } public function addFiles(array | string | Generator $filePaths): self { if (is_string($filePaths)) { $filePaths = [$filePaths]; } foreach ($filePaths as $filePath) { if (! empty($filePath)) { file_put_contents($this->manifestPath, $filePath.PHP_EOL, FILE_APPEND); } } return $this; } public function files(): Generator | array { $file = new SplFileObject($this->path()); while (! $file->eof()) { $filePath = $file->fgets(); if (! empty($filePath)) { yield trim($filePath); } } } public function count(): int { $file = new SplFileObject($this->manifestPath, 'r'); $file->seek(PHP_INT_MAX); return $file->key(); } } laravel-backup/src/Tasks/Backup/DbDumperFactory.php 0000644 00000010212 15002154456 0016257 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Exception; use Illuminate\Database\ConfigurationUrlParser; use Illuminate\Support\Arr; use Illuminate\Support\Str; use Spatie\Backup\Exceptions\CannotCreateDbDumper; use Spatie\DbDumper\Databases\MongoDb; use Spatie\DbDumper\Databases\MySql; use Spatie\DbDumper\Databases\PostgreSql; use Spatie\DbDumper\Databases\Sqlite; use Spatie\DbDumper\DbDumper; class DbDumperFactory { protected static array $custom = []; public static function createFromConnection(string $dbConnectionName): DbDumper { $parser = new ConfigurationUrlParser(); if (config("database.connections.{$dbConnectionName}") === null) { throw CannotCreateDbDumper::unsupportedDriver($dbConnectionName); } try { $dbConfig = $parser->parseConfiguration(config("database.connections.{$dbConnectionName}")); } catch (Exception) { throw CannotCreateDbDumper::unsupportedDriver($dbConnectionName); } if (isset($dbConfig['read'])) { $dbConfig = Arr::except( array_merge($dbConfig, $dbConfig['read']), ['read', 'write'] ); } $dbDumper = static::forDriver($dbConfig['driver'] ?? '') ->setHost(Arr::first(Arr::wrap($dbConfig['host'] ?? ''))) ->setDbName($dbConfig['connect_via_database'] ?? $dbConfig['database']) ->setUserName($dbConfig['username'] ?? '') ->setPassword($dbConfig['password'] ?? ''); if ($dbDumper instanceof MySql) { $dbDumper ->setDefaultCharacterSet($dbConfig['charset'] ?? '') ->setGtidPurged($dbConfig['dump']['mysql_gtid_purged'] ?? 'AUTO'); } if ($dbDumper instanceof MongoDb) { $dbDumper->setAuthenticationDatabase($dbConfig['dump']['mongodb_user_auth'] ?? ''); } if (isset($dbConfig['port'])) { $dbDumper = $dbDumper->setPort($dbConfig['port']); } if (isset($dbConfig['dump'])) { $dbDumper = static::processExtraDumpParameters($dbConfig['dump'], $dbDumper); } if (isset($dbConfig['unix_socket'])) { $dbDumper = $dbDumper->setSocket($dbConfig['unix_socket']); } return $dbDumper; } public static function extend(string $driver, callable $callback) { static::$custom[$driver] = $callback; } protected static function forDriver($dbDriver): DbDumper { $driver = strtolower($dbDriver); if (isset(static::$custom[$driver])) { return (static::$custom[$driver])(); } return match ($driver) { 'mysql', 'mariadb' => new MySql(), 'pgsql' => new PostgreSql(), 'sqlite' => new Sqlite(), 'mongodb' => new MongoDb(), default => throw CannotCreateDbDumper::unsupportedDriver($driver), }; } protected static function processExtraDumpParameters(array $dumpConfiguration, DbDumper $dbDumper): DbDumper { collect($dumpConfiguration)->each(function ($configValue, $configName) use ($dbDumper) { $methodName = lcfirst(Str::studly(is_numeric($configName) ? $configValue : $configName)); $methodValue = is_numeric($configName) ? null : $configValue; $methodName = static::determineValidMethodName($dbDumper, $methodName); if (method_exists($dbDumper, $methodName)) { static::callMethodOnDumper($dbDumper, $methodName, $methodValue); } }); return $dbDumper; } protected static function callMethodOnDumper(DbDumper $dbDumper, string $methodName, $methodValue): DbDumper { if (! $methodValue) { $dbDumper->$methodName(); return $dbDumper; } $dbDumper->$methodName($methodValue); return $dbDumper; } protected static function determineValidMethodName(DbDumper $dbDumper, string $methodName): string { return collect([$methodName, 'set'.ucfirst($methodName)]) ->first(fn (string $methodName) => method_exists($dbDumper, $methodName), ''); } } laravel-backup/src/Tasks/Backup/Zip.php 0000644 00000005534 15002154456 0014002 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Illuminate\Support\Str; use Spatie\Backup\Helpers\Format; use ZipArchive; class Zip { protected ZipArchive $zipFile; protected int $fileCount = 0; protected string $pathToZip; public static function createForManifest(Manifest $manifest, string $pathToZip): self { $relativePath = config('backup.backup.source.files.relative_path') ? rtrim(config('backup.backup.source.files.relative_path'), DIRECTORY_SEPARATOR) . DIRECTORY_SEPARATOR : false; $zip = new static($pathToZip); $zip->open(); foreach ($manifest->files() as $file) { $zip->add($file, self::determineNameOfFileInZip($file, $pathToZip, $relativePath)); } $zip->close(); return $zip; } protected static function determineNameOfFileInZip(string $pathToFile, string $pathToZip, string $relativePath) { $fileDirectory = pathinfo($pathToFile, PATHINFO_DIRNAME) . DIRECTORY_SEPARATOR; $zipDirectory = pathinfo($pathToZip, PATHINFO_DIRNAME) . DIRECTORY_SEPARATOR; if (Str::startsWith($fileDirectory, $zipDirectory)) { return str_replace($zipDirectory, '', $pathToFile); } if ($relativePath && $relativePath != DIRECTORY_SEPARATOR && Str::startsWith($fileDirectory, $relativePath)) { return str_replace($relativePath, '', $pathToFile); } return $pathToFile; } public function __construct(string $pathToZip) { $this->zipFile = new ZipArchive(); $this->pathToZip = $pathToZip; $this->open(); } public function path(): string { return $this->pathToZip; } public function size(): float { if ($this->fileCount === 0) { return 0; } return filesize($this->pathToZip); } public function humanReadableSize(): string { return Format::humanReadableSize($this->size()); } public function open(): void { $this->zipFile->open($this->pathToZip, ZipArchive::CREATE); } public function close(): void { $this->zipFile->close(); } public function add(string | iterable $files, string $nameInZip = null): self { if (is_array($files)) { $nameInZip = null; } if (is_string($files)) { $files = [$files]; } foreach ($files as $file) { if (is_dir($file)) { $this->zipFile->addEmptyDir(ltrim($nameInZip ?: $file, DIRECTORY_SEPARATOR)); } if (is_file($file)) { $this->zipFile->addFile($file, ltrim($nameInZip, DIRECTORY_SEPARATOR)).PHP_EOL; } $this->fileCount++; } return $this; } public function count(): int { return $this->fileCount; } } laravel-backup/src/Tasks/Backup/BackupJobFactory.php 0000644 00000002445 15002154456 0016426 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestinationFactory; class BackupJobFactory { public static function createFromArray(array $config): BackupJob { return (new BackupJob()) ->setFileSelection(static::createFileSelection($config['backup']['source']['files'])) ->setDbDumpers(static::createDbDumpers($config['backup']['source']['databases'])) ->setBackupDestinations(BackupDestinationFactory::createFromArray($config['backup'])); } protected static function createFileSelection(array $sourceFiles): FileSelection { return FileSelection::create($sourceFiles['include']) ->excludeFilesFrom($sourceFiles['exclude']) ->shouldFollowLinks(isset($sourceFiles['follow_links']) && $sourceFiles['follow_links']) ->shouldIgnoreUnreadableDirs(Arr::get($sourceFiles, 'ignore_unreadable_directories', false)); } protected static function createDbDumpers(array $dbConnectionNames): Collection { return collect($dbConnectionNames)->mapWithKeys( fn (string $dbConnectionName) => [$dbConnectionName => DbDumperFactory::createFromConnection($dbConnectionName)] ); } } laravel-backup/src/Tasks/Backup/FileSelection.php 0000644 00000007516 15002154456 0015767 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Generator; use Illuminate\Support\Collection; use Illuminate\Support\Str; use Symfony\Component\Finder\Finder; class FileSelection { protected Collection $includeFilesAndDirectories; protected Collection $excludeFilesAndDirectories; protected bool $shouldFollowLinks = false; protected bool $shouldIgnoreUnreadableDirs = false; public static function create(array | string $includeFilesAndDirectories = []): self { return new static($includeFilesAndDirectories); } public function __construct(array | string $includeFilesAndDirectories = []) { $this->includeFilesAndDirectories = collect($includeFilesAndDirectories); $this->excludeFilesAndDirectories = collect(); } public function excludeFilesFrom(array | string $excludeFilesAndDirectories): self { $this->excludeFilesAndDirectories = $this->excludeFilesAndDirectories->merge($this->sanitize($excludeFilesAndDirectories)); return $this; } public function shouldFollowLinks(bool $shouldFollowLinks): self { $this->shouldFollowLinks = $shouldFollowLinks; return $this; } public function shouldIgnoreUnreadableDirs(bool $ignoreUnreadableDirs): self { $this->shouldIgnoreUnreadableDirs = $ignoreUnreadableDirs; return $this; } public function selectedFiles(): Generator | array { if ($this->includeFilesAndDirectories->isEmpty()) { return []; } $finder = (new Finder()) ->ignoreDotFiles(false) ->ignoreVCS(false); if ($this->shouldFollowLinks) { $finder->followLinks(); } if ($this->shouldIgnoreUnreadableDirs) { $finder->ignoreUnreadableDirs(); } foreach ($this->includedFiles() as $includedFile) { yield $includedFile; } if (! count($this->includedDirectories())) { return []; } $finder->in($this->includedDirectories()); foreach ($finder->getIterator() as $file) { if ($this->shouldExclude($file)) { continue; } yield $file->getPathname(); } } protected function includedFiles(): array { return $this ->includeFilesAndDirectories ->filter(fn ($path) => is_file($path))->toArray(); } protected function includedDirectories(): array { return $this ->includeFilesAndDirectories ->reject(fn ($path) => is_file($path))->toArray(); } protected function shouldExclude(string $path): bool { $path = realpath($path); if (is_dir($path)) { $path .= DIRECTORY_SEPARATOR ; } foreach ($this->excludeFilesAndDirectories as $excludedPath) { if (Str::startsWith($path, $excludedPath.(is_dir($excludedPath) ? DIRECTORY_SEPARATOR : ''))) { if ($path != $excludedPath && is_file($excludedPath)) { continue; } return true; } } return false; } protected function sanitize(string | array $paths): Collection { return collect($paths) ->reject(fn ($path) => $path === '') ->flatMap(fn ($path) => $this->getMatchingPaths($path)) ->map(fn ($path) => realpath($path)) ->reject(fn ($path) => $path === false); } protected function getMatchingPaths(string $path): array { if ($this->canUseGlobBrace($path)) { return glob(str_replace('*', '{.[!.],}*', $path), GLOB_BRACE); } return glob($path); } protected function canUseGlobBrace(string $path): bool { return strpos($path, '*') !== false && defined('GLOB_BRACE'); } } laravel-backup/src/Tasks/Backup/BackupJob.php 0000644 00000023660 15002154456 0015100 0 ustar 00 <?php namespace Spatie\Backup\Tasks\Backup; use Carbon\Carbon; use Exception; use Generator; use Illuminate\Console\Command; use Illuminate\Support\Collection; use Spatie\Backup\BackupDestination\BackupDestination; use Spatie\Backup\Events\BackupHasFailed; use Spatie\Backup\Events\BackupManifestWasCreated; use Spatie\Backup\Events\BackupWasSuccessful; use Spatie\Backup\Events\BackupZipWasCreated; use Spatie\Backup\Events\DumpingDatabase; use Spatie\Backup\Exceptions\InvalidBackupJob; use Spatie\DbDumper\Compressors\GzipCompressor; use Spatie\DbDumper\Databases\MongoDb; use Spatie\DbDumper\Databases\Sqlite; use Spatie\DbDumper\DbDumper; use Spatie\SignalAwareCommand\Facades\Signal; use Spatie\TemporaryDirectory\TemporaryDirectory; class BackupJob { public const FILENAME_FORMAT = 'Y-m-d-H-i-s.\z\i\p'; protected FileSelection $fileSelection; protected Collection $dbDumpers; protected Collection $backupDestinations; protected string $filename; protected TemporaryDirectory $temporaryDirectory; protected bool $sendNotifications = true; protected bool $signals = true; public function __construct() { $this ->dontBackupFilesystem() ->dontBackupDatabases() ->setDefaultFilename(); $this->backupDestinations = new Collection(); } public function dontBackupFilesystem(): self { $this->fileSelection = FileSelection::create(); return $this; } public function onlyDbName(array $allowedDbNames): self { $this->dbDumpers = $this->dbDumpers->filter( fn (DbDumper $dbDumper, string $connectionName) => in_array($connectionName, $allowedDbNames) ); return $this; } public function dontBackupDatabases(): self { $this->dbDumpers = new Collection(); return $this; } public function disableNotifications(): self { $this->sendNotifications = false; return $this; } public function disableSignals(): self { $this->signals = false; return $this; } public function setDefaultFilename(): self { $this->filename = Carbon::now()->format(static::FILENAME_FORMAT); return $this; } public function setFileSelection(FileSelection $fileSelection): self { $this->fileSelection = $fileSelection; return $this; } public function setDbDumpers(Collection $dbDumpers): self { $this->dbDumpers = $dbDumpers; return $this; } public function setFilename(string $filename): self { $this->filename = $filename; return $this; } public function onlyBackupTo(string $diskName): self { $this->backupDestinations = $this->backupDestinations->filter( fn (BackupDestination $backupDestination) => $backupDestination->diskName() === $diskName ); if (! count($this->backupDestinations)) { throw InvalidBackupJob::destinationDoesNotExist($diskName); } return $this; } public function setBackupDestinations(Collection $backupDestinations): self { $this->backupDestinations = $backupDestinations; return $this; } public function run(): void { $temporaryDirectoryPath = config('backup.backup.temporary_directory') ?? storage_path('app/backup-temp'); $this->temporaryDirectory = (new TemporaryDirectory($temporaryDirectoryPath)) ->name('temp') ->force() ->create() ->empty(); if ($this->signals) { Signal::handle(SIGINT, function (Command $command) { $command->info('Cleaning up temporary directory...'); $this->temporaryDirectory->delete(); }); } try { if (! count($this->backupDestinations)) { throw InvalidBackupJob::noDestinationsSpecified(); } $manifest = $this->createBackupManifest(); if (! $manifest->count()) { throw InvalidBackupJob::noFilesToBeBackedUp(); } $zipFile = $this->createZipContainingEveryFileInManifest($manifest); $this->copyToBackupDestinations($zipFile); } catch (Exception $exception) { consoleOutput()->error("Backup failed because {$exception->getMessage()}." . PHP_EOL . $exception->getTraceAsString()); $this->sendNotification(new BackupHasFailed($exception)); $this->temporaryDirectory->delete(); throw $exception; } $this->temporaryDirectory->delete(); if ($this->signals) { Signal::clearHandlers(SIGINT); } } protected function createBackupManifest(): Manifest { $databaseDumps = $this->dumpDatabases(); consoleOutput()->info('Determining files to backup...'); $manifest = Manifest::create($this->temporaryDirectory->path('manifest.txt')) ->addFiles($databaseDumps) ->addFiles($this->filesToBeBackedUp()); $this->sendNotification(new BackupManifestWasCreated($manifest)); return $manifest; } public function filesToBeBackedUp(): Generator { $this->fileSelection->excludeFilesFrom($this->directoriesUsedByBackupJob()); return $this->fileSelection->selectedFiles(); } protected function directoriesUsedByBackupJob(): array { return $this->backupDestinations ->filter(fn (BackupDestination $backupDestination) => $backupDestination->filesystemType() === 'localfilesystemadapter') ->map( fn (BackupDestination $backupDestination) => $backupDestination->disk()->path('') . $backupDestination->backupName() ) ->each(fn (string $backupDestinationDirectory) => $this->fileSelection->excludeFilesFrom($backupDestinationDirectory)) ->push($this->temporaryDirectory->path()) ->toArray(); } protected function createZipContainingEveryFileInManifest(Manifest $manifest): string { consoleOutput()->info("Zipping {$manifest->count()} files and directories..."); $pathToZip = $this->temporaryDirectory->path(config('backup.backup.destination.filename_prefix') . $this->filename); $zip = Zip::createForManifest($manifest, $pathToZip); consoleOutput()->info("Created zip containing {$zip->count()} files and directories. Size is {$zip->humanReadableSize()}"); if ($this->sendNotifications) { $this->sendNotification(new BackupZipWasCreated($pathToZip)); } else { app()->call('\Spatie\Backup\Listeners\EncryptBackupArchive@handle', ['event' => new BackupZipWasCreated($pathToZip)]); } return $pathToZip; } /** * Dumps the databases to the given directory. * Returns an array with paths to the dump files. * * @return array */ protected function dumpDatabases(): array { return $this->dbDumpers ->map(function (DbDumper $dbDumper, $key) { consoleOutput()->info("Dumping database {$dbDumper->getDbName()}..."); $dbType = mb_strtolower(basename(str_replace('\\', '/', get_class($dbDumper)))); $dbName = $dbDumper->getDbName(); if ($dbDumper instanceof Sqlite) { $dbName = $key . '-database'; } $fileName = "{$dbType}-{$dbName}.{$this->getExtension($dbDumper)}"; if (config('backup.backup.gzip_database_dump')) { $dbDumper->useCompressor(new GzipCompressor()); $fileName .= '.' . $dbDumper->getCompressorExtension(); } if ($compressor = config('backup.backup.database_dump_compressor')) { $dbDumper->useCompressor(new $compressor()); $fileName .= '.' . $dbDumper->getCompressorExtension(); } $temporaryFilePath = $this->temporaryDirectory->path('db-dumps' . DIRECTORY_SEPARATOR . $fileName); event(new DumpingDatabase($dbDumper)); $dbDumper->dumpToFile($temporaryFilePath); return $temporaryFilePath; }) ->toArray(); } protected function copyToBackupDestinations(string $path): void { $this->backupDestinations ->each(function (BackupDestination $backupDestination) use ($path) { try { if (! $backupDestination->isReachable()) { throw new Exception("Could not connect to disk {$backupDestination->diskName()} because: {$backupDestination->connectionError()}"); } consoleOutput()->info("Copying zip to disk named {$backupDestination->diskName()}..."); $backupDestination->write($path); consoleOutput()->info("Successfully copied zip to disk named {$backupDestination->diskName()}."); $this->sendNotification(new BackupWasSuccessful($backupDestination)); } catch (Exception $exception) { consoleOutput()->error("Copying zip failed because: {$exception->getMessage()}."); $this->sendNotification(new BackupHasFailed($exception, $backupDestination)); throw $exception; } }); } protected function sendNotification($notification): void { if ($this->sendNotifications) { rescue( fn () => event($notification), fn () => consoleOutput()->error('Sending notification failed') ); } } protected function getExtension(DbDumper $dbDumper): string { if ($extension = config('backup.backup.database_dump_file_extension')) { return $extension; } return $dbDumper instanceof MongoDb ? 'archive' : 'sql'; } } laravel-backup/src/Listeners/EncryptBackupArchive.php 0000644 00000002611 15002154456 0016763 0 ustar 00 <?php namespace Spatie\Backup\Listeners; use Spatie\Backup\Events\BackupZipWasCreated; use ZipArchive; class EncryptBackupArchive { public function handle(BackupZipWasCreated $event): void { if (! self::shouldEncrypt()) { return; } $zip = new ZipArchive(); $zip->open($event->pathToZip); $this->encrypt($zip); $zip->close(); } protected function encrypt(ZipArchive $zip): void { $zip->setPassword(static::getPassword()); foreach (range(0, $zip->numFiles - 1) as $i) { $zip->setEncryptionIndex($i, static::getAlgorithm()); } } public static function shouldEncrypt(): bool { $password = static::getPassword(); $algorithm = static::getAlgorithm(); if ($password === null) { return false; } if (! is_int($algorithm)) { return false; } return true; } protected static function getPassword(): ?string { return config('backup.backup.password'); } protected static function getAlgorithm(): ?int { $encryption = config('backup.backup.encryption'); if ($encryption === 'default') { $encryption = defined("\ZipArchive::EM_AES_256") ? ZipArchive::EM_AES_256 : null; } return $encryption; } } laravel-backup/src/Helpers/RightAlignedTableStyle.php 0000644 00000000346 15002154456 0016676 0 ustar 00 <?php namespace Spatie\Backup\Helpers; use Symfony\Component\Console\Helper\TableStyle; class RightAlignedTableStyle extends TableStyle { public function __construct() { $this->setPadType(STR_PAD_LEFT); } } laravel-backup/src/Helpers/ConsoleOutput.php 0000644 00000001106 15002154456 0015162 0 ustar 00 <?php namespace Spatie\Backup\Helpers; use Illuminate\Console\Command; /** * @phpstan-ignore-next-line * @mixin \Illuminate\Console\Concerns\InteractsWithIO */ class ConsoleOutput { protected ?Command $command = null; public function setCommand(Command $command): void { $this->command = $command; } public function __call(string $method, array $arguments) { $consoleOutput = app(static::class); if (! $consoleOutput->command) { return; } $consoleOutput->command->$method($arguments[0]); } } laravel-backup/src/Helpers/Format.php 0000644 00000001361 15002154456 0013572 0 ustar 00 <?php namespace Spatie\Backup\Helpers; use Carbon\Carbon; class Format { public static function humanReadableSize(float $sizeInBytes): string { $units = ['B', 'KB', 'MB', 'GB', 'TB']; if ($sizeInBytes === 0.0) { return '0 '.$units[1]; } for ($i = 0; $sizeInBytes > 1024; $i++) { $sizeInBytes /= 1024; } return round($sizeInBytes, 2).' '.$units[$i]; } public static function emoji(bool $bool): string { return $bool ? '✅' : '❌'; } public static function ageInDays(Carbon $date): string { return number_format(round($date->diffInMinutes() / (24 * 60), 2), 2).' ('.$date->diffForHumans().')'; } } laravel-backup/src/Helpers/functions.php 0000644 00000000203 15002154456 0014344 0 ustar 00 <?php use Spatie\Backup\Helpers\ConsoleOutput; function consoleOutput(): ConsoleOutput { return app(ConsoleOutput::class); } laravel-backup/src/Helpers/File.php 0000644 00000002271 15002154456 0013222 0 ustar 00 <?php namespace Spatie\Backup\Helpers; use Exception; use Illuminate\Contracts\Filesystem\Filesystem; class File { protected static array $allowedMimeTypes = [ 'application/zip', 'application/x-zip', 'application/x-gzip', ]; public function isZipFile(?Filesystem $disk, string $path): bool { if ($this->hasZipExtension($path)) { return true; } return $this->hasAllowedMimeType($disk, $path); } protected function hasZipExtension(string $path): bool { return pathinfo($path, PATHINFO_EXTENSION) === 'zip'; } protected function hasAllowedMimeType(?Filesystem $disk, string $path): bool { return in_array($this->mimeType($disk, $path), self::$allowedMimeTypes); } protected function mimeType(?Filesystem $disk, string $path): bool | string { try { if ($disk && method_exists($disk, 'mimeType')) { return $disk->mimeType($path) ?: false; } } catch (Exception) { // Some drivers throw exceptions when checking mime types, we'll // just fallback to `false`. } return false; } } laravel-backup/LICENSE.md 0000644 00000002102 15002154456 0011036 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-backup/config/backup.php 0000644 00000022271 15002154456 0012666 0 ustar 00 <?php return [ 'backup' => [ /* * The name of this application. You can use this name to monitor * the backups. */ 'name' => env('APP_NAME', 'laravel-backup'), 'source' => [ 'files' => [ /* * The list of directories and files that will be included in the backup. */ 'include' => [ base_path(), ], /* * These directories and files will be excluded from the backup. * * Directories used by the backup process will automatically be excluded. */ 'exclude' => [ base_path('vendor'), base_path('node_modules'), ], /* * Determines if symlinks should be followed. */ 'follow_links' => false, /* * Determines if it should avoid unreadable folders. */ 'ignore_unreadable_directories' => false, /* * This path is used to make directories in resulting zip-file relative * Set to `null` to include complete absolute path * Example: base_path() */ 'relative_path' => null, ], /* * The names of the connections to the databases that should be backed up * MySQL, PostgreSQL, SQLite and Mongo databases are supported. * * The content of the database dump may be customized for each connection * by adding a 'dump' key to the connection settings in config/database.php. * E.g. * 'mysql' => [ * ... * 'dump' => [ * 'excludeTables' => [ * 'table_to_exclude_from_backup', * 'another_table_to_exclude' * ] * ], * ], * * If you are using only InnoDB tables on a MySQL server, you can * also supply the useSingleTransaction option to avoid table locking. * * E.g. * 'mysql' => [ * ... * 'dump' => [ * 'useSingleTransaction' => true, * ], * ], * * For a complete list of available customization options, see https://github.com/spatie/db-dumper */ 'databases' => [ 'mysql', ], ], /* * The database dump can be compressed to decrease disk space usage. * * Out of the box Laravel-backup supplies * Spatie\DbDumper\Compressors\GzipCompressor::class. * * You can also create custom compressor. More info on that here: * https://github.com/spatie/db-dumper#using-compression * * If you do not want any compressor at all, set it to null. */ 'database_dump_compressor' => null, /* * The file extension used for the database dump files. * * If not specified, the file extension will be .archive for MongoDB and .sql for all other databases * The file extension should be specified without a leading . */ 'database_dump_file_extension' => '', 'destination' => [ /* * The filename prefix used for the backup zip file. */ 'filename_prefix' => '', /* * The disk names on which the backups will be stored. */ 'disks' => [ 'local', ], ], /* * The directory where the temporary files will be stored. */ 'temporary_directory' => storage_path('app/backup-temp'), /* * The password to be used for archive encryption. * Set to `null` to disable encryption. */ 'password' => env('BACKUP_ARCHIVE_PASSWORD'), /* * The encryption algorithm to be used for archive encryption. * You can set it to `null` or `false` to disable encryption. * * When set to 'default', we'll use ZipArchive::EM_AES_256 if it is * available on your system. */ 'encryption' => 'default', ], /* * You can get notified when specific events occur. Out of the box you can use 'mail' and 'slack'. * For Slack you need to install laravel/slack-notification-channel. * * You can also use your own notification classes, just make sure the class is named after one of * the `Spatie\Backup\Notifications\Notifications` classes. */ 'notifications' => [ 'notifications' => [ \Spatie\Backup\Notifications\Notifications\BackupHasFailedNotification::class => ['mail'], \Spatie\Backup\Notifications\Notifications\UnhealthyBackupWasFoundNotification::class => ['mail'], \Spatie\Backup\Notifications\Notifications\CleanupHasFailedNotification::class => ['mail'], \Spatie\Backup\Notifications\Notifications\BackupWasSuccessfulNotification::class => ['mail'], \Spatie\Backup\Notifications\Notifications\HealthyBackupWasFoundNotification::class => ['mail'], \Spatie\Backup\Notifications\Notifications\CleanupWasSuccessfulNotification::class => ['mail'], ], /* * Here you can specify the notifiable to which the notifications should be sent. The default * notifiable will use the variables specified in this config file. */ 'notifiable' => \Spatie\Backup\Notifications\Notifiable::class, 'mail' => [ 'to' => 'your@example.com', 'from' => [ 'address' => env('MAIL_FROM_ADDRESS', 'hello@example.com'), 'name' => env('MAIL_FROM_NAME', 'Example'), ], ], 'slack' => [ 'webhook_url' => '', /* * If this is set to null the default channel of the webhook will be used. */ 'channel' => null, 'username' => null, 'icon' => null, ], 'discord' => [ 'webhook_url' => '', /* * If this is an empty string, the name field on the webhook will be used. */ 'username' => '', /* * If this is an empty string, the avatar on the webhook will be used. */ 'avatar_url' => '', ], ], /* * Here you can specify which backups should be monitored. * If a backup does not meet the specified requirements the * UnHealthyBackupWasFound event will be fired. */ 'monitor_backups' => [ [ 'name' => env('APP_NAME', 'laravel-backup'), 'disks' => ['local'], 'health_checks' => [ \Spatie\Backup\Tasks\Monitor\HealthChecks\MaximumAgeInDays::class => 1, \Spatie\Backup\Tasks\Monitor\HealthChecks\MaximumStorageInMegabytes::class => 5000, ], ], /* [ 'name' => 'name of the second app', 'disks' => ['local', 's3'], 'health_checks' => [ \Spatie\Backup\Tasks\Monitor\HealthChecks\MaximumAgeInDays::class => 1, \Spatie\Backup\Tasks\Monitor\HealthChecks\MaximumStorageInMegabytes::class => 5000, ], ], */ ], 'cleanup' => [ /* * The strategy that will be used to cleanup old backups. The default strategy * will keep all backups for a certain amount of days. After that period only * a daily backup will be kept. After that period only weekly backups will * be kept and so on. * * No matter how you configure it the default strategy will never * delete the newest backup. */ 'strategy' => \Spatie\Backup\Tasks\Cleanup\Strategies\DefaultStrategy::class, 'default_strategy' => [ /* * The number of days for which backups must be kept. */ 'keep_all_backups_for_days' => 7, /* * The number of days for which daily backups must be kept. */ 'keep_daily_backups_for_days' => 16, /* * The number of weeks for which one weekly backup must be kept. */ 'keep_weekly_backups_for_weeks' => 8, /* * The number of months for which one monthly backup must be kept. */ 'keep_monthly_backups_for_months' => 4, /* * The number of years for which one yearly backup must be kept. */ 'keep_yearly_backups_for_years' => 2, /* * After cleaning up the backups remove the oldest backup until * this amount of megabytes has been reached. */ 'delete_oldest_backups_when_using_more_megabytes_than' => 5000, ], ], ]; laravel-backup/phpstan.neon.dist 0000644 00000000453 15002154456 0012741 0 ustar 00 includes: - phpstan-baseline.neon parameters: level: 5 paths: - src - config tmpDir: build/phpstan checkOctaneCompatibility: true checkModelProperties: true checkMissingIterableValueType: false ignoreErrors: - '#Unsafe usage of new static#' temporary-directory/composer.json 0000644 00000002053 15002154456 0013314 0 ustar 00 { "name": "spatie/temporary-directory", "description": "Easily create, use and destroy temporary directories", "license": "MIT", "keywords": [ "spatie", "php", "temporary-directory" ], "authors": [ { "name": "Alex Vanderbist", "email": "alex@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "homepage": "https://github.com/spatie/temporary-directory", "require": { "php": "^8.0" }, "require-dev": { "phpunit/phpunit": "^9.5" }, "minimum-stability": "dev", "prefer-stable": true, "autoload": { "psr-4": { "Spatie\\TemporaryDirectory\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\TemporaryDirectory\\Test\\": "tests" } }, "config": { "sort-packages": true }, "scripts": { "test": "vendor/bin/phpunit", "test-coverage": "vendor/bin/phpunit --coverage-html coverage" } } temporary-directory/README.md 0000644 00000013252 15002154456 0012054 0 ustar 00 # Quickly create, use and delete temporary directories [](https://packagist.org/packages/spatie/temporary-directory)  [](LICENSE.md) [](https://packagist.org/packages/spatie/temporary-directory) This package allows you to quickly create, use and delete a temporary directory in the system's temporary directory. Here's a quick example on how to create a temporary directory and delete it: ```php use Spatie\TemporaryDirectory\TemporaryDirectory; $temporaryDirectory = (new TemporaryDirectory())->create(); // Get a path inside the temporary directory $temporaryDirectory->path('temporaryfile.txt'); // Delete the temporary directory and all the files inside it $temporaryDirectory->delete(); ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/temporary-directory.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/temporary-directory) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation You can install the package via composer: ```bash composer require spatie/temporary-directory ``` ## Usage ### Creating a temporary directory To create a temporary directory simply call the `create` method on a `TemporaryDirectory` object. ```php (new TemporaryDirectory())->create(); ``` Alternatively, use the static `make` method on a `TemporaryDirectory` object. ```php TemporaryDirectory::make(); ``` By default, the temporary directory will be created in a timestamped directory in your system's temporary directory (usually `/tmp`). ### Naming your temporary directory If you want to use a custom name for your temporary directory instead of the timestamp call the `name` method with a string `$name` argument before the `create` method. ```php (new TemporaryDirectory()) ->name($name) ->create(); ``` By default an exception will be thrown if a directory already exists with the given argument. You can override this behaviour by calling the `force` method in combination with the `name` method. ```php (new TemporaryDirectory()) ->name($name) ->force() ->create(); ``` ### Setting a custom location for a temporary directory You can set a custom location in which your temporary directory will be created by passing a string `$location` argument to the `TemporaryDirectory` constructor. ```php (new TemporaryDirectory($location)) ->create(); ``` The `make` method also accepts a `$location` argument. ```php TemporaryDirectory::make($location); ``` Finally, you can call the `location` method with a `$location` argument. ```php (new TemporaryDirectory()) ->location($location) ->create(); ``` ### Determining paths within the temporary directory You can use the `path` method to determine the full path to a file or directory in the temporary directory: ```php $temporaryDirectory = (new TemporaryDirectory())->create(); $temporaryDirectory->path('dumps/datadump.dat'); // return /tmp/1485941876276/dumps/datadump.dat ``` ### Emptying a temporary directory Use the `empty` method to delete all the files inside the temporary directory. ```php $temporaryDirectory->empty(); ``` ### Deleting a temporary directory Once you're done processing your temporary data you can delete the entire temporary directory using the `delete` method. All files inside of it will be deleted. ```php $temporaryDirectory->delete(); ``` ### Deleting a temporary directory when the object is destroyed If you want to automatically have the filesystem directory deleted when the object instance has no more references in its defined scope, you can enable `deleteWhenDestroyed()` on the TemporaryDirectory object. ```php function handleTemporaryFiles() { $temporaryDirectory = (new TemporaryDirectory()) ->deleteWhenDestroyed() ->create(); // ... use the temporary directory return; // no need to manually call $temporaryDirectory->delete()! } handleTemporaryFiles(); ``` You can also call `unset()` on an object instance. ## Testing ```bash composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Postcardware You're free to use this package, but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/en/opensource/postcards). ## Credits - [Alex Vanderbist](https://github.com/AlexVanderbist) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. temporary-directory/src/Exceptions/InvalidDirectoryName.php 0000644 00000000430 15002154456 0020264 0 ustar 00 <?php namespace Spatie\TemporaryDirectory\Exceptions; class InvalidDirectoryName extends \Exception { public static function create(string $directoryName): static { return new static("The directory name `{$directoryName}` contains invalid characters."); } } temporary-directory/src/Exceptions/PathAlreadyExists.php 0000644 00000000350 15002154456 0017607 0 ustar 00 <?php namespace Spatie\TemporaryDirectory\Exceptions; class PathAlreadyExists extends \Exception { public static function create(string $path): static { return new static("Path `{$path}` already exists."); } } temporary-directory/src/TemporaryDirectory.php 0000644 00000011276 15002154456 0015750 0 ustar 00 <?php namespace Spatie\TemporaryDirectory; use FilesystemIterator; use Spatie\TemporaryDirectory\Exceptions\InvalidDirectoryName; use Spatie\TemporaryDirectory\Exceptions\PathAlreadyExists; use Throwable; class TemporaryDirectory { protected string $location; protected string $name = ''; protected bool $forceCreate = false; protected bool $deleteWhenDestroyed = false; public function __construct(string $location = '') { $this->location = $this->sanitizePath($location); } public static function make(string $location = ''): self { return (new self($location))->create(); } public function create(): self { if (empty($this->location)) { $this->location = $this->getSystemTemporaryDirectory(); } if (empty($this->name)) { $this->name = mt_rand().'-'.str_replace([' ', '.'], '', microtime()); } if ($this->forceCreate && file_exists($this->getFullPath())) { $this->deleteDirectory($this->getFullPath()); } if ($this->exists()) { throw PathAlreadyExists::create($this->getFullPath()); } mkdir($this->getFullPath(), 0777, true); return $this; } public function force(): self { $this->forceCreate = true; return $this; } public function name(string $name): self { $this->name = $this->sanitizeName($name); return $this; } public function location(string $location): self { $this->location = $this->sanitizePath($location); return $this; } public function path(string $pathOrFilename = ''): string { if (empty($pathOrFilename)) { return $this->getFullPath(); } $path = $this->getFullPath().DIRECTORY_SEPARATOR.trim($pathOrFilename, '/'); $directoryPath = $this->removeFilenameFromPath($path); if (! file_exists($directoryPath)) { mkdir($directoryPath, 0777, true); } return $path; } public function empty(): self { $this->deleteDirectory($this->getFullPath()); mkdir($this->getFullPath(), 0777, true); return $this; } public function delete(): bool { return $this->deleteDirectory($this->getFullPath()); } public function exists(): bool { return file_exists($this->getFullPath()); } protected function getFullPath(): string { return $this->location.(! empty($this->name) ? DIRECTORY_SEPARATOR.$this->name : ''); } protected function isValidDirectoryName(string $directoryName): bool { return strpbrk($directoryName, '\\/?%*:|"<>') === false; } protected function getSystemTemporaryDirectory(): string { return rtrim(sys_get_temp_dir(), DIRECTORY_SEPARATOR); } protected function sanitizePath(string $path): string { $path = rtrim($path); return rtrim($path, DIRECTORY_SEPARATOR); } protected function sanitizeName(string $name): string { if (! $this->isValidDirectoryName($name)) { throw InvalidDirectoryName::create($name); } return trim($name); } protected function removeFilenameFromPath(string $path): string { if (! $this->isFilePath($path)) { return $path; } return substr($path, 0, strrpos($path, DIRECTORY_SEPARATOR)); } protected function isFilePath(string $path): bool { return str_contains($path, '.'); } protected function deleteDirectory(string $path): bool { try { if (is_link($path)) { return unlink($path); } if (! file_exists($path)) { return true; } if (! is_dir($path)) { return unlink($path); } foreach (new FilesystemIterator($path) as $item) { if (! $this->deleteDirectory((string) $item)) { return false; } } /* * By forcing a php garbage collection cycle using gc_collect_cycles() we can ensure * that the rmdir does not fail due to files still being reserved in memory. */ gc_collect_cycles(); return rmdir($path); } catch (Throwable) { return false; } } public function deleteWhenDestroyed(bool $deleteWhenDestroyed = true): self { $this->deleteWhenDestroyed = $deleteWhenDestroyed; return $this; } public function __destruct() { if ($this->deleteWhenDestroyed) { $this->delete(); } } } temporary-directory/LICENSE.md 0000644 00000002102 15002154456 0012171 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-package-tools/composer.json 0000644 00000002377 15002154456 0013456 0 ustar 00 { "name": "spatie/laravel-package-tools", "description": "Tools for creating Laravel packages", "keywords": [ "spatie", "laravel-package-tools" ], "homepage": "https://github.com/spatie/laravel-package-tools", "license": "MIT", "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "role": "Developer" } ], "require": { "php": "^8.0", "illuminate/contracts": "^9.28|^10.0|^11.0" }, "require-dev": { "mockery/mockery": "^1.5", "orchestra/testbench": "^7.7|^8.0", "pestphp/pest": "^1.22", "phpunit/phpunit": "^9.5.24", "spatie/pest-plugin-test-time": "^1.1" }, "autoload": { "psr-4": { "Spatie\\LaravelPackageTools\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\LaravelPackageTools\\Tests\\": "tests" } }, "scripts": { "test": "vendor/bin/pest", "test-coverage": "vendor/bin/pest --coverage" }, "config": { "sort-packages": true, "allow-plugins": { "pestphp/pest-plugin": true } }, "minimum-stability": "dev", "prefer-stable": true } laravel-package-tools/README.md 0000644 00000043253 15002154456 0012211 0 ustar 00 # Tools for creating Laravel packages [](https://packagist.org/packages/spatie/laravel-package-tools)  [](https://packagist.org/packages/spatie/laravel-package-tools) This package contains a `PackageServiceProvider` that you can use in your packages to easily register config files, migrations, and more. Here's an example of how it can be used. ```php use Spatie\LaravelPackageTools\PackageServiceProvider; use Spatie\LaravelPackageTools\Package; use MyPackage\ViewComponents\Alert; use Spatie\LaravelPackageTools\Commands\InstallCommand; class YourPackageServiceProvider extends PackageServiceProvider { public function configurePackage(Package $package): void { $package ->name('your-package-name') ->hasConfigFile() ->hasViews() ->hasViewComponent('spatie', Alert::class) ->hasViewComposer('*', MyViewComposer::class) ->sharesDataWithAllViews('downloads', 3) ->hasTranslations() ->hasAssets() ->publishesServiceProvider('MyProviderName') ->hasRoute('web') ->hasMigration('create_package_tables') ->hasCommand(YourCoolPackageCommand::class) ->hasInstallCommand(function(InstallCommand $command) { $command ->publishConfigFile() ->publishAssets() ->publishMigrations() ->copyAndRegisterServiceProviderInApp() ->askToStarRepoOnGitHub(); }); } } ``` Under the hood it will do the necessary work to register the necessary things and make all sorts of files publishable. ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/laravel-package-tools.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/laravel-package-tools) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Getting started This package is opinionated on how you should structure your package. To get started easily, consider using [our package-skeleton repo](https://github.com/spatie/package-skeleton-laravel) to start your package. The skeleton is structured perfectly to work perfectly with the `PackageServiceProvider` in this package. ## Usage In your package you should let your service provider extend `Spatie\LaravelPackageTools\PackageServiceProvider`. ```php use Spatie\LaravelPackageTools\PackageServiceProvider; use Spatie\LaravelPackageTools\Package; class YourPackageServiceProvider extends PackageServiceProvider { public function configurePackage(Package $package) : void { $package->name('your-package-name'); } } ``` Passing the package name to `name` is mandatory. ### Working with a config file To register a config file, you should create a php file with your package name in the `config` directory of your package. In this example it should be at `<package root>/config/your-package-name.php`. If your package name starts with `laravel-`, we expect that your config file does not contain that prefix. So if your package name is `laravel-cool-package`, the config file should be named `cool-package.php`. To register that config file, call `hasConfigFile()` on `$package` in the `configurePackage` method. ```php $package ->name('your-package-name') ->hasConfigFile(); ``` The `hasConfigFile` method will also make the config file publishable. Users of your package will be able to publish the config file with this command. ```bash php artisan vendor:publish --tag=your-package-name-config ``` Should your package have multiple config files, you can pass their names as an array to `hasConfigFile` ```php $package ->name('your-package-name') ->hasConfigFile(['my-config-file', 'another-config-file']); ``` ### Working with views Any views your package provides, should be placed in the `<package root>/resources/views` directory. You can register these views with the `hasViews` command. ```php $package ->name('your-package-name') ->hasViews(); ``` This will register your views with Laravel. If you have a view `<package root>/resources/views/myView.blade.php`, you can use it like this: `view('your-package-name::myView')`. Of course, you can also use subdirectories to organise your views. A view located at `<package root>/resources/views/subdirectory/myOtherView.blade.php` can be used with `view('your-package-name::subdirectory.myOtherView')`. #### Using a custom view namespace You can pass a custom view namespace to the `hasViews` method. ```php $package ->name('your-package-name') ->hasViews('custom-view-namespace'); ``` You can now use the views of the package like this: ```php view('custom-view-namespace::myView'); ``` #### Publishing the views Calling `hasViews` will also make views publishable. Users of your package will be able to publish the views with this command: ```bash php artisan vendor:publish --tag=your-package-name-views ``` > **Note:** > > If you use custom view namespace then you should change your publish command like this: ```bash php artisan vendor:publish --tag=custom-view-namespace-views ``` ### Sharing global data with views You can share data with all views using the `sharesDataWithAllViews` method. This will make the shared variable available to all views. ```php $package ->name('your-package-name') ->sharesDataWithAllViews('companyName', 'Spatie'); ``` ### Working with Blade view components Any Blade view components that your package provides should be placed in the `<package root>/src/Components` directory. You can register these views with the `hasViewComponents` command. ```php $package ->name('your-package-name') ->hasViewComponents('spatie', Alert::class); ``` This will register your view components with Laravel. In the case of `Alert::class`, it can be referenced in views as `<x-spatie-alert />`, where `spatie` is the prefix you provided during registration. Calling `hasViewComponents` will also make view components publishable, and will be published to `app/Views/Components/vendor/<package name>`. Users of your package will be able to publish the view components with this command: ```bash php artisan vendor:publish --tag=your-package-name-components ``` ### Working with view composers You can register any view composers that your project uses with the `hasViewComposers` method. You may also register a callback that receives a `$view` argument instead of a classname. To register a view composer with all views, use an asterisk as the view name `'*'`. ```php $package ->name('your-package-name') ->hasViewComposer('viewName', MyViewComposer::class) ->hasViewComposer('*', function($view) { $view->with('sharedVariable', 123); }); ``` ### Working with inertia components Any `.vue` or `.jsx` files your package provides, should be placed in the `<package root>/resources/js/Pages` directory. You can register these components with the `hasInertiaComponents` command. ```php $package ->name('your-package-name') ->hasInertiaComponents(); ``` This will register your components with Laravel. The user should publish the inertia components manually or using the [installer-command](#adding-an-installer-command) in order to use them. If you have an inertia component `<package root>/resources/js/Pages/myComponent.vue`, you can use it like this: `Inertia::render('YourPackageName/myComponent')`. Of course, you can also use subdirectories to organise your components. #### Publishing inertia components Calling `hasInertiaComponents` will also make inertia components publishable. Users of your package will be able to publish the views with this command: ```bash php artisan vendor:publish --tag=your-package-name-inertia-components ``` Also, the inertia components are available in a convenient way with your package [installer-command](#adding-an-installer-command) ### Working with translations Any translations your package provides, should be placed in the `<package root>/resources/lang/<language-code>` directory. You can register these translations with the `hasTranslations` command. ```php $package ->name('your-package-name') ->hasTranslations(); ``` This will register the translations with Laravel. Assuming you save this translation file at `<package root>/resources/lang/en/translations.php`... ```php return [ 'translatable' => 'translation', ]; ``` ... your package and users will be able to retrieve the translation with: ```php trans('your-package-name::translations.translatable'); // returns 'translation' ``` If your package name starts with `laravel-` then you should leave that off in the example above. Coding with translation strings as keys, you should create JSON files in `<package root>/resources/lang/<language-code>.json`. For example, creating `<package root>/resources/lang/it.json` file like so: ```json { "Hello!": "Ciao!" } ``` ...the output of... ```php trans('Hello!'); ``` ...will be `Ciao!` if the application uses the Italian language. Calling `hasTranslations` will also make translations publishable. Users of your package will be able to publish the translations with this command: ```bash php artisan vendor:publish --tag=your-package-name-translations ``` ### Working with assets Any assets your package provides, should be placed in the `<package root>/resources/dist/` directory. You can make these assets publishable the `hasAssets` method. ```php $package ->name('your-package-name') ->hasAssets(); ``` Users of your package will be able to publish the assets with this command: ```bash php artisan vendor:publish --tag=your-package-name-assets ``` This will copy over the assets to the `public/vendor/<your-package-name>` directory in the app where your package is installed in. ### Working with migrations The `PackageServiceProvider` assumes that any migrations are placed in this directory: `<package root>/database/migrations`. Inside that directory you can put any migrations. To register your migration, you should pass its name without the extension to the `hasMigration` table. If your migration file is called `create_my_package_tables.php.stub` you can register them like this: ```php $package ->name('your-package-name') ->hasMigration('create_my_package_tables'); ``` Should your package contain multiple migration files, you can just call `hasMigration` multiple times or use `hasMigrations`. ```php $package ->name('your-package-name') ->hasMigrations(['my_package_tables', 'some_other_migration']); ``` Calling `hasMigration` will also make migrations publishable. Users of your package will be able to publish the migrations with this command: ```bash php artisan vendor:publish --tag=your-package-name-migrations ``` Like you might expect, published migration files will be prefixed with the current datetime. You can also enable the migrations to be registered without needing the users of your package to publish them: ```php $package ->name('your-package-name') ->hasMigrations(['my_package_tables', 'some_other_migration']) ->runsMigrations(); ``` ### Working with a publishable service provider Some packages need an example service provider to be copied into the `app\Providers` directory of the Laravel app. Think of for instance, the `laravel/horizon` package that copies an `HorizonServiceProvider` into your app with some sensible defaults. ```php $package ->name('your-package-name') ->publishesServiceProvider($nameOfYourServiceProvider); ``` The file that will be copied to the app should be stored in your package in `/resources/stubs/{$nameOfYourServiceProvider}.php.stub`. When your package is installed into an app, running this command... ```bash php artisan vendor:publish --tag=your-package-name-provider ``` ... will copy `/resources/stubs/{$nameOfYourServiceProvider}.php.stub` in your package to `app/Providers/{$nameOfYourServiceProvider}.php` in the app of the user. ### Registering commands You can register any command you package provides with the `hasCommand` function. ```php $package ->name('your-package-name') ->hasCommand(YourCoolPackageCommand::class); ```` If your package provides multiple commands, you can either use `hasCommand` multiple times, or pass an array to `hasCommands` ```php $package ->name('your-package-name') ->hasCommands([ YourCoolPackageCommand::class, YourOtherCoolPackageCommand::class, ]); ``` ### Adding an installer command Instead of letting your users manually publishing config files, migrations, and other files manually, you could opt to add an install command that does all this work in one go. Packages like Laravel Horizon and Livewire provide such commands. When using Laravel Package Tools, you don't have to write an `InstallCommand` yourself. Instead, you can simply call, `hasInstallCommand` and configure it using a closure. Here's an example. ```php use Spatie\LaravelPackageTools\PackageServiceProvider; use Spatie\LaravelPackageTools\Package; use Spatie\LaravelPackageTools\Commands\InstallCommand; class YourPackageServiceProvider extends PackageServiceProvider { public function configurePackage(Package $package): void { $package ->name('your-package-name') ->hasConfigFile() ->hasMigration('create_package_tables') ->publishesServiceProvider('MyServiceProviderName') ->hasInstallCommand(function(InstallCommand $command) { $command ->publishConfigFile() ->publishAssets() ->publishMigrations() ->askToRunMigrations() ->copyAndRegisterServiceProviderInApp() ->askToStarRepoOnGitHub('your-vendor/your-repo-name') }); } } ``` With this in place, the package user can call this command: ```bash php artisan your-package-name:install ``` Using the code above, that command will: - publish the config file - publish the assets - publish the migrations - copy the `/resources/stubs/MyProviderName.php.stub` from your package to `app/Providers/MyServiceProviderName.php`, and also register that provider in `config/app.php` - ask if migrations should be run now - prompt the user to open up `https://github.com/'your-vendor/your-repo-name'` in the browser in order to star it You can also call `startWith` and `endWith` on the `InstallCommand`. They will respectively be executed at the start and end when running `php artisan your-package-name:install`. You can use this to perform extra work or display extra output. ```php use Spatie\LaravelPackageTools\Commands\InstallCommand; public function configurePackage(Package $package): void { $package // ... configure package ->hasInstallCommand(function(InstallCommand $command) { $command ->startWith(function(InstallCommand $command) { $command->info('Hello, and welcome to my great new package!'); }) ->publishConfigFile() ->publishAssets() ->publishMigrations() ->askToRunMigrations() ->copyAndRegisterServiceProviderInApp() ->askToStarRepoOnGitHub('your-vendor/your-repo-name') ->endWith(function(InstallCommand $command) { $command->info('Have a great day!'); }) }); } ``` ### Working with routes The `PackageServiceProvider` assumes that any route files are placed in this directory: `<package root>/routes`. Inside that directory you can put any route files. To register your route, you should pass its name without the extension to the `hasRoute` method. If your route file is called `web.php` you can register them like this: ```php $package ->name('your-package-name') ->hasRoute('web'); ``` Should your package contain multiple route files, you can just call `hasRoute` multiple times or use `hasRoutes`. ```php $package ->name('your-package-name') ->hasRoutes(['web', 'admin']); ``` ### Using lifecycle hooks You can put any custom logic your package needs while starting up in one of these methods: - `registeringPackage`: will be called at the start of the `register` method of `PackageServiceProvider` - `packageRegistered`: will be called at the end of the `register` method of `PackageServiceProvider` - `bootingPackage`: will be called at the start of the `boot` method of `PackageServiceProvider` - `packageBooted`: will be called at the end of the `boot` method of `PackageServiceProvider` ## Testing ```bash composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. laravel-package-tools/src/Exceptions/InvalidPackage.php 0000644 00000000445 15002154456 0017211 0 ustar 00 <?php namespace Spatie\LaravelPackageTools\Exceptions; use Exception; class InvalidPackage extends Exception { public static function nameIsRequired(): self { return new static('This package does not have a name. You can set one with `$package->name("yourName")`'); } } laravel-package-tools/src/PackageServiceProvider.php 0000644 00000016317 15002154456 0016622 0 ustar 00 <?php namespace Spatie\LaravelPackageTools; use Carbon\Carbon; use Illuminate\Support\Facades\View; use Illuminate\Support\ServiceProvider; use Illuminate\Support\Str; use ReflectionClass; use Spatie\LaravelPackageTools\Exceptions\InvalidPackage; abstract class PackageServiceProvider extends ServiceProvider { protected Package $package; abstract public function configurePackage(Package $package): void; public function register() { $this->registeringPackage(); $this->package = $this->newPackage(); $this->package->setBasePath($this->getPackageBaseDir()); $this->configurePackage($this->package); if (empty($this->package->name)) { throw InvalidPackage::nameIsRequired(); } foreach ($this->package->configFileNames as $configFileName) { $this->mergeConfigFrom($this->package->basePath("/../config/{$configFileName}.php"), $configFileName); } $this->packageRegistered(); return $this; } public function newPackage(): Package { return new Package(); } public function boot() { $this->bootingPackage(); if ($this->package->hasTranslations) { $langPath = 'vendor/' . $this->package->shortName(); $langPath = (function_exists('lang_path')) ? lang_path($langPath) : resource_path('lang/' . $langPath); } if ($this->app->runningInConsole()) { foreach ($this->package->configFileNames as $configFileName) { $this->publishes([ $this->package->basePath("/../config/{$configFileName}.php") => config_path("{$configFileName}.php"), ], "{$this->package->shortName()}-config"); } if ($this->package->hasViews) { $this->publishes([ $this->package->basePath('/../resources/views') => base_path("resources/views/vendor/{$this->packageView($this->package->viewNamespace)}"), ], "{$this->packageView($this->package->viewNamespace)}-views"); } if ($this->package->hasInertiaComponents) { $packageDirectoryName = Str::of($this->packageView($this->package->viewNamespace))->studly()->remove('-')->value(); $this->publishes([ $this->package->basePath('/../resources/js/Pages') => base_path("resources/js/Pages/{$packageDirectoryName}"), ], "{$this->packageView($this->package->viewNamespace)}-inertia-components"); } $now = Carbon::now(); foreach ($this->package->migrationFileNames as $migrationFileName) { $filePath = $this->package->basePath("/../database/migrations/{$migrationFileName}.php"); if (! file_exists($filePath)) { // Support for the .stub file extension $filePath .= '.stub'; } $this->publishes([ $filePath => $this->generateMigrationName( $migrationFileName, $now->addSecond() ), ], "{$this->package->shortName()}-migrations"); if ($this->package->runsMigrations) { $this->loadMigrationsFrom($filePath); } } if ($this->package->hasTranslations) { $this->publishes([ $this->package->basePath('/../resources/lang') => $langPath, ], "{$this->package->shortName()}-translations"); } if ($this->package->hasAssets) { $this->publishes([ $this->package->basePath('/../resources/dist') => public_path("vendor/{$this->package->shortName()}"), ], "{$this->package->shortName()}-assets"); } } if (! empty($this->package->commands)) { $this->commands($this->package->commands); } if (! empty($this->package->consoleCommands) && $this->app->runningInConsole()) { $this->commands($this->package->consoleCommands); } if ($this->package->hasTranslations) { $this->loadTranslationsFrom( $this->package->basePath('/../resources/lang/'), $this->package->shortName() ); $this->loadJsonTranslationsFrom($this->package->basePath('/../resources/lang/')); $this->loadJsonTranslationsFrom($langPath); } if ($this->package->hasViews) { $this->loadViewsFrom($this->package->basePath('/../resources/views'), $this->package->viewNamespace()); } foreach ($this->package->viewComponents as $componentClass => $prefix) { $this->loadViewComponentsAs($prefix, [$componentClass]); } if (count($this->package->viewComponents)) { $this->publishes([ $this->package->basePath('/Components') => base_path("app/View/Components/vendor/{$this->package->shortName()}"), ], "{$this->package->name}-components"); } if ($this->package->publishableProviderName) { $this->publishes([ $this->package->basePath("/../resources/stubs/{$this->package->publishableProviderName}.php.stub") => base_path("app/Providers/{$this->package->publishableProviderName}.php"), ], "{$this->package->shortName()}-provider"); } foreach ($this->package->routeFileNames as $routeFileName) { $this->loadRoutesFrom("{$this->package->basePath('/../routes/')}{$routeFileName}.php"); } foreach ($this->package->sharedViewData as $name => $value) { View::share($name, $value); } foreach ($this->package->viewComposers as $viewName => $viewComposer) { View::composer($viewName, $viewComposer); } $this->packageBooted(); return $this; } public static function generateMigrationName(string $migrationFileName, Carbon $now): string { $migrationsPath = 'migrations/'; $len = strlen($migrationFileName) + 4; if (Str::contains($migrationFileName, '/')) { $migrationsPath .= Str::of($migrationFileName)->beforeLast('/')->finish('/'); $migrationFileName = Str::of($migrationFileName)->afterLast('/'); } foreach (glob(database_path("{$migrationsPath}*.php")) as $filename) { if ((substr($filename, -$len) === $migrationFileName . '.php')) { return $filename; } } return database_path($migrationsPath . $now->format('Y_m_d_His') . '_' . Str::of($migrationFileName)->snake()->finish('.php')); } public function registeringPackage() { } public function packageRegistered() { } public function bootingPackage() { } public function packageBooted() { } protected function getPackageBaseDir(): string { $reflector = new ReflectionClass(get_class($this)); return dirname($reflector->getFileName()); } public function packageView(?string $namespace) { return is_null($namespace) ? $this->package->shortName() : $this->package->viewNamespace; } } laravel-package-tools/src/Package.php 0000644 00000012435 15002154456 0013563 0 ustar 00 <?php namespace Spatie\LaravelPackageTools; use Illuminate\Support\Str; use Spatie\LaravelPackageTools\Commands\InstallCommand; class Package { public string $name; public array $configFileNames = []; public bool $hasViews = false; public bool $hasInertiaComponents = false; public ?string $viewNamespace = null; public bool $hasTranslations = false; public bool $hasAssets = false; public bool $runsMigrations = false; public array $migrationFileNames = []; public array $routeFileNames = []; public array $commands = []; public array $consoleCommands = []; public array $viewComponents = []; public array $sharedViewData = []; public array $viewComposers = []; public string $basePath; public ?string $publishableProviderName = null; public function name(string $name): static { $this->name = $name; return $this; } public function hasConfigFile($configFileName = null): static { $configFileName ??= $this->shortName(); if (! is_array($configFileName)) { $configFileName = [$configFileName]; } $this->configFileNames = $configFileName; return $this; } public function publishesServiceProvider(string $providerName): static { $this->publishableProviderName = $providerName; return $this; } public function hasInstallCommand($callable): static { $installCommand = new InstallCommand($this); $callable($installCommand); $this->consoleCommands[] = $installCommand; return $this; } public function shortName(): string { return Str::after($this->name, 'laravel-'); } public function hasViews(string $namespace = null): static { $this->hasViews = true; $this->viewNamespace = $namespace; return $this; } public function hasInertiaComponents(string $namespace = null): static { $this->hasInertiaComponents = true; $this->viewNamespace = $namespace; return $this; } public function hasViewComponent(string $prefix, string $viewComponentName): static { $this->viewComponents[$viewComponentName] = $prefix; return $this; } public function hasViewComponents(string $prefix, ...$viewComponentNames): static { foreach ($viewComponentNames as $componentName) { $this->viewComponents[$componentName] = $prefix; } return $this; } public function sharesDataWithAllViews(string $name, $value): static { $this->sharedViewData[$name] = $value; return $this; } public function hasViewComposer($view, $viewComposer): static { if (! is_array($view)) { $view = [$view]; } foreach ($view as $viewName) { $this->viewComposers[$viewName] = $viewComposer; } return $this; } public function hasTranslations(): static { $this->hasTranslations = true; return $this; } public function hasAssets(): static { $this->hasAssets = true; return $this; } public function runsMigrations(bool $runsMigrations = true): static { $this->runsMigrations = $runsMigrations; return $this; } public function hasMigration(string $migrationFileName): static { $this->migrationFileNames[] = $migrationFileName; return $this; } public function hasMigrations(...$migrationFileNames): static { $this->migrationFileNames = array_merge( $this->migrationFileNames, collect($migrationFileNames)->flatten()->toArray() ); return $this; } public function hasCommand(string $commandClassName): static { $this->commands[] = $commandClassName; return $this; } public function hasCommands(...$commandClassNames): static { $this->commands = array_merge($this->commands, collect($commandClassNames)->flatten()->toArray()); return $this; } public function hasConsoleCommand(string $commandClassName): static { $this->consoleCommands[] = $commandClassName; return $this; } public function hasConsoleCommands(...$commandClassNames): static { $this->consoleCommands = array_merge($this->consoleCommands, collect($commandClassNames)->flatten()->toArray()); return $this; } public function hasRoute(string $routeFileName): static { $this->routeFileNames[] = $routeFileName; return $this; } public function hasRoutes(...$routeFileNames): static { $this->routeFileNames = array_merge($this->routeFileNames, collect($routeFileNames)->flatten()->toArray()); return $this; } public function basePath(string $directory = null): string { if ($directory === null) { return $this->basePath; } return $this->basePath . DIRECTORY_SEPARATOR . ltrim($directory, DIRECTORY_SEPARATOR); } public function viewNamespace(): string { return $this->viewNamespace ?? $this->shortName(); } public function setBasePath(string $path): static { $this->basePath = $path; return $this; } } laravel-package-tools/src/Commands/InstallCommand.php 0000644 00000011202 15002154456 0016665 0 ustar 00 <?php namespace Spatie\LaravelPackageTools\Commands; use Closure; use Illuminate\Console\Command; use Illuminate\Support\Str; use Spatie\LaravelPackageTools\Package; class InstallCommand extends Command { protected Package $package; public ?Closure $startWith = null; protected array $publishes = []; protected bool $askToRunMigrations = false; protected bool $copyServiceProviderInApp = false; protected ?string $starRepo = null; public ?Closure $endWith = null; public $hidden = true; public function __construct(Package $package) { $this->signature = $package->shortName() . ':install'; $this->description = 'Install ' . $package->name; $this->package = $package; parent::__construct(); } public function handle() { if ($this->startWith) { ($this->startWith)($this); } foreach ($this->publishes as $tag) { $name = str_replace('-', ' ', $tag); $this->comment("Publishing {$name}..."); $this->callSilently("vendor:publish", [ '--tag' => "{$this->package->shortName()}-{$tag}", ]); } if ($this->askToRunMigrations) { if ($this->confirm('Would you like to run the migrations now?')) { $this->comment('Running migrations...'); $this->call('migrate'); } } if ($this->copyServiceProviderInApp) { $this->comment('Publishing service provider...'); $this->copyServiceProviderInApp(); } if ($this->starRepo) { if ($this->confirm('Would you like to star our repo on GitHub?')) { $repoUrl = "https://github.com/{$this->starRepo}"; if (PHP_OS_FAMILY == 'Darwin') { exec("open {$repoUrl}"); } if (PHP_OS_FAMILY == 'Windows') { exec("start {$repoUrl}"); } if (PHP_OS_FAMILY == 'Linux') { exec("xdg-open {$repoUrl}"); } } } $this->info("{$this->package->shortName()} has been installed!"); if ($this->endWith) { ($this->endWith)($this); } } public function publish(string ...$tag): self { $this->publishes = array_merge($this->publishes, $tag); return $this; } public function publishConfigFile(): self { return $this->publish('config'); } public function publishAssets(): self { return $this->publish('assets'); } public function publishInertiaComponents(): self { return $this->publish('inertia-components'); } public function publishMigrations(): self { return $this->publish('migrations'); } public function askToRunMigrations(): self { $this->askToRunMigrations = true; return $this; } public function copyAndRegisterServiceProviderInApp(): self { $this->copyServiceProviderInApp = true; return $this; } public function askToStarRepoOnGitHub($vendorSlashRepoName): self { $this->starRepo = $vendorSlashRepoName; return $this; } public function startWith($callable): self { $this->startWith = $callable; return $this; } public function endWith($callable): self { $this->endWith = $callable; return $this; } protected function copyServiceProviderInApp(): self { $providerName = $this->package->publishableProviderName; if (! $providerName) { return $this; } $this->callSilent('vendor:publish', ['--tag' => $this->package->shortName() . '-provider']); $namespace = Str::replaceLast('\\', '', $this->laravel->getNamespace()); $appConfig = file_get_contents(config_path('app.php')); $class = '\\Providers\\' . $providerName . '::class'; if (Str::contains($appConfig, $namespace . $class)) { return $this; } file_put_contents(config_path('app.php'), str_replace( "{$namespace}\\Providers\\BroadcastServiceProvider::class,", "{$namespace}\\Providers\\BroadcastServiceProvider::class," . PHP_EOL . " {$namespace}{$class},", $appConfig )); file_put_contents(app_path('Providers/' . $providerName . '.php'), str_replace( "namespace App\Providers;", "namespace {$namespace}\Providers;", file_get_contents(app_path('Providers/' . $providerName . '.php')) )); return $this; } } laravel-package-tools/LICENSE.md 0000644 00000002076 15002154456 0012334 0 ustar 00 The MIT License (MIT) Copyright (c) spatie <freek@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. flysystem-dropbox/CONTRIBUTING.md 0000644 00000005631 15002154456 0012516 0 ustar 00 # Contributing Contributions are **welcome** and will be fully **credited**. Please read and understand the contribution guide before creating an issue or pull request. ## Etiquette This project is open source, and as such, the maintainers give their free time to build and maintain the source code held within. They make the code freely available in the hope that it will be of use to other developers. It would be extremely unfair for them to suffer abuse or anger for their hard work. Please be considerate towards maintainers when raising issues or presenting pull requests. Let's show the world that developers are civilized and selfless people. It's the duty of the maintainer to ensure that all submissions to the project are of sufficient quality to benefit the project. Many developers have different skillsets, strengths, and weaknesses. Respect the maintainer's decision, and do not be upset or abusive if your submission is not used. ## Viability When requesting or submitting new features, first consider whether it might be useful to others. Open source projects are used by many developers, who may have entirely different needs to your own. Think about whether or not your feature is likely to be used by other users of the project. ## Procedure Before filing an issue: - Attempt to replicate the problem, to ensure that it wasn't a coincidental incident. - Check to make sure your feature suggestion isn't already present within the project. - Check the pull requests tab to ensure that the bug doesn't have a fix in progress. - Check the pull requests tab to ensure that the feature isn't already in progress. Before submitting a pull request: - Check the codebase to ensure that your feature doesn't already exist. - Check the pull requests to ensure that another person hasn't already submitted the feature or fix. ## Requirements If the project maintainer has any additional requirements, you will find them listed here. - **[PSR-2 Coding Standard](https://github.com/php-fig/fig-standards/blob/master/accepted/PSR-2-coding-style-guide.md)** - The easiest way to apply the conventions is to install [PHP Code Sniffer](http://pear.php.net/package/PHP_CodeSniffer). - **Add tests!** - Your patch won't be accepted if it doesn't have tests. - **Document any change in behaviour** - Make sure the `README.md` and any other relevant documentation are kept up-to-date. - **Consider our release cycle** - We try to follow [SemVer v2.0.0](http://semver.org/). Randomly breaking public APIs is not an option. - **One pull request per feature** - If you want to do more than one thing, send multiple pull requests. - **Send coherent history** - Make sure each individual commit in your pull request is meaningful. If you had to make multiple intermediate commits while developing, please [squash them](http://www.git-scm.com/book/en/v2/Git-Tools-Rewriting-History#Changing-Multiple-Commit-Messages) before submitting. **Happy coding**! flysystem-dropbox/composer.json 0000644 00000002134 15002154456 0013002 0 ustar 00 { "name": "spatie/flysystem-dropbox", "description": "Flysystem Adapter for the Dropbox v2 API", "keywords": [ "spatie", "flysystem-dropbox", "flysystem", "dropbox", "v2", "api" ], "homepage": "https://github.com/spatie/flysystem-dropbox", "license": "MIT", "authors": [ { "name": "Alex Vanderbist", "email": "alex.vanderbist@gmail.com", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^8.0", "league/flysystem": "^2.0.4 || ^3.0.0", "spatie/dropbox-api": "^1.17.1" }, "require-dev": { "phpspec/prophecy-phpunit": "^2.0.1", "phpunit/phpunit": "^9.5.4" }, "autoload": { "psr-4": { "Spatie\\FlysystemDropbox\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\FlysystemDropbox\\Test\\": "tests" } }, "scripts": { "test": "vendor/bin/phpunit" }, "config": { "sort-packages": true } } flysystem-dropbox/README.md 0000644 00000006560 15002154456 0011546 0 ustar 00 # Flysystem adapter for the Dropbox API [](https://packagist.org/packages/spatie/flysystem-dropbox) [](https://github.com/spatie/flysystem-dropbox/actions/workflows/run-tests.yml) [](https://packagist.org/packages/spatie/flysystem-dropbox) This package contains a [Flysystem](https://flysystem.thephpleague.com/) adapter for Dropbox. Under the hood, the [Dropbox API v2](https://www.dropbox.com/developers/documentation/http/overview) is used. ## Using Flystem v1 If you're using Flysystem v1, then use [v1 of flysystem-dropbox](https://github.com/spatie/flysystem-dropbox/tree/v1). ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/flysystem-dropbox.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/flysystem-dropbox) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation You can install the package via composer: ``` bash composer require spatie/flysystem-dropbox ``` ## Usage The first thing you need to do is to get an authorization token at Dropbox. A token can be generated in the [App Console](https://www.dropbox.com/developers/apps) for any Dropbox API app. You'll find more info at [the Dropbox Developer Blog](https://blogs.dropbox.com/developers/2014/05/generate-an-access-token-for-your-own-account/). ```php use League\Flysystem\Filesystem; use Spatie\Dropbox\Client; use Spatie\FlysystemDropbox\DropboxAdapter; $client = new Client($authorizationToken); $adapter = new DropboxAdapter($client); $filesystem = new Filesystem($adapter, ['case_sensitive' => false]); ``` Note: Because Dropbox is not case-sensitive you’ll need to set the 'case_sensitive' option to false. ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently. ## Testing ``` bash composer test ``` ## Contributing Please see [CONTRIBUTING](CONTRIBUTING.md) for details. ## Security If you discover any security related issues, please email freek@spatie.be instead of using the issue tracker. ## Postcardware You're free to use this package (it's [MIT-licensed](LICENSE.md)), but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/en/opensource/postcards). ## Credits - [Alex Vanderbist](https://github.com/AlexVanderbist) - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. flysystem-dropbox/.github/CONTRIBUTING.md 0000644 00000005634 15002154456 0014061 0 ustar 00 # Contributing Contributions are **welcome** and will be fully **credited**. Please read and understand the contribution guide before creating an issue or pull request. ## Etiquette This project is open source, and as such, the maintainers give their free time to build and maintain the source code held within. They make the code freely available in the hope that it will be of use to other developers. It would be extremely unfair for them to suffer abuse or anger for their hard work. Please be considerate towards maintainers when raising issues or presenting pull requests. Let's show the world that developers are civilized and selfless people. It's the duty of the maintainer to ensure that all submissions to the project are of sufficient quality to benefit the project. Many developers have different skillsets, strengths, and weaknesses. Respect the maintainer's decision, and do not be upset or abusive if your submission is not used. ## Viability When requesting or submitting new features, first consider whether it might be useful to others. Open source projects are used by many developers, who may have entirely different needs to your own. Think about whether or not your feature is likely to be used by other users of the project. ## Procedure Before filing an issue: - Attempt to replicate the problem, to ensure that it wasn't a coincidental incident. - Check to make sure your feature suggestion isn't already present within the project. - Check the pull requests tab to ensure that the bug doesn't have a fix in progress. - Check the pull requests tab to ensure that the feature isn't already in progress. Before submitting a pull request: - Check the codebase to ensure that your feature doesn't already exist. - Check the pull requests to ensure that another person hasn't already submitted the feature or fix. ## Requirements If the project maintainer has any additional requirements, you will find them listed here. - **[PSR-2 Coding Standard](https://github.com/php-fig/fig-standards/blob/master/accepted/PSR-2-coding-style-guide.md)** - The easiest way to apply the conventions is to install [PHP Code Sniffer](https://pear.php.net/package/PHP_CodeSniffer). - **Add tests!** - Your patch won't be accepted if it doesn't have tests. - **Document any change in behaviour** - Make sure the `README.md` and any other relevant documentation are kept up-to-date. - **Consider our release cycle** - We try to follow [SemVer v2.0.0](https://semver.org/). Randomly breaking public APIs is not an option. - **One pull request per feature** - If you want to do more than one thing, send multiple pull requests. - **Send coherent history** - Make sure each individual commit in your pull request is meaningful. If you had to make multiple intermediate commits while developing, please [squash them](https://www.git-scm.com/book/en/v2/Git-Tools-Rewriting-History#Changing-Multiple-Commit-Messages) before submitting. **Happy coding**! flysystem-dropbox/.github/ISSUE_TEMPLATE/config.yml 0000644 00000000501 15002154456 0015767 0 ustar 00 blank_issues_enabled: true contact_links: - name: Ask a Question url: https://github.com/spatie/ray/discussions/new?category=q-a about: Ask the community for help - name: Feature Request url: https://github.com/spatie/ray/discussions/new?category=ideas about: Share ideas for new features flysystem-dropbox/.github/SECURITY.md 0000644 00000000201 15002154456 0013402 0 ustar 00 # Security Policy If you discover any security related issues, please email freek@spatie.be instead of using the issue tracker. flysystem-dropbox/.github/workflows/run-tests.yml 0000644 00000002044 15002154456 0016344 0 ustar 00 name: Tests on: [push, pull_request] jobs: test: runs-on: ${{ matrix.os }} strategy: fail-fast: true matrix: os: [ubuntu-latest] php: [8.1, 8.0] dependency-version: [prefer-lowest, prefer-stable] name: P${{ matrix.php }} - ${{ matrix.dependency-version }} - ${{ matrix.os }} steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup PHP uses: shivammathur/setup-php@v2 with: php-version: ${{ matrix.php }} extensions: dom, curl, libxml, mbstring, zip, pcntl, pdo, sqlite, pdo_sqlite, bcmath, soap, intl, gd, exif, iconv, imagick coverage: none - name: Setup Problem Matches run: | echo "::add-matcher::${{ runner.tool_cache }}/php.json" echo "::add-matcher::${{ runner.tool_cache }}/phpunit.json" - name: Install dependencies run: composer update --${{ matrix.dependency-version }} --prefer-dist --no-interaction - name: Execute tests run: vendor/bin/phpunit flysystem-dropbox/.github/workflows/php-cs-fixer.yml 0000644 00000001000 15002154456 0016674 0 ustar 00 name: Check & fix styling on: [push] jobs: php-cs-fixer: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 with: ref: ${{ github.head_ref }} - name: Run PHP CS Fixer uses: docker://oskarstark/php-cs-fixer-ga with: args: --config=.php-cs-fixer.dist.php --allow-risky=yes - name: Commit changes uses: stefanzweifel/git-auto-commit-action@v4 with: commit_message: Fix styling flysystem-dropbox/.github/FUNDING.yml 0000644 00000000100 15002154456 0013424 0 ustar 00 github: spatie custom: https://spatie.be/open-source/support-us flysystem-dropbox/src/DropboxAdapter.php 0000644 00000021644 15002154456 0014505 0 ustar 00 <?php namespace Spatie\FlysystemDropbox; use League\Flysystem; use League\Flysystem\Config; use League\Flysystem\DirectoryAttributes; use League\Flysystem\FileAttributes; use League\Flysystem\FilesystemException; use League\Flysystem\PathPrefixer; use League\Flysystem\StorageAttributes; use League\Flysystem\UnableToCheckExistence; use League\Flysystem\UnableToCopyFile; use League\Flysystem\UnableToCreateDirectory; use League\Flysystem\UnableToDeleteFile; use League\Flysystem\UnableToMoveFile; use League\Flysystem\UnableToReadFile; use League\Flysystem\UnableToRetrieveMetadata; use League\Flysystem\UnableToSetVisibility; use League\Flysystem\UnableToWriteFile; use League\MimeTypeDetection\FinfoMimeTypeDetector; use League\MimeTypeDetection\MimeTypeDetector; use Spatie\Dropbox\Client; use Spatie\Dropbox\Exceptions\BadRequest; class DropboxAdapter implements Flysystem\FilesystemAdapter { /** @var \Spatie\Dropbox\Client */ protected $client; /** @var \League\Flysystem\PathPrefixer */ protected $prefixer; /** @var \League\MimeTypeDetection\MimeTypeDetector */ protected $mimeTypeDetector; public function __construct( Client $client, string $prefix = '', MimeTypeDetector $mimeTypeDetector = null ) { $this->client = $client; $this->prefixer = new PathPrefixer($prefix); $this->mimeTypeDetector = $mimeTypeDetector ?: new FinfoMimeTypeDetector(); } public function getClient(): Client { return $this->client; } public function fileExists(string $path): bool { $location = $this->applyPathPrefix($path); try { $this->client->getMetadata($location); return true; } catch (BadRequest $exception) { return false; } } public function directoryExists(string $path): bool { return $this->fileExists($path); } /** * @inheritDoc */ public function write(string $path, string $contents, Config $config): void { $location = $this->applyPathPrefix($path); try { $this->client->upload($location, $contents, 'overwrite'); } catch (BadRequest $e) { throw UnableToWriteFile::atLocation($location, $e->getMessage(), $e); } } /** * @inheritDoc */ public function writeStream(string $path, $contents, Config $config): void { $location = $this->applyPathPrefix($path); try { $this->client->upload($location, $contents, 'overwrite'); } catch (BadRequest $e) { throw UnableToWriteFile::atLocation($location, $e->getMessage(), $e); } } /** * @inheritDoc */ public function read(string $path): string { $object = $this->readStream($path); $contents = stream_get_contents($object); fclose($object); unset($object); return $contents; } /** * @inheritDoc */ public function readStream(string $path) { $location = $this->applyPathPrefix($path); try { $stream = $this->client->download($location); } catch (BadRequest $e) { throw UnableToReadFile::fromLocation($location, $e->getMessage(), $e); } return $stream; } /** * @inheritDoc */ public function delete(string $path): void { $location = $this->applyPathPrefix($path); try { $this->client->delete($location); } catch (BadRequest $e) { throw UnableToDeleteFile::atLocation($location, $e->getMessage(), $e); } } /** * @inheritDoc */ public function deleteDirectory(string $path): void { $location = $this->applyPathPrefix($path); try { $this->client->delete($location); } catch (UnableToDeleteFile $e) { throw Flysystem\UnableToDeleteDirectory::atLocation($location, $e->getPrevious()->getMessage(), $e); } } /** * @inheritDoc */ public function createDirectory(string $path, Config $config): void { $location = $this->applyPathPrefix($path); try { $this->client->createFolder($location); } catch (BadRequest $e) { throw UnableToCreateDirectory::atLocation($location, $e->getMessage()); } } /** * @inheritDoc */ public function setVisibility(string $path, string $visibility): void { throw UnableToSetVisibility::atLocation($path, 'Adapter does not support visibility controls.'); } /** * @inheritDoc */ public function visibility(string $path): FileAttributes { // Noop return new FileAttributes($path); } /** * @inheritDoc */ public function mimeType(string $path): FileAttributes { return new FileAttributes( $path, null, null, null, $this->mimeTypeDetector->detectMimeTypeFromPath($path) ); } /** * @inheritDoc */ public function lastModified(string $path): FileAttributes { $location = $this->applyPathPrefix($path); try { $response = $this->client->getMetadata($location); } catch (BadRequest $e) { throw UnableToRetrieveMetadata::lastModified($location, $e->getMessage()); } $timestamp = (isset($response['server_modified'])) ? strtotime($response['server_modified']) : null; return new FileAttributes( $path, null, null, $timestamp ); } /** * @inheritDoc */ public function fileSize(string $path): FileAttributes { $location = $this->applyPathPrefix($path); try { $response = $this->client->getMetadata($location); } catch (BadRequest $e) { throw UnableToRetrieveMetadata::lastModified($location, $e->getMessage()); } return new FileAttributes( $path, $response['size'] ?? null ); } /** * {@inheritDoc} */ public function listContents(string $path = '', bool $deep = false): iterable { foreach ($this->iterateFolderContents($path, $deep) as $entry) { $storageAttrs = $this->normalizeResponse($entry); // Avoid including the base directory itself if ($storageAttrs->isDir() && $storageAttrs->path() === $path) { continue; } yield $storageAttrs; } } protected function iterateFolderContents(string $path = '', bool $deep = false): \Generator { $location = $this->applyPathPrefix($path); try { $result = $this->client->listFolder($location, $deep); } catch (BadRequest $e) { return; } yield from $result['entries']; while ($result['has_more']) { $result = $this->client->listFolderContinue($result['cursor']); yield from $result['entries']; } } protected function normalizeResponse(array $response): StorageAttributes { $timestamp = (isset($response['server_modified'])) ? strtotime($response['server_modified']) : null; if ($response['.tag'] === 'folder') { $normalizedPath = ltrim($this->prefixer->stripDirectoryPrefix($response['path_display']), '/'); return new DirectoryAttributes( $normalizedPath, null, $timestamp ); } $normalizedPath = ltrim($this->prefixer->stripPrefix($response['path_display']), '/'); return new FileAttributes( $normalizedPath, $response['size'] ?? null, null, $timestamp, $this->mimeTypeDetector->detectMimeTypeFromPath($normalizedPath) ); } /** * @inheritDoc */ public function move(string $source, string $destination, Config $config): void { $path = $this->applyPathPrefix($source); $newPath = $this->applyPathPrefix($destination); try { $this->client->move($path, $newPath); } catch (BadRequest $e) { throw UnableToMoveFile::fromLocationTo($path, $newPath, $e); } } /** * @inheritDoc */ public function copy(string $source, string $destination, Config $config): void { $path = $this->applyPathPrefix($source); $newPath = $this->applyPathPrefix($destination); try { $this->client->copy($path, $newPath); } catch (BadRequest $e) { throw UnableToCopyFile::fromLocationTo($path, $newPath, $e); } } protected function applyPathPrefix($path): string { return '/'.trim($this->prefixer->prefixPath($path), '/'); } public function getUrl(string $path): string { return $this->client->getTemporaryLink($path); } } flysystem-dropbox/LICENSE.md 0000644 00000002102 15002154456 0011657 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. flysystem-dropbox/.php-cs-fixer.dist.php 0000644 00000002377 15002154456 0014327 0 ustar 00 <?php $finder = Symfony\Component\Finder\Finder::create() ->in([ __DIR__ . '/src', __DIR__ . '/tests', ]) ->name('*.php') ->notName('*.blade.php') ->ignoreDotFiles(true) ->ignoreVCS(true); return PhpCsFixer\Config::create() ->setRules([ '@PSR2' => true, 'array_syntax' => ['syntax' => 'short'], 'ordered_imports' => ['sortAlgorithm' => 'alpha'], 'no_unused_imports' => true, 'not_operator_with_successor_space' => true, 'trailing_comma_in_multiline_array' => true, 'phpdoc_scalar' => true, 'unary_operator_spaces' => true, 'binary_operator_spaces' => true, 'blank_line_before_statement' => [ 'statements' => ['break', 'continue', 'declare', 'return', 'throw', 'try'], ], 'phpdoc_single_line_var_spacing' => true, 'phpdoc_var_without_name' => true, 'class_attributes_separation' => [ 'elements' => [ 'method', ], ], 'method_argument_space' => [ 'on_multiline' => 'ensure_fully_multiline', 'keep_multiple_spaces_after_comma' => true, ], 'single_trait_insert_per_statement' => true, ]) ->setFinder($finder); flysystem-dropbox/CHANGELOG.md 0000644 00000002375 15002154456 0012100 0 ustar 00 # Changelog All notable changes to `flysystem-dropbox` will be documented in this file ## 2.0.4 - 2021-04-26 - avoid listing the base directory itself in listContents calls (#73) ## 2.0.3 - 2021-04-25 - make listing a non-created directory not throw an exception (#72) ## 2.0.2 - 2021-03-31 - use generator in listContents call for upstream compliance (#66) ## 2.0.1 - 2021-03-31 - fix bugs discovered after real-world use (#63) ## 2.0.0 - 2021-03-28 - add support from Flysystem v2 ## 1.2.3 - 2020-12-27 - add support for PHP 8 ## 1.2.2 - 2019-12-04 - fix `createSharedLinkWithSettings` ## 1.2.1 - 2019-09-14 - fix minimum dep ## 1.2.0 - 2019-09-13 - add `getUrl` method ## 1.1.0 - 2019-05-31 - add `createSharedLinkWithSettings` ## 1.0.6 - 2017-11-18 - determine mimetype from filename ## 1.0.5 - 2017-10-21 - do not throw an exception when listing a non-existing directory ## 1.0.4 - 2017-10-19 - make sure all files are retrieved when calling `listContents` ## 1.0.3 - 2017-05-18 - reverts changes made in 1.0.2 ## 1.0.2 - 2017-05-18 - fix for files with different casings not showing up ## 1.0.1 - 2017-05-09 - add `size` key. `bytes` is deprecated and will be removed in the next major version. ## 1.0.0 - 2017-04-19 - initial release backtrace/composer.json 0000644 00000002644 15002154456 0011215 0 ustar 00 { "name": "spatie/backtrace", "description": "A better backtrace", "keywords": [ "spatie", "backtrace" ], "homepage": "https://github.com/spatie/backtrace", "license": "MIT", "authors": [ { "name": "Freek Van de Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^7.3|^8.0" }, "require-dev": { "ext-json": "*", "phpunit/phpunit": "^9.3", "spatie/phpunit-snapshot-assertions": "^4.2", "symfony/var-dumper": "^5.1" }, "autoload": { "psr-4": { "Spatie\\Backtrace\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\Backtrace\\Tests\\": "tests" } }, "scripts": { "psalm": "vendor/bin/psalm", "test": "vendor/bin/phpunit", "test-coverage": "vendor/bin/phpunit --coverage-html coverage", "format": "vendor/bin/php-cs-fixer fix --allow-risky=yes" }, "config": { "sort-packages": true }, "minimum-stability": "dev", "prefer-stable": true, "funding": [ { "type": "github", "url": "https://github.com/sponsors/spatie" }, { "type": "other", "url": "https://spatie.be/open-source/support-us" } ] } backtrace/README.md 0000644 00000014545 15002154456 0007755 0 ustar 00 # A better PHP backtrace [](https://packagist.org/packages/spatie/backtrace)  [](https://packagist.org/packages/spatie/backtrace) To get the backtrace in PHP you can use the `debug_backtrace` function. By default, it can be hard to work with. The reported function name for a frame is skewed: it belongs to the previous frame. Also, options need to be passed using a bitmask. This package provides a better way than `debug_backtrace` to work with a back trace. Here's an example: ```php // returns an array with `Spatie\Backtrace\Frame` instances $frames = Spatie\Backtrace\Backtrace::create()->frames(); $firstFrame = $frames[0]; $firstFrame->file; // returns the file name $firstFrame->lineNumber; // returns the line number $firstFrame->class; // returns the class name ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/backtrace.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/backtrace) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation You can install the package via composer: ```bash composer require spatie/backtrace ``` ## Usage This is how you can create a backtrace instance: ```php $backtrace = Spatie\Backtrace\Backtrace::create(); ``` ### Getting the frames To get all the frames you can call `frames`. ```php $frames = $backtrace->frames(); // contains an array with `Spatie\Backtrace\Frame` instances ``` A `Spatie\Backtrace\Frame` has these properties: - `file`: the name of the file - `lineNumber`: the line number - `arguments`: the arguments used for this frame. Will be `null` if `withArguments` was not used. - `class`: the class name for this frame. Will be `null` if the frame concerns a function. - `method`: the method used in this frame - `applicationFrame`: contains `true` is this frame belongs to your application, and `false` if it belongs to a file in the vendor directory ### Collecting arguments For performance reasons, the frames of the back trace will not contain the arguments of the called functions. If you want to add those use the `withArguments` method. ```php $backtrace = Spatie\Backtrace\Backtrace::create()->withArguments(); ``` #### Reducing arguments For viewing purposes, arguments can be reduced to a string: ```php $backtrace = Spatie\Backtrace\Backtrace::create()->withArguments()->reduceArguments(); ``` By default, some typical types will be reduced to a string. You can define your own reduction algorithm per type by implementing an `ArgumentReducer`: ```php class DateTimeWithOtherFormatArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof DateTimeInterface) { return UnReducedArgument::create(); } return new ReducedArgument( $argument->format('d/m/y H:i'), get_class($argument), ); } } ``` This is a copy of the built-in argument reducer for `DateTimeInterface` where we've updated the format. An `UnReducedArgument` object is returned when the argument is not of the expected type. A `ReducedArgument` object is returned with the reduced value of the argument and the original type of the argument. The reducer can be used as such: ```php $backtrace = Spatie\Backtrace\Backtrace::create()->withArguments()->reduceArguments( Spatie\Backtrace\Arguments\ArgumentReducers::default([ new DateTimeWithOtherFormatArgumentReducer() ]) ); ``` Which will first execute the new reducer and then the default ones. ### Setting the application path You can use the `applicationPath` to pass the base path of your app. This value will be used to determine whether a frame is an application frame, or a vendor frame. Here's an example using a Laravel specific function. ```php $backtrace = Spatie\Backtrace\Backtrace::create()->applicationPath(base_path()); ``` ### Getting a certain part of a trace If you only want to have the frames starting from a particular frame in the backtrace you can use the `startingFromFrame` method: ```php use Spatie\Backtrace\Backtrace; use Spatie\Backtrace\Frame; $frames = Backtrace::create() ->startingFromFrame(function (Frame $frame) { return $frame->class === MyClass::class; }) ->frames(); ``` With this code, all frames before the frame that concerns `MyClass` will have been filtered out. Alternatively, you can use the `offset` method, which will skip the given number of frames. In this example the first 2 frames will not end up in `$frames`. ```php $frames = Spatie\Backtrace\Backtrace::create() ->offset(2) ->frames(); ``` ### Limiting the number of frames To only get a specific number of frames use the `limit` function. In this example, we'll only get the first two frames. ```php $frames = Spatie\Backtrace\Backtrace::create() ->limit(2) ->frames(); ``` ### Getting a backtrace for a throwable Here's how you can get a backtrace for a throwable. ```php $frames = Spatie\Backtrace\Backtrace::createForThrowable($throwable) ``` Because we will use the backtrace that is already available the throwable, the frames will always contain the arguments used. ## Testing ``` bash composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Freek Van de Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. backtrace/src/Frame.php 0000644 00000003141 15002154456 0011016 0 ustar 00 <?php namespace Spatie\Backtrace; class Frame { /** @var string */ public $file; /** @var int */ public $lineNumber; /** @var array|null */ public $arguments = null; /** @var bool */ public $applicationFrame; /** @var string|null */ public $method; /** @var string|null */ public $class; public function __construct( string $file, int $lineNumber, ?array $arguments, string $method = null, string $class = null, bool $isApplicationFrame = false ) { $this->file = $file; $this->lineNumber = $lineNumber; $this->arguments = $arguments; $this->method = $method; $this->class = $class; $this->applicationFrame = $isApplicationFrame; } public function getSnippet(int $lineCount): array { return (new CodeSnippet()) ->surroundingLine($this->lineNumber) ->snippetLineCount($lineCount) ->get($this->file); } public function getSnippetAsString(int $lineCount): string { return (new CodeSnippet()) ->surroundingLine($this->lineNumber) ->snippetLineCount($lineCount) ->getAsString($this->file); } public function getSnippetProperties(int $lineCount): array { $snippet = $this->getSnippet($lineCount); return array_map(function (int $lineNumber) use ($snippet) { return [ 'line_number' => $lineNumber, 'text' => $snippet[$lineNumber], ]; }, array_keys($snippet)); } } backtrace/src/Backtrace.php 0000644 00000015103 15002154456 0011644 0 ustar 00 <?php namespace Spatie\Backtrace; use Closure; use Spatie\Backtrace\Arguments\ArgumentReducers; use Spatie\Backtrace\Arguments\ReduceArgumentsAction; use Spatie\Backtrace\Arguments\Reducers\ArgumentReducer; use Throwable; class Backtrace { /** @var bool */ protected $withArguments = false; /** @var bool */ protected $reduceArguments = false; /** @var array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null */ protected $argumentReducers = null; /** @var bool */ protected $withObject = false; /** @var string|null */ protected $applicationPath; /** @var int */ protected $offset = 0; /** @var int */ protected $limit = 0; /** @var \Closure|null */ protected $startingFromFrameClosure = null; /** @var \Throwable|null */ protected $throwable = null; public static function create(): self { return new static(); } public static function createForThrowable(Throwable $throwable): self { return (new static())->forThrowable($throwable); } protected function forThrowable(Throwable $throwable): self { $this->throwable = $throwable; return $this; } public function withArguments( bool $withArguments = true ): self { $this->withArguments = $withArguments; return $this; } /** * @param array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null $argumentReducers * * @return $this */ public function reduceArguments( $argumentReducers = null ): self { $this->reduceArguments = true; $this->argumentReducers = $argumentReducers; return $this; } public function withObject(): self { $this->withObject = true; return $this; } public function applicationPath(string $applicationPath): self { $this->applicationPath = rtrim($applicationPath, '/'); return $this; } public function offset(int $offset): self { $this->offset = $offset; return $this; } public function limit(int $limit): self { $this->limit = $limit; return $this; } public function startingFromFrame(Closure $startingFromFrameClosure) { $this->startingFromFrameClosure = $startingFromFrameClosure; return $this; } /** * @return \Spatie\Backtrace\Frame[] */ public function frames(): array { $rawFrames = $this->getRawFrames(); return $this->toFrameObjects($rawFrames); } public function firstApplicationFrameIndex(): ?int { foreach ($this->frames() as $index => $frame) { if ($frame->applicationFrame) { return $index; } } return null; } protected function getRawFrames(): array { if ($this->throwable) { return $this->throwable->getTrace(); } $options = null; if (! $this->withArguments) { $options = $options | DEBUG_BACKTRACE_IGNORE_ARGS; } if ($this->withObject()) { $options = $options | DEBUG_BACKTRACE_PROVIDE_OBJECT; } $limit = $this->limit; if ($limit !== 0) { $limit += 3; } return debug_backtrace($options, $limit); } /** * @return \Spatie\Backtrace\Frame[] */ protected function toFrameObjects(array $rawFrames): array { $currentFile = $this->throwable ? $this->throwable->getFile() : ''; $currentLine = $this->throwable ? $this->throwable->getLine() : 0; $arguments = $this->withArguments ? [] : null; $frames = []; $reduceArgumentsAction = new ReduceArgumentsAction($this->resolveArgumentReducers()); foreach ($rawFrames as $rawFrame) { $frames[] = new Frame( $currentFile, $currentLine, $arguments, $rawFrame['function'] ?? null, $rawFrame['class'] ?? null, $this->isApplicationFrame($currentFile) ); $arguments = $this->withArguments ? $rawFrame['args'] ?? null : null; if ($this->reduceArguments) { $arguments = $reduceArgumentsAction->execute( $rawFrame['class'] ?? null, $rawFrame['function'] ?? null, $arguments ); } $currentFile = $rawFrame['file'] ?? 'unknown'; $currentLine = $rawFrame['line'] ?? 0; } $frames[] = new Frame( $currentFile, $currentLine, [], '[top]' ); $frames = $this->removeBacktracePackageFrames($frames); if ($closure = $this->startingFromFrameClosure) { $frames = $this->startAtFrameFromClosure($frames, $closure); } $frames = array_slice($frames, $this->offset, $this->limit === 0 ? PHP_INT_MAX : $this->limit); return array_values($frames); } protected function isApplicationFrame(string $frameFilename): bool { $relativeFile = str_replace('\\', DIRECTORY_SEPARATOR, $frameFilename); if (! empty($this->applicationPath)) { $relativeFile = array_reverse(explode($this->applicationPath ?? '', $frameFilename, 2))[0]; } if (strpos($relativeFile, DIRECTORY_SEPARATOR.'vendor') === 0) { return false; } return true; } protected function removeBacktracePackageFrames(array $frames): array { return $this->startAtFrameFromClosure($frames, function (Frame $frame) { return $frame->class !== static::class; }); } /** * @param \Spatie\Backtrace\Frame[] $frames * @param \Closure $closure * * @return array */ protected function startAtFrameFromClosure(array $frames, Closure $closure): array { foreach ($frames as $i => $frame) { $foundStartingFrame = $closure($frame); if ($foundStartingFrame) { return $frames; } unset($frames[$i]); } return $frames; } protected function resolveArgumentReducers(): ArgumentReducers { if ($this->argumentReducers === null) { return ArgumentReducers::default(); } if ($this->argumentReducers instanceof ArgumentReducers) { return $this->argumentReducers; } return ArgumentReducers::create($this->argumentReducers); } } backtrace/src/CodeSnippet.php 0000644 00000003777 15002154456 0012220 0 ustar 00 <?php namespace Spatie\Backtrace; use RuntimeException; class CodeSnippet { /** @var int */ protected $surroundingLine = 1; /** @var int */ protected $snippetLineCount = 9; public function surroundingLine(int $surroundingLine): self { $this->surroundingLine = $surroundingLine; return $this; } public function snippetLineCount(int $snippetLineCount): self { $this->snippetLineCount = $snippetLineCount; return $this; } public function get(string $fileName): array { if (! file_exists($fileName)) { return []; } try { $file = new File($fileName); [$startLineNumber, $endLineNumber] = $this->getBounds($file->numberOfLines()); $code = []; $line = $file->getLine($startLineNumber); $currentLineNumber = $startLineNumber; while ($currentLineNumber <= $endLineNumber) { $code[$currentLineNumber] = rtrim(substr($line, 0, 250)); $line = $file->getNextLine(); $currentLineNumber++; } return $code; } catch (RuntimeException $exception) { return []; } } public function getAsString(string $fileName): string { $snippet = $this->get($fileName); $snippetStrings = array_map(function (string $line, string $number) { return "{$number} {$line}"; }, $snippet, array_keys($snippet)); return implode(PHP_EOL, $snippetStrings); } protected function getBounds(int $totalNumberOfLineInFile): array { $startLine = max($this->surroundingLine - floor($this->snippetLineCount / 2), 1); $endLine = $startLine + ($this->snippetLineCount - 1); if ($endLine > $totalNumberOfLineInFile) { $endLine = $totalNumberOfLineInFile; $startLine = max($endLine - ($this->snippetLineCount - 1), 1); } return [$startLine, $endLine]; } } backtrace/src/Arguments/ProvidedArgument.php 0000644 00000006111 15002154456 0015210 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments; use ReflectionParameter; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\TruncatedReducedArgument; class ProvidedArgument { /** @var string */ public $name; /** @var bool */ public $passedByReference = false; /** @var bool */ public $isVariadic = false; /** @var bool */ public $hasDefaultValue = false; /** @var mixed */ public $defaultValue = null; /** @var bool */ public $defaultValueUsed = false; /** @var bool */ public $truncated = false; /** @var mixed */ public $reducedValue = null; /** @var string|null */ public $originalType = null; public static function fromReflectionParameter(ReflectionParameter $parameter): self { return new self( $parameter->getName(), $parameter->isPassedByReference(), $parameter->isVariadic(), $parameter->isDefaultValueAvailable(), $parameter->isDefaultValueAvailable() ? $parameter->getDefaultValue() : null, ); } public static function fromNonReflectableParameter( int $index ): self { return new self( "arg{$index}", false, ); } public function __construct( string $name, bool $passedByReference = false, bool $isVariadic = false, bool $hasDefaultValue = false, $defaultValue = null, bool $defaultValueUsed = false, bool $truncated = false, $reducedValue = null, ?string $originalType = null ) { $this->originalType = $originalType; $this->reducedValue = $reducedValue; $this->truncated = $truncated; $this->defaultValueUsed = $defaultValueUsed; $this->defaultValue = $defaultValue; $this->hasDefaultValue = $hasDefaultValue; $this->isVariadic = $isVariadic; $this->passedByReference = $passedByReference; $this->name = $name; if ($this->isVariadic) { $this->defaultValue = []; } } public function setReducedArgument( ReducedArgument $reducedArgument ): self { $this->reducedValue = $reducedArgument->value; $this->originalType = $reducedArgument->originalType; if ($reducedArgument instanceof TruncatedReducedArgument) { $this->truncated = true; } return $this; } public function defaultValueUsed(): self { $this->defaultValueUsed = true; $this->originalType = get_debug_type($this->defaultValue); return $this; } public function toArray(): array { return [ 'name' => $this->name, 'value' => $this->defaultValueUsed ? $this->defaultValue : $this->reducedValue, 'original_type' => $this->originalType, 'passed_by_reference' => $this->passedByReference, 'is_variadic' => $this->isVariadic, 'truncated' => $this->truncated, ]; } } backtrace/src/Arguments/ArgumentReducers.php 0000644 00000005444 15002154456 0015220 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments; use Spatie\Backtrace\Arguments\Reducers\ArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\ArrayArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\BaseTypeArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\ClosureArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\DateTimeArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\DateTimeZoneArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\EnumArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\MinimalArrayArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\SensitiveParameterArrayReducer; use Spatie\Backtrace\Arguments\Reducers\StdClassArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\StringableArgumentReducer; use Spatie\Backtrace\Arguments\Reducers\SymphonyRequestArgumentReducer; class ArgumentReducers { /** @var array<int, ArgumentReducer> */ public $argumentReducers = []; /** * @param array<ArgumentReducer|class-string<ArgumentReducer>> $argumentReducers */ public static function create(array $argumentReducers): self { return new self(array_map( function ($argumentReducer) { /** @var $argumentReducer ArgumentReducer|class-string<ArgumentReducer> */ return $argumentReducer instanceof ArgumentReducer ? $argumentReducer : new $argumentReducer(); }, $argumentReducers )); } public static function default(array $extra = []): self { return new self(static::defaultReducers($extra)); } public static function minimal(array $extra = []): self { return new self(static::minimalReducers($extra)); } /** * @param array<int, ArgumentReducer> $argumentReducers */ protected function __construct(array $argumentReducers) { $this->argumentReducers = $argumentReducers; } protected static function defaultReducers(array $extra = []): array { return array_merge($extra, [ new BaseTypeArgumentReducer(), new ArrayArgumentReducer(), new StdClassArgumentReducer(), new EnumArgumentReducer(), new ClosureArgumentReducer(), new SensitiveParameterArrayReducer(), new DateTimeArgumentReducer(), new DateTimeZoneArgumentReducer(), new SymphonyRequestArgumentReducer(), new StringableArgumentReducer(), ]); } protected static function minimalReducers(array $extra = []): array { return array_merge($extra, [ new BaseTypeArgumentReducer(), new MinimalArrayArgumentReducer(), new EnumArgumentReducer(), new ClosureArgumentReducer(), new SensitiveParameterArrayReducer(), ]); } } backtrace/src/Arguments/ReduceArgumentPayloadAction.php 0000644 00000002266 15002154456 0017322 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; class ReduceArgumentPayloadAction { /** @var \Spatie\Backtrace\Arguments\ArgumentReducers */ protected $argumentReducers; public function __construct( ArgumentReducers $argumentReducers ) { $this->argumentReducers = $argumentReducers; } public function reduce($argument, bool $includeObjectType = false): ReducedArgument { foreach ($this->argumentReducers->argumentReducers as $reducer) { $reduced = $reducer->execute($argument); if ($reduced instanceof ReducedArgument) { return $reduced; } } if (gettype($argument) === 'object' && $includeObjectType) { return new ReducedArgument( 'object ('.get_class($argument).')', get_debug_type($argument), ); } if (gettype($argument) === 'object') { return new ReducedArgument('object', get_debug_type($argument), ); } return new ReducedArgument( $argument, get_debug_type($argument), ); } } backtrace/src/Arguments/ReducedArgument/ReducedArgumentContract.php 0000644 00000000145 15002154456 0021564 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\ReducedArgument; interface ReducedArgumentContract { } backtrace/src/Arguments/ReducedArgument/TruncatedReducedArgument.php 0000644 00000000172 15002154456 0021740 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\ReducedArgument; class TruncatedReducedArgument extends ReducedArgument { } backtrace/src/Arguments/ReducedArgument/ReducedArgument.php 0000644 00000000652 15002154456 0020071 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\ReducedArgument; class ReducedArgument implements ReducedArgumentContract { /** @var mixed */ public $value; /** @var string */ public $originalType; /** * @param mixed $value */ public function __construct( $value, string $originalType ) { $this->originalType = $originalType; $this->value = $value; } } backtrace/src/Arguments/ReducedArgument/UnReducedArgument.php 0000644 00000000647 15002154456 0020400 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\ReducedArgument; class UnReducedArgument implements ReducedArgumentContract { /** @var self|null */ private static $instance = null; private function __construct() { } public static function create(): self { if (self::$instance !== null) { return self::$instance; } return self::$instance = new self(); } } backtrace/src/Arguments/ReducedArgument/VariadicReducedArgument.php 0000644 00000000771 15002154456 0021536 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\ReducedArgument; use Exception; class VariadicReducedArgument extends ReducedArgument { public function __construct(array $value) { foreach ($value as $key => $item) { if (! $item instanceof ReducedArgument) { throw new Exception('VariadicReducedArgument must be an array of ReducedArgument'); } $value[$key] = $item->value; } parent::__construct($value, 'array'); } } backtrace/src/Arguments/ReduceArgumentsAction.php 0000644 00000007234 15002154456 0016173 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments; use ReflectionException; use ReflectionFunction; use ReflectionMethod; use ReflectionParameter; use Spatie\Backtrace\Arguments\ReducedArgument\VariadicReducedArgument; use Throwable; class ReduceArgumentsAction { /** @var ArgumentReducers */ protected $argumentReducers; /** @var ReduceArgumentPayloadAction */ protected $reduceArgumentPayloadAction; public function __construct( ArgumentReducers $argumentReducers ) { $this->argumentReducers = $argumentReducers; $this->reduceArgumentPayloadAction = new ReduceArgumentPayloadAction($argumentReducers); } public function execute( ?string $class, ?string $method, ?array $frameArguments ): ?array { try { if ($frameArguments === null) { return null; } $parameters = $this->getParameters($class, $method); if ($parameters === null) { $arguments = []; foreach ($frameArguments as $index => $argument) { $arguments[$index] = ProvidedArgument::fromNonReflectableParameter($index) ->setReducedArgument($this->reduceArgumentPayloadAction->reduce($argument)) ->toArray(); } return $arguments; } $arguments = array_map( function ($argument) { return $this->reduceArgumentPayloadAction->reduce($argument); }, $frameArguments, ); $argumentsCount = count($arguments); $hasVariadicParameter = false; foreach ($parameters as $index => $parameter) { if ($index + 1 > $argumentsCount) { $parameter->defaultValueUsed(); } elseif ($parameter->isVariadic) { $parameter->setReducedArgument(new VariadicReducedArgument(array_slice($arguments, $index))); $hasVariadicParameter = true; } else { $parameter->setReducedArgument($arguments[$index]); } $parameters[$index] = $parameter->toArray(); } if ($this->moreArgumentsProvidedThanParameters($arguments, $parameters, $hasVariadicParameter)) { for ($i = count($parameters); $i < count($arguments); $i++) { $parameters[$i] = ProvidedArgument::fromNonReflectableParameter(count($parameters)) ->setReducedArgument($arguments[$i]) ->toArray(); } } return $parameters; } catch (Throwable $e) { return null; } } /** @return null|Array<\Spatie\Backtrace\Arguments\ProvidedArgument> */ protected function getParameters( ?string $class, ?string $method ): ?array { try { $reflection = $class !== null ? new ReflectionMethod($class, $method) : new ReflectionFunction($method); } catch (ReflectionException $e) { return null; } return array_map( function (ReflectionParameter $reflectionParameter) { return ProvidedArgument::fromReflectionParameter($reflectionParameter); }, $reflection->getParameters(), ); } protected function moreArgumentsProvidedThanParameters( array $arguments, array $parameters, bool $hasVariadicParameter ): bool { return count($arguments) > count($parameters) && ! $hasVariadicParameter; } } backtrace/src/Arguments/Reducers/StringableArgumentReducer.php 0000644 00000001172 15002154456 0020616 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; use Stringable; class StringableArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof Stringable) { return UnReducedArgument::create(); } return new ReducedArgument( (string) $argument, get_class($argument), ); } } backtrace/src/Arguments/Reducers/SensitiveParameterArrayReducer.php 0000644 00000001312 15002154456 0021626 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use SensitiveParameterValue; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class SensitiveParameterArrayReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof SensitiveParameterValue) { return UnReducedArgument::create(); } return new ReducedArgument( 'SensitiveParameterValue('.get_debug_type($argument->getValue()).')', get_class($argument) ); } } backtrace/src/Arguments/Reducers/MinimalArrayArgumentReducer.php 0000644 00000001142 15002154456 0021106 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class MinimalArrayArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if(! is_array($argument)) { return UnReducedArgument::create(); } return new ReducedArgument( 'array (size='.count($argument).')', 'array' ); } } backtrace/src/Arguments/Reducers/DateTimeArgumentReducer.php 0000644 00000001226 15002154456 0020220 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use DateTimeInterface; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class DateTimeArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof DateTimeInterface) { return UnReducedArgument::create(); } return new ReducedArgument( $argument->format('d M Y H:i:s e'), get_class($argument), ); } } backtrace/src/Arguments/Reducers/SymphonyRequestArgumentReducer.php 0000644 00000001267 15002154456 0021730 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; use Symfony\Component\HttpFoundation\Request; class SymphonyRequestArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if(! $argument instanceof Request) { return UnReducedArgument::create(); } return new ReducedArgument( "{$argument->getMethod()} {$argument->getUri()}", get_class($argument), ); } } backtrace/src/Arguments/Reducers/BaseTypeArgumentReducer.php 0000644 00000001305 15002154456 0020236 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class BaseTypeArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (is_int($argument) || is_float($argument) || is_bool($argument) || is_string($argument) || $argument === null ) { return new ReducedArgument($argument, get_debug_type($argument)); } return UnReducedArgument::create(); } } backtrace/src/Arguments/Reducers/ArrayArgumentReducer.php 0000644 00000003170 15002154456 0017602 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ArgumentReducers; use Spatie\Backtrace\Arguments\ReduceArgumentPayloadAction; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\TruncatedReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class ArrayArgumentReducer implements ReducedArgumentContract { /** @var int */ protected $maxArraySize = 25; /** @var \Spatie\Backtrace\Arguments\ReduceArgumentPayloadAction */ protected $reduceArgumentPayloadAction; public function __construct() { $this->reduceArgumentPayloadAction = new ReduceArgumentPayloadAction(ArgumentReducers::minimal()); } public function execute($argument): ReducedArgumentContract { if (! is_array($argument)) { return UnReducedArgument::create(); } return $this->reduceArgument($argument, 'array'); } protected function reduceArgument(array $argument, string $originalType): ReducedArgumentContract { foreach ($argument as $key => $value) { $argument[$key] = $this->reduceArgumentPayloadAction->reduce( $value, true )->value; } if (count($argument) > $this->maxArraySize) { return new TruncatedReducedArgument( array_slice($argument, 0, $this->maxArraySize), 'array' ); } return new ReducedArgument($argument, $originalType); } } backtrace/src/Arguments/Reducers/ClosureArgumentReducer.php 0000644 00000001715 15002154456 0020143 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Closure; use ReflectionFunction; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class ClosureArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof Closure) { return UnReducedArgument::create(); } $reflection = new ReflectionFunction($argument); if ($reflection->getFileName() && $reflection->getStartLine() && $reflection->getEndLine()) { return new ReducedArgument( "{$reflection->getFileName()}:{$reflection->getStartLine()}-{$reflection->getEndLine()}", 'Closure' ); } return new ReducedArgument("{$reflection->getFileName()}", 'Closure'); } } backtrace/src/Arguments/Reducers/StdClassArgumentReducer.php 0000644 00000001020 15002154456 0020234 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; use stdClass; class StdClassArgumentReducer extends ArrayArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof stdClass) { return UnReducedArgument::create(); } return parent::reduceArgument((array) $argument, stdClass::class); } } backtrace/src/Arguments/Reducers/ArgumentReducer.php 0000644 00000000415 15002154456 0016602 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; interface ArgumentReducer { /** * @param mixed $argument */ public function execute($argument): ReducedArgumentContract; } backtrace/src/Arguments/Reducers/DateTimeZoneArgumentReducer.php 0000644 00000001202 15002154456 0021046 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use DateTimeZone; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; class DateTimeZoneArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof DateTimeZone) { return UnReducedArgument::create(); } return new ReducedArgument( $argument->getName(), get_class($argument), ); } } backtrace/src/Arguments/Reducers/EnumArgumentReducer.php 0000644 00000001207 15002154456 0017427 0 ustar 00 <?php namespace Spatie\Backtrace\Arguments\Reducers; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgument; use Spatie\Backtrace\Arguments\ReducedArgument\ReducedArgumentContract; use Spatie\Backtrace\Arguments\ReducedArgument\UnReducedArgument; use UnitEnum; class EnumArgumentReducer implements ArgumentReducer { public function execute($argument): ReducedArgumentContract { if (! $argument instanceof UnitEnum) { return UnReducedArgument::create(); } return new ReducedArgument( get_class($argument).'::'.$argument->name, get_class($argument), ); } } backtrace/src/File.php 0000644 00000001335 15002154456 0010646 0 ustar 00 <?php namespace Spatie\Backtrace; use SplFileObject; class File { /** @var \SplFileObject */ protected $file; public function __construct(string $path) { $this->file = new SplFileObject($path); } public function numberOfLines(): int { $this->file->seek(PHP_INT_MAX); return $this->file->key() + 1; } public function getLine(int $lineNumber = null): string { if (is_null($lineNumber)) { return $this->getNextLine(); } $this->file->seek($lineNumber - 1); return $this->file->current(); } public function getNextLine(): string { $this->file->next(); return $this->file->current(); } } backtrace/LICENSE.md 0000644 00000002102 15002154456 0010064 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-signal-aware-command/composer.json 0000644 00000003371 15002154456 0014706 0 ustar 00 { "name": "spatie/laravel-signal-aware-command", "description": "Handle signals in artisan commands", "keywords": [ "spatie", "laravel", "laravel-signal-aware-command" ], "homepage": "https://github.com/spatie/laravel-signal-aware-command", "license": "MIT", "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "role": "Developer" } ], "require": { "php": "^8.0", "spatie/laravel-package-tools": "^1.4.3", "illuminate/contracts": "^8.35|^9.0|^10.0" }, "require-dev": { "ext-pcntl": "*", "brianium/paratest": "^6.2", "nunomaduro/collision": "^5.3|^6.0", "orchestra/testbench": "^6.16|^7.0|^8.0", "pestphp/pest-plugin-laravel": "^1.3", "phpunit/phpunit": "^9.5", "spatie/laravel-ray": "^1.17" }, "autoload": { "psr-4": { "Spatie\\SignalAwareCommand\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\SignalAwareCommand\\Tests\\": "tests" } }, "scripts": { "psalm": "vendor/bin/psalm", "test": "./vendor/bin/pest", "test-coverage": "vendor/bin/pest --coverage-html coverage" }, "config": { "sort-packages": true, "allow-plugins": { "pestphp/pest-plugin": true } }, "extra": { "laravel": { "providers": [ "Spatie\\SignalAwareCommand\\SignalAwareCommandServiceProvider" ], "aliases": { "Signal": "Spatie\\SignalAwareCommand\\Facades\\Signal" } } }, "minimum-stability": "dev", "prefer-stable": true } laravel-signal-aware-command/README.md 0000644 00000015544 15002154456 0013450 0 ustar 00 [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/support-ukraine.svg?t=1" />](https://supportukrainenow.org) # Handle signals in Artisan commands [](https://packagist.org/packages/spatie/laravel-signal-aware-command) [](https://github.com/spatie/laravel-signal-aware-command/actions?query=workflow%3ATests+branch%3Amain) [](https://github.com/spatie/laravel-signal-aware-command/actions?query=workflow%3A"Check+%26+fix+styling"+branch%3Amain) [](https://packagist.org/packages/spatie/laravel-signal-aware-command) Using this package you can easily handle signals like `SIGINT`, `SIGTERM` in your Laravel app. Here's a quick example where the `SIGINT` signal is handled. ```php use Spatie\SignalAwareCommand\SignalAwareCommand; class YourCommand extends SignalAwareCommand { protected $signature = 'your-command'; public function handle() { $this->info('Command started...'); sleep(100); } public function onSigint() { // will be executed when you stop the command $this->info('You stopped the command!'); } } ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/laravel-signal-aware-command.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/package-laravel-signal-aware-command-laravel) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation You can install the package via composer: ```bash composer require spatie/laravel-signal-aware-command ``` ## Usage In order to make an Artisan command signal aware you need to let it extend `SignalAwareCommand`. ```php use Spatie\SignalAwareCommand\SignalAwareCommand; class YourCommand extends SignalAwareCommand { // your code } ``` ### Handling signals There are three ways to handle signals: - on the command itself - via the `Signal` facade - using the `SignalReceived` event #### On the command To handle signals on the command itself, you need to let your command extend `SignalAwareCommand`. Next, define a method that starts with `on` followed by the name of the signal. Here's an example where the `SIGINT` signal is handled. ```php use Spatie\SignalAwareCommand\SignalAwareCommand; class YourCommand extends SignalAwareCommand { protected $signature = 'your-command'; public function handle() { $this->info('Command started...'); sleep(100); } public function onSigint() { // will be executed when you stop the command $this->info('You stopped the command!'); } } ``` #### Via the `Signal` facade Using the `Signal` facade you can register signal handling code anywhere in your app. First, you need to define the signals you want to handle in your command in the `handlesSignals` property. ```php use Spatie\SignalAwareCommand\SignalAwareCommand; class YourCommand extends SignalAwareCommand { protected $signature = 'your-command'; protected $handlesSignals = [SIGINT]; public function handle() { (new SomeOtherClass())->performSomeWork(); sleep(100); } } ``` In any class you'd like you can use the `Signal` facade to register code that should be executed when a signal is received. ```php use Illuminate\Console\Command; use Spatie\SignalAwareCommand\Facades\Signal; class SomeOtherClass { public function performSomeWork() { Signal::handle(SIGINT, function(Command $commandThatReceivedSignal) { $commandThatReceivedSignal->info('Received the SIGINT signal!'); }) } } ``` You can call `clearHandlers` if you want to remove a handler that was previously registered. ```php use Spatie\SignalAwareCommand\Facades\Signal; public function performSomeWork() { Signal::handle(SIGNINT, function() { // perform cleanup }); $this->doSomeWork(); // at this point doSomeWork was executed without any problems // running a cleanup isn't necessary anymore Signal::clearHandlers(SIGINT); } ``` To clear all handlers for all signals use `Signal::clearHandlers()`. #### Using the `SignalReceived` event Whenever a signal is received, the `Spatie\SignalAwareCommand\Events\SignalReceived` event is fired. To register which events you want to receive you must define a `handlesSignals` property on your command. Here's an example where we register listening for the `SIGINT` signal. ```php use Spatie\SignalAwareCommand\SignalAwareCommand class YourCommand extends SignalAwareCommand { protected $signature = 'your-command'; protected $handlesSignals = [SIGINT]; public function handle() { (new SomeOtherClass())->performSomeWork(); sleep(100); } } ``` In any class you'd like you can listen for the `SignalReceived` event. ```php use Spatie\SignalAwareCommand\Events\SignalReceived; use Spatie\SignalAwareCommand\Signals; class SomeOtherClass { public function performSomeWork() { Event::listen(function(SignalReceived $event) { $signalNumber = $event->signal; $signalName = Signals::getSignalName($signalNumber); $event->command->info("Received the {$signalName} signal"); }); } } ``` ## Learn how this package was built The foundations of this pacakge were coded up in [this live stream on YouTube](https://www.youtube.com/watch?v=D9hxQoD47jI). ## Testing ```bash composer test ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. laravel-signal-aware-command/.php_cs.dist.php 0000644 00000002541 15002154456 0015167 0 ustar 00 <?php $finder = Symfony\Component\Finder\Finder::create() ->notPath('bootstrap/*') ->notPath('storage/*') ->notPath('resources/view/mail/*') ->in([ __DIR__ . '/src', __DIR__ . '/tests', ]) ->name('*.php') ->notName('*.blade.php') ->ignoreDotFiles(true) ->ignoreVCS(true); return (new PhpCsFixer\Config()) ->setRules([ '@PSR12' => true, 'array_syntax' => ['syntax' => 'short'], 'ordered_imports' => ['sort_algorithm' => 'alpha'], 'no_unused_imports' => true, 'not_operator_with_successor_space' => true, 'trailing_comma_in_multiline' => true, 'phpdoc_scalar' => true, 'unary_operator_spaces' => true, 'binary_operator_spaces' => true, 'blank_line_before_statement' => [ 'statements' => ['break', 'continue', 'declare', 'return', 'throw', 'try'], ], 'phpdoc_single_line_var_spacing' => true, 'phpdoc_var_without_name' => true, 'class_attributes_separation' => [ 'elements' => [ 'method' => 'one', ], ], 'method_argument_space' => [ 'on_multiline' => 'ensure_fully_multiline', 'keep_multiple_spaces_after_comma' => true, ], 'single_trait_insert_per_statement' => true, ]) ->setFinder($finder); laravel-signal-aware-command/src/Signal.php 0000644 00000001500 15002154456 0014671 0 ustar 00 <?php namespace Spatie\SignalAwareCommand; use Illuminate\Console\Command; class Signal { protected array $registeredSignalHandlers = []; public function handle(int $signal, callable $callable): self { $this->registeredSignalHandlers[$signal][] = $callable; return $this; } public function executeSignalHandlers(int $signal, Command $command): self { foreach ($this->registeredSignalHandlers[$signal] ?? [] as $signalHandler) { $signalHandler($command); } return $this; } public function clearHandlers(int $signal = null): self { if (is_null($signal)) { $this->registeredSignalHandlers = []; return $this; } $this->registeredSignalHandlers[$signal] = []; return $this; } } laravel-signal-aware-command/src/Events/SignalReceived.php 0000644 00000000331 15002154456 0017605 0 ustar 00 <?php namespace Spatie\SignalAwareCommand\Events; use Illuminate\Console\Command; class SignalReceived { public function __construct( public int $signal, public Command $command ) { } } laravel-signal-aware-command/src/SignalAwareCommandServiceProvider.php 0000644 00000000672 15002154456 0022215 0 ustar 00 <?php namespace Spatie\SignalAwareCommand; use Spatie\LaravelPackageTools\Package; use Spatie\LaravelPackageTools\PackageServiceProvider; class SignalAwareCommandServiceProvider extends PackageServiceProvider { public function configurePackage(Package $package): void { $package->name('signal-aware-command'); $this->app->singleton(Signal::class, function () { return new Signal(); }); } } laravel-signal-aware-command/src/Signals.php 0000644 00000001674 15002154456 0015070 0 ustar 00 <?php namespace Spatie\SignalAwareCommand; use Illuminate\Support\Str; class Signals { public static function getSignalName(int $lookingForSignalNumber): ?string { foreach (get_defined_constants(true)['pcntl'] as $signalName => $signalNumber) { if ($signalNumber !== $lookingForSignalNumber) { continue; } if (Str::startsWith($signalName, 'SIG_')) { continue; } if (! Str::startsWith($signalName, 'SIG')) { continue; } return $signalName; } return null; } public static function getSignalForName(string $lookingForSignalName): ?int { foreach (get_defined_constants(true)['pcntl'] as $signalName => $signalNumber) { if ($signalName === $lookingForSignalName) { return $signalNumber; } } return null; } } laravel-signal-aware-command/src/SignalAwareCommand.php 0000644 00000003316 15002154456 0017157 0 ustar 00 <?php namespace Spatie\SignalAwareCommand; use Illuminate\Console\Command; use Illuminate\Support\Str; use Spatie\SignalAwareCommand\Events\SignalReceived; use Symfony\Component\Console\Command\SignalableCommandInterface; abstract class SignalAwareCommand extends Command implements SignalableCommandInterface { public function getSubscribedSignals(): array { return array_merge($this->autoDiscoverSignals(), $this->handlesSignals ?? []); } public function handleSignal(int $signal): void { event(new SignalReceived($signal, $this)); $this ->executeRegisteredSignalHandlers($signal) ->handleSignalMethodOnCommandClass($signal); } protected function executeRegisteredSignalHandlers(int $signal): self { app(Signal::class)->executeSignalHandlers($signal, $this); return $this; } protected function handleSignalMethodOnCommandClass(int $signal): self { if (! $signalName = Signals::getSignalName($signal)) { return $this; } $methodName = Str::camel("on {$signalName}"); if (! method_exists($this, $methodName)) { return $this; } $this->$methodName($signal); return $this; } protected function autoDiscoverSignals(): array { return collect(get_class_methods($this)) ->filter(fn (string $methodName) => Str::startsWith($methodName, 'on')) ->map(function (string $methodName) { $possibleSignalName = Str::of($methodName)->after('on')->upper(); return Signals::getSignalForName($possibleSignalName); }) ->filter() ->toArray(); } } laravel-signal-aware-command/src/Facades/Signal.php 0000644 00000000505 15002154456 0016223 0 ustar 00 <?php namespace Spatie\SignalAwareCommand\Facades; use Illuminate\Support\Facades\Facade; use Spatie\SignalAwareCommand\Signal as SignalClass; /** * @see \Spatie\SignalAwareCommand\Signal */ class Signal extends Facade { protected static function getFacadeAccessor() { return SignalClass::class; } } laravel-signal-aware-command/LICENSE.md 0000644 00000002076 15002154456 0013571 0 ustar 00 The MIT License (MIT) Copyright (c) spatie <freek@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. dropbox-api/composer.json 0000644 00000002566 15002154456 0011525 0 ustar 00 { "name": "spatie/dropbox-api", "description": "A minimal implementation of Dropbox API v2", "keywords": [ "spatie", "dropbox-api", "dropbox", "api", "v2" ], "homepage": "https://github.com/spatie/dropbox-api", "license": "MIT", "authors": [ { "name": "Alex Vanderbist", "email": "alex.vanderbist@gmail.com", "homepage": "https://spatie.be", "role": "Developer" }, { "name": "Freek Van der Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^8.1", "ext-json": "*", "graham-campbell/guzzle-factory": "^4.0.2|^5.0|^6.0|^7.0", "guzzlehttp/guzzle": "^6.2|^7.0" }, "require-dev": { "laravel/pint": "^1.10.1", "phpstan/phpstan": "^1.10.16", "phpunit/phpunit": "^9.4" }, "autoload": { "psr-4": { "Spatie\\Dropbox\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\Dropbox\\Test\\": "tests" } }, "scripts": { "test": "vendor/bin/phpunit", "pint": "vendor/bin/pint", "phpstan": "vendor/bin/phpstan analyze" }, "config": { "sort-packages": true } } dropbox-api/README.md 0000644 00000015030 15002154456 0010250 0 ustar 00 # A minimal implementation of Dropbox API v2 [](https://packagist.org/packages/spatie/dropbox-api) [](https://github.com/spatie/dropbox-api/actions?query=workflow%3ATests+branch%3Amaster) [](https://github.com/spatie/dropbox-api/actions?query=workflow%3A"Check+%26+fix+styling"+branch%3Amaster) [](https://packagist.org/packages/spatie/dropbox-api) This is a minimal PHP implementation of the [Dropbox API v2](https://www.dropbox.com/developers/documentation/http/overview). It contains only the methods needed for [our flysystem-dropbox adapter](https://github.com/spatie/flysystem-dropbox). We are open however to PRs that add extra methods to the client. Here are a few examples on how you can use the package: ```php $client = new Spatie\Dropbox\Client($authorizationToken); //create a folder $client->createFolder($path); //list a folder $client->listFolder($path); //get a temporary link $client->getTemporaryLink($path); ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/dropbox-api.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/dropbox-api) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Installation You can install the package via composer: ``` bash composer require spatie/dropbox-api ``` ## Usage The first thing you need to do is get an authorization token at Dropbox. Unlike [other companies](https://google.com) Dropbox has made this very easy. You can just generate a token in the [App Console](https://www.dropbox.com/developers/apps) for any Dropbox API app. You'll find more info at [the Dropbox Developer Blog](https://blogs.dropbox.com/developers/2014/05/generate-an-access-token-for-your-own-account/). With an authorization token you can instantiate a `Spatie\Dropbox\Client`. ```php $client = new Spatie\Dropbox\Client($authorizationToken); ``` or alternatively you can implement `Spatie\Dropbox\TokenProvider` which will provide the access-token from its `TokenProvider->getToken(): string` method. If you use oauth2 to authenticate and to acquire refresh-tokens and access-tokens, (like [thephpleague/oauth2-client](https://github.com/thephpleague/oauth2-client)), you can create an adapter that internally takes care of token-expiration and refreshing tokens, and at runtime will supply the access-token via the `TokenProvider->getToken(): string` method. *(Dropbox announced they will be moving to short-lived access_tokens mid 2021).* ```php // implements Spatie\Dropbox\TokenProvider $tokenProvider = new AutoRefreshingDropBoxTokenService($refreshToken); $client = new Spatie\Dropbox\Client($tokenProvider); ``` or alternatively you can authenticate as an App using your App Key & Secret. ```php $client = new Spatie\Dropbox\Client([$appKey, $appSecret]); ``` If you only need to access the public endpoints you can instantiate `Spatie\Dropbox\Client` without any arguments. ```php $client = new Spatie\Dropbox\Client(); ``` ## Dropbox Endpoints Look in [the source code of `Spatie\Dropbox\Client`](https://github.com/spatie/dropbox-api/blob/master/src/Client.php) to discover the methods you can use. Here's an example: ```php $content = 'hello, world'; $client->upload('/dropboxpath/filename.txt', $content, $mode='add'); $from = '/dropboxpath/somefile.txt'; $to = '/dropboxpath/archive/somefile.txt'; $client->move($from, $to); ``` If the destination filename already exists, dropbox will throw an Exception with 'to/conflict/file/..' The ``upload()`` and ``move()`` methods have an optional extra 'autorename' argument to try and let dropbox automatically rename the file if there is such a conflict. Here's an example: ```php $from = '/dropboxpath/somefile.txt'; $to = '/dropboxpath/archive/somefile.txt'; $client->move($from, $to, $autorename=true); // with autorename results in 'somefile (1).txt' ``` If you do not find your favorite method, you can directly use the `contentEndpointRequest` and `rpcEndpointRequest` functions. ```php public function contentEndpointRequest(string $endpoint, array $arguments, $body): ResponseInterface public function rpcEndpointRequest(string $endpoint, array $parameters): array ``` Here's an example: ```php $client->rpcEndpointRequest('search', ['path' => '', 'query' => 'bat cave']); ``` If you need to change the subdomain of the endpoint URL used in the API request, you can prefix the endpoint path with `subdomain::`. Here's an example: ```php $client->rpcEndpointRequest('content::files/get_thumbnail_batch', $parameters); ``` ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently. ## Testing ``` bash composer test ``` ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. You can run `composer pint` to run the code style fixer, and `composer phpstan` to run the static analysis. ## Security If you've found a bug regarding security please mail [security@spatie.be](mailto:security@spatie.be) instead of using the issue tracker. ## Postcardware You're free to use this package (it's [MIT-licensed](LICENSE.md)), but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/en/opensource/postcards). ## Credits - [Alex Vanderbist](https://github.com/AlexVanderbist) - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. dropbox-api/.github/ISSUE_TEMPLATE/config.yml 0000644 00000000720 15002154456 0014504 0 ustar 00 blank_issues_enabled: false contact_links: - name: Ask a question url: https://github.com/spatie/dropbox-api/discussions/new?category=q-a about: Ask the community for help - name: Request a feature url: https://github.com/spatie/dropbox-api/discussions/new?category=ideas about: Share ideas for new features - name: Report a bug url: https://github.com/spatie/dropbox-api/issues/new about: Report a reproducable bug dropbox-api/.github/dependabot.yml 0000644 00000000170 15002154456 0013160 0 ustar 00 version: 2 updates: - package-ecosystem: "github-actions" directory: "/" schedule: interval: "weekly" dropbox-api/.github/workflows/static-analysis.yml 0000644 00000001214 15002154456 0016220 0 ustar 00 name: Static Analysis on: [push, pull_request] jobs: phpstan: name: PHPStan (PHP ${{ matrix.php-version }}) runs-on: ubuntu-latest strategy: matrix: php-version: - '8.2' steps: - name: Checkout code uses: actions/checkout@v3 - name: Install PHP uses: shivammathur/setup-php@v2 with: coverage: none php-version: ${{ matrix.php-version }} tools: cs2pr - name: Install Composer dependencies uses: ramsey/composer-install@v2 - name: Run PHPStan run: 'vendor/bin/phpstan analyse --error-format=checkstyle | cs2pr' dropbox-api/.github/workflows/run-tests.yml 0000644 00000001546 15002154456 0015064 0 ustar 00 name: Tests on: [push, pull_request] jobs: test: runs-on: ubuntu-latest strategy: fail-fast: true matrix: php: [8.2, 8.1] stability: [lowest, highest] name: PHP ${{ matrix.php }} - ${{ matrix.stability }} deps steps: - name: Checkout code uses: actions/checkout@v3 - name: Setup PHP uses: shivammathur/setup-php@v2 with: php-version: ${{ matrix.php }} coverage: none - name: Setup problem matchers run: | echo "::add-matcher::${{ runner.tool_cache }}/php.json" echo "::add-matcher::${{ runner.tool_cache }}/phpunit.json" - name: Install dependencies uses: ramsey/composer-install@v2 with: dependency-versions: ${{ matrix.stability }} - name: Execute tests run: vendor/bin/phpunit dropbox-api/.github/workflows/code-style.yml 0000644 00000000772 15002154456 0015170 0 ustar 00 name: Check & fix styling on: push: paths: - '**.php' permissions: contents: write jobs: php-code-styling: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v3 with: ref: ${{ github.head_ref }} - name: Fix PHP code style issues uses: aglipanci/laravel-pint-action@2.3.0 - name: Commit changes uses: stefanzweifel/git-auto-commit-action@v4 with: commit_message: Fix styling dropbox-api/.github/workflows/dependabot-auto-merge.yml 0000644 00000002775 15002154456 0017275 0 ustar 00 name: dependabot-auto-merge on: pull_request_target permissions: pull-requests: write contents: write jobs: dependabot: runs-on: ubuntu-latest if: ${{ github.actor == 'dependabot[bot]' }} steps: - name: Dependabot metadata id: metadata uses: dependabot/fetch-metadata@v1.5.1 with: github-token: "${{ secrets.GITHUB_TOKEN }}" compat-lookup: true - name: Auto-merge Dependabot PRs for semver-minor updates if: ${{steps.metadata.outputs.update-type == 'version-update:semver-minor'}} run: gh pr merge --auto --merge "$PR_URL" env: PR_URL: ${{github.event.pull_request.html_url}} GITHUB_TOKEN: ${{secrets.GITHUB_TOKEN}} - name: Auto-merge Dependabot PRs for semver-patch updates if: ${{steps.metadata.outputs.update-type == 'version-update:semver-patch'}} run: gh pr merge --auto --merge "$PR_URL" env: PR_URL: ${{github.event.pull_request.html_url}} GITHUB_TOKEN: ${{secrets.GITHUB_TOKEN}} - name: Auto-merge Dependabot PRs for Action major versions when compatibility is higher than 90% if: ${{steps.metadata.outputs.package-ecosystem == 'github_actions' && steps.metadata.outputs.update-type == 'version-update:semver-major' && steps.metadata.outputs.compatibility-score >= 90}} run: gh pr merge --auto --merge "$PR_URL" env: PR_URL: ${{github.event.pull_request.html_url}} GH_TOKEN: ${{secrets.GITHUB_TOKEN}} dropbox-api/.github/workflows/update-changelog.yml 0000644 00000001205 15002154456 0016317 0 ustar 00 name: "Update Changelog" on: release: types: [released] jobs: update: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 with: ref: main - name: Update Changelog uses: stefanzweifel/changelog-updater-action@v1 with: latest-version: ${{ github.event.release.name }} release-notes: ${{ github.event.release.body }} - name: Commit updated CHANGELOG uses: stefanzweifel/git-auto-commit-action@v4 with: branch: main commit_message: Update CHANGELOG file_pattern: CHANGELOG.md dropbox-api/.github/FUNDING.yml 0000644 00000000100 15002154456 0012136 0 ustar 00 github: spatie custom: https://spatie.be/open-source/support-us dropbox-api/src/Exceptions/BadRequest.php 0000644 00000001035 15002154456 0014451 0 ustar 00 <?php namespace Spatie\Dropbox\Exceptions; use Exception; use Psr\Http\Message\ResponseInterface; class BadRequest extends Exception { public ?string $dropboxCode = null; public function __construct(public ResponseInterface $response) { $body = json_decode($response->getBody(), true); if ($body !== null) { if (isset($body['error']['.tag'])) { $this->dropboxCode = $body['error']['.tag']; } parent::__construct($body['error_summary']); } } } dropbox-api/src/InMemoryTokenProvider.php 0000644 00000000361 15002154456 0014545 0 ustar 00 <?php namespace Spatie\Dropbox; class InMemoryTokenProvider implements TokenProvider { public function __construct(protected string $token) { } public function getToken(): string { return $this->token; } } dropbox-api/src/Client.php 0000644 00000060604 15002154456 0011516 0 ustar 00 <?php namespace Spatie\Dropbox; use Exception; use GrahamCampbell\GuzzleFactory\GuzzleFactory; use GuzzleHttp\Client as GuzzleClient; use GuzzleHttp\ClientInterface; use GuzzleHttp\Exception\ClientException; use GuzzleHttp\Exception\RequestException; use GuzzleHttp\Psr7; use GuzzleHttp\Psr7\PumpStream; use GuzzleHttp\Psr7\StreamWrapper; use Psr\Http\Message\ResponseInterface; use Psr\Http\Message\StreamInterface; use Spatie\Dropbox\Exceptions\BadRequest; class Client { public const THUMBNAIL_FORMAT_JPEG = 'jpeg'; public const THUMBNAIL_FORMAT_PNG = 'png'; public const THUMBNAIL_SIZE_XS = 'w32h32'; public const THUMBNAIL_SIZE_S = 'w64h64'; public const THUMBNAIL_SIZE_M = 'w128h128'; public const THUMBNAIL_SIZE_L = 'w640h480'; public const THUMBNAIL_SIZE_XL = 'w1024h768'; public const MAX_CHUNK_SIZE = 1024 * 1024 * 150; public const UPLOAD_SESSION_START = 0; public const UPLOAD_SESSION_APPEND = 1; private ?TokenProvider $tokenProvider = null; protected ?string $namespaceId = null; protected ?string $appKey = null; protected ?string $appSecret = null; protected ClientInterface $client; protected int $maxChunkSize; /** * @param string|array<string>|TokenProvider|null $accessTokenOrAppCredentials * @param int $maxChunkSize Set max chunk size per request (determines when to switch from "one shot upload" to upload session and defines chunk size for uploads via session). * @param int $maxUploadChunkRetries How many times to retry an upload session start or append after RequestException. * @param ?string $teamMemberId The team member ID to be specified for Dropbox business accounts */ public function __construct( string|array|TokenProvider|null $accessTokenOrAppCredentials = null, ClientInterface $client = null, int $maxChunkSize = self::MAX_CHUNK_SIZE, protected int $maxUploadChunkRetries = 0, protected ?string $teamMemberId = null, ) { if (is_array($accessTokenOrAppCredentials)) { [$this->appKey, $this->appSecret] = $accessTokenOrAppCredentials; } if ($accessTokenOrAppCredentials instanceof TokenProvider) { $this->tokenProvider = $accessTokenOrAppCredentials; } if (is_string($accessTokenOrAppCredentials)) { $this->tokenProvider = new InMemoryTokenProvider($accessTokenOrAppCredentials); } $this->client = $client ?? new GuzzleClient(['handler' => GuzzleFactory::handler()]); $this->maxChunkSize = ($maxChunkSize < self::MAX_CHUNK_SIZE ? max($maxChunkSize, 1) : self::MAX_CHUNK_SIZE); } /** * Copy a file or folder to a different location in the user's Dropbox. * * If the source path is a folder all its contents will be copied. * * @return array<string, string> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-copy_v2 */ public function copy(string $fromPath, string $toPath): array { $parameters = [ 'from_path' => $this->normalizePath($fromPath), 'to_path' => $this->normalizePath($toPath), ]; return $this->rpcEndpointRequest('files/copy_v2', $parameters); } /** * Create a folder at a given path. * * @return array<string, string> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-create_folder */ public function createFolder(string $path): array { $parameters = [ 'path' => $this->normalizePath($path), ]; $object = $this->rpcEndpointRequest('files/create_folder', $parameters); $object['.tag'] = 'folder'; return $object; } /** * Create a shared link with custom settings. * * If no settings are given then the default visibility is RequestedVisibility.public. * The resolved visibility, though, may depend on other aspects such as team and * shared folder settings). Only for paid users. * * @param array<string, string> $settings * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#sharing-create_shared_link_with_settings */ public function createSharedLinkWithSettings(string $path, array $settings = []): array { $parameters = [ 'path' => $this->normalizePath($path), ]; if (count($settings)) { $parameters = array_merge(compact('settings'), $parameters); } return $this->rpcEndpointRequest('sharing/create_shared_link_with_settings', $parameters); } /** * Search a file or folder in the user's Dropbox. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-search */ public function search(string $query, bool $includeHighlights = false): array { $parameters = [ 'query' => $query, 'include_highlights' => $includeHighlights, ]; return $this->rpcEndpointRequest('files/search_v2', $parameters); } /** * List shared links. * * For empty path returns a list of all shared links. For non-empty path * returns a list of all shared links with access to the given path. * * If direct_only is set true, only direct links to the path will be returned, otherwise * it may return link to the path itself and parent folders as described on docs. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#sharing-list_shared_links */ public function listSharedLinks(string $path = null, bool $direct_only = false, string $cursor = null): array { $parameters = [ 'path' => $path ? $this->normalizePath($path) : null, 'cursor' => $cursor, 'direct_only' => $direct_only, ]; $body = $this->rpcEndpointRequest('sharing/list_shared_links', $parameters); return $body['links']; } /** * Delete the file or folder at a given path. * * If the path is a folder, all its contents will be deleted too. * A successful response indicates that the file or folder was deleted. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-delete */ public function delete(string $path): array { $parameters = [ 'path' => $this->normalizePath($path), ]; return $this->rpcEndpointRequest('files/delete', $parameters); } /** * Download a file from a user's Dropbox. * * * @return resource * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-download */ public function download(string $path) { $arguments = [ 'path' => $this->normalizePath($path), ]; $response = $this->contentEndpointRequest('files/download', $arguments); return StreamWrapper::getResource($response->getBody()); } /** * Download a folder from the user's Dropbox, as a zip file. * The folder must be less than 20 GB in size and have fewer than 10,000 total files. * The input cannot be a single file. Any single file must be less than 4GB in size. * * * @return resource * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-download_zip */ public function downloadZip(string $path) { $arguments = [ 'path' => $this->normalizePath($path), ]; $response = $this->contentEndpointRequest('files/download_zip', $arguments); return StreamWrapper::getResource($response->getBody()); } /** * Returns the metadata for a file or folder. * * Note: Metadata for the root folder is unsupported. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-get_metadata */ public function getMetadata(string $path): array { $parameters = [ 'path' => $this->normalizePath($path), ]; return $this->rpcEndpointRequest('files/get_metadata', $parameters); } /** * Get a temporary link to stream content of a file. * * This link will expire in four hours and afterwards you will get 410 Gone. * Content-Type of the link is determined automatically by the file's mime type. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-get_temporary_link */ public function getTemporaryLink(string $path): string { $parameters = [ 'path' => $this->normalizePath($path), ]; $body = $this->rpcEndpointRequest('files/get_temporary_link', $parameters); return $body['link']; } /** * Get a thumbnail for an image. * * This method currently supports files with the following file extensions: * jpg, jpeg, png, tiff, tif, gif and bmp. * * Photos that are larger than 20MB in size won't be converted to a thumbnail. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-get_thumbnail */ public function getThumbnail(string $path, string $format = 'jpeg', string $size = 'w64h64'): string { $arguments = [ 'path' => $this->normalizePath($path), 'format' => $format, 'size' => $size, ]; $response = $this->contentEndpointRequest('files/get_thumbnail', $arguments); return (string) $response->getBody(); } /** * Starts returning the contents of a folder. * * If the result's ListFolderResult.has_more field is true, call * list_folder/continue with the returned ListFolderResult.cursor to retrieve more entries. * * Note: auth.RateLimitError may be returned if multiple list_folder or list_folder/continue calls * with same parameters are made simultaneously by same API app for same user. If your app implements * retry logic, please hold off the retry until the previous request finishes. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-list_folder */ public function listFolder(string $path = '', bool $recursive = false): array { $parameters = [ 'path' => $this->normalizePath($path), 'recursive' => $recursive, ]; return $this->rpcEndpointRequest('files/list_folder', $parameters); } /** * Once a cursor has been retrieved from list_folder, use this to paginate through all files and * retrieve updates to the folder, following the same rules as documented for list_folder. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-list_folder-continue */ public function listFolderContinue(string $cursor = ''): array { return $this->rpcEndpointRequest('files/list_folder/continue', compact('cursor')); } /** * Move a file or folder to a different location in the user's Dropbox. * * If the source path is a folder all its contents will be moved. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-move_v2 */ public function move(string $fromPath, string $toPath, bool $autorename = false): array { $parameters = [ 'from_path' => $this->normalizePath($fromPath), 'to_path' => $this->normalizePath($toPath), 'autorename' => $autorename, ]; return $this->rpcEndpointRequest('files/move_v2', $parameters); } /** * The file should be uploaded in chunks if its size exceeds the 150 MB threshold * or if the resource size could not be determined (eg. a popen() stream). * * @param string|resource $contents */ protected function shouldUploadChunked(mixed $contents): bool { $size = is_string($contents) ? strlen($contents) : fstat($contents)['size']; if ($this->isPipe($contents)) { return true; } return $size > $this->maxChunkSize; } /** * Check if the contents is a pipe stream (not seekable, no size defined). * * @param string|resource $contents */ protected function isPipe(mixed $contents): bool { return is_resource($contents) && (fstat($contents)['mode'] & 010000) != 0; } /** * Create a new file with the contents provided in the request. * * Do not use this to upload a file larger than 150 MB. Instead, create an upload session with upload_session/start. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-upload * * @param string|resource $contents * @return array<mixed> */ public function upload(string $path, mixed $contents, string $mode = 'add', bool $autorename = false): array { if ($this->shouldUploadChunked($contents)) { return $this->uploadChunked($path, $contents, $mode); } $arguments = [ 'path' => $this->normalizePath($path), 'mode' => $mode, 'autorename' => $autorename, ]; $response = $this->contentEndpointRequest('files/upload', $arguments, $contents); $metadata = json_decode($response->getBody(), true); $metadata['.tag'] = 'file'; return $metadata; } /** * Upload file split in chunks. This allows uploading large files, since * Dropbox API v2 limits the content size to 150MB. * * The chunk size will affect directly the memory usage, so be careful. * Large chunks tends to speed up the upload, while smaller optimizes memory usage. * * @param string|resource $contents * @return array<mixed> */ public function uploadChunked(string $path, mixed $contents, string $mode = 'add', ?int $chunkSize = null): array { if ($chunkSize === null || $chunkSize > $this->maxChunkSize) { $chunkSize = $this->maxChunkSize; } $stream = $this->getStream($contents); $cursor = $this->uploadChunk(self::UPLOAD_SESSION_START, $stream, $chunkSize, null); while (! $stream->eof()) { $cursor = $this->uploadChunk(self::UPLOAD_SESSION_APPEND, $stream, $chunkSize, $cursor); } return $this->uploadSessionFinish('', $cursor, $path, $mode); } /** * @throws Exception */ protected function uploadChunk(int $type, StreamInterface &$stream, int $chunkSize, ?UploadSessionCursor $cursor = null): UploadSessionCursor { $maximumTries = $stream->isSeekable() ? $this->maxUploadChunkRetries : 0; $pos = $stream->tell(); $tries = 0; tryUpload: try { $tries++; $chunkStream = new Psr7\LimitStream($stream, $chunkSize, $stream->tell()); if ($type === self::UPLOAD_SESSION_START) { return $this->uploadSessionStart($chunkStream); } if ($type === self::UPLOAD_SESSION_APPEND && $cursor !== null) { return $this->uploadSessionAppend($chunkStream, $cursor); } throw new Exception('Invalid type'); } catch (RequestException $exception) { if ($tries < $maximumTries) { // rewind $stream->seek($pos, SEEK_SET); goto tryUpload; } throw $exception; } } /** * Upload sessions allow you to upload a single file in one or more requests, * for example where the size of the file is greater than 150 MB. * This call starts a new upload session with the given data. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-upload_session-start * * @param string|resource|StreamInterface $contents */ public function uploadSessionStart(mixed $contents, bool $close = false): UploadSessionCursor { $arguments = compact('close'); $response = json_decode( $this->contentEndpointRequest('files/upload_session/start', $arguments, $contents)->getBody(), true ); return new UploadSessionCursor($response['session_id'], ($contents instanceof StreamInterface ? $contents->tell() : strlen($contents))); } /** * Append more data to an upload session. * When the parameter close is set, this call will close the session. * A single request should not upload more than 150 MB. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-upload_session-append_v2 */ public function uploadSessionAppend(string|StreamInterface $contents, UploadSessionCursor $cursor, bool $close = false): UploadSessionCursor { $arguments = compact('cursor', 'close'); $pos = $contents instanceof StreamInterface ? $contents->tell() : 0; $this->contentEndpointRequest('files/upload_session/append_v2', $arguments, $contents); $cursor->offset += $contents instanceof StreamInterface ? ($contents->tell() - $pos) : strlen($contents); return $cursor; } /** * Finish an upload session and save the uploaded data to the given file path. * A single request should not upload more than 150 MB. * * @link https://www.dropbox.com/developers/documentation/http/documentation#files-upload_session-finish * * @param string|resource|StreamInterface $contents * @return array<mixed> */ public function uploadSessionFinish(mixed $contents, UploadSessionCursor $cursor, string $path, string $mode = 'add', bool $autorename = false, bool $mute = false): array { $arguments = compact('cursor'); $arguments['commit'] = compact('path', 'mode', 'autorename', 'mute'); $response = $this->contentEndpointRequest( 'files/upload_session/finish', $arguments, ($contents == '') ? null : $contents ); $metadata = json_decode($response->getBody(), true); $metadata['.tag'] = 'file'; return $metadata; } /** * Get Account Info for current authenticated user. * * @return array<mixed> * * @link https://www.dropbox.com/developers/documentation/http/documentation#users-get_current_account */ public function getAccountInfo(): array { return $this->rpcEndpointRequest('users/get_current_account'); } /** * Revoke current access token. * * @link https://www.dropbox.com/developers/documentation/http/documentation#auth-token-revoke */ public function revokeToken(): void { $this->rpcEndpointRequest('auth/token/revoke'); } protected function normalizePath(string $path): string { if (preg_match("/^id:.*|^rev:.*|^(ns:[0-9]+(\/.*)?)/", $path) === 1) { return $path; } $path = trim($path, '/'); return ($path === '') ? '' : '/'.$path; } protected function getEndpointUrl(string $subdomain, string $endpoint): string { if (count($parts = explode('::', $endpoint)) === 2) { [$subdomain, $endpoint] = $parts; } return "https://{$subdomain}.dropboxapi.com/2/{$endpoint}"; } /** * @param array<string, mixed> $arguments * @param string|resource|StreamInterface $body * * @throws \Exception */ public function contentEndpointRequest(string $endpoint, array $arguments, mixed $body = '', bool $isRefreshed = false): ResponseInterface { $headers = ['Dropbox-API-Arg' => json_encode($arguments)]; if ($body !== '') { $headers['Content-Type'] = 'application/octet-stream'; } try { $response = $this->client->request('POST', $this->getEndpointUrl('content', $endpoint), [ 'headers' => $this->getHeaders($headers), 'body' => $body, ]); } catch (ClientException $exception) { if ( $isRefreshed || ! $this->tokenProvider instanceof RefreshableTokenProvider || ! $this->tokenProvider->refresh($exception) ) { throw $this->determineException($exception); } $response = $this->contentEndpointRequest($endpoint, $arguments, $body, true); } return $response; } /** * @param array<string, string|bool|array<string>>|null $parameters * @return array<mixed> */ public function rpcEndpointRequest(string $endpoint, array $parameters = null, bool $isRefreshed = false): array { try { $options = ['headers' => $this->getHeaders()]; if ($parameters) { $options['json'] = $parameters; } $response = $this->client->request('POST', $this->getEndpointUrl('api', $endpoint), $options); } catch (ClientException $exception) { if ( $isRefreshed || ! $this->tokenProvider instanceof RefreshableTokenProvider || ! $this->tokenProvider->refresh($exception) ) { throw $this->determineException($exception); } return $this->rpcEndpointRequest($endpoint, $parameters, true); } return json_decode($response->getBody(), true) ?? []; } protected function determineException(ClientException $exception): Exception { if (in_array($exception->getResponse()->getStatusCode(), [400, 409])) { return new BadRequest($exception->getResponse()); } return $exception; } /** * @param string|resource $contents */ protected function getStream(mixed $contents): StreamInterface { if ($this->isPipe($contents)) { /* @var resource $contents */ return new PumpStream(function ($length) use ($contents) { $data = fread($contents, $length); if (strlen($data) === 0) { return false; } return $data; }); } return Psr7\Utils::streamFor($contents); } /** * Get the access token. */ public function getAccessToken(): string { return $this->tokenProvider->getToken(); } /** * Set the access token. */ public function setAccessToken(string $accessToken): self { $this->tokenProvider = new InMemoryTokenProvider($accessToken); return $this; } /** * Set the namespace ID. */ public function setNamespaceId(string $namespaceId): self { $this->namespaceId = $namespaceId; return $this; } /** * Get the HTTP headers. * * @param array<string, string> $headers * @return array<string, string> */ protected function getHeaders(array $headers = []): array { $auth = []; if ($this->tokenProvider || ($this->appKey && $this->appSecret)) { $auth = $this->tokenProvider ? $this->getHeadersForBearerToken($this->tokenProvider->getToken()) : $this->getHeadersForCredentials(); } if ($this->teamMemberId) { $auth = array_merge( $auth, [ 'Dropbox-API-Select-User' => $this->teamMemberId, ] ); } if ($this->namespaceId) { $headers = array_merge( $headers, [ 'Dropbox-API-Path-Root' => json_encode([ '.tag' => 'namespace_id', 'namespace_id' => $this->namespaceId, ]), ] ); } return array_merge($auth, $headers); } /** * @return array{Authorization: string} */ protected function getHeadersForBearerToken(string $token): array { return [ 'Authorization' => "Bearer {$token}", ]; } /** * @return array{Authorization: string} */ protected function getHeadersForCredentials(): array { return [ 'Authorization' => 'Basic '.base64_encode("{$this->appKey}:{$this->appSecret}"), ]; } } dropbox-api/src/RefreshableTokenProvider.php 0000644 00000000416 15002154456 0015231 0 ustar 00 <?php namespace Spatie\Dropbox; use GuzzleHttp\Exception\ClientException; interface RefreshableTokenProvider extends TokenProvider { /** * @return bool Whether the token was refreshed. */ public function refresh(ClientException $exception): bool; } dropbox-api/src/TokenProvider.php 0000644 00000000146 15002154456 0013066 0 ustar 00 <?php namespace Spatie\Dropbox; interface TokenProvider { public function getToken(): string; } dropbox-api/src/UploadSessionCursor.php 0000644 00000000703 15002154456 0014260 0 ustar 00 <?php namespace Spatie\Dropbox; class UploadSessionCursor { public function __construct( /** * The upload session ID (returned by upload_session/start). */ public string $session_id, /** * The amount of data that has been uploaded so far. We use this to make sure upload data isn't lost or duplicated in the event of a network error. */ public int $offset = 0, ) { } } dropbox-api/LICENSE.md 0000644 00000002102 15002154456 0010371 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. dropbox-api/CHANGELOG.md 0000644 00000011513 15002154456 0010604 0 ustar 00 # Changelog All notable changes to `dropbox-api` will be documented in this file ## 1.21.2 - 2023-06-07 ### What's Changed - Bump dependabot/fetch-metadata from 1.3.6 to 1.4.0 by @dependabot in https://github.com/spatie/dropbox-api/pull/115 - Bump dependabot/fetch-metadata from 1.4.0 to 1.5.0 by @dependabot in https://github.com/spatie/dropbox-api/pull/117 - Bump dependabot/fetch-metadata from 1.5.0 to 1.5.1 by @dependabot in https://github.com/spatie/dropbox-api/pull/118 - Fix README badges by @jmsche in https://github.com/spatie/dropbox-api/pull/120 - Allow graham-campbell/guzzle-factory v7 by @jmsche in https://github.com/spatie/dropbox-api/pull/119 - Fix PHPUnit errors & failures by @jmsche in https://github.com/spatie/dropbox-api/pull/121 **Full Changelog**: https://github.com/spatie/dropbox-api/compare/1.21.1...1.21.2 ## 1.21.1 - 2023-03-17 ### What's Changed - Add PHP 8.2 support by @patinthehat in https://github.com/spatie/dropbox-api/pull/110 - Add dependabot automation by @patinthehat in https://github.com/spatie/dropbox-api/pull/109 - Update Dependabot Automation by @patinthehat in https://github.com/spatie/dropbox-api/pull/112 - Bump dependabot/fetch-metadata from 1.3.5 to 1.3.6 by @dependabot in https://github.com/spatie/dropbox-api/pull/113 - Allow graham-campbell/guzzle-factory v6 by @jmsche in https://github.com/spatie/dropbox-api/pull/114 ### New Contributors - @patinthehat made their first contribution in https://github.com/spatie/dropbox-api/pull/110 - @dependabot made their first contribution in https://github.com/spatie/dropbox-api/pull/113 **Full Changelog**: https://github.com/spatie/dropbox-api/compare/1.21.0...1.21.1 ## 1.21.0 - 2022-09-27 ### What's Changed - Add ability to set the namespace ID for requests by @rstefanic in https://github.com/spatie/dropbox-api/pull/105 ### New Contributors - @rstefanic made their first contribution in https://github.com/spatie/dropbox-api/pull/105 **Full Changelog**: https://github.com/spatie/dropbox-api/compare/1.20.2...1.21.0 ## 1.20.2 - 2022-06-24 ### What's Changed - uploadSessionStart and uploadSessionFinish can accept resource by @dmitryuk in https://github.com/spatie/dropbox-api/pull/102 ### New Contributors - @dmitryuk made their first contribution in https://github.com/spatie/dropbox-api/pull/102 **Full Changelog**: https://github.com/spatie/dropbox-api/compare/1.20.1...1.20.2 ## 1.20.1 - 2022-03-29 ## What's Changed - Fix refreshable token response by @einarsozols in https://github.com/spatie/dropbox-api/pull/100 ## New Contributors - @einarsozols made their first contribution in https://github.com/spatie/dropbox-api/pull/100 **Full Changelog**: https://github.com/spatie/dropbox-api/compare/1.20.0...1.20.1 ## Unreleased - Added refreshable token provider interface. ## 1.19.1 - 2021-07-04 - fix compability with guzzlehttp/psr7 2.0 (#91) ## 1.19.0 - 2021-06-18 - add autoRename parameter for move() method (#89) ## 1.18.0 - 2021-05-27 - add autorename option to upload method (#86) ## 1.17.1 - 2021-03-01 - allow graham-campbell/guzzle-factory v5 (#79) ## 1.17.0 - 2020-12-08 - `TokenProvider` interface for accesstokens (#76) ## 1.16.1 - 2020-11-27 - allow PHP 8 ## 1.16.0 - 2020-09-25 - allow the Client to work with Dropbox business accounts ## 1.15.0 - 2020-07-09 - allow Guzzle 7 (#70) ## 1.14.0 - 2020-05-11 - add support for app authentication and no authentication ## 1.13.0 - 2020-05-03 - added `downloadZip` (#66) ## 1.12.0 - 2020-02-04 - add `search` method ## 1.11.1 - 2019-12-12 - make compatible with PHP 7.4 ## 1.11.0 - 2019-07-04 - add `$response` to `BadRequest` ## 1.10.0 - 2019-07-01 - move retry stuff to package ## 1.9.0 - 2019-05-21 - make guzzle retry 5xx and 429 responses ## 1.8.0 - 2019-04-13 - add `getEndpointUrl` - drop support for PHP 7.0 ## 1.7.1 - 2019-02-13 - fix for `createSharedLinkWithSettings` with empty settings ## 1.7.0 - 2019-02-06 - add getter and setter for the access token ## 1.6.6 - 2018-07-19 - fix for piped streams ## 1.6.5 - 2018-01-15 - adjust `normalizePath` to allow id/rev/ns to be queried ## 1.6.4 - 2017-12-05 - fix max chunk size ## 1.6.1 - 2017-07-28 - fix for finishing upload session ## 1.6.0 - 2017-07-28 - add various new methods to enable chuncked uploads ## 1.5.3 - 2017-07-28 - use recommended `move_v2` method to move files ## 1.5.2 - 2017-07-17 - add missing parameters to `listSharedLinks` method ## 1.5.1 - 2017-07-17 - fix broken `revokeToken` and `getAccountInfo` ## 1.5.0 - 2017-07-11 - add `revokeToken` and `getAccountInfo` ## 1.4.0 - 2017-07-11 - add `listSharedLinks` ## 1.3.0 - 2017-07-04 - add error code to thrown exception ## 1.2.0 - 2017-04-29 - added `createSharedLinkWithSettings` ## 1.1.0 - 2017-04-22 - added `listFolderContinue` ## 1.0.1 - 2017-04-19 - Bugfix: set default value for request body ## 1.0.0 - 2017-04-19 - initial release laravel-ignition/composer.json 0000644 00000005062 15002154456 0012537 0 ustar 00 { "name": "spatie/laravel-ignition", "description": "A beautiful error page for Laravel applications.", "keywords": [ "error", "page", "laravel", "flare" ], "authors": [ { "name": "Spatie", "email": "info@spatie.be", "role": "Developer" } ], "homepage": "https://flareapp.io/ignition", "license": "MIT", "require": { "php": "^8.0", "ext-curl": "*", "ext-json": "*", "ext-mbstring": "*", "monolog/monolog": "^2.3", "spatie/ignition": "^1.4.1", "spatie/flare-client-php": "^1.0.1", "symfony/console": "^5.0|^6.0", "symfony/var-dumper": "^5.0|^6.0", "illuminate/support": "^8.77|^9.27" }, "require-dev": { "filp/whoops": "^2.14", "livewire/livewire": "^2.8|dev-develop", "mockery/mockery": "^1.4", "nunomaduro/larastan": "^1.0", "orchestra/testbench": "^6.23|^7.0", "pestphp/pest": "^1.20", "phpstan/extension-installer": "^1.1", "phpstan/phpstan-deprecation-rules": "^1.0", "phpstan/phpstan-phpunit": "^1.0", "spatie/laravel-ray": "^1.27" }, "config": { "sort-packages": true, "allow-plugins": { "phpstan/extension-installer": true, "pestphp/pest-plugin": true } }, "extra": { "laravel": { "providers": [ "Spatie\\LaravelIgnition\\IgnitionServiceProvider" ], "aliases": { "Flare": "Spatie\\LaravelIgnition\\Facades\\Flare" } } }, "autoload": { "psr-4": { "Spatie\\LaravelIgnition\\": "src" }, "files": [ "src/helpers.php" ] }, "autoload-dev": { "psr-4": { "Spatie\\LaravelIgnition\\Tests\\": "tests" } }, "minimum-stability": "dev", "prefer-stable": true, "scripts": { "analyse": "vendor/bin/phpstan analyse", "baseline": "vendor/bin/phpstan --generate-baseline", "format": "vendor/bin/php-cs-fixer fix --allow-risky=yes", "test": "vendor/bin/pest", "test-coverage": "vendor/bin/phpunit --coverage-html coverage" }, "support": { "issues": "https://github.com/spatie/laravel-ignition/issues", "forum": "https://twitter.com/flareappio", "source": "https://github.com/spatie/laravel-ignition", "docs": "https://flareapp.io/docs/ignition-for-laravel/introduction" } } laravel-ignition/README.md 0000644 00000005476 15002154456 0011305 0 ustar 00 # Ignition: a beautiful error page for Laravel apps [](https://packagist.org/packages/spatie/laravel-ignition)  [](https://packagist.org/packages/spatie/laravel-ignition) [Ignition](https://flareapp.io/docs/ignition-for-laravel/introduction) is a beautiful and customizable error page for Laravel applications. It is the default error page for new Laravel applications. It also allows to publicly share your errors on [Flare](https://flareapp.io). If configured with a valid Flare API key, your errors in production applications will be tracked, and you'll get notified when they happen. `spatie/laravel-ignition` works for Laravel 8 and 9 applications running on PHP 8.0 and above. Looking for Ignition for Laravel 5.x, 6.x or 7.x or old PHP versions? `facade/ignition` is still compatible. 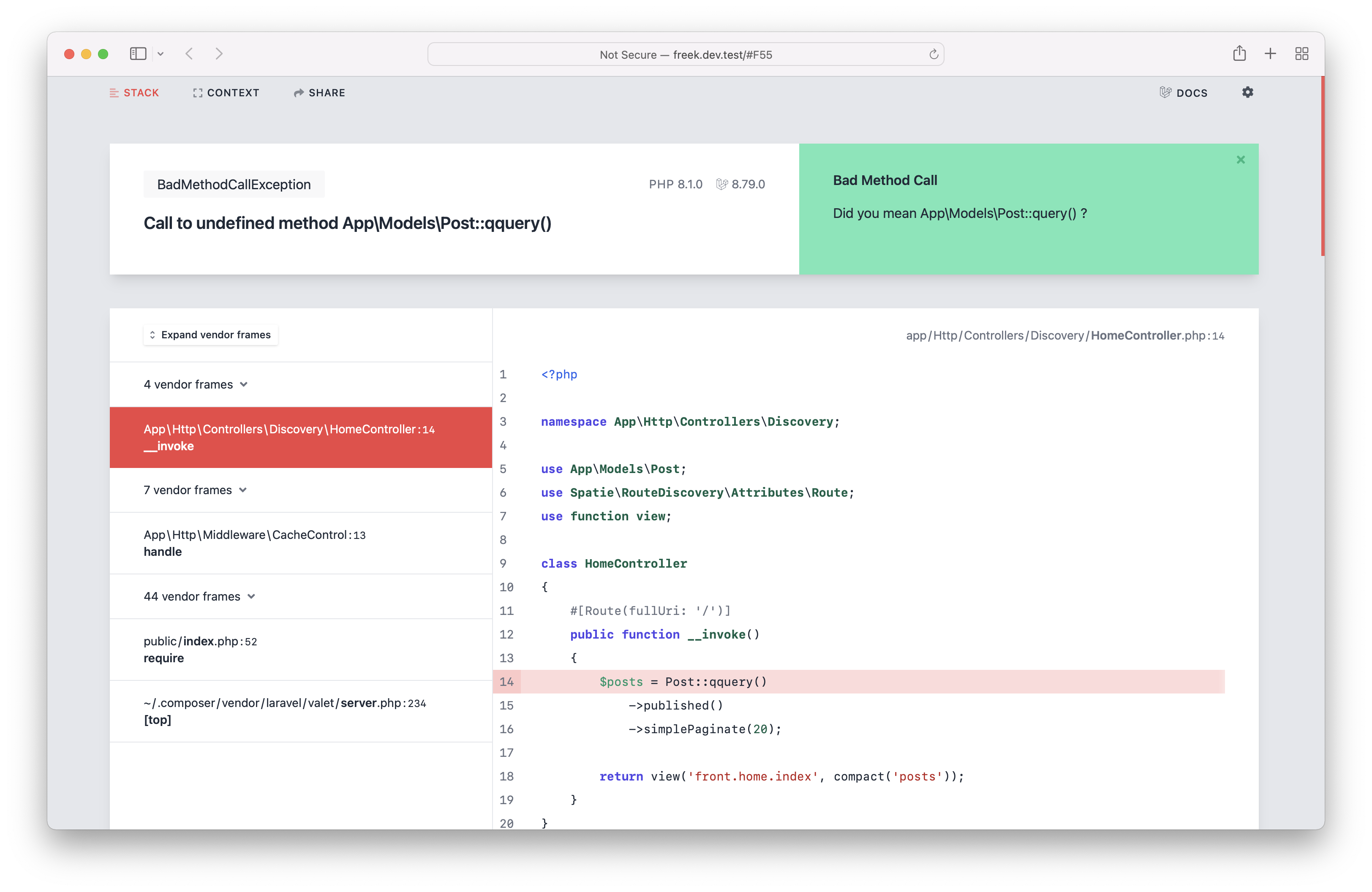 ## Are you a visual learner? In [this video on YouTube](https://youtu.be/LEY0N0Bteew?t=739), you'll see a demo of all of the features. Do know more about the design decisions we made, read [this blog post](https://freek.dev/2168-ignition-the-most-beautiful-error-page-for-laravel-and-php-got-a-major-redesign). ## Official Documentation The official documentation for Ignition can be found on the [Flare website](https://flareapp.io/docs/ignition-for-laravel/installation). ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/laravel-ignition.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/laravel-ignition) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ### Changelog Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security Vulnerabilities Please review [our security policy](../../security/policy) on how to report security vulnerabilities. ## Credits - [Spatie](https://spatie.be) - [All Contributors](../../contributors) ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. laravel-ignition/src/FlareMiddleware/AddExceptionInformation.php 0000644 00000002140 15002154456 0021113 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Illuminate\Database\QueryException; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; class AddExceptionInformation implements FlareMiddleware { public function handle(Report $report, $next) { $throwable = $report->getThrowable(); $this->addUserDefinedContext($report); if (! $throwable instanceof QueryException) { return $next($report); } $report->group('exception', [ 'raw_sql' => $throwable->getSql(), ]); return $next($report); } private function addUserDefinedContext(Report $report): void { $throwable = $report->getThrowable(); if ($throwable === null) { return; } if (! method_exists($throwable, 'context')) { return; } $context = $throwable->context(); if (! is_array($context)) { return; } foreach ($context as $key => $value) { $report->context($key, $value); } } } laravel-ignition/src/FlareMiddleware/AddEnvironmentInformation.php 0000644 00000001333 15002154456 0021464 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Closure; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; class AddEnvironmentInformation implements FlareMiddleware { public function handle(Report $report, Closure $next) { $report->frameworkVersion(app()->version()); $report->group('env', [ 'laravel_version' => app()->version(), 'laravel_locale' => app()->getLocale(), 'laravel_config_cached' => app()->configurationIsCached(), 'app_debug' => config('app.debug'), 'app_env' => config('app.env'), 'php_version' => phpversion(), ]); return $next($report); } } laravel-ignition/src/FlareMiddleware/AddJobs.php 0000644 00000001117 15002154456 0015647 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; use Spatie\LaravelIgnition\Recorders\JobRecorder\JobRecorder; class AddJobs implements FlareMiddleware { protected JobRecorder $jobRecorder; public function __construct() { $this->jobRecorder = app(JobRecorder::class); } public function handle(Report $report, $next) { if ($job = $this->jobRecorder->getJob()) { $report->group('job', $job); } return $next($report); } } laravel-ignition/src/FlareMiddleware/AddLogs.php 0000644 00000001057 15002154456 0015661 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; use Spatie\LaravelIgnition\Recorders\LogRecorder\LogRecorder; class AddLogs implements FlareMiddleware { protected LogRecorder $logRecorder; public function __construct() { $this->logRecorder = app(LogRecorder::class); } public function handle(Report $report, $next) { $report->group('logs', $this->logRecorder->getLogMessages()); return $next($report); } } laravel-ignition/src/FlareMiddleware/AddQueries.php 0000644 00000000754 15002154456 0016375 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Spatie\FlareClient\Report; use Spatie\LaravelIgnition\Recorders\QueryRecorder\QueryRecorder; class AddQueries { protected QueryRecorder $queryRecorder; public function __construct() { $this->queryRecorder = app(QueryRecorder::class); } public function handle(Report $report, $next) { $report->group('queries', $this->queryRecorder->getQueries()); return $next($report); } } laravel-ignition/src/FlareMiddleware/AddDumps.php 0000644 00000001107 15002154456 0016041 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Closure; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; use Spatie\LaravelIgnition\Recorders\DumpRecorder\DumpRecorder; class AddDumps implements FlareMiddleware { protected DumpRecorder $dumpRecorder; public function __construct() { $this->dumpRecorder = app(DumpRecorder::class); } public function handle(Report $report, Closure $next) { $report->group('dumps', $this->dumpRecorder->getDumps()); return $next($report); } } laravel-ignition/src/FlareMiddleware/AddNotifierName.php 0000644 00000000617 15002154456 0017336 0 ustar 00 <?php namespace Spatie\LaravelIgnition\FlareMiddleware; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\Report; class AddNotifierName implements FlareMiddleware { public const NOTIFIER_NAME = 'Laravel Client'; public function handle(Report $report, $next) { $report->notifierName(static::NOTIFIER_NAME); return $next($report); } } laravel-ignition/src/Renderers/IgnitionExceptionRenderer.php 0000644 00000001005 15002154456 0020365 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Renderers; use Illuminate\Contracts\Foundation\ExceptionRenderer; class IgnitionExceptionRenderer implements ExceptionRenderer { protected ErrorPageRenderer $errorPageHandler; public function __construct(ErrorPageRenderer $errorPageHandler) { $this->errorPageHandler = $errorPageHandler; } public function render($throwable) { ob_start(); $this->errorPageHandler->render($throwable); return ob_get_clean(); } } laravel-ignition/src/Renderers/ErrorPageRenderer.php 0000644 00000002746 15002154456 0016631 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Renderers; use Spatie\FlareClient\Flare; use Spatie\Ignition\Config\IgnitionConfig; use Spatie\Ignition\Contracts\SolutionProviderRepository; use Spatie\Ignition\Ignition; use Spatie\LaravelIgnition\ContextProviders\LaravelContextProviderDetector; use Spatie\LaravelIgnition\Solutions\SolutionTransformers\LaravelSolutionTransformer; use Spatie\LaravelIgnition\Support\LaravelDocumentationLinkFinder; use Throwable; class ErrorPageRenderer { public function render(Throwable $throwable): void { $vitejsAutoRefresh = ''; if (class_exists('Illuminate\Foundation\Vite')) { $vite = app(\Illuminate\Foundation\Vite::class); if (is_file($vite->hotFile())) { $vitejsAutoRefresh = $vite->__invoke([]); } } app(Ignition::class) ->resolveDocumentationLink( fn (Throwable $throwable) => (new LaravelDocumentationLinkFinder())->findLinkForThrowable($throwable) ) ->setFlare(app(Flare::class)) ->setConfig(app(IgnitionConfig::class)) ->setSolutionProviderRepository(app(SolutionProviderRepository::class)) ->setContextProviderDetector(new LaravelContextProviderDetector()) ->setSolutionTransformerClass(LaravelSolutionTransformer::class) ->applicationPath(base_path()) ->addCustomHtmlToHead($vitejsAutoRefresh) ->renderException($throwable); } } laravel-ignition/src/Renderers/IgnitionWhoopsHandler.php 0000644 00000002104 15002154456 0017516 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Renderers; use Error; use ErrorException; use Throwable; use Whoops\Handler\Handler; class IgnitionWhoopsHandler extends Handler { protected ErrorPageRenderer $errorPageHandler; protected Throwable $exception; public function __construct(ErrorPageRenderer $errorPageHandler) { $this->errorPageHandler = $errorPageHandler; } public function handle(): ?int { try { $this->errorPageHandler->render($this->exception); } catch (Error $error) { // Errors aren't caught by Whoops. // Convert the error to an exception and throw again. throw new ErrorException( $error->getMessage(), $error->getCode(), 1, $error->getFile(), $error->getLine(), $error ); } return Handler::QUIT; } /** @param \Throwable $exception */ public function setException($exception): void { $this->exception = $exception; } } laravel-ignition/src/Exceptions/CannotExecuteSolutionForNonLocalIp.php 0000644 00000001500 15002154456 0022317 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Exceptions; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\Ignition\Contracts\Solution; use Symfony\Component\HttpKernel\Exception\HttpException; class CannotExecuteSolutionForNonLocalIp extends HttpException implements ProvidesSolution { public static function make(): self { return new self(403, 'Solutions cannot be run from your current IP address.'); } public function getSolution(): Solution { return BaseSolution::create() ->setSolutionTitle('Checking your environment settings') ->setSolutionDescription("Solutions can only be executed by requests from a local IP address. Keep in mind that `APP_DEBUG` should set to false on any production environment."); } } laravel-ignition/src/Exceptions/ViewException.php 0000644 00000002307 15002154456 0016226 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Exceptions; use ErrorException; use Spatie\FlareClient\Contracts\ProvidesFlareContext; use Spatie\LaravelIgnition\Recorders\DumpRecorder\HtmlDumper; class ViewException extends ErrorException implements ProvidesFlareContext { /** @var array<string, mixed> */ protected array $viewData = []; protected string $view = ''; /** * @param array<string, mixed> $data * * @return void */ public function setViewData(array $data): void { $this->viewData = $data; } /** @return array<string, mixed> */ public function getViewData(): array { return $this->viewData; } public function setView(string $path): void { $this->view = $path; } protected function dumpViewData(mixed $variable): string { return (new HtmlDumper())->dumpVariable($variable); } /** @return array<string, mixed> */ public function context(): array { $context = [ 'view' => [ 'view' => $this->view, ], ]; $context['view']['data'] = array_map([$this, 'dumpViewData'], $this->viewData); return $context; } } laravel-ignition/src/Exceptions/InvalidConfig.php 0000644 00000001705 15002154456 0016152 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Exceptions; use Exception; use Monolog\Logger; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\Ignition\Contracts\Solution; class InvalidConfig extends Exception implements ProvidesSolution { public static function invalidLogLevel(string $logLevel): self { return new self("Invalid log level `{$logLevel}` specified."); } public function getSolution(): Solution { $validLogLevels = array_map( fn (string $level) => strtolower($level), array_keys(Logger::getLevels()) ); $validLogLevelsString = implode(',', $validLogLevels); return BaseSolution::create() ->setSolutionTitle('You provided an invalid log level') ->setSolutionDescription("Please change the log level in your `config/logging.php` file. Valid log levels are {$validLogLevelsString}."); } } laravel-ignition/src/Exceptions/ViewExceptionWithSolution.php 0000644 00000000713 15002154456 0020616 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Exceptions; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\Ignition\Contracts\Solution; class ViewExceptionWithSolution extends ViewException implements ProvidesSolution { protected Solution $solution; public function setSolution(Solution $solution): void { $this->solution = $solution; } public function getSolution(): Solution { return $this->solution; } } laravel-ignition/src/Support/RunnableSolutionsGuard.php 0000644 00000002053 15002154456 0017437 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; class RunnableSolutionsGuard { /** * Check if runnable solutions are allowed based on the current * environment and config. * * @return bool */ public static function check(): bool { if (! config('app.debug')) { // Never run solutions in when debug mode is not enabled. return false; } if (config('ignition.enable_runnable_solutions') !== null) { // Allow enabling or disabling runnable solutions regardless of environment // if the IGNITION_ENABLE_RUNNABLE_SOLUTIONS env var is explicitly set. return config('ignition.enable_runnable_solutions'); } if (! app()->environment('local') && ! app()->environment('development')) { // Never run solutions on non-local environments. This avoids exposing // applications that are somehow APP_ENV=production with APP_DEBUG=true. return false; } return config('app.debug'); } } laravel-ignition/src/Support/Composer/Composer.php 0000644 00000000516 15002154456 0016346 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support\Composer; interface Composer { /** @return array<string, mixed> */ public function getClassMap(): array; /** @return array<string, mixed> */ public function getPrefixes(): array; /** @return array<string, mixed> */ public function getPrefixesPsr4(): array; } laravel-ignition/src/Support/Composer/ComposerClassMap.php 0000644 00000007355 15002154456 0020002 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support\Composer; use function app_path; use function base_path; use Illuminate\Support\Str; use Symfony\Component\Finder\Finder; use Symfony\Component\Finder\SplFileInfo; class ComposerClassMap { /** @var \Spatie\LaravelIgnition\Support\Composer\Composer */ protected object $composer; protected string $basePath; public function __construct(?string $autoloaderPath = null) { $autoloaderPath = $autoloaderPath ?? base_path('/vendor/autoload.php'); $this->composer = file_exists($autoloaderPath) ? require $autoloaderPath : new FakeComposer(); $this->basePath = app_path(); } /** @return array<string, string> */ public function listClasses(): array { $classes = $this->composer->getClassMap(); return array_merge($classes, $this->listClassesInPsrMaps()); } public function searchClassMap(string $missingClass): ?string { foreach ($this->composer->getClassMap() as $fqcn => $file) { $basename = basename($file, '.php'); if ($basename === $missingClass) { return $fqcn; } } return null; } /** @return array<string, mixed> */ public function listClassesInPsrMaps(): array { // TODO: This is incorrect. Doesnt list all fqcns. Need to parse namespace? e.g. App\LoginController is wrong $prefixes = array_merge( $this->composer->getPrefixes(), $this->composer->getPrefixesPsr4() ); $classes = []; foreach ($prefixes as $namespace => $directories) { foreach ($directories as $directory) { if (file_exists($directory)) { $files = (new Finder) ->in($directory) ->files() ->name('*.php'); foreach ($files as $file) { if ($file instanceof SplFileInfo) { $fqcn = $this->getFullyQualifiedClassNameFromFile($namespace, $file); $classes[$fqcn] = $file->getRelativePathname(); } } } } } return $classes; } public function searchPsrMaps(string $missingClass): ?string { $prefixes = array_merge( $this->composer->getPrefixes(), $this->composer->getPrefixesPsr4() ); foreach ($prefixes as $namespace => $directories) { foreach ($directories as $directory) { if (file_exists($directory)) { $files = (new Finder) ->in($directory) ->files() ->name('*.php'); foreach ($files as $file) { if ($file instanceof SplFileInfo) { $basename = basename($file->getRelativePathname(), '.php'); if ($basename === $missingClass) { return $namespace . basename($file->getRelativePathname(), '.php'); } } } } } } return null; } protected function getFullyQualifiedClassNameFromFile(string $rootNamespace, SplFileInfo $file): string { $class = trim(str_replace($this->basePath, '', (string)$file->getRealPath()), DIRECTORY_SEPARATOR); $class = str_replace( [DIRECTORY_SEPARATOR, 'App\\'], ['\\', app()->getNamespace()], ucfirst(Str::replaceLast('.php', '', $class)) ); return $rootNamespace . $class; } } laravel-ignition/src/Support/Composer/FakeComposer.php 0000644 00000000674 15002154456 0017142 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support\Composer; class FakeComposer implements Composer { /** @return array<string, mixed> */ public function getClassMap(): array { return []; } /** @return array<string, mixed> */ public function getPrefixes(): array { return []; } /** @return array<string, mixed> */ public function getPrefixesPsr4(): array { return []; } } laravel-ignition/src/Support/LivewireComponentParser.php 0000644 00000005735 15002154456 0017626 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; use Illuminate\Support\Collection; use Illuminate\Support\Str; use Livewire\LivewireManager; use ReflectionClass; use ReflectionMethod; use ReflectionProperty; class LivewireComponentParser { protected string $componentClass; protected ReflectionClass $reflectionClass; public static function create(string $componentAlias): self { return new self($componentAlias); } public function __construct(protected string $componentAlias) { $this->componentClass = app(LivewireManager::class)->getClass($this->componentAlias); $this->reflectionClass = new ReflectionClass($this->componentClass); } public function getComponentClass(): string { return $this->componentClass; } public function getPropertyNamesLike(string $similar): Collection { $properties = collect($this->reflectionClass->getProperties(ReflectionProperty::IS_PUBLIC)) // @phpstan-ignore-next-line ->reject(fn (ReflectionProperty $reflectionProperty) => $reflectionProperty->class !== $this->reflectionClass->name) ->map(fn (ReflectionProperty $reflectionProperty) => $reflectionProperty->name); $computedProperties = collect($this->reflectionClass->getMethods(ReflectionMethod::IS_PUBLIC)) // @phpstan-ignore-next-line ->reject(fn (ReflectionMethod $reflectionMethod) => $reflectionMethod->class !== $this->reflectionClass->name) ->filter(fn (ReflectionMethod $reflectionMethod) => str_starts_with($reflectionMethod->name, 'get') && str_ends_with($reflectionMethod->name, 'Property')) ->map(fn (ReflectionMethod $reflectionMethod) => lcfirst(Str::of($reflectionMethod->name)->after('get')->before('Property'))); return $this->filterItemsBySimilarity( $properties->merge($computedProperties), $similar ); } public function getMethodNamesLike(string $similar): Collection { $methods = collect($this->reflectionClass->getMethods(ReflectionMethod::IS_PUBLIC)) // @phpstan-ignore-next-line ->reject(fn (ReflectionMethod $reflectionMethod) => $reflectionMethod->class !== $this->reflectionClass->name) ->map(fn (ReflectionMethod $reflectionMethod) => $reflectionMethod->name); return $this->filterItemsBySimilarity($methods, $similar); } protected function filterItemsBySimilarity(Collection $items, string $similar): Collection { return $items ->map(function (string $name) use ($similar) { similar_text($similar, $name, $percentage); return ['match' => $percentage, 'value' => $name]; }) ->sortByDesc('match') ->filter(function (array $item) { return $item['match'] > 40; }) ->map(function (array $item) { return $item['value']; }) ->values(); } } laravel-ignition/src/Support/StringComparator.php 0000644 00000003041 15002154456 0016262 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; use Illuminate\Support\Collection; class StringComparator { /** * @param array<int|string, string> $strings * @param string $input * @param int $sensitivity * * @return string|null */ public static function findClosestMatch(array $strings, string $input, int $sensitivity = 4): ?string { $closestDistance = -1; $closestMatch = null; foreach ($strings as $string) { $levenshteinDistance = levenshtein($input, $string); if ($levenshteinDistance === 0) { $closestMatch = $string; $closestDistance = 0; break; } if ($levenshteinDistance <= $closestDistance || $closestDistance < 0) { $closestMatch = $string; $closestDistance = $levenshteinDistance; } } if ($closestDistance <= $sensitivity) { return $closestMatch; } return null; } /** * @param array<int, string> $strings * @param string $input * * @return string|null */ public static function findSimilarText(array $strings, string $input): ?string { if (empty($strings)) { return null; } return Collection::make($strings) ->sortByDesc(function (string $string) use ($input) { similar_text($input, $string, $percentage); return $percentage; }) ->first(); } } laravel-ignition/src/Support/SentReports.php 0000644 00000002172 15002154456 0015260 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; use Illuminate\Support\Arr; use Spatie\FlareClient\Report; class SentReports { /** @var array<int, Report> */ protected array $reports = []; public function add(Report $report): self { $this->reports[] = $report; return $this; } /** @return array<int, Report> */ public function all(): array { return $this->reports; } /** @return array<int, string> */ public function uuids(): array { return array_map(fn (Report $report) => $report->trackingUuid(), $this->reports); } /** @return array<int, string> */ public function urls(): array { return array_map(function (string $trackingUuid) { return "https://flareapp.io/tracked-occurrence/{$trackingUuid}"; }, $this->uuids()); } public function latestUuid(): ?string { return Arr::last($this->reports)?->trackingUuid(); } public function latestUrl(): ?string { return Arr::last($this->urls()); } public function clear(): void { $this->reports = []; } } laravel-ignition/src/Support/LaravelVersion.php 0000644 00000000264 15002154456 0015724 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; class LaravelVersion { public static function major(): string { return explode('.', app()->version())[0]; } } laravel-ignition/src/Support/LaravelDocumentationLinkFinder.php 0000644 00000004114 15002154456 0021054 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; use Illuminate\Support\Collection; use Illuminate\Support\Str; use Spatie\LaravelIgnition\Exceptions\ViewException; use Throwable; class LaravelDocumentationLinkFinder { public function findLinkForThrowable(Throwable $throwable): ?string { if ($throwable instanceof ViewException) { $throwable = $throwable->getPrevious(); } $majorVersion = LaravelVersion::major(); if (str_contains($throwable->getMessage(), Collection::class)) { return "https://laravel.com/docs/{$majorVersion}.x/collections#available-methods"; } $type = $this->getType($throwable); if (! $type) { return null; } return match ($type) { 'Auth' => "https://laravel.com/docs/{$majorVersion}.x/authentication", 'Broadcasting' => "https://laravel.com/docs/{$majorVersion}.x/broadcasting", 'Container' => "https://laravel.com/docs/{$majorVersion}.x/container", 'Database' => "https://laravel.com/docs/{$majorVersion}.x/eloquent", 'Pagination' => "https://laravel.com/docs/{$majorVersion}.x/pagination", 'Queue' => "https://laravel.com/docs/{$majorVersion}.x/queues", 'Routing' => "https://laravel.com/docs/{$majorVersion}.x/routing", 'Session' => "https://laravel.com/docs/{$majorVersion}.x/session", 'Validation' => "https://laravel.com/docs/{$majorVersion}.x/validation", 'View' => "https://laravel.com/docs/{$majorVersion}.x/views", default => null, }; } protected function getType(?Throwable $throwable): ?string { if (! $throwable) { return null; } if (str_contains($throwable::class, 'Illuminate')) { return Str::between($throwable::class, 'Illuminate\\', '\\'); } if (str_contains($throwable->getMessage(), 'Illuminate')) { return explode('\\', Str::between($throwable->getMessage(), 'Illuminate\\', '\\'))[0]; } return null; } } laravel-ignition/src/Support/FlareLogHandler.php 0000644 00000005221 15002154456 0015757 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Support; use InvalidArgumentException; use Monolog\Handler\AbstractProcessingHandler; use Monolog\Logger; use Spatie\FlareClient\Flare; use Spatie\FlareClient\Report; use Throwable; class FlareLogHandler extends AbstractProcessingHandler { protected Flare $flare; protected SentReports $sentReports; protected int $minimumReportLogLevel = Logger::ERROR; public function __construct(Flare $flare, SentReports $sentReports, $level = Logger::DEBUG, $bubble = true) { $this->flare = $flare; $this->sentReports = $sentReports; parent::__construct($level, $bubble); } public function setMinimumReportLogLevel(int $level): void { if (! in_array($level, Logger::getLevels())) { throw new InvalidArgumentException('The given minimum log level is not supported.'); } $this->minimumReportLogLevel = $level; } protected function write(array $record): void { if (! $this->shouldReport($record)) { return; } if ($this->hasException($record)) { $report = $this->flare->report($record['context']['exception']); if ($report) { $this->sentReports->add($report); } return; } if (config('flare.send_logs_as_events')) { if ($this->hasValidLogLevel($record)) { $this->flare->reportMessage( $record['message'], 'Log ' . Logger::getLevelName($record['level']), function (Report $flareReport) use ($record) { foreach ($record['context'] as $key => $value) { $flareReport->context($key, $value); } } ); } } } /** * @param array<string, mixed> $report * * @return bool */ protected function shouldReport(array $report): bool { if (! config('flare.key')) { return false; } return $this->hasException($report) || $this->hasValidLogLevel($report); } /** * @param array<string, mixed> $report * * @return bool */ protected function hasException(array $report): bool { $context = $report['context']; return isset($context['exception']) && $context['exception'] instanceof Throwable; } /** * @param array<string, mixed> $report * * @return bool */ protected function hasValidLogLevel(array $report): bool { return $report['level'] >= $this->minimumReportLogLevel; } } laravel-ignition/src/Solutions/UseDefaultValetDbCredentialsSolution.php 0000644 00000003150 15002154456 0022526 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Illuminate\Support\Str; use Spatie\Ignition\Contracts\RunnableSolution; class UseDefaultValetDbCredentialsSolution implements RunnableSolution { public function getSolutionActionDescription(): string { return 'Pressing the button will change `DB_USER` and `DB_PASSWORD` in your `.env` file.'; } public function getRunButtonText(): string { return 'Use default Valet credentials'; } public function getSolutionTitle(): string { return 'Could not connect to database'; } public function run(array $parameters = []): void { if (! file_exists(base_path('.env'))) { return; } $this->ensureLineExists('DB_USERNAME', 'root'); $this->ensureLineExists('DB_PASSWORD', ''); } protected function ensureLineExists(string $key, string $value): void { $envPath = base_path('.env'); $envLines = array_map(fn (string $envLine) => Str::startsWith($envLine, $key) ? "{$key}={$value}".PHP_EOL : $envLine, file($envPath) ?: []); file_put_contents($envPath, implode('', $envLines)); } public function getRunParameters(): array { return []; } public function getDocumentationLinks(): array { return [ 'Valet documentation' => 'https://laravel.com/docs/master/valet', ]; } public function getSolutionDescription(): string { return 'You seem to be using Valet, but the .env file does not contain the right default database credentials.'; } } laravel-ignition/src/Solutions/LivewireDiscoverSolution.php 0000644 00000002353 15002154456 0020336 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Livewire\LivewireComponentsFinder; use Spatie\Ignition\Contracts\RunnableSolution; class LivewireDiscoverSolution implements RunnableSolution { protected string $customTitle; public function __construct(string $customTitle = '') { $this->customTitle = $customTitle; } public function getSolutionTitle(): string { return $this->customTitle; } public function getSolutionDescription(): string { return 'You might have forgotten to discover your Livewire components.'; } public function getDocumentationLinks(): array { return [ 'Livewire: Artisan Commands' => 'https://laravel-livewire.com/docs/2.x/artisan-commands', ]; } public function getRunParameters(): array { return []; } public function getSolutionActionDescription(): string { return 'You can discover your Livewire components using `php artisan livewire:discover`.'; } public function getRunButtonText(): string { return 'Run livewire:discover'; } public function run(array $parameters = []): void { app(LivewireComponentsFinder::class)->build(); } } laravel-ignition/src/Solutions/SuggestUsingCorrectDbNameSolution.php 0000644 00000001460 15002154456 0022067 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestUsingCorrectDbNameSolution implements Solution { public function getSolutionTitle(): string { return 'Database name seems incorrect'; } public function getSolutionDescription(): string { $defaultDatabaseName = env('DB_DATABASE'); return "You're using the default database name `$defaultDatabaseName`. This database does not exist.\n\nEdit the `.env` file and use the correct database name in the `DB_DATABASE` key."; } /** @return array<string, string> */ public function getDocumentationLinks(): array { return [ 'Database: Getting Started docs' => 'https://laravel.com/docs/master/database#configuration', ]; } } laravel-ignition/src/Solutions/RunMigrationsSolution.php 0000644 00000002305 15002154456 0017647 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Illuminate\Support\Facades\Artisan; use Spatie\Ignition\Contracts\RunnableSolution; class RunMigrationsSolution implements RunnableSolution { protected string $customTitle; public function __construct(string $customTitle = '') { $this->customTitle = $customTitle; } public function getSolutionTitle(): string { return $this->customTitle; } public function getSolutionDescription(): string { return 'You might have forgotten to run your database migrations.'; } public function getDocumentationLinks(): array { return [ 'Database: Running Migrations docs' => 'https://laravel.com/docs/master/migrations#running-migrations', ]; } public function getRunParameters(): array { return []; } public function getSolutionActionDescription(): string { return 'You can try to run your migrations using `php artisan migrate`.'; } public function getRunButtonText(): string { return 'Run migrations'; } public function run(array $parameters = []): void { Artisan::call('migrate'); } } laravel-ignition/src/Solutions/SuggestCorrectVariableNameSolution.php 0000644 00000001605 15002154456 0022262 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestCorrectVariableNameSolution implements Solution { protected ?string $variableName; protected ?string $viewFile; protected ?string $suggested; public function __construct(string $variableName = null, string $viewFile = null, string $suggested = null) { $this->variableName = $variableName; $this->viewFile = $viewFile; $this->suggested = $suggested; } public function getSolutionTitle(): string { return 'Possible typo $'.$this->variableName; } public function getDocumentationLinks(): array { return []; } public function getSolutionDescription(): string { return "Did you mean `$$this->suggested`?"; } public function isRunnable(): bool { return false; } } laravel-ignition/src/Solutions/SolutionTransformers/LaravelSolutionTransformer.php 0000644 00000003306 15002154456 0025103 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionTransformers; use Spatie\Ignition\Contracts\RunnableSolution; use Spatie\Ignition\Solutions\SolutionTransformer; use Spatie\LaravelIgnition\Http\Controllers\ExecuteSolutionController; use Throwable; class LaravelSolutionTransformer extends SolutionTransformer { /** @return array<string|mixed> */ public function toArray(): array { $baseProperties = parent::toArray(); if (! $this->isRunnable()) { return $baseProperties; } /** @var RunnableSolution $solution Type shenanigans */ $solution = $this->solution; $runnableProperties = [ 'is_runnable' => true, 'action_description' => $solution->getSolutionActionDescription(), 'run_button_text' => $solution->getRunButtonText(), 'execute_endpoint' => $this->executeEndpoint(), 'run_parameters' => $solution->getRunParameters(), ]; return array_merge($baseProperties, $runnableProperties); } protected function isRunnable(): bool { if (! $this->solution instanceof RunnableSolution) { return false; } if (! $this->executeEndpoint()) { return false; } return true; } protected function executeEndpoint(): ?string { try { // The action class needs to be prefixed with a `\` to Laravel from trying // to add its own global namespace from RouteServiceProvider::$namespace. return action('\\'.ExecuteSolutionController::class); } catch (Throwable $exception) { report($exception); return null; } } } laravel-ignition/src/Solutions/SuggestImportSolution.php 0000644 00000001154 15002154456 0017663 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestImportSolution implements Solution { protected string $class; public function __construct(string $class = '') { $this->class = $class; } public function getSolutionTitle(): string { return 'A class import is missing'; } public function getSolutionDescription(): string { return 'You have a missing class import. Try importing this class: `'.$this->class.'`.'; } public function getDocumentationLinks(): array { return []; } } laravel-ignition/src/Solutions/SolutionProviders/UndefinedLivewireMethodSolutionProvider.php 0000644 00000003115 15002154456 0027044 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Livewire\Exceptions\MethodNotFoundException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\SuggestLivewireMethodNameSolution; use Spatie\LaravelIgnition\Support\LivewireComponentParser; use Throwable; class UndefinedLivewireMethodSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { return $throwable instanceof MethodNotFoundException; } public function getSolutions(Throwable $throwable): array { ['methodName' => $methodName, 'component' => $component] = $this->getMethodAndComponent($throwable); if ($methodName === null || $component === null) { return []; } $parsed = LivewireComponentParser::create($component); return $parsed->getMethodNamesLike($methodName) ->map(function (string $suggested) use ($parsed, $methodName) { return new SuggestLivewireMethodNameSolution( $methodName, $parsed->getComponentClass(), $suggested ); }) ->toArray(); } /** @return array<string, string|null> */ protected function getMethodAndComponent(Throwable $throwable): array { preg_match_all('/\[([\d\w\-_]*)\]/m', $throwable->getMessage(), $matches, PREG_SET_ORDER); return [ 'methodName' => $matches[0][1] ?? null, 'component' => $matches[1][1] ?? null, ]; } } laravel-ignition/src/Solutions/SolutionProviders/RouteNotDefinedSolutionProvider.php 0000644 00000003170 15002154456 0025332 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Facades\Route; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Support\StringComparator; use Symfony\Component\Routing\Exception\RouteNotFoundException; use Throwable; class RouteNotDefinedSolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/Route \[(.*)\] not defined/m'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof RouteNotFoundException) { return false; } return (bool)preg_match(self::REGEX, $throwable->getMessage(), $matches); } public function getSolutions(Throwable $throwable): array { preg_match(self::REGEX, $throwable->getMessage(), $matches); $missingRoute = $matches[1] ?? null; $suggestedRoute = $this->findRelatedRoute($missingRoute); if ($suggestedRoute) { return [ BaseSolution::create("{$missingRoute} was not defined.") ->setSolutionDescription("Did you mean `{$suggestedRoute}`?"), ]; } return [ BaseSolution::create("{$missingRoute} was not defined.") ->setSolutionDescription('Are you sure that the route is defined'), ]; } protected function findRelatedRoute(string $missingRoute): ?string { Route::getRoutes()->refreshNameLookups(); return StringComparator::findClosestMatch(array_keys(Route::getRoutes()->getRoutesByName()), $missingRoute); } } laravel-ignition/src/Solutions/SolutionProviders/MissingColumnSolutionProvider.php 0000644 00000001731 15002154456 0025064 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Database\QueryException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\RunMigrationsSolution; use Throwable; class MissingColumnSolutionProvider implements HasSolutionsForThrowable { /** * See https://dev.mysql.com/doc/refman/8.0/en/server-error-reference.html#error_er_bad_field_error. */ const MYSQL_BAD_FIELD_CODE = '42S22'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof QueryException) { return false; } return $this->isBadTableErrorCode($throwable->getCode()); } protected function isBadTableErrorCode(string $code): bool { return $code === static::MYSQL_BAD_FIELD_CODE; } public function getSolutions(Throwable $throwable): array { return [new RunMigrationsSolution('A column was not found')]; } } laravel-ignition/src/Solutions/SolutionProviders/MissingAppKeySolutionProvider.php 0000644 00000001252 15002154456 0025016 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use RuntimeException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\GenerateAppKeySolution; use Throwable; class MissingAppKeySolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof RuntimeException) { return false; } return $throwable->getMessage() === 'No application encryption key has been specified.'; } public function getSolutions(Throwable $throwable): array { return [new GenerateAppKeySolution()]; } } laravel-ignition/src/Solutions/SolutionProviders/DefaultDbNameSolutionProvider.php 0000644 00000001620 15002154456 0024725 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Database\QueryException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\SuggestUsingCorrectDbNameSolution; use Throwable; class DefaultDbNameSolutionProvider implements HasSolutionsForThrowable { const MYSQL_UNKNOWN_DATABASE_CODE = 1049; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof QueryException) { return false; } if ($throwable->getCode() !== self::MYSQL_UNKNOWN_DATABASE_CODE) { return false; } if (! in_array(env('DB_DATABASE'), ['homestead', 'laravel'])) { return false; } return true; } public function getSolutions(Throwable $throwable): array { return [new SuggestUsingCorrectDbNameSolution()]; } } laravel-ignition/src/Solutions/SolutionProviders/ViewNotFoundSolutionProvider.php 0000644 00000007224 15002154456 0024667 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Arr; use Illuminate\Support\Facades\View; use InvalidArgumentException; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Exceptions\ViewException; use Spatie\LaravelIgnition\Support\StringComparator; use Symfony\Component\Finder\Finder; use Symfony\Component\Finder\SplFileInfo; use Throwable; class ViewNotFoundSolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/View \[(.*)\] not found/m'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof InvalidArgumentException && ! $throwable instanceof ViewException) { return false; } return (bool)preg_match(self::REGEX, $throwable->getMessage(), $matches); } public function getSolutions(Throwable $throwable): array { preg_match(self::REGEX, $throwable->getMessage(), $matches); $missingView = $matches[1] ?? null; $suggestedView = $this->findRelatedView($missingView); if ($suggestedView) { return [ BaseSolution::create() ->setSolutionTitle("{$missingView} was not found.") ->setSolutionDescription("Did you mean `{$suggestedView}`?"), ]; } return [ BaseSolution::create() ->setSolutionTitle("{$missingView} was not found.") ->setSolutionDescription('Are you sure the view exists and is a `.blade.php` file?'), ]; } protected function findRelatedView(string $missingView): ?string { $views = $this->getAllViews(); return StringComparator::findClosestMatch($views, $missingView); } /** @return array<int, string> */ protected function getAllViews(): array { /** @var \Illuminate\View\FileViewFinder $fileViewFinder */ $fileViewFinder = View::getFinder(); $extensions = $fileViewFinder->getExtensions(); $viewsForHints = collect($fileViewFinder->getHints()) ->flatMap(function ($paths, string $namespace) use ($extensions) { $paths = Arr::wrap($paths); return collect($paths) ->flatMap(fn (string $path) => $this->getViewsInPath($path, $extensions)) ->map(fn (string $view) => "{$namespace}::{$view}") ->toArray(); }); $viewsForViewPaths = collect($fileViewFinder->getPaths()) ->flatMap(fn (string $path) => $this->getViewsInPath($path, $extensions)); return $viewsForHints->merge($viewsForViewPaths)->toArray(); } /** * @param string $path * @param array<int, string> $extensions * * @return array<int, string> */ protected function getViewsInPath(string $path, array $extensions): array { $filePatterns = array_map(fn (string $extension) => "*.{$extension}", $extensions); $extensionsWithDots = array_map(fn (string $extension) => ".{$extension}", $extensions); $files = (new Finder()) ->in($path) ->files(); foreach ($filePatterns as $filePattern) { $files->name($filePattern); } $views = []; foreach ($files as $file) { if ($file instanceof SplFileInfo) { $view = $file->getRelativePathname(); $view = str_replace($extensionsWithDots, '', $view); $view = str_replace('/', '.', $view); $views[] = $view; } } return $views; } } laravel-ignition/src/Solutions/SolutionProviders/MissingLivewireComponentSolutionProvider.php 0000644 00000002116 15002154456 0027276 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Livewire\Exceptions\ComponentNotFoundException; use Livewire\LivewireComponentsFinder; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\LivewireDiscoverSolution; use Throwable; class MissingLivewireComponentSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { if (! $this->livewireIsInstalled()) { return false; } if (! $throwable instanceof ComponentNotFoundException) { return false; } return true; } public function getSolutions(Throwable $throwable): array { return [new LivewireDiscoverSolution('A Livewire component was not found')]; } public function livewireIsInstalled(): bool { if (! class_exists(ComponentNotFoundException::class)) { return false; } if (! class_exists(LivewireComponentsFinder::class)) { return false; } return true; } } laravel-ignition/src/Solutions/SolutionProviders/UndefinedLivewirePropertySolutionProvider.php 0000644 00000003213 15002154456 0027447 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Livewire\Exceptions\PropertyNotFoundException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\SuggestLivewirePropertyNameSolution; use Spatie\LaravelIgnition\Support\LivewireComponentParser; use Throwable; class UndefinedLivewirePropertySolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { return $throwable instanceof PropertyNotFoundException; } public function getSolutions(Throwable $throwable): array { ['variable' => $variable, 'component' => $component] = $this->getMethodAndComponent($throwable); if ($variable === null || $component === null) { return []; } $parsed = LivewireComponentParser::create($component); return $parsed->getPropertyNamesLike($variable) ->map(function (string $suggested) use ($parsed, $variable) { return new SuggestLivewirePropertyNameSolution( $variable, $parsed->getComponentClass(), '$'.$suggested ); }) ->toArray(); } /** * @param \Throwable $throwable * * @return array<string, string|null> */ protected function getMethodAndComponent(Throwable $throwable): array { preg_match_all('/\[([\d\w\-_\$]*)\]/m', $throwable->getMessage(), $matches, PREG_SET_ORDER, 0); return [ 'variable' => $matches[0][1] ?? null, 'component' => $matches[1][1] ?? null, ]; } } laravel-ignition/src/Solutions/SolutionProviders/MissingMixManifestSolutionProvider.php 0000644 00000001307 15002154456 0026052 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Str; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class MissingMixManifestSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { return Str::startsWith($throwable->getMessage(), 'Mix manifest not found'); } public function getSolutions(Throwable $throwable): array { return [ BaseSolution::create('Missing Mix Manifest File') ->setSolutionDescription('Did you forget to run `npm install && npm run dev`?'), ]; } } laravel-ignition/src/Solutions/SolutionProviders/RunningLaravelDuskInProductionProvider.php 0000644 00000002122 15002154456 0026647 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Exception; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class RunningLaravelDuskInProductionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof Exception) { return false; } return $throwable->getMessage() === 'It is unsafe to run Dusk in production.'; } public function getSolutions(Throwable $throwable): array { return [ BaseSolution::create() ->setSolutionTitle('Laravel Dusk should not be run in production.') ->setSolutionDescription('Install the dependencies with the `--no-dev` flag.'), BaseSolution::create() ->setSolutionTitle('Laravel Dusk can be run in other environments.') ->setSolutionDescription('Consider setting the `APP_ENV` to something other than `production` like `local` for example.'), ]; } } laravel-ignition/src/Solutions/SolutionProviders/UndefinedViewVariableSolutionProvider.php 0000644 00000006760 15002154456 0026506 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\Ignition\Contracts\Solution; use Spatie\LaravelIgnition\Exceptions\ViewException; use Spatie\LaravelIgnition\Solutions\MakeViewVariableOptionalSolution; use Spatie\LaravelIgnition\Solutions\SuggestCorrectVariableNameSolution; use Throwable; class UndefinedViewVariableSolutionProvider implements HasSolutionsForThrowable { protected string $variableName; protected string $viewFile; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof ViewException) { return false; } return $this->getNameAndView($throwable) !== null; } public function getSolutions(Throwable $throwable): array { $solutions = []; /** @phpstan-ignore-next-line */ extract($this->getNameAndView($throwable)); if (! isset($variableName)) { return []; } if (isset($viewFile)) { /** @phpstan-ignore-next-line */ $solutions = $this->findCorrectVariableSolutions($throwable, $variableName, $viewFile); $solutions[] = $this->findOptionalVariableSolution($variableName, $viewFile); } return $solutions; } /** * @param \Spatie\LaravelIgnition\Exceptions\ViewException $throwable * @param string $variableName * @param string $viewFile * * @return array<int, \Spatie\Ignition\Contracts\Solution> */ protected function findCorrectVariableSolutions( ViewException $throwable, string $variableName, string $viewFile ): array { return collect($throwable->getViewData()) ->map(function ($value, $key) use ($variableName) { similar_text($variableName, $key, $percentage); return ['match' => $percentage, 'value' => $value]; }) ->sortByDesc('match') ->filter(fn ($var) => $var['match'] > 40) ->keys() ->map(fn ($suggestion) => new SuggestCorrectVariableNameSolution($variableName, $viewFile, $suggestion)) ->map(function ($solution) { return $solution->isRunnable() ? $solution : BaseSolution::create($solution->getSolutionTitle()) ->setSolutionDescription($solution->getSolutionDescription()); }) ->toArray(); } protected function findOptionalVariableSolution(string $variableName, string $viewFile): Solution { $optionalSolution = new MakeViewVariableOptionalSolution($variableName, $viewFile); return $optionalSolution->isRunnable() ? $optionalSolution : BaseSolution::create($optionalSolution->getSolutionTitle()) ->setSolutionDescription($optionalSolution->getSolutionDescription()); } /** * @param \Throwable $throwable * * @return array<string, string>|null */ protected function getNameAndView(Throwable $throwable): ?array { $pattern = '/Undefined variable:? (.*?) \(View: (.*?)\)/'; preg_match($pattern, $throwable->getMessage(), $matches); if (count($matches) === 3) { [, $variableName, $viewFile] = $matches; $variableName = ltrim($variableName, '$'); return compact('variableName', 'viewFile'); } return null; } } laravel-ignition/src/Solutions/SolutionProviders/UnknownValidationSolutionProvider.php 0000644 00000004734 15002154456 0025755 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use BadMethodCallException; use Illuminate\Support\Collection; use Illuminate\Support\Str; use Illuminate\Validation\Validator; use ReflectionClass; use ReflectionMethod; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Support\StringComparator; use Throwable; class UnknownValidationSolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/Illuminate\\\\Validation\\\\Validator::(?P<method>validate(?!(Attribute|UsingCustomRule))[A-Z][a-zA-Z]+)/m'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof BadMethodCallException) { return false; } return ! is_null($this->getMethodFromExceptionMessage($throwable->getMessage())); } public function getSolutions(Throwable $throwable): array { return [ BaseSolution::create() ->setSolutionTitle('Unknown Validation Rule') ->setSolutionDescription($this->getSolutionDescription($throwable)), ]; } protected function getSolutionDescription(Throwable $throwable): string { $method = (string)$this->getMethodFromExceptionMessage($throwable->getMessage()); $possibleMethod = StringComparator::findSimilarText( $this->getAvailableMethods()->toArray(), $method ); if (empty($possibleMethod)) { return ''; } $rule = Str::snake(str_replace('validate', '', $possibleMethod)); return "Did you mean `{$rule}` ?"; } protected function getMethodFromExceptionMessage(string $message): ?string { if (! preg_match(self::REGEX, $message, $matches)) { return null; } return $matches['method']; } protected function getAvailableMethods(): Collection { $class = new ReflectionClass(Validator::class); $extensions = Collection::make((app('validator')->make([], []))->extensions) ->keys() ->map(fn (string $extension) => 'validate'.Str::studly($extension)); return Collection::make($class->getMethods()) ->filter(fn (ReflectionMethod $method) => preg_match('/(validate(?!(Attribute|UsingCustomRule))[A-Z][a-zA-Z]+)/', $method->name)) ->map(fn (ReflectionMethod $method) => $method->name) ->merge($extensions); } } laravel-ignition/src/Solutions/SolutionProviders/GenericLaravelExceptionSolutionProvider.php 0000644 00000003427 15002154456 0027043 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Broadcasting\BroadcastException; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Support\LaravelVersion; use Throwable; class GenericLaravelExceptionSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { return ! is_null($this->getSolutionTexts($throwable)); } public function getSolutions(Throwable $throwable): array { if (! $texts = $this->getSolutionTexts($throwable)) { return []; } $solution = BaseSolution::create($texts['title']) ->setSolutionDescription($texts['description']) ->setDocumentationLinks($texts['links']); return ([$solution]); } /** * @param \Throwable $throwable * * @return array<string, mixed>|null */ protected function getSolutionTexts(Throwable $throwable) : ?array { foreach ($this->getSupportedExceptions() as $supportedClass => $texts) { if ($throwable instanceof $supportedClass) { return $texts; } } return null; } /** @return array<string, mixed> */ protected function getSupportedExceptions(): array { $majorVersion = LaravelVersion::major(); return [ BroadcastException::class => [ 'title' => 'Here are some links that might help solve this problem', 'description' => '', 'links' => [ 'Laravel docs on authentication' => "https://laravel.com/docs/{$majorVersion}.x/authentication", ], ], ]; } } laravel-ignition/src/Solutions/SolutionProviders/SolutionProviderRepository.php 0000644 00000006025 15002154456 0024455 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Collection; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\Contracts\SolutionProviderRepository as SolutionProviderRepositoryContract; use Throwable; class SolutionProviderRepository implements SolutionProviderRepositoryContract { protected Collection $solutionProviders; /** * @param array<int, ProvidesSolution> $solutionProviders */ public function __construct(array $solutionProviders = []) { $this->solutionProviders = Collection::make($solutionProviders); } public function registerSolutionProvider(string $solutionProviderClass): SolutionProviderRepositoryContract { $this->solutionProviders->push($solutionProviderClass); return $this; } public function registerSolutionProviders(array $solutionProviderClasses): SolutionProviderRepositoryContract { $this->solutionProviders = $this->solutionProviders->merge($solutionProviderClasses); return $this; } public function getSolutionsForThrowable(Throwable $throwable): array { $solutions = []; if ($throwable instanceof Solution) { $solutions[] = $throwable; } if ($throwable instanceof ProvidesSolution) { $solutions[] = $throwable->getSolution(); } /** @phpstan-ignore-next-line */ $providedSolutions = $this->solutionProviders ->filter(function (string $solutionClass) { if (! in_array(HasSolutionsForThrowable::class, class_implements($solutionClass) ?: [])) { return false; } if (in_array($solutionClass, config('ignition.ignored_solution_providers', []))) { return false; } return true; }) ->map(fn (string $solutionClass) => app($solutionClass)) ->filter(function (HasSolutionsForThrowable $solutionProvider) use ($throwable) { try { return $solutionProvider->canSolve($throwable); } catch (Throwable $e) { return false; } }) ->map(function (HasSolutionsForThrowable $solutionProvider) use ($throwable) { try { return $solutionProvider->getSolutions($throwable); } catch (Throwable $e) { return []; } }) ->flatten() ->toArray(); return array_merge($solutions, $providedSolutions); } public function getSolutionForClass(string $solutionClass): ?Solution { if (! class_exists($solutionClass)) { return null; } if (! in_array(Solution::class, class_implements($solutionClass) ?: [])) { return null; } return app($solutionClass); } } laravel-ignition/src/Solutions/SolutionProviders/LazyLoadingViolationSolutionProvider.php 0000644 00000002573 15002154456 0026404 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Database\LazyLoadingViolationException; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Support\LaravelVersion; use Throwable; class LazyLoadingViolationSolutionProvider implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { if ($throwable instanceof LazyLoadingViolationException) { return true; } if (! $previous = $throwable->getPrevious()) { return false; } return $previous instanceof LazyLoadingViolationException; } public function getSolutions(Throwable $throwable): array { $majorVersion = LaravelVersion::major(); return [BaseSolution::create( 'Lazy loading was disabled to detect N+1 problems' ) ->setSolutionDescription( 'Either avoid lazy loading the relation or allow lazy loading.' ) ->setDocumentationLinks([ 'Read the docs on preventing lazy loading' => "https://laravel.com/docs/{$majorVersion}.x/eloquent-relationships#preventing-lazy-loading", 'Watch a video on how to deal with the N+1 problem' => 'https://www.youtube.com/watch?v=ZE7KBeraVpc', ]),]; } } laravel-ignition/src/Solutions/SolutionProviders/MissingViteManifestSolutionProvider.php 0000644 00000003403 15002154456 0026223 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Str; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\Ignition\Contracts\Solution; use Throwable; class MissingViteManifestSolutionProvider implements HasSolutionsForThrowable { protected array $links = [ 'Asset bundling with Vite' => 'https://laravel.com/docs/9.x/vite#running-vite', ]; public function canSolve(Throwable $throwable): bool { return Str::startsWith($throwable->getMessage(), 'Vite manifest not found'); } public function getSolutions(Throwable $throwable): array { return [ $this->getSolution(), ]; } public function getSolution(): Solution { /** @var string */ $baseCommand = collect([ 'pnpm-lock.yaml' => 'pnpm', 'yarn.lock' => 'yarn', ])->first(fn ($_, $lockfile) => file_exists(base_path($lockfile)), 'npm run'); return app()->environment('local') ? $this->getLocalSolution($baseCommand) : $this->getProductionSolution($baseCommand); } protected function getLocalSolution(string $baseCommand): Solution { return BaseSolution::create('Start the development server') ->setSolutionDescription("Run `{$baseCommand} dev` in your terminal and refresh the page.") ->setDocumentationLinks($this->links); } protected function getProductionSolution(string $baseCommand): Solution { return BaseSolution::create('Build the production assets') ->setSolutionDescription("Run `{$baseCommand} build` in your deployment script.") ->setDocumentationLinks($this->links); } } laravel-ignition/src/Solutions/SolutionProviders/TableNotFoundSolutionProvider.php 0000644 00000001730 15002154456 0025000 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Database\QueryException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\RunMigrationsSolution; use Throwable; class TableNotFoundSolutionProvider implements HasSolutionsForThrowable { /** * See https://dev.mysql.com/doc/refman/8.0/en/server-error-reference.html#error_er_bad_table_error. */ const MYSQL_BAD_TABLE_CODE = '42S02'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof QueryException) { return false; } return $this->isBadTableErrorCode($throwable->getCode()); } protected function isBadTableErrorCode(string $code): bool { return $code === static::MYSQL_BAD_TABLE_CODE; } public function getSolutions(Throwable $throwable): array { return [new RunMigrationsSolution('A table was not found')]; } } laravel-ignition/src/Solutions/SolutionProviders/MissingImportSolutionProvider.php 0000644 00000002646 15002154456 0025107 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\SuggestImportSolution; use Spatie\LaravelIgnition\Support\Composer\ComposerClassMap; use Throwable; class MissingImportSolutionProvider implements HasSolutionsForThrowable { protected ?string $foundClass; protected ComposerClassMap $composerClassMap; public function canSolve(Throwable $throwable): bool { $pattern = '/Class \'([^\s]+)\' not found/m'; if (! preg_match($pattern, $throwable->getMessage(), $matches)) { return false; } $class = $matches[1]; $this->composerClassMap = new ComposerClassMap(); $this->search($class); return ! is_null($this->foundClass); } /** * @param \Throwable $throwable * * @return array<int, SuggestImportSolution> */ public function getSolutions(Throwable $throwable): array { if (is_null($this->foundClass)) { return []; } return [new SuggestImportSolution($this->foundClass)]; } protected function search(string $missingClass): void { $this->foundClass = $this->composerClassMap->searchClassMap($missingClass); if (is_null($this->foundClass)) { $this->foundClass = $this->composerClassMap->searchPsrMaps($missingClass); } } } laravel-ignition/src/Solutions/SolutionProviders/IncorrectValetDbCredentialsSolutionProvider.php 0000644 00000003142 15002154456 0027643 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Database\QueryException; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Solutions\UseDefaultValetDbCredentialsSolution; use Throwable; class IncorrectValetDbCredentialsSolutionProvider implements HasSolutionsForThrowable { const MYSQL_ACCESS_DENIED_CODE = 1045; public function canSolve(Throwable $throwable): bool { if (PHP_OS !== 'Darwin') { return false; } if (! $throwable instanceof QueryException) { return false; } if (! $this->isAccessDeniedCode($throwable->getCode())) { return false; } if (! $this->envFileExists()) { return false; } if (! $this->isValetInstalled()) { return false; } if ($this->usingCorrectDefaultCredentials()) { return false; } return true; } public function getSolutions(Throwable $throwable): array { return [new UseDefaultValetDbCredentialsSolution()]; } protected function envFileExists(): bool { return file_exists(base_path('.env')); } protected function isAccessDeniedCode(string $code): bool { return $code === static::MYSQL_ACCESS_DENIED_CODE; } protected function isValetInstalled(): bool { return file_exists('/usr/local/bin/valet'); } protected function usingCorrectDefaultCredentials(): bool { return env('DB_USERNAME') === 'root' && env('DB_PASSWORD') === ''; } } laravel-ignition/src/Solutions/SolutionProviders/InvalidRouteActionSolutionProvider.php 0000644 00000005623 15002154456 0026044 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions\SolutionProviders; use Illuminate\Support\Str; use Spatie\Ignition\Contracts\BaseSolution; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Spatie\LaravelIgnition\Support\Composer\ComposerClassMap; use Spatie\LaravelIgnition\Support\StringComparator; use Throwable; use UnexpectedValueException; class InvalidRouteActionSolutionProvider implements HasSolutionsForThrowable { protected const REGEX = '/\[([a-zA-Z\\\\]+)\]/m'; public function canSolve(Throwable $throwable): bool { if (! $throwable instanceof UnexpectedValueException) { return false; } if (! preg_match(self::REGEX, $throwable->getMessage(), $matches)) { return false; } return Str::startsWith($throwable->getMessage(), 'Invalid route action: '); } public function getSolutions(Throwable $throwable): array { preg_match(self::REGEX, $throwable->getMessage(), $matches); $invalidController = $matches[1] ?? null; $suggestedController = $this->findRelatedController($invalidController); if ($suggestedController === $invalidController) { return [ BaseSolution::create("`{$invalidController}` is not invokable.") ->setSolutionDescription("The controller class `{$invalidController}` is not invokable. Did you forget to add the `__invoke` method or is the controller's method missing in your routes file?"), ]; } if ($suggestedController) { return [ BaseSolution::create("`{$invalidController}` was not found.") ->setSolutionDescription("Controller class `{$invalidController}` for one of your routes was not found. Did you mean `{$suggestedController}`?"), ]; } return [ BaseSolution::create("`{$invalidController}` was not found.") ->setSolutionDescription("Controller class `{$invalidController}` for one of your routes was not found. Are you sure this controller exists and is imported correctly?"), ]; } protected function findRelatedController(string $invalidController): ?string { $composerClassMap = app(ComposerClassMap::class); $controllers = collect($composerClassMap->listClasses()) ->filter(function (string $file, string $fqcn) { return Str::endsWith($fqcn, 'Controller'); }) ->mapWithKeys(function (string $file, string $fqcn) { return [$fqcn => class_basename($fqcn)]; }) ->toArray(); $basenameMatch = StringComparator::findClosestMatch($controllers, $invalidController, 4); $controllers = array_flip($controllers); $fqcnMatch = StringComparator::findClosestMatch($controllers, $invalidController, 4); return $fqcnMatch ?? $basenameMatch; } } laravel-ignition/src/Solutions/GenerateAppKeySolution.php 0000644 00000002000 15002154456 0017702 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Illuminate\Support\Facades\Artisan; use Spatie\Ignition\Contracts\RunnableSolution; class GenerateAppKeySolution implements RunnableSolution { public function getSolutionTitle(): string { return 'Your app key is missing'; } public function getDocumentationLinks(): array { return [ 'Laravel installation' => 'https://laravel.com/docs/master/installation#configuration', ]; } public function getSolutionActionDescription(): string { return 'Generate your application encryption key using `php artisan key:generate`.'; } public function getRunButtonText(): string { return 'Generate app key'; } public function getSolutionDescription(): string { return ''; } public function getRunParameters(): array { return []; } public function run(array $parameters = []): void { Artisan::call('key:generate'); } } laravel-ignition/src/Solutions/MakeViewVariableOptionalSolution.php 0000644 00000007362 15002154456 0021742 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Illuminate\Support\Facades\Blade; use Illuminate\Support\Str; use Spatie\Ignition\Contracts\RunnableSolution; class MakeViewVariableOptionalSolution implements RunnableSolution { protected ?string $variableName; protected ?string $viewFile; public function __construct(string $variableName = null, string $viewFile = null) { $this->variableName = $variableName; $this->viewFile = $viewFile; } public function getSolutionTitle(): string { return "$$this->variableName is undefined"; } public function getDocumentationLinks(): array { return []; } public function getSolutionActionDescription(): string { $output = [ 'Make the variable optional in the blade template.', "Replace `{{ $$this->variableName }}` with `{{ $$this->variableName ?? '' }}`", ]; return implode(PHP_EOL, $output); } public function getRunButtonText(): string { return 'Make variable optional'; } public function getSolutionDescription(): string { return ''; } public function getRunParameters(): array { return [ 'variableName' => $this->variableName, 'viewFile' => $this->viewFile, ]; } /** * @param array<string, mixed> $parameters * * @return bool */ public function isRunnable(array $parameters = []): bool { return $this->makeOptional($this->getRunParameters()) !== false; } /** * @param array<string, string> $parameters * * @return void */ public function run(array $parameters = []): void { $output = $this->makeOptional($parameters); if ($output !== false) { file_put_contents($parameters['viewFile'], $output); } } protected function isSafePath(string $path): bool { if (! Str::startsWith($path, ['/', './'])) { return false; } if (! Str::endsWith($path, '.blade.php')) { return false; } return true; } /** * @param array<string, string> $parameters * * @return bool|string */ public function makeOptional(array $parameters = []): bool|string { if (! $this->isSafePath($parameters['viewFile'])) { return false; } $originalContents = (string)file_get_contents($parameters['viewFile']); $newContents = str_replace('$'.$parameters['variableName'], '$'.$parameters['variableName']." ?? ''", $originalContents); $originalTokens = token_get_all(Blade::compileString($originalContents)); $newTokens = token_get_all(Blade::compileString($newContents)); $expectedTokens = $this->generateExpectedTokens($originalTokens, $parameters['variableName']); if ($expectedTokens !== $newTokens) { return false; } return $newContents; } /** * @param array<int, mixed> $originalTokens * @param string $variableName * * @return array<int, mixed> */ protected function generateExpectedTokens(array $originalTokens, string $variableName): array { $expectedTokens = []; foreach ($originalTokens as $token) { $expectedTokens[] = $token; if ($token[0] === T_VARIABLE && $token[1] === '$'.$variableName) { $expectedTokens[] = [T_WHITESPACE, ' ', $token[2]]; $expectedTokens[] = [T_COALESCE, '??', $token[2]]; $expectedTokens[] = [T_WHITESPACE, ' ', $token[2]]; $expectedTokens[] = [T_CONSTANT_ENCAPSED_STRING, "''", $token[2]]; } } return $expectedTokens; } } laravel-ignition/src/Solutions/SuggestLivewirePropertyNameSolution.php 0000644 00000001311 15002154456 0022540 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestLivewirePropertyNameSolution implements Solution { public function __construct( protected string $variableName, protected string $componentClass, protected string $suggested, ) { } public function getSolutionTitle(): string { return "Possible typo {$this->variableName}"; } public function getDocumentationLinks(): array { return []; } public function getSolutionDescription(): string { return "Did you mean `$this->suggested`?"; } public function isRunnable(): bool { return false; } } laravel-ignition/src/Solutions/SuggestLivewireMethodNameSolution.php 0000644 00000001370 15002154456 0022141 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Solutions; use Spatie\Ignition\Contracts\Solution; class SuggestLivewireMethodNameSolution implements Solution { public function __construct( protected string $methodName, protected string $componentClass, protected string $suggested ) { } public function getSolutionTitle(): string { return "Possible typo `{$this->componentClass}::{$this->methodName}`"; } public function getDocumentationLinks(): array { return []; } public function getSolutionDescription(): string { return "Did you mean `{$this->componentClass}::{$this->suggested}`?"; } public function isRunnable(): bool { return false; } } laravel-ignition/src/helpers.php 0000644 00000000773 15002154456 0012763 0 ustar 00 <?php use Spatie\LaravelIgnition\Renderers\ErrorPageRenderer; if (! function_exists('ddd')) { function ddd() { $args = func_get_args(); if (count($args) === 0) { throw new Exception('You should pass at least 1 argument to `ddd`'); } call_user_func_array('dump', $args); $renderer = app()->make(ErrorPageRenderer::class); $exception = new Exception('Dump, Die, Debug'); $renderer->render($exception); die(); } } laravel-ignition/src/Commands/SolutionMakeCommand.php 0000644 00000001554 15002154456 0016771 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Commands; use Illuminate\Console\GeneratorCommand; use Symfony\Component\Console\Input\InputOption; class SolutionMakeCommand extends GeneratorCommand { protected $name = 'ignition:make-solution'; protected $description = 'Create a new custom Ignition solution class'; protected $type = 'Solution'; protected function getStub(): string { return $this->option('runnable') ? __DIR__.'/stubs/runnable-solution.stub' : __DIR__.'/stubs/solution.stub'; } protected function getDefaultNamespace($rootNamespace) { return "{$rootNamespace}\\Solutions"; } /** @return array<int, mixed> */ protected function getOptions(): array { return [ ['runnable', null, InputOption::VALUE_NONE, 'Create runnable solution'], ]; } } laravel-ignition/src/Commands/stubs/solution.stub 0000644 00000000552 15002154456 0016257 0 ustar 00 <?php namespace DummyNamespace; use Spatie\Ignition\Contracts\Solution; class DummyClass implements Solution { public function getSolutionTitle(): string { return ''; } public function getSolutionDescription(): string { return ''; } public function getDocumentationLinks(): array { return []; } } laravel-ignition/src/Commands/stubs/solution-provider.stub 0000644 00000000534 15002154456 0020107 0 ustar 00 <?php namespace DummyNamespace; use Spatie\Ignition\Contracts\HasSolutionsForThrowable; use Throwable; class DummyClass implements HasSolutionsForThrowable { public function canSolve(Throwable $throwable): bool { return false; } public function getSolutions(Throwable $throwable): array { return []; } } laravel-ignition/src/Commands/stubs/runnable-solution.stub 0000644 00000001272 15002154456 0020063 0 ustar 00 <?php namespace DummyNamespace; use Spatie\Ignition\Contracts\RunnableSolution; class DummyClass implements RunnableSolution { public function getSolutionTitle(): string { return ''; } public function getDocumentationLinks(): array { return []; } public function getSolutionActionDescription(): string { return ''; } public function getRunButtonText(): string { return ''; } public function getSolutionDescription(): string { return ''; } public function getRunParameters(): array { return []; } public function run(array $parameters = []) { // } } laravel-ignition/src/Commands/TestCommand.php 0000644 00000010617 15002154456 0015276 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Commands; use Composer\InstalledVersions; use Exception; use Illuminate\Config\Repository; use Illuminate\Console\Command; use Illuminate\Log\LogManager; use Spatie\FlareClient\Flare; use Spatie\FlareClient\Http\Exceptions\BadResponseCode; class TestCommand extends Command { protected $signature = 'flare:test'; protected $description = 'Send a test notification to Flare'; protected Repository $config; public function handle(Repository $config): void { $this->config = $config; $this->checkFlareKey(); if (app()->make('log') instanceof LogManager) { $this->checkFlareLogger(); } $this->sendTestException(); } protected function checkFlareKey(): self { $message = empty($this->config->get('flare.key')) ? '❌ Flare key not specified. Make sure you specify a value in the `key` key of the `flare` config file.' : '✅ Flare key specified'; $this->info($message); return $this; } public function checkFlareLogger(): self { $defaultLogChannel = $this->config->get('logging.default'); $activeStack = $this->config->get("logging.channels.{$defaultLogChannel}"); if (is_null($activeStack)) { $this->info("❌ The default logging channel `{$defaultLogChannel}` is not configured in the `logging` config file"); } if (! isset($activeStack['channels']) || ! in_array('flare', $activeStack['channels'])) { $this->info("❌ The logging channel `{$defaultLogChannel}` does not contain the 'flare' channel"); } if (is_null($this->config->get('logging.channels.flare'))) { $this->info('❌ There is no logging channel named `flare` in the `logging` config file'); } if ($this->config->get('logging.channels.flare.driver') !== 'flare') { $this->info('❌ The `flare` logging channel defined in the `logging` config file is not set to `flare`.'); } $this->info('✅ The Flare logging driver was configured correctly.'); return $this; } protected function sendTestException(): void { $testException = new Exception('This is an exception to test if the integration with Flare works.'); try { app(Flare::class)->sendTestReport($testException); $this->info(''); } catch (Exception $exception) { $this->warn('❌ We were unable to send an exception to Flare. '); if ($exception instanceof BadResponseCode) { $this->info(''); $message = 'Unknown error'; $body = $exception->response->getBody(); if (is_array($body) && isset($body['message'])) { $message = $body['message']; } $this->warn("{$exception->response->getHttpResponseCode()} - {$message}"); } else { $this->warn($exception->getMessage()); } $this->warn('Make sure that your key is correct and that you have a valid subscription.'); $this->info(''); $this->info('For more info visit the docs on https://flareapp.io/docs/ignition-for-laravel/introduction'); $this->info('You can see the status page of Flare at https://status.flareapp.io'); $this->info('Flare support can be reached at support@flareapp.io'); $this->line(''); $this->line('Extra info'); $this->table([], [ ['Platform', PHP_OS], ['PHP', phpversion()], ['Laravel', app()->version()], ['spatie/ignition', InstalledVersions::getVersion('spatie/ignition')], ['spatie/laravel-ignition', InstalledVersions::getVersion('spatie/laravel-ignition')], ['spatie/flare-client-php', InstalledVersions::getVersion('spatie/flare-client-php')], /** @phpstan-ignore-next-line */ ['Curl', curl_version()['version'] ?? 'Unknown'], /** @phpstan-ignore-next-line */ ['SSL', curl_version()['ssl_version'] ?? 'Unknown'], ]); if ($this->output->isVerbose()) { throw $exception; } return; } $this->info('We tried to send an exception to Flare. Please check if it arrived!'); } } laravel-ignition/src/Commands/SolutionProviderMakeCommand.php 0000644 00000001103 15002154456 0020472 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Commands; use Illuminate\Console\GeneratorCommand; class SolutionProviderMakeCommand extends GeneratorCommand { protected $name = 'ignition:make-solution-provider'; protected $description = 'Create a new custom Ignition solution provider class'; protected $type = 'Solution Provider'; protected function getStub(): string { return __DIR__.'/stubs/solution-provider.stub'; } protected function getDefaultNamespace($rootNamespace) { return "{$rootNamespace}\\SolutionProviders"; } } laravel-ignition/src/Views/ViewExceptionMapper.php 0000644 00000013732 15002154456 0016353 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Views; use Exception; use Illuminate\Contracts\View\Engine; use Illuminate\Foundation\Application; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Illuminate\View\Engines\PhpEngine; use Illuminate\View\ViewException; use ReflectionClass; use ReflectionProperty; use Spatie\Ignition\Contracts\ProvidesSolution; use Spatie\LaravelIgnition\Exceptions\ViewException as IgnitionViewException; use Spatie\LaravelIgnition\Exceptions\ViewExceptionWithSolution; use Throwable; class ViewExceptionMapper { protected Engine $compilerEngine; protected BladeSourceMapCompiler $bladeSourceMapCompiler; protected array $knownPaths; public function __construct(BladeSourceMapCompiler $bladeSourceMapCompiler) { $resolver = app('view.engine.resolver'); $this->compilerEngine = $resolver->resolve('blade'); $this->bladeSourceMapCompiler = $bladeSourceMapCompiler; } public function map(ViewException $viewException): IgnitionViewException { $baseException = $this->getRealException($viewException); if ($baseException instanceof IgnitionViewException) { return $baseException; } preg_match('/\(View: (?P<path>.*?)\)/', $viewException->getMessage(), $matches); $compiledViewPath = $matches['path']; $exception = $this->createException($baseException); if ($baseException instanceof ProvidesSolution) { /** @var ViewExceptionWithSolution $exception */ $exception->setSolution($baseException->getSolution()); } $this->modifyViewsInTrace($exception); $exception->setView($compiledViewPath); $exception->setViewData($this->getViewData($exception)); return $exception; } protected function createException(Throwable $baseException): IgnitionViewException { $viewExceptionClass = $baseException instanceof ProvidesSolution ? ViewExceptionWithSolution::class : IgnitionViewException::class; $viewFile = $this->findCompiledView($baseException->getFile()); $file = $viewFile ?? $baseException->getFile(); $line = $viewFile ? $this->getBladeLineNumber($file, $baseException->getLine()) : $baseException->getLine(); return new $viewExceptionClass( $baseException->getMessage(), 0, 1, $file, $line, $baseException ); } protected function modifyViewsInTrace(IgnitionViewException $exception): void { $trace = Collection::make($exception->getPrevious()->getTrace()) ->map(function ($trace) { if ($originalPath = $this->findCompiledView(Arr::get($trace, 'file', ''))) { $trace['file'] = $originalPath; $trace['line'] = $this->getBladeLineNumber($trace['file'], $trace['line']); } return $trace; })->toArray(); $traceProperty = new ReflectionProperty('Exception', 'trace'); $traceProperty->setAccessible(true); $traceProperty->setValue($exception, $trace); } /** * Look at the previous exceptions to find the original exception. * This is usually the first Exception that is not a ViewException. */ protected function getRealException(Throwable $exception): Throwable { $rootException = $exception->getPrevious() ?? $exception; while ($rootException instanceof ViewException && $rootException->getPrevious()) { $rootException = $rootException->getPrevious(); } return $rootException; } protected function findCompiledView(string $compiledPath): ?string { $this->knownPaths ??= $this->getKnownPaths(); return $this->knownPaths[$compiledPath] ?? null; } protected function getKnownPaths(): array { $compilerEngineReflection = new ReflectionClass($this->compilerEngine); if (! $compilerEngineReflection->hasProperty('lastCompiled') && $compilerEngineReflection->hasProperty('engine')) { $compilerEngine = $compilerEngineReflection->getProperty('engine'); $compilerEngine->setAccessible(true); $compilerEngine = $compilerEngine->getValue($this->compilerEngine); $lastCompiled = new ReflectionProperty($compilerEngine, 'lastCompiled'); $lastCompiled->setAccessible(true); $lastCompiled = $lastCompiled->getValue($compilerEngine); } else { $lastCompiled = $compilerEngineReflection->getProperty('lastCompiled'); $lastCompiled->setAccessible(true); $lastCompiled = $lastCompiled->getValue($this->compilerEngine); } $knownPaths = []; foreach ($lastCompiled as $lastCompiledPath) { $compiledPath = $this->compilerEngine->getCompiler()->getCompiledPath($lastCompiledPath); $knownPaths[realpath($compiledPath ?? $lastCompiledPath)] = realpath($lastCompiledPath); } return $knownPaths; } protected function getBladeLineNumber(string $view, int $compiledLineNumber): int { return $this->bladeSourceMapCompiler->detectLineNumber($view, $compiledLineNumber); } protected function getViewData(Throwable $exception): array { foreach ($exception->getTrace() as $frame) { if (Arr::get($frame, 'class') === PhpEngine::class) { $data = Arr::get($frame, 'args.1', []); return $this->filterViewData($data); } } return []; } protected function filterViewData(array $data): array { // By default, Laravel views get two data keys: // __env and app. We try to filter them out. return array_filter($data, function ($value, $key) { if ($key === 'app') { return ! $value instanceof Application; } return $key !== '__env'; }, ARRAY_FILTER_USE_BOTH); } } laravel-ignition/src/Views/BladeSourceMapCompiler.php 0000644 00000010660 15002154456 0016733 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Views; use Illuminate\View\Compilers\BladeCompiler; use Throwable; class BladeSourceMapCompiler { protected BladeCompiler $bladeCompiler; public function __construct() { $this->bladeCompiler = app('blade.compiler'); } public function detectLineNumber(string $filename, int $compiledLineNumber): int { $map = $this->compileSourcemap((string)file_get_contents($filename)); return $this->findClosestLineNumberMapping($map, $compiledLineNumber); } protected function compileSourcemap(string $value): string { try { $value = $this->addEchoLineNumbers($value); $value = $this->addStatementLineNumbers($value); $value = $this->addBladeComponentLineNumbers($value); $value = $this->bladeCompiler->compileString($value); return $this->trimEmptyLines($value); } catch (Throwable $e) { report($e); return $value; } } protected function addEchoLineNumbers(string $value): string { $echoPairs = [['{{', '}}'], ['{{{', '}}}'], ['{!!', '!!}']]; foreach ($echoPairs as $pair) { // Matches {{ $value }}, {!! $value !!} and {{{ $value }}} depending on $pair $pattern = sprintf('/(@)?%s\s*(.+?)\s*%s(\r?\n)?/s', $pair[0], $pair[1]); if (preg_match_all($pattern, $value, $matches, PREG_OFFSET_CAPTURE)) { foreach (array_reverse($matches[0]) as $match) { $position = mb_strlen(substr($value, 0, $match[1])); $value = $this->insertLineNumberAtPosition($position, $value); } } } return $value; } protected function addStatementLineNumbers(string $value): string { // Matches @bladeStatements() like @if, @component(...), @etc; $shouldInsertLineNumbers = preg_match_all( '/\B@(@?\w+(?:::\w+)?)([ \t]*)(\( ( (?>[^()]+) | (?3) )* \))?/x', $value, $matches, PREG_OFFSET_CAPTURE ); if ($shouldInsertLineNumbers) { foreach (array_reverse($matches[0]) as $match) { $position = mb_strlen(substr($value, 0, $match[1])); $value = $this->insertLineNumberAtPosition($position, $value); } } return $value; } protected function addBladeComponentLineNumbers(string $value): string { // Matches the start of `<x-blade-component` $shouldInsertLineNumbers = preg_match_all( '/<\s*x[-:]([\w\-:.]*)/mx', $value, $matches, PREG_OFFSET_CAPTURE ); if ($shouldInsertLineNumbers) { foreach (array_reverse($matches[0]) as $match) { $position = mb_strlen(substr($value, 0, $match[1])); $value = $this->insertLineNumberAtPosition($position, $value); } } return $value; } protected function insertLineNumberAtPosition(int $position, string $value): string { $before = mb_substr($value, 0, $position); $lineNumber = count(explode("\n", $before)); return mb_substr($value, 0, $position)."|---LINE:{$lineNumber}---|".mb_substr($value, $position); } protected function trimEmptyLines(string $value): string { $value = preg_replace('/^\|---LINE:([0-9]+)---\|$/m', '', $value); return ltrim((string)$value, PHP_EOL); } protected function findClosestLineNumberMapping(string $map, int $compiledLineNumber): int { $map = explode("\n", $map); // Max 20 lines between compiled and source line number. // Blade components can span multiple lines and the compiled line number is often // a couple lines below the source-mapped `<x-component>` code. $maxDistance = 20; $pattern = '/\|---LINE:(?P<line>[0-9]+)---\|/m'; $lineNumberToCheck = $compiledLineNumber - 1; while (true) { if ($lineNumberToCheck < $compiledLineNumber - $maxDistance) { // Something wrong. Return the $compiledLineNumber (unless it's out of range) return min($compiledLineNumber, count($map)); } if (preg_match($pattern, $map[$lineNumberToCheck] ?? '', $matches)) { return (int)$matches['line']; } $lineNumberToCheck--; } } } laravel-ignition/src/ignition-routes.php 0000644 00000001413 15002154456 0014450 0 ustar 00 <?php use Illuminate\Support\Facades\Route; use Spatie\LaravelIgnition\Http\Controllers\ExecuteSolutionController; use Spatie\LaravelIgnition\Http\Controllers\HealthCheckController; use Spatie\LaravelIgnition\Http\Controllers\UpdateConfigController; use Spatie\LaravelIgnition\Http\Middleware\RunnableSolutionsEnabled; Route::group([ 'as' => 'ignition.', 'prefix' => config('ignition.housekeeping_endpoint_prefix'), 'middleware' => [RunnableSolutionsEnabled::class], ], function () { Route::get('health-check', HealthCheckController::class)->name('healthCheck'); Route::post('execute-solution', ExecuteSolutionController::class) ->name('executeSolution'); Route::post('update-config', UpdateConfigController::class)->name('updateConfig'); }); laravel-ignition/src/Recorders/LogRecorder/LogRecorder.php 0000644 00000003730 15002154456 0017663 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\LogRecorder; use Illuminate\Contracts\Foundation\Application; use Illuminate\Log\Events\MessageLogged; use Throwable; class LogRecorder { /** @var \Spatie\LaravelIgnition\Recorders\LogRecorder\LogMessage[] */ protected array $logMessages = []; protected Application $app; protected ?int $maxLogs; public function __construct(Application $app, ?int $maxLogs = null) { $this->app = $app; $this->maxLogs = $maxLogs; } public function start(): self { /** @phpstan-ignore-next-line */ $this->app['events']->listen(MessageLogged::class, [$this, 'record']); return $this; } public function record(MessageLogged $event): void { if ($this->shouldIgnore($event)) { return; } $this->logMessages[] = LogMessage::fromMessageLoggedEvent($event); if (is_int($this->maxLogs)) { $this->logMessages = array_slice($this->logMessages, -$this->maxLogs); } } /** @return array<array<int,string>> */ public function getLogMessages(): array { return $this->toArray(); } /** @return array<int, mixed> */ public function toArray(): array { $logMessages = []; foreach ($this->logMessages as $log) { $logMessages[] = $log->toArray(); } return $logMessages; } protected function shouldIgnore(mixed $event): bool { if (! isset($event->context['exception'])) { return false; } if (! $event->context['exception'] instanceof Throwable) { return false; } return true; } public function reset(): void { $this->logMessages = []; } public function getMaxLogs(): ?int { return $this->maxLogs; } public function setMaxLogs(?int $maxLogs): self { $this->maxLogs = $maxLogs; return $this; } } laravel-ignition/src/Recorders/LogRecorder/LogMessage.php 0000644 00000002364 15002154456 0017504 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\LogRecorder; use Illuminate\Log\Events\MessageLogged; class LogMessage { protected ?string $message; protected string $level; /** @var array<string, string> */ protected array $context = []; protected ?float $microtime; /** * @param string|null $message * @param string $level * @param array<string, string> $context * @param float|null $microtime */ public function __construct( ?string $message, string $level, array $context = [], ?float $microtime = null ) { $this->message = $message; $this->level = $level; $this->context = $context; $this->microtime = $microtime ?? microtime(true); } public static function fromMessageLoggedEvent(MessageLogged $event): self { return new self( $event->message, $event->level, $event->context ); } /** @return array<string, mixed> */ public function toArray(): array { return [ 'message' => $this->message, 'level' => $this->level, 'context' => $this->context, 'microtime' => $this->microtime, ]; } } laravel-ignition/src/Recorders/QueryRecorder/Query.php 0000644 00000003157 15002154456 0017150 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\QueryRecorder; use Illuminate\Database\Events\QueryExecuted; class Query { protected string $sql; protected float $time; protected string $connectionName; /** @var array<string, string>|null */ protected ?array $bindings; protected float $microtime; public static function fromQueryExecutedEvent(QueryExecuted $queryExecuted, bool $reportBindings = false): self { return new self( $queryExecuted->sql, $queryExecuted->time, /** @phpstan-ignore-next-line */ $queryExecuted->connectionName ?? '', $reportBindings ? $queryExecuted->bindings : null ); } /** * @param string $sql * @param float $time * @param string $connectionName * @param array<string, string>|null $bindings * @param float|null $microtime */ protected function __construct( string $sql, float $time, string $connectionName, ?array $bindings = null, ?float $microtime = null ) { $this->sql = $sql; $this->time = $time; $this->connectionName = $connectionName; $this->bindings = $bindings; $this->microtime = $microtime ?? microtime(true); } /** * @return array<string, mixed> */ public function toArray(): array { return [ 'sql' => $this->sql, 'time' => $this->time, 'connection_name' => $this->connectionName, 'bindings' => $this->bindings, 'microtime' => $this->microtime, ]; } } laravel-ignition/src/Recorders/QueryRecorder/QueryRecorder.php 0000644 00000003662 15002154456 0020637 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\QueryRecorder; use Illuminate\Contracts\Foundation\Application; use Illuminate\Database\Events\QueryExecuted; class QueryRecorder { /** @var \Spatie\LaravelIgnition\Recorders\QueryRecorder\Query[] */ protected array $queries = []; protected Application $app; protected bool $reportBindings = true; protected ?int $maxQueries; public function __construct( Application $app, bool $reportBindings = true, ?int $maxQueries = 200 ) { $this->app = $app; $this->reportBindings = $reportBindings; $this->maxQueries = $maxQueries; } public function start(): self { /** @phpstan-ignore-next-line */ $this->app['events']->listen(QueryExecuted::class, [$this, 'record']); return $this; } public function record(QueryExecuted $queryExecuted): void { $this->queries[] = Query::fromQueryExecutedEvent($queryExecuted, $this->reportBindings); if (is_int($this->maxQueries)) { $this->queries = array_slice($this->queries, -$this->maxQueries); } } /** * @return array<int, array<string, mixed>> */ public function getQueries(): array { $queries = []; foreach ($this->queries as $query) { $queries[] = $query->toArray(); } return $queries; } public function reset(): void { $this->queries = []; } public function getReportBindings(): bool { return $this->reportBindings; } public function setReportBindings(bool $reportBindings): self { $this->reportBindings = $reportBindings; return $this; } public function getMaxQueries(): ?int { return $this->maxQueries; } public function setMaxQueries(?int $maxQueries): self { $this->maxQueries = $maxQueries; return $this; } } laravel-ignition/src/Recorders/DumpRecorder/Dump.php 0000644 00000001421 15002154456 0016540 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\DumpRecorder; class Dump { protected string $htmlDump; protected ?string $file; protected ?int $lineNumber; protected float $microtime; public function __construct(string $htmlDump, ?string $file, ?int $lineNumber, ?float $microtime = null) { $this->htmlDump = $htmlDump; $this->file = $file; $this->lineNumber = $lineNumber; $this->microtime = $microtime ?? microtime(true); } /** @return array<string, mixed> */ public function toArray(): array { return [ 'html_dump' => $this->htmlDump, 'file' => $this->file, 'line_number' => $this->lineNumber, 'microtime' => $this->microtime, ]; } } laravel-ignition/src/Recorders/DumpRecorder/MultiDumpHandler.php 0000644 00000000765 15002154456 0021063 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\DumpRecorder; class MultiDumpHandler { /** @var array<int, callable|null> */ protected array $handlers = []; public function dump(mixed $value): void { foreach ($this->handlers as $handler) { if ($handler) { $handler($value); } } } public function addHandler(callable $callable = null): self { $this->handlers[] = $callable; return $this; } } laravel-ignition/src/Recorders/DumpRecorder/DumpHandler.php 0000644 00000000704 15002154456 0020041 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\DumpRecorder; use Symfony\Component\VarDumper\Cloner\VarCloner; class DumpHandler { protected DumpRecorder $dumpRecorder; public function __construct(DumpRecorder $dumpRecorder) { $this->dumpRecorder = $dumpRecorder; } public function dump(mixed $value): void { $data = (new VarCloner)->cloneVar($value); $this->dumpRecorder->record($data); } } laravel-ignition/src/Recorders/DumpRecorder/HtmlDumper.php 0000644 00000001363 15002154456 0017721 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\DumpRecorder; use Symfony\Component\VarDumper\Cloner\Data; use Symfony\Component\VarDumper\Cloner\VarCloner; use Symfony\Component\VarDumper\Dumper\HtmlDumper as BaseHtmlDumper; class HtmlDumper extends BaseHtmlDumper { protected $dumpHeader = ''; public function dumpVariable($variable): string { $cloner = new VarCloner(); $clonedData = $cloner->cloneVar($variable)->withMaxDepth(3); return $this->dump($clonedData); } public function dump(Data $data, $output = null, array $extraDisplayOptions = []): string { return (string)parent::dump($data, true, [ 'maxDepth' => 3, 'maxStringLength' => 160, ]); } } laravel-ignition/src/Recorders/DumpRecorder/DumpRecorder.php 0000644 00000007424 15002154456 0020237 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\DumpRecorder; use Illuminate\Contracts\Foundation\Application; use Illuminate\Support\Arr; use ReflectionMethod; use ReflectionProperty; use Symfony\Component\VarDumper\Cloner\Data; use Symfony\Component\VarDumper\VarDumper; class DumpRecorder { /** @var array<array<int,mixed>> */ protected array $dumps = []; protected Application $app; protected static bool $registeredHandler = false; public function __construct(Application $app) { $this->app = $app; } public function start(): self { $multiDumpHandler = new MultiDumpHandler(); $this->app->singleton(MultiDumpHandler::class, fn () => $multiDumpHandler); if (! self::$registeredHandler) { static::$registeredHandler = true; $this->ensureOriginalHandlerExists(); $originalHandler = VarDumper::setHandler(fn ($dumpedVariable) => $multiDumpHandler->dump($dumpedVariable)); $multiDumpHandler?->addHandler($originalHandler); $multiDumpHandler->addHandler(fn ($var) => (new DumpHandler($this))->dump($var)); } return $this; } public function record(Data $data): void { $backtrace = debug_backtrace(DEBUG_BACKTRACE_IGNORE_ARGS, 11); $sourceFrame = $this->findSourceFrame($backtrace); $file = (string) Arr::get($sourceFrame, 'file'); $lineNumber = (int) Arr::get($sourceFrame, 'line'); $htmlDump = (new HtmlDumper())->dump($data); $this->dumps[] = new Dump($htmlDump, $file, $lineNumber); } public function getDumps(): array { return $this->toArray(); } public function reset() { $this->dumps = []; } public function toArray(): array { $dumps = []; foreach ($this->dumps as $dump) { $dumps[] = $dump->toArray(); } return $dumps; } /* * Only the `VarDumper` knows how to create the orignal HTML or CLI VarDumper. * Using reflection and the private VarDumper::register() method we can force it * to create and register a new VarDumper::$handler before we'll overwrite it. * Of course, we only need to do this if there isn't a registered VarDumper::$handler. * * @throws \ReflectionException */ protected function ensureOriginalHandlerExists(): void { $reflectionProperty = new ReflectionProperty(VarDumper::class, 'handler'); $reflectionProperty->setAccessible(true); $handler = $reflectionProperty->getValue(); if (! $handler) { // No handler registered yet, so we'll force VarDumper to create one. $reflectionMethod = new ReflectionMethod(VarDumper::class, 'register'); $reflectionMethod->setAccessible(true); $reflectionMethod->invoke(null); } } /** * Find the first meaningful stack frame that is not the `DumpRecorder` itself. * * @template T of array{class?: class-string, function?: string, line?: int, file?: string} * * @param array<T> $stacktrace * * @return null|T */ protected function findSourceFrame(array $stacktrace): ?array { $seenVarDumper = false; foreach ($stacktrace as $frame) { // Keep looping until we're past the VarDumper::dump() call in Symfony's helper functions file. if (Arr::get($frame, 'class') === VarDumper::class && Arr::get($frame, 'function') === 'dump') { $seenVarDumper = true; continue; } if (! $seenVarDumper) { continue; } // Return the next frame in the stack after the VarDumper::dump() call: return $frame; } return null; } } laravel-ignition/src/Recorders/JobRecorder/JobRecorder.php 0000644 00000011600 15002154456 0017640 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Recorders\JobRecorder; use DateTime; use Error; use Exception; use Illuminate\Contracts\Encryption\Encrypter; use Illuminate\Contracts\Foundation\Application; use Illuminate\Contracts\Queue\Job; use Illuminate\Queue\CallQueuedClosure; use Illuminate\Queue\Events\JobExceptionOccurred; use Illuminate\Queue\Jobs\RedisJob; use Illuminate\Support\Str; use ReflectionClass; use ReflectionProperty; use RuntimeException; class JobRecorder { protected ?Job $job = null; public function __construct( protected Application $app, protected int $maxChainedJobReportingDepth = 5, ) { } public function start(): self { /** @phpstan-ignore-next-line */ $this->app['events']->listen(JobExceptionOccurred::class, [$this, 'record']); return $this; } public function record(JobExceptionOccurred $event): void { $this->job = $event->job; } /** * @return array<string, mixed>|null */ public function getJob(): ?array { if ($this->job === null) { return null; } return array_merge( $this->getJobProperties(), [ 'name' => $this->job->resolveName(), 'connection' => $this->job->getConnectionName(), 'queue' => $this->job->getQueue(), ] ); } public function reset(): void { $this->job = null; } protected function getJobProperties(): array { $payload = collect($this->resolveJobPayload()); $properties = []; foreach ($payload as $key => $value) { if (! in_array($key, ['job', 'data', 'displayName'])) { $properties[$key] = $value; } } if ($pushedAt = DateTime::createFromFormat('U.u', $payload->get('pushedAt', ''))) { $properties['pushedAt'] = $pushedAt->format(DATE_ATOM); } try { $properties['data'] = $this->resolveCommandProperties( $this->resolveObjectFromCommand($payload['data']['command']), $this->maxChainedJobReportingDepth ); } catch (Exception $exception) { } return $properties; } protected function resolveJobPayload(): array { if (! $this->job instanceof RedisJob) { return $this->job->payload(); } try { return json_decode($this->job->getReservedJob(), true, 512, JSON_THROW_ON_ERROR); } catch (Exception $e) { return $this->job->payload(); } } protected function resolveCommandProperties(object $command, int $maxChainDepth): array { $propertiesToIgnore = ['job', 'closure']; $properties = collect((new ReflectionClass($command))->getProperties()) ->reject(function (ReflectionProperty $property) use ($propertiesToIgnore) { return in_array($property->name, $propertiesToIgnore); }) ->mapWithKeys(function (ReflectionProperty $property) use ($command) { try { $property->setAccessible(true); return [$property->name => $property->getValue($command)]; } catch (Error $error) { return [$property->name => 'uninitialized']; } }); if ($properties->has('chained')) { $properties['chained'] = $this->resolveJobChain($properties->get('chained'), $maxChainDepth); } return $properties->all(); } /** * @param array<string, mixed> $chainedCommands * @param int $maxDepth * * @return array */ protected function resolveJobChain(array $chainedCommands, int $maxDepth): array { if ($maxDepth === 0) { return ['Ignition stopped recording jobs after this point since the max chain depth was reached']; } return array_map( function (string $command) use ($maxDepth) { $commandObject = $this->resolveObjectFromCommand($command); return [ 'name' => $commandObject instanceof CallQueuedClosure ? $commandObject->displayName() : get_class($commandObject), 'data' => $this->resolveCommandProperties($commandObject, $maxDepth - 1), ]; }, $chainedCommands ); } // Taken from Illuminate\Queue\CallQueuedHandler protected function resolveObjectFromCommand(string $command): object { if (Str::startsWith($command, 'O:')) { return unserialize($command); } if ($this->app->bound(Encrypter::class)) { /** @phpstan-ignore-next-line */ return unserialize($this->app[Encrypter::class]->decrypt($command)); } throw new RuntimeException('Unable to extract job payload.'); } } laravel-ignition/src/IgnitionServiceProvider.php 0000644 00000027175 15002154456 0016142 0 ustar 00 <?php namespace Spatie\LaravelIgnition; use Illuminate\Contracts\Debug\ExceptionHandler; use Illuminate\Foundation\Application; use Illuminate\Support\Facades\Log; use Illuminate\Support\ServiceProvider; use Illuminate\View\ViewException; use Laravel\Octane\Events\RequestReceived; use Laravel\Octane\Events\RequestTerminated; use Laravel\Octane\Events\TaskReceived; use Laravel\Octane\Events\TickReceived; use Monolog\Logger; use Spatie\FlareClient\Flare; use Spatie\FlareClient\FlareMiddleware\AddSolutions; use Spatie\Ignition\Config\FileConfigManager; use Spatie\Ignition\Config\IgnitionConfig; use Spatie\Ignition\Contracts\ConfigManager; use Spatie\Ignition\Contracts\SolutionProviderRepository as SolutionProviderRepositoryContract; use Spatie\Ignition\Ignition; use Spatie\LaravelIgnition\Commands\SolutionMakeCommand; use Spatie\LaravelIgnition\Commands\SolutionProviderMakeCommand; use Spatie\LaravelIgnition\Commands\TestCommand; use Spatie\LaravelIgnition\ContextProviders\LaravelContextProviderDetector; use Spatie\LaravelIgnition\Exceptions\InvalidConfig; use Spatie\LaravelIgnition\FlareMiddleware\AddJobs; use Spatie\LaravelIgnition\FlareMiddleware\AddLogs; use Spatie\LaravelIgnition\FlareMiddleware\AddQueries; use Spatie\LaravelIgnition\Recorders\DumpRecorder\DumpRecorder; use Spatie\LaravelIgnition\Recorders\JobRecorder\JobRecorder; use Spatie\LaravelIgnition\Recorders\LogRecorder\LogRecorder; use Spatie\LaravelIgnition\Recorders\QueryRecorder\QueryRecorder; use Spatie\LaravelIgnition\Renderers\IgnitionExceptionRenderer; use Spatie\LaravelIgnition\Renderers\IgnitionWhoopsHandler; use Spatie\LaravelIgnition\Solutions\SolutionProviders\SolutionProviderRepository; use Spatie\LaravelIgnition\Support\FlareLogHandler; use Spatie\LaravelIgnition\Support\SentReports; use Spatie\LaravelIgnition\Views\ViewExceptionMapper; class IgnitionServiceProvider extends ServiceProvider { public function register(): void { $this->registerConfig(); $this->registerFlare(); $this->registerIgnition(); $this->registerRenderer(); $this->registerRecorders(); $this->registerLogHandler(); } public function boot() { if ($this->app->runningInConsole()) { $this->registerCommands(); $this->publishConfigs(); } $this->registerRoutes(); $this->configureTinker(); $this->configureOctane(); $this->registerViewExceptionMapper(); $this->startRecorders(); $this->configureQueue(); } protected function registerConfig(): void { $this->mergeConfigFrom(__DIR__ . '/../config/flare.php', 'flare'); $this->mergeConfigFrom(__DIR__ . '/../config/ignition.php', 'ignition'); } protected function registerCommands(): void { if ($this->app['config']->get('flare.key')) { $this->commands([ TestCommand::class, ]); } if ($this->app['config']->get('ignition.register_commands')) { $this->commands([ SolutionMakeCommand::class, SolutionProviderMakeCommand::class, ]); } } protected function publishConfigs(): void { $this->publishes([ __DIR__ . '/../config/ignition.php' => config_path('ignition.php'), ], 'ignition-config'); $this->publishes([ __DIR__ . '/../config/flare.php' => config_path('flare.php'), ], 'flare-config'); } protected function registerRenderer(): void { if (interface_exists('Whoops\Handler\HandlerInterface')) { $this->app->bind( 'Whoops\Handler\HandlerInterface', fn (Application $app) => $app->make(IgnitionWhoopsHandler::class) ); } if (interface_exists('Illuminate\Contracts\Foundation\ExceptionRenderer')) { $this->app->bind( 'Illuminate\Contracts\Foundation\ExceptionRenderer', fn (Application $app) => $app->make(IgnitionExceptionRenderer::class) ); } } protected function registerFlare(): void { $this->app->singleton(Flare::class, function () { return Flare::make() ->setApiToken(config('flare.key') ?? '') ->setBaseUrl(config('flare.base_url', 'https://flareapp.io/api')) ->applicationPath(base_path()) ->setStage(app()->environment()) ->setContextProviderDetector(new LaravelContextProviderDetector()) ->registerMiddleware($this->getFlareMiddleware()) ->registerMiddleware(new AddSolutions(new SolutionProviderRepository($this->getSolutionProviders()))); }); $this->app->singleton(SentReports::class); } protected function registerIgnition(): void { $this->app->singleton( ConfigManager::class, fn () => new FileConfigManager(config('ignition.settings_file_path', '')) ); $ignitionConfig = (new IgnitionConfig()) ->merge(config('ignition', [])) ->loadConfigFile(); $solutionProviders = $this->getSolutionProviders(); $solutionProviderRepository = new SolutionProviderRepository($solutionProviders); $this->app->singleton(IgnitionConfig::class, fn () => $ignitionConfig); $this->app->singleton(SolutionProviderRepositoryContract::class, fn () => $solutionProviderRepository); $this->app->singleton( Ignition::class, fn () => (new Ignition()) ->applicationPath(base_path()) ); } protected function registerRecorders(): void { $this->app->singleton(DumpRecorder::class); $this->app->singleton(LogRecorder::class, function (Application $app): LogRecorder { return new LogRecorder( $app, config()->get('flare.flare_middleware.' . AddLogs::class . '.maximum_number_of_collected_logs') ); }); $this->app->singleton( QueryRecorder::class, function (Application $app): QueryRecorder { return new QueryRecorder( $app, config('flare.flare_middleware.' . AddQueries::class . '.report_query_bindings', true), config('flare.flare_middleware.' . AddQueries::class . '.maximum_number_of_collected_queries', 200) ); } ); $this->app->singleton(JobRecorder::class, function (Application $app): JobRecorder { return new JobRecorder( $app, config('flare.flare_middleware.' . AddJobs::class . '.max_chained_job_reporting_depth', 5) ); }); } public function configureTinker(): void { if (! $this->app->runningInConsole()) { if (isset($_SERVER['argv']) && ['artisan', 'tinker'] === $_SERVER['argv']) { app(Flare::class)->sendReportsImmediately(); } } } protected function configureOctane(): void { if (isset($_SERVER['LARAVEL_OCTANE'])) { $this->setupOctane(); } } protected function registerViewExceptionMapper(): void { $handler = $this->app->make(ExceptionHandler::class); if (! method_exists($handler, 'map')) { return; } $handler->map(function (ViewException $viewException) { return $this->app->make(ViewExceptionMapper::class)->map($viewException); }); } protected function registerRoutes(): void { $this->loadRoutesFrom(realpath(__DIR__ . '/ignition-routes.php')); } protected function registerLogHandler(): void { $this->app->singleton('flare.logger', function ($app) { $handler = new FlareLogHandler( $app->make(Flare::class), $app->make(SentReports::class), ); $logLevelString = config('logging.channels.flare.level', 'error'); $logLevel = $this->getLogLevel($logLevelString); $handler->setMinimumReportLogLevel($logLevel); return tap( new Logger('Flare'), fn (Logger $logger) => $logger->pushHandler($handler) ); }); Log::extend('flare', fn ($app) => $app['flare.logger']); } protected function startRecorders(): void { foreach ($this->app->config['ignition.recorders'] ?? [] as $recorder) { $this->app->make($recorder)->start(); } } protected function configureQueue(): void { if (! $this->app->bound('queue')) { return; } $queue = $this->app->get('queue'); // Reset before executing a queue job to make sure the job's log/query/dump recorders are empty. // When using a sync queue this also reports the queued reports from previous exceptions. $queue->before(function () { $this->resetFlareAndLaravelIgnition(); app(Flare::class)->sendReportsImmediately(); }); // Send queued reports (and reset) after executing a queue job. $queue->after(function () { $this->resetFlareAndLaravelIgnition(); }); // Note: the $queue->looping() event can't be used because it's not triggered on Vapor } protected function getLogLevel(string $logLevelString): int { $logLevel = Logger::getLevels()[strtoupper($logLevelString)] ?? null; if (! $logLevel) { throw InvalidConfig::invalidLogLevel($logLevelString); } return $logLevel; } protected function getFlareMiddleware(): array { return collect(config('flare.flare_middleware')) ->map(function ($value, $key) { if (is_string($key)) { $middlewareClass = $key; $parameters = $value ?? []; } else { $middlewareClass = $value; $parameters = []; } return new $middlewareClass(...array_values($parameters)); }) ->values() ->toArray(); } protected function getSolutionProviders(): array { return collect(config('ignition.solution_providers')) ->reject( fn (string $class) => in_array($class, config('ignition.ignored_solution_providers')) ) ->toArray(); } protected function setupOctane(): void { $this->app['events']->listen(RequestReceived::class, function () { $this->resetFlareAndLaravelIgnition(); }); $this->app['events']->listen(TaskReceived::class, function () { $this->resetFlareAndLaravelIgnition(); }); $this->app['events']->listen(TickReceived::class, function () { $this->resetFlareAndLaravelIgnition(); }); $this->app['events']->listen(RequestTerminated::class, function () { $this->resetFlareAndLaravelIgnition(); }); } protected function resetFlareAndLaravelIgnition(): void { $this->app->get(SentReports::class)->clear(); $this->app->get(Ignition::class)->reset(); if (config('flare.flare_middleware.' . AddLogs::class)) { $this->app->make(LogRecorder::class)->reset(); } if (config('flare.flare_middleware.' . AddQueries::class)) { $this->app->make(QueryRecorder::class)->reset(); } if (config('flare.flare_middleware.' . AddJobs::class)) { $this->app->make(JobRecorder::class)->reset(); } $this->app->make(DumpRecorder::class)->reset(); } } laravel-ignition/src/Facades/Flare.php 0000644 00000001246 15002154456 0013674 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Facades; use Illuminate\Support\Facades\Facade; use Spatie\LaravelIgnition\Support\SentReports; /** * @method static void glow(string $name, string $messageLevel = \Spatie\FlareClient\Enums\MessageLevels::INFO, array $metaData = []) * @method static void context($key, $value) * @method static void group(string $groupName, array $properties) * * @see \Spatie\FlareClient\Flare */ class Flare extends Facade { protected static function getFacadeAccessor() { return \Spatie\FlareClient\Flare::class; } public static function sentReports(): SentReports { return app(SentReports::class); } } laravel-ignition/src/Http/Middleware/RunnableSolutionsEnabled.php 0000644 00000000532 15002154456 0021247 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Middleware; use Closure; use Spatie\LaravelIgnition\Support\RunnableSolutionsGuard; class RunnableSolutionsEnabled { public function handle($request, Closure $next) { if (! RunnableSolutionsGuard::check()) { abort(404); } return $next($request); } } laravel-ignition/src/Http/Controllers/ExecuteSolutionController.php 0000644 00000002645 15002154456 0021751 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Controllers; use Illuminate\Foundation\Validation\ValidatesRequests; use Spatie\Ignition\Contracts\SolutionProviderRepository; use Spatie\LaravelIgnition\Exceptions\CannotExecuteSolutionForNonLocalIp; use Spatie\LaravelIgnition\Http\Requests\ExecuteSolutionRequest; use Spatie\LaravelIgnition\Support\RunnableSolutionsGuard; class ExecuteSolutionController { use ValidatesRequests; public function __invoke( ExecuteSolutionRequest $request, SolutionProviderRepository $solutionProviderRepository ) { $this ->ensureRunnableSolutionsEnabled() ->ensureLocalRequest(); $solution = $request->getRunnableSolution(); $solution->run($request->get('parameters', [])); return response()->noContent(); } public function ensureRunnableSolutionsEnabled(): self { // Should already be checked in middleware but we want to be 100% certain. abort_unless(RunnableSolutionsGuard::check(), 400); return $this; } public function ensureLocalRequest(): self { $ipIsPublic = filter_var( request()->ip(), FILTER_VALIDATE_IP, FILTER_FLAG_IPV4 | FILTER_FLAG_NO_PRIV_RANGE | FILTER_FLAG_NO_RES_RANGE ); if ($ipIsPublic) { throw CannotExecuteSolutionForNonLocalIp::make(); } return $this; } } laravel-ignition/src/Http/Controllers/UpdateConfigController.php 0000644 00000000605 15002154456 0021154 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Controllers; use Spatie\Ignition\Config\IgnitionConfig; use Spatie\LaravelIgnition\Http\Requests\UpdateConfigRequest; class UpdateConfigController { public function __invoke(UpdateConfigRequest $request) { $result = (new IgnitionConfig())->saveValues($request->validated()); return response()->json($result); } } laravel-ignition/src/Http/Controllers/HealthCheckController.php 0000644 00000000767 15002154456 0020760 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Controllers; use Illuminate\Support\Facades\Artisan; use Illuminate\Support\Str; class HealthCheckController { public function __invoke() { return [ 'can_execute_commands' => $this->canExecuteCommands(), ]; } protected function canExecuteCommands(): bool { Artisan::call('help', ['--version']); $output = Artisan::output(); return Str::contains($output, app()->version()); } } laravel-ignition/src/Http/Requests/ExecuteSolutionRequest.php 0000644 00000001747 15002154456 0020565 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Requests; use Illuminate\Foundation\Http\FormRequest; use Spatie\Ignition\Contracts\RunnableSolution; use Spatie\Ignition\Contracts\Solution; use Spatie\Ignition\Contracts\SolutionProviderRepository; class ExecuteSolutionRequest extends FormRequest { public function rules(): array { return [ 'solution' => 'required', 'parameters' => 'array', ]; } public function getSolution(): Solution { $solution = app(SolutionProviderRepository::class) ->getSolutionForClass($this->get('solution')); abort_if(is_null($solution), 404, 'Solution could not be found'); return $solution; } public function getRunnableSolution(): RunnableSolution { $solution = $this->getSolution(); if (! $solution instanceof RunnableSolution) { abort(404, 'Runnable solution could not be found'); } return $solution; } } laravel-ignition/src/Http/Requests/UpdateConfigRequest.php 0000644 00000000654 15002154456 0017772 0 ustar 00 <?php namespace Spatie\LaravelIgnition\Http\Requests; use Illuminate\Foundation\Http\FormRequest; use Illuminate\Validation\Rule; class UpdateConfigRequest extends FormRequest { public function rules(): array { return [ 'theme' => ['required', Rule::in(['light', 'dark', 'auto'])], 'editor' => ['required'], 'hide_solutions' => ['required', 'boolean'], ]; } } laravel-ignition/src/ContextProviders/LaravelContextProviderDetector.php 0000644 00000001636 15002154456 0023002 0 ustar 00 <?php namespace Spatie\LaravelIgnition\ContextProviders; use Illuminate\Http\Request; use Livewire\LivewireManager; use Spatie\FlareClient\Context\ContextProvider; use Spatie\FlareClient\Context\ContextProviderDetector; class LaravelContextProviderDetector implements ContextProviderDetector { public function detectCurrentContext(): ContextProvider { if (app()->runningInConsole()) { return new LaravelConsoleContextProvider($_SERVER['argv'] ?? []); } $request = app(Request::class); if ($this->isRunningLiveWire($request)) { return new LaravelLivewireRequestContextProvider($request, app(LivewireManager::class)); } return new LaravelRequestContextProvider($request); } protected function isRunningLiveWire(Request $request): bool { return $request->hasHeader('x-livewire') && $request->hasHeader('referer'); } } laravel-ignition/src/ContextProviders/LaravelRequestContextProvider.php 0000644 00000005314 15002154456 0022656 0 ustar 00 <?php namespace Spatie\LaravelIgnition\ContextProviders; use Illuminate\Database\Eloquent\Model; use Illuminate\Http\Request as LaravelRequest; use Spatie\FlareClient\Context\RequestContextProvider; use Symfony\Component\HttpFoundation\Request as SymphonyRequest; use Throwable; class LaravelRequestContextProvider extends RequestContextProvider { protected null|SymphonyRequest|LaravelRequest $request; public function __construct(LaravelRequest $request) { $this->request = $request; } /** @return null|array<string, mixed> */ public function getUser(): array|null { try { /** @var object|null $user */ /** @phpstan-ignore-next-line */ $user = $this->request?->user(); if (! $user) { return null; } } catch (Throwable) { return null; } try { if (method_exists($user, 'toFlare')) { return $user->toFlare(); } if (method_exists($user, 'toArray')) { return $user->toArray(); } } catch (Throwable $e) { return null; } return null; } /** @return null|array<string, mixed> */ public function getRoute(): array|null { /** * @phpstan-ignore-next-line * @var \Illuminate\Routing\Route|null $route */ $route = $this->request->route(); if (! $route) { return null; } return [ 'route' => $route->getName(), 'routeParameters' => $this->getRouteParameters(), 'controllerAction' => $route->getActionName(), 'middleware' => array_values($route->gatherMiddleware() ?? []), ]; } /** @return array<int, mixed> */ protected function getRouteParameters(): array { try { /** @phpstan-ignore-next-line */ return collect(optional($this->request->route())->parameters ?? []) ->map(fn ($parameter) => $parameter instanceof Model ? $parameter->withoutRelations() : $parameter) ->map(function ($parameter) { return method_exists($parameter, 'toFlare') ? $parameter->toFlare() : $parameter; }) ->toArray(); } catch (Throwable) { return []; } } /** @return array<int, mixed> */ public function toArray(): array { $properties = parent::toArray(); if ($route = $this->getRoute()) { $properties['route'] = $route; } if ($user = $this->getUser()) { $properties['user'] = $user; } return $properties; } } laravel-ignition/src/ContextProviders/LaravelConsoleContextProvider.php 0000644 00000000272 15002154456 0022626 0 ustar 00 <?php namespace Spatie\LaravelIgnition\ContextProviders; use Spatie\FlareClient\Context\ConsoleContextProvider; class LaravelConsoleContextProvider extends ConsoleContextProvider { } laravel-ignition/src/ContextProviders/LaravelLivewireRequestContextProvider.php 0000644 00000005370 15002154456 0024367 0 ustar 00 <?php namespace Spatie\LaravelIgnition\ContextProviders; use Exception; use Illuminate\Http\Request; use Illuminate\Support\Arr; use Livewire\LivewireManager; class LaravelLivewireRequestContextProvider extends LaravelRequestContextProvider { public function __construct( Request $request, protected LivewireManager $livewireManager ) { parent::__construct($request); } /** @return array<string, string> */ public function getRequest(): array { $properties = parent::getRequest(); $properties['method'] = $this->livewireManager->originalMethod(); $properties['url'] = $this->livewireManager->originalUrl(); return $properties; } /** @return array<int|string, mixed> */ public function toArray(): array { $properties = parent::toArray(); $properties['livewire'] = $this->getLivewireInformation(); return $properties; } /** @return array<string, mixed> */ protected function getLivewireInformation(): array { /** @phpstan-ignore-next-line */ $componentId = $this->request->input('fingerprint.id'); /** @phpstan-ignore-next-line */ $componentAlias = $this->request->input('fingerprint.name'); if ($componentAlias === null) { return []; } try { $componentClass = $this->livewireManager->getClass($componentAlias); } catch (Exception $e) { $componentClass = null; } return [ 'component_class' => $componentClass, 'component_alias' => $componentAlias, 'component_id' => $componentId, 'data' => $this->resolveData(), 'updates' => $this->resolveUpdates(), ]; } /** @return array<string, mixed> */ protected function resolveData(): array { /** @phpstan-ignore-next-line */ $data = $this->request->input('serverMemo.data') ?? []; /** @phpstan-ignore-next-line */ $dataMeta = $this->request->input('serverMemo.dataMeta') ?? []; foreach ($dataMeta['modelCollections'] ?? [] as $key => $value) { $data[$key] = array_merge($data[$key] ?? [], $value); } foreach ($dataMeta['models'] ?? [] as $key => $value) { $data[$key] = array_merge($data[$key] ?? [], $value); } return $data; } /** @return array<string, mixed> */ protected function resolveUpdates(): array { /** @phpstan-ignore-next-line */ $updates = $this->request->input('updates') ?? []; return array_map(function (array $update) { $update['payload'] = Arr::except($update['payload'] ?? [], ['id']); return $update; }, $updates); } } laravel-ignition/LICENSE.md 0000644 00000002075 15002154456 0011422 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-ignition/config/flare.php 0000644 00000005061 15002154456 0013063 0 ustar 00 <?php use Spatie\FlareClient\FlareMiddleware\AddGitInformation; use Spatie\FlareClient\FlareMiddleware\RemoveRequestIp; use Spatie\FlareClient\FlareMiddleware\CensorRequestBodyFields; use Spatie\FlareClient\FlareMiddleware\CensorRequestHeaders; use Spatie\LaravelIgnition\FlareMiddleware\AddDumps; use Spatie\LaravelIgnition\FlareMiddleware\AddEnvironmentInformation; use Spatie\LaravelIgnition\FlareMiddleware\AddExceptionInformation; use Spatie\LaravelIgnition\FlareMiddleware\AddJobs; use Spatie\LaravelIgnition\FlareMiddleware\AddLogs; use Spatie\LaravelIgnition\FlareMiddleware\AddQueries; use Spatie\LaravelIgnition\FlareMiddleware\AddNotifierName; return [ /* | |-------------------------------------------------------------------------- | Flare API key |-------------------------------------------------------------------------- | | Specify Flare's API key below to enable error reporting to the service. | | More info: https://flareapp.io/docs/general/projects | */ 'key' => env('FLARE_KEY'), /* |-------------------------------------------------------------------------- | Middleware |-------------------------------------------------------------------------- | | These middleware will modify the contents of the report sent to Flare. | */ 'flare_middleware' => [ RemoveRequestIp::class, AddGitInformation::class, AddNotifierName::class, AddEnvironmentInformation::class, AddExceptionInformation::class, AddDumps::class, AddLogs::class => [ 'maximum_number_of_collected_logs' => 200, ], AddQueries::class => [ 'maximum_number_of_collected_queries' => 200, 'report_query_bindings' => true, ], AddJobs::class => [ 'max_chained_job_reporting_depth' => 5, ], CensorRequestBodyFields::class => [ 'censor_fields' => [ 'password', 'password_confirmation', ], ], CensorRequestHeaders::class => [ 'headers' => [ 'API-KEY', ] ] ], /* |-------------------------------------------------------------------------- | Reporting log statements |-------------------------------------------------------------------------- | | If this setting is `false` log statements won't be sent as events to Flare, | no matter which error level you specified in the Flare log channel. | */ 'send_logs_as_events' => true, ]; laravel-ignition/config/ignition.php 0000644 00000022710 15002154456 0013612 0 ustar 00 <?php use Spatie\Ignition\Solutions\SolutionProviders\BadMethodCallSolutionProvider; use Spatie\Ignition\Solutions\SolutionProviders\MergeConflictSolutionProvider; use Spatie\Ignition\Solutions\SolutionProviders\UndefinedPropertySolutionProvider; use Spatie\LaravelIgnition\Recorders\DumpRecorder\DumpRecorder; use Spatie\LaravelIgnition\Recorders\JobRecorder\JobRecorder; use Spatie\LaravelIgnition\Recorders\LogRecorder\LogRecorder; use Spatie\LaravelIgnition\Recorders\QueryRecorder\QueryRecorder; use Spatie\LaravelIgnition\Solutions\SolutionProviders\DefaultDbNameSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\GenericLaravelExceptionSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\IncorrectValetDbCredentialsSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\InvalidRouteActionSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingAppKeySolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingColumnSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingImportSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingLivewireComponentSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingMixManifestSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\MissingViteManifestSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\RunningLaravelDuskInProductionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\TableNotFoundSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\UndefinedViewVariableSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\UnknownValidationSolutionProvider; use Spatie\LaravelIgnition\Solutions\SolutionProviders\ViewNotFoundSolutionProvider; return [ /* |-------------------------------------------------------------------------- | Editor |-------------------------------------------------------------------------- | | Choose your preferred editor to use when clicking any edit button. | | Supported: "phpstorm", "vscode", "vscode-insiders", "textmate", "emacs", | "sublime", "atom", "nova", "macvim", "idea", "netbeans", | "xdebug" | */ 'editor' => env('IGNITION_EDITOR', 'phpstorm'), /* |-------------------------------------------------------------------------- | Theme |-------------------------------------------------------------------------- | | Here you may specify which theme Ignition should use. | | Supported: "light", "dark", "auto" | */ 'theme' => env('IGNITION_THEME', 'auto'), /* |-------------------------------------------------------------------------- | Sharing |-------------------------------------------------------------------------- | | You can share local errors with colleagues or others around the world. | Sharing is completely free and doesn't require an account on Flare. | | If necessary, you can completely disable sharing below. | */ 'enable_share_button' => env('IGNITION_SHARING_ENABLED', true), /* |-------------------------------------------------------------------------- | Register Ignition commands |-------------------------------------------------------------------------- | | Ignition comes with an additional make command that lets you create | new solution classes more easily. To keep your default Laravel | installation clean, this command is not registered by default. | | You can enable the command registration below. | */ 'register_commands' => env('REGISTER_IGNITION_COMMANDS', false), /* |-------------------------------------------------------------------------- | Solution Providers |-------------------------------------------------------------------------- | | You may specify a list of solution providers (as fully qualified class | names) that shouldn't be loaded. Ignition will ignore these classes | and possible solutions provided by them will never be displayed. | */ 'solution_providers' => [ // from spatie/ignition BadMethodCallSolutionProvider::class, MergeConflictSolutionProvider::class, UndefinedPropertySolutionProvider::class, // from spatie/laravel-ignition IncorrectValetDbCredentialsSolutionProvider::class, MissingAppKeySolutionProvider::class, DefaultDbNameSolutionProvider::class, TableNotFoundSolutionProvider::class, MissingImportSolutionProvider::class, InvalidRouteActionSolutionProvider::class, ViewNotFoundSolutionProvider::class, RunningLaravelDuskInProductionProvider::class, MissingColumnSolutionProvider::class, UnknownValidationSolutionProvider::class, MissingMixManifestSolutionProvider::class, MissingViteManifestSolutionProvider::class, MissingLivewireComponentSolutionProvider::class, UndefinedViewVariableSolutionProvider::class, GenericLaravelExceptionSolutionProvider::class, ], /* |-------------------------------------------------------------------------- | Ignored Solution Providers |-------------------------------------------------------------------------- | | You may specify a list of solution providers (as fully qualified class | names) that shouldn't be loaded. Ignition will ignore these classes | and possible solutions provided by them will never be displayed. | */ 'ignored_solution_providers' => [ ], /* |-------------------------------------------------------------------------- | Runnable Solutions |-------------------------------------------------------------------------- | | Some solutions that Ignition displays are runnable and can perform | various tasks. By default, runnable solutions are only enabled when your | app has debug mode enabled and the environment is `local` or | `development`. | | Using the `IGNITION_ENABLE_RUNNABLE_SOLUTIONS` environment variable, you | can override this behaviour and enable or disable runnable solutions | regardless of the application's environment. | | Default: env('IGNITION_ENABLE_RUNNABLE_SOLUTIONS') | */ 'enable_runnable_solutions' => env('IGNITION_ENABLE_RUNNABLE_SOLUTIONS'), /* |-------------------------------------------------------------------------- | Remote Path Mapping |-------------------------------------------------------------------------- | | If you are using a remote dev server, like Laravel Homestead, Docker, or | even a remote VPS, it will be necessary to specify your path mapping. | | Leaving one, or both of these, empty or null will not trigger the remote | URL changes and Ignition will treat your editor links as local files. | | "remote_sites_path" is an absolute base path for your sites or projects | in Homestead, Vagrant, Docker, or another remote development server. | | Example value: "/home/vagrant/Code" | | "local_sites_path" is an absolute base path for your sites or projects | on your local computer where your IDE or code editor is running on. | | Example values: "/Users/<name>/Code", "C:\Users\<name>\Documents\Code" | */ 'remote_sites_path' => env('IGNITION_REMOTE_SITES_PATH', base_path()), 'local_sites_path' => env('IGNITION_LOCAL_SITES_PATH', ''), /* |-------------------------------------------------------------------------- | Housekeeping Endpoint Prefix |-------------------------------------------------------------------------- | | Ignition registers a couple of routes when it is enabled. Below you may | specify a route prefix that will be used to host all internal links. | */ 'housekeeping_endpoint_prefix' => '_ignition', /* |-------------------------------------------------------------------------- | Settings File |-------------------------------------------------------------------------- | | Ignition allows you to save your settings to a specific global file. | | If no path is specified, a file with settings will be saved to the user's | home directory. The directory depends on the OS and its settings but it's | typically `~/.ignition.json`. In this case, the settings will be applied | to all of your projects where Ignition is used and the path is not | specified. | | However, if you want to store your settings on a project basis, or you | want to keep them in another directory, you can specify a path where | the settings file will be saved. The path should be an existing directory | with correct write access. | For example, create a new `ignition` folder in the storage directory and | use `storage_path('ignition')` as the `settings_file_path`. | | Default value: '' (empty string) */ 'settings_file_path' => '', /* |-------------------------------------------------------------------------- | Recorders |-------------------------------------------------------------------------- | | Ignition registers a couple of recorders when it is enabled. Below you may | specify a recorders will be used to record specific events. | */ 'recorders' => [ DumpRecorder::class, JobRecorder::class, LogRecorder::class, QueryRecorder::class ] ]; laravel-permission/composer.json 0000644 00000003224 15002154456 0013105 0 ustar 00 { "name": "spatie/laravel-permission", "description": "Permission handling for Laravel 6.0 and up", "license": "MIT", "keywords": [ "spatie", "laravel", "permission", "permissions", "roles", "acl", "rbac", "security" ], "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "homepage": "https://github.com/spatie/laravel-permission", "require": { "php": "^7.3|^8.0", "illuminate/auth": "^7.0|^8.0|^9.0|^10.0", "illuminate/container": "^7.0|^8.0|^9.0|^10.0", "illuminate/contracts": "^7.0|^8.0|^9.0|^10.0", "illuminate/database": "^7.0|^8.0|^9.0|^10.0" }, "require-dev": { "orchestra/testbench": "^5.0|^6.0|^7.0|^8.0", "phpunit/phpunit": "^9.4", "predis/predis": "^1.1" }, "minimum-stability": "dev", "prefer-stable": true, "autoload": { "psr-4": { "Spatie\\Permission\\": "src" }, "files": [ "src/helpers.php" ] }, "autoload-dev": { "psr-4": { "Spatie\\Permission\\Test\\": "tests" } }, "config": { "sort-packages": true }, "extra": { "branch-alias": { "dev-main": "5.x-dev", "dev-master": "5.x-dev" }, "laravel": { "providers": [ "Spatie\\Permission\\PermissionServiceProvider" ] } }, "scripts": { "test": "phpunit" } } laravel-permission/database/migrations/create_permission_tables.php.stub 0000644 00000014630 15002154456 0023040 0 ustar 00 <?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; use Spatie\Permission\PermissionRegistrar; class CreatePermissionTables extends Migration { /** * Run the migrations. * * @return void */ public function up() { $tableNames = config('permission.table_names'); $columnNames = config('permission.column_names'); $teams = config('permission.teams'); if (empty($tableNames)) { throw new \Exception('Error: config/permission.php not loaded. Run [php artisan config:clear] and try again.'); } if ($teams && empty($columnNames['team_foreign_key'] ?? null)) { throw new \Exception('Error: team_foreign_key on config/permission.php not loaded. Run [php artisan config:clear] and try again.'); } Schema::create($tableNames['permissions'], function (Blueprint $table) { $table->bigIncrements('id'); // permission id $table->string('name'); // For MySQL 8.0 use string('name', 125); $table->string('guard_name'); // For MySQL 8.0 use string('guard_name', 125); $table->timestamps(); $table->unique(['name', 'guard_name']); }); Schema::create($tableNames['roles'], function (Blueprint $table) use ($teams, $columnNames) { $table->bigIncrements('id'); // role id if ($teams || config('permission.testing')) { // permission.testing is a fix for sqlite testing $table->unsignedBigInteger($columnNames['team_foreign_key'])->nullable(); $table->index($columnNames['team_foreign_key'], 'roles_team_foreign_key_index'); } $table->string('name'); // For MySQL 8.0 use string('name', 125); $table->string('guard_name'); // For MySQL 8.0 use string('guard_name', 125); $table->timestamps(); if ($teams || config('permission.testing')) { $table->unique([$columnNames['team_foreign_key'], 'name', 'guard_name']); } else { $table->unique(['name', 'guard_name']); } }); Schema::create($tableNames['model_has_permissions'], function (Blueprint $table) use ($tableNames, $columnNames, $teams) { $table->unsignedBigInteger(PermissionRegistrar::$pivotPermission); $table->string('model_type'); $table->unsignedBigInteger($columnNames['model_morph_key']); $table->index([$columnNames['model_morph_key'], 'model_type'], 'model_has_permissions_model_id_model_type_index'); $table->foreign(PermissionRegistrar::$pivotPermission) ->references('id') // permission id ->on($tableNames['permissions']) ->onDelete('cascade'); if ($teams) { $table->unsignedBigInteger($columnNames['team_foreign_key']); $table->index($columnNames['team_foreign_key'], 'model_has_permissions_team_foreign_key_index'); $table->primary([$columnNames['team_foreign_key'], PermissionRegistrar::$pivotPermission, $columnNames['model_morph_key'], 'model_type'], 'model_has_permissions_permission_model_type_primary'); } else { $table->primary([PermissionRegistrar::$pivotPermission, $columnNames['model_morph_key'], 'model_type'], 'model_has_permissions_permission_model_type_primary'); } }); Schema::create($tableNames['model_has_roles'], function (Blueprint $table) use ($tableNames, $columnNames, $teams) { $table->unsignedBigInteger(PermissionRegistrar::$pivotRole); $table->string('model_type'); $table->unsignedBigInteger($columnNames['model_morph_key']); $table->index([$columnNames['model_morph_key'], 'model_type'], 'model_has_roles_model_id_model_type_index'); $table->foreign(PermissionRegistrar::$pivotRole) ->references('id') // role id ->on($tableNames['roles']) ->onDelete('cascade'); if ($teams) { $table->unsignedBigInteger($columnNames['team_foreign_key']); $table->index($columnNames['team_foreign_key'], 'model_has_roles_team_foreign_key_index'); $table->primary([$columnNames['team_foreign_key'], PermissionRegistrar::$pivotRole, $columnNames['model_morph_key'], 'model_type'], 'model_has_roles_role_model_type_primary'); } else { $table->primary([PermissionRegistrar::$pivotRole, $columnNames['model_morph_key'], 'model_type'], 'model_has_roles_role_model_type_primary'); } }); Schema::create($tableNames['role_has_permissions'], function (Blueprint $table) use ($tableNames) { $table->unsignedBigInteger(PermissionRegistrar::$pivotPermission); $table->unsignedBigInteger(PermissionRegistrar::$pivotRole); $table->foreign(PermissionRegistrar::$pivotPermission) ->references('id') // permission id ->on($tableNames['permissions']) ->onDelete('cascade'); $table->foreign(PermissionRegistrar::$pivotRole) ->references('id') // role id ->on($tableNames['roles']) ->onDelete('cascade'); $table->primary([PermissionRegistrar::$pivotPermission, PermissionRegistrar::$pivotRole], 'role_has_permissions_permission_id_role_id_primary'); }); app('cache') ->store(config('permission.cache.store') != 'default' ? config('permission.cache.store') : null) ->forget(config('permission.cache.key')); } /** * Reverse the migrations. * * @return void */ public function down() { $tableNames = config('permission.table_names'); if (empty($tableNames)) { throw new \Exception('Error: config/permission.php not found and defaults could not be merged. Please publish the package configuration before proceeding, or drop the tables manually.'); } Schema::drop($tableNames['role_has_permissions']); Schema::drop($tableNames['model_has_roles']); Schema::drop($tableNames['model_has_permissions']); Schema::drop($tableNames['roles']); Schema::drop($tableNames['permissions']); } } laravel-permission/database/migrations/add_teams_fields.php.stub 0000644 00000007733 15002154456 0021250 0 ustar 00 <?php use Illuminate\Support\Facades\DB; use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; use Spatie\Permission\PermissionRegistrar; class AddTeamsFields extends Migration { /** * Run the migrations. * * @return void */ public function up() { $teams = config('permission.teams'); $tableNames = config('permission.table_names'); $columnNames = config('permission.column_names'); if (! $teams) { return; } if (empty($tableNames)) { throw new \Exception('Error: config/permission.php not loaded. Run [php artisan config:clear] and try again.'); } if (empty($columnNames['team_foreign_key'] ?? null)) { throw new \Exception('Error: team_foreign_key on config/permission.php not loaded. Run [php artisan config:clear] and try again.'); } if (! Schema::hasColumn($tableNames['roles'], $columnNames['team_foreign_key'])) { Schema::table($tableNames['roles'], function (Blueprint $table) use ($columnNames) { $table->unsignedBigInteger($columnNames['team_foreign_key'])->nullable()->after('id'); $table->index($columnNames['team_foreign_key'], 'roles_team_foreign_key_index'); $table->dropUnique('roles_name_guard_name_unique'); $table->unique([$columnNames['team_foreign_key'], 'name', 'guard_name']); }); } if (! Schema::hasColumn($tableNames['model_has_permissions'], $columnNames['team_foreign_key'])) { Schema::table($tableNames['model_has_permissions'], function (Blueprint $table) use ($tableNames, $columnNames) { $table->unsignedBigInteger($columnNames['team_foreign_key'])->default('1'); $table->index($columnNames['team_foreign_key'], 'model_has_permissions_team_foreign_key_index'); if (DB::getDriverName() !== 'sqlite') { $table->dropForeign([PermissionRegistrar::$pivotPermission]); } $table->dropPrimary(); $table->primary([$columnNames['team_foreign_key'], PermissionRegistrar::$pivotPermission, $columnNames['model_morph_key'], 'model_type'], 'model_has_permissions_permission_model_type_primary'); if (DB::getDriverName() !== 'sqlite') { $table->foreign(PermissionRegistrar::$pivotPermission) ->references('id')->on($tableNames['permissions'])->onDelete('cascade'); } }); } if (! Schema::hasColumn($tableNames['model_has_roles'], $columnNames['team_foreign_key'])) { Schema::table($tableNames['model_has_roles'], function (Blueprint $table) use ($tableNames, $columnNames) { $table->unsignedBigInteger($columnNames['team_foreign_key'])->default('1');; $table->index($columnNames['team_foreign_key'], 'model_has_roles_team_foreign_key_index'); if (DB::getDriverName() !== 'sqlite') { $table->dropForeign([PermissionRegistrar::$pivotRole]); } $table->dropPrimary(); $table->primary([$columnNames['team_foreign_key'], PermissionRegistrar::$pivotRole, $columnNames['model_morph_key'], 'model_type'], 'model_has_roles_role_model_type_primary'); if (DB::getDriverName() !== 'sqlite') { $table->foreign(PermissionRegistrar::$pivotRole) ->references('id')->on($tableNames['roles'])->onDelete('cascade'); } }); } app('cache') ->store(config('permission.cache.store') != 'default' ? config('permission.cache.store') : null) ->forget(config('permission.cache.key')); } /** * Reverse the migrations. * * @return void */ public function down() { } } laravel-permission/README.md 0000644 00000011662 15002154456 0011647 0 ustar 00 <p align="center"><img src="/art/socialcard.png" alt="Social Card of Laravel Permission"></p> # Associate users with permissions and roles ### Sponsor <table> <tr> <td><img src="https://user-images.githubusercontent.com/6287961/92889815-d64c3d80-f416-11ea-894a-b4de7e8f7eef.png"></td> <td>If you want to quickly add authentication and authorization to Laravel projects, feel free to check Auth0's Laravel SDK and free plan at <a href="https://auth0.com/developers?utm_source=GHsponsor&utm_medium=GHsponsor&utm_campaign=laravel-permission&utm_content=auth">https://auth0.com/developers</a>.</td> </tr> </table> [](https://packagist.org/packages/spatie/laravel-permission) [](https://github.com/spatie/laravel-permission/actions?query=workflow%3ATests+branch%3Amain) [](https://packagist.org/packages/spatie/laravel-permission) ## Documentation, Installation, and Usage Instructions See the [documentation](https://spatie.be/docs/laravel-permission/) for detailed installation and usage instructions. ## What It Does This package allows you to manage user permissions and roles in a database. Once installed you can do stuff like this: ```php // Adding permissions to a user $user->givePermissionTo('edit articles'); // Adding permissions via a role $user->assignRole('writer'); $role->givePermissionTo('edit articles'); ``` Because all permissions will be registered on [Laravel's gate](https://laravel.com/docs/authorization), you can check if a user has a permission with Laravel's default `can` function: ```php $user->can('edit articles'); ``` ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/laravel-permission.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/laravel-permission) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ### Testing ``` bash composer test ``` ### Changelog Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ### Security If you discover any security-related issues, please email [security@spatie.be](mailto:security@spatie.be) instead of using the issue tracker. ## Postcardware You're free to use this package, but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/en/opensource/postcards). ## Credits - [Chris Brown](https://github.com/drbyte) - [Freek Van der Herten](https://github.com/freekmurze) - [All Contributors](../../contributors) This package is heavily based on [Jeffrey Way](https://twitter.com/jeffrey_way)'s awesome [Laracasts](https://laracasts.com) lessons on [permissions and roles](https://laracasts.com/series/whats-new-in-laravel-5-1/episodes/16). His original code can be found [in this repo on GitHub](https://github.com/laracasts/laravel-5-roles-and-permissions-demo). Special thanks to [Alex Vanderbist](https://github.com/AlexVanderbist) who greatly helped with `v2`, and to [Chris Brown](https://github.com/drbyte) for his longtime support helping us maintain the package. And a special thanks to [Caneco](https://twitter.com/caneco) for the logo ✨ ## Alternatives - [Povilas Korop](https://twitter.com/@povilaskorop) did an excellent job listing the alternatives [in an article on Laravel News](https://laravel-news.com/two-best-roles-permissions-packages). In that same article, he compares laravel-permission to [Joseph Silber](https://github.com/JosephSilber)'s [Bouncer]((https://github.com/JosephSilber/bouncer)), which in our book is also an excellent package. - [ultraware/roles](https://github.com/ultraware/roles) takes a slightly different approach to its features. - [santigarcor/laratrust](https://github.com/santigarcor/laratrust) implements team support - [zizaco/entrust](https://github.com/zizaco/entrust) offers some wildcard pattern matching ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. laravel-permission/src/Exceptions/WildcardPermissionNotProperlyFormatted.php 0000644 00000000500 15002154456 0023664 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class WildcardPermissionNotProperlyFormatted extends InvalidArgumentException { public static function create(string $permission) { return new static("Wildcard permission `{$permission}` is not properly formatted."); } } laravel-permission/src/Exceptions/GuardDoesNotMatch.php 0000644 00000000630 15002154456 0017315 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use Illuminate\Support\Collection; use InvalidArgumentException; class GuardDoesNotMatch extends InvalidArgumentException { public static function create(string $givenGuard, Collection $expectedGuards) { return new static("The given role or permission should use guard `{$expectedGuards->implode(', ')}` instead of `{$givenGuard}`."); } } laravel-permission/src/Exceptions/UnauthorizedException.php 0000644 00000003660 15002154456 0020350 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use Symfony\Component\HttpKernel\Exception\HttpException; class UnauthorizedException extends HttpException { private $requiredRoles = []; private $requiredPermissions = []; public static function forRoles(array $roles): self { $message = 'User does not have the right roles.'; if (config('permission.display_role_in_exception')) { $message .= ' Necessary roles are '.implode(', ', $roles); } $exception = new static(403, $message, null, []); $exception->requiredRoles = $roles; return $exception; } public static function forPermissions(array $permissions): self { $message = 'User does not have the right permissions.'; if (config('permission.display_permission_in_exception')) { $message .= ' Necessary permissions are '.implode(', ', $permissions); } $exception = new static(403, $message, null, []); $exception->requiredPermissions = $permissions; return $exception; } public static function forRolesOrPermissions(array $rolesOrPermissions): self { $message = 'User does not have any of the necessary access rights.'; if (config('permission.display_permission_in_exception') && config('permission.display_role_in_exception')) { $message .= ' Necessary roles or permissions are '.implode(', ', $rolesOrPermissions); } $exception = new static(403, $message, null, []); $exception->requiredPermissions = $rolesOrPermissions; return $exception; } public static function notLoggedIn(): self { return new static(403, 'User is not logged in.', null, []); } public function getRequiredRoles(): array { return $this->requiredRoles; } public function getRequiredPermissions(): array { return $this->requiredPermissions; } } laravel-permission/src/Exceptions/WildcardPermissionNotImplementsContract.php 0000644 00000000510 15002154456 0024016 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class WildcardPermissionNotImplementsContract extends InvalidArgumentException { public static function create() { return new static('Wildcard permission class must implements Spatie\Permission\Contracts\Wildcard contract'); } } laravel-permission/src/Exceptions/RoleDoesNotExist.php 0000644 00000000616 15002154456 0017220 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class RoleDoesNotExist extends InvalidArgumentException { public static function named(string $roleName) { return new static("There is no role named `{$roleName}`."); } public static function withId(int $roleId) { return new static("There is no role with id `{$roleId}`."); } } laravel-permission/src/Exceptions/PermissionDoesNotExist.php 0000644 00000001035 15002154456 0020443 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class PermissionDoesNotExist extends InvalidArgumentException { public static function create(string $permissionName, string $guardName = '') { return new static("There is no permission named `{$permissionName}` for guard `{$guardName}`."); } public static function withId(int $permissionId, string $guardName = '') { return new static("There is no [permission] with id `{$permissionId}` for guard `{$guardName}`."); } } laravel-permission/src/Exceptions/PermissionAlreadyExists.php 0000644 00000000523 15002154456 0020635 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class PermissionAlreadyExists extends InvalidArgumentException { public static function create(string $permissionName, string $guardName) { return new static("A `{$permissionName}` permission already exists for guard `{$guardName}`."); } } laravel-permission/src/Exceptions/RoleAlreadyExists.php 0000644 00000000473 15002154456 0017412 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class RoleAlreadyExists extends InvalidArgumentException { public static function create(string $roleName, string $guardName) { return new static("A role `{$roleName}` already exists for guard `{$guardName}`."); } } laravel-permission/src/Exceptions/WildcardPermissionInvalidArgument.php 0000644 00000000463 15002154456 0022622 0 ustar 00 <?php namespace Spatie\Permission\Exceptions; use InvalidArgumentException; class WildcardPermissionInvalidArgument extends InvalidArgumentException { public static function create() { return new static('Wildcard permission must be string, permission id or permission instance'); } } laravel-permission/src/Middlewares/RoleOrPermissionMiddleware.php 0000644 00000001501 15002154456 0021370 0 ustar 00 <?php namespace Spatie\Permission\Middlewares; use Closure; use Illuminate\Support\Facades\Auth; use Spatie\Permission\Exceptions\UnauthorizedException; class RoleOrPermissionMiddleware { public function handle($request, Closure $next, $roleOrPermission, $guard = null) { $authGuard = Auth::guard($guard); if ($authGuard->guest()) { throw UnauthorizedException::notLoggedIn(); } $rolesOrPermissions = is_array($roleOrPermission) ? $roleOrPermission : explode('|', $roleOrPermission); if (! $authGuard->user()->hasAnyRole($rolesOrPermissions) && ! $authGuard->user()->hasAnyPermission($rolesOrPermissions)) { throw UnauthorizedException::forRolesOrPermissions($rolesOrPermissions); } return $next($request); } } laravel-permission/src/Middlewares/PermissionMiddleware.php 0000644 00000001347 15002154456 0020255 0 ustar 00 <?php namespace Spatie\Permission\Middlewares; use Closure; use Spatie\Permission\Exceptions\UnauthorizedException; class PermissionMiddleware { public function handle($request, Closure $next, $permission, $guard = null) { $authGuard = app('auth')->guard($guard); if ($authGuard->guest()) { throw UnauthorizedException::notLoggedIn(); } $permissions = is_array($permission) ? $permission : explode('|', $permission); foreach ($permissions as $permission) { if ($authGuard->user()->can($permission)) { return $next($request); } } throw UnauthorizedException::forPermissions($permissions); } } laravel-permission/src/Middlewares/RoleMiddleware.php 0000644 00000001223 15002154456 0017017 0 ustar 00 <?php namespace Spatie\Permission\Middlewares; use Closure; use Illuminate\Support\Facades\Auth; use Spatie\Permission\Exceptions\UnauthorizedException; class RoleMiddleware { public function handle($request, Closure $next, $role, $guard = null) { $authGuard = Auth::guard($guard); if ($authGuard->guest()) { throw UnauthorizedException::notLoggedIn(); } $roles = is_array($role) ? $role : explode('|', $role); if (! $authGuard->user()->hasAnyRole($roles)) { throw UnauthorizedException::forRoles($roles); } return $next($request); } } laravel-permission/src/helpers.php 0000644 00000001663 15002154456 0013332 0 ustar 00 <?php if (! function_exists('getModelForGuard')) { /** * @return string|null */ function getModelForGuard(string $guard) { return collect(config('auth.guards')) ->map(function ($guard) { if (! isset($guard['provider'])) { return; } return config("auth.providers.{$guard['provider']}.model"); })->get($guard); } } if (! function_exists('setPermissionsTeamId')) { /** * @param int|string|\Illuminate\Database\Eloquent\Model $id */ function setPermissionsTeamId($id) { app(\Spatie\Permission\PermissionRegistrar::class)->setPermissionsTeamId($id); } } if (! function_exists('getPermissionsTeamId')) { /** * @return int|string */ function getPermissionsTeamId() { return app(\Spatie\Permission\PermissionRegistrar::class)->getPermissionsTeamId(); } } laravel-permission/src/Contracts/Role.php 0000644 00000002165 15002154456 0014527 0 ustar 00 <?php namespace Spatie\Permission\Contracts; use Illuminate\Database\Eloquent\Relations\BelongsToMany; interface Role { /** * A role may be given various permissions. */ public function permissions(): BelongsToMany; /** * Find a role by its name and guard name. * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\RoleDoesNotExist */ public static function findByName(string $name, $guardName): self; /** * Find a role by its id and guard name. * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\RoleDoesNotExist */ public static function findById(int $id, $guardName): self; /** * Find or create a role by its name and guard name. * * @param string|null $guardName */ public static function findOrCreate(string $name, $guardName): self; /** * Determine if the user may perform the given permission. * * @param string|\Spatie\Permission\Contracts\Permission $permission */ public function hasPermissionTo($permission): bool; } laravel-permission/src/Contracts/Permission.php 0000644 00000001630 15002154456 0015752 0 ustar 00 <?php namespace Spatie\Permission\Contracts; use Illuminate\Database\Eloquent\Relations\BelongsToMany; interface Permission { /** * A permission can be applied to roles. */ public function roles(): BelongsToMany; /** * Find a permission by its name. * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\PermissionDoesNotExist */ public static function findByName(string $name, $guardName): self; /** * Find a permission by its id. * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\PermissionDoesNotExist */ public static function findById(int $id, $guardName): self; /** * Find or Create a permission by its name and guard name. * * @param string|null $guardName */ public static function findOrCreate(string $name, $guardName): self; } laravel-permission/src/Contracts/Wildcard.php 0000644 00000000262 15002154456 0015353 0 ustar 00 <?php namespace Spatie\Permission\Contracts; interface Wildcard { /** * @param string|Wildcard $permission */ public function implies($permission): bool; } laravel-permission/src/Commands/CreateRole.php 0000644 00000004142 15002154456 0015451 0 ustar 00 <?php namespace Spatie\Permission\Commands; use Illuminate\Console\Command; use Spatie\Permission\Contracts\Permission as PermissionContract; use Spatie\Permission\Contracts\Role as RoleContract; use Spatie\Permission\PermissionRegistrar; class CreateRole extends Command { protected $signature = 'permission:create-role {name : The name of the role} {guard? : The name of the guard} {permissions? : A list of permissions to assign to the role, separated by | } {--team-id=}'; protected $description = 'Create a role'; public function handle() { $roleClass = app(RoleContract::class); $teamIdAux = getPermissionsTeamId(); setPermissionsTeamId($this->option('team-id') ?: null); if (! PermissionRegistrar::$teams && $this->option('team-id')) { $this->warn('Teams feature disabled, argument --team-id has no effect. Either enable it in permissions config file or remove --team-id parameter'); return; } $role = $roleClass::findOrCreate($this->argument('name'), $this->argument('guard')); setPermissionsTeamId($teamIdAux); $teams_key = PermissionRegistrar::$teamsKey; if (PermissionRegistrar::$teams && $this->option('team-id') && is_null($role->$teams_key)) { $this->warn("Role `{$role->name}` already exists on the global team; argument --team-id has no effect"); } $role->givePermissionTo($this->makePermissions($this->argument('permissions'))); $this->info("Role `{$role->name}` ".($role->wasRecentlyCreated ? 'created' : 'updated')); } /** * @param array|null|string $string */ protected function makePermissions($string = null) { if (empty($string)) { return; } $permissionClass = app(PermissionContract::class); $permissions = explode('|', $string); $models = []; foreach ($permissions as $permission) { $models[] = $permissionClass::findOrCreate(trim($permission), $this->argument('guard')); } return collect($models); } } laravel-permission/src/Commands/CreatePermission.php 0000644 00000001336 15002154456 0016702 0 ustar 00 <?php namespace Spatie\Permission\Commands; use Illuminate\Console\Command; use Spatie\Permission\Contracts\Permission as PermissionContract; class CreatePermission extends Command { protected $signature = 'permission:create-permission {name : The name of the permission} {guard? : The name of the guard}'; protected $description = 'Create a permission'; public function handle() { $permissionClass = app(PermissionContract::class); $permission = $permissionClass::findOrCreate($this->argument('name'), $this->argument('guard')); $this->info("Permission `{$permission->name}` ".($permission->wasRecentlyCreated ? 'created' : 'already exists')); } } laravel-permission/src/Commands/UpgradeForTeams.php 0000644 00000006436 15002154456 0016464 0 ustar 00 <?php namespace Spatie\Permission\Commands; use Illuminate\Console\Command; use Illuminate\Support\Facades\Config; class UpgradeForTeams extends Command { protected $signature = 'permission:setup-teams'; protected $description = 'Setup the teams feature by generating the associated migration.'; protected $migrationSuffix = 'add_teams_fields.php'; public function handle() { if (! Config::get('permission.teams')) { $this->error('Teams feature is disabled in your permission.php file.'); $this->warn('Please enable the teams setting in your configuration.'); return; } $this->line(''); $this->info('The teams feature setup is going to add a migration and a model'); $existingMigrations = $this->alreadyExistingMigrations(); if ($existingMigrations) { $this->line(''); $this->warn($this->getExistingMigrationsWarning($existingMigrations)); } $this->line(''); if (! $this->confirm('Proceed with the migration creation?', 'yes')) { return; } $this->line(''); $this->line('Creating migration'); if ($this->createMigration()) { $this->info('Migration created successfully.'); } else { $this->error( "Couldn't create migration.\n". 'Check the write permissions within the database/migrations directory.' ); } $this->line(''); } /** * Create the migration. * * @return bool */ protected function createMigration() { try { $migrationStub = __DIR__."/../../database/migrations/{$this->migrationSuffix}.stub"; copy($migrationStub, $this->getMigrationPath()); return true; } catch (\Throwable $e) { $this->error($e->getMessage()); return false; } } /** * Build a warning regarding possible duplication * due to already existing migrations. * * @return string */ protected function getExistingMigrationsWarning(array $existingMigrations) { if (count($existingMigrations) > 1) { $base = "Setup teams migrations already exist.\nFollowing files were found: "; } else { $base = "Setup teams migration already exists.\nFollowing file was found: "; } return $base.array_reduce($existingMigrations, function ($carry, $fileName) { return $carry."\n - ".$fileName; }); } /** * Check if there is another migration * with the same suffix. * * @return array */ protected function alreadyExistingMigrations() { $matchingFiles = glob($this->getMigrationPath('*')); return array_map(function ($path) { return basename($path); }, $matchingFiles); } /** * Get the migration path. * * The date parameter is optional for ability * to provide a custom value or a wildcard. * * @param string|null $date * @return string */ protected function getMigrationPath($date = null) { $date = $date ?: date('Y_m_d_His'); return database_path("migrations/{$date}_{$this->migrationSuffix}"); } } laravel-permission/src/Commands/Show.php 0000644 00000005745 15002154456 0014356 0 ustar 00 <?php namespace Spatie\Permission\Commands; use Illuminate\Console\Command; use Illuminate\Support\Collection; use Spatie\Permission\Contracts\Permission as PermissionContract; use Spatie\Permission\Contracts\Role as RoleContract; use Symfony\Component\Console\Helper\TableCell; class Show extends Command { protected $signature = 'permission:show {guard? : The name of the guard} {style? : The display style (default|borderless|compact|box)}'; protected $description = 'Show a table of roles and permissions per guard'; public function handle() { $permissionClass = app(PermissionContract::class); $roleClass = app(RoleContract::class); $teamsEnabled = config('permission.teams'); $team_key = config('permission.column_names.team_foreign_key'); $style = $this->argument('style') ?? 'default'; $guard = $this->argument('guard'); if ($guard) { $guards = Collection::make([$guard]); } else { $guards = $permissionClass::pluck('guard_name')->merge($roleClass::pluck('guard_name'))->unique(); } foreach ($guards as $guard) { $this->info("Guard: $guard"); $roles = $roleClass::whereGuardName($guard) ->with('permissions') ->when($teamsEnabled, function ($q) use ($team_key) { $q->orderBy($team_key); }) ->orderBy('name')->get()->mapWithKeys(function ($role) use ($teamsEnabled, $team_key) { return [$role->name.'_'.($teamsEnabled ? ($role->$team_key ?: '') : '') => [ 'permissions' => $role->permissions->pluck('id'), $team_key => $teamsEnabled ? $role->$team_key : null, ]]; }); $permissions = $permissionClass::whereGuardName($guard)->orderBy('name')->pluck('name', 'id'); $body = $permissions->map(function ($permission, $id) use ($roles) { return $roles->map(function (array $role_data) use ($id) { return $role_data['permissions']->contains($id) ? ' ✔' : ' ·'; })->prepend($permission); }); if ($teamsEnabled) { $teams = $roles->groupBy($team_key)->values()->map(function ($group, $id) { return new TableCell('Team ID: '.($id ?: 'NULL'), ['colspan' => $group->count()]); }); } $this->table( array_merge([ isset($teams) ? $teams->prepend(new TableCell(''))->toArray() : [], $roles->keys()->map(function ($val) { $name = explode('_', $val); array_pop($name); return implode('_', $name); }) ->prepend('')->toArray(), ]), $body->toArray(), $style ); } } } laravel-permission/src/Commands/CacheReset.php 0000644 00000001011 15002154456 0015422 0 ustar 00 <?php namespace Spatie\Permission\Commands; use Illuminate\Console\Command; use Spatie\Permission\PermissionRegistrar; class CacheReset extends Command { protected $signature = 'permission:cache-reset'; protected $description = 'Reset the permission cache'; public function handle() { if (app(PermissionRegistrar::class)->forgetCachedPermissions()) { $this->info('Permission cache flushed.'); } else { $this->error('Unable to flush cache.'); } } } laravel-permission/src/PermissionServiceProvider.php 0000644 00000014105 15002154456 0017047 0 ustar 00 <?php namespace Spatie\Permission; use Illuminate\Filesystem\Filesystem; use Illuminate\Routing\Route; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Illuminate\Support\ServiceProvider; use Illuminate\View\Compilers\BladeCompiler; use Spatie\Permission\Contracts\Permission as PermissionContract; use Spatie\Permission\Contracts\Role as RoleContract; class PermissionServiceProvider extends ServiceProvider { public function boot(PermissionRegistrar $permissionLoader) { $this->offerPublishing(); $this->registerMacroHelpers(); $this->registerCommands(); $this->registerModelBindings(); if ($this->app->config['permission.register_permission_check_method']) { $permissionLoader->clearClassPermissions(); $permissionLoader->registerPermissions(); } $this->app->singleton(PermissionRegistrar::class, function ($app) use ($permissionLoader) { return $permissionLoader; }); } public function register() { $this->mergeConfigFrom( __DIR__.'/../config/permission.php', 'permission' ); $this->callAfterResolving('blade.compiler', function (BladeCompiler $bladeCompiler) { $this->registerBladeExtensions($bladeCompiler); }); } protected function offerPublishing() { if (! function_exists('config_path')) { // function not available and 'publish' not relevant in Lumen return; } $this->publishes([ __DIR__.'/../config/permission.php' => config_path('permission.php'), ], 'permission-config'); $this->publishes([ __DIR__.'/../database/migrations/create_permission_tables.php.stub' => $this->getMigrationFileName('create_permission_tables.php'), ], 'permission-migrations'); } protected function registerCommands() { $this->commands([ Commands\CacheReset::class, Commands\CreateRole::class, Commands\CreatePermission::class, Commands\Show::class, Commands\UpgradeForTeams::class, ]); } protected function registerModelBindings() { $config = $this->app->config['permission.models']; if (! $config) { return; } $this->app->bind(PermissionContract::class, $config['permission']); $this->app->bind(RoleContract::class, $config['role']); } public static function bladeMethodWrapper($method, $role, $guard = null) { return auth($guard)->check() && auth($guard)->user()->{$method}($role); } protected function registerBladeExtensions($bladeCompiler) { $bladeCompiler->directive('role', function ($arguments) { return "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {$arguments})): ?>"; }); $bladeCompiler->directive('elserole', function ($arguments) { return "<?php elseif(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {$arguments})): ?>"; }); $bladeCompiler->directive('endrole', function () { return '<?php endif; ?>'; }); $bladeCompiler->directive('hasrole', function ($arguments) { return "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {$arguments})): ?>"; }); $bladeCompiler->directive('endhasrole', function () { return '<?php endif; ?>'; }); $bladeCompiler->directive('hasanyrole', function ($arguments) { return "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasAnyRole', {$arguments})): ?>"; }); $bladeCompiler->directive('endhasanyrole', function () { return '<?php endif; ?>'; }); $bladeCompiler->directive('hasallroles', function ($arguments) { return "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasAllRoles', {$arguments})): ?>"; }); $bladeCompiler->directive('endhasallroles', function () { return '<?php endif; ?>'; }); $bladeCompiler->directive('unlessrole', function ($arguments) { return "<?php if(! \\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {$arguments})): ?>"; }); $bladeCompiler->directive('endunlessrole', function () { return '<?php endif; ?>'; }); $bladeCompiler->directive('hasexactroles', function ($arguments) { return "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasExactRoles', {$arguments})): ?>"; }); $bladeCompiler->directive('endhasexactroles', function () { return '<?php endif; ?>'; }); } protected function registerMacroHelpers() { if (! method_exists(Route::class, 'macro')) { // Lumen return; } Route::macro('role', function ($roles = []) { $roles = implode('|', Arr::wrap($roles)); $this->middleware("role:$roles"); return $this; }); Route::macro('permission', function ($permissions = []) { $permissions = implode('|', Arr::wrap($permissions)); $this->middleware("permission:$permissions"); return $this; }); } /** * Returns existing migration file if found, else uses the current timestamp. */ protected function getMigrationFileName($migrationFileName): string { $timestamp = date('Y_m_d_His'); $filesystem = $this->app->make(Filesystem::class); return Collection::make($this->app->databasePath().DIRECTORY_SEPARATOR.'migrations'.DIRECTORY_SEPARATOR) ->flatMap(function ($path) use ($filesystem, $migrationFileName) { return $filesystem->glob($path.'*_'.$migrationFileName); }) ->push($this->app->databasePath()."/migrations/{$timestamp}_{$migrationFileName}") ->first(); } } laravel-permission/src/Models/Role.php 0000644 00000014572 15002154456 0014017 0 ustar 00 <?php namespace Spatie\Permission\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Relations\BelongsToMany; use Spatie\Permission\Contracts\Role as RoleContract; use Spatie\Permission\Exceptions\GuardDoesNotMatch; use Spatie\Permission\Exceptions\RoleAlreadyExists; use Spatie\Permission\Exceptions\RoleDoesNotExist; use Spatie\Permission\Guard; use Spatie\Permission\PermissionRegistrar; use Spatie\Permission\Traits\HasPermissions; use Spatie\Permission\Traits\RefreshesPermissionCache; /** * @property int $id * @property string $name * @property string $guard_name * @property ?\Illuminate\Support\Carbon $created_at * @property ?\Illuminate\Support\Carbon $updated_at */ class Role extends Model implements RoleContract { use HasPermissions; use RefreshesPermissionCache; protected $guarded = []; public function __construct(array $attributes = []) { $attributes['guard_name'] = $attributes['guard_name'] ?? config('auth.defaults.guard'); parent::__construct($attributes); $this->guarded[] = $this->primaryKey; } public function getTable() { return config('permission.table_names.roles', parent::getTable()); } public static function create(array $attributes = []) { $attributes['guard_name'] = $attributes['guard_name'] ?? Guard::getDefaultName(static::class); $params = ['name' => $attributes['name'], 'guard_name' => $attributes['guard_name']]; if (PermissionRegistrar::$teams) { if (array_key_exists(PermissionRegistrar::$teamsKey, $attributes)) { $params[PermissionRegistrar::$teamsKey] = $attributes[PermissionRegistrar::$teamsKey]; } else { $attributes[PermissionRegistrar::$teamsKey] = getPermissionsTeamId(); } } if (static::findByParam($params)) { throw RoleAlreadyExists::create($attributes['name'], $attributes['guard_name']); } return static::query()->create($attributes); } /** * A role may be given various permissions. */ public function permissions(): BelongsToMany { return $this->belongsToMany( config('permission.models.permission'), config('permission.table_names.role_has_permissions'), PermissionRegistrar::$pivotRole, PermissionRegistrar::$pivotPermission ); } /** * A role belongs to some users of the model associated with its guard. */ public function users(): BelongsToMany { return $this->morphedByMany( getModelForGuard($this->attributes['guard_name'] ?? config('auth.defaults.guard')), 'model', config('permission.table_names.model_has_roles'), PermissionRegistrar::$pivotRole, config('permission.column_names.model_morph_key') ); } /** * Find a role by its name and guard name. * * @param string|null $guardName * @return \Spatie\Permission\Contracts\Role|\Spatie\Permission\Models\Role * * @throws \Spatie\Permission\Exceptions\RoleDoesNotExist */ public static function findByName(string $name, $guardName = null): RoleContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $role = static::findByParam(['name' => $name, 'guard_name' => $guardName]); if (! $role) { throw RoleDoesNotExist::named($name); } return $role; } /** * Find a role by its id (and optionally guardName). * * @param string|null $guardName * @return \Spatie\Permission\Contracts\Role|\Spatie\Permission\Models\Role */ public static function findById(int $id, $guardName = null): RoleContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $role = static::findByParam([(new static())->getKeyName() => $id, 'guard_name' => $guardName]); if (! $role) { throw RoleDoesNotExist::withId($id); } return $role; } /** * Find or create role by its name (and optionally guardName). * * @param string|null $guardName * @return \Spatie\Permission\Contracts\Role|\Spatie\Permission\Models\Role */ public static function findOrCreate(string $name, $guardName = null): RoleContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $role = static::findByParam(['name' => $name, 'guard_name' => $guardName]); if (! $role) { return static::query()->create(['name' => $name, 'guard_name' => $guardName] + (PermissionRegistrar::$teams ? [PermissionRegistrar::$teamsKey => getPermissionsTeamId()] : [])); } return $role; } protected static function findByParam(array $params = []) { $query = static::query(); if (PermissionRegistrar::$teams) { $query->where(function ($q) use ($params) { $q->whereNull(PermissionRegistrar::$teamsKey) ->orWhere(PermissionRegistrar::$teamsKey, $params[PermissionRegistrar::$teamsKey] ?? getPermissionsTeamId()); }); unset($params[PermissionRegistrar::$teamsKey]); } foreach ($params as $key => $value) { $query->where($key, $value); } return $query->first(); } /** * Determine if the user may perform the given permission. * * @param string|Permission $permission * * @throws \Spatie\Permission\Exceptions\GuardDoesNotMatch */ public function hasPermissionTo($permission): bool { if (config('permission.enable_wildcard_permission', false)) { return $this->hasWildcardPermission($permission, $this->getDefaultGuardName()); } $permissionClass = $this->getPermissionClass(); if (is_string($permission)) { $permission = $permissionClass->findByName($permission, $this->getDefaultGuardName()); } if (is_int($permission)) { $permission = $permissionClass->findById($permission, $this->getDefaultGuardName()); } if (! $this->getGuardNames()->contains($permission->guard_name)) { throw GuardDoesNotMatch::create($permission->guard_name, $this->getGuardNames()); } return $this->permissions->contains($permission->getKeyName(), $permission->getKey()); } } laravel-permission/src/Models/Permission.php 0000644 00000011622 15002154456 0015237 0 ustar 00 <?php namespace Spatie\Permission\Models; use Illuminate\Database\Eloquent\Collection; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Relations\BelongsToMany; use Spatie\Permission\Contracts\Permission as PermissionContract; use Spatie\Permission\Exceptions\PermissionAlreadyExists; use Spatie\Permission\Exceptions\PermissionDoesNotExist; use Spatie\Permission\Guard; use Spatie\Permission\PermissionRegistrar; use Spatie\Permission\Traits\HasRoles; use Spatie\Permission\Traits\RefreshesPermissionCache; /** * @property int $id * @property string $name * @property string $guard_name * @property ?\Illuminate\Support\Carbon $created_at * @property ?\Illuminate\Support\Carbon $updated_at */ class Permission extends Model implements PermissionContract { use HasRoles; use RefreshesPermissionCache; protected $guarded = []; public function __construct(array $attributes = []) { $attributes['guard_name'] = $attributes['guard_name'] ?? config('auth.defaults.guard'); parent::__construct($attributes); $this->guarded[] = $this->primaryKey; } public function getTable() { return config('permission.table_names.permissions', parent::getTable()); } public static function create(array $attributes = []) { $attributes['guard_name'] = $attributes['guard_name'] ?? Guard::getDefaultName(static::class); $permission = static::getPermission(['name' => $attributes['name'], 'guard_name' => $attributes['guard_name']]); if ($permission) { throw PermissionAlreadyExists::create($attributes['name'], $attributes['guard_name']); } return static::query()->create($attributes); } /** * A permission can be applied to roles. */ public function roles(): BelongsToMany { return $this->belongsToMany( config('permission.models.role'), config('permission.table_names.role_has_permissions'), PermissionRegistrar::$pivotPermission, PermissionRegistrar::$pivotRole ); } /** * A permission belongs to some users of the model associated with its guard. */ public function users(): BelongsToMany { return $this->morphedByMany( getModelForGuard($this->attributes['guard_name'] ?? config('auth.defaults.guard')), 'model', config('permission.table_names.model_has_permissions'), PermissionRegistrar::$pivotPermission, config('permission.column_names.model_morph_key') ); } /** * Find a permission by its name (and optionally guardName). * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\PermissionDoesNotExist */ public static function findByName(string $name, $guardName = null): PermissionContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $permission = static::getPermission(['name' => $name, 'guard_name' => $guardName]); if (! $permission) { throw PermissionDoesNotExist::create($name, $guardName); } return $permission; } /** * Find a permission by its id (and optionally guardName). * * @param string|null $guardName * * @throws \Spatie\Permission\Exceptions\PermissionDoesNotExist */ public static function findById(int $id, $guardName = null): PermissionContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $permission = static::getPermission([(new static())->getKeyName() => $id, 'guard_name' => $guardName]); if (! $permission) { throw PermissionDoesNotExist::withId($id, $guardName); } return $permission; } /** * Find or create permission by its name (and optionally guardName). * * @param string|null $guardName */ public static function findOrCreate(string $name, $guardName = null): PermissionContract { $guardName = $guardName ?? Guard::getDefaultName(static::class); $permission = static::getPermission(['name' => $name, 'guard_name' => $guardName]); if (! $permission) { return static::query()->create(['name' => $name, 'guard_name' => $guardName]); } return $permission; } /** * Get the current cached permissions. */ protected static function getPermissions(array $params = [], bool $onlyOne = false): Collection { return app(PermissionRegistrar::class) ->setPermissionClass(static::class) ->getPermissions($params, $onlyOne); } /** * Get the current cached first permission. * * @return \Spatie\Permission\Contracts\Permission */ protected static function getPermission(array $params = []): ?PermissionContract { return static::getPermissions($params, true)->first(); } } laravel-permission/src/WildcardPermission.php 0000644 00000005371 15002154456 0015472 0 ustar 00 <?php namespace Spatie\Permission; use Illuminate\Support\Collection; use Spatie\Permission\Contracts\Wildcard; use Spatie\Permission\Exceptions\WildcardPermissionNotProperlyFormatted; class WildcardPermission implements Wildcard { /** @var string */ public const WILDCARD_TOKEN = '*'; /** @var string */ public const PART_DELIMITER = '.'; /** @var string */ public const SUBPART_DELIMITER = ','; /** @var string */ protected $permission; /** @var Collection */ protected $parts; public function __construct(string $permission) { $this->permission = $permission; $this->parts = collect(); $this->setParts(); } /** * @param string|WildcardPermission $permission */ public function implies($permission): bool { if (is_string($permission)) { $permission = new static($permission); } $otherParts = $permission->getParts(); $i = 0; $partsCount = $this->getParts()->count(); foreach ($otherParts as $otherPart) { if ($partsCount - 1 < $i) { return true; } if (! $this->parts->get($i)->contains(static::WILDCARD_TOKEN) && ! $this->containsAll($this->parts->get($i), $otherPart)) { return false; } $i++; } for ($i; $i < $partsCount; $i++) { if (! $this->parts->get($i)->contains(static::WILDCARD_TOKEN)) { return false; } } return true; } protected function containsAll(Collection $part, Collection $otherPart): bool { foreach ($otherPart->toArray() as $item) { if (! $part->contains($item)) { return false; } } return true; } public function getParts(): Collection { return $this->parts; } /** * Sets the different parts and subparts from permission string. */ protected function setParts(): void { if (empty($this->permission) || $this->permission == null) { throw WildcardPermissionNotProperlyFormatted::create($this->permission); } $parts = collect(explode(static::PART_DELIMITER, $this->permission)); $parts->each(function ($item, $key) { $subParts = collect(explode(static::SUBPART_DELIMITER, $item)); if ($subParts->isEmpty() || $subParts->contains('')) { throw WildcardPermissionNotProperlyFormatted::create($this->permission); } $this->parts->add($subParts); }); if ($this->parts->isEmpty()) { throw WildcardPermissionNotProperlyFormatted::create($this->permission); } } } laravel-permission/src/PermissionRegistrar.php 0000644 00000025166 15002154456 0015707 0 ustar 00 <?php namespace Spatie\Permission; use Illuminate\Cache\CacheManager; use Illuminate\Contracts\Auth\Access\Authorizable; use Illuminate\Contracts\Auth\Access\Gate; use Illuminate\Contracts\Cache\Repository; use Illuminate\Contracts\Cache\Store; use Illuminate\Database\Eloquent\Collection; use Spatie\Permission\Contracts\Permission; use Spatie\Permission\Contracts\Role; class PermissionRegistrar { /** @var \Illuminate\Contracts\Cache\Repository */ protected $cache; /** @var \Illuminate\Cache\CacheManager */ protected $cacheManager; /** @var string */ protected $permissionClass; /** @var string */ protected $roleClass; /** @var \Illuminate\Database\Eloquent\Collection */ protected $permissions; /** @var string */ public static $pivotRole; /** @var string */ public static $pivotPermission; /** @var \DateInterval|int */ public static $cacheExpirationTime; /** @var bool */ public static $teams; /** @var string */ public static $teamsKey; /** @var int|string */ protected $teamId = null; /** @var string */ public static $cacheKey; /** @var array */ private $cachedRoles = []; /** @var array */ private $alias = []; /** @var array */ private $except = []; /** * PermissionRegistrar constructor. */ public function __construct(CacheManager $cacheManager) { $this->permissionClass = config('permission.models.permission'); $this->roleClass = config('permission.models.role'); $this->cacheManager = $cacheManager; $this->initializeCache(); } public function initializeCache() { self::$cacheExpirationTime = config('permission.cache.expiration_time') ?: \DateInterval::createFromDateString('24 hours'); self::$teams = config('permission.teams', false); self::$teamsKey = config('permission.column_names.team_foreign_key'); self::$cacheKey = config('permission.cache.key'); self::$pivotRole = config('permission.column_names.role_pivot_key') ?: 'role_id'; self::$pivotPermission = config('permission.column_names.permission_pivot_key') ?: 'permission_id'; $this->cache = $this->getCacheStoreFromConfig(); } protected function getCacheStoreFromConfig(): Repository { // the 'default' fallback here is from the permission.php config file, // where 'default' means to use config(cache.default) $cacheDriver = config('permission.cache.store', 'default'); // when 'default' is specified, no action is required since we already have the default instance if ($cacheDriver === 'default') { return $this->cacheManager->store(); } // if an undefined cache store is specified, fallback to 'array' which is Laravel's closest equiv to 'none' if (! \array_key_exists($cacheDriver, config('cache.stores'))) { $cacheDriver = 'array'; } return $this->cacheManager->store($cacheDriver); } /** * Set the team id for teams/groups support, this id is used when querying permissions/roles * * @param int|string|\Illuminate\Database\Eloquent\Model $id */ public function setPermissionsTeamId($id) { if ($id instanceof \Illuminate\Database\Eloquent\Model) { $id = $id->getKey(); } $this->teamId = $id; } /** * @return int|string */ public function getPermissionsTeamId() { return $this->teamId; } /** * Register the permission check method on the gate. * We resolve the Gate fresh here, for benefit of long-running instances. */ public function registerPermissions(): bool { app(Gate::class)->before(function (Authorizable $user, string $ability) { if (method_exists($user, 'checkPermissionTo')) { return $user->checkPermissionTo($ability) ?: null; } }); return true; } /** * Flush the cache. */ public function forgetCachedPermissions() { $this->permissions = null; return $this->cache->forget(self::$cacheKey); } /** * Clear class permissions. * This is only intended to be called by the PermissionServiceProvider on boot, * so that long-running instances like Swoole don't keep old data in memory. */ public function clearClassPermissions() { $this->permissions = null; } /** * Load permissions from cache * This get cache and turns array into \Illuminate\Database\Eloquent\Collection */ private function loadPermissions() { if ($this->permissions) { return; } $this->permissions = $this->cache->remember(self::$cacheKey, self::$cacheExpirationTime, function () { return $this->getSerializedPermissionsForCache(); }); // fallback for old cache method, must be removed on next mayor version if (! isset($this->permissions['alias'])) { $this->forgetCachedPermissions(); $this->loadPermissions(); return; } $this->alias = $this->permissions['alias']; $this->hydrateRolesCache(); $this->permissions = $this->getHydratedPermissionCollection(); $this->cachedRoles = $this->alias = $this->except = []; } /** * Get the permissions based on the passed params. */ public function getPermissions(array $params = [], bool $onlyOne = false): Collection { $this->loadPermissions(); $method = $onlyOne ? 'first' : 'filter'; $permissions = $this->permissions->$method(static function ($permission) use ($params) { foreach ($params as $attr => $value) { if ($permission->getAttribute($attr) != $value) { return false; } } return true; }); if ($onlyOne) { $permissions = new Collection($permissions ? [$permissions] : []); } return $permissions; } /** * Get an instance of the permission class. */ public function getPermissionClass(): Permission { return app($this->permissionClass); } public function setPermissionClass($permissionClass) { $this->permissionClass = $permissionClass; config()->set('permission.models.permission', $permissionClass); app()->bind(Permission::class, $permissionClass); return $this; } /** * Get an instance of the role class. */ public function getRoleClass(): Role { return app($this->roleClass); } public function setRoleClass($roleClass) { $this->roleClass = $roleClass; config()->set('permission.models.role', $roleClass); app()->bind(Role::class, $roleClass); return $this; } public function getCacheRepository(): Repository { return $this->cache; } public function getCacheStore(): Store { return $this->cache->getStore(); } protected function getPermissionsWithRoles(): Collection { return $this->getPermissionClass()->select()->with('roles')->get(); } /** * Changes array keys with alias */ private function aliasedArray($model): array { return collect(is_array($model) ? $model : $model->getAttributes())->except($this->except) ->keyBy(function ($value, $key) { return $this->alias[$key] ?? $key; })->all(); } /** * Array for cache alias */ private function aliasModelFields($newKeys = []): void { $i = 0; $alphas = ! count($this->alias) ? range('a', 'h') : range('j', 'p'); foreach (array_keys($newKeys->getAttributes()) as $value) { if (! isset($this->alias[$value])) { $this->alias[$value] = $alphas[$i++] ?? $value; } } $this->alias = array_diff_key($this->alias, array_flip($this->except)); } /* * Make the cache smaller using an array with only required fields */ private function getSerializedPermissionsForCache() { $this->except = config('permission.cache.column_names_except', ['created_at', 'updated_at', 'deleted_at']); $permissions = $this->getPermissionsWithRoles() ->map(function ($permission) { if (! $this->alias) { $this->aliasModelFields($permission); } return $this->aliasedArray($permission) + $this->getSerializedRoleRelation($permission); })->all(); $roles = array_values($this->cachedRoles); $this->cachedRoles = []; return ['alias' => array_flip($this->alias)] + compact('permissions', 'roles'); } private function getSerializedRoleRelation($permission) { if (! $permission->roles->count()) { return []; } if (! isset($this->alias['roles'])) { $this->alias['roles'] = 'r'; $this->aliasModelFields($permission->roles[0]); } return [ 'r' => $permission->roles->map(function ($role) { if (! isset($this->cachedRoles[$role->getKey()])) { $this->cachedRoles[$role->getKey()] = $this->aliasedArray($role); } return $role->getKey(); })->all(), ]; } private function getHydratedPermissionCollection() { $permissionClass = $this->getPermissionClass(); $permissionInstance = new $permissionClass(); return Collection::make( array_map(function ($item) use ($permissionInstance) { return $permissionInstance->newInstance([], true) ->setRawAttributes($this->aliasedArray(array_diff_key($item, ['r' => 0])), true) ->setRelation('roles', $this->getHydratedRoleCollection($item['r'] ?? [])); }, $this->permissions['permissions']) ); } private function getHydratedRoleCollection(array $roles) { return Collection::make(array_values( array_intersect_key($this->cachedRoles, array_flip($roles)) )); } private function hydrateRolesCache() { $roleClass = $this->getRoleClass(); $roleInstance = new $roleClass(); array_map(function ($item) use ($roleInstance) { $role = $roleInstance->newInstance([], true) ->setRawAttributes($this->aliasedArray($item), true); $this->cachedRoles[$role->getKey()] = $role; }, $this->permissions['roles']); $this->permissions['roles'] = []; } } laravel-permission/src/Traits/HasPermissions.php 0000644 00000041005 15002154456 0016077 0 ustar 00 <?php namespace Spatie\Permission\Traits; use Illuminate\Database\Eloquent\Builder; use Illuminate\Database\Eloquent\Relations\BelongsToMany; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Spatie\Permission\Contracts\Permission; use Spatie\Permission\Contracts\Role; use Spatie\Permission\Contracts\Wildcard; use Spatie\Permission\Exceptions\GuardDoesNotMatch; use Spatie\Permission\Exceptions\PermissionDoesNotExist; use Spatie\Permission\Exceptions\WildcardPermissionInvalidArgument; use Spatie\Permission\Exceptions\WildcardPermissionNotImplementsContract; use Spatie\Permission\Guard; use Spatie\Permission\PermissionRegistrar; use Spatie\Permission\WildcardPermission; trait HasPermissions { /** @var string */ private $permissionClass; /** @var string */ private $wildcardClass; public static function bootHasPermissions() { static::deleting(function ($model) { if (method_exists($model, 'isForceDeleting') && ! $model->isForceDeleting()) { return; } if (is_a($model, Permission::class)) { return; } $teams = PermissionRegistrar::$teams; PermissionRegistrar::$teams = false; $model->permissions()->detach(); PermissionRegistrar::$teams = $teams; }); } public function getPermissionClass() { if (! isset($this->permissionClass)) { $this->permissionClass = app(PermissionRegistrar::class)->getPermissionClass(); } return $this->permissionClass; } protected function getWildcardClass() { if (! is_null($this->wildcardClass)) { return $this->wildcardClass; } $this->wildcardClass = false; if (config('permission.enable_wildcard_permission', false)) { $this->wildcardClass = config('permission.wildcard_permission', WildcardPermission::class); if (! is_subclass_of($this->wildcardClass, Wildcard::class)) { throw WildcardPermissionNotImplementsContract::create(); } } return $this->wildcardClass; } /** * A model may have multiple direct permissions. */ public function permissions(): BelongsToMany { $relation = $this->morphToMany( config('permission.models.permission'), 'model', config('permission.table_names.model_has_permissions'), config('permission.column_names.model_morph_key'), PermissionRegistrar::$pivotPermission ); if (! PermissionRegistrar::$teams) { return $relation; } return $relation->wherePivot(PermissionRegistrar::$teamsKey, getPermissionsTeamId()); } /** * Scope the model query to certain permissions only. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions */ public function scopePermission(Builder $query, $permissions): Builder { $permissions = $this->convertToPermissionModels($permissions); $rolesWithPermissions = array_unique(array_reduce($permissions, function ($result, $permission) { return array_merge($result, $permission->roles->all()); }, [])); return $query->where(function (Builder $query) use ($permissions, $rolesWithPermissions) { $query->whereHas('permissions', function (Builder $subQuery) use ($permissions) { $permissionClass = $this->getPermissionClass(); $key = (new $permissionClass())->getKeyName(); $subQuery->whereIn(config('permission.table_names.permissions').".$key", \array_column($permissions, $key)); }); if (count($rolesWithPermissions) > 0) { $query->orWhereHas('roles', function (Builder $subQuery) use ($rolesWithPermissions) { $roleClass = $this->getRoleClass(); $key = (new $roleClass())->getKeyName(); $subQuery->whereIn(config('permission.table_names.roles').".$key", \array_column($rolesWithPermissions, $key)); }); } }); } /** * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions * * @throws \Spatie\Permission\Exceptions\PermissionDoesNotExist */ protected function convertToPermissionModels($permissions): array { if ($permissions instanceof Collection) { $permissions = $permissions->all(); } return array_map(function ($permission) { if ($permission instanceof Permission) { return $permission; } $method = is_string($permission) ? 'findByName' : 'findById'; return $this->getPermissionClass()->{$method}($permission, $this->getDefaultGuardName()); }, Arr::wrap($permissions)); } /** * Find a permission. * * @param string|int|\Spatie\Permission\Contracts\Permission $permission * @return \Spatie\Permission\Contracts\Permission * * @throws PermissionDoesNotExist */ public function filterPermission($permission, $guardName = null) { $permissionClass = $this->getPermissionClass(); if (is_string($permission)) { $permission = $permissionClass->findByName( $permission, $guardName ?? $this->getDefaultGuardName() ); } if (is_int($permission)) { $permission = $permissionClass->findById( $permission, $guardName ?? $this->getDefaultGuardName() ); } if (! $permission instanceof Permission) { throw new PermissionDoesNotExist(); } return $permission; } /** * Determine if the model may perform the given permission. * * @param string|int|\Spatie\Permission\Contracts\Permission $permission * @param string|null $guardName * * @throws PermissionDoesNotExist */ public function hasPermissionTo($permission, $guardName = null): bool { if ($this->getWildcardClass()) { return $this->hasWildcardPermission($permission, $guardName); } $permission = $this->filterPermission($permission, $guardName); return $this->hasDirectPermission($permission) || $this->hasPermissionViaRole($permission); } /** * Validates a wildcard permission against all permissions of a user. * * @param string|int|\Spatie\Permission\Contracts\Permission $permission * @param string|null $guardName */ protected function hasWildcardPermission($permission, $guardName = null): bool { $guardName = $guardName ?? $this->getDefaultGuardName(); if (is_int($permission)) { $permission = $this->getPermissionClass()->findById($permission, $guardName); } if ($permission instanceof Permission) { $permission = $permission->name; } if (! is_string($permission)) { throw WildcardPermissionInvalidArgument::create(); } $WildcardPermissionClass = $this->getWildcardClass(); foreach ($this->getAllPermissions() as $userPermission) { if ($guardName !== $userPermission->guard_name) { continue; } $userPermission = new $WildcardPermissionClass($userPermission->name); if ($userPermission->implies($permission)) { return true; } } return false; } /** * An alias to hasPermissionTo(), but avoids throwing an exception. * * @param string|int|\Spatie\Permission\Contracts\Permission $permission * @param string|null $guardName */ public function checkPermissionTo($permission, $guardName = null): bool { try { return $this->hasPermissionTo($permission, $guardName); } catch (PermissionDoesNotExist $e) { return false; } } /** * Determine if the model has any of the given permissions. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection ...$permissions */ public function hasAnyPermission(...$permissions): bool { $permissions = collect($permissions)->flatten(); foreach ($permissions as $permission) { if ($this->checkPermissionTo($permission)) { return true; } } return false; } /** * Determine if the model has all of the given permissions. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection ...$permissions */ public function hasAllPermissions(...$permissions): bool { $permissions = collect($permissions)->flatten(); foreach ($permissions as $permission) { if (! $this->checkPermissionTo($permission)) { return false; } } return true; } /** * Determine if the model has, via roles, the given permission. */ protected function hasPermissionViaRole(Permission $permission): bool { return $this->hasRole($permission->roles); } /** * Determine if the model has the given permission. * * @param string|int|\Spatie\Permission\Contracts\Permission $permission * * @throws PermissionDoesNotExist */ public function hasDirectPermission($permission): bool { $permission = $this->filterPermission($permission); return $this->permissions->contains($permission->getKeyName(), $permission->getKey()); } /** * Return all the permissions the model has via roles. */ public function getPermissionsViaRoles(): Collection { return $this->loadMissing('roles', 'roles.permissions') ->roles->flatMap(function ($role) { return $role->permissions; })->sort()->values(); } /** * Return all the permissions the model has, both directly and via roles. */ public function getAllPermissions(): Collection { /** @var Collection $permissions */ $permissions = $this->permissions; if (method_exists($this, 'roles')) { $permissions = $permissions->merge($this->getPermissionsViaRoles()); } return $permissions->sort()->values(); } /** * Returns permissions ids as array keys * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions * @return array */ public function collectPermissions(...$permissions) { return collect($permissions) ->flatten() ->reduce(function ($array, $permission) { if (empty($permission)) { return $array; } $permission = $this->getStoredPermission($permission); if (! $permission instanceof Permission) { return $array; } $this->ensureModelSharesGuard($permission); $array[$permission->getKey()] = PermissionRegistrar::$teams && ! is_a($this, Role::class) ? [PermissionRegistrar::$teamsKey => getPermissionsTeamId()] : []; return $array; }, []); } /** * Grant the given permission(s) to a role. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions * @return $this */ public function givePermissionTo(...$permissions) { $permissions = $this->collectPermissions(...$permissions); $model = $this->getModel(); if ($model->exists) { $this->permissions()->sync($permissions, false); $model->load('permissions'); } else { $class = \get_class($model); $class::saved( function ($object) use ($permissions, $model) { if ($model->getKey() != $object->getKey()) { return; } $model->permissions()->sync($permissions, false); $model->load('permissions'); } ); } if (is_a($this, get_class(app(PermissionRegistrar::class)->getRoleClass()))) { $this->forgetCachedPermissions(); } return $this; } /** * Remove all current permissions and set the given ones. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions * @return $this */ public function syncPermissions(...$permissions) { $this->permissions()->detach(); return $this->givePermissionTo($permissions); } /** * Revoke the given permission(s). * * @param \Spatie\Permission\Contracts\Permission|\Spatie\Permission\Contracts\Permission[]|string|string[] $permission * @return $this */ public function revokePermissionTo($permission) { $this->permissions()->detach($this->getStoredPermission($permission)); if (is_a($this, get_class(app(PermissionRegistrar::class)->getRoleClass()))) { $this->forgetCachedPermissions(); } $this->load('permissions'); return $this; } public function getPermissionNames(): Collection { return $this->permissions->pluck('name'); } /** * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection $permissions * @return \Spatie\Permission\Contracts\Permission|\Spatie\Permission\Contracts\Permission[]|\Illuminate\Support\Collection */ protected function getStoredPermission($permissions) { $permissionClass = $this->getPermissionClass(); if (is_numeric($permissions)) { return $permissionClass->findById($permissions, $this->getDefaultGuardName()); } if (is_string($permissions)) { return $permissionClass->findByName($permissions, $this->getDefaultGuardName()); } if (is_array($permissions)) { $permissions = array_map(function ($permission) use ($permissionClass) { return is_a($permission, get_class($permissionClass)) ? $permission->name : $permission; }, $permissions); return $permissionClass ->whereIn('name', $permissions) ->whereIn('guard_name', $this->getGuardNames()) ->get(); } return $permissions; } /** * @param \Spatie\Permission\Contracts\Permission|\Spatie\Permission\Contracts\Role $roleOrPermission * * @throws \Spatie\Permission\Exceptions\GuardDoesNotMatch */ protected function ensureModelSharesGuard($roleOrPermission) { if (! $this->getGuardNames()->contains($roleOrPermission->guard_name)) { throw GuardDoesNotMatch::create($roleOrPermission->guard_name, $this->getGuardNames()); } } protected function getGuardNames(): Collection { return Guard::getNames($this); } protected function getDefaultGuardName(): string { return Guard::getDefaultName($this); } /** * Forget the cached permissions. */ public function forgetCachedPermissions() { app(PermissionRegistrar::class)->forgetCachedPermissions(); } /** * Check if the model has All of the requested Direct permissions. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection ...$permissions */ public function hasAllDirectPermissions(...$permissions): bool { $permissions = collect($permissions)->flatten(); foreach ($permissions as $permission) { if (! $this->hasDirectPermission($permission)) { return false; } } return true; } /** * Check if the model has Any of the requested Direct permissions. * * @param string|int|array|\Spatie\Permission\Contracts\Permission|\Illuminate\Support\Collection ...$permissions */ public function hasAnyDirectPermission(...$permissions): bool { $permissions = collect($permissions)->flatten(); foreach ($permissions as $permission) { if ($this->hasDirectPermission($permission)) { return true; } } return false; } } laravel-permission/src/Traits/RefreshesPermissionCache.php 0000644 00000000664 15002154456 0020061 0 ustar 00 <?php namespace Spatie\Permission\Traits; use Spatie\Permission\PermissionRegistrar; trait RefreshesPermissionCache { public static function bootRefreshesPermissionCache() { static::saved(function () { app(PermissionRegistrar::class)->forgetCachedPermissions(); }); static::deleted(function () { app(PermissionRegistrar::class)->forgetCachedPermissions(); }); } } laravel-permission/src/Traits/HasRoles.php 0000644 00000024140 15002154456 0014651 0 ustar 00 <?php namespace Spatie\Permission\Traits; use Illuminate\Database\Eloquent\Builder; use Illuminate\Database\Eloquent\Relations\BelongsToMany; use Illuminate\Support\Arr; use Illuminate\Support\Collection; use Spatie\Permission\Contracts\Permission; use Spatie\Permission\Contracts\Role; use Spatie\Permission\PermissionRegistrar; trait HasRoles { use HasPermissions; /** @var string */ private $roleClass; public static function bootHasRoles() { static::deleting(function ($model) { if (method_exists($model, 'isForceDeleting') && ! $model->isForceDeleting()) { return; } $teams = PermissionRegistrar::$teams; PermissionRegistrar::$teams = false; $model->roles()->detach(); PermissionRegistrar::$teams = $teams; }); } public function getRoleClass() { if (! isset($this->roleClass)) { $this->roleClass = app(PermissionRegistrar::class)->getRoleClass(); } return $this->roleClass; } /** * A model may have multiple roles. */ public function roles(): BelongsToMany { $relation = $this->morphToMany( config('permission.models.role'), 'model', config('permission.table_names.model_has_roles'), config('permission.column_names.model_morph_key'), PermissionRegistrar::$pivotRole ); if (! PermissionRegistrar::$teams) { return $relation; } return $relation->wherePivot(PermissionRegistrar::$teamsKey, getPermissionsTeamId()) ->where(function ($q) { $teamField = config('permission.table_names.roles').'.'.PermissionRegistrar::$teamsKey; $q->whereNull($teamField)->orWhere($teamField, getPermissionsTeamId()); }); } /** * Scope the model query to certain roles only. * * @param string|int|array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection $roles * @param string $guard */ public function scopeRole(Builder $query, $roles, $guard = null): Builder { if ($roles instanceof Collection) { $roles = $roles->all(); } $roles = array_map(function ($role) use ($guard) { if ($role instanceof Role) { return $role; } $method = is_numeric($role) ? 'findById' : 'findByName'; return $this->getRoleClass()->{$method}($role, $guard ?: $this->getDefaultGuardName()); }, Arr::wrap($roles)); return $query->whereHas('roles', function (Builder $subQuery) use ($roles) { $roleClass = $this->getRoleClass(); $key = (new $roleClass())->getKeyName(); $subQuery->whereIn(config('permission.table_names.roles').".$key", \array_column($roles, $key)); }); } /** * Assign the given role to the model. * * @param array|string|int|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection ...$roles * @return $this */ public function assignRole(...$roles) { $roles = collect($roles) ->flatten() ->reduce(function ($array, $role) { if (empty($role)) { return $array; } $role = $this->getStoredRole($role); if (! $role instanceof Role) { return $array; } $this->ensureModelSharesGuard($role); $array[$role->getKey()] = PermissionRegistrar::$teams && ! is_a($this, Permission::class) ? [PermissionRegistrar::$teamsKey => getPermissionsTeamId()] : []; return $array; }, []); $model = $this->getModel(); if ($model->exists) { $this->roles()->sync($roles, false); $model->load('roles'); } else { $class = \get_class($model); $class::saved( function ($object) use ($roles, $model) { if ($model->getKey() != $object->getKey()) { return; } $model->roles()->sync($roles, false); $model->load('roles'); } ); } if (is_a($this, get_class($this->getPermissionClass()))) { $this->forgetCachedPermissions(); } return $this; } /** * Revoke the given role from the model. * * @param string|int|\Spatie\Permission\Contracts\Role $role */ public function removeRole($role) { $this->roles()->detach($this->getStoredRole($role)); $this->load('roles'); if (is_a($this, get_class($this->getPermissionClass()))) { $this->forgetCachedPermissions(); } return $this; } /** * Remove all current roles and set the given ones. * * @param array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection|string|int ...$roles * @return $this */ public function syncRoles(...$roles) { $this->roles()->detach(); return $this->assignRole($roles); } /** * Determine if the model has (one of) the given role(s). * * @param string|int|array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection $roles */ public function hasRole($roles, string $guard = null): bool { $this->loadMissing('roles'); if (is_string($roles) && false !== strpos($roles, '|')) { $roles = $this->convertPipeToArray($roles); } if (is_string($roles)) { return $guard ? $this->roles->where('guard_name', $guard)->contains('name', $roles) : $this->roles->contains('name', $roles); } if (is_int($roles)) { $roleClass = $this->getRoleClass(); $key = (new $roleClass())->getKeyName(); return $guard ? $this->roles->where('guard_name', $guard)->contains($key, $roles) : $this->roles->contains($key, $roles); } if ($roles instanceof Role) { return $this->roles->contains($roles->getKeyName(), $roles->getKey()); } if (is_array($roles)) { foreach ($roles as $role) { if ($this->hasRole($role, $guard)) { return true; } } return false; } return $roles->intersect($guard ? $this->roles->where('guard_name', $guard) : $this->roles)->isNotEmpty(); } /** * Determine if the model has any of the given role(s). * * Alias to hasRole() but without Guard controls * * @param string|int|array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection $roles */ public function hasAnyRole(...$roles): bool { return $this->hasRole($roles); } /** * Determine if the model has all of the given role(s). * * @param string|array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection $roles */ public function hasAllRoles($roles, string $guard = null): bool { $this->loadMissing('roles'); if (is_string($roles) && false !== strpos($roles, '|')) { $roles = $this->convertPipeToArray($roles); } if (is_string($roles)) { return $guard ? $this->roles->where('guard_name', $guard)->contains('name', $roles) : $this->roles->contains('name', $roles); } if ($roles instanceof Role) { return $this->roles->contains($roles->getKeyName(), $roles->getKey()); } $roles = collect()->make($roles)->map(function ($role) { return $role instanceof Role ? $role->name : $role; }); return $roles->intersect( $guard ? $this->roles->where('guard_name', $guard)->pluck('name') : $this->getRoleNames() ) == $roles; } /** * Determine if the model has exactly all of the given role(s). * * @param string|array|\Spatie\Permission\Contracts\Role|\Illuminate\Support\Collection $roles */ public function hasExactRoles($roles, string $guard = null): bool { $this->loadMissing('roles'); if (is_string($roles) && false !== strpos($roles, '|')) { $roles = $this->convertPipeToArray($roles); } if (is_string($roles)) { $roles = [$roles]; } if ($roles instanceof Role) { $roles = [$roles->name]; } $roles = collect()->make($roles)->map(function ($role) { return $role instanceof Role ? $role->name : $role; }); return $this->roles->count() == $roles->count() && $this->hasAllRoles($roles, $guard); } /** * Return all permissions directly coupled to the model. */ public function getDirectPermissions(): Collection { return $this->permissions; } public function getRoleNames(): Collection { $this->loadMissing('roles'); return $this->roles->pluck('name'); } protected function getStoredRole($role): Role { $roleClass = $this->getRoleClass(); if (is_numeric($role)) { return $roleClass->findById($role, $this->getDefaultGuardName()); } if (is_string($role)) { return $roleClass->findByName($role, $this->getDefaultGuardName()); } return $role; } protected function convertPipeToArray(string $pipeString) { $pipeString = trim($pipeString); if (strlen($pipeString) <= 2) { return $pipeString; } $quoteCharacter = substr($pipeString, 0, 1); $endCharacter = substr($quoteCharacter, -1, 1); if ($quoteCharacter !== $endCharacter) { return explode('|', $pipeString); } if (! in_array($quoteCharacter, ["'", '"'])) { return explode('|', $pipeString); } return explode('|', trim($pipeString, $quoteCharacter)); } } laravel-permission/src/Guard.php 0000644 00000005035 15002154456 0012727 0 ustar 00 <?php namespace Spatie\Permission; use Illuminate\Database\Eloquent\Model; use Illuminate\Support\Collection; class Guard { /** * Return a collection of guard names suitable for the $model, * as indicated by the presence of a $guard_name property or a guardName() method on the model. * * @param string|Model $model model class object or name */ public static function getNames($model): Collection { $class = is_object($model) ? get_class($model) : $model; if (is_object($model)) { if (\method_exists($model, 'guardName')) { $guardName = $model->guardName(); } else { $guardName = $model->getAttributeValue('guard_name'); } } if (! isset($guardName)) { $guardName = (new \ReflectionClass($class))->getDefaultProperties()['guard_name'] ?? null; } if ($guardName) { return collect($guardName); } return self::getConfigAuthGuards($class); } /** * Get list of relevant guards for the $class model based on config(auth) settings. * * Lookup flow: * - get names of models for guards defined in auth.guards where a provider is set * - filter for provider models matching the model $class being checked (important for Lumen) * - keys() gives just the names of the matched guards * - return collection of guard names */ protected static function getConfigAuthGuards(string $class): Collection { return collect(config('auth.guards')) ->map(function ($guard) { if (! isset($guard['provider'])) { return null; } return config("auth.providers.{$guard['provider']}.model"); }) ->filter(function ($model) use ($class) { return $class === $model; }) ->keys(); } /** * Lookup a guard name relevant for the $class model and the current user. * * @param string|Model $class model class object or name * @return string guard name */ public static function getDefaultName($class): string { $default = config('auth.defaults.guard'); $possible_guards = static::getNames($class); // return current-detected auth.defaults.guard if it matches one of those that have been checked if ($possible_guards->contains($default)) { return $default; } return $possible_guards->first() ?: $default; } } laravel-permission/LICENSE.md 0000644 00000002102 15002154456 0011761 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-permission/ide.json 0000644 00000004636 15002154456 0012027 0 ustar 00 { "$schema": "https://laravel-ide.com/schema/laravel-ide-v2.json", "blade": { "directives": [ { "name": "role", "prefix": "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {", "suffix": "})): ?>" }, { "name": "elserole", "prefix": "<?php elseif(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {", "suffix": "})): ?>" }, { "name": "endrole", "prefix": "", "suffix": "" }, { "name": "hasrole", "prefix": "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {", "suffix": "})): ?>" }, { "name": "endhasrole", "prefix": "", "suffix": "" }, { "name": "hasanyrole", "prefix": "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasAnyRole', {", "suffix": "})): ?>" }, { "name": "endhasanyrole", "prefix": "", "suffix": "" }, { "name": "hasallroles", "prefix": "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasAllRoles', {", "suffix": "})): ?>" }, { "name": "endhasallroles", "prefix": "", "suffix": "" }, { "name": "unlessrole", "prefix": "<?php if(! \\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasRole', {", "suffix": "})): ?>" }, { "name": "endunlessrole", "prefix": "", "suffix": "" }, { "name": "hasexactroles", "prefix": "<?php if(\\Spatie\\Permission\\PermissionServiceProvider::bladeMethodWrapper('hasExactRoles', {", "suffix": "})): ?>" }, { "name": "endhasexactroles", "prefix": "", "suffix": "" } ] } } laravel-permission/config/permission.php 0000644 00000012714 15002154456 0014535 0 ustar 00 <?php return [ 'models' => [ /* * When using the "HasPermissions" trait from this package, we need to know which * Eloquent model should be used to retrieve your permissions. Of course, it * is often just the "Permission" model but you may use whatever you like. * * The model you want to use as a Permission model needs to implement the * `Spatie\Permission\Contracts\Permission` contract. */ 'permission' => Spatie\Permission\Models\Permission::class, /* * When using the "HasRoles" trait from this package, we need to know which * Eloquent model should be used to retrieve your roles. Of course, it * is often just the "Role" model but you may use whatever you like. * * The model you want to use as a Role model needs to implement the * `Spatie\Permission\Contracts\Role` contract. */ 'role' => Spatie\Permission\Models\Role::class, ], 'table_names' => [ /* * When using the "HasRoles" trait from this package, we need to know which * table should be used to retrieve your roles. We have chosen a basic * default value but you may easily change it to any table you like. */ 'roles' => 'roles', /* * When using the "HasPermissions" trait from this package, we need to know which * table should be used to retrieve your permissions. We have chosen a basic * default value but you may easily change it to any table you like. */ 'permissions' => 'permissions', /* * When using the "HasPermissions" trait from this package, we need to know which * table should be used to retrieve your models permissions. We have chosen a * basic default value but you may easily change it to any table you like. */ 'model_has_permissions' => 'model_has_permissions', /* * When using the "HasRoles" trait from this package, we need to know which * table should be used to retrieve your models roles. We have chosen a * basic default value but you may easily change it to any table you like. */ 'model_has_roles' => 'model_has_roles', /* * When using the "HasRoles" trait from this package, we need to know which * table should be used to retrieve your roles permissions. We have chosen a * basic default value but you may easily change it to any table you like. */ 'role_has_permissions' => 'role_has_permissions', ], 'column_names' => [ /* * Change this if you want to name the related pivots other than defaults */ 'role_pivot_key' => null, //default 'role_id', 'permission_pivot_key' => null, //default 'permission_id', /* * Change this if you want to name the related model primary key other than * `model_id`. * * For example, this would be nice if your primary keys are all UUIDs. In * that case, name this `model_uuid`. */ 'model_morph_key' => 'model_id', /* * Change this if you want to use the teams feature and your related model's * foreign key is other than `team_id`. */ 'team_foreign_key' => 'team_id', ], /* * When set to true, the method for checking permissions will be registered on the gate. * Set this to false, if you want to implement custom logic for checking permissions. */ 'register_permission_check_method' => true, /* * When set to true the package implements teams using the 'team_foreign_key'. If you want * the migrations to register the 'team_foreign_key', you must set this to true * before doing the migration. If you already did the migration then you must make a new * migration to also add 'team_foreign_key' to 'roles', 'model_has_roles', and * 'model_has_permissions'(view the latest version of package's migration file) */ 'teams' => false, /* * When set to true, the required permission names are added to the exception * message. This could be considered an information leak in some contexts, so * the default setting is false here for optimum safety. */ 'display_permission_in_exception' => false, /* * When set to true, the required role names are added to the exception * message. This could be considered an information leak in some contexts, so * the default setting is false here for optimum safety. */ 'display_role_in_exception' => false, /* * By default wildcard permission lookups are disabled. */ 'enable_wildcard_permission' => false, 'cache' => [ /* * By default all permissions are cached for 24 hours to speed up performance. * When permissions or roles are updated the cache is flushed automatically. */ 'expiration_time' => \DateInterval::createFromDateString('24 hours'), /* * The cache key used to store all permissions. */ 'key' => 'spatie.permission.cache', /* * You may optionally indicate a specific cache driver to use for permission and * role caching using any of the `store` drivers listed in the cache.php config * file. Using 'default' here means to use the `default` set in cache.php. */ 'store' => 'default', ], ]; data-transfer-object/composer.json 0000644 00000002130 15002154456 0013263 0 ustar 00 { "name": "spatie/data-transfer-object", "description": "Data transfer objects with batteries included", "keywords": [ "spatie", "data-transfer-object" ], "homepage": "https://github.com/spatie/data-transfer-object", "license": "MIT", "authors": [ { "name": "Brent Roose", "email": "brent@spatie.be", "homepage": "https://spatie.be", "role": "Developer" } ], "require": { "php": "^8.0" }, "require-dev": { "illuminate/collections": "^8.36", "larapack/dd": "^1.1", "phpunit/phpunit": "^9.5.5", "jetbrains/phpstorm-attributes": "^1.0" }, "autoload": { "psr-4": { "Spatie\\DataTransferObject\\": "src" } }, "autoload-dev": { "psr-4": { "Spatie\\DataTransferObject\\Tests\\": "tests" } }, "scripts": { "test": "vendor/bin/phpunit", "test-coverage": "vendor/bin/phpunit --coverage-html coverage" }, "config": { "sort-packages": true } } data-transfer-object/README.md 0000644 00000032432 15002154456 0012030 0 ustar 00 [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/support-ukraine.svg?t=1" />](https://supportukrainenow.org) # Data transfer objects with batteries included [](https://packagist.org/packages/spatie/data-transfer-object)  [](https://packagist.org/packages/spatie/data-transfer-object) ## Installation You can install the package via composer: ```bash composer require spatie/data-transfer-object ``` * **Note**: v3 of this package only supports `php:^8.0`. If you're looking for the older version, check out [v2](https://github.com/spatie/data-transfer-object/tree/v2). ## Support us [<img src="https://github-ads.s3.eu-central-1.amazonaws.com/data-transfer-object.jpg?t=1" width="419px" />](https://spatie.be/github-ad-click/data-transfer-object) We invest a lot of resources into creating [best in class open source packages](https://spatie.be/open-source). You can support us by [buying one of our paid products](https://spatie.be/open-source/support-us). We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on [our contact page](https://spatie.be/about-us). We publish all received postcards on [our virtual postcard wall](https://spatie.be/open-source/postcards). ## Usage The goal of this package is to make constructing objects from arrays of (serialized) data as easy as possible. Here's what a DTO looks like: ```php use Spatie\DataTransferObject\Attributes\MapFrom; use Spatie\DataTransferObject\DataTransferObject; class MyDTO extends DataTransferObject { public OtherDTO $otherDTO; public OtherDTOCollection $collection; #[CastWith(ComplexObjectCaster::class)] public ComplexObject $complexObject; public ComplexObjectWithCast $complexObjectWithCast; #[NumberBetween(1, 100)] public int $a; #[MapFrom('address.city')] public string $city; } ``` You could construct this DTO like so: ```php $dto = new MyDTO( a: 5, collection: [ ['id' => 1], ['id' => 2], ['id' => 3], ], complexObject: [ 'name' => 'test', ], complexObjectWithCast: [ 'name' => 'test', ], otherDTO: ['id' => 5], ); ``` Let's discuss all possibilities one by one. ## Named arguments Constructing a DTO can be done with named arguments. It's also possible to still use the old array notation. This example is equivalent to the one above. ```php $dto = new MyDTO([ 'a' => 5, 'collection' => [ ['id' => 1], ['id' => 2], ['id' => 3], ], 'complexObject' => [ 'name' => 'test', ], 'complexObjectWithCast' => [ 'name' => 'test', ], 'otherDTO' => ['id' => 5], ]); ``` ## Value casts If a DTO has a property that is another DTO or a DTO collection, the package will take care of automatically casting arrays of data to those DTOs: ```php $dto = new MyDTO( collection: [ // This will become an object of class OtherDTOCollection ['id' => 1], ['id' => 2], // Each item will be an instance of OtherDTO ['id' => 3], ], otherDTO: ['id' => 5], // This data will be cast to OtherDTO ); ``` ### Custom casters You can build your own caster classes, which will take whatever input they are given, and will cast that input to the desired result. Take a look at the `ComplexObject`: ```php class ComplexObject { public string $name; } ``` And its caster `ComplexObjectCaster`: ```php use Spatie\DataTransferObject\Caster; class ComplexObjectCaster implements Caster { /** * @param array|mixed $value * * @return mixed */ public function cast(mixed $value): ComplexObject { return new ComplexObject( name: $value['name'] ); } } ``` ### Class-specific casters Instead of specifying which caster should be used for each property, you can also define that caster on the target class itself: ```php class MyDTO extends DataTransferObject { public ComplexObjectWithCast $complexObjectWithCast; } ``` ```php #[CastWith(ComplexObjectWithCastCaster::class)] class ComplexObjectWithCast { public string $name; } ``` ### Default casters It's possible to define default casters on a DTO class itself. These casters will be used whenever a property with a given type is encountered within the DTO class. ```php #[ DefaultCast(DateTimeImmutable::class, DateTimeImmutableCaster::class), DefaultCast(MyEnum::class, EnumCaster::class), ] abstract class BaseDataTransferObject extends DataTransferObject { public MyEnum $status; // EnumCaster will be used public DateTimeImmutable $date; // DateTimeImmutableCaster will be used } ``` ### Using custom caster arguments Any caster can be passed custom arguments, the built-in [`ArrayCaster` implementation](https://github.com/spatie/data-transfer-object/blob/master/src/Casters/ArrayCaster.php) is a good example of how this may be used. Using named arguments when passing input to your caster will help make your code more clear, but they are not required. For example: ```php /** @var \Spatie\DataTransferObject\Tests\Foo[] */ #[CastWith(ArrayCaster::class, itemType: Foo::class)] public array $collectionWithNamedArguments; /** @var \Spatie\DataTransferObject\Tests\Foo[] */ #[CastWith(ArrayCaster::class, Foo::class)] public array $collectionWithoutNamedArguments; ``` Note that the first argument passed to the caster constructor is always the array with type(s) of the value being casted. All other arguments will be the ones passed as extra arguments in the `CastWith` attribute. ## Validation This package doesn't offer any specific validation functionality, but it does give you a way to build your own validation attributes. For example, `NumberBetween` is a user-implemented validation attribute: ```php class MyDTO extends DataTransferObject { #[NumberBetween(1, 100)] public int $a; } ``` It works like this under the hood: ```php #[Attribute(Attribute::TARGET_PROPERTY | Attribute::IS_REPEATABLE)] class NumberBetween implements Validator { public function __construct( private int $min, private int $max ) { } public function validate(mixed $value): ValidationResult { if ($value < $this->min) { return ValidationResult::invalid("Value should be greater than or equal to {$this->min}"); } if ($value > $this->max) { return ValidationResult::invalid("Value should be less than or equal to {$this->max}"); } return ValidationResult::valid(); } } ``` ## Mapping You can map a DTO property from a source property with a different name using the `#[MapFrom]` attribute. It works with a "dot" notation property name or an index. ```php class PostDTO extends DataTransferObject { #[MapFrom('postTitle')] public string $title; #[MapFrom('user.name')] public string $author; } $dto = new PostDTO([ 'postTitle' => 'Hello world', 'user' => [ 'name' => 'John Doe' ] ]); ``` ```php class UserDTO extends DataTransferObject { #[MapFrom(0)] public string $firstName; #[MapFrom(1)] public string $lastName; } $dto = new UserDTO(['John', 'Doe']); ``` Sometimes you also want to map them during the transformation to Array. A typical usecase would be transformation from camel case to snake case. For that you can use the `#[MapTo]` attribute. ```php class UserDTO extends DataTransferObject { #[MapFrom(0)] #[MapTo('first_name')] public string $firstName; #[MapFrom(1)] #[MapTo('last_name')] public string $lastName; } $dto = new UserDTO(['John', 'Doe']); $dto->toArray() // ['first_name' => 'John', 'last_name'=> 'Doe']; $dto->only('first_name')->toArray() // ['first_name' => 'John']; ``` ## Strict DTOs The previous version of this package added the `FlexibleDataTransferObject` class which allowed you to ignore properties that didn't exist on the DTO. This behaviour has been changed, all DTOs are flexible now by default, but you can make them strict by using the `#[Strict]` attribute: ```php class NonStrictDto extends DataTransferObject { public string $name; } // This works new NonStrictDto( name: 'name', unknown: 'unknown' ); ``` ```php use \Spatie\DataTransferObject\Attributes\Strict; #[Strict] class StrictDto extends DataTransferObject { public string $name; } // This throws a \Spatie\DataTransferObject\Exceptions\UnknownProperties exception new StrictDto( name: 'name', unknown: 'unknown' ); ``` ## Helper functions There are also some helper functions provided for working with multiple properties at once. ```php $postData->all(); $postData ->only('title', 'body') ->toArray(); $postData ->except('author') ->toArray(); ``` Note that `all()` will simply return all properties, while `toArray()` will cast nested DTOs to arrays as well. You can chain the `except()` and `only()` methods: ```php $postData ->except('title') ->except('body') ->toArray(); ``` It's important to note that `except()` and `only()` are immutable, they won't change the original data transfer object. ## Immutable DTOs and cloning This package doesn't force immutable objects since PHP doesn't support them, but you're always encouraged to keep your DTOs immutable. To help you, there's a `clone` method on every DTO which accepts data to override: ```php $clone = $original->clone(other: ['name' => 'a']); ``` Note that no data in `$original` is changed. ## Collections of DTOs This version removes the `DataTransferObjectCollection` class. Instead you can use simple casters and your own collection classes. Here's an example of casting a collection of DTOs to an array of DTOs: ```php class Bar extends DataTransferObject { /** @var \Spatie\DataTransferObject\Tests\Foo[] */ #[CastWith(FooArrayCaster::class)] public array $collectionOfFoo; } class Foo extends DataTransferObject { public string $name; } ``` ```php class FooArrayCaster implements Caster { public function cast(mixed $value): array { if (! is_array($value)) { throw new Exception("Can only cast arrays to Foo"); } return array_map( fn (array $data) => new Foo(...$data), $value ); } } ``` If you don't want the redundant typehint, or want extended collection functionality; you could create your own collection classes using any collection implementation. In this example, we use Laravel's: ```php class Bar extends DataTransferObject { #[CastWith(FooCollectionCaster::class)] public CollectionOfFoo $collectionOfFoo; } class Foo extends DataTransferObject { public string $name; } ``` ```php use Illuminate\Support\Collection; class CollectionOfFoo extends Collection { // Add the correct return type here for static analyzers to know which type of array this is public function offsetGet($key): Foo { return parent::offsetGet($key); } } ``` ```php class FooCollectionCaster implements Caster { public function cast(mixed $value): CollectionOfFoo { return new CollectionOfFoo(array_map( fn (array $data) => new Foo(...$data), $value )); } } ``` ## Simple arrays of DTOs For a simple array of DTOs, or an object that implements PHP's built-in `ArrayAccess`, consider using the `ArrayCaster` which requires an item type to be provided: ```php class Bar extends DataTransferObject { /** @var \Spatie\DataTransferObject\Tests\Foo[] */ #[CastWith(ArrayCaster::class, itemType: Foo::class)] public array $collectionOfFoo; } ``` ## Testing ``` bash composer test ``` ### Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ### Security If you've found a bug regarding security please mail [security@spatie.be](mailto:security@spatie.be) instead of using the issue tracker. ## Postcardware You're free to use this package, but if it makes it to your production environment we highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. Our address is: Spatie, Kruikstraat 22, 2018 Antwerp, Belgium. We publish all received postcards [on our company website](https://spatie.be/en/opensource/postcards). ## External tools - [json2dto](https://json2dto.atymic.dev): a GUI to convert JSON objects to DTO classes (with nesting support). Also provides a [CLI tool](https://github.com/atymic/json2dto#cli-tool) for local usage. - [Data Transfer Object Factory](https://github.com/anteris-dev/data-transfer-object-factory): Intelligently generates a DTO instance using the correct content for your properties based on its name and type. ## Credits - [Brent Roose](https://github.com/brendt) - [All Contributors](../../contributors) Our `Arr` class contains functions copied from Laravels `Arr` helper. ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. data-transfer-object/.github/workflows/style.yml 0000644 00000001130 15002154456 0016020 0 ustar 00 name: Check & fix styling on: [push] jobs: php-cs-fixer: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 with: ref: ${{ github.head_ref }} - name: Run PHP CS Fixer uses: docker://oskarstark/php-cs-fixer-ga with: args: --config=.php-cs-fixer.dist.php --allow-risky=yes - name: Commit changes uses: stefanzweifel/git-auto-commit-action@v4 with: commit_message: Fix styling data-transfer-object/.github/workflows/update-changelog.yml 0000644 00000001205 15002154456 0020072 0 ustar 00 name: "Update Changelog" on: release: types: [released] jobs: update: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 with: ref: main - name: Update Changelog uses: stefanzweifel/changelog-updater-action@v1 with: latest-version: ${{ github.event.release.name }} release-notes: ${{ github.event.release.body }} - name: Commit updated CHANGELOG uses: stefanzweifel/git-auto-commit-action@v4 with: branch: main commit_message: Update CHANGELOG file_pattern: CHANGELOG.md data-transfer-object/.github/workflows/test.yml 0000644 00000002012 15002154456 0015637 0 ustar 00 name: Test on: [push, pull_request] jobs: test: runs-on: ${{ matrix.os }} strategy: fail-fast: true matrix: os: [ubuntu-latest] php: [8.0, 8.1] dependency-version: [prefer-lowest, prefer-stable] name: PHP ${{ matrix.php }} - ${{ matrix.dependency-version }} - ${{ matrix.os }} steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup PHP uses: shivammathur/setup-php@v2 with: php-version: ${{ matrix.php }} extensions: mbstring, sqlite, pdo_sqlite, iconv coverage: none - name: Setup problem matchers run: | echo "::add-matcher::${{ runner.tool_cache }}/php.json" echo "::add-matcher::${{ runner.tool_cache }}/phpunit.json" - name: Install dependencies run: | composer update --${{ matrix.dependency-version }} --prefer-dist --no-interaction - name: Execute tests run: vendor/bin/phpunit data-transfer-object/.github/FUNDING.yml 0000644 00000000100 15002154456 0013711 0 ustar 00 github: spatie custom: https://spatie.be/open-source/support-us data-transfer-object/src/Arr.php 0000644 00000004516 15002154456 0012577 0 ustar 00 <?php namespace Spatie\DataTransferObject; use ArrayAccess; class Arr { public static function only($array, $keys): array { return array_intersect_key($array, array_flip((array) $keys)); } public static function except($array, $keys): array { return static::forget($array, $keys); } public static function forget($array, $keys): array { $keys = (array) $keys; if (count($keys) === 0) { return $array; } foreach ($keys as $key) { // If the exact key exists in the top-level, remove it if (static::exists($array, $key)) { unset($array[$key]); continue; } // Check if the key is using dot-notation if (! str_contains($key, '.')) { continue; } // If we are dealing with dot-notation, recursively handle i $parts = explode('.', $key); $key = array_shift($parts); if (static::exists($array, $key) && static::accessible($array[$key])) { $array[$key] = static::forget($array[$key], implode('.', $parts)); if (count($array[$key]) === 0) { unset($array[$key]); } } } return $array; } public static function get($array, $key, $default = null) { if (! static::accessible($array)) { return $default; } if (is_null($key)) { return $array; } if (static::exists($array, $key)) { return $array[$key]; } if (strpos($key, '.') === false) { return $array[$key] ?? $default; } foreach (explode('.', $key) as $segment) { if (static::accessible($array) && static::exists($array, $segment)) { $array = $array[$segment]; } else { return $default; } } return $array; } public static function accessible($value) { return is_array($value) || $value instanceof ArrayAccess; } public static function exists($array, $key): bool { if ($array instanceof ArrayAccess) { return $array->offsetExists($key); } return array_key_exists($key, $array); } } data-transfer-object/src/Caster.php 0000644 00000000161 15002154456 0013264 0 ustar 00 <?php namespace Spatie\DataTransferObject; interface Caster { public function cast(mixed $value): mixed; } data-transfer-object/src/Exceptions/InvalidCasterClass.php 0000644 00000000607 15002154456 0017707 0 ustar 00 <?php namespace Spatie\DataTransferObject\Exceptions; use Exception; use Spatie\DataTransferObject\Caster; class InvalidCasterClass extends Exception { public function __construct(string $className) { $expected = Caster::class; parent::__construct( "Class `{$className}` doesn't implement {$expected} and can't be used as a caster" ); } } data-transfer-object/src/Exceptions/UnknownProperties.php 0000644 00000000515 15002154456 0017703 0 ustar 00 <?php namespace Spatie\DataTransferObject\Exceptions; use Exception; class UnknownProperties extends Exception { public static function new(string $dtoClass, array $fields): self { $properties = json_encode($fields); return new self("Unknown properties provided to `{$dtoClass}`: {$properties}"); } } data-transfer-object/src/Exceptions/ValidationException.php 0000644 00000001464 15002154456 0020144 0 ustar 00 <?php namespace Spatie\DataTransferObject\Exceptions; use Exception; use Spatie\DataTransferObject\DataTransferObject; class ValidationException extends Exception { public function __construct( public DataTransferObject $dataTransferObject, public array $validationErrors, ) { $className = $dataTransferObject::class; $messages = []; foreach ($validationErrors as $fieldName => $errorsForField) { /** @var \Spatie\DataTransferObject\Validation\ValidationResult $errorForField */ foreach ($errorsForField as $errorForField) { $messages[] = "\t - `{$className}->{$fieldName}`: {$errorForField->message}"; } } parent::__construct("Validation errors:" . PHP_EOL . implode(PHP_EOL, $messages)); } } data-transfer-object/src/Validator.php 0000644 00000000277 15002154456 0014000 0 ustar 00 <?php namespace Spatie\DataTransferObject; use Spatie\DataTransferObject\Validation\ValidationResult; interface Validator { public function validate(mixed $value): ValidationResult; } data-transfer-object/src/Reflection/DataTransferObjectClass.php 0000644 00000004524 15002154456 0020637 0 ustar 00 <?php namespace Spatie\DataTransferObject\Reflection; use ReflectionClass; use ReflectionProperty; use Spatie\DataTransferObject\Attributes\Strict; use Spatie\DataTransferObject\DataTransferObject; use Spatie\DataTransferObject\Exceptions\ValidationException; class DataTransferObjectClass { private ReflectionClass $reflectionClass; private DataTransferObject $dataTransferObject; private bool $isStrict; public function __construct(DataTransferObject $dataTransferObject) { $this->reflectionClass = new ReflectionClass($dataTransferObject); $this->dataTransferObject = $dataTransferObject; } /** * @return \Spatie\DataTransferObject\Reflection\DataTransferObjectProperty[] */ public function getProperties(): array { $publicProperties = array_filter( $this->reflectionClass->getProperties(ReflectionProperty::IS_PUBLIC), fn (ReflectionProperty $property) => ! $property->isStatic() ); return array_map( fn (ReflectionProperty $property) => new DataTransferObjectProperty( $this->dataTransferObject, $property ), $publicProperties ); } public function validate(): void { $validationErrors = []; foreach ($this->getProperties() as $property) { $validators = $property->getValidators(); foreach ($validators as $validator) { $result = $validator->validate($property->getValue()); if ($result->isValid) { continue; } $validationErrors[$property->name][] = $result; } } if (count($validationErrors)) { throw new ValidationException($this->dataTransferObject, $validationErrors); } } public function isStrict(): bool { if (! isset($this->isStrict)) { $attribute = null; $reflectionClass = $this->reflectionClass; while ($attribute === null && $reflectionClass !== false) { $attribute = $reflectionClass->getAttributes(Strict::class)[0] ?? null; $reflectionClass = $reflectionClass->getParentClass(); } $this->isStrict = $attribute !== null; } return $this->isStrict; } } data-transfer-object/src/Reflection/DataTransferObjectProperty.php 0000644 00000012044 15002154456 0021412 0 ustar 00 <?php namespace Spatie\DataTransferObject\Reflection; use JetBrains\PhpStorm\Immutable; use ReflectionAttribute; use ReflectionClass; use ReflectionNamedType; use ReflectionProperty; use ReflectionType; use ReflectionUnionType; use Spatie\DataTransferObject\Attributes\CastWith; use Spatie\DataTransferObject\Attributes\DefaultCast; use Spatie\DataTransferObject\Attributes\MapFrom; use Spatie\DataTransferObject\Caster; use Spatie\DataTransferObject\DataTransferObject; use Spatie\DataTransferObject\Validator; class DataTransferObjectProperty { #[Immutable] public string $name; private DataTransferObject $dataTransferObject; private ReflectionProperty $reflectionProperty; private ?Caster $caster; public function __construct( DataTransferObject $dataTransferObject, ReflectionProperty $reflectionProperty ) { $this->dataTransferObject = $dataTransferObject; $this->reflectionProperty = $reflectionProperty; $this->name = $this->resolveMappedProperty(); $this->caster = $this->resolveCaster(); } public function setValue(mixed $value): void { if ($this->caster && $value !== null) { $value = $this->caster->cast($value); } $this->reflectionProperty->setValue($this->dataTransferObject, $value); } /** * @return \Spatie\DataTransferObject\Validator[] */ public function getValidators(): array { $attributes = $this->reflectionProperty->getAttributes( Validator::class, ReflectionAttribute::IS_INSTANCEOF ); return array_map( fn (ReflectionAttribute $attribute) => $attribute->newInstance(), $attributes ); } public function getValue(): mixed { return $this->reflectionProperty->getValue($this->dataTransferObject); } public function getDefaultValue(): mixed { return $this->reflectionProperty->getDefaultValue(); } private function resolveCaster(): ?Caster { $attributes = $this->reflectionProperty->getAttributes(CastWith::class); if (! count($attributes)) { $attributes = $this->resolveCasterFromType(); } if (! count($attributes)) { return $this->resolveCasterFromDefaults(); } /** @var \Spatie\DataTransferObject\Attributes\CastWith $attribute */ $attribute = $attributes[0]->newInstance(); return new $attribute->casterClass( array_map(fn ($type) => $this->resolveTypeName($type), $this->extractTypes()), ...$attribute->args ); } private function resolveCasterFromType(): array { foreach ($this->extractTypes() as $type) { $name = $this->resolveTypeName($type); if (! class_exists($name)) { continue; } $reflectionClass = new ReflectionClass($name); do { $attributes = $reflectionClass->getAttributes(CastWith::class); $reflectionClass = $reflectionClass->getParentClass(); } while (! count($attributes) && $reflectionClass); if (count($attributes) > 0) { return $attributes; } } return []; } private function resolveCasterFromDefaults(): ?Caster { $defaultCastAttributes = []; $class = $this->reflectionProperty->getDeclaringClass(); do { array_push($defaultCastAttributes, ...$class->getAttributes(DefaultCast::class)); $class = $class->getParentClass(); } while ($class !== false); if (! count($defaultCastAttributes)) { return null; } foreach ($defaultCastAttributes as $defaultCastAttribute) { /** @var \Spatie\DataTransferObject\Attributes\DefaultCast $defaultCast */ $defaultCast = $defaultCastAttribute->newInstance(); if ($defaultCast->accepts($this->reflectionProperty)) { return $defaultCast->resolveCaster(); } } return null; } private function resolveMappedProperty(): string | int { $attributes = $this->reflectionProperty->getAttributes(MapFrom::class); if (! count($attributes)) { return $this->reflectionProperty->name; } return $attributes[0]->newInstance()->name; } /** * @return ReflectionNamedType[] */ private function extractTypes(): array { $type = $this->reflectionProperty->getType(); if (! $type) { return []; } return match ($type::class) { ReflectionNamedType::class => [$type], ReflectionUnionType::class => $type->getTypes(), }; } private function resolveTypeName(ReflectionType $type): string { return match ($type->getName()) { 'self' => $this->dataTransferObject::class, 'parent' => get_parent_class($this->dataTransferObject), default => $type->getName(), }; } } data-transfer-object/src/Casters/DataTransferObjectCaster.php 0000644 00000001051 15002154456 0020315 0 ustar 00 <?php namespace Spatie\DataTransferObject\Casters; use Spatie\DataTransferObject\Caster; use Spatie\DataTransferObject\DataTransferObject; class DataTransferObjectCaster implements Caster { public function __construct( private array $classNames ) { } public function cast(mixed $value): DataTransferObject { foreach ($this->classNames as $className) { if ($value instanceof $className) { return $value; } } return new $this->classNames[0]($value); } } data-transfer-object/src/Casters/ArrayCaster.php 0000644 00000003551 15002154456 0015675 0 ustar 00 <?php namespace Spatie\DataTransferObject\Casters; use ArrayAccess; use LogicException; use Spatie\DataTransferObject\Caster; use Traversable; class ArrayCaster implements Caster { public function __construct( private array $types, private string $itemType, ) { } public function cast(mixed $value): array | ArrayAccess { foreach ($this->types as $type) { if ($type == 'array') { return $this->mapInto( destination: [], items: $value ); } if (is_subclass_of($type, ArrayAccess::class)) { return $this->mapInto( destination: new $type(), items: $value ); } } throw new LogicException( "Caster [ArrayCaster] may only be used to cast arrays or objects that implement ArrayAccess." ); } private function mapInto(array | ArrayAccess $destination, mixed $items): array | ArrayAccess { if ($destination instanceof ArrayAccess && ! is_subclass_of($destination, Traversable::class)) { throw new LogicException( "Caster [ArrayCaster] may only be used to cast ArrayAccess objects that are traversable." ); } foreach ($items as $key => $item) { $destination[$key] = $this->castItem($item); } return $destination; } private function castItem(mixed $data) { if ($data instanceof $this->itemType) { return $data; } if (is_array($data)) { return new $this->itemType(...$data); } throw new LogicException( "Caster [ArrayCaster] each item must be an array or an instance of the specified item type [{$this->itemType}]." ); } } data-transfer-object/src/Casters/EnumCaster.php 0000644 00000001512 15002154456 0015516 0 ustar 00 <?php namespace Spatie\DataTransferObject\Casters; use LogicException; use Spatie\DataTransferObject\Caster; class EnumCaster implements Caster { public function __construct( private array $types, private string $enumType ) { } public function cast(mixed $value): mixed { if ($value instanceof $this->enumType) { return $value; } if (! is_subclass_of($this->enumType, 'BackedEnum')) { throw new LogicException("Caster [EnumCaster] may only be used to cast backed enums. Received [$this->enumType]."); } $castedValue = $this->enumType::tryFrom($value); if ($castedValue === null) { throw new LogicException("Couldn't cast enum [$this->enumType] with value [$value]"); } return $castedValue; } } data-transfer-object/src/Validation/ValidationResult.php 0000644 00000001057 15002154456 0017433 0 ustar 00 <?php namespace Spatie\DataTransferObject\Validation; use JetBrains\PhpStorm\Immutable; class ValidationResult { public function __construct( #[Immutable] public bool $isValid, #[Immutable] public ?string $message = null ) { } public static function valid(): self { return new self( isValid: true, ); } public static function invalid(string $message): self { return new self( isValid: false, message: $message, ); } } data-transfer-object/src/DataTransferObject.php 0000644 00000006234 15002154456 0015557 0 ustar 00 <?php namespace Spatie\DataTransferObject; use ReflectionClass; use ReflectionProperty; use Spatie\DataTransferObject\Attributes\CastWith; use Spatie\DataTransferObject\Attributes\MapTo; use Spatie\DataTransferObject\Casters\DataTransferObjectCaster; use Spatie\DataTransferObject\Exceptions\UnknownProperties; use Spatie\DataTransferObject\Reflection\DataTransferObjectClass; #[CastWith(DataTransferObjectCaster::class)] abstract class DataTransferObject { protected array $exceptKeys = []; protected array $onlyKeys = []; public function __construct(...$args) { if (is_array($args[0] ?? null)) { $args = $args[0]; } $class = new DataTransferObjectClass($this); foreach ($class->getProperties() as $property) { $property->setValue(Arr::get($args, $property->name, $property->getDefaultValue())); $args = Arr::forget($args, $property->name); } if ($class->isStrict() && count($args)) { throw UnknownProperties::new(static::class, array_keys($args)); } $class->validate(); } public static function arrayOf(array $arrayOfParameters): array { return array_map( fn (mixed $parameters) => new static($parameters), $arrayOfParameters ); } public function all(): array { $data = []; $class = new ReflectionClass(static::class); $properties = $class->getProperties(ReflectionProperty::IS_PUBLIC); foreach ($properties as $property) { if ($property->isStatic()) { continue; } $mapToAttribute = $property->getAttributes(MapTo::class); $name = count($mapToAttribute) ? $mapToAttribute[0]->newInstance()->name : $property->getName(); $data[$name] = $property->getValue($this); } return $data; } public function only(string ...$keys): static { $dataTransferObject = clone $this; $dataTransferObject->onlyKeys = [...$this->onlyKeys, ...$keys]; return $dataTransferObject; } public function except(string ...$keys): static { $dataTransferObject = clone $this; $dataTransferObject->exceptKeys = [...$this->exceptKeys, ...$keys]; return $dataTransferObject; } public function clone(...$args): static { return new static(...array_merge($this->toArray(), $args)); } public function toArray(): array { if (count($this->onlyKeys)) { $array = Arr::only($this->all(), $this->onlyKeys); } else { $array = Arr::except($this->all(), $this->exceptKeys); } $array = $this->parseArray($array); return $array; } protected function parseArray(array $array): array { foreach ($array as $key => $value) { if ($value instanceof DataTransferObject) { $array[$key] = $value->toArray(); continue; } if (! is_array($value)) { continue; } $array[$key] = $this->parseArray($value); } return $array; } } data-transfer-object/src/Attributes/MapTo.php 0000644 00000000347 15002154456 0015217 0 ustar 00 <?php namespace Spatie\DataTransferObject\Attributes; use Attribute; #[Attribute(Attribute::TARGET_CLASS | Attribute::TARGET_PROPERTY)] class MapTo { public function __construct( public string $name, ) { } } data-transfer-object/src/Attributes/DefaultCast.php 0000644 00000002241 15002154456 0016371 0 ustar 00 <?php namespace Spatie\DataTransferObject\Attributes; use Attribute; use JetBrains\PhpStorm\Immutable; use ReflectionNamedType; use ReflectionProperty; use ReflectionUnionType; use Spatie\DataTransferObject\Caster; #[Attribute(Attribute::TARGET_CLASS | Attribute::IS_REPEATABLE)] class DefaultCast { public function __construct( #[Immutable] private string $targetClass, #[Immutable] private string $casterClass, ) { } public function accepts(ReflectionProperty $property): bool { $type = $property->getType(); /** @var \ReflectionNamedType[]|null $types */ $types = match ($type::class) { ReflectionNamedType::class => [$type], ReflectionUnionType::class => $type->getTypes(), default => null, }; if (! $types) { return false; } foreach ($types as $type) { if ($type->getName() !== $this->targetClass) { continue; } return true; } return false; } public function resolveCaster(): Caster { return new $this->casterClass(); } } data-transfer-object/src/Attributes/Strict.php 0000644 00000000177 15002154456 0015450 0 ustar 00 <?php namespace Spatie\DataTransferObject\Attributes; use Attribute; #[Attribute(Attribute::TARGET_CLASS)] class Strict { } data-transfer-object/src/Attributes/MapFrom.php 0000644 00000000357 15002154456 0015541 0 ustar 00 <?php namespace Spatie\DataTransferObject\Attributes; use Attribute; #[Attribute(Attribute::TARGET_CLASS | Attribute::TARGET_PROPERTY)] class MapFrom { public function __construct( public string | int $name, ) { } } data-transfer-object/src/Attributes/CastWith.php 0000644 00000001055 15002154456 0015722 0 ustar 00 <?php namespace Spatie\DataTransferObject\Attributes; use Attribute; use Spatie\DataTransferObject\Caster; use Spatie\DataTransferObject\Exceptions\InvalidCasterClass; #[Attribute(Attribute::TARGET_CLASS | Attribute::TARGET_PROPERTY)] class CastWith { public array $args; public function __construct( public string $casterClass, mixed ...$args ) { if (! is_subclass_of($this->casterClass, Caster::class)) { throw new InvalidCasterClass($this->casterClass); } $this->args = $args; } } data-transfer-object/LICENSE.md 0000644 00000002102 15002154456 0012144 0 ustar 00 The MIT License (MIT) Copyright (c) Spatie bvba <info@spatie.be> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. data-transfer-object/.php-cs-fixer.dist.php 0000644 00000002400 15002154456 0014577 0 ustar 00 <?php $finder = Symfony\Component\Finder\Finder::create() ->notPath('docs/*') ->notPath('vendor') ->in([ __DIR__.'/src', __DIR__.'/tests', ]) ->name('*.php') ->ignoreDotFiles(true) ->ignoreVCS(true); return (new PhpCsFixer\Config()) ->setRules([ '@PSR12' => true, 'array_syntax' => ['syntax' => 'short'], 'ordered_imports' => ['sort_algorithm' => 'alpha'], 'no_unused_imports' => true, 'not_operator_with_successor_space' => true, 'trailing_comma_in_multiline' => true, 'phpdoc_scalar' => true, 'unary_operator_spaces' => true, 'binary_operator_spaces' => true, 'logical_operators' => true, 'blank_line_before_statement' => [ 'statements' => ['break', 'continue', 'declare', 'return', 'throw', 'try'], ], 'phpdoc_single_line_var_spacing' => true, 'phpdoc_var_without_name' => true, 'class_attributes_separation' => [ 'elements' => [ 'method' => 'one' ], ], 'method_argument_space' => [ 'on_multiline' => 'ensure_fully_multiline', 'keep_multiple_spaces_after_comma' => true, ], ]) ->setFinder($finder); data-transfer-object/CHANGELOG.md 0000644 00000015527 15002154456 0012370 0 ustar 00 # Changelog All notable changes to `data-transfer-object` will be documented in this file ## 3.9.0 - 2022-09-14 ### What's Changed - allow enum casting not only enum value casting by @regnerisch in https://github.com/spatie/data-transfer-object/pull/301 ### New Contributors - @regnerisch made their first contribution in https://github.com/spatie/data-transfer-object/pull/301 **Full Changelog**: https://github.com/spatie/data-transfer-object/compare/3.8.1...3.9.0 ## 3.8.1 - 2022-06-02 ### What's Changed - Fixed bug #249 by @gerpo in https://github.com/spatie/data-transfer-object/pull/251 **Full Changelog**: https://github.com/spatie/data-transfer-object/compare/3.8.0...3.8.1 ## 3.8.0 - 2022-06-02 ### What's Changed - Added enum caster by @Elnadrion in https://github.com/spatie/data-transfer-object/pull/277 ### New Contributors - @Raja-Omer-Mustafa made their first contribution in https://github.com/spatie/data-transfer-object/pull/271 - @Elnadrion made their first contribution in https://github.com/spatie/data-transfer-object/pull/277 **Full Changelog**: https://github.com/spatie/data-transfer-object/compare/3.7.3...3.8.0 ## 3.7.3 - 2022-01-10 ## What's Changed - Stop suggesting phpstan/phpstan by @ymilin in https://github.com/spatie/data-transfer-object/pull/264 ## New Contributors - @sergiy-petrov made their first contribution in https://github.com/spatie/data-transfer-object/pull/246 - @damms005 made their first contribution in https://github.com/spatie/data-transfer-object/pull/255 - @ymilin made their first contribution in https://github.com/spatie/data-transfer-object/pull/264 **Full Changelog**: https://github.com/spatie/data-transfer-object/compare/3.7.2...3.7.3 ## 3.7.2 - 2021-09-17 - `#[Strict]` is passed down the inheritance chain so children are strict when parent is strict (#239) ## 3.7.1 - 2021-09-09 - Cast properties with self or parent type (#236) ## 3.7.0 - 2021-08-26 - Add `#[MapTo]` support (#233) ## 3.6.2 - 2021-08-25 - Correct behavior of Arr::forget with dot keys (#231) ## 3.6.1 - 2021-08-17 - Fix array assignment bug with strict dto's (#225) ## 3.6.0 - 2021-08-12 - Support mapped properties (#224) ## 3.5.0 - 2021-08-11 - Support union types in casters (#210) ## 3.4.0 - 2021-08-10 - Fix for an empty value being created when casting `ArrayAccess` objects (#216) - Add logic exception when attempting to cast `ArrayAccess` objects that are not traversable (#216) - Allow the `ArrayCaster` to retain values that are already instances of the `itemType` (#217) ## 3.3.0 - 2021-06-01 - Expose DTO and validation error array in ValidationException (#213) ## 3.2.0 - 2021-05-31 - Support generic casters (#199) - Add `ArrayCaster` - Add casting of objects that implement `ArrayAccess` to the `ArrayCaster` (#206) - Fix for caster subclass check (#204) ## 3.1.1 - 2021-04-26 - Make `DefaultCast` repeatable (#202) ## 3.1.0 - 2021-04-21 - Add `DataTransferObject::clone(...$args)` ## 3.0.4 - 2021-04-14 - Support union types (#185) - Resolve default cast from parent classes (#189) - Support default values (#191) ## 3.0.3 - 2021-04-08 - Fix when nested DTO have casted field (#178) ## 3.0.2 - 2021-04-02 - Allow valid DTOs to be passed to caster (#177) ## 3.0.1 - 2021-04-02 - Fix for null values with casters ## 3.0.0 - 2021-04-02 This package now focuses only on object creation by adding easy-to-use casting and data validation functionality. All runtime type checks are gone in favour of the improved type system in PHP 8. - Require `php:^8.0` - Removed all runtime type checking functionality, you should use typed properties and a static analysis tool like Psalm or PhpStan - Removed `Spatie\DataTransferObject\DataTransferObjectCollection` - Removed `Spatie\DataTransferObject\FlexibleDataTransferObject`, all DTOs are now considered flexible - Removed runtime immutable DTOs, you should use static analysis instead - Added `Spatie\DataTransferObject\Validator` - Added `Spatie\DataTransferObject\Validation\ValidationResult` - Added `#[DefaultCast]` - Added `#[CastWith]` - Added `Spatie\DataTransferObject\Caster` - Added `#[Strict]` ## 2.8.3 - 2021-02-12 - Add support for using `collection` internally ## 2.8.2 - 2021-02-11 This might be a breaking change, but it was required for a bugfix - Prevent DataTransferObjectCollection from iterating over array copy (#166) ## 2.8.1 - 2021-02-10 - Fix for incorrect return type (#164) ## 2.8.0 - 2021-01-27 - Allow the traversal of collections with string keys ## 2.7.0 - 2021-01-21 - Cast nested collections (#117) ## 2.6.0 - 2020-11-26 - Support PHP 8 ## 2.5.0 - 2020-08-28 - Group type errors (#130) ## 2.4.0 - 2020-08-28 - Support for `array<int, string>` syntax (#136) ## 2.3.0 - 2020-08-19 - Add PHPStan extension to support `checkUninitializedProperties: true` (#135) ## 2.2.1 - 2020-05-13 - Validator for typed 7.4 properties (#109) ## 2.2.0 - 2020-05-08 - Add support for typed properties to DTO casting in PHP 7.4 ## 2.0.0 - 2020-04-28 - Bump minimum required PHP version to 7.4 - Support for nested immutable DTOs (#86) ## 1.13.3 - 2020-01-29 - Ignore static properties when serializing (#88) ## 1.13.2 - 2020-01-08 - DataTransferObjectError::invalidType : get actual type before mutating $value for the error message (#81) ## 1.13.1 - 2020-01-08 - Improve extendability of DTOs (#80) ## 1.13.0 - 2020-01-08 - Ignore static properties (#82) - Add `DataTransferObject::arrayOf` (#83) ## 1.12.0 - 2019-12-19 - Improved performance by adding a cache (#79) - Add `FlexibleDataTransferObject` which allows for unknown properties to be ignored ## 1.11.0 - 2019-11-28 (#71) - Add `iterable` and `iterable<\Type>` support ## 1.10.0 - 2019-10-16 - Allow a DTO to be constructed without an array (#68) ## 1.9.1 - 2019-10-03 - Improve type error message ## 1.9.0 - 2019-08-30 - Add DataTransferObjectCollection::items() ## 1.8.0 - 2019-03-18 - Support immutability ## 1.7.1 - 2019-02-11 - Fixes #47, allowing empty dto's to be cast to using an empty array. ## 1.7.0 - 2019-02-04 - Nested array DTO casting supported. ## 1.6.6 - 2018-12-04 - Properly support `float`. ## 1.6.5 - 2018-11-20 - Fix uninitialised error with default value. ## 1.6.4 - 2018-11-15 - Don't use `allValues` anymore. ## 1.6.3 - 2018-11-14 - Support nested collections in collections - Cleanup code ## 1.6.2 - 2018-11-14 - Remove too much magic in nested array casting ## 1.6.1 - 2018-11-14 - Support nested `toArray` in collections. ## 1.6.0 - 2018-11-14 - Support nested `toArray`. ## 1.5.1 - 2018-11-07 - Add strict type declarations ## 1.5.0 - 2018-11-07 - Add auto casting of nested DTOs ## 1.4.0 - 2018-11-05 - Rename to data-transfer-object ## 1.2.0 - 2018-10-30 - Add uninitialized errors. ## 1.1.1 - 2018-10-25 - Support instanceof on interfaces when type checking ## 1.1.0 - 2018-10-24 - proper support for collections of value objects ## 1.0.0 - 2018-10-24 - initial release flare-client-php/composer.json 0000644 00000003456 15002154456 0012432 0 ustar 00 { "name": "spatie/flare-client-php", "description": "Send PHP errors to Flare", "keywords": [ "spatie", "flare", "exception", "reporting" ], "homepage": "https://github.com/spatie/flare-client-php", "license": "MIT", "require": { "php": "^8.0", "illuminate/pipeline": "^8.0|^9.0|^10.0|^11.0", "spatie/backtrace": "^1.5.2", "symfony/http-foundation": "^5.2|^6.0|^7.0", "symfony/mime": "^5.2|^6.0|^7.0", "symfony/process": "^5.2|^6.0|^7.0", "symfony/var-dumper": "^5.2|^6.0|^7.0" }, "require-dev": { "dms/phpunit-arraysubset-asserts": "^0.5.0", "phpstan/extension-installer": "^1.1", "phpstan/phpstan-deprecation-rules": "^1.0", "phpstan/phpstan-phpunit": "^1.0", "spatie/phpunit-snapshot-assertions": "^4.0|^5.0", "pestphp/pest": "^1.20|^2.0" }, "autoload": { "psr-4": { "Spatie\\FlareClient\\": "src" }, "files": [ "src/helpers.php" ] }, "autoload-dev": { "psr-4": { "Spatie\\FlareClient\\Tests\\": "tests" } }, "scripts": { "analyse": "vendor/bin/phpstan analyse", "baseline": "vendor/bin/phpstan analyse --generate-baseline", "format": "vendor/bin/php-cs-fixer fix --allow-risky=yes", "test": "vendor/bin/pest", "test-coverage": "vendor/bin/phpunit --coverage-html coverage" }, "config": { "sort-packages": true, "allow-plugins": { "pestphp/pest-plugin": true, "phpstan/extension-installer": true } }, "prefer-stable": true, "minimum-stability": "dev", "extra": { "branch-alias": { "dev-main": "1.3.x-dev" } } } flare-client-php/README.md 0000644 00000003400 15002154456 0011154 0 ustar 00 # Send PHP errors to Flare [](https://packagist.org/packages/spatie/flare-client-php) [](https://github.com/spatie/flare-client-php/actions/workflows/run-tests.yml) [](https://github.com/spatie/flare-client-php/actions/workflows/phpstan.yml) [](https://packagist.org/packages/spatie/flare-client-php) This repository contains the PHP client to send errors and exceptions to [Flare](https://flareapp.io). The client can be installed using composer and works for PHP 8.0 and above. Using Laravel? You probably want to use [Ignition for Laravel](https://github.com/spatie/laravel-ignition). It comes with a beautiful error page and has the Flare client built in. 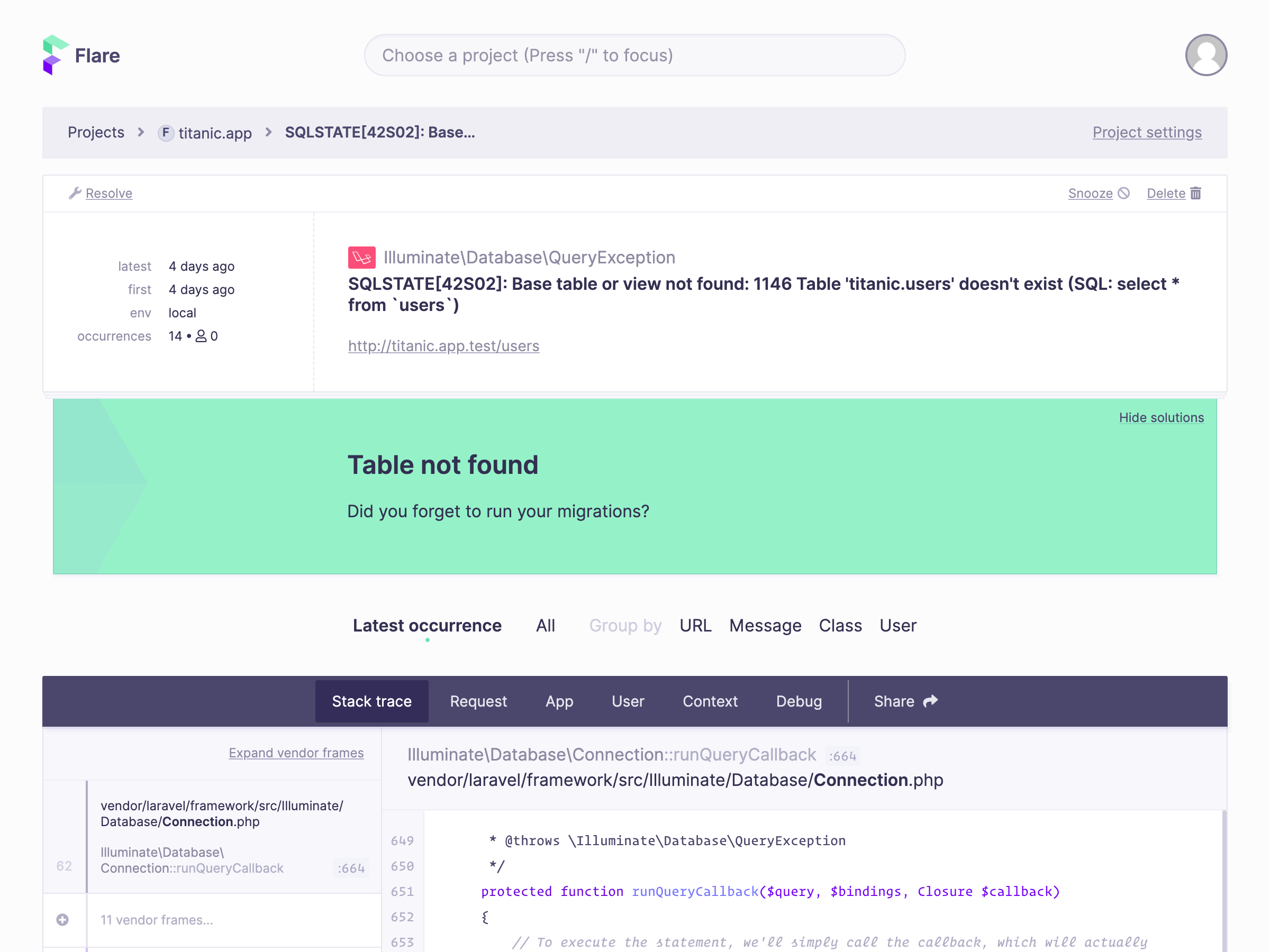 ## Documentation You can find the documentation of this package at [the docs of Flare](https://flareapp.io/docs/flare/general/welcome-to-flare). ## Changelog Please see [CHANGELOG](CHANGELOG.md) for more information on what has changed recently. ## Testing ``` bash composer test ``` ## Contributing Please see [CONTRIBUTING](https://github.com/spatie/.github/blob/main/CONTRIBUTING.md) for details. ## Security If you discover any security related issues, please email support@flareapp.io instead of using the issue tracker. ## License The MIT License (MIT). Please see [License File](LICENSE.md) for more information. flare-client-php/src/FlareMiddleware/RemoveRequestIp.php 0000644 00000000557 15002154456 0017335 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Spatie\FlareClient\Report; class RemoveRequestIp implements FlareMiddleware { public function handle(Report $report, $next) { $context = $report->allContext(); $context['request']['ip'] = null; $report->userProvidedContext($context); return $next($report); } } flare-client-php/src/FlareMiddleware/CensorRequestBodyFields.php 0000644 00000001275 15002154456 0021003 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Spatie\FlareClient\Report; class CensorRequestBodyFields implements FlareMiddleware { protected array $fieldNames = []; public function __construct(array $fieldNames) { $this->fieldNames = $fieldNames; } public function handle(Report $report, $next) { $context = $report->allContext(); foreach ($this->fieldNames as $fieldName) { if (isset($context['request_data']['body'][$fieldName])) { $context['request_data']['body'][$fieldName] = '<CENSORED>'; } } $report->userProvidedContext($context); return $next($report); } } flare-client-php/src/FlareMiddleware/AddGitInformation.php 0000644 00000004073 15002154456 0017575 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Closure; use Spatie\FlareClient\Report; use Symfony\Component\Process\Process; use Throwable; class AddGitInformation { protected ?string $baseDir = null; public function handle(Report $report, Closure $next) { try { $this->baseDir = $this->getGitBaseDirectory(); if (! $this->baseDir) { return $next($report); } $report->group('git', [ 'hash' => $this->hash(), 'message' => $this->message(), 'tag' => $this->tag(), 'remote' => $this->remote(), 'isDirty' => ! $this->isClean(), ]); } catch (Throwable) { } return $next($report); } protected function hash(): ?string { return $this->command("git log --pretty=format:'%H' -n 1") ?: null; } protected function message(): ?string { return $this->command("git log --pretty=format:'%s' -n 1") ?: null; } protected function tag(): ?string { return $this->command('git describe --tags --abbrev=0') ?: null; } protected function remote(): ?string { return $this->command('git config --get remote.origin.url') ?: null; } protected function isClean(): bool { return empty($this->command('git status -s')); } protected function getGitBaseDirectory(): ?string { /** @var Process $process */ $process = Process::fromShellCommandline("echo $(git rev-parse --show-toplevel)")->setTimeout(1); $process->run(); if (! $process->isSuccessful()) { return null; } $directory = trim($process->getOutput()); if (! file_exists($directory)) { return null; } return $directory; } protected function command($command) { $process = Process::fromShellCommandline($command, $this->baseDir)->setTimeout(1); $process->run(); return trim($process->getOutput()); } } flare-client-php/src/FlareMiddleware/AddEnvironmentInformation.php 0000644 00000000537 15002154456 0021357 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Closure; use Spatie\FlareClient\Report; class AddEnvironmentInformation implements FlareMiddleware { public function handle(Report $report, Closure $next) { $report->group('env', [ 'php_version' => phpversion(), ]); return $next($report); } } flare-client-php/src/FlareMiddleware/AddSolutions.php 0000644 00000001472 15002154456 0016643 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Closure; use Spatie\FlareClient\Report; use Spatie\Ignition\Contracts\SolutionProviderRepository; class AddSolutions implements FlareMiddleware { protected SolutionProviderRepository $solutionProviderRepository; public function __construct(SolutionProviderRepository $solutionProviderRepository) { $this->solutionProviderRepository = $solutionProviderRepository; } public function handle(Report $report, Closure $next) { if ($throwable = $report->getThrowable()) { $solutions = $this->solutionProviderRepository->getSolutionsForThrowable($throwable); foreach ($solutions as $solution) { $report->addSolution($solution); } } return $next($report); } } flare-client-php/src/FlareMiddleware/AddNotifierName.php 0000644 00000000521 15002154456 0017216 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Spatie\FlareClient\Report; class AddNotifierName implements FlareMiddleware { public const NOTIFIER_NAME = 'Flare Client'; public function handle(Report $report, $next) { $report->notifierName(static::NOTIFIER_NAME); return $next($report); } } flare-client-php/src/FlareMiddleware/FlareMiddleware.php 0000644 00000000274 15002154456 0017261 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Closure; use Spatie\FlareClient\Report; interface FlareMiddleware { public function handle(Report $report, Closure $next); } flare-client-php/src/FlareMiddleware/CensorRequestHeaders.php 0000644 00000001264 15002154456 0020330 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use Spatie\FlareClient\Report; class CensorRequestHeaders implements FlareMiddleware { protected array $headers = []; public function __construct(array $headers) { $this->headers = $headers; } public function handle(Report $report, $next) { $context = $report->allContext(); foreach ($this->headers as $header) { $header = strtolower($header); if (isset($context['headers'][$header])) { $context['headers'][$header] = '<CENSORED>'; } } $report->userProvidedContext($context); return $next($report); } } flare-client-php/src/FlareMiddleware/AddDocumentationLinks.php 0000644 00000002503 15002154456 0020452 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; use ArrayObject; use Closure; use Spatie\FlareClient\Report; class AddDocumentationLinks implements FlareMiddleware { protected ArrayObject $documentationLinkResolvers; public function __construct(ArrayObject $documentationLinkResolvers) { $this->documentationLinkResolvers = $documentationLinkResolvers; } public function handle(Report $report, Closure $next) { if (! $throwable = $report->getThrowable()) { return $next($report); } $links = $this->getLinks($throwable); if (count($links)) { $report->addDocumentationLinks($links); } return $next($report); } /** @return array<int, string> */ protected function getLinks(\Throwable $throwable): array { $allLinks = []; foreach ($this->documentationLinkResolvers as $resolver) { $resolvedLinks = $resolver($throwable); if (is_null($resolvedLinks)) { continue; } if (is_string($resolvedLinks)) { $resolvedLinks = [$resolvedLinks]; } foreach ($resolvedLinks as $link) { $allLinks[] = $link; } } return array_values(array_unique($allLinks)); } } flare-client-php/src/FlareMiddleware/AddGlows.php 0000644 00000001107 15002154456 0015732 0 ustar 00 <?php namespace Spatie\FlareClient\FlareMiddleware; namespace Spatie\FlareClient\FlareMiddleware; use Closure; use Spatie\FlareClient\Glows\GlowRecorder; use Spatie\FlareClient\Report; class AddGlows implements FlareMiddleware { protected GlowRecorder $recorder; public function __construct(GlowRecorder $recorder) { $this->recorder = $recorder; } public function handle(Report $report, Closure $next) { foreach ($this->recorder->glows() as $glow) { $report->addGlow($glow); } return $next($report); } } flare-client-php/src/Frame.php 0000644 00000001361 15002154456 0012233 0 ustar 00 <?php namespace Spatie\FlareClient; use Spatie\Backtrace\Frame as SpatieFrame; class Frame { public static function fromSpatieFrame( SpatieFrame $frame, ): self { return new self($frame); } public function __construct( protected SpatieFrame $frame, ) { } public function toArray(): array { return [ 'file' => $this->frame->file, 'line_number' => $this->frame->lineNumber, 'method' => $this->frame->method, 'class' => $this->frame->class, 'code_snippet' => $this->frame->getSnippet(30), 'arguments' => $this->frame->arguments, 'application_frame' => $this->frame->applicationFrame, ]; } } flare-client-php/src/View.php 0000644 00000002642 15002154456 0012116 0 ustar 00 <?php namespace Spatie\FlareClient; use Symfony\Component\VarDumper\Cloner\VarCloner; use Symfony\Component\VarDumper\Dumper\HtmlDumper; class View { protected string $file; /** @var array<string, mixed> */ protected array $data = []; /** * @param string $file * @param array<string, mixed> $data */ public function __construct(string $file, array $data = []) { $this->file = $file; $this->data = $data; } /** * @param string $file * @param array<string, mixed> $data * * @return self */ public static function create(string $file, array $data = []): self { return new self($file, $data); } protected function dumpViewData(mixed $variable): string { $cloner = new VarCloner(); $dumper = new HtmlDumper(); $dumper->setDumpHeader(''); $output = fopen('php://memory', 'r+b'); if (! $output) { return ''; } $dumper->dump($cloner->cloneVar($variable)->withMaxDepth(1), $output, [ 'maxDepth' => 1, 'maxStringLength' => 160, ]); return (string)stream_get_contents($output, -1, 0); } /** @return array<string, mixed> */ public function toArray(): array { return [ 'file' => $this->file, 'data' => array_map([$this, 'dumpViewData'], $this->data), ]; } } flare-client-php/src/Glows/GlowRecorder.php 0000644 00000001026 15002154456 0014670 0 ustar 00 <?php namespace Spatie\FlareClient\Glows; class GlowRecorder { const GLOW_LIMIT = 30; /** @var array<int, Glow> */ protected array $glows = []; public function record(Glow $glow): void { $this->glows[] = $glow; $this->glows = array_slice($this->glows, static::GLOW_LIMIT * -1, static::GLOW_LIMIT); } /** @return array<int, Glow> */ public function glows(): array { return $this->glows; } public function reset(): void { $this->glows = []; } } flare-client-php/src/Glows/Glow.php 0000644 00000002363 15002154456 0013207 0 ustar 00 <?php namespace Spatie\FlareClient\Glows; use Spatie\FlareClient\Concerns\UsesTime; use Spatie\FlareClient\Enums\MessageLevels; class Glow { use UsesTime; protected string $name; /** @var array<int, mixed> */ protected array $metaData = []; protected string $messageLevel; protected float $microtime; /** * @param string $name * @param string $messageLevel * @param array<int, mixed> $metaData * @param float|null $microtime */ public function __construct( string $name, string $messageLevel = MessageLevels::INFO, array $metaData = [], ?float $microtime = null ) { $this->name = $name; $this->messageLevel = $messageLevel; $this->metaData = $metaData; $this->microtime = $microtime ?? microtime(true); } /** * @return array{time: int, name: string, message_level: string, meta_data: array, microtime: float} */ public function toArray(): array { return [ 'time' => $this->getCurrentTime(), 'name' => $this->name, 'message_level' => $this->messageLevel, 'meta_data' => $this->metaData, 'microtime' => $this->microtime, ]; } } flare-client-php/src/Solutions/ReportSolution.php 0000644 00000002220 15002154456 0016203 0 ustar 00 <?php namespace Spatie\FlareClient\Solutions; use Spatie\Ignition\Contracts\RunnableSolution; use Spatie\Ignition\Contracts\Solution as SolutionContract; class ReportSolution { protected SolutionContract $solution; public function __construct(SolutionContract $solution) { $this->solution = $solution; } public static function fromSolution(SolutionContract $solution): self { return new self($solution); } /** * @return array<string, mixed> */ public function toArray(): array { $isRunnable = ($this->solution instanceof RunnableSolution); return [ 'class' => get_class($this->solution), 'title' => $this->solution->getSolutionTitle(), 'description' => $this->solution->getSolutionDescription(), 'links' => $this->solution->getDocumentationLinks(), /** @phpstan-ignore-next-line */ 'action_description' => $isRunnable ? $this->solution->getSolutionActionDescription() : null, 'is_runnable' => $isRunnable, 'ai_generated' => $this->solution->aiGenerated ?? false, ]; } } flare-client-php/src/helpers.php 0000644 00000001246 15002154456 0012645 0 ustar 00 <?php if (! function_exists('array_merge_recursive_distinct')) { /** * @param array<int|string, mixed> $array1 * @param array<int|string, mixed> $array2 * * @return array<int|string, mixed> */ function array_merge_recursive_distinct(array &$array1, array &$array2): array { $merged = $array1; foreach ($array2 as $key => &$value) { if (is_array($value) && isset($merged[$key]) && is_array($merged[$key])) { $merged[$key] = array_merge_recursive_distinct($merged[$key], $value); } else { $merged[$key] = $value; } } return $merged; } } flare-client-php/src/Flare.php 0000644 00000027714 15002154456 0012244 0 ustar 00 <?php namespace Spatie\FlareClient; use Error; use ErrorException; use Exception; use Illuminate\Contracts\Container\Container; use Illuminate\Pipeline\Pipeline; use Spatie\Backtrace\Arguments\ArgumentReducers; use Spatie\Backtrace\Arguments\Reducers\ArgumentReducer; use Spatie\FlareClient\Concerns\HasContext; use Spatie\FlareClient\Context\BaseContextProviderDetector; use Spatie\FlareClient\Context\ContextProviderDetector; use Spatie\FlareClient\Enums\MessageLevels; use Spatie\FlareClient\FlareMiddleware\AddEnvironmentInformation; use Spatie\FlareClient\FlareMiddleware\AddGlows; use Spatie\FlareClient\FlareMiddleware\CensorRequestBodyFields; use Spatie\FlareClient\FlareMiddleware\FlareMiddleware; use Spatie\FlareClient\FlareMiddleware\RemoveRequestIp; use Spatie\FlareClient\Glows\Glow; use Spatie\FlareClient\Glows\GlowRecorder; use Spatie\FlareClient\Http\Client; use Throwable; class Flare { use HasContext; protected Client $client; protected Api $api; /** @var array<int, FlareMiddleware|class-string<FlareMiddleware>> */ protected array $middleware = []; protected GlowRecorder $recorder; protected ?string $applicationPath = null; protected ContextProviderDetector $contextDetector; protected $previousExceptionHandler = null; /** @var null|callable */ protected $previousErrorHandler = null; /** @var null|callable */ protected $determineVersionCallable = null; protected ?int $reportErrorLevels = null; /** @var null|callable */ protected $filterExceptionsCallable = null; /** @var null|callable */ protected $filterReportsCallable = null; protected ?string $stage = null; protected ?string $requestId = null; protected ?Container $container = null; /** @var array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null */ protected null|array|ArgumentReducers $argumentReducers = null; protected bool $withStackFrameArguments = true; public static function make( string $apiKey = null, ContextProviderDetector $contextDetector = null ): self { $client = new Client($apiKey); return new self($client, $contextDetector); } public function setApiToken(string $apiToken): self { $this->client->setApiToken($apiToken); return $this; } public function apiTokenSet(): bool { return $this->client->apiTokenSet(); } public function setBaseUrl(string $baseUrl): self { $this->client->setBaseUrl($baseUrl); return $this; } public function setStage(?string $stage): self { $this->stage = $stage; return $this; } public function sendReportsImmediately(): self { $this->api->sendReportsImmediately(); return $this; } public function determineVersionUsing(callable $determineVersionCallable): self { $this->determineVersionCallable = $determineVersionCallable; return $this; } public function reportErrorLevels(int $reportErrorLevels): self { $this->reportErrorLevels = $reportErrorLevels; return $this; } public function filterExceptionsUsing(callable $filterExceptionsCallable): self { $this->filterExceptionsCallable = $filterExceptionsCallable; return $this; } public function filterReportsUsing(callable $filterReportsCallable): self { $this->filterReportsCallable = $filterReportsCallable; return $this; } /** @param array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null $argumentReducers */ public function argumentReducers(null|array|ArgumentReducers $argumentReducers): self { $this->argumentReducers = $argumentReducers; return $this; } public function withStackFrameArguments(bool $withStackFrameArguments = true): self { $this->withStackFrameArguments = $withStackFrameArguments; return $this; } public function version(): ?string { if (! $this->determineVersionCallable) { return null; } return ($this->determineVersionCallable)(); } /** * @param \Spatie\FlareClient\Http\Client $client * @param \Spatie\FlareClient\Context\ContextProviderDetector|null $contextDetector * @param array<int, FlareMiddleware> $middleware */ public function __construct( Client $client, ContextProviderDetector $contextDetector = null, array $middleware = [], ) { $this->client = $client; $this->recorder = new GlowRecorder(); $this->contextDetector = $contextDetector ?? new BaseContextProviderDetector(); $this->middleware = $middleware; $this->api = new Api($this->client); $this->registerDefaultMiddleware(); } /** @return array<int, FlareMiddleware|class-string<FlareMiddleware>> */ public function getMiddleware(): array { return $this->middleware; } public function setContextProviderDetector(ContextProviderDetector $contextDetector): self { $this->contextDetector = $contextDetector; return $this; } public function setContainer(Container $container): self { $this->container = $container; return $this; } public function registerFlareHandlers(): self { $this->registerExceptionHandler(); $this->registerErrorHandler(); return $this; } public function registerExceptionHandler(): self { /** @phpstan-ignore-next-line */ $this->previousExceptionHandler = set_exception_handler([$this, 'handleException']); return $this; } public function registerErrorHandler(): self { $this->previousErrorHandler = set_error_handler([$this, 'handleError']); return $this; } protected function registerDefaultMiddleware(): self { return $this->registerMiddleware([ new AddGlows($this->recorder), new AddEnvironmentInformation(), ]); } /** * @param FlareMiddleware|array<FlareMiddleware>|class-string<FlareMiddleware>|callable $middleware * * @return $this */ public function registerMiddleware($middleware): self { if (! is_array($middleware)) { $middleware = [$middleware]; } $this->middleware = array_merge($this->middleware, $middleware); return $this; } /** * @return array<int,FlareMiddleware|class-string<FlareMiddleware>> */ public function getMiddlewares(): array { return $this->middleware; } /** * @param string $name * @param string $messageLevel * @param array<int, mixed> $metaData * * @return $this */ public function glow( string $name, string $messageLevel = MessageLevels::INFO, array $metaData = [] ): self { $this->recorder->record(new Glow($name, $messageLevel, $metaData)); return $this; } public function handleException(Throwable $throwable): void { $this->report($throwable); if ($this->previousExceptionHandler && is_callable($this->previousExceptionHandler)) { call_user_func($this->previousExceptionHandler, $throwable); } } /** * @return mixed */ public function handleError(mixed $code, string $message, string $file = '', int $line = 0) { $exception = new ErrorException($message, 0, $code, $file, $line); $this->report($exception); if ($this->previousErrorHandler) { return call_user_func( $this->previousErrorHandler, $message, $code, $file, $line ); } } public function applicationPath(string $applicationPath): self { $this->applicationPath = $applicationPath; return $this; } public function report(Throwable $throwable, callable $callback = null, Report $report = null): ?Report { if (! $this->shouldSendReport($throwable)) { return null; } $report ??= $this->createReport($throwable); if (! is_null($callback)) { call_user_func($callback, $report); } $this->recorder->reset(); $this->sendReportToApi($report); return $report; } protected function shouldSendReport(Throwable $throwable): bool { if (isset($this->reportErrorLevels) && $throwable instanceof Error) { return (bool) ($this->reportErrorLevels & $throwable->getCode()); } if (isset($this->reportErrorLevels) && $throwable instanceof ErrorException) { return (bool) ($this->reportErrorLevels & $throwable->getSeverity()); } if ($this->filterExceptionsCallable && $throwable instanceof Exception) { return (bool) (call_user_func($this->filterExceptionsCallable, $throwable)); } return true; } public function reportMessage(string $message, string $logLevel, callable $callback = null): void { $report = $this->createReportFromMessage($message, $logLevel); if (! is_null($callback)) { call_user_func($callback, $report); } $this->sendReportToApi($report); } public function sendTestReport(Throwable $throwable): void { $this->api->sendTestReport($this->createReport($throwable)); } protected function sendReportToApi(Report $report): void { if ($this->filterReportsCallable) { if (! call_user_func($this->filterReportsCallable, $report)) { return; } } try { $this->api->report($report); } catch (Exception $exception) { } } public function reset(): void { $this->api->sendQueuedReports(); $this->userProvidedContext = []; $this->recorder->reset(); } protected function applyAdditionalParameters(Report $report): void { $report ->stage($this->stage) ->messageLevel($this->messageLevel) ->setApplicationPath($this->applicationPath) ->userProvidedContext($this->userProvidedContext); } public function anonymizeIp(): self { $this->registerMiddleware(new RemoveRequestIp()); return $this; } /** * @param array<int, string> $fieldNames * * @return $this */ public function censorRequestBodyFields(array $fieldNames): self { $this->registerMiddleware(new CensorRequestBodyFields($fieldNames)); return $this; } public function createReport(Throwable $throwable): Report { $report = Report::createForThrowable( $throwable, $this->contextDetector->detectCurrentContext(), $this->applicationPath, $this->version(), $this->argumentReducers, $this->withStackFrameArguments ); return $this->applyMiddlewareToReport($report); } public function createReportFromMessage(string $message, string $logLevel): Report { $report = Report::createForMessage( $message, $logLevel, $this->contextDetector->detectCurrentContext(), $this->applicationPath, $this->argumentReducers, $this->withStackFrameArguments ); return $this->applyMiddlewareToReport($report); } protected function applyMiddlewareToReport(Report $report): Report { $this->applyAdditionalParameters($report); $middleware = array_map(function ($singleMiddleware) { return is_string($singleMiddleware) ? new $singleMiddleware : $singleMiddleware; }, $this->middleware); $report = (new Pipeline()) ->send($report) ->through($middleware) ->then(fn ($report) => $report); return $report; } } flare-client-php/src/Report.php 0000644 00000025375 15002154456 0012467 0 ustar 00 <?php namespace Spatie\FlareClient; use ErrorException; use Spatie\Backtrace\Arguments\ArgumentReducers; use Spatie\Backtrace\Arguments\Reducers\ArgumentReducer; use Spatie\Backtrace\Backtrace; use Spatie\Backtrace\Frame as SpatieFrame; use Spatie\FlareClient\Concerns\HasContext; use Spatie\FlareClient\Concerns\UsesTime; use Spatie\FlareClient\Context\ContextProvider; use Spatie\FlareClient\Contracts\ProvidesFlareContext; use Spatie\FlareClient\Glows\Glow; use Spatie\FlareClient\Solutions\ReportSolution; use Spatie\Ignition\Contracts\Solution; use Spatie\LaravelIgnition\Exceptions\ViewException; use Throwable; class Report { use UsesTime; use HasContext; protected Backtrace $stacktrace; protected string $exceptionClass = ''; protected string $message = ''; /** @var array<int, array{time: int, name: string, message_level: string, meta_data: array, microtime: float}> */ protected array $glows = []; /** @var array<int, array<int|string, mixed>> */ protected array $solutions = []; /** @var array<int, string> */ public array $documentationLinks = []; protected ContextProvider $context; protected ?string $applicationPath = null; protected ?string $applicationVersion = null; /** @var array<int|string, mixed> */ protected array $userProvidedContext = []; /** @var array<int|string, mixed> */ protected array $exceptionContext = []; protected ?Throwable $throwable = null; protected string $notifierName = 'Flare Client'; protected ?string $languageVersion = null; protected ?string $frameworkVersion = null; protected ?int $openFrameIndex = null; protected string $trackingUuid; protected ?View $view; public static ?string $fakeTrackingUuid = null; /** @param array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null $argumentReducers */ public static function createForThrowable( Throwable $throwable, ContextProvider $context, ?string $applicationPath = null, ?string $version = null, null|array|ArgumentReducers $argumentReducers = null, bool $withStackTraceArguments = true, ): self { $stacktrace = Backtrace::createForThrowable($throwable) ->withArguments($withStackTraceArguments) ->reduceArguments($argumentReducers) ->applicationPath($applicationPath ?? ''); return (new self()) ->setApplicationPath($applicationPath) ->throwable($throwable) ->useContext($context) ->exceptionClass(self::getClassForThrowable($throwable)) ->message($throwable->getMessage()) ->stackTrace($stacktrace) ->exceptionContext($throwable) ->setApplicationVersion($version); } protected static function getClassForThrowable(Throwable $throwable): string { /** @phpstan-ignore-next-line */ if ($throwable::class === ViewException::class) { /** @phpstan-ignore-next-line */ if ($previous = $throwable->getPrevious()) { return get_class($previous); } } return get_class($throwable); } /** @param array<class-string<ArgumentReducer>|ArgumentReducer>|ArgumentReducers|null $argumentReducers */ public static function createForMessage( string $message, string $logLevel, ContextProvider $context, ?string $applicationPath = null, null|array|ArgumentReducers $argumentReducers = null, bool $withStackTraceArguments = true, ): self { $stacktrace = Backtrace::create() ->withArguments($withStackTraceArguments) ->reduceArguments($argumentReducers) ->applicationPath($applicationPath ?? ''); return (new self()) ->setApplicationPath($applicationPath) ->message($message) ->useContext($context) ->exceptionClass($logLevel) ->stacktrace($stacktrace) ->openFrameIndex($stacktrace->firstApplicationFrameIndex()); } public function __construct() { $this->trackingUuid = self::$fakeTrackingUuid ?? $this->generateUuid(); } public function trackingUuid(): string { return $this->trackingUuid; } public function exceptionClass(string $exceptionClass): self { $this->exceptionClass = $exceptionClass; return $this; } public function getExceptionClass(): string { return $this->exceptionClass; } public function throwable(Throwable $throwable): self { $this->throwable = $throwable; return $this; } public function getThrowable(): ?Throwable { return $this->throwable; } public function message(string $message): self { $this->message = $message; return $this; } public function getMessage(): string { return $this->message; } public function stacktrace(Backtrace $stacktrace): self { $this->stacktrace = $stacktrace; return $this; } public function getStacktrace(): Backtrace { return $this->stacktrace; } public function notifierName(string $notifierName): self { $this->notifierName = $notifierName; return $this; } public function languageVersion(string $languageVersion): self { $this->languageVersion = $languageVersion; return $this; } public function frameworkVersion(string $frameworkVersion): self { $this->frameworkVersion = $frameworkVersion; return $this; } public function useContext(ContextProvider $request): self { $this->context = $request; return $this; } public function openFrameIndex(?int $index): self { $this->openFrameIndex = $index; return $this; } public function setApplicationPath(?string $applicationPath): self { $this->applicationPath = $applicationPath; return $this; } public function getApplicationPath(): ?string { return $this->applicationPath; } public function setApplicationVersion(?string $applicationVersion): self { $this->applicationVersion = $applicationVersion; return $this; } public function getApplicationVersion(): ?string { return $this->applicationVersion; } public function view(?View $view): self { $this->view = $view; return $this; } public function addGlow(Glow $glow): self { $this->glows[] = $glow->toArray(); return $this; } public function addSolution(Solution $solution): self { $this->solutions[] = ReportSolution::fromSolution($solution)->toArray(); return $this; } /** * @param array<int, string> $documentationLinks * * @return $this */ public function addDocumentationLinks(array $documentationLinks): self { $this->documentationLinks = $documentationLinks; return $this; } /** * @param array<int|string, mixed> $userProvidedContext * * @return $this */ public function userProvidedContext(array $userProvidedContext): self { $this->userProvidedContext = $userProvidedContext; return $this; } /** * @return array<int|string, mixed> */ public function allContext(): array { $context = $this->context->toArray(); $context = array_merge_recursive_distinct($context, $this->exceptionContext); return array_merge_recursive_distinct($context, $this->userProvidedContext); } protected function exceptionContext(Throwable $throwable): self { if ($throwable instanceof ProvidesFlareContext) { $this->exceptionContext = $throwable->context(); } return $this; } /** * @return array<int|string, mixed> */ protected function stracktraceAsArray(): array { return array_map( fn (SpatieFrame $frame) => Frame::fromSpatieFrame($frame)->toArray(), $this->cleanupStackTraceForError($this->stacktrace->frames()), ); } /** * @param array<SpatieFrame> $frames * * @return array<SpatieFrame> */ protected function cleanupStackTraceForError(array $frames): array { if ($this->throwable === null || get_class($this->throwable) !== ErrorException::class) { return $frames; } $firstErrorFrameIndex = null; $restructuredFrames = array_values(array_slice($frames, 1)); // remove the first frame where error was created foreach ($restructuredFrames as $index => $frame) { if ($frame->file === $this->throwable->getFile()) { $firstErrorFrameIndex = $index; break; } } if ($firstErrorFrameIndex === null) { return $frames; } $restructuredFrames[$firstErrorFrameIndex]->arguments = null; // Remove error arguments return array_values(array_slice($restructuredFrames, $firstErrorFrameIndex)); } /** * @return array<string, mixed> */ public function toArray(): array { return [ 'notifier' => $this->notifierName ?? 'Flare Client', 'language' => 'PHP', 'framework_version' => $this->frameworkVersion, 'language_version' => $this->languageVersion ?? phpversion(), 'exception_class' => $this->exceptionClass, 'seen_at' => $this->getCurrentTime(), 'message' => $this->message, 'glows' => $this->glows, 'solutions' => $this->solutions, 'documentation_links' => $this->documentationLinks, 'stacktrace' => $this->stracktraceAsArray(), 'context' => $this->allContext(), 'stage' => $this->stage, 'message_level' => $this->messageLevel, 'open_frame_index' => $this->openFrameIndex, 'application_path' => $this->applicationPath, 'application_version' => $this->applicationVersion, 'tracking_uuid' => $this->trackingUuid, ]; } /* * Found on https://stackoverflow.com/questions/2040240/php-function-to-generate-v4-uuid/15875555#15875555 */ protected function generateUuid(): string { // Generate 16 bytes (128 bits) of random data or use the data passed into the function. $data = random_bytes(16); // Set version to 0100 $data[6] = chr(ord($data[6]) & 0x0f | 0x40); // Set bits 6-7 to 10 $data[8] = chr(ord($data[8]) & 0x3f | 0x80); // Output the 36 character UUID. return vsprintf('%s%s-%s-%s-%s-%s%s%s', str_split(bin2hex($data), 4)); } } flare-client-php/src/Contracts/ProvidesFlareContext.php 0000644 00000000261 15002154456 0017251 0 ustar 00 <?php namespace Spatie\FlareClient\Contracts; interface ProvidesFlareContext { /** * @return array<int|string, mixed> */ public function context(): array; } flare-client-php/src/Time/SystemTime.php 0000644 00000000330 15002154456 0014175 0 ustar 00 <?php namespace Spatie\FlareClient\Time; use DateTimeImmutable; class SystemTime implements Time { public function getCurrentTime(): int { return (new DateTimeImmutable())->getTimestamp(); } } flare-client-php/src/Time/Time.php 0000644 00000000151 15002154456 0012771 0 ustar 00 <?php namespace Spatie\FlareClient\Time; interface Time { public function getCurrentTime(): int; } flare-client-php/src/Context/ConsoleContextProvider.php 0000644 00000001034 15002154456 0017304 0 ustar 00 <?php namespace Spatie\FlareClient\Context; class ConsoleContextProvider implements ContextProvider { /** * @var array<string, mixed> */ protected array $arguments = []; /** * @param array<string, mixed> $arguments */ public function __construct(array $arguments = []) { $this->arguments = $arguments; } /** * @return array<int|string, mixed> */ public function toArray(): array { return [ 'arguments' => $this->arguments, ]; } } flare-client-php/src/Context/BaseContextProviderDetector.php 0000644 00000001273 15002154456 0020253 0 ustar 00 <?php namespace Spatie\FlareClient\Context; class BaseContextProviderDetector implements ContextProviderDetector { public function detectCurrentContext(): ContextProvider { if ($this->runningInConsole()) { return new ConsoleContextProvider($_SERVER['argv'] ?? []); } return new RequestContextProvider(); } protected function runningInConsole(): bool { if (isset($_ENV['APP_RUNNING_IN_CONSOLE'])) { return $_ENV['APP_RUNNING_IN_CONSOLE'] === 'true'; } if (isset($_ENV['FLARE_FAKE_WEB_REQUEST'])) { return false; } return in_array(php_sapi_name(), ['cli', 'phpdb']); } } flare-client-php/src/Context/RequestContextProvider.php 0000644 00000007324 15002154456 0017342 0 ustar 00 <?php namespace Spatie\FlareClient\Context; use RuntimeException; use Symfony\Component\HttpFoundation\File\UploadedFile; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\Mime\Exception\InvalidArgumentException; use Throwable; class RequestContextProvider implements ContextProvider { protected ?Request $request; public function __construct(Request $request = null) { $this->request = $request ?? Request::createFromGlobals(); } /** * @return array<string, mixed> */ public function getRequest(): array { return [ 'url' => $this->request->getUri(), 'ip' => $this->request->getClientIp(), 'method' => $this->request->getMethod(), 'useragent' => $this->request->headers->get('User-Agent'), ]; } /** * @return array<int, mixed> */ protected function getFiles(): array { if (is_null($this->request->files)) { return []; } return $this->mapFiles($this->request->files->all()); } /** * @param array<int, mixed> $files * * @return array<string, string> */ protected function mapFiles(array $files): array { return array_map(function ($file) { if (is_array($file)) { return $this->mapFiles($file); } if (! $file instanceof UploadedFile) { return; } try { $fileSize = $file->getSize(); } catch (RuntimeException $e) { $fileSize = 0; } try { $mimeType = $file->getMimeType(); } catch (InvalidArgumentException $e) { $mimeType = 'undefined'; } return [ 'pathname' => $file->getPathname(), 'size' => $fileSize, 'mimeType' => $mimeType, ]; }, $files); } /** * @return array<string, mixed> */ public function getSession(): array { try { $session = $this->request->getSession(); } catch (Throwable $exception) { $session = []; } return $session ? $this->getValidSessionData($session) : []; } protected function getValidSessionData($session): array { if (! method_exists($session, 'all')) { return []; } try { json_encode($session->all()); } catch (Throwable $e) { return []; } return $session->all(); } /** * @return array<int|string, mixed */ public function getCookies(): array { return $this->request->cookies->all(); } /** * @return array<string, mixed> */ public function getHeaders(): array { /** @var array<string, list<string|null>> $headers */ $headers = $this->request->headers->all(); return array_filter( array_map( fn (array $header) => $header[0], $headers ) ); } /** * @return array<string, mixed> */ public function getRequestData(): array { return [ 'queryString' => $this->request->query->all(), 'body' => $this->request->request->all(), 'files' => $this->getFiles(), ]; } /** @return array<string, mixed> */ public function toArray(): array { return [ 'request' => $this->getRequest(), 'request_data' => $this->getRequestData(), 'headers' => $this->getHeaders(), 'cookies' => $this->getCookies(), 'session' => $this->getSession(), ]; } } flare-client-php/src/Context/ContextProviderDetector.php 0000644 00000000221 15002154456 0017450 0 ustar 00 <?php namespace Spatie\FlareClient\Context; interface ContextProviderDetector { public function detectCurrentContext(): ContextProvider; } flare-client-php/src/Context/ContextProvider.php 0000644 00000000252 15002154456 0015762 0 ustar 00 <?php namespace Spatie\FlareClient\Context; interface ContextProvider { /** * @return array<int, string|mixed> */ public function toArray(): array; } flare-client-php/src/Api.php 0000644 00000003506 15002154456 0011715 0 ustar 00 <?php namespace Spatie\FlareClient; use Exception; use Spatie\FlareClient\Http\Client; use Spatie\FlareClient\Truncation\ReportTrimmer; class Api { protected Client $client; protected bool $sendReportsImmediately = false; /** @var array<int, Report> */ protected array $queue = []; public function __construct(Client $client) { $this->client = $client; register_shutdown_function([$this, 'sendQueuedReports']); } public function sendReportsImmediately(): self { $this->sendReportsImmediately = true; return $this; } public function report(Report $report): void { try { $this->sendReportsImmediately ? $this->sendReportToApi($report) : $this->addReportToQueue($report); } catch (Exception $e) { // } } public function sendTestReport(Report $report): self { $this->sendReportToApi($report); return $this; } protected function addReportToQueue(Report $report): self { $this->queue[] = $report; return $this; } public function sendQueuedReports(): void { try { foreach ($this->queue as $report) { $this->sendReportToApi($report); } } catch (Exception $e) { // } finally { $this->queue = []; } } protected function sendReportToApi(Report $report): void { $payload = $this->truncateReport($report->toArray()); $this->client->post('reports', $payload); } /** * @param array<int|string, mixed> $payload * * @return array<int|string, mixed> */ protected function truncateReport(array $payload): array { return (new ReportTrimmer())->trim($payload); } } flare-client-php/src/Enums/MessageLevels.php 0000644 00000000323 15002154456 0015024 0 ustar 00 <?php namespace Spatie\FlareClient\Enums; class MessageLevels { const INFO = 'info'; const DEBUG = 'debug'; const WARNING = 'warning'; const ERROR = 'error'; const CRITICAL = 'critical'; } flare-client-php/src/Concerns/HasContext.php 0000644 00000002501 15002154456 0015030 0 ustar 00 <?php namespace Spatie\FlareClient\Concerns; trait HasContext { protected ?string $messageLevel = null; protected ?string $stage = null; /** * @var array<string, mixed> */ protected array $userProvidedContext = []; public function stage(?string $stage): self { $this->stage = $stage; return $this; } public function messageLevel(?string $messageLevel): self { $this->messageLevel = $messageLevel; return $this; } /** * @param string $groupName * @param mixed $default * * @return array<int, mixed> */ public function getGroup(string $groupName = 'context', $default = []): array { return $this->userProvidedContext[$groupName] ?? $default; } public function context(string $key, mixed $value): self { return $this->group('context', [$key => $value]); } /** * @param string $groupName * @param array<string, mixed> $properties * * @return $this */ public function group(string $groupName, array $properties): self { $group = $this->userProvidedContext[$groupName] ?? []; $this->userProvidedContext[$groupName] = array_merge_recursive_distinct( $group, $properties ); return $this; } } flare-client-php/src/Concerns/UsesTime.php 0000644 00000000633 15002154456 0014512 0 ustar 00 <?php namespace Spatie\FlareClient\Concerns; use Spatie\FlareClient\Time\SystemTime; use Spatie\FlareClient\Time\Time; trait UsesTime { public static Time $time; public static function useTime(Time $time): void { self::$time = $time; } public function getCurrentTime(): int { $time = self::$time ?? new SystemTime(); return $time->getCurrentTime(); } } flare-client-php/src/Http/Exceptions/BadResponse.php 0000644 00000000653 15002154456 0016451 0 ustar 00 <?php namespace Spatie\FlareClient\Http\Exceptions; use Exception; use Spatie\FlareClient\Http\Response; class BadResponse extends Exception { public Response $response; public static function createForResponse(Response $response): self { $exception = new self("Could not perform request because: {$response->getError()}"); $exception->response = $response; return $exception; } } flare-client-php/src/Http/Exceptions/InvalidData.php 0000644 00000000411 15002154456 0016414 0 ustar 00 <?php namespace Spatie\FlareClient\Http\Exceptions; use Spatie\FlareClient\Http\Response; class InvalidData extends BadResponseCode { public static function getMessageForResponse(Response $response): string { return 'Invalid data found'; } } flare-client-php/src/Http/Exceptions/NotFound.php 0000644 00000000375 15002154456 0016001 0 ustar 00 <?php namespace Spatie\FlareClient\Http\Exceptions; use Spatie\FlareClient\Http\Response; class NotFound extends BadResponseCode { public static function getMessageForResponse(Response $response): string { return 'Not found'; } } flare-client-php/src/Http/Exceptions/MissingParameter.php 0000644 00000000403 15002154456 0017507 0 ustar 00 <?php namespace Spatie\FlareClient\Http\Exceptions; use Exception; class MissingParameter extends Exception { public static function create(string $parameterName): self { return new self("`$parameterName` is a required parameter"); } } flare-client-php/src/Http/Exceptions/BadResponseCode.php 0000644 00000001436 15002154456 0017244 0 ustar 00 <?php namespace Spatie\FlareClient\Http\Exceptions; use Exception; use Spatie\FlareClient\Http\Response; class BadResponseCode extends Exception { public Response $response; /** * @var array<int, mixed> */ public array $errors = []; public static function createForResponse(Response $response): self { $exception = new self(static::getMessageForResponse($response)); $exception->response = $response; $bodyErrors = isset($response->getBody()['errors']) ? $response->getBody()['errors'] : []; $exception->errors = $bodyErrors; return $exception; } public static function getMessageForResponse(Response $response): string { return "Response code {$response->getHttpResponseCode()} returned"; } } flare-client-php/src/Http/Response.php 0000644 00000001535 15002154456 0013721 0 ustar 00 <?php namespace Spatie\FlareClient\Http; class Response { protected mixed $headers; protected mixed $body; protected mixed $error; public function __construct(mixed $headers, mixed $body, mixed $error) { $this->headers = $headers; $this->body = $body; $this->error = $error; } public function getHeaders(): mixed { return $this->headers; } public function getBody(): mixed { return $this->body; } public function hasBody(): bool { return $this->body != false; } public function getError(): mixed { return $this->error; } public function getHttpResponseCode(): ?int { if (! isset($this->headers['http_code'])) { return null; } return (int) $this->headers['http_code']; } } flare-client-php/src/Http/Client.php 0000644 00000013665 15002154456 0013350 0 ustar 00 <?php namespace Spatie\FlareClient\Http; use Spatie\FlareClient\Http\Exceptions\BadResponseCode; use Spatie\FlareClient\Http\Exceptions\InvalidData; use Spatie\FlareClient\Http\Exceptions\MissingParameter; use Spatie\FlareClient\Http\Exceptions\NotFound; class Client { protected ?string $apiToken; protected ?string $baseUrl; protected int $timeout; protected $lastRequest = null; public function __construct( ?string $apiToken = null, string $baseUrl = 'https://reporting.flareapp.io/api', int $timeout = 10 ) { $this->apiToken = $apiToken; if (! $baseUrl) { throw MissingParameter::create('baseUrl'); } $this->baseUrl = $baseUrl; if (! $timeout) { throw MissingParameter::create('timeout'); } $this->timeout = $timeout; } public function setApiToken(string $apiToken): self { $this->apiToken = $apiToken; return $this; } public function apiTokenSet(): bool { return ! empty($this->apiToken); } public function setBaseUrl(string $baseUrl): self { $this->baseUrl = $baseUrl; return $this; } /** * @param string $url * @param array $arguments * * @return array|false */ public function get(string $url, array $arguments = []) { return $this->makeRequest('get', $url, $arguments); } /** * @param string $url * @param array $arguments * * @return array|false */ public function post(string $url, array $arguments = []) { return $this->makeRequest('post', $url, $arguments); } /** * @param string $url * @param array $arguments * * @return array|false */ public function patch(string $url, array $arguments = []) { return $this->makeRequest('patch', $url, $arguments); } /** * @param string $url * @param array $arguments * * @return array|false */ public function put(string $url, array $arguments = []) { return $this->makeRequest('put', $url, $arguments); } /** * @param string $method * @param array $arguments * * @return array|false */ public function delete(string $method, array $arguments = []) { return $this->makeRequest('delete', $method, $arguments); } /** * @param string $httpVerb * @param string $url * @param array $arguments * * @return array */ protected function makeRequest(string $httpVerb, string $url, array $arguments = []) { $queryString = http_build_query([ 'key' => $this->apiToken, ]); $fullUrl = "{$this->baseUrl}/{$url}?{$queryString}"; $headers = [ 'x-api-token: '.$this->apiToken, ]; $response = $this->makeCurlRequest($httpVerb, $fullUrl, $headers, $arguments); if ($response->getHttpResponseCode() === 422) { throw InvalidData::createForResponse($response); } if ($response->getHttpResponseCode() === 404) { throw NotFound::createForResponse($response); } if ($response->getHttpResponseCode() !== 200 && $response->getHttpResponseCode() !== 204) { throw BadResponseCode::createForResponse($response); } return $response->getBody(); } public function makeCurlRequest(string $httpVerb, string $fullUrl, array $headers = [], array $arguments = []): Response { $curlHandle = $this->getCurlHandle($fullUrl, $headers); switch ($httpVerb) { case 'post': curl_setopt($curlHandle, CURLOPT_POST, true); $this->attachRequestPayload($curlHandle, $arguments); break; case 'get': curl_setopt($curlHandle, CURLOPT_URL, $fullUrl.'&'.http_build_query($arguments)); break; case 'delete': curl_setopt($curlHandle, CURLOPT_CUSTOMREQUEST, 'DELETE'); break; case 'patch': curl_setopt($curlHandle, CURLOPT_CUSTOMREQUEST, 'PATCH'); $this->attachRequestPayload($curlHandle, $arguments); break; case 'put': curl_setopt($curlHandle, CURLOPT_CUSTOMREQUEST, 'PUT'); $this->attachRequestPayload($curlHandle, $arguments); break; } $body = json_decode(curl_exec($curlHandle), true); $headers = curl_getinfo($curlHandle); $error = curl_error($curlHandle); return new Response($headers, $body, $error); } protected function attachRequestPayload(&$curlHandle, array $data) { $encoded = json_encode($data); $this->lastRequest['body'] = $encoded; curl_setopt($curlHandle, CURLOPT_POSTFIELDS, $encoded); } /** * @param string $fullUrl * @param array $headers * * @return resource */ protected function getCurlHandle(string $fullUrl, array $headers = []) { $curlHandle = curl_init(); curl_setopt($curlHandle, CURLOPT_URL, $fullUrl); curl_setopt($curlHandle, CURLOPT_HTTPHEADER, array_merge([ 'Accept: application/json', 'Content-Type: application/json', ], $headers)); curl_setopt($curlHandle, CURLOPT_USERAGENT, 'Laravel/Flare API 1.0'); curl_setopt($curlHandle, CURLOPT_RETURNTRANSFER, true); curl_setopt($curlHandle, CURLOPT_TIMEOUT, $this->timeout); curl_setopt($curlHandle, CURLOPT_SSL_VERIFYPEER, true); curl_setopt($curlHandle, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_0); curl_setopt($curlHandle, CURLOPT_ENCODING, ''); curl_setopt($curlHandle, CURLINFO_HEADER_OUT, true); curl_setopt($curlHandle, CURLOPT_FOLLOWLOCATION, true); curl_setopt($curlHandle, CURLOPT_MAXREDIRS, 1); return $curlHandle; } } flare-client-php/src/Truncation/TrimStringsStrategy.php 0000644 00000002240 15002154456 0017334 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; class TrimStringsStrategy extends AbstractTruncationStrategy { /** * @return array<int, int> */ public static function thresholds(): array { return [1024, 512, 256]; } /** * @param array<int|string, mixed> $payload * * @return array<int|string, mixed> */ public function execute(array $payload): array { foreach (static::thresholds() as $threshold) { if (! $this->reportTrimmer->needsToBeTrimmed($payload)) { break; } $payload = $this->trimPayloadString($payload, $threshold); } return $payload; } /** * @param array<int|string, mixed> $payload * @param int $threshold * * @return array<int|string, mixed> */ protected function trimPayloadString(array $payload, int $threshold): array { array_walk_recursive($payload, function (&$value) use ($threshold) { if (is_string($value) && strlen($value) > $threshold) { $value = substr($value, 0, $threshold); } }); return $payload; } } flare-client-php/src/Truncation/AbstractTruncationStrategy.php 0000644 00000000443 15002154456 0020664 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; abstract class AbstractTruncationStrategy implements TruncationStrategy { protected ReportTrimmer $reportTrimmer; public function __construct(ReportTrimmer $reportTrimmer) { $this->reportTrimmer = $reportTrimmer; } } flare-client-php/src/Truncation/TrimContextItemsStrategy.php 0000644 00000002662 15002154456 0020341 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; class TrimContextItemsStrategy extends AbstractTruncationStrategy { /** * @return array<int, int> */ public static function thresholds(): array { return [100, 50, 25, 10]; } /** * @param array<int|string, mixed> $payload * * @return array<int|string, mixed> */ public function execute(array $payload): array { foreach (static::thresholds() as $threshold) { if (! $this->reportTrimmer->needsToBeTrimmed($payload)) { break; } $payload['context'] = $this->iterateContextItems($payload['context'], $threshold); } return $payload; } /** * @param array<int|string, mixed> $contextItems * @param int $threshold * * @return array<int|string, mixed> */ protected function iterateContextItems(array $contextItems, int $threshold): array { array_walk($contextItems, [$this, 'trimContextItems'], $threshold); return $contextItems; } protected function trimContextItems(mixed &$value, mixed $key, int $threshold): mixed { if (is_array($value)) { if (count($value) > $threshold) { $value = array_slice($value, $threshold * -1, $threshold); } array_walk($value, [$this, 'trimContextItems'], $threshold); } return $value; } } flare-client-php/src/Truncation/ReportTrimmer.php 0000644 00000002405 15002154456 0016142 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; class ReportTrimmer { protected static int $maxPayloadSize = 524288; /** @var array<int, class-string<\Spatie\FlareClient\Truncation\TruncationStrategy>> */ protected array $strategies = [ TrimStringsStrategy::class, TrimStackFrameArgumentsStrategy::class, TrimContextItemsStrategy::class, ]; /** * @param array<int|string, mixed> $payload * * @return array<int|string, mixed> */ public function trim(array $payload): array { foreach ($this->strategies as $strategy) { if (! $this->needsToBeTrimmed($payload)) { break; } $payload = (new $strategy($this))->execute($payload); } return $payload; } /** * @param array<int|string, mixed> $payload * * @return bool */ public function needsToBeTrimmed(array $payload): bool { return strlen((string)json_encode($payload)) > self::getMaxPayloadSize(); } public static function getMaxPayloadSize(): int { return self::$maxPayloadSize; } public static function setMaxPayloadSize(int $maxPayloadSize): void { self::$maxPayloadSize = $maxPayloadSize; } } flare-client-php/src/Truncation/TruncationStrategy.php 0000644 00000000365 15002154456 0017203 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; interface TruncationStrategy { /** * @param array<int|string, mixed> $payload * * @return array<int|string, mixed> */ public function execute(array $payload): array; } flare-client-php/src/Truncation/TrimStackFrameArgumentsStrategy.php 0000644 00000000531 15002154456 0021612 0 ustar 00 <?php namespace Spatie\FlareClient\Truncation; class TrimStackFrameArgumentsStrategy implements TruncationStrategy { public function execute(array $payload): array { for ($i = 0; $i < count($payload['stacktrace']); $i++) { $payload['stacktrace'][$i]['arguments'] = null; } return $payload; } } flare-client-php/LICENSE.md 0000644 00000002102 15002154456 0011277 0 ustar 00 The MIT License (MIT) Copyright (c) Facade <info@facade.company> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. laravel-activitylog/.php_cs.dist 0000644 00000001426 15002154456 0012752 0 ustar 00 <?php $finder = \PhpCsFixer\Finder::create() ->notPath('bootstrap/*') ->notPath('storage/*') ->notPath('resources/view/mail/*') ->in([ __DIR__.'/src', __DIR__.'/tests', ]) ->name('*.php') ->notName('*.blade.php') ->ignoreDotFiles(true) ->ignoreVCS(true); return \PhpCsFixer\Config::create() ->setRules(array_merge(require '.php_cs.laravel', [ '@PSR2' => true, '@PSR12' => true, 'no_unused_imports' => true, 'phpdoc_to_comment' => false, 'phpdoc_order' => true, 'phpdoc_separation' => true, 'simplified_null_return' => false, ])) ->setLineEnding("\n") ->setIndent(str_repeat(' ', 4)) ->setUsingCache(false) ->setRiskyAllowed(true) ->setFinder($finder); laravel-activitylog/composer.json 0000644 00000003711 15002154456 0013254 0 ustar 00 { "name": "spatie/laravel-activitylog", "description": "A very simple activity logger to monitor the users of your website or application", "license": "MIT", "keywords": [ "spatie", "log", "user", "activity", "laravel" ], "authors": [ { "name": "Freek Van der Herten", "email": "freek@spatie.be", "homepage": "https://spatie.be", "role": "Developer" }, { "name": "Sebastian De Deyne", "email": "sebastian@spatie.be", "homepage": "https://spatie.be", "role": "Developer" }, { "name": "Tom Witkowski", "email": "dev.gummibeer@gmail.com", "homepage": "https://gummibeer.de", "role": "Developer" } ], "homepage": "https://github.com/spatie/activitylog", "require": { "php": "^8.0", "illuminate/config": "^8.0 || ^9.0 || ^10.0", "illuminate/database": "^8.69 || ^9.27 || ^10.0", "illuminate/support": "^8.0 || ^9.0 || ^10.0", "spatie/laravel-package-tools": "^1.6.3" }, "require-dev": { "ext-json": "*", "orchestra/testbench": "^6.23 || ^7.0 || ^8.0", "pestphp/pest": "^1.20" }, "minimum-stability": "dev", "prefer-stable": true, "autoload": { "psr-4": { "Spatie\\Activitylog\\": "src" }, "files": [ "src/helpers.php" ] }, "autoload-dev": { "psr-4": { "Spatie\\Activitylog\\Test\\": "tests" } }, "config": { "allow-plugins": { "pestphp/pest-plugin": true }, "sort-packages": true }, "extra": { "laravel": { "providers": [ "Spatie\\Activitylog\\ActivitylogServiceProvider" ] } }, "scripts": { "test": "vendor/bin/pest" } } laravel-activitylog/UPGRADING.md 0000644 00000004444 15002154456 0012400 0 ustar 00 ## From v3 to v4 ``` bash composer require spatie/laravel-activitylog "^4.0.0" ``` ### Publish migrations & migrate new tables ``` bash php artisan vendor:publish --provider="Spatie\Activitylog\ActivitylogServiceProvider" --tag="activitylog-migrations" php artisan migrate ``` ### Model event Logging - All models now need to define a `getActivitylogOptions()` method to configure and return the models options as a `LogOptions` instance. - To control what attributes are logged, instead of defining a `$logAttributes` property this is defined in the `getActivitylogOptions()` method using the `logOnly()` method of `LogOptions`. - The `getDescriptionForEvent()` method is no longer used to customize the description. Instead, use the `setDescriptionForEvent()` method for `LogOptions` class. - When customizing the log's name instead of defining a `$logName` property, call the `useLogName()` method when configuring the `LogOptions`. - Instead of the `$ignoreChangedAttributes` property the ` dontLogIfAttributesChangedOnly()` method should be used. - If you only need to log the dirty attributes use `logOnlyDirty()` since the `$logOnlyDirty` property is no longer used. - For instances where you do not want to store empty log events use `dontSubmitEmptyLogs()` instead of setting `$submitEmptyLogs` to `false`. - When you use a `*` (wildcard) and want to ignore specific elements use the `dontLogIfAttributesChangedOnly()` method instead of the `$logAttributesToIgnore` property. ## From v2 to v3 - if you are using a custom `Activity` model, you should let it implement the new `Spatie\Activitylog\Contracts\Activity` interface - the preferred way to get changes on an `Activity` model is through the `changes` property instead of the `changes()` function. Change all usages from `$activity->changes()` to `$activity->changes` - the `activity` relation of the `CausesActivity` trait has been renamed to `actions`. Rename all uses from `$user->activity` to `$user->actions` - the `activity` relation of the `LogsActivity` trait has been renamed to `activities`. Rename all uses from `$yourModel->activity` to `$yourModel->activities`. - the deprecated `loggedActivity` relation has been removed. Use `activities` instead. - the `HasActivity` trait has been removed. Use both `CausesActivity` and `LogsActivity` traits instead. laravel-activitylog/art/logomark.svg 0000644 00000001644 15002154456 0013657 0 ustar 00 <svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 100 100" width="100" height="100"> <linearGradient id="a" x1="21.25" x2="121.25" y1="-21.25" y2="78.75" gradientUnits="userSpaceOnUse"> <stop offset="0" stop-color="#A3C8D4"/> <stop offset=".95" stop-color="#197593"/> </linearGradient> <path fill="url(#a)" d="M100 100h-15v-85h-85v-15h100z"/> <linearGradient id="b" x1="14" x2="72" y1="50" y2="50" gradientUnits="userSpaceOnUse"> <stop offset="0" stop-color="#104C60"/> <stop offset="1" stop-color="#0B3542"/> </linearGradient> <path fill="url(#b)" d="M14 28h58v44h-44v-15h29v-14h-43z"/> <linearGradient id="c" x1="-10.75" x2="68.25" y1="38.75" y2="117.75" gradientUnits="userSpaceOnUse"> <stop offset="0" stop-color="#0F4658"/> <stop offset="1" stop-color="#277D99"/> </linearGradient> <path fill="url(#c)" d="M0 100v-72h15v57h71v15z"/> </svg> laravel-activitylog/art/logomark@3x.png 0000644 00000011503 15002154456 0014212 0 ustar 00 �PNG IHDR , , y}�u tEXtSoftware Adobe ImageReadyq�e<